text
stringlengths 2
99.9k
| meta
dict |
---|---|
<ul>
<li>br</li>
<li>em</li>
<li>strong</li>
<li>code</li>
<li>a</li>
<li>img</li>
<li>hr</li>
<li>ul, ol</li>
<li>pre</li>
<li>div</li>
<li>p</li>
<li>blockquote</li>
<li>h1 ~ h6</li>
</ul>
| {
"pile_set_name": "Github"
} |
export class KeyboardControls {
constructor(visualizer, bindings) {
this.visualizer = visualizer;
this.keyState = {};
this.keyBindings = bindings;
this.el = null;
this._onKeyUp = null;
this._onKeyDown = null;
}
attach(el) {
if (!el) el = document.body;
this.el = el;
// Make element focusable
el.querySelector("canvas").setAttribute("tabindex", 0);
this._onKeyUp = (e) => {
if (document.activeElement.tagName == "INPUT") return;
if (typeof this.keyBindings[e.code] !== "undefined") {
const event = this.keyBindings[e.code];
if (typeof event === "function") {
event();
}
else {
this.keyState[event] = false;
if (!this.visualizer.isPlaying() &&
Object.values(this.keyState).every((v) => !v)) {
}
}
e.preventDefault();
}
};
this._onKeyDown = (e) => {
if (document.activeElement.tagName == "INPUT") return;
if (typeof this.keyBindings[e.code] !== "undefined") {
const event = this.keyBindings[e.code];
if (typeof event !== "function") {
this.keyState[event] = true;
if (!this.visualizer.isPlaying()) {
// Run the Pixi event loop while keys are down
this.visualizer.application.start();
}
}
e.preventDefault();
}
};
el.addEventListener("keyup", this._onKeyUp);
el.addEventListener("keydown", this._onKeyDown);
}
destroy() {
this.el.removeEventListener("keyup", this._onKeyUp);
this.el.removeEventListener("keydown", this._onKeyDown);
this.visualizer = null;
}
handleKeys(dt) {
dt *= this.visualizer.timeStep * this.visualizer.scrubSpeed * 50;
if (this.keyState["scrubBackwards"]) {
this.visualizer.pause();
this.visualizer.advanceTime(-dt);
this.visualizer.render();
}
else if (this.keyState["scrubForwards"]) {
this.visualizer.pause();
this.visualizer.advanceTime(dt);
this.visualizer.render(dt);
}
if (this.keyState["panUp"]) {
this.visualizer.camera.panBy(0, 1);
}
else if (this.keyState["panDown"]) {
this.visualizer.camera.panBy(0, -1);
}
if (this.keyState["panLeft"]) {
this.visualizer.camera.panBy(1, 0);
}
else if (this.keyState["panRight"]) {
this.visualizer.camera.panBy(-1, 0);
}
if (this.keyState["zoomIn"]) {
this.visualizer.camera.zoomBy(0.5, 0.5, dt);
}
else if (this.keyState["zoomOut"]) {
this.visualizer.camera.zoomBy(0.5, 0.5, -dt);
}
}
}
| {
"pile_set_name": "Github"
} |
export function union<T>(...sets: Set<T>[]): Set<T> {
const result = new Set<T>()
for (const set of sets) {
for (const item of set) {
result.add(item)
}
}
return result
}
export function intersection<T>(set: Set<T>, ...sets: Set<T>[]): Set<T> {
const result = new Set<T>()
top: for (const item of set) {
for (const other of sets) {
if (!other.has(item))
continue top
}
result.add(item)
}
return result
}
export function difference<T>(set: Set<T>, ...sets: Set<T>[]): Set<T> {
const result = new Set<T>(set)
for (const item of union(...sets)) {
result.delete(item)
}
return result
}
| {
"pile_set_name": "Github"
} |
/*
* Copyright 2010-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.rekognition.model.transform;
import com.amazonaws.services.rekognition.model.*;
import com.amazonaws.transform.SimpleTypeJsonUnmarshallers.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.json.AwsJsonReader;
/**
* JSON unmarshaller for POJO Emotion
*/
class EmotionJsonUnmarshaller implements Unmarshaller<Emotion, JsonUnmarshallerContext> {
public Emotion unmarshall(JsonUnmarshallerContext context) throws Exception {
AwsJsonReader reader = context.getReader();
if (!reader.isContainer()) {
reader.skipValue();
return null;
}
Emotion emotion = new Emotion();
reader.beginObject();
while (reader.hasNext()) {
String name = reader.nextName();
if (name.equals("Type")) {
emotion.setType(StringJsonUnmarshaller.getInstance()
.unmarshall(context));
} else if (name.equals("Confidence")) {
emotion.setConfidence(FloatJsonUnmarshaller.getInstance()
.unmarshall(context));
} else {
reader.skipValue();
}
}
reader.endObject();
return emotion;
}
private static EmotionJsonUnmarshaller instance;
public static EmotionJsonUnmarshaller getInstance() {
if (instance == null)
instance = new EmotionJsonUnmarshaller();
return instance;
}
}
| {
"pile_set_name": "Github"
} |
/* TEMPLATE GENERATED TESTCASE FILE
Filename: CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53a.c
Label Definition File: CWE122_Heap_Based_Buffer_Overflow__c_CWE805.string.label.xml
Template File: sources-sink-53a.tmpl.c
*/
/*
* @description
* CWE: 122 Heap Based Buffer Overflow
* BadSource: Allocate using malloc() and set data pointer to a small buffer
* GoodSource: Allocate using malloc() and set data pointer to a large buffer
* Sink: snprintf
* BadSink : Copy string to data using snprintf
* Flow Variant: 53 Data flow: data passed as an argument from one function through two others to a fourth; all four functions are in different source files
*
* */
#include "std_testcase.h"
#include <wchar.h>
#ifdef _WIN32
#define SNPRINTF _snwprintf
#else
#define SNPRINTF snprintf
#endif
#ifndef OMITBAD
/* bad function declaration */
void CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53b_badSink(wchar_t * data);
void CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53_bad()
{
wchar_t * data;
data = NULL;
/* FLAW: Allocate and point data to a small buffer that is smaller than the large buffer used in the sinks */
data = (wchar_t *)malloc(50*sizeof(wchar_t));
data[0] = L'\0'; /* null terminate */
CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53b_badSink(data);
}
#endif /* OMITBAD */
#ifndef OMITGOOD
/* good function declaration */
void CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53b_goodG2BSink(wchar_t * data);
/* goodG2B uses the GoodSource with the BadSink */
static void goodG2B()
{
wchar_t * data;
data = NULL;
/* FIX: Allocate and point data to a large buffer that is at least as large as the large buffer used in the sink */
data = (wchar_t *)malloc(100*sizeof(wchar_t));
data[0] = L'\0'; /* null terminate */
CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53b_goodG2BSink(data);
}
void CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53_good()
{
goodG2B();
}
#endif /* OMITGOOD */
/* Below is the main(). It is only used when building this testcase on
* its own for testing or for building a binary to use in testing binary
* analysis tools. It is not used when compiling all the testcases as one
* application, which is how source code analysis tools are tested.
*/
#ifdef INCLUDEMAIN
int main(int argc, char * argv[])
{
/* seed randomness */
srand( (unsigned)time(NULL) );
#ifndef OMITGOOD
printLine("Calling good()...");
CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53_good();
printLine("Finished good()");
#endif /* OMITGOOD */
#ifndef OMITBAD
printLine("Calling bad()...");
CWE122_Heap_Based_Buffer_Overflow__c_CWE805_wchar_t_snprintf_53_bad();
printLine("Finished bad()");
#endif /* OMITBAD */
return 0;
}
#endif
| {
"pile_set_name": "Github"
} |
/*=============================================================================
Copyright (c) 2011 Eric Niebler
Distributed under the Boost Software License, Version 1.0. (See accompanying
file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
==============================================================================*/
#if !defined(BOOST_FUSION_ACCUMULATE_FWD_HPP_INCLUDED)
#define BOOST_FUSION_ACCUMULATE_FWD_HPP_INCLUDED
#include <boost/fusion/support/config.hpp>
#include <boost/fusion/support/is_sequence.hpp>
#include <boost/utility/enable_if.hpp>
namespace boost { namespace fusion
{
namespace result_of
{
template <typename Sequence, typename State, typename F>
struct accumulate;
}
template <typename Sequence, typename State, typename F>
BOOST_FUSION_GPU_ENABLED
typename
lazy_enable_if<
traits::is_sequence<Sequence>
, result_of::accumulate<Sequence, State const, F>
>::type
accumulate(Sequence& seq, State const& state, F f);
template <typename Sequence, typename State, typename F>
BOOST_FUSION_GPU_ENABLED
typename
lazy_enable_if<
traits::is_sequence<Sequence>
, result_of::accumulate<Sequence const, State const, F>
>::type
accumulate(Sequence const& seq, State const& state, F f);
}}
#endif
| {
"pile_set_name": "Github"
} |
// Copyright (c) 2014-present, Facebook, Inc. All rights reserved.
//
// You are hereby granted a non-exclusive, worldwide, royalty-free license to use,
// copy, modify, and distribute this software in source code or binary form for use
// in connection with the web services and APIs provided by Facebook.
