instruction
stringclasses 2
values | input
stringlengths 0
1k
| output
stringclasses 2
values | metadata
dict |
---|---|---|---|
Analyze this document content | These files were obtained from the creators of the AssistantBench benchmark and modified slightly. You can find the latest version at [https://huggingface.co/spaces/AssistantBench/leaderboard/tree/main/evaluation](https://huggingface.co/spaces/AssistantBench/leaderboard/tree/main/evaluation)
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/AssistantBench/Scripts/evaluate_utils/readme.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | from typing import List, Set, Tuple, Union, Callable
import numpy as np
from scipy.optimize import linear_sum_assignment
def _align_bags(
predicted: List[Set[str]],
gold: List[Set[str]],
method: Callable[[object, object], float],
) -> List[float]:
"""
Takes gold and predicted answer sets and first finds the optimal 1-1 alignment
between them and gets maximum metric values over all the answers.
"""
scores = np.zeros([len(gold), len(predicted)])
for gold_index, gold_item in enumerate(gold):
for pred_index, pred_item in enumerate(predicted):
scores[gold_index, pred_index] = method(pred_item, gold_item)
row_ind, col_ind = linear_sum_assignment(-scores)
max_scores = np.zeros([max(len(gold), len(predicted))])
for row, column in zip(row_ind, col_ind):
max_scores[row] = max(max_scores[row], scores[row, column])
return max_scores
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/AssistantBench/Scripts/evaluate_utils/utils.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import logging
import os
import re
import tiktoken
from openai import AzureOpenAI
from typing import List
from autogen_core import AgentId, AgentProxy, TopicId
from autogen_core import SingleThreadedAgentRuntime
from autogen_core.logging import EVENT_LOGGER_NAME
from autogen_core.models import (
ChatCompletionClient,
UserMessage,
LLMMessage,
)
from autogen_core import DefaultSubscription, DefaultTopicId
from autogen_ext.code_executors.local import LocalCommandLineCodeExecutor
from autogen_core.models import AssistantMessage
from autogen_magentic_one.markdown_browser import MarkdownConverter, UnsupportedFormatException
from autogen_magentic_one.agents.coder import Coder, Executor
from autogen_magentic_one.agents.orchestrator import LedgerOrchestrator
from autogen_magentic_one.messages import BroadcastMessage
from autogen_magentic_one.agents.multimodal_web_surfer import MultimodalWebSurfer
from autogen_magentic_one.agents.file_surfer import FileSurfer
from | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/AssistantBench/Templates/MagenticOne/scenario.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | # GAIA Benchmark
This scenario implements the [GAIA](https://arxiv.org/abs/2311.12983) agent benchmark. Before you begin, make sure you have followed instruction in `../README.md` to prepare your environment.
### Setup Environment Variables for AgBench
Navigate to GAIA
```bash
cd benchmarks/GAIA
```
Create a file called ENV.json with the following (required) contents (If you're using MagenticOne)
```json
{
"BING_API_KEY": "REPLACE_WITH_YOUR_BING_API_KEY",
"HOMEPAGE": "https://www.bing.com/",
"WEB_SURFER_DEBUG_DIR": "/autogen/debug",
"CHAT_COMPLETION_KWARGS_JSON": "{\"api_version\": \"2024-02-15-preview\", \"azure_endpoint\": \"YOUR_ENDPOINT/\", \"model_capabilities\": {\"function_calling\": true, \"json_output\": true, \"vision\": true}, \"azure_ad_token_provider\": \"DEFAULT\", \"model\": \"gpt-4o-2024-05-13\"}",
"CHAT_COMPLETION_PROVIDER": "azure"
}
```
You can also use the openai client by replacing the last two entries in the ENV file by:
- `CHAT_COMPLET | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/GAIA/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | import os
import sys
import re
from agbench.tabulate_cmd import default_tabulate
import json
import pandas as pd
import sqlite3
import glob
import numpy as np
EXCLUDE_DIR_NAMES = ["__pycache__"]
def normalize_answer(a):
# Lower case
# Trim (left and right)
# standardize comma separated values
# Replace multiple spaces with one space
# Remove trailing punctuation
norm_answer = ", ".join(a.strip().lower().split(","))
norm_answer = re.sub(r"[\.\!\?]+$", "", re.sub(r"\s+", " ", norm_answer))
return norm_answer
def scorer(instance_dir):
# Read the expected answer
expected_answer_file = os.path.join(instance_dir, "expected_answer.txt")
if not os.path.isfile(expected_answer_file):
return None
expected_answer = None
with open(expected_answer_file, "rt") as fh:
expected_answer = fh.read().strip()
# Read the console
console_log_file = os.path.join(instance_dir, "console_log.txt")
if not os.path.isfile(console_ | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/GAIA/Scripts/custom_tabulate.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | #
# Run this file to download the human_eval dataset, and create a corresponding testbed scenario:
# (default: ../scenarios/human_eval_two_agents_gpt4.jsonl and ./scenarios/human_eval_two_agents_gpt35.jsonl)
#
import json
import os
import re
import sys
from huggingface_hub import snapshot_download
SCRIPT_PATH = os.path.realpath(__file__)
SCRIPT_NAME = os.path.basename(SCRIPT_PATH)
SCRIPT_DIR = os.path.dirname(SCRIPT_PATH)
SCENARIO_DIR = os.path.realpath(os.path.join(SCRIPT_DIR, os.path.pardir))
TEMPLATES_DIR = os.path.join(SCENARIO_DIR, "Templates")
TASKS_DIR = os.path.join(SCENARIO_DIR, "Tasks")
DOWNLOADS_DIR = os.path.join(SCENARIO_DIR, "Downloads")
REPO_DIR = os.path.join(DOWNLOADS_DIR, "GAIA")
def download_gaia():
"""Download the GAIA benchmark from Hugging Face."""
if not os.path.isdir(DOWNLOADS_DIR):
os.mkdir(DOWNLOADS_DIR)
"""Download the GAIA dataset from Hugging Face Hub"""
snapshot_download(
repo_id="gaia-benchmark/GAIA",
repo_t | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/GAIA/Scripts/init_tasks.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import logging
import os
import re
import tiktoken
from openai import AzureOpenAI
from typing import List
from autogen_core import AgentId, AgentProxy, TopicId
from autogen_core import SingleThreadedAgentRuntime
from autogen_core import EVENT_LOGGER_NAME
from autogen_core.models import (
ChatCompletionClient,
ModelCapabilities,
UserMessage,
LLMMessage,
)
from autogen_core import DefaultSubscription, DefaultTopicId
from autogen_ext.code_executors.local import LocalCommandLineCodeExecutor
from autogen_core.models import AssistantMessage
from autogen_magentic_one.markdown_browser import MarkdownConverter, UnsupportedFormatException
from autogen_magentic_one.agents.coder import Coder, Executor
from autogen_magentic_one.agents.orchestrator import LedgerOrchestrator
from autogen_magentic_one.messages import BroadcastMessage
from autogen_magentic_one.agents.multimodal_web_surfer import MultimodalWebSurfer
from autogen_magentic_one.agents.file_surfer import F | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/GAIA/Templates/MagenticOne/scenario.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | # HumanEval Benchmark
This scenario implements a modified version of the [HumanEval](https://arxiv.org/abs/2107.03374) benchmark.
Compared to the original benchmark, there are **two key differences** here:
- A chat model rather than a completion model is used.
- The agents get pass/fail feedback about their implementations, and can keep trying until they succeed or run out of tokens or turns.
## Running the tasks
Navigate to HumanEval
```bash
cd benchmarks/HumanEval
```
Create a file called ENV.json with the following (required) contents (If you're using MagenticOne)
```json
{
"CHAT_COMPLETION_KWARGS_JSON": "{\"api_version\": \"2024-02-15-preview\", \"azure_endpoint\": \"YOUR_ENDPOINT/\", \"model_capabilities\": {\"function_calling\": true, \"json_output\": true, \"vision\": true}, \"azure_ad_token_provider\": \"DEFAULT\", \"model\": \"gpt-4o-2024-05-13\"}",
"CHAT_COMPLETION_PROVIDER": "azure"
}
```
You can also use the openai client by replacing the last two entries i | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/HumanEval/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | import os
import sys
from agbench.tabulate_cmd import default_tabulate
def main(args):
default_tabulate(args)
if __name__ == "__main__" and __package__ is None:
main(sys.argv)
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/HumanEval/Scripts/custom_tabulate.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | #
# Run this file to download the human_eval dataset, and create a corresponding testbed scenario:
# (default: ../scenarios/human_eval_two_agents_gpt4.jsonl and ./scenarios/human_eval_two_agents_gpt35.jsonl)
#
import base64
import gzip
import io
import json
import os
import re
import requests
URL = "https://github.com/openai/human-eval/raw/master/data/HumanEval.jsonl.gz"
SCRIPT_PATH = os.path.realpath(__file__)
SCRIPT_NAME = os.path.basename(SCRIPT_PATH)
SCRIPT_DIR = os.path.dirname(SCRIPT_PATH)
SCENARIO_DIR = os.path.realpath(os.path.join(SCRIPT_DIR, os.path.pardir))
TEMPLATES_DIR = os.path.join(SCENARIO_DIR, "Templates")
TASKS_DIR = os.path.join(SCENARIO_DIR, "Tasks")
# A selected subset of HumanEval problems to work with during development
# Deprecated 2/5/2024 -- Use subsample instead
REDUCED_SET = [
"HumanEval/2",
"HumanEval/26",
"HumanEval/32",
"HumanEval/33",
"HumanEval/36",
"HumanEval/38",
"HumanEval/41",
"HumanEval/50",
"HumanEval/56" | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/HumanEval/Scripts/init_tasks.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import logging
from autogen_core import AgentId, AgentProxy, TopicId
from autogen_core import SingleThreadedAgentRuntime
from autogen_core import EVENT_LOGGER_NAME
from autogen_core import DefaultSubscription, DefaultTopicId
from autogen_ext.code_executors.local import LocalCommandLineCodeExecutor
from autogen_core.models import (
UserMessage,
)
from autogen_magentic_one.agents.coder import Coder, Executor
from autogen_magentic_one.agents.orchestrator import RoundRobinOrchestrator
from autogen_magentic_one.messages import BroadcastMessage, OrchestrationEvent
from autogen_magentic_one.utils import create_completion_client_from_env
async def main() -> None:
# Create the runtime.
runtime = SingleThreadedAgentRuntime()
# Create the AzureOpenAI client
client = create_completion_client_from_env()
# Register agents.
await runtime.register(
"Coder",
lambda: Coder(model_client=client),
subscriptions=lambda: [DefaultSubscri | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/HumanEval/Templates/MagenticOne/scenario.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | # Disable ruff linter for template files
# ruff: noqa: F821 E722
import sys
__TEST__
def run_tests(candidate):
try:
check(candidate)
# We can search for this string in the output
print("ALL TESTS PASSED !#!#")
except AssertionError:
sys.exit("SOME TESTS FAILED - TRY AGAIN !#!#")
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/HumanEval/Templates/MagenticOne/unit_tests.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | # WebArena Benchmark
This scenario implements the [WebArena](https://github.com/web-arena-x/webarena/tree/main) benchmark. The evaluation code has been modified from WebArena in [evaluation_harness](Templates/Common/evaluation_harness) we retain the License from WebArena and include it here [LICENSE](Templates/Common/evaluation_harness/LICENSE).