//
// As with any software that integrates with the Facebook platform, your use of
// this software is subject to the Facebook Developer Principles and Policies
// [http://developers.facebook.com/policy/]. This copyright notice shall be
// included in all copies or substantial portions of the software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
// FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
// COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
// IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
// CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
//
// @generated
//
"com_accountkit_account_verified" = "Korisnički račun potvrđen!";
"com_accountkit_button_begin" = "Započni";
"com_accountkit_button_cancel" = "Odustani";
"com_accountkit_button_confirm" = "Potvrdi";
"com_accountkit_button_continue" = "Nastavi";
"com_accountkit_button_edit" = "UREDI";
"com_accountkit_button_log_in" = "Prijavi se";
"com_accountkit_button_next" = "Dalje";
"com_accountkit_button_ok" = "U redu";
"com_accountkit_button_resend_code_in" = "Ponovo pošaljite SMS za %1$d";
"com_accountkit_button_resend_sms" = "Ponovo pošalji SMS";
"com_accountkit_button_send" = "Pošalji";
"com_accountkit_button_send_code_in_call" = "Primite poziv";
"com_accountkit_button_send_code_in_call_details" = "Preuzmite kôd u glasovnom pozivu";
"com_accountkit_button_send_code_in_call_from_facebook_details" = "Preuzmite kôd u glasovnom pozivu iz Facebooka";
"com_accountkit_button_send_code_in_fb" = "Preuzmite kôd na Facebooku";
"com_accountkit_button_send_code_in_fb_details" = "Kôd će stići u obliku obavijesti s Facebooka";
"com_accountkit_button_start" = "Početak";
"com_accountkit_button_start_over" = "Pokušajte ponovno";
"com_accountkit_button_submit" = "Pošalji";
"com_accountkit_check_email" = "Otvorite e-poštu";
"com_accountkit_code_sent_to" = "Potvrdite svoj broj mobitela %1$@";
"com_accountkit_confirmation_code_agreement" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a> te <a href=\"%4$@\">pravila o upotrebi kolačića</a>.";
"com_accountkit_confirmation_code_agreement_app_privacy_policy" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a>, <a href=\"%4$@\">pravila o upotrebi kolačića</a> i <a href=\"%5$@\">Pravila o zaštiti privatnosti</a> koje primjenjuje %6$@.";
"com_accountkit_confirmation_code_agreement_app_privacy_policy_and_terms" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a>, <a href=\"%4$@\">pravila o upotrebi kolačića</a> i <a href=\"%5$@\">Pravila o zaštiti privatnosti</a>, kao i <a href=\"%6$@\">Uvjeta pružanja usluge</a> koje primjenjuje %7$@.";
"com_accountkit_confirmation_code_agreement_app_privacy_policy_and_terms_instant_verification" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a>, <a href=\"%4$@\">pravila o upotrebi kolačića</a> te <a href=\"%5$@\">Pravila o zaštiti privatnosti</a> te <a href=\"%6$@\">Uvjeta pružanja usluge</a> koje primjenjuje %7$@. <a href=\"%8$@\">Saznajte više</a> o tome kako smo potvrdili vaš račun.";
"com_accountkit_confirmation_code_agreement_app_privacy_policy_instant_verification" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a>, <a href=\"%4$@\">pravila o upotrebi kolačića</a> te <a href=\"%5$@\">Pravila o zaštiti privatnosti</a> koje primjenjuje %6$@. <a href=\"%7$@\">Saznajte više</a> o tome kako smo potvrdili vaš račun.";
"com_accountkit_confirmation_code_agreement_instant_verification" = "Dodirnite %1$@ za prihvaćanje Facebookovih <a href=\"%2$@\">Uvjeta</a>, <a href=\"%3$@\">Pravila o upotrebi podataka</a> te <a href=\"%4$@\">pravila o upotrebi kolačića</a>. <a href=\"%5$@\">Saznajte više</a> o tome kako smo potvrdili vaš račun.";
"com_accountkit_confirmation_code_text" = "Nije mi stigao kôd";
"com_accountkit_confirmation_code_title" = "Unesite svoj kôd";
"com_accountkit_email_invalid" = "Adresa e-pošte nije točna. Pokušajte ponovo.";
"com_accountkit_email_loading_title" = "Potvrđujemo adresu e-pošte...";
"com_accountkit_email_login_retry_title" = "Potvrdite adresu e-pošte";
"com_accountkit_email_login_text" = "Dodirnite %1$@ kako biste dobili potvrdu putem e-pošte od Facebookova alata Account Kit i lakše upotrebljavali %2$@. Za to vam ne treba korisnički račun za Facebook. <a href=\"%3$@\">Saznajte kako se Facebook služi vašim podacima</a>.";
"com_accountkit_email_login_title" = "Unesite adresu e-pošte";
"com_accountkit_email_not_received" = "Ponovno pošalji poruku e-pošte";
"com_accountkit_email_verify_title" = "Otvorite poruku e-pošte i potvrdite adresu e-pošte";
"com_accountkit_enter_code_sent_to" = "Unesite kôd poslan na %1$@";
"com_accountkit_error_title" = "Došlo je do sistemske pogreške. Pokušajte ponovo.";
"com_accountkit_facebook_code_entry_title" = "Unesite svoj kôd s Facebooka";
"com_accountkit_ios_button_back" = "Natrag";
"com_accountkit_ios_country_code_title" = "Odaberite svoju državu";
"com_accountkit_ios_phone_login_phone_number_placeholder" = "Broj";
"com_accountkit_ios_phone_login_text" = "Dodirnite %1$@ kako biste SMS-om dobili potvrdu od Facebookova alata Account Kit. %2$@ vam polakšava registraciju s pomoću Facebookove tehnologije, ali ne morate imati korisnički račun za Facebook. Možda se naplaćuje cijena za SMS i prijenos podataka. <a href=\"%3$@\">Saznajte kako Facebook upotrebljava vaše podatke</a>.";
"com_accountkit_ios_phone_sending_sms" = "Slanje SMS-a s kodom";
"com_accountkit_logging_in" = "Prijava u tijeku\U2026";
"com_accountkit_other_ways_to_get_code" = "Drugi načini za preuzimanje koda:";
"com_accountkit_phone_error_title" = "Unesite valjani broj mobitela.";
"com_accountkit_phone_loading_title" = "Potvrda broja...";
"com_accountkit_phone_login_retry_title" = "Potvrdite broj mobitela";
"com_accountkit_phone_login_text" = "Dodirnite %1$@ kako biste potvrdili korisnički račun za Facebookov alat Account Kit. Za njega vam ne treba korisnički račun za Facebook. Možda ćete primiti SMS radi potvrde broja telefona. Možda se naplaćuje naknada za slanje poruka i prijenos podataka. <a href=\"%2$@\">Saznajte kako se Facebook služi vašim podacima</a>.";
"com_accountkit_phone_login_title" = "Unesite broj mobilnog telefona";
"com_accountkit_phone_sending_code_on_fb_title" = "Slanje koda na Facebooku...";
"com_accountkit_resend_check" = "Provjerite dolazne SMS-e";
"com_accountkit_resend_email_text" = "Pošalji novu poruku e-pošte";
"com_accountkit_resend_title" = "Ako niste primili kôd:";
"com_accountkit_return_title" = "Vraćamo vas u %1$@...";
"com_accountkit_sent_title" = "Poslano!";
"com_accountkit_success_title" = "Potvrđeno!";
"com_accountkit_toolbar_title" = "Prijavite se u aplikaciju %1$@";
"com_accountkit_verify_confirmation_code_title" = "Nismo mogli potvrditi kôd. Pokušajte ponovo:";
"com_accountkit_verify_title" = "Potvrda koda...";
"com_accountkit_voice_call_code_entry_title" = "Unesite svoj kôd s Voice Calla";
| {
"pile_set_name": "Github"
} |
<!DOCTYPE HTML>
<!-- NewPage -->
<html lang="en">
<head>
<!-- Generated by javadoc -->
<title>JTabbedPane.ModelListener (Java SE 12 & JDK 12 )</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta name="keywords" content="javax.swing.JTabbedPane.ModelListener class">
<meta name="keywords" content="stateChanged()">
<link rel="stylesheet" type="text/css" href="../../../stylesheet.css" title="Style">
<link rel="stylesheet" type="text/css" href="../../../jquery/jquery-ui.css" title="Style">
<script type="text/javascript" src="../../../script.js"></script>
<script type="text/javascript" src="../../../jquery/jszip/dist/jszip.min.js"></script>
<script type="text/javascript" src="../../../jquery/jszip-utils/dist/jszip-utils.min.js"></script>
<!--[if IE]>
<script type="text/javascript" src="../../../jquery/jszip-utils/dist/jszip-utils-ie.min.js"></script>
<![endif]-->
<script type="text/javascript" src="../../../jquery/jquery-3.3.1.js"></script>
<script type="text/javascript" src="../../../jquery/jquery-migrate-3.0.1.js"></script>
<script type="text/javascript" src="../../../jquery/jquery-ui.js"></script>
</head>
<body>
<script type="text/javascript"><!--
try {
if (location.href.indexOf('is-external=true') == -1) {
parent.document.title="JTabbedPane.ModelListener (Java SE 12 & JDK 12 )";
}
}
catch(err) {
}
//-->
var pathtoroot = "../../../";
var useModuleDirectories = true;
loadScripts(document, 'script');</script>
<noscript>
<div>JavaScript is disabled on your browser.</div>
</noscript>
<header role="banner">
<nav role="navigation">
<div class="fixedNav">
<!-- ========= START OF TOP NAVBAR ======= -->
<div class="topNav"><a id="navbar.top">
<!-- -->
</a>
<div class="skipNav"><a href="#skip.navbar.top" title="Skip navigation links">Skip navigation links</a></div>
<a id="navbar.top.firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../index.html">Overview</a></li>
<li><a href="../../module-summary.html">Module</a></li>
<li><a href="package-summary.html">Package</a></li>
<li class="navBarCell1Rev">Class</li>
<li><a href="class-use/JTabbedPane.ModelListener.html">Use</a></li>
<li><a href="package-tree.html">Tree</a></li>
<li><a href="../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../help-doc.html">Help</a></li>
</ul>
<div class="aboutLanguage"><div style="margin-top: 14px;"><strong>Java SE 12 & JDK 12</strong> </div></div>
</div>
<div class="subNav">
<div>
<ul class="subNavList">
<li>Summary: </li>
<li>Nested | </li>
<li>Field | </li>
<li><a href="#constructor.summary">Constr</a> | </li>
<li><a href="#method.summary">Method</a></li>
</ul>
<ul class="subNavList">
<li>Detail: </li>
<li>Field | </li>
<li><a href="#constructor.detail">Constr</a> | </li>
<li>Method</li>
</ul>
</div>
<ul class="navListSearch">
<li><label for="search">SEARCH:</label>
<input type="text" id="search" value="search" disabled="disabled">
<input type="reset" id="reset" value="reset" disabled="disabled">
</li>
</ul>
</div>
<a id="skip.navbar.top">
<!-- -->
</a>
<!-- ========= END OF TOP NAVBAR ========= -->
</div>
<div class="navPadding"> </div>
<script type="text/javascript"><!--
$('.navPadding').css('padding-top', $('.fixedNav').css("height"));
//-->
</script>
</nav>
</header>
<!-- ======== START OF CLASS DATA ======== -->
<main role="main">
<div class="header">
<div class="subTitle"><span class="moduleLabelInType">Module</span> <a href="../../module-summary.html">java.desktop</a></div>
<div class="subTitle"><span class="packageLabelInType">Package</span> <a href="package-summary.html">javax.swing</a></div>
<h2 title="Class JTabbedPane.ModelListener" class="title">Class JTabbedPane.ModelListener</h2>
</div>
<div class="contentContainer">
<ul class="inheritance">
<li><a href="../../../java.base/java/lang/Object.html" title="class in java.lang">java.lang.Object</a></li>
<li>
<ul class="inheritance">
<li>javax.swing.JTabbedPane.ModelListener</li>
</ul>
</li>
</ul>
<div class="description">
<ul class="blockList">
<li class="blockList">
<dl>
<dt>All Implemented Interfaces:</dt>
<dd><code><a href="../../../java.base/java/io/Serializable.html" title="interface in java.io">Serializable</a></code>, <code><a href="../../../java.base/java/util/EventListener.html" title="interface in java.util">EventListener</a></code>, <code><a href="event/ChangeListener.html" title="interface in javax.swing.event">ChangeListener</a></code></dd>
</dl>
<dl>
<dt>Enclosing class:</dt>
<dd><a href="JTabbedPane.html" title="class in javax.swing">JTabbedPane</a></dd>
</dl>
<hr>
<pre>protected class <span class="typeNameLabel">JTabbedPane.ModelListener</span>
extends <a href="../../../java.base/java/lang/Object.html" title="class in java.lang">Object</a>
implements <a href="event/ChangeListener.html" title="interface in javax.swing.event">ChangeListener</a>, <a href="../../../java.base/java/io/Serializable.html" title="interface in java.io">Serializable</a></pre>
<div class="block">We pass <code>ModelChanged</code> events along to the listeners with
the tabbedpane (instead of the model itself) as the event source.</div>
</li>
</ul>
</div>
<div class="summary">
<ul class="blockList">
<li class="blockList">
<!-- ======== CONSTRUCTOR SUMMARY ======== -->
<section role="region">
<ul class="blockList">
<li class="blockList"><a id="constructor.summary">
<!-- -->
</a>
<h3>Constructor Summary</h3>
<div class="memberSummary">
<table>
<caption><span>Constructors</span><span class="tabEnd"> </span></caption>
<tr>
<th class="colFirst" scope="col">Modifier</th>
<th class="colSecond" scope="col">Constructor</th>
<th class="colLast" scope="col">Description</th>
</tr>
<tbody>
<tr class="altColor">
<td class="colFirst"><code>protected </code></td>
<th class="colConstructorName" scope="row"><code><span class="memberNameLink"><a href="#%3Cinit%3E()">ModelListener</a></span>()</code></th>
<td class="colLast"> </td>
</tr>
</tbody>
</table>
</div>
</li>
</ul>
</section>
<!-- ========== METHOD SUMMARY =========== -->
<section role="region">
<ul class="blockList">
<li class="blockList"><a id="method.summary">
<!-- -->
</a>
<h3>Method Summary</h3>
<ul class="blockList">
<li class="blockList"><a id="methods.inherited.from.class.java.lang.Object">
<!-- -->
</a>
<h3>Methods declared in class java.lang.<a href="../../../java.base/java/lang/Object.html" title="class in java.lang">Object</a></h3>
<code><a href="../../../java.base/java/lang/Object.html#clone()">clone</a>, <a href="../../../java.base/java/lang/Object.html#equals(java.lang.Object)">equals</a>, <a href="../../../java.base/java/lang/Object.html#finalize()">finalize</a>, <a href="../../../java.base/java/lang/Object.html#getClass()">getClass</a>, <a href="../../../java.base/java/lang/Object.html#hashCode()">hashCode</a>, <a href="../../../java.base/java/lang/Object.html#notify()">notify</a>, <a href="../../../java.base/java/lang/Object.html#notifyAll()">notifyAll</a>, <a href="../../../java.base/java/lang/Object.html#toString()">toString</a>, <a href="../../../java.base/java/lang/Object.html#wait()">wait</a>, <a href="../../../java.base/java/lang/Object.html#wait(long)">wait</a>, <a href="../../../java.base/java/lang/Object.html#wait(long,int)">wait</a></code></li>
</ul>
<ul class="blockList">
<li class="blockList"><a id="methods.inherited.from.class.javax.swing.event.ChangeListener">
<!-- -->
</a>
<h3>Methods declared in interface javax.swing.event.<a href="event/ChangeListener.html" title="interface in javax.swing.event">ChangeListener</a></h3>
<code><a href="event/ChangeListener.html#stateChanged(javax.swing.event.ChangeEvent)">stateChanged</a></code></li>
</ul>
</li>
</ul>
</section>
</li>
</ul>
</div>
<div class="details">
<ul class="blockList">
<li class="blockList">
<!-- ========= CONSTRUCTOR DETAIL ======== -->
<section role="region">
<ul class="blockList">
<li class="blockList"><a id="constructor.detail">
<!-- -->
</a>
<h3>Constructor Detail</h3>
<a id="<init>()">
<!-- -->
</a>
<ul class="blockListLast">
<li class="blockList">
<h4>ModelListener</h4>
<pre>protected ModelListener()</pre>
</li>
</ul>
</li>
</ul>
</section>
</li>
</ul>
</div>
</div>
</main>
<!-- ========= END OF CLASS DATA ========= -->
<footer role="contentinfo">
<nav role="navigation">
<!-- ======= START OF BOTTOM NAVBAR ====== -->
<div class="bottomNav"><a id="navbar.bottom">
<!-- -->
</a>
<div class="skipNav"><a href="#skip.navbar.bottom" title="Skip navigation links">Skip navigation links</a></div>
<a id="navbar.bottom.firstrow">
<!-- -->
</a>
<ul class="navList" title="Navigation">
<li><a href="../../../index.html">Overview</a></li>
<li><a href="../../module-summary.html">Module</a></li>
<li><a href="package-summary.html">Package</a></li>
<li class="navBarCell1Rev">Class</li>
<li><a href="class-use/JTabbedPane.ModelListener.html">Use</a></li>
<li><a href="package-tree.html">Tree</a></li>
<li><a href="../../../deprecated-list.html">Deprecated</a></li>
<li><a href="../../../index-files/index-1.html">Index</a></li>
<li><a href="../../../help-doc.html">Help</a></li>
</ul>
<div class="aboutLanguage"><div style="margin-top: 14px;"><strong>Java SE 12 & JDK 12</strong> </div></div>
</div>
<div class="subNav">
<div>
<ul class="subNavList">
<li>Summary: </li>
<li>Nested | </li>
<li>Field | </li>
<li><a href="#constructor.summary">Constr</a> | </li>
<li><a href="#method.summary">Method</a></li>
</ul>
<ul class="subNavList">
<li>Detail: </li>
<li>Field | </li>
<li><a href="#constructor.detail">Constr</a> | </li>
<li>Method</li>
</ul>
</div>
</div>
<a id="skip.navbar.bottom">
<!-- -->
</a>
<!-- ======== END OF BOTTOM NAVBAR ======= -->
</nav>
<p class="legalCopy"><small><a href="https://bugreport.java.com/bugreport/">Report a bug or suggest an enhancement</a><br> For further API reference and developer documentation see the <a href="https://docs.oracle.com/pls/topic/lookup?ctx=javase12.0.2&id=homepage" target="_blank">Java SE Documentation</a>, which contains more detailed, developer-targeted descriptions with conceptual overviews, definitions of terms, workarounds, and working code examples.<br> Java is a trademark or registered trademark of Oracle and/or its affiliates in the US and other countries.<br> <a href="../../../../legal/copyright.html">Copyright</a> © 1993, 2019, Oracle and/or its affiliates, 500 Oracle Parkway, Redwood Shores, CA 94065 USA.<br>All rights reserved. Use is subject to <a href="https://www.oracle.com/technetwork/java/javase/terms/license/java12.0.2speclicense.html">license terms</a> and the <a href="https://www.oracle.com/technetwork/java/redist-137594.html">documentation redistribution policy</a>. <!-- Version 12.0.2+10 --></small></p>
</footer>
</body>
</html>
| {
"pile_set_name": "Github"
} |
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;; Copyright (C) Kongsberg Oil & Gas Technologies. All rights reserved.