## References
Zhou, Shuyan, Frank F. Xu, Hao Zhu, Xuhui Zhou, Robert Lo, Abishek Sridhar, Xianyi Cheng et al. "Webarena: A realistic web environment for building autonomous agents." arXiv preprint arXiv:2307.13854 (2023). | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | import os
import sys
import re
from agbench.tabulate_cmd import default_tabulate
def scorer(instance_dir):
# Read the console
console_log_file = os.path.join(instance_dir, "console_log.txt")
if not os.path.isfile(console_log_file):
return None
console_log = ""
with open(console_log_file, "rt") as fh:
console_log = fh.read()
final_score = None
m = re.search(r"FINAL SCORE:(.*?)\n", console_log, re.DOTALL)
if m:
final_score = m.group(1).strip()
# Missing the final answer line
if final_score is None:
return None
else:
return float(final_score) > 0
def main(args):
default_tabulate(args, scorer=scorer)
if __name__ == "__main__" and __package__ is None:
main(sys.argv)
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Scripts/custom_tabulate.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | #
# Run this file to download the human_eval dataset, and create a corresponding testbed scenario:
# (default: ../scenarios/human_eval_two_agents_gpt4.jsonl and ./scenarios/human_eval_two_agents_gpt35.jsonl)
#
import requests
import tarfile
import hashlib
import io
import json
import os
import re
import sys
URL = "https://raw.githubusercontent.com/web-arena-x/webarena/main/config_files/test.raw.json"
SCRIPT_PATH = os.path.realpath(__file__)
SCRIPT_NAME = os.path.basename(SCRIPT_PATH)
SCRIPT_DIR = os.path.dirname(SCRIPT_PATH)
SCENARIO_DIR = os.path.realpath(os.path.join(SCRIPT_DIR, os.path.pardir))
TEMPLATES_DIR = os.path.join(SCENARIO_DIR, "Templates")
TASKS_DIR = os.path.join(SCENARIO_DIR, "Tasks")
DOWNLOADS_DIR = os.path.join(SCENARIO_DIR, "Downloads")
def download():
"""Download the WebArena dataset (if not already downloaded).
Return a JSON list of problem instances."""
if not os.path.isdir(DOWNLOADS_DIR):
os.mkdir(DOWNLOADS_DIR)
json_file = os.path. | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Scripts/init_tasks.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from .evaluators import *
from .helper_functions import (
shopping_get_latest_order_url,
shopping_get_sku_latest_review_author,
shopping_get_sku_latest_review_rating,
)
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/Common/evaluation_harness/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | # websites domain
import os
REDDIT = os.environ.get("REDDIT", "")
SHOPPING = os.environ.get("SHOPPING", "")
SHOPPING_ADMIN = os.environ.get("SHOPPING_ADMIN", "")
GITLAB = os.environ.get("GITLAB", "")
WIKIPEDIA = os.environ.get("WIKIPEDIA", "")
MAP = os.environ.get("MAP", "")
HOMEPAGE = os.environ.get("HOMEPAGE", "")
REDDIT_USERNAME = os.environ.get("REDDIT_USERNAME", "")
REDDIT_PASSWORD = os.environ.get("REDDIT_PASSWORD", "")
GITLAB_USERNAME = os.environ.get("GITLAB_USERNAME", "")
GITLAB_PASSWORD = os.environ.get("GITLAB_PASSWORD", "")
SHOPPING_USERNAME = os.environ.get("SHOPPING_USERNAME", "")
SHOPPING_PASSWORD = os.environ.get("SHOPPING_PASSWORD", "")
SHOPPING_ADMIN_USERNAME = os.environ.get("SHOPPING_ADMIN_USERNAME", "")
SHOPPING_ADMIN_PASSWORD = os.environ.get("SHOPPING_ADMIN_PASSWORD", "")
assert REDDIT and SHOPPING and SHOPPING_ADMIN and GITLAB and WIKIPEDIA and MAP and HOMEPAGE, (
"Please setup the URLs to each site. Current: \n"
+ f"Reddit: {REDDIT}\n"
+ f"Sho | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/Common/evaluation_harness/env_config.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """From WebArena. base class for evaluation"""
# answer string match
import collections
import html
import importlib
import json
import time
import urllib
import inspect
from pathlib import Path
from typing import Any, Tuple, Union, TypedDict, Dict
from beartype import beartype
from nltk.tokenize import word_tokenize # type: ignore
from playwright.async_api import CDPSession, Page
import numpy as np
import numpy.typing as npt
from .helper_functions import (
PseudoPage,
gitlab_get_project_memeber_role,
llm_fuzzy_match,
llm_ua_match,
reddit_get_post_url,
shopping_get_latest_order_url,
shopping_get_sku_latest_review_author,
shopping_get_sku_latest_review_rating,
)
# Subset used for evaluation (added by: adamfo)
#####################################################################
class Action(TypedDict):
answer: str
Observation = str | npt.NDArray[np.uint8]
class StateInfo(TypedDict):
observation: dict[str, Observation]
info: Dict[str | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/Common/evaluation_harness/evaluators.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """From WebArena with minor modifications. Implements helper functions to assist evaluation cases where other evaluators are not suitable."""
import json
from typing import Any
from urllib.parse import urlparse
import requests
from playwright.async_api import Page
from .env_config import (
ACCOUNTS,
GITLAB,
MAP,
REDDIT,
SHOPPING,
SHOPPING_ADMIN,
WIKIPEDIA,
)
from .openai_utils import (
generate_from_openai_chat_completion,
)
import autogen
def shopping_get_auth_token() -> str:
response = requests.post(
url=f"{SHOPPING}/rest/default/V1/integration/admin/token",
headers={"content-type": "application/json"},
data=json.dumps(
{
"username": ACCOUNTS["shopping_site_admin"]["username"],
"password": ACCOUNTS["shopping_site_admin"]["password"],
}
),
)
token: str = response.json()
return token
def shopping_get_latest_order_url() -> str:
"""Get the l | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/Common/evaluation_harness/helper_functions.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """Tools to generate from OpenAI prompts.
Adopted from https://github.com/zeno-ml/zeno-build/"""
import asyncio
import logging
import os
import random
import time
from typing import Any
import aiolimiter
import openai
from openai import AsyncOpenAI, OpenAI
client = None
aclient = None
if "OPENAI_API_KEY" not in os.environ and "OAI_CONFIG_LIST" not in os.environ:
raise ValueError("Neither OPENAI_API_KEY nor OAI_CONFIG_LIST is defined in the environment.")
if "OPENAI_API_KEY" in os.environ:
client = OpenAI(api_key=os.environ["OPENAI_API_KEY"])
aclient = AsyncOpenAI(api_key=os.environ["OPENAI_API_KEY"])
from tqdm.asyncio import tqdm_asyncio
def retry_with_exponential_backoff( # type: ignore
func,
initial_delay: float = 1,
exponential_base: float = 2,
jitter: bool = True,
max_retries: int = 3,
errors: tuple[Any] = (
openai.RateLimitError,
openai.BadRequestError,
openai.InternalServerError,
),
):
"""Retry a function | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/Common/evaluation_harness/openai_utils.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import logging
import json
import os
import re
import nltk
from typing import Any, Dict, List, Tuple, Union
from autogen_core import AgentId, AgentProxy, TopicId
from autogen_core import SingleThreadedAgentRuntime
from autogen_core import EVENT_LOGGER_NAME
from autogen_core import DefaultSubscription, DefaultTopicId
from autogen_ext.code_executors.local import LocalCommandLineCodeExecutor
from autogen_core.models import (
ChatCompletionClient,
UserMessage,
SystemMessage,
LLMMessage,
)
from autogen_magentic_one.markdown_browser import MarkdownConverter, UnsupportedFormatException
from autogen_magentic_one.agents.coder import Coder, Executor
from autogen_magentic_one.agents.orchestrator import RoundRobinOrchestrator, LedgerOrchestrator
from autogen_magentic_one.messages import BroadcastMessage, OrchestrationEvent, RequestReplyMessage, ResetMessage, DeactivateMessage
from autogen_magentic_one.agents.multimodal_web_surfer import MultimodalWebSurfer
from aut | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/benchmarks/WebArena/Templates/MagenticOne/scenario.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from .version import __version__
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from .cli import main
if __name__ == "__main__":
main()
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/__main__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import sys
from typing import Callable, List, Optional, Sequence
from typing_extensions import TypedDict
from .remove_missing_cmd import remove_missing_cli
from .run_cmd import run_cli
from .tabulate_cmd import tabulate_cli
from .version import __version__
class CommandSpec(TypedDict):
command: str
description: str
function: Optional[Callable[[Sequence[str]], None]]
def main(args: Optional[List[str]] = None) -> None:
if args is None:
args = sys.argv[:] # Shallow copy
invocation_cmd = "autogenbench"
version_string = f"AutoGenBench version {__version__}"
commands: List[CommandSpec] = [
{
"command": "run",
"description": "run a given benchmark configuration",
"function": run_cli,
},
{
"command": "tabulate",
"description": "tabulate the results of a previous run",
"function": tabulate_cli,
},
{
"command": "remove_missing",
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/cli.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import importlib.util
import os
import sys
from types import ModuleType
def load_module(module_path: str) -> ModuleType:
module_name = os.path.basename(module_path).replace(".py", "")
spec = importlib.util.spec_from_file_location(module_name, module_path)
if spec is None:
raise ValueError(f"Could not load module from path: {module_path}")
module = importlib.util.module_from_spec(spec)
sys.modules[module_name] = module
assert spec.loader is not None
spec.loader.exec_module(module)
return module
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/load_module.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import argparse
import os
import shutil
import sys
from typing import Sequence
def default_scorer(instance_dir: str) -> bool:
"""
returns True if the instance_dir has the expected ending pattern in the console_log.txt file
"""
console_log = os.path.join(instance_dir, "console_log.txt")
if os.path.isfile(console_log):
with open(console_log, "rt") as fh:
content = fh.read()
# Use a regular expression to match the expected ending pattern
has_final_answer = "FINAL ANSWER:" in content
has_scenario_complete = "SCENARIO.PY COMPLETE !#!#" in content
has_run_complete = "RUN.SH COMPLETE !#!#" in content
# if so, return False
last_10_lines = content.splitlines()[-10:]
last_10_lines_joined = "\n".join(last_10_lines)
has_error_in_last_10_lines = "Error code" in last_10_lines_joined
has_all = has_final_answer and has_scenario_complete and has_run_compl | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/remove_missing_cmd.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import argparse
import errno
import json
import logging
import os
import pathlib
import random
import shutil
import subprocess
import sys
import time
import traceback
from multiprocessing import Pool
from typing import Any, Callable, Dict, List, Mapping, Optional, Sequence, Tuple, Union, cast
import docker
from azure.core.exceptions import ClientAuthenticationError
from azure.identity import DefaultAzureCredential, get_bearer_token_provider
from docker.errors import APIError, DockerException, ImageNotFound
from typing_extensions import TypedDict
from .version import __version__
# Figure out where everything is
SCRIPT_PATH = os.path.realpath(__file__)
SCRIPT_NAME = os.path.basename(SCRIPT_PATH)
SCRIPT_DIR = os.path.dirname(SCRIPT_PATH)
TASK_TIMEOUT = 60 * 120 # 120 minutes
BASE_TEMPLATE_PATH = os.path.join(SCRIPT_DIR, "template")
RESOURCES_PATH = os.path.join(SCRIPT_DIR, "res")
# What platform are we running?
IS_WIN32 = sys.platform == "win32"
# This is the tag given to the imag | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/run_cmd.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import argparse
import os
import sys
from copy import deepcopy
from typing import Any, Callable, List, Optional, Sequence, Tuple
import tabulate as tb
from .load_module import load_module
# Figure out where everything is
SCRIPT_PATH = os.path.realpath(__file__)
SCRIPT_NAME = os.path.basename(SCRIPT_PATH)
SCRIPT_DIR = os.path.dirname(SCRIPT_PATH)
TABULATE_FILE = "custom_tabulate.py"
SUCCESS_STRINGS = [
"ALL TESTS PASSED !#!#",
]
EXCLUDE_DIR_NAMES = ["__pycache__"]
def find_tabulate_module(search_dir: str, stop_dir: Optional[str] = None) -> Optional[str]:
"""Hunt for the tabulate script."""