;;; Written by [email protected], 2000-09-26.
;;; Eval following region
;; Make scene-graph and first viewer
(define text (new-sotext3))
(define interpolatefloat (new-sointerpolatefloat))
(-> (-> text 'string) 'connectFrom (-> interpolatefloat 'output))
(define viewer (new-soxtexaminerviewer))
(-> viewer 'setscenegraph text)
(-> viewer 'show)
;;; End initial eval-region
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;; Test input fields of SoInterpolateFloat engine, play around ;;;;;;;;;;;;
(set-mfield-values! (-> interpolatefloat 'input0) 0 '(0 1 2 3))
(set-mfield-values! (-> interpolatefloat 'input1) 0 '(1 2 0 3))
(-> (-> interpolatefloat 'alpha) 'setvalue 0.1)
;; Copy the scenegraph.
(define viewer-copy (new-soxtexaminerviewer))
(-> viewer-copy 'setscenegraph (-> (-> viewer 'getscenegraph) 'copy 1))
(-> viewer-copy 'show)
;; Export scenegraph with engine.
(define writeaction (new-sowriteaction))
(-> writeaction 'apply (-> viewer 'getscenegraph))
;; Read scenegraph with engine in it.
(let ((buffer "#Inventor V2.1 ascii\n\n Text3 { string \"\" = InterpolateFloat { alpha 0.7 input0 [ 0, 1 ] input1 [ 2, 3] } . output }")
(input (new-soinput)))
(-> input 'setbuffer (void-cast buffer) (string-length buffer))
(let ((sceneroot (sodb::readall input)))
(-> viewer 'setscenegraph sceneroot)
(-> viewer 'viewall)))
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;; Confirmed and potential bugs. ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;; Fixed bugs and false alarms (ex-possible bugs) ;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
;;;;; scratch area ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
(-> (-> text 'justification) 'setValue SoText3::CENTER)
(-> (-> text 'parts) 'setValue SoText3::ALL)
(-> (-> text 'string) 'disconnect)
(-> viewer 'viewAll)
| {
"pile_set_name": "Github"
} |
// SPDX-License-Identifier: GPL-2.0
#include <sys/types.h>
#include <errno.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <regex.h>
#include "../../../util/debug.h"
#include "../../../util/header.h"
static inline void
cpuid(unsigned int op, unsigned int *a, unsigned int *b, unsigned int *c,
unsigned int *d)
{
__asm__ __volatile__ (".byte 0x53\n\tcpuid\n\t"
"movl %%ebx, %%esi\n\t.byte 0x5b"
: "=a" (*a),
"=S" (*b),
"=c" (*c),
"=d" (*d)
: "a" (op));
}
static int
__get_cpuid(char *buffer, size_t sz, const char *fmt)
{
unsigned int a, b, c, d, lvl;
int family = -1, model = -1, step = -1;
int nb;
char vendor[16];
cpuid(0, &lvl, &b, &c, &d);
strncpy(&vendor[0], (char *)(&b), 4);
strncpy(&vendor[4], (char *)(&d), 4);
strncpy(&vendor[8], (char *)(&c), 4);
vendor[12] = '\0';
if (lvl >= 1) {
cpuid(1, &a, &b, &c, &d);
family = (a >> 8) & 0xf; /* bits 11 - 8 */
model = (a >> 4) & 0xf; /* Bits 7 - 4 */
step = a & 0xf;
/* extended family */
if (family == 0xf)
family += (a >> 20) & 0xff;
/* extended model */
if (family >= 0x6)
model += ((a >> 16) & 0xf) << 4;
}
nb = scnprintf(buffer, sz, fmt, vendor, family, model, step);
/* look for end marker to ensure the entire data fit */
if (strchr(buffer, '$')) {
buffer[nb-1] = '\0';
return 0;
}
return ENOBUFS;
}
int
get_cpuid(char *buffer, size_t sz)
{
return __get_cpuid(buffer, sz, "%s,%u,%u,%u$");
}
char *
get_cpuid_str(struct perf_pmu *pmu __maybe_unused)
{
char *buf = malloc(128);
if (buf && __get_cpuid(buf, 128, "%s-%u-%X-%X$") < 0) {
free(buf);
return NULL;
}
return buf;
}
/* Full CPUID format for x86 is vendor-family-model-stepping */
static bool is_full_cpuid(const char *id)
{
const char *tmp = id;
int count = 0;
while ((tmp = strchr(tmp, '-')) != NULL) {
count++;
tmp++;
}
if (count == 3)
return true;
return false;
}
int strcmp_cpuid_str(const char *mapcpuid, const char *id)
{
regex_t re;
regmatch_t pmatch[1];
int match;
bool full_mapcpuid = is_full_cpuid(mapcpuid);
bool full_cpuid = is_full_cpuid(id);
/*
* Full CPUID format is required to identify a platform.
* Error out if the cpuid string is incomplete.
*/
if (full_mapcpuid && !full_cpuid) {
pr_info("Invalid CPUID %s. Full CPUID is required, "
"vendor-family-model-stepping\n", id);
return 1;
}
if (regcomp(&re, mapcpuid, REG_EXTENDED) != 0) {
/* Warn unable to generate match particular string. */
pr_info("Invalid regular expression %s\n", mapcpuid);
return 1;
}
match = !regexec(&re, id, 1, pmatch, 0);
regfree(&re);
if (match) {
size_t match_len = (pmatch[0].rm_eo - pmatch[0].rm_so);
size_t cpuid_len;
/* If the full CPUID format isn't required,
* ignoring the stepping.
*/
if (!full_mapcpuid && full_cpuid)
cpuid_len = strrchr(id, '-') - id;
else
cpuid_len = strlen(id);
/* Verify the entire string matched. */
if (match_len == cpuid_len)
return 0;
}
return 1;
}
| {
"pile_set_name": "Github"
} |
#ifdef __OBJC__
#import <UIKit/UIKit.h>
#else
#ifndef FOUNDATION_EXPORT
#if defined(__cplusplus)
#define FOUNDATION_EXPORT extern "C"
#else
#define FOUNDATION_EXPORT extern
#endif
#endif
#endif
FOUNDATION_EXPORT double QuickLayoutVersionNumber;
FOUNDATION_EXPORT const unsigned char QuickLayoutVersionString[];
| {
"pile_set_name": "Github"
} |
//
// Deprecated.swift
// RxDataSources
//
// Created by Krunoslav Zaher on 10/8/17.
// Copyright © 2017 kzaher. All rights reserved.
//
#if os(iOS) || os(tvOS)
extension CollectionViewSectionedDataSource {
@available(*, deprecated, renamed: "configureSupplementaryView")
public var supplementaryViewFactory: ConfigureSupplementaryView? {
get {
return self.configureSupplementaryView
}
set {
self.configureSupplementaryView = newValue
}
}
}
#endif
| {
"pile_set_name": "Github"
} |
'use strict';
angular.module("ngLocale", [], ["$provide", function($provide) {
var PLURAL_CATEGORY = {ZERO: "zero", ONE: "one", TWO: "two", FEW: "few", MANY: "many", OTHER: "other"};
$provide.value("$locale", {
"DATETIME_FORMATS": {
"AMPMS": [
"AM",
"PM"
],
"DAY": [
"dimanche",
"lundi",
"mardi",
"mercredi",
"jeudi",
"vendredi",
"samedi"
],
"ERANAMES": [
"avant J\u00e9sus-Christ",
"apr\u00e8s J\u00e9sus-Christ"
],
"ERAS": [
"av. J.-C.",
"ap. J.-C."
],
"FIRSTDAYOFWEEK": 0,
"MONTH": [
"janvier",
"f\u00e9vrier",
"mars",
"avril",
"mai",
"juin",
"juillet",
"ao\u00fbt",
"septembre",
"octobre",
"novembre",
"d\u00e9cembre"
],
"SHORTDAY": [
"dim.",
"lun.",
"mar.",
"mer.",
"jeu.",
"ven.",
"sam."
],
"SHORTMONTH": [
"janv.",
"f\u00e9vr.",
"mars",
"avr.",
"mai",
"juin",
"juil.",
"ao\u00fbt",
"sept.",
"oct.",
"nov.",
"d\u00e9c."