search_dir = os.path.abspath(search_dir)
if not os.path.isdir(search_dir):
raise ValueError(f"'{search_dir}' is not a directory.")
stop_dir = None if stop_dir is None else os.path.abspath(stop_dir)
while True:
path = os.path.join(search_dir, TABULATE_FILE)
if os.path.isfile(path):
return path
path = os.path.join(search_dir | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/tabulate_cmd.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | __version__ = "0.0.1a1"
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/agbench/src/agbench/version.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | # AutoGen AgentChat
- [Documentation](https://microsoft.github.io/autogen/dev/user-guide/agentchat-user-guide/index.html)
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | """
This module provides the main entry point for the autogen_agentchat package.
It includes logger names for trace and event logs, and retrieves the package version.
"""
import importlib.metadata
TRACE_LOGGER_NAME = "autogen_agentchat"
"""Logger name for trace logs."""
EVENT_LOGGER_NAME = "autogen_agentchat.events"
"""Logger name for event logs."""
__version__ = importlib.metadata.version("autogen_agentchat")
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """
This module defines various message types used for agent-to-agent communication.
Each message type inherits either from the BaseChatMessage class or BaseAgentEvent
class and includes specific fields relevant to the type of message being sent.
"""
from abc import ABC
from typing import List, Literal
from autogen_core import FunctionCall, Image
from autogen_core.models import FunctionExecutionResult, RequestUsage
from pydantic import BaseModel, ConfigDict, Field
from typing_extensions import Annotated, deprecated
class BaseMessage(BaseModel, ABC):
"""Base class for all message types."""
source: str
"""The name of the agent that sent this message."""
models_usage: RequestUsage | None = None
"""The model client usage incurred when producing this message."""
model_config = ConfigDict(arbitrary_types_allowed=True)
class BaseChatMessage(BaseMessage, ABC):
"""Base class for chat messages."""
pass
class BaseAgentEvent(BaseMessage, ABC):
"""Bas | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/messages.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """
This module initializes various pre-defined agents provided by the package.
BaseChatAgent is the base class for all agents in AgentChat.
"""
from ._assistant_agent import AssistantAgent, Handoff # type: ignore
from ._base_chat_agent import BaseChatAgent
from ._code_executor_agent import CodeExecutorAgent
from ._coding_assistant_agent import CodingAssistantAgent
from ._society_of_mind_agent import SocietyOfMindAgent
from ._tool_use_assistant_agent import ToolUseAssistantAgent
from ._user_proxy_agent import UserProxyAgent
__all__ = [
"BaseChatAgent",
"AssistantAgent",
"Handoff",
"CodeExecutorAgent",
"CodingAssistantAgent",
"ToolUseAssistantAgent",
"SocietyOfMindAgent",
"UserProxyAgent",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import json
import logging
import warnings
from typing import (
Any,
AsyncGenerator,
Awaitable,
Callable,
Dict,
List,
Mapping,
Sequence,
)
from autogen_core import CancellationToken, FunctionCall
from autogen_core.model_context import (
ChatCompletionContext,
UnboundedChatCompletionContext,
)
from autogen_core.models import (
AssistantMessage,
ChatCompletionClient,
FunctionExecutionResult,
FunctionExecutionResultMessage,
SystemMessage,
UserMessage,
)
from autogen_core.tools import FunctionTool, Tool
from typing_extensions import deprecated
from .. import EVENT_LOGGER_NAME
from ..base import Handoff as HandoffBase
from ..base import Response
from ..messages import (
AgentEvent,
ChatMessage,
HandoffMessage,
MultiModalMessage,
TextMessage,
ToolCallExecutionEvent,
ToolCallRequestEvent,
ToolCallSummaryMessage,
)
from ..state import AssistantAgentState
from ._base_chat_agent impor | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_assistant_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from abc import ABC, abstractmethod
from typing import Any, AsyncGenerator, List, Mapping, Sequence
from autogen_core import CancellationToken
from ..base import ChatAgent, Response, TaskResult
from ..messages import (
AgentEvent,
BaseChatMessage,
ChatMessage,
TextMessage,
)
from ..state import BaseState
class BaseChatAgent(ChatAgent, ABC):
"""Base class for a chat agent.
This abstract class provides a base implementation for a :class:`ChatAgent`.
To create a new chat agent, subclass this class and implement the
:meth:`on_messages`, :meth:`on_reset`, and :attr:`produced_message_types`.
If streaming is required, also implement the :meth:`on_messages_stream` method.
An agent is considered stateful and maintains its state between calls to
the :meth:`on_messages` or :meth:`on_messages_stream` methods.
The agent should store its state in the
agent instance. The agent should also implement the :meth:`on_reset` method
to reset the | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_base_chat_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import re
from typing import List, Sequence
from autogen_core import CancellationToken
from autogen_core.code_executor import CodeBlock, CodeExecutor
from ..base import Response
from ..messages import ChatMessage, TextMessage
from ._base_chat_agent import BaseChatAgent
def _extract_markdown_code_blocks(markdown_text: str) -> List[CodeBlock]:
pattern = re.compile(r"```(?:\s*([\w\+\-]+))?\n([\s\S]*?)```")
matches = pattern.findall(markdown_text)
code_blocks: List[CodeBlock] = []
for match in matches:
language = match[0].strip() if match[0] else ""
code_content = match[1]
code_blocks.append(CodeBlock(code=code_content, language=language))
return code_blocks
class CodeExecutorAgent(BaseChatAgent):
"""An agent that extracts and executes code snippets found in received messages and returns the output.
It is typically used within a team with another agent that generates code snippets to be executed.
.. note::
It is recom | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_code_executor_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import warnings
from autogen_core.models import (
ChatCompletionClient,
)
from ._assistant_agent import AssistantAgent
class CodingAssistantAgent(AssistantAgent):
"""[DEPRECATED] An agent that provides coding assistance using an LLM model client.
It responds with a StopMessage when 'terminate' is detected in the response.
"""
def __init__(
self,
name: str,
model_client: ChatCompletionClient,
*,
description: str = "A helpful and general-purpose AI assistant that has strong language skills, Python skills, and Linux command line skills.",
system_message: str = """You are a helpful AI assistant.
Solve tasks using your coding and language skills.
In the following cases, suggest python code (in a python coding block) or shell script (in a sh coding block) for the user to execute.
1. When you need to collect info, use the code to output the info you need, for example, browse or search the web, download/read a file, | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_coding_assistant_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Any, AsyncGenerator, List, Mapping, Sequence
from autogen_core import CancellationToken
from autogen_core.models import ChatCompletionClient, LLMMessage, SystemMessage, UserMessage
from autogen_agentchat.base import Response
from autogen_agentchat.state import SocietyOfMindAgentState
from ..base import TaskResult, Team
from ..messages import (
AgentEvent,
BaseChatMessage,
ChatMessage,
TextMessage,
)
from ._base_chat_agent import BaseChatAgent
class SocietyOfMindAgent(BaseChatAgent):
"""An agent that uses an inner team of agents to generate responses.
Each time the agent's :meth:`on_messages` or :meth:`on_messages_stream`
method is called, it runs the inner team of agents and then uses the
model client to generate a response based on the inner team's messages.
Once the response is generated, the agent resets the inner team by
calling :meth:`Team.reset`.
Args:
name (str): The name of the agent.
team (Te | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_society_of_mind_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import logging
import warnings
from typing import Any, Awaitable, Callable, List
from autogen_core.models import (
ChatCompletionClient,
)
from autogen_core.tools import Tool
from .. import EVENT_LOGGER_NAME
from ._assistant_agent import AssistantAgent
event_logger = logging.getLogger(EVENT_LOGGER_NAME)
class ToolUseAssistantAgent(AssistantAgent):
"""[DEPRECATED] An agent that provides assistance with tool use.
It responds with a StopMessage when 'terminate' is detected in the response.
Args:
name (str): The name of the agent.
model_client (ChatCompletionClient): The model client to use for inference.
registered_tools (List[Tool | Callable[..., Any] | Callable[..., Awaitable[Any]]): The tools to register with the agent.
description (str, optional): The description of the agent.
system_message (str, optional): The system message for the model.
"""
def __init__(
self,
name: str,
model_client: | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_tool_use_assistant_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from inspect import iscoroutinefunction
from typing import Awaitable, Callable, Optional, Sequence, Union, cast
from aioconsole import ainput # type: ignore
from autogen_core import CancellationToken
from ..base import Response
from ..messages import ChatMessage, HandoffMessage, TextMessage
from ._base_chat_agent import BaseChatAgent
# Define input function types more precisely
SyncInputFunc = Callable[[str], str]
AsyncInputFunc = Callable[[str, Optional[CancellationToken]], Awaitable[str]]
InputFuncType = Union[SyncInputFunc, AsyncInputFunc]
# TODO: ainput doesn't seem to play nicely with jupyter.
# No input window appears in this case.
async def cancellable_input(prompt: str, cancellation_token: Optional[CancellationToken]) -> str:
task: asyncio.Task[str] = asyncio.create_task(ainput(prompt)) # type: ignore
if cancellation_token is not None:
cancellation_token.link_future(task)
return await task
class UserProxyAgent(BaseChatAgent):
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/agents/_user_proxy_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from ._chat_agent import ChatAgent, Response
from ._handoff import Handoff
from ._task import TaskResult, TaskRunner
from ._team import Team
from ._termination import TerminatedException, TerminationCondition
__all__ = [
"ChatAgent",
"Response",
"Team",
"TerminatedException",
"TerminationCondition",
"TaskResult",
"TaskRunner",
"Handoff",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from dataclasses import dataclass
from typing import Any, AsyncGenerator, Mapping, Protocol, Sequence, runtime_checkable
from autogen_core import CancellationToken
from ..messages import AgentEvent, ChatMessage
from ._task import TaskRunner
@dataclass(kw_only=True)
class Response:
"""A response from calling :meth:`ChatAgent.on_messages`."""
chat_message: ChatMessage
"""A chat message produced by the agent as the response."""
inner_messages: Sequence[AgentEvent | ChatMessage] | None = None
"""Inner messages produced by the agent, they can be :class:`AgentEvent`
or :class:`ChatMessage`."""