],
"STANDALONEMONTH": [
"janvier",
"f\u00e9vrier",
"mars",
"avril",
"mai",
"juin",
"juillet",
"ao\u00fbt",
"septembre",
"octobre",
"novembre",
"d\u00e9cembre"
],
"WEEKENDRANGE": [
5,
6
],
"fullDate": "EEEE d MMMM y",
"longDate": "d MMMM y",
"medium": "d MMM y HH:mm:ss",
"mediumDate": "d MMM y",
"mediumTime": "HH:mm:ss",
"short": "dd/MM/y HH:mm",
"shortDate": "dd/MM/y",
"shortTime": "HH:mm"
},
"NUMBER_FORMATS": {
"CURRENCY_SYM": "\u20ac",
"DECIMAL_SEP": ",",
"GROUP_SEP": "\u00a0",
"PATTERNS": [
{
"gSize": 3,
"lgSize": 3,
"maxFrac": 3,
"minFrac": 0,
"minInt": 1,
"negPre": "-",
"negSuf": "",
"posPre": "",
"posSuf": ""
},
{
"gSize": 3,
"lgSize": 3,
"maxFrac": 2,
"minFrac": 2,
"minInt": 1,
"negPre": "-",
"negSuf": "\u00a0\u00a4",
"posPre": "",
"posSuf": "\u00a0\u00a4"
}
]
},
"id": "fr-gp",
"localeID": "fr_GP",
"pluralCat": function(n, opt_precision) { var i = n | 0; if (i == 0 || i == 1) { return PLURAL_CATEGORY.ONE; } return PLURAL_CATEGORY.OTHER;}
});
}]);
| {
"pile_set_name": "Github"
} |
#![allow(unused_imports)]
use super::*;
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
extern "C" {
# [wasm_bindgen (extends = :: js_sys :: Object , js_name = CustomElementRegistry , typescript_type = "CustomElementRegistry")]
#[derive(Debug, Clone, PartialEq, Eq)]
#[doc = "The `CustomElementRegistry` class."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`*"]
pub type CustomElementRegistry;
# [wasm_bindgen (catch , method , structural , js_class = "CustomElementRegistry" , js_name = define)]
#[doc = "The `define()` method."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry/define)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`*"]
pub fn define(
this: &CustomElementRegistry,
name: &str,
function_constructor: &::js_sys::Function,
) -> Result<(), JsValue>;
#[cfg(feature = "ElementDefinitionOptions")]
# [wasm_bindgen (catch , method , structural , js_class = "CustomElementRegistry" , js_name = define)]
#[doc = "The `define()` method."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry/define)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`, `ElementDefinitionOptions`*"]
pub fn define_with_options(
this: &CustomElementRegistry,
name: &str,
function_constructor: &::js_sys::Function,
options: &ElementDefinitionOptions,
) -> Result<(), JsValue>;
# [wasm_bindgen (method , structural , js_class = "CustomElementRegistry" , js_name = get)]
#[doc = "The `get()` method."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry/get)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`*"]
pub fn get(this: &CustomElementRegistry, name: &str) -> ::wasm_bindgen::JsValue;
#[cfg(feature = "Node")]
# [wasm_bindgen (method , structural , js_class = "CustomElementRegistry" , js_name = upgrade)]
#[doc = "The `upgrade()` method."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry/upgrade)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`, `Node`*"]
pub fn upgrade(this: &CustomElementRegistry, root: &Node);
# [wasm_bindgen (catch , method , structural , js_class = "CustomElementRegistry" , js_name = whenDefined)]
#[doc = "The `whenDefined()` method."]
#[doc = ""]
#[doc = "[MDN Documentation](https://developer.mozilla.org/en-US/docs/Web/API/CustomElementRegistry/whenDefined)"]
#[doc = ""]
#[doc = "*This API requires the following crate features to be activated: `CustomElementRegistry`*"]
pub fn when_defined(
this: &CustomElementRegistry,
name: &str,
) -> Result<::js_sys::Promise, JsValue>;
}
| {
"pile_set_name": "Github"
} |
// +build !appengine
package mail
import (
"bytes"
"encoding/base64"
"errors"
"fmt"
"io"
"mime/multipart"
"net/smtp"
"net/textproto"
"strings"
"sort"
"gnd.la/util/stringutil"
)
var (
errNoAdminEmail = errors.New("mail.Admin specified as a mail destinary, but no mail.AdminEmail() has been set")
crlf = []byte("\r\n")
)
func joinAddrs(addrs []string) (string, error) {
var values []string
for _, v := range addrs {
if v == Admin {
addr := AdminEmail()
if addr == "" {
return "", errNoAdminEmail
}
addrs, err := ParseAddressList(addr)
if err != nil {
return "", fmt.Errorf("invalid admin address %q: %s", addr, err)
}
values = append(values, addrs...)
continue
}
values = append(values, v)
}
return strings.Join(values, ", "), nil
}
func makeBoundary() string {
return "Gondola-Boundary-" + stringutil.Random(32)
}
func sendMail(to []string, cc []string, bcc []string, msg *Message) error {
from := Config.DefaultFrom
server := Config.MailServer
if msg.Server != "" {
server = msg.Server
}
if msg.From != "" {
from = msg.From
}
if from == "" {
return errNoFrom
}
var auth smtp.Auth
cram, username, password, server := parseServer(server)
if username != "" || password != "" {
if cram {
auth = smtp.CRAMMD5Auth(username, password)
} else {
auth = smtp.PlainAuth("", username, password, server)
}
}
var buf bytes.Buffer
headers := msg.Headers
if headers == nil {
headers = make(Headers)
}
if msg.Subject != "" {
headers["Subject"] = msg.Subject
}
headers["From"] = from
if msg.ReplyTo != "" {
headers["Reply-To"] = msg.ReplyTo
}
var err error
if len(to) > 0 {
headers["To"], err = joinAddrs(to)
if err != nil {
return err
}
for ii, v := range to {
if v == Admin {
if Config.AdminEmail == "" {
return errNoAdminEmail
}
to[ii] = Config.AdminEmail
}
}
}
if len(cc) > 0 {
headers["Cc"], err = joinAddrs(cc)
if err != nil {
return err
}
}
if len(bcc) > 0 {
headers["Bcc"], err = joinAddrs(bcc)
if err != nil {
return err
}
}
hk := make([]string, 0, len(headers))
for k := range headers {
hk = append(hk, k)
}
sort.Strings(hk)
for _, k := range hk {
buf.WriteString(fmt.Sprintf("%s: %s\r\n", k, headers[k]))
}
buf.WriteString("MIME-Version: 1.0\r\n")
mw := multipart.NewWriter(&buf)
mw.SetBoundary(makeBoundary())
// Create a multipart mixed first
fmt.Fprintf(&buf, "Content-Type: multipart/mixed;\r\n\tboundary=%q\r\n\r\n", mw.Boundary())
var bodyWriter *multipart.Writer
if msg.TextBody != "" && msg.HTMLBody != "" {
boundary := makeBoundary()
outerHeader := make(textproto.MIMEHeader)
// First part is a multipart/alternative, which contains the text and html bodies
outerHeader.Set("Content-Type", fmt.Sprintf("multipart/alternative; boundary=%q", boundary))
iw, err := mw.CreatePart(outerHeader)
if err != nil {
return err
}
bodyWriter = multipart.NewWriter(iw)
bodyWriter.SetBoundary(boundary)
} else {
bodyWriter = mw
}
if msg.TextBody != "" {
textHeader := make(textproto.MIMEHeader)
textHeader.Set("Content-Type", "text/plain; charset=UTF-8")
tpw, err := bodyWriter.CreatePart(textHeader)
if err != nil {
return err
}
if _, err := io.WriteString(tpw, msg.TextBody); err != nil {
return err
}
tpw.Write(crlf)
tpw.Write(crlf)
}
attached := make(map[*Attachment]bool)
if msg.HTMLBody != "" {
var htmlAttachments []*Attachment
for _, v := range msg.Attachments {
if v.ContentID != "" && strings.Contains(msg.HTMLBody, fmt.Sprintf("cid:%s", v.ContentID)) {
htmlAttachments = append(htmlAttachments, v)
attached[v] = true
}
}
var htmlWriter *multipart.Writer
if len(htmlAttachments) > 0 {
relatedHeader := make(textproto.MIMEHeader)
relatedBoundary := makeBoundary()
relatedHeader.Set("Content-Type", fmt.Sprintf("multipart/related; boundary=%q; type=\"text/html\"", relatedBoundary))
rw, err := bodyWriter.CreatePart(relatedHeader)
if err != nil {
return err
}
htmlWriter = multipart.NewWriter(rw)
htmlWriter.SetBoundary(relatedBoundary)
} else {
htmlWriter = bodyWriter
}
htmlHeader := make(textproto.MIMEHeader)
htmlHeader.Set("Content-Type", "text/html; charset=UTF-8")
thw, err := htmlWriter.CreatePart(htmlHeader)
if err != nil {
return err
}
if _, err := io.WriteString(thw, msg.HTMLBody); err != nil {
return err
}
thw.Write(crlf)
thw.Write(crlf)
for _, v := range htmlAttachments {
attachmentHeader := make(textproto.MIMEHeader)
attachmentHeader.Set("Content-Disposition", "inline")
attachmentHeader.Set("Content-Id", fmt.Sprintf("<%s>", v.ContentID))
attachmentHeader.Set("Content-Transfer-Encoding", "base64")
attachmentHeader.Set("Content-Type", v.ContentType)
aw, err := htmlWriter.CreatePart(attachmentHeader)
if err != nil {
return err
}
b := make([]byte, base64.StdEncoding.EncodedLen(len(v.Data)))
base64.StdEncoding.Encode(b, v.Data)
aw.Write(b)
}
if htmlWriter != bodyWriter {
if err := htmlWriter.Close(); err != nil {
return err
}
}
}
if bodyWriter != mw {
if err := bodyWriter.Close(); err != nil {
return err
}
}
for _, v := range msg.Attachments {
if attached[v] {
continue
}
attachmentHeader := make(textproto.MIMEHeader)