@runtime_checkable
class ChatAgent(TaskRunner, Protocol):
"""Protocol for a chat agent."""
@property
def name(self) -> str:
"""The name of the agent. This is used by team to uniquely identify
the agent. It should be unique within the team."""
...
@property
def description(self) -> str:
"""The description of the agent. | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/_chat_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import logging
from typing import Any, Dict
from autogen_core.tools import FunctionTool, Tool
from pydantic import BaseModel, Field, model_validator
from .. import EVENT_LOGGER_NAME
event_logger = logging.getLogger(EVENT_LOGGER_NAME)
class Handoff(BaseModel):
"""Handoff configuration."""
target: str
"""The name of the target agent to handoff to."""
description: str = Field(default="")
"""The description of the handoff such as the condition under which it should happen and the target agent's ability.
If not provided, it is generated from the target agent's name."""
name: str = Field(default="")
"""The name of this handoff configuration. If not provided, it is generated from the target agent's name."""
message: str = Field(default="")
"""The message to the target agent.
If not provided, it is generated from the target agent's name."""
@model_validator(mode="before")
@classmethod
def set_defaults(cls, values: Dict[str, Any] | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/_handoff.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from dataclasses import dataclass
from typing import AsyncGenerator, Protocol, Sequence
from autogen_core import CancellationToken
from ..messages import AgentEvent, ChatMessage
@dataclass
class TaskResult:
"""Result of running a task."""
messages: Sequence[AgentEvent | ChatMessage]
"""Messages produced by the task."""
stop_reason: str | None = None
"""The reason the task stopped."""
class TaskRunner(Protocol):
"""A task runner."""
async def run(
self,
*,
task: str | ChatMessage | Sequence[ChatMessage] | None = None,
cancellation_token: CancellationToken | None = None,
) -> TaskResult:
"""Run the task and return the result.
The task can be a string, a single message, or a sequence of messages.
The runner is stateful and a subsequent call to this method will continue
from where the previous call left off. If the task is not specified,
the runner will continue with the curre | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/_task.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Any, Mapping, Protocol
from ._task import TaskRunner
class Team(TaskRunner, Protocol):
async def reset(self) -> None:
"""Reset the team and all its participants to its initial state."""
...
async def save_state(self) -> Mapping[str, Any]:
"""Save the current state of the team."""
...
async def load_state(self, state: Mapping[str, Any]) -> None:
"""Load the state of the team."""
...
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/_team.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from abc import ABC, abstractmethod
from typing import List, Sequence
from ..messages import AgentEvent, ChatMessage, StopMessage
class TerminatedException(BaseException): ...
class TerminationCondition(ABC):
"""A stateful condition that determines when a conversation should be terminated.
A termination condition is a callable that takes a sequence of ChatMessage objects
since the last time the condition was called, and returns a StopMessage if the
conversation should be terminated, or None otherwise.
Once a termination condition has been reached, it must be reset before it can be used again.
Termination conditions can be combined using the AND and OR operators.
Example:
.. code-block:: python
import asyncio
from autogen_agentchat.conditions import MaxMessageTermination, TextMentionTermination
async def main() -> None:
# Terminate the conversation after 10 turns or if the | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/base/_termination.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """
This module provides various termination conditions for controlling the behavior of
multi-agent teams.
"""
from ._terminations import (
ExternalTermination,
HandoffTermination,
MaxMessageTermination,
SourceMatchTermination,
StopMessageTermination,
TextMentionTermination,
TimeoutTermination,
TokenUsageTermination,
)
__all__ = [
"MaxMessageTermination",
"TextMentionTermination",
"StopMessageTermination",
"TokenUsageTermination",
"HandoffTermination",
"TimeoutTermination",
"ExternalTermination",
"SourceMatchTermination",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/conditions/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import time
from typing import List, Sequence
from ..base import TerminatedException, TerminationCondition
from ..messages import AgentEvent, ChatMessage, HandoffMessage, MultiModalMessage, StopMessage
class StopMessageTermination(TerminationCondition):
"""Terminate the conversation if a StopMessage is received."""
def __init__(self) -> None:
self._terminated = False
@property
def terminated(self) -> bool:
return self._terminated
async def __call__(self, messages: Sequence[AgentEvent | ChatMessage]) -> StopMessage | None:
if self._terminated:
raise TerminatedException("Termination condition has already been reached")
for message in messages:
if isinstance(message, StopMessage):
self._terminated = True
return StopMessage(content="Stop message received", source="StopMessageTermination")
return None
async def reset(self) -> None:
self._terminated = False
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/conditions/_terminations.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """State management for agents, teams and termination conditions."""
from ._states import (
AssistantAgentState,
BaseGroupChatManagerState,
BaseState,
ChatAgentContainerState,
MagenticOneOrchestratorState,
RoundRobinManagerState,
SelectorManagerState,
SocietyOfMindAgentState,
SwarmManagerState,
TeamState,
)
__all__ = [
"BaseState",
"AssistantAgentState",
"BaseGroupChatManagerState",
"ChatAgentContainerState",
"RoundRobinManagerState",
"SelectorManagerState",
"SwarmManagerState",
"MagenticOneOrchestratorState",
"TeamState",
"SocietyOfMindAgentState",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/state/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Annotated, Any, List, Mapping, Optional
from pydantic import BaseModel, Field
from ..messages import (
AgentEvent,
ChatMessage,
)
# Ensures pydantic can distinguish between types of events & messages.
_AgentMessage = Annotated[AgentEvent | ChatMessage, Field(discriminator="type")]
class BaseState(BaseModel):
"""Base class for all saveable state"""
type: str = Field(default="BaseState")
version: str = Field(default="1.0.0")
class AssistantAgentState(BaseState):
"""State for an assistant agent."""
llm_context: Mapping[str, Any] = Field(default_factory=lambda: dict([("messages", [])]))
type: str = Field(default="AssistantAgentState")
class TeamState(BaseState):
"""State for a team of agents."""
agent_states: Mapping[str, Any] = Field(default_factory=dict)
team_id: str = Field(default="")
type: str = Field(default="TeamState")
class BaseGroupChatManagerState(BaseState):
"""Base state for all group chat man | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/state/_states.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import AsyncGenerator, TypeVar
from typing_extensions import deprecated
from ..base import Response, TaskResult
from ..conditions import (
ExternalTermination as ExternalTerminationAlias,
)
from ..conditions import (
HandoffTermination as HandoffTerminationAlias,
)
from ..conditions import (
MaxMessageTermination as MaxMessageTerminationAlias,
)
from ..conditions import (
SourceMatchTermination as SourceMatchTerminationAlias,
)
from ..conditions import (
StopMessageTermination as StopMessageTerminationAlias,
)
from ..conditions import (
TextMentionTermination as TextMentionTerminationAlias,
)
from ..conditions import (
TimeoutTermination as TimeoutTerminationAlias,
)
from ..conditions import (
TokenUsageTermination as TokenUsageTerminationAlias,
)
from ..messages import AgentEvent, ChatMessage
from ..ui import Console as ConsoleAlias
@deprecated("Moved to autogen_agentchat.conditions.ExternalTermination. Will remove this in 0.4.0.", stack | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/task/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """
This module provides implementation of various pre-defined multi-agent teams.
Each team inherits from the BaseGroupChat class.
"""
from ._group_chat._base_group_chat import BaseGroupChat
from ._group_chat._magentic_one import MagenticOneGroupChat
from ._group_chat._round_robin_group_chat import RoundRobinGroupChat
from ._group_chat._selector_group_chat import SelectorGroupChat
from ._group_chat._swarm_group_chat import Swarm
__all__ = [
"BaseGroupChat",
"RoundRobinGroupChat",
"SelectorGroupChat",
"Swarm",
"MagenticOneGroupChat",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
|
Analyze this code content | import asyncio
import logging
import uuid
from abc import ABC, abstractmethod
from typing import Any, AsyncGenerator, Callable, List, Mapping, Sequence
from autogen_core import (
AgentId,
AgentInstantiationContext,
AgentRuntime,
AgentType,
CancellationToken,
ClosureAgent,
MessageContext,
SingleThreadedAgentRuntime,
TypeSubscription,
)
from autogen_core._closure_agent import ClosureContext
from ... import EVENT_LOGGER_NAME
from ...base import ChatAgent, TaskResult, Team, TerminationCondition
from ...messages import AgentEvent, BaseChatMessage, ChatMessage, TextMessage
from ...state import TeamState
from ._chat_agent_container import ChatAgentContainer
from ._events import GroupChatMessage, GroupChatReset, GroupChatStart, GroupChatTermination
from ._sequential_routed_agent import SequentialRoutedAgent
event_logger = logging.getLogger(EVENT_LOGGER_NAME)
class BaseGroupChat(Team, ABC):
"""The base class for group chat teams.
To implement a | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_base_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from abc import ABC, abstractmethod
from typing import Any, List
from autogen_core import DefaultTopicId, MessageContext, event, rpc
from ...base import TerminationCondition
from ...messages import AgentEvent, ChatMessage, StopMessage
from ._events import (
GroupChatAgentResponse,
GroupChatRequestPublish,
GroupChatReset,
GroupChatStart,
GroupChatTermination,
)
from ._sequential_routed_agent import SequentialRoutedAgent
class BaseGroupChatManager(SequentialRoutedAgent, ABC):
"""Base class for a group chat manager that manages a group chat with multiple participants.
It is the responsibility of the caller to ensure:
- All participants must subscribe to the group chat topic and each of their own topics.
- The group chat manager must subscribe to the group chat topic.
- The agent types of the participants must be unique.
- For each participant, the agent type must be the same as the topic type.
Without the above condition | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_base_group_chat_manager.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Any, List, Mapping
from autogen_core import DefaultTopicId, MessageContext, event, rpc
from ...base import ChatAgent, Response
from ...messages import ChatMessage
from ...state import ChatAgentContainerState
from ._events import GroupChatAgentResponse, GroupChatMessage, GroupChatRequestPublish, GroupChatReset, GroupChatStart
from ._sequential_routed_agent import SequentialRoutedAgent
class ChatAgentContainer(SequentialRoutedAgent):
"""A core agent class that delegates message handling to an
:class:`autogen_agentchat.base.ChatAgent` so that it can be used in a
group chat team.
Args:
parent_topic_type (str): The topic type of the parent orchestrator.
output_topic_type (str): The topic type for the output.
agent (ChatAgent): The agent to delegate message handling to.
"""
def __init__(self, parent_topic_type: str, output_topic_type: str, agent: ChatAgent) -> None:
super().__init__(description=agent.description | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_chat_agent_container.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import List
from pydantic import BaseModel
from ...base import Response
from ...messages import AgentEvent, ChatMessage, StopMessage
class GroupChatStart(BaseModel):
"""A request to start a group chat."""
messages: List[ChatMessage] | None = None
"""An optional list of messages to start the group chat."""
class GroupChatAgentResponse(BaseModel):
"""A response published to a group chat."""
agent_response: Response
"""The response from an agent."""
class GroupChatRequestPublish(BaseModel):
"""A request to publish a message to a group chat."""