attachmentHeader.Set("Content-Disposition", fmt.Sprintf("attachment; filename=%q", v.Name))
attachmentHeader.Set("Content-Transfer-Encoding", "base64")
attachmentHeader.Set("Content-Type", v.ContentType)
aw, err := mw.CreatePart(attachmentHeader)
if err != nil {
return err
}
b := make([]byte, base64.StdEncoding.EncodedLen(len(v.Data)))
base64.StdEncoding.Encode(b, v.Data)
aw.Write(b)
}
if err := mw.Close(); err != nil {
return err
}
if server == "echo" {
printer(buf.String())
return nil
}
return smtp.SendMail(server, auth, from, to, buf.Bytes())
}
func parseServer(server string) (bool, string, string, string) {
// Check if the server includes authentication info
cram := false
var username string
var password string
if idx := strings.LastIndex(server, "@"); idx >= 0 {
var credentials string
credentials, server = server[:idx], server[idx+1:]
if strings.HasPrefix(credentials, "cram?") {
credentials = credentials[5:]
cram = true
}
colon := strings.Index(credentials, ":")
if colon >= 0 {
username = credentials[:colon]
if colon < len(credentials)-1 {
password = credentials[colon+1:]
}
} else {
username = credentials
}
}
return cram, username, password, server
}
| {
"pile_set_name": "Github"
} |
# --------------------------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# --------------------------------------------------------------------------------------------
from azure.cli.core.commands import CliCommandType
from ._format import (
registry_output_format,
usage_output_format,
policy_output_format,
credential_output_format,
webhook_output_format,
webhook_get_config_output_format,
webhook_list_events_output_format,
webhook_ping_output_format,
replication_output_format,
endpoints_output_format,
build_output_format,
task_output_format,
task_identity_format,
taskrun_output_format,
run_output_format,
helm_list_output_format,
helm_show_output_format,
scope_map_output_format,
token_output_format,
token_credential_output_format,
agentpool_output_format
)
from ._client_factory import (
cf_acr_registries,
cf_acr_replications,
cf_acr_webhooks,
cf_acr_tasks,
cf_acr_taskruns,
cf_acr_runs,
cf_acr_scope_maps,
cf_acr_tokens,
cf_acr_token_credentials,
cf_acr_private_endpoint_connections,
cf_acr_agentpool
)
def load_command_table(self, _): # pylint: disable=too-many-statements
acr_custom_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.custom#{}',
table_transformer=registry_output_format,
client_factory=cf_acr_registries
)
acr_login_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.custom#{}'
)
acr_import_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.import#{}',
client_factory=cf_acr_registries
)
acr_policy_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.policy#{}',
table_transformer=policy_output_format,
client_factory=cf_acr_registries
)
acr_cred_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.credential#{}',
table_transformer=credential_output_format,
client_factory=cf_acr_registries
)
acr_repo_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.repository#{}'
)
acr_webhook_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.webhook#{}',
table_transformer=webhook_output_format,
client_factory=cf_acr_webhooks
)
acr_replication_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.replication#{}',
table_transformer=replication_output_format,
client_factory=cf_acr_replications
)
acr_build_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.build#{}',
table_transformer=build_output_format,
client_factory=cf_acr_runs
)
acr_run_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.run#{}',
table_transformer=run_output_format,
client_factory=cf_acr_runs
)
acr_pack_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.pack#{}',
table_transformer=run_output_format,
client_factory=cf_acr_runs
)
acr_task_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.task#{}',
table_transformer=task_output_format,
client_factory=cf_acr_tasks
)
acr_taskrun_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.taskrun#{}',
table_transformer=taskrun_output_format,
client_factory=cf_acr_taskruns
)
acr_helm_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.helm#{}'
)
acr_network_rule_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.network_rule#{}',
client_factory=cf_acr_registries
)
acr_check_health_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.check_health#{}'
)
acr_scope_map_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.scope_map#{}',
table_transformer=scope_map_output_format,
client_factory=cf_acr_scope_maps
)
acr_token_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.token#{}',
table_transformer=token_output_format,
client_factory=cf_acr_tokens
)
acr_token_credential_generate_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.token#{}',
table_transformer=token_credential_output_format,
client_factory=cf_acr_token_credentials
)
acr_agentpool_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.agentpool#{}',
table_transformer=agentpool_output_format,
client_factory=cf_acr_agentpool
)
acr_private_endpoint_connection_util = CliCommandType(
operations_tmpl='azure.cli.command_modules.acr.private_endpoint_connection#{}',
client_factory=cf_acr_private_endpoint_connections
)
with self.command_group('acr', acr_custom_util) as g:
g.command('check-name', 'acr_check_name', table_transformer=None)
g.command('list', 'acr_list')
g.command('create', 'acr_create')
g.command('delete', 'acr_delete')
g.show_command('show', 'acr_show')
g.command('show-usage', 'acr_show_usage', table_transformer=usage_output_format)
g.command('show-endpoints', 'acr_show_endpoints', table_transformer=endpoints_output_format)
g.generic_update_command('update',
getter_name='acr_update_get',
setter_name='acr_update_set',
custom_func_name='acr_update_custom',
custom_func_type=acr_custom_util,
client_factory=cf_acr_registries)
with self.command_group('acr', acr_login_util) as g:
g.command('login', 'acr_login')
with self.command_group('acr', acr_import_util) as g:
g.command('import', 'acr_import')
with self.command_group('acr credential', acr_cred_util) as g:
g.show_command('show', 'acr_credential_show')
g.command('renew', 'acr_credential_renew')
with self.command_group('acr repository', acr_repo_util) as g:
g.command('list', 'acr_repository_list')
g.command('show-tags', 'acr_repository_show_tags')
g.command('show-manifests', 'acr_repository_show_manifests')
g.show_command('show', 'acr_repository_show')
g.command('update', 'acr_repository_update')
g.command('delete', 'acr_repository_delete')
g.command('untag', 'acr_repository_untag')
with self.command_group('acr webhook', acr_webhook_util) as g:
g.command('list', 'acr_webhook_list')
g.command('create', 'acr_webhook_create')
g.command('delete', 'acr_webhook_delete')
g.show_command('show', 'acr_webhook_show')
g.command('get-config', 'acr_webhook_get_config', table_transformer=webhook_get_config_output_format)
g.command('list-events', 'acr_webhook_list_events', table_transformer=webhook_list_events_output_format)
g.command('ping', 'acr_webhook_ping', table_transformer=webhook_ping_output_format)
g.generic_update_command('update',
getter_name='acr_webhook_update_get',
setter_name='acr_webhook_update_set',
custom_func_name='acr_webhook_update_custom',
custom_func_type=acr_webhook_util,
client_factory=cf_acr_webhooks)
with self.command_group('acr replication', acr_replication_util) as g:
g.command('list', 'acr_replication_list')
g.command('create', 'acr_replication_create')
g.command('delete', 'acr_replication_delete')
g.show_command('show', 'acr_replication_show')
g.generic_update_command('update',
getter_name='acr_replication_update_get',
setter_name='acr_replication_update_set',
custom_func_name='acr_replication_update_custom',
custom_func_type=acr_replication_util,
client_factory=cf_acr_replications)
with self.command_group('acr', acr_build_util) as g:
g.command('build', 'acr_build', supports_no_wait=True)
with self.command_group('acr', acr_run_util) as g:
g.command('run', 'acr_run', supports_no_wait=True)
with self.command_group('acr pack', acr_pack_util, is_preview=True) as g:
g.command('build', 'acr_pack_build', supports_no_wait=True)
with self.command_group('acr task', acr_task_util) as g:
g.command('create', 'acr_task_create')
g.show_command('show', 'acr_task_show')
g.command('list', 'acr_task_list')
g.command('delete', 'acr_task_delete')
g.command('update', 'acr_task_update')
g.command('identity assign', 'acr_task_identity_assign')