...
class GroupChatMessage(BaseModel):
"""A message from a group chat."""
message: AgentEvent | ChatMessage
"""The message that was published."""
class GroupChatTermination(BaseModel):
"""A message indicating that a group chat has terminated."""
message: StopMessage
"""The stop message that indicates the reason of termination."""
class GroupChatReset(BaseModel):
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_events.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Any, Callable, List, Mapping
from ...base import ChatAgent, TerminationCondition
from ...messages import AgentEvent, ChatMessage
from ...state import RoundRobinManagerState
from ._base_group_chat import BaseGroupChat
from ._base_group_chat_manager import BaseGroupChatManager
class RoundRobinGroupChatManager(BaseGroupChatManager):
"""A group chat manager that selects the next speaker in a round-robin fashion."""
def __init__(
self,
group_topic_type: str,
output_topic_type: str,
participant_topic_types: List[str],
participant_descriptions: List[str],
termination_condition: TerminationCondition | None,
max_turns: int | None = None,
) -> None:
super().__init__(
group_topic_type,
output_topic_type,
participant_topic_types,
participant_descriptions,
termination_condition,
max_turns,
)
self._next_speaker_inde | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_round_robin_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import logging
import re
from typing import Any, Callable, Dict, List, Mapping, Sequence
from autogen_core.models import ChatCompletionClient, SystemMessage
from ... import TRACE_LOGGER_NAME
from ...base import ChatAgent, TerminationCondition
from ...messages import (
AgentEvent,
BaseAgentEvent,
ChatMessage,
MultiModalMessage,
)
from ...state import SelectorManagerState
from ._base_group_chat import BaseGroupChat
from ._base_group_chat_manager import BaseGroupChatManager
trace_logger = logging.getLogger(TRACE_LOGGER_NAME)
class SelectorGroupChatManager(BaseGroupChatManager):
"""A group chat manager that selects the next speaker using a ChatCompletion
model and a custom selector function."""
def __init__(
self,
group_topic_type: str,
output_topic_type: str,
participant_topic_types: List[str],
participant_descriptions: List[str],
termination_condition: TerminationCondition | None,
max_turns: int | N | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_selector_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from typing import Any
from autogen_core import MessageContext, RoutedAgent
class FIFOLock:
"""A lock that ensures coroutines acquire the lock in the order they request it."""
def __init__(self) -> None:
self._queue = asyncio.Queue[asyncio.Event]()
self._locked = False
async def acquire(self) -> None:
# If the lock is not held by any coroutine, set the lock to be held
# by the current coroutine.
if not self._locked:
self._locked = True
return
# If the lock is held by another coroutine, create an event and put it
# in the queue. Wait for the event to be set.
event = asyncio.Event()
await self._queue.put(event)
await event.wait()
def release(self) -> None:
if not self._queue.empty():
# If there are events in the queue, get the next event and set it.
next_event = self._queue.get_nowait()
next_event.set()
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_sequential_routed_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from typing import Any, Callable, List, Mapping
from ...base import ChatAgent, TerminationCondition
from ...messages import AgentEvent, ChatMessage, HandoffMessage
from ...state import SwarmManagerState
from ._base_group_chat import BaseGroupChat
from ._base_group_chat_manager import BaseGroupChatManager
class SwarmGroupChatManager(BaseGroupChatManager):
"""A group chat manager that selects the next speaker based on handoff message only."""
def __init__(
self,
group_topic_type: str,
output_topic_type: str,
participant_topic_types: List[str],
participant_descriptions: List[str],
termination_condition: TerminationCondition | None,
max_turns: int | None,
) -> None:
super().__init__(
group_topic_type,
output_topic_type,
participant_topic_types,
participant_descriptions,
termination_condition,
max_turns,
)
self._current_speak | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_swarm_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | from ._magentic_one_group_chat import MagenticOneGroupChat
__all__ = [
"MagenticOneGroupChat",
]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_magentic_one/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import logging
from typing import Callable, List
from autogen_core.models import ChatCompletionClient
from .... import EVENT_LOGGER_NAME, TRACE_LOGGER_NAME
from ....base import ChatAgent, TerminationCondition
from .._base_group_chat import BaseGroupChat
from ._magentic_one_orchestrator import MagenticOneOrchestrator
from ._prompts import ORCHESTRATOR_FINAL_ANSWER_PROMPT
trace_logger = logging.getLogger(TRACE_LOGGER_NAME)
event_logger = logging.getLogger(EVENT_LOGGER_NAME)
class MagenticOneGroupChat(BaseGroupChat):
"""A team that runs a group chat with participants managed by the MagenticOneOrchestrator.
The orchestrator handles the conversation flow, ensuring that the task is completed
efficiently by managing the participants' interactions.
The orchestrator is based on the Magentic-One architecture, which is a generalist multi-agent system for solving complex tasks (see references below).
Args:
participants (List[ChatAgent]): The participants in the | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_magentic_one/_magentic_one_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import json
import logging
from typing import Any, Dict, List, Mapping
from autogen_core import AgentId, CancellationToken, DefaultTopicId, Image, MessageContext, event, rpc
from autogen_core.models import (
AssistantMessage,
ChatCompletionClient,
LLMMessage,
UserMessage,
)
from .... import TRACE_LOGGER_NAME
from ....base import Response, TerminationCondition
from ....messages import (
AgentEvent,
ChatMessage,
HandoffMessage,
MultiModalMessage,
StopMessage,
TextMessage,
ToolCallExecutionEvent,
ToolCallRequestEvent,
ToolCallSummaryMessage,
)
from ....state import MagenticOneOrchestratorState
from .._base_group_chat_manager import BaseGroupChatManager
from .._events import (
GroupChatAgentResponse,
GroupChatMessage,
GroupChatRequestPublish,
GroupChatReset,
GroupChatStart,
GroupChatTermination,
)
from ._prompts import (
ORCHESTRATOR_FINAL_ANSWER_PROMPT,
ORCHESTRATOR_PROGRESS_LEDGER_PROMPT,
ORCHESTRAT | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_magentic_one/_magentic_one_orchestrator.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | ORCHESTRATOR_SYSTEM_MESSAGE = ""
ORCHESTRATOR_TASK_LEDGER_FACTS_PROMPT = """Below I will present you a request. Before we begin addressing the request, please answer the following pre-survey to the best of your ability. Keep in mind that you are Ken Jennings-level with trivia, and Mensa-level with puzzles, so there should be a deep well to draw from.
Here is the request:
{task}
Here is the pre-survey:
1. Please list any specific facts or figures that are GIVEN in the request itself. It is possible that there are none.
2. Please list any facts that may need to be looked up, and WHERE SPECIFICALLY they might be found. In some cases, authoritative sources are mentioned in the request itself.
3. Please list any facts that may need to be derived (e.g., via logical deduction, simulation, or computation)
4. Please list any facts that are recalled from memory, hunches, well-reasoned guesses, etc.
When answering this survey, keep in mind that "facts" will typically be spe | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/teams/_group_chat/_magentic_one/_prompts.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """
This module implements utility classes for formatting/printing agent messages.
"""
from ._console import Console
__all__ = ["Console"]
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/ui/__init__.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import os
import sys
import time
from typing import AsyncGenerator, List, Optional, TypeVar, cast
from autogen_core import Image
from autogen_core.models import RequestUsage
from autogen_agentchat.base import Response, TaskResult
from autogen_agentchat.messages import AgentEvent, ChatMessage, MultiModalMessage
def _is_running_in_iterm() -> bool:
return os.getenv("TERM_PROGRAM") == "iTerm.app"
def _is_output_a_tty() -> bool:
return sys.stdout.isatty()
T = TypeVar("T", bound=TaskResult | Response)
async def Console(
stream: AsyncGenerator[AgentEvent | ChatMessage | T, None],
*,
no_inline_images: bool = False,
) -> T:
"""
Consumes the message stream from :meth:`~autogen_agentchat.base.TaskRunner.run_stream`
or :meth:`~autogen_agentchat.base.ChatAgent.on_messages_stream` and renders the messages to the console.
Returns the last processed TaskResult or Response.
Args:
stream (AsyncGenerator[AgentEvent | ChatMessage | TaskResult, Non | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/src/autogen_agentchat/ui/_console.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import json
import logging
from typing import Any, AsyncGenerator, List
import pytest
from autogen_agentchat import EVENT_LOGGER_NAME
from autogen_agentchat.agents import AssistantAgent
from autogen_agentchat.base import Handoff, TaskResult
from autogen_agentchat.messages import (
ChatMessage,
HandoffMessage,
MultiModalMessage,
TextMessage,
ToolCallExecutionEvent,
ToolCallRequestEvent,
ToolCallSummaryMessage,
)
from autogen_core import Image
from autogen_core.model_context import BufferedChatCompletionContext
from autogen_core.models import LLMMessage
from autogen_core.models._model_client import ModelFamily
from autogen_core.tools import FunctionTool
from autogen_ext.models.openai import OpenAIChatCompletionClient
from openai.resources.chat.completions import AsyncCompletions
from openai.types.chat.chat_completion import ChatCompletion, Choice
from openai.types.chat.chat_completion_chunk import ChatCompletionChunk
from openai.types.chat.chat | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_assistant_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import pytest
from autogen_agentchat.agents import CodeExecutorAgent
from autogen_agentchat.base import Response
from autogen_agentchat.messages import TextMessage
from autogen_core import CancellationToken
from autogen_ext.code_executors.local import LocalCommandLineCodeExecutor
@pytest.mark.asyncio
async def test_basic_code_execution() -> None:
"""Test basic code execution"""
agent = CodeExecutorAgent(name="code_executor", code_executor=LocalCommandLineCodeExecutor())
messages = [
TextMessage(
content="""
```python
import math
number = 42
square_root = math.sqrt(number)
print("%0.3f" % (square_root,))
```
""".strip(),
source="assistant",
)
]
response = await agent.on_messages(messages, CancellationToken())
assert isinstance(response, Response)
assert isinstance(response.chat_message, TextMessage)
assert response.chat_message.content.strip() == "6.481"
assert response.chat_message.source == "code_executo | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_code_executor_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import json
import logging
import tempfile
from typing import Any, AsyncGenerator, List, Sequence
import pytest
from autogen_agentchat import EVENT_LOGGER_NAME
from autogen_agentchat.agents import (
AssistantAgent,
BaseChatAgent,
CodeExecutorAgent,
)
from autogen_agentchat.base import Handoff, Response, TaskResult
from autogen_agentchat.conditions import HandoffTermination, MaxMessageTermination, TextMentionTermination
from autogen_agentchat.messages import (
AgentEvent,
ChatMessage,
HandoffMessage,
MultiModalMessage,
StopMessage,
TextMessage,
ToolCallExecutionEvent,
ToolCallRequestEvent,
ToolCallSummaryMessage,
)
from autogen_agentchat.teams import (
RoundRobinGroupChat,
SelectorGroupChat,
Swarm,
)
from autogen_agentchat.teams._group_chat._round_robin_group_chat import RoundRobinGroupChatManager
from autogen_agentchat.teams._group_chat._selector_group_chat import SelectorGroupChatManager
from autogen_agentchat | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import json
import logging
from typing import Sequence
import pytest
from autogen_agentchat import EVENT_LOGGER_NAME
from autogen_agentchat.agents import (
BaseChatAgent,
)
from autogen_agentchat.base import Response
from autogen_agentchat.messages import (
ChatMessage,
TextMessage,
)
from autogen_agentchat.teams import (
MagenticOneGroupChat,
)
from autogen_agentchat.teams._group_chat._magentic_one._magentic_one_orchestrator import MagenticOneOrchestrator
from autogen_core import AgentId, CancellationToken
from autogen_ext.models.replay import ReplayChatCompletionClient
from utils import FileLogHandler
logger = logging.getLogger(EVENT_LOGGER_NAME)
logger.setLevel(logging.DEBUG)
logger.addHandler(FileLogHandler("test_magentic_one_group_chat.log"))
class _EchoAgent(BaseChatAgent):
def __init__(self, name: str, description: str) -> None:
super().__init__(name, description)
self._last_message: str | None = None
self._total_mes | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_magentic_one_group_chat.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import random
from dataclasses import dataclass
from typing import List
import pytest
from autogen_agentchat.teams._group_chat._sequential_routed_agent import SequentialRoutedAgent
from autogen_core import (
AgentId,
DefaultTopicId,
MessageContext,
SingleThreadedAgentRuntime,
default_subscription,
message_handler,
)
@dataclass
class Message:
content: str
@default_subscription
class _TestAgent(SequentialRoutedAgent):
def __init__(self, description: str) -> None:
super().__init__(description=description)
self.messages: List[Message] = []
@message_handler
async def handle_content_publish(self, message: Message, ctx: MessageContext) -> None:
# Sleep a random amount of time to simulate processing time.
await asyncio.sleep(random.random() / 100)
self.messages.append(message)
@pytest.mark.asyncio
async def test_sequential_routed_agent() -> None:
runtime = SingleThreadedAgentRuntime()
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_sequential_routed_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from typing import Any, AsyncGenerator, List
import pytest
from autogen_agentchat.agents import AssistantAgent, SocietyOfMindAgent
from autogen_agentchat.conditions import MaxMessageTermination
from autogen_agentchat.teams import RoundRobinGroupChat
from autogen_ext.models.openai import OpenAIChatCompletionClient
from openai.resources.chat.completions import AsyncCompletions
from openai.types.chat.chat_completion import ChatCompletion, Choice
from openai.types.chat.chat_completion_chunk import ChatCompletionChunk
from openai.types.chat.chat_completion_message import ChatCompletionMessage
from openai.types.completion_usage import CompletionUsage
class _MockChatCompletion:
def __init__(self, chat_completions: List[ChatCompletion]) -> None:
self._saved_chat_completions = chat_completions
self._curr_index = 0
async def mock_create(
self, *args: Any, **kwargs: Any
) -> ChatCompletion | AsyncGenerator[ChatCompletionChunk, None]:
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_society_of_mind_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
import pytest
from autogen_agentchat.base import TerminatedException
from autogen_agentchat.conditions import (
ExternalTermination,
HandoffTermination,
MaxMessageTermination,
SourceMatchTermination,
StopMessageTermination,
TextMentionTermination,
TimeoutTermination,
TokenUsageTermination,
)
from autogen_agentchat.messages import HandoffMessage, StopMessage, TextMessage
from autogen_core.models import RequestUsage
@pytest.mark.asyncio
async def test_handoff_termination() -> None:
termination = HandoffTermination("target")
assert await termination([]) is None
await termination.reset()
assert await termination([TextMessage(content="Hello", source="user")]) is None
await termination.reset()
assert await termination([HandoffMessage(target="target", source="user", content="Hello")]) is not None
assert termination.terminated
await termination.reset()
assert await termination([HandoffMessage(target="another" | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_termination_condition.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import asyncio
from typing import Optional, Sequence
import pytest
from autogen_agentchat.agents import UserProxyAgent
from autogen_agentchat.base import Response
from autogen_agentchat.messages import ChatMessage, HandoffMessage, TextMessage
from autogen_core import CancellationToken
@pytest.mark.asyncio
async def test_basic_input() -> None:
"""Test basic message handling with custom input"""
def custom_input(prompt: str) -> str:
return "The height of the eiffel tower is 324 meters. Aloha!"
agent = UserProxyAgent(name="test_user", input_func=custom_input)
messages = [TextMessage(content="What is the height of the eiffel tower?", source="assistant")]
response = await agent.on_messages(messages, CancellationToken())
assert isinstance(response, Response)
assert isinstance(response.chat_message, TextMessage)
assert response.chat_message.content == "The height of the eiffel tower is 324 meters. Aloha!"
assert response.chat_message.source = | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/test_userproxy_agent.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | import json
import logging
import sys
from datetime import datetime
from pydantic import BaseModel
class FileLogHandler(logging.Handler):
def __init__(self, filename: str) -> None:
super().__init__()
self.filename = filename
self.file_handler = logging.FileHandler(filename)
def emit(self, record: logging.LogRecord) -> None:
ts = datetime.fromtimestamp(record.created).isoformat()
if isinstance(record.msg, BaseModel):
record.msg = json.dumps(
{
"timestamp": ts,
"message": record.msg.model_dump(),
"type": record.msg.__class__.__name__,
},
)
self.file_handler.emit(record)
class ConsoleLogHandler(logging.Handler):
def emit(self, record: logging.LogRecord) -> None:
ts = datetime.fromtimestamp(record.created).isoformat()
if isinstance(record.msg, BaseModel):
record.msg = json.dumps(
| Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-agentchat/tests/utils.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | # AutoGen Core
- [Documentation](https://microsoft.github.io/autogen/dev/user-guide/core-user-guide/index.html)
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ## Building the AutoGen Documentation
AutoGen documentation is based on the sphinx documentation system and uses the myst-parser to render markdown files. It uses the [pydata-sphinx-theme](https://pydata-sphinx-theme.readthedocs.io/en/latest/) to style the documentation.
### Prerequisites
Ensure you have all of the dev dependencies for the `autogen-core` package installed. You can install them by running the following command from the root of the python repository:
```bash
uv sync
source .venv/bin/activate
```
## Building Docs
To build the documentation, run the following command from the root of the python repository:
```bash
poe --directory ./packages/autogen-core/ docs-build
```
To serve the documentation locally, run the following command from the root of the python repository:
```bash
poe --directory ./packages/autogen-core/ docs-serve
```
[!NOTE]
Sphinx will only rebuild files that have changed since the last build. If you want to force a full rebuild, you can delete th | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/README.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | from pathlib import Path
from string import Template
import sys
THIS_FILE_DIR = Path(__file__).parent
# Contains single text template $to_url
HTML_PAGE_TEMPLATE_FILE = THIS_FILE_DIR / "redirect_template.html"
HTML_REDIRECT_TEMPLATE = HTML_PAGE_TEMPLATE_FILE.open("r").read()
REDIRECT_URLS_FILE = THIS_FILE_DIR / "redirect_urls.txt"
def generate_redirect(file_to_write: str, new_url: str, base_dir: Path):
# Create a new redirect page
redirect_page = Template(HTML_REDIRECT_TEMPLATE).substitute(to_url=new_url)
# If the url ends with /, add index.html
if file_to_write.endswith("/"):
file_to_write += "index.html"
else:
file_to_write += "/index.html"
if file_to_write.startswith("/"):
file_to_write = file_to_write[1:]
# Create the path to the redirect page
redirect_page_path = base_dir / file_to_write
# Create the directory if it doesn't exist
redirect_page_path.parent.mkdir(parents=True, exist_ok=True)
# Write the redi | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/redirects/redirects.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | # Configuration file for the Sphinx documentation builder.
#
# For the full list of built-in configuration values, see the documentation:
# https://www.sphinx-doc.org/en/master/usage/configuration.html
from sphinx.application import Sphinx
from typing import Any, Dict
from pathlib import Path
import sys
import os
# -- Project information -----------------------------------------------------
# https://www.sphinx-doc.org/en/master/usage/configuration.html#project-information
import autogen_core
project = "autogen_core"
copyright = "2024, Microsoft"
author = "Microsoft"
version = "0.4"
release = autogen_core.__version__
sys.path.append(str(Path(".").resolve()))
# -- General configuration ---------------------------------------------------
# https://www.sphinx-doc.org/en/master/usage/configuration.html#general-configuration
extensions = [
"sphinx.ext.napoleon",
"sphinx.ext.autodoc",
"sphinx.ext.autosummary",
"sphinx.ext.todo",
"sphinx.ext.viewcode",
"sphinx.e | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/conf.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
Top-level documentation for AutoGen, a framework for developing applications using AI agents
html_theme.sidebar_secondary.remove: false
sd_hide_title: true
---
<style>
.hero-title {
font-size: 60px;
font-weight: bold;
margin: 2rem auto 0;
}
.wip-card {
border: 1px solid var(--pst-color-success);
background-color: var(--pst-color-success-bg);
border-radius: .25rem;
padding: 0.3rem;
display: flex;
justify-content: center;
align-items: center;
margin-bottom: 1rem;
}
</style>
# AutoGen
<div class="container">
<div class="row text-center">
<div class="col-sm-12">
<h1 class="hero-title">
AutoGen
</h1>
<h3>
A framework for building AI agents and multi-agent applications
</h3>
</div>
</div>
</div>
<div style="margin-top: 2rem;">
::::{grid}
:gutter: 2
:::{grid-item-card}
:shadow: none
:margin: 2 0 0 0
:columns: 12 12 12 12
<div class="sd-card-title sd-font-weight-bold docutils">
{fas}`people-group;pst-color | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this code content | # Modified from: https://github.com/kai687/sphinxawesome-codelinter
import tempfile
from typing import AbstractSet, Any, Iterable
from docutils import nodes
from sphinx.application import Sphinx
from sphinx.builders import Builder
from sphinx.util import logging
from sphinx.util.console import darkgreen, darkred, red, teal, faint # type: ignore[attr-defined]
from pygments import highlight # type: ignore
from pygments.lexers import PythonLexer
from pygments.formatters import TerminalFormatter
logger = logging.getLogger(__name__)
__version__ = "0.1.0"
class CodeLinter(Builder):
"""Iterate over all ``literal_block`` nodes.
pipe them into any command line tool that
can read from standard input.
"""
name = "code_lint"
allow_parallel = True
def init(self) -> None:
"""Initialize."""
self._had_errors = False
pass
def get_outdated_docs(self) -> str | Iterable[str]:
"""Check for outdated files.
Return an iterab | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/_extension/code_lint.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this code content | """A directive to generate a gallery of images from structured data.
Generating a gallery of images that are all the same size is a common
pattern in documentation, and this can be cumbersome if the gallery is
generated programmatically. This directive wraps this particular use-case
in a helper-directive to generate it with a single YAML configuration file.
It currently exists for maintainers of the pydata-sphinx-theme,
but might be abstracted into a standalone package if it proves useful.