g.command('identity remove', 'acr_task_identity_remove')
g.command('identity show', 'acr_task_identity_show', table_transformer=task_identity_format)
g.command('credential add', 'acr_task_credential_add')
g.command('credential update', 'acr_task_credential_update')
g.command('credential remove', 'acr_task_credential_remove')
g.command('credential list', 'acr_task_credential_list')
g.command('timer add', 'acr_task_timer_add')
g.command('timer update', 'acr_task_timer_update')
g.command('timer remove', 'acr_task_timer_remove')
g.command('timer list', 'acr_task_timer_list')
g.command('run', 'acr_task_run', client_factory=cf_acr_runs,
table_transformer=run_output_format, supports_no_wait=True)
g.command('list-runs', 'acr_task_list_runs', client_factory=cf_acr_runs,
table_transformer=run_output_format)
g.command('show-run', 'acr_task_show_run', client_factory=cf_acr_runs,
table_transformer=run_output_format)
g.command('cancel-run', 'acr_task_cancel_run', client_factory=cf_acr_runs,
table_transformer=None)
g.command('update-run', 'acr_task_update_run', client_factory=cf_acr_runs,
table_transformer=run_output_format)
g.command('logs', 'acr_task_logs', client_factory=cf_acr_runs,
table_transformer=None)
with self.command_group('acr taskrun', acr_taskrun_util, is_preview=True) as g:
g.command('list', 'acr_taskrun_list')
g.command('delete', 'acr_taskrun_delete')
g.show_command('show', 'acr_taskrun_show')
g.command('logs', 'acr_taskrun_logs', client_factory=cf_acr_runs,
table_transformer=None)
with self.command_group('acr config content-trust', acr_policy_util) as g:
g.show_command('show', 'acr_config_content_trust_show')
g.command('update', 'acr_config_content_trust_update')
with self.command_group('acr config retention', acr_policy_util, is_preview=True) as g:
g.show_command('show', 'acr_config_retention_show')
g.command('update', 'acr_config_retention_update')
def _helm_deprecate_message(self):
msg = "This {} has been deprecated and will be removed in future release.".format(self.object_type)
msg += " Use '{}' instead.".format(self.redirect)
msg += " For more information go to"
msg += " https://aka.ms/acr/helm"
return msg
with self.command_group('acr helm', acr_helm_util,
deprecate_info=self.deprecate(redirect="helm v3",
message_func=_helm_deprecate_message)) as g:
g.command('list', 'acr_helm_list', table_transformer=helm_list_output_format)
g.show_command('show', 'acr_helm_show', table_transformer=helm_show_output_format)
g.command('delete', 'acr_helm_delete')
g.command('push', 'acr_helm_push')
g.command('repo add', 'acr_helm_repo_add')
g.command('install-cli', 'acr_helm_install_cli', is_preview=True)
with self.command_group('acr network-rule', acr_network_rule_util) as g:
g.command('list', 'acr_network_rule_list')
g.command('add', 'acr_network_rule_add')
g.command('remove', 'acr_network_rule_remove')
with self.command_group('acr', acr_check_health_util) as g:
g.command('check-health', 'acr_check_health')
with self.command_group('acr scope-map', acr_scope_map_util, is_preview=True) as g:
g.command('create', 'acr_scope_map_create')
g.command('delete', 'acr_scope_map_delete')
g.command('update', 'acr_scope_map_update')
g.show_command('show', 'acr_scope_map_show')
g.command('list', 'acr_scope_map_list')
with self.command_group('acr token', acr_token_util, is_preview=True) as g:
g.command('create', 'acr_token_create')
g.command('delete', 'acr_token_delete')
g.command('update', 'acr_token_update')
g.show_command('show', 'acr_token_show')
g.command('list', 'acr_token_list')
g.command('credential delete', 'acr_token_credential_delete')
with self.command_group('acr token credential', acr_token_credential_generate_util) as g:
g.command('generate', 'acr_token_credential_generate')
with self.command_group('acr agentpool', acr_agentpool_util, is_preview=True) as g:
g.command('create', 'acr_agentpool_create', supports_no_wait=True)
g.command('update', 'acr_agentpool_update', supports_no_wait=True)
g.command('delete', 'acr_agentpool_delete', supports_no_wait=True)
g.command('list', 'acr_agentpool_list')
g.show_command('show', 'acr_agentpool_show')
with self.command_group('acr private-endpoint-connection', acr_private_endpoint_connection_util) as g:
g.command('delete', 'delete')
g.show_command('show', 'show')
g.command('list', 'list_connections')
g.command('approve', 'approve')
g.command('reject', 'reject')
with self.command_group('acr private-link-resource', acr_custom_util) as g:
g.command('list', 'list_private_link_resources')
with self.command_group('acr identity', acr_custom_util) as g:
g.show_command('show', 'show_identity')
g.command('assign', 'assign_identity')
g.command('remove', 'remove_identity')
with self.command_group('acr encryption', acr_custom_util) as g:
g.show_command('show', 'show_encryption')
g.command('rotate-key', "rotate_key")
| {
"pile_set_name": "Github"
} |
fileFormatVersion: 2
guid: f2526e443ce2efb4cb419bda6a034df6
timeCreated: 1490642887
licenseType: Pro
NativeFormatImporter:
userData:
assetBundleName:
assetBundleVariant:
| {
"pile_set_name": "Github"
} |
//===--- SwiftPrivateThreadExtras.swift -----------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2018 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
//
// This file contains wrappers for pthread APIs that are less painful to use
// than the C APIs.
//
//===----------------------------------------------------------------------===//
#if canImport(Darwin)
import Darwin
#elseif canImport(Glibc)
import Glibc
#elseif os(Windows)
import MSVCRT
import WinSDK
#endif
/// An abstract base class to encapsulate the context necessary to invoke
/// a block from pthread_create.
internal class ThreadBlockContext {
/// Execute the block, and return an `UnsafeMutablePointer` to memory
/// allocated with `UnsafeMutablePointer.alloc` containing the result of the
/// block.
func run() -> UnsafeMutableRawPointer { fatalError("abstract") }
}
internal class ThreadBlockContextImpl<Argument, Result>: ThreadBlockContext {
let block: (Argument) -> Result
let arg: Argument
init(block: @escaping (Argument) -> Result, arg: Argument) {
self.block = block
self.arg = arg
super.init()
}
override func run() -> UnsafeMutableRawPointer {
let result = UnsafeMutablePointer<Result>.allocate(capacity: 1)
result.initialize(to: block(arg))
return UnsafeMutableRawPointer(result)
}
}
/// Entry point for `pthread_create` that invokes a block context.
internal func invokeBlockContext(
_ contextAsVoidPointer: UnsafeMutableRawPointer?
) -> UnsafeMutableRawPointer! {
// The context is passed in +1; we're responsible for releasing it.
let context = Unmanaged<ThreadBlockContext>
.fromOpaque(contextAsVoidPointer!)
.takeRetainedValue()
return context.run()
}
#if os(Windows)
public typealias ThreadHandle = HANDLE
#else
public typealias ThreadHandle = pthread_t
#if os(Linux) || os(Android)
internal func _make_pthread_t() -> pthread_t {
return pthread_t()
}
#else
internal func _make_pthread_t() -> pthread_t? {
return nil
}
#endif
#endif
/// Block-based wrapper for `pthread_create`.
public func _stdlib_thread_create_block<Argument, Result>(
_ start_routine: @escaping (Argument) -> Result,
_ arg: Argument
) -> (CInt, ThreadHandle?) {
let context = ThreadBlockContextImpl(block: start_routine, arg: arg)
// We hand ownership off to `invokeBlockContext` through its void context
// argument.
let contextAsVoidPointer = Unmanaged.passRetained(context).toOpaque()
#if os(Windows)
let threadID =
_beginthreadex(nil, 0, { invokeBlockContext($0)!
.assumingMemoryBound(to: UInt32.self).pointee },
contextAsVoidPointer, 0, nil)
if threadID == 0 {
return (errno, nil)
} else {
return (0, ThreadHandle(bitPattern: threadID))
}
#else
var threadID = _make_pthread_t()
let result = pthread_create(&threadID, nil,
{ invokeBlockContext($0) }, contextAsVoidPointer)
if result == 0 {
return (result, threadID)
} else {
return (result, nil)
}
#endif
}
/// Block-based wrapper for `pthread_join`.
public func _stdlib_thread_join<Result>(
_ thread: ThreadHandle,
_ resultType: Result.Type
) -> (CInt, Result?) {
#if os(Windows)
let result = WaitForSingleObject(thread, INFINITE)
guard result == WAIT_OBJECT_0 else { return (CInt(result), nil) }
var dwResult: DWORD = 0
GetExitCodeThread(thread, &dwResult)
CloseHandle(thread)
let value: Result = withUnsafePointer(to: &dwResult) {
$0.withMemoryRebound(to: Result.self, capacity: 1) {
$0.pointee
}
}
return (CInt(result), value)
#else
var threadResultRawPtr: UnsafeMutableRawPointer?