"""
from pathlib import Path
from typing import Any, ClassVar, Dict, List
from docutils import nodes
from docutils.parsers.rst import directives
from sphinx.application import Sphinx
from sphinx.util import logging
from sphinx.util.docutils import SphinxDirective
from yaml import safe_load
logger = logging.getLogger(__name__)
TEMPLATE_GRID = """
`````{{grid}} {columns}
{options}
{content}
`````
"""
GRID_CARD = """
````{{grid-item-card}} {title}
{options}
{content}
````
"""
class GalleryG | Code analysis and explanation | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/_extension/gallery_directive.py",
"file_type": ".py",
"source_type": "code"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
AutoGen is a community-driven project. Learn how to get involved, contribute, and connect with the community.
---
# API Reference
```{toctree}
:hidden:
:caption: AutoGen AgentChat
python/autogen_agentchat
python/autogen_agentchat.messages
python/autogen_agentchat.agents
python/autogen_agentchat.teams
python/autogen_agentchat.base
python/autogen_agentchat.conditions
python/autogen_agentchat.ui
python/autogen_agentchat.state
```
```{toctree}
:hidden:
:caption: AutoGen Core
python/autogen_core
python/autogen_core.code_executor
python/autogen_core.models
python/autogen_core.model_context
python/autogen_core.tools
python/autogen_core.tool_agent
python/autogen_core.exceptions
python/autogen_core.logging
```
```{toctree}
:hidden:
:caption: AutoGen Extensions
python/autogen_ext.agents.magentic_one
python/autogen_ext.agents.openai
python/autogen_ext.agents.web_surfer
python/autogen_ext.agents.file_surfer
python/autogen_ext.agents. | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/reference/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
User Guide for AgentChat, a high-level API for AutoGen
---
# AgentChat
AgentChat is a high-level API for building multi-agent applications.
It is built on top of the [`autogen-core`](../core-user-guide/index.md) package.
For beginner users, AgentChat is the recommended starting point.
For advanced users, [`autogen-core`](../core-user-guide/index.md)'s event-driven
programming model provides more flexibility and control over the underlying components.
AgentChat provides intuitive defaults, such as **Agents** with preset
behaviors and **Teams** with predefined [multi-agent design patterns](../core-user-guide/design-patterns/intro.md).
::::{grid} 2 2 2 2
:gutter: 3
:::{grid-item-card} {fas}`download;pst-color-primary` Installation
:link: ./installation.html
How to install AgentChat
:::
:::{grid-item-card} {fas}`rocket;pst-color-primary` Quickstart
:link: ./quickstart.html
Build your first agent
:::
:::{grid-item-card} {fas | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/agentchat-user-guide/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
Installing AutoGen AgentChat
---
# Installation
## Create a Virtual Environment (optional)
When installing AgentChat locally, we recommend using a virtual environment for the installation. This will ensure that the dependencies for AgentChat are isolated from the rest of your system.
``````{tab-set}
`````{tab-item} venv
Create and activate:
```bash
python3 -m venv .venv
source .venv/bin/activate
```
To deactivate later, run:
```bash
deactivate
```
`````
`````{tab-item} conda
[Install Conda](https://docs.conda.io/projects/conda/en/stable/user-guide/install/index.html) if you have not already.
Create and activate:
```bash
conda create -n autogen python=3.10
conda activate autogen
```
To deactivate later, run:
```bash
conda deactivate
```
`````
``````
## Install Using pip
Install the `autogen-agentchat` package using pip:
```bash
pip install "autogen-agentchat==0.4.0.dev13"
```
```{note}
Python 3.10 or la | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/agentchat-user-guide/installation.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Migration Guide for v0.2 to v0.4
This is a migration guide for users of the `v0.2.*` versions of `autogen-agentchat`
to the `v0.4` version, which introduces a new set of APIs and features.
The `v0.4` version contains breaking changes. Please read this guide carefully.
We still maintain the `v0.2` version in the `0.2` branch; however,
we highly recommend you upgrade to the `v0.4` version.
> **Note**: We no longer have admin access to the `pyautogen` PyPI package, and
> the releases from that package are no longer from Microsoft since version 0.2.34.
> To continue use the `v0.2` version of AutoGen, install it using `autogen-agentchat~=0.2`.
> Please read our [clarification statement](https://github.com/microsoft/autogen/discussions/4217) regarding forks.
## What is `v0.4`?
Since the release of AutoGen in 2023, we have intensively listened to our community and users from small startups and large enterprises, gathering much feedback. Based on that feedback, we built AutoGen `v0.4`, a | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/agentchat-user-guide/migration-guide.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
Examples built using AgentChat, a high-level api for AutoGen
---
# Examples
A list of examples to help you get started with AgentChat.
:::::{grid} 2 2 2 3
::::{grid-item-card} Travel Planning
:img-top: ../../../images/example-travel.jpeg
:img-alt: travel planning example
:link: ./travel-planning.html
^^^
Generating a travel plan using multiple agents.
::::
::::{grid-item-card} Company Research
:img-top: ../../../images/example-company.jpg
:img-alt: company research example
:link: ./company-research.html
^^^
Generating a company research report using multiple agents with tools.
::::
::::{grid-item-card} Literature Review
:img-top: ../../../images/example-literature.jpg
:img-alt: literature review example
:link: ./literature-review.html
^^^
Generating a literature review using agents with tools.
::::
:::::
```{toctree}
:maxdepth: 1
:hidden:
travel-planning
company-research
literature-review
```
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/agentchat-user-guide/examples/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
FAQ for AutoGen Studio - A low code tool for building and debugging multi-agent systems
---
# FAQ
## Q: How do I specify the directory where files(e.g. database) are stored?
A: You can specify the directory where files are stored by setting the `--appdir` argument when running the application. For example, `autogenstudio ui --appdir /path/to/folder`. This will store the database (default) and other files in the specified directory e.g. `/path/to/folder/database.sqlite`.
## Q: Can I use other models with AutoGen Studio?
Yes. AutoGen standardizes on the openai model api format, and you can use any api server that offers an openai compliant endpoint.
AutoGen Studio is based on declaritive specifications which applies to models as well. Agents can include a model_client field which specifies the model endpoint details including `model`, `api_key`, `base_url`, `model type`.
An example of the openai model client is shown below: | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/autogenstudio-user-guide/faq.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
User Guide for AutoGen Studio - A low code tool for building and debugging multi-agent systems
---
# AutoGen Studio
[](https://badge.fury.io/py/autogenstudio)
[](https://pepy.tech/project/autogenstudio)
AutoGen Studio is a low-code interface built to help you rapidly prototype AI agents, enhance them with tools, compose them into teams and interact with them to accomplish tasks. It is built on [AutoGen AgentChat](https://microsoft.github.io/autogen) - a high-level API for building multi-agent applications.
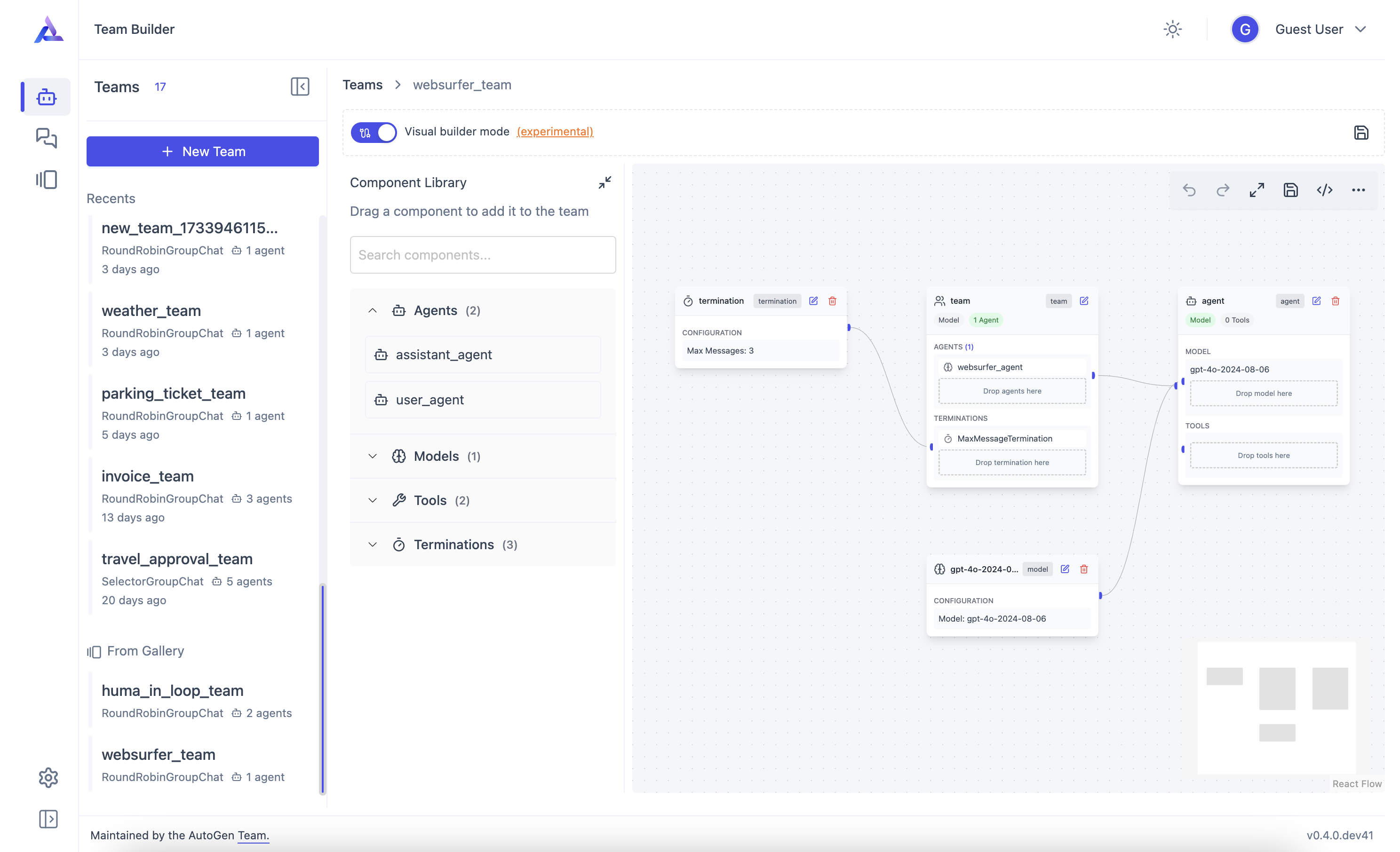
Code for AutoGen Studio is on GitHub at [microsoft/autogen](https://github.com/microsoft/autogen/tree/main/python/packages/autogen-studio)
```{caution}
Auto | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/autogenstudio-user-guide/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
User Guide for AutoGen Studio - A low code tool for building and debugging multi-agent systems
---
# Installation
There are two ways to install AutoGen Studio - from PyPi or from source. We **recommend installing from PyPi** unless you plan to modify the source code.
1. **Install from PyPi**
We recommend using a virtual environment (e.g., conda) to avoid conflicts with existing Python packages. With Python 3.10 or newer active in your virtual environment, use pip to install AutoGen Studio:
```bash
pip install -U autogenstudio
```
2. **Install from Source**
> Note: This approach requires some familiarity with building interfaces in React.
If you prefer to install from source, ensure you have Python 3.10+ and Node.js (version above 14.15.0) installed. Here's how you get started:
- Clone the AutoGen Studio repository and install its Python dependencies:
```bash
pip install -e .
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/autogenstudio-user-guide/installation.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
Usage for AutoGen Studio - A low code tool for building and debugging multi-agent systems
---
# Usage
The expected usage behavior is that developers use the provided Team Builder interface to to define teams - create agents, attach tools and models to agents, and define termination conditions. Once the team is defined, users can run the team in the Playground to interact with the team to accomplish tasks.
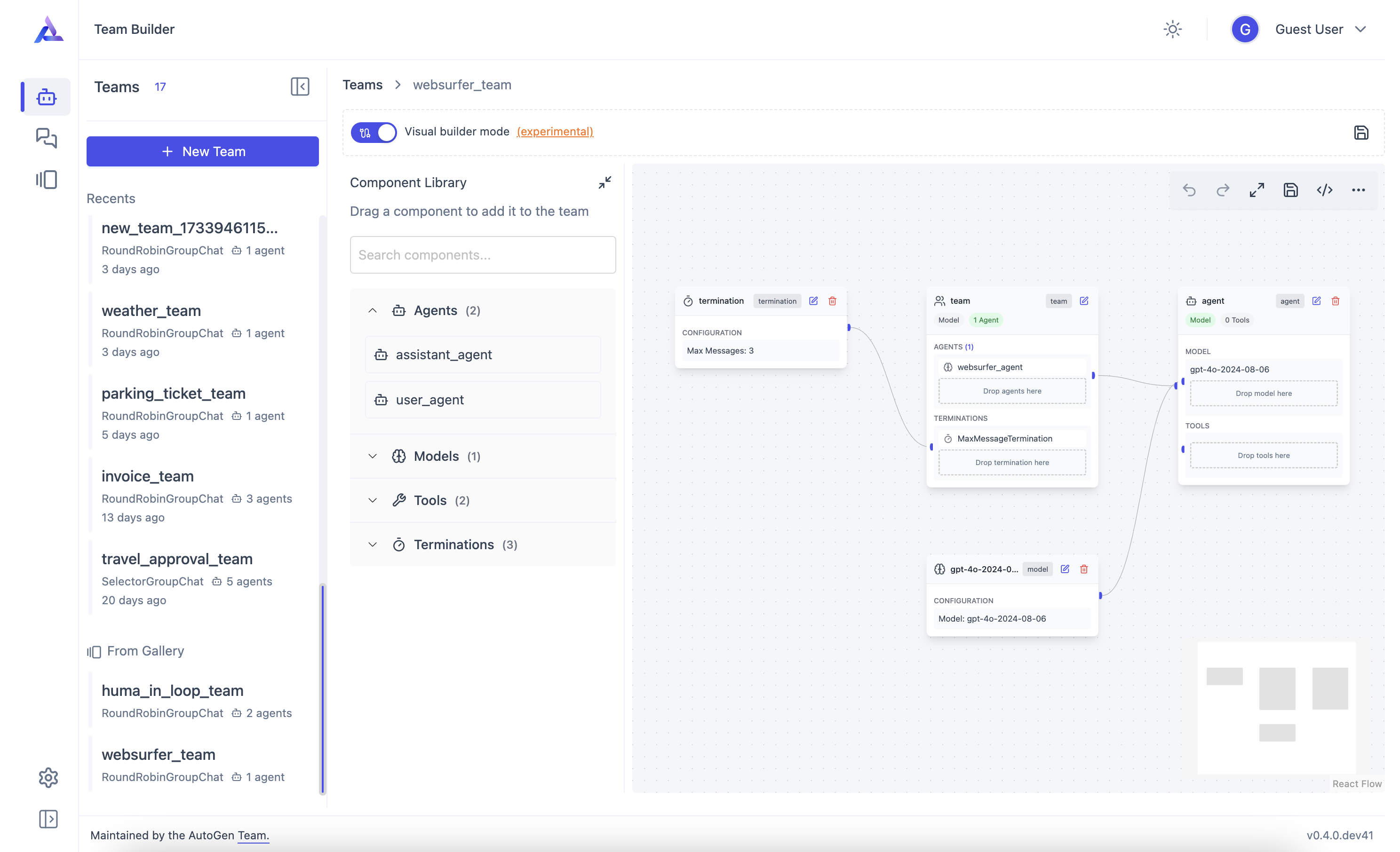
## Building an Agent Team
AutoGen Studio is tied very closely with all of the component abstractions provided by AutoGen AgentChat. This includes - {py:class}`~autogen_agentchat.teams`, {py:class}`~autogen_agentchat.agents`, {py:class}`~autogen_core.models`, {py:class}`~autogen_core.tools`, termination {py:class}`~autogen_agentchat.conditions`.
Users can define these components | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/autogenstudio-user-guide/usage.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # FAQs
## How do I get the underlying agent instance?
Agents might be distributed across multiple machines, so the underlying agent instance is intentionally discouraged from being accessed. If the agent is definitely running on the same machine, you can access the agent instance by calling {py:meth}`autogen_core.AgentRuntime.try_get_underlying_agent_instance` on the `AgentRuntime`. If the agent is not available this will throw an exception.
## How do I call call a function on an agent?
Since the instance itself is not accessible, you can't call a function on an agent directly. Instead, you should create a type to represent the function call and its arguments, and then send that message to the agent. Then in the agent, create a handler for that message type and implement the required logic. This also supports returning a response to the caller.
This allows your agent to work in a distributed environment a well as a local one.
## Why do I need to use a factory to register an agent | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/faqs.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | ---
myst:
html_meta:
"description lang=en": |
User Guide for AutoGen Core, a framework for building multi-agent applications with AI agents.
---
# Core
```{toctree}
:maxdepth: 1
:hidden:
installation
quickstart
```
```{toctree}
:maxdepth: 1
:hidden:
:caption: Core Concepts
core-concepts/agent-and-multi-agent-application
core-concepts/architecture
core-concepts/application-stack
core-concepts/agent-identity-and-lifecycle
core-concepts/topic-and-subscription
```
```{toctree}
:maxdepth: 1
:hidden:
:caption: Framework Guide
framework/agent-and-agent-runtime
framework/message-and-communication
framework/model-clients
framework/tools
framework/logging
framework/telemetry
framework/command-line-code-executors
framework/distributed-agent-runtime
framework/component-config
```
```{toctree}
:maxdepth: 1
:hidden:
:caption: Multi-Agent Design Patterns
design-patterns/intro
design-patterns/concurrent-agents
design-patterns/sequential-workflow
design-patterns/group-chat
design-p | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Installation
## Install using pip
Install the `autogen-core` package using pip:
```bash
pip install "autogen-core==0.4.0.dev13"
```
```{note}
Python 3.10 or later is required.
```
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/installation.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Azure OpenAI with AAD Auth
This guide will show you how to use the Azure OpenAI client with Azure Active Directory (AAD) authentication.
The identity used must be assigned the [**Cognitive Services OpenAI User**](https://learn.microsoft.com/en-us/azure/ai-services/openai/how-to/role-based-access-control#cognitive-services-openai-user) role.
## Install Azure Identity client
The Azure identity client is used to authenticate with Azure Active Directory.
```sh
pip install azure-identity
```
## Using the Model Client
```python
from autogen_ext.models.openai import AzureOpenAIChatCompletionClient
from azure.identity import DefaultAzureCredential, get_bearer_token_provider
# Create the token provider
token_provider = get_bearer_token_provider(
DefaultAzureCredential(), "https://cognitiveservices.azure.com/.default"
)
client = AzureOpenAIChatCompletionClient(
azure_deployment="{your-azure-deployment}",
model="{model-name, such as gpt-4o}",
api_version="2024-02-01",
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/cookbook/azure-openai-with-aad-auth.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Cookbook
This section contains a collection of recipes that demonstrate how to use the Core API features.
## List of recipes
```{toctree}
:maxdepth: 1
azure-openai-with-aad-auth
termination-with-intervention
tool-use-with-intervention
extracting-results-with-an-agent
openai-assistant-agent
langgraph-agent
llamaindex-agent
local-llms-ollama-litellm
instrumenting
topic-subscription-scenarios
structured-output-agent
llm-usage-logger
```
| Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/cookbook/index.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Instrumentating your code locally
AutoGen supports instrumenting your code using [OpenTelemetry](https://opentelemetry.io). This allows you to collect traces and logs from your code and send them to a backend of your choice.
While debugging, you can use a local backend such as [Aspire](https://aspiredashboard.com/) or [Jaeger](https://www.jaegertracing.io/). In this guide we will use Aspire as an example.
## Setting up Aspire
Follow the instructions [here](https://learn.microsoft.com/en-us/dotnet/aspire/fundamentals/dashboard/overview?tabs=bash#standalone-mode) to set up Aspire in standalone mode. This will require Docker to be installed on your machine.
## Instrumenting your code
Once you have a dashboard set up, now it's a matter of sending traces and logs to it. You can follow the steps in the [Telemetry Guide](../framework/telemetry.md) to set up the opentelemetry sdk and exporter.
After instrumenting your code with the Aspire Dashboard running, you should see traces and l | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/cookbook/instrumenting.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | # Agent and Multi-Agent Applications
An **agent** is a software entity that communicates via messages, maintains its own state, and performs actions in response to received messages or changes in its state. These actions may modify the agent’s state and produce external effects, such as updating message logs, sending new messages, executing code, or making API calls.
Many software systems can be modeled as a collection of independent agents that interact with one another. Examples include:
- Sensors on a factory floor
- Distributed services powering web applications
- Business workflows involving multiple stakeholders
- AI agents, such as those powered by language models (e.g., GPT-4), which can write code, interface with external systems, and communicate with other agents.
These systems, composed of multiple interacting agents, are referred to as **multi-agent applications**.
> **Note:**
> AI agents typically use language models as part of their software stack to interpret mess | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/core-concepts/agent-and-multi-agent-application.md",
"file_type": ".md",
"source_type": "document"
} |
Analyze this document content | (agentid_and_lifecycle)=
# Agent Identity and Lifecycle
The agent runtime manages agents' identities
and lifecycles.
Application does not create agents directly, rather,
it registers an agent type with a factory function for
agent instances.
In this section, we explain how agents are identified
and created by the runtime.
## Agent ID
Agent ID uniquely identifies an agent instance within
an agent runtime -- including distributed runtime.
It is the "address" of the agent instance for receiving messages.
It has two components: agent type and agent key.
```{note}
Agent ID = (Agent Type, Agent Key)
```
The agent type is not an agent class.
It associates an agent with a specific
factory function, which produces instances of agents
of the same agent type.
For example, different factory functions can produce the same
agent class but with different constructor parameters.
The agent key is an instance identifier
for the given agent type.
Agent IDs can be converted to and from strings. the f | Document summary and key points | {
"file_path": "multi_repo_data/autogen/python/packages/autogen-core/docs/src/user-guide/core-user-guide/core-concepts/agent-identity-and-lifecycle.md",
"file_type": ".md",
"source_type": "document"
} |