let result = pthread_join(thread, &threadResultRawPtr)
if result == 0 {
let threadResultPtr = threadResultRawPtr!.assumingMemoryBound(
to: Result.self)
let threadResult = threadResultPtr.pointee
threadResultPtr.deinitialize(count: 1)
threadResultPtr.deallocate()
return (result, threadResult)
} else {
return (result, nil)
}
#endif
}
public class _stdlib_Barrier {
var _threadBarrier: _stdlib_thread_barrier_t
var _threadBarrierPtr: UnsafeMutablePointer<_stdlib_thread_barrier_t> {
return _getUnsafePointerToStoredProperties(self)
.assumingMemoryBound(to: _stdlib_thread_barrier_t.self)
}
public init(threadCount: Int) {
self._threadBarrier = _stdlib_thread_barrier_t()
let ret = _stdlib_thread_barrier_init(
_threadBarrierPtr, CUnsignedInt(threadCount))
if ret != 0 {
fatalError("_stdlib_thread_barrier_init() failed")
}
}
deinit {
_stdlib_thread_barrier_destroy(_threadBarrierPtr)
}
public func wait() {
let ret = _stdlib_thread_barrier_wait(_threadBarrierPtr)
if !(ret == 0 || ret == _stdlib_THREAD_BARRIER_SERIAL_THREAD) {
fatalError("_stdlib_thread_barrier_wait() failed")
}
}
}
| {
"pile_set_name": "Github"
} |
version https://git-lfs.github.com/spec/v1
oid sha256:2aa3ff0c0b8e1a5f7ce4fce717d6632d3687f95d420401bb2f5fe3dde75cab09
size 6557
| {
"pile_set_name": "Github"
} |
package plugins.core.combat;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import akka.actor.ActorRef;
import akka.actor.ActorSelection;
import akka.actor.Props;
import akka.actor.UntypedActor;
import io.gamemachine.chat.ChatSubscriptions;
import io.gamemachine.core.ActorUtil;
import io.gamemachine.core.GameEntityManager;
import io.gamemachine.core.GameEntityManagerService;
import io.gamemachine.core.GameMachineLoader;
import io.gamemachine.core.PlayerMessage;
import io.gamemachine.grid.Grid;
import io.gamemachine.grid.GridService;
import io.gamemachine.messages.CombatLog;
import io.gamemachine.messages.GameMessage;
import io.gamemachine.messages.PlayerSkill;
import io.gamemachine.messages.RegionInfo;
import io.gamemachine.messages.StatusEffect;
import io.gamemachine.messages.StatusEffectTarget;
import io.gamemachine.messages.TrackData;
import io.gamemachine.messages.Zone;
import io.gamemachine.regions.ZoneService;
public class StatusEffectManager extends UntypedActor {
private static final Logger logger = LoggerFactory.getLogger(StatusEffectManager.class);
public static String name = StatusEffectManager.class.getSimpleName();
public static boolean combatLogEnabled = true;
public static List<GridSet> gridsets = new ArrayList<GridSet>();
public static Set<String> handlerZones = new HashSet<String>();
public static void tell(String gridName, String zone, StatusEffectTarget statusEffectTarget, ActorRef sender) {
String actorName = null;
if (!hasEffects(statusEffectTarget)) {
logger.warn("No effects found for skill " + statusEffectTarget.skillRequest.playerSkill.id);
return;
}
if (activeEffectCount(statusEffectTarget) > 0) {
actorName = ActiveEffectHandler.actorName(gridName, zone);
ActorSelection sel = ActorUtil.getSelectionByName(actorName);
sel.tell(statusEffectTarget.clone(), sender);
}
if (passiveEffectCount(statusEffectTarget) > 0) {
actorName = PassiveEffectHandler.actorName(gridName, zone);
ActorSelection sel = ActorUtil.getSelectionByName(actorName);
sel.tell(statusEffectTarget.clone(), sender);
}
}
private static boolean hasEffects(StatusEffectTarget statusEffectTarget) {
if (StatusEffectData.skillEffects.containsKey(statusEffectTarget.skillRequest.playerSkill.id)) {
return true;
} else {
return false;
}
}
private static int passiveEffectCount(StatusEffectTarget statusEffectTarget) {
int count = 0;
for (StatusEffect statusEffect : StatusEffectData.skillEffects.get(statusEffectTarget.skillRequest.playerSkill.id)) {
if (statusEffect.type == StatusEffect.Type.AttributeMaxDecrease || statusEffect.type == StatusEffect.Type.AttributeMaxIncrease) {
count++;
}
}
return count;
}
private static int activeEffectCount(StatusEffectTarget statusEffectTarget) {
int count = 0;
for (StatusEffect statusEffect : StatusEffectData.skillEffects.get(statusEffectTarget.skillRequest.playerSkill.id)) {
if (statusEffect.type == StatusEffect.Type.AttributeDecrease || statusEffect.type == StatusEffect.Type.AttributeIncrease) {
count++;
}
}
return count;
}
public static int getEffectValue(StatusEffect statusEffect, PlayerSkill playerSkill, String characterId) {
GameEntityManager gameEntityManager = GameEntityManagerService.instance().getGameEntityManager();
return gameEntityManager.getEffectValue(statusEffect, playerSkill.id, characterId);
}
public static boolean inGroup(String playerId) {
String playerGroup = ChatSubscriptions.playerGroup(playerId);
if (playerGroup.equals("nogroup")) {
return false;
} else {
return true;
}
}
public static void skillUsed(PlayerSkill playerSkill, String characterId) {
GameEntityManager gameEntityManager = GameEntityManagerService.instance().getGameEntityManager();
gameEntityManager.skillUsed(playerSkill.id, characterId);
}
public static String playerGroup(String playerId) {
return ChatSubscriptions.playerGroup(playerId);
}
public static boolean inSameGroup(String playerId, String otherId) {
if (!inGroup(playerId)) {
return false;
}
String otherGroup = ChatSubscriptions.playerGroup(otherId);
if (playerGroup(playerId).equals(otherGroup)) {
return true;
} else {
return false;
}
}
public static boolean DeductCost(VitalsProxy vitalsProxy, StatusEffect statusEffect) {
if (statusEffect.resourceCost == 0) {
return true;
}
if (statusEffect.resource == StatusEffect.Resource.ResourceStamina) {
if (vitalsProxy.get("stamina") < statusEffect.resourceCost) {
//logger.warn("Insufficient stamina needed " + statusEffect.resourceCost);
return false;
}
vitalsProxy.subtract("stamina", statusEffect.resourceCost);
} else if (statusEffect.resource == StatusEffect.Resource.ResourceMagic) {
if (vitalsProxy.get("magic") < statusEffect.resourceCost) {
//logger.warn("Insufficient magic needed " + statusEffect.resourceCost);
return false;
}
vitalsProxy.subtract("magic", statusEffect.resourceCost);
}
return true;
}
public static void sendCombatDamage(VitalsProxy origin, VitalsProxy target, int value, CombatLog.Type type, String zone) {
GameEntityManager gameEntityManager = GameEntityManagerService.instance().getGameEntityManager();
gameEntityManager.combatDamage(origin, target, value, type);
if (!combatLogEnabled) {
return;
}
GameMessage msg = new GameMessage();
msg.combatLog = new CombatLog();
msg.combatLog.origin = origin.getEntityId();
msg.combatLog.target = target.getEntityId();
msg.combatLog.value = value;
msg.combatLog.type = type;
Grid grid = GridService.getInstance().getGrid(zone, "default");
TrackData trackData = grid.get(msg.combatLog.origin);
if (trackData != null) {
PlayerMessage.tell(msg, trackData.id);
}
trackData = grid.get(msg.combatLog.target);
if (trackData != null) {
PlayerMessage.tell(msg, trackData.id);
}
}
public StatusEffectManager() {
createDefaultEffectHandlers();
}
private void createDefaultEffectHandlers() {
int zoneCount = RegionInfo.db().findAll().size();
if (zoneCount == 0) {
logger.warn("Combat system requires at least one zone configured");
return;
}
for (Zone zone : ZoneService.staticZones()) {
createEffectHandler(zone.name);
}
}
public static void createEffectHandler(String zone) {
if (handlerZones.contains(zone)) {
logger.warn("effect handler already created for zone " + zone);
return;
}
GridSet gridSet = new GridSet();
gridSet.zone = zone;
GridService.getInstance().createForZone(zone);
gridSet.playerGrid = GridService.getInstance().getGrid(zone, "default");
gridSet.objectGrid = GridService.getInstance().getGrid(zone, "build_objects");
gridsets.add(gridSet);
GameMachineLoader.getActorSystem().actorOf(Props.create(ActiveEffectHandler.class, "default", zone),
ActiveEffectHandler.actorName("default", zone));
GameMachineLoader.getActorSystem().actorOf(Props.create(ActiveEffectHandler.class, "build_objects", zone),
ActiveEffectHandler.actorName("build_objects", zone));
GameMachineLoader.getActorSystem().actorOf(Props.create(PassiveEffectHandler.class, "default", zone),
PassiveEffectHandler.actorName("default", zone));
GameMachineLoader.getActorSystem().actorOf(Props.create(PassiveEffectHandler.class, "build_objects", zone),
PassiveEffectHandler.actorName("build_objects", zone));
GameMachineLoader.getActorSystem().actorOf(Props.create(VitalsRegen.class, zone),
VitalsRegen.actorName(zone));
handlerZones.add(zone);
}
@Override
public void onReceive(Object arg0) throws Exception {
// TODO Auto-generated method stub
}
}
| {
"pile_set_name": "Github"
} |
package com.trinity.sample.view.foucs
enum class AutoFocusTrigger {
GESTURE,
METHOD
}
| {
"pile_set_name": "Github"
} |
Escreva um **servidor** HTTP que recebe apenas requisições de POST e converte os caracteres no corpo da requisição para caixa-alta e retorna-os para o cliente.
Seu servidor deve "escutar" na porta provida a você pelo primeiro argumento para seu programa.
----------------------------------------------------------------------
## DICAS
Ainda que você não esteja restrito ao uso das capacidades de streaming dos objetos `request` e `response`, será muito mais fácil se você decidir usá-las.
Existe um grande número de pacotes diferentes no npm que você pode usar para *"transformar"* um streaming de dados enquanto ele está sendo passado. Para esse exercício, o pacote `through2-map` oferece a API mais simples.
`through2-map` permite que você crie um *stream transformador* usando apenas uma única função que recebe um bloco de dados e retorna um outro bloco de dados. Ela é designada para funcionar como um `Array#map()`, só que para streams:
```js
const map = require('through2-map')
inStream.pipe(map(function (chunk) {
return chunk.toString().split('').reverse().join('')
})).pipe(outStream)
```
No exemplo acima, a data que estamos recebendo de `inStream` é convertida para uma String (se já não estiver nesse formato), os caracteres são revertidos e o resultado é passado para o `outStream`. Sendo assim nós fizemos um reversor de caracteres! Lembre-se que o tamanho do bloco é determinado pelo fluxo e você tem muito pouco controle sobre ele para os dados que está recebendo.
Para instalar `through2-map` type:
```sh
$ npm install through2-map
```
Se você não possuir uma conexão à Internet, simplesmente crie uma pasta `node_modules` e copie o diretório inteiro para o módulo que você quiser usar de dentro do diretório de instalação do {{appname}}:
{rootdir:/node_modules/through2-map}
A documentação do through2-map foi instalada junto com o {appname} no seu sistema e você pode lê-los apontando seu navegador para cá:
{rootdir:/docs/through2-map.html}
| {
"pile_set_name": "Github"
} |
// mkerrors.sh -m32
// Code generated by the command above; see README.md. DO NOT EDIT.
// +build 386,darwin
// Code generated by cmd/cgo -godefs; DO NOT EDIT.
// cgo -godefs -- -m32 _const.go
package unix
import "syscall"
const (
AF_APPLETALK = 0x10
AF_CCITT = 0xa
AF_CHAOS = 0x5
AF_CNT = 0x15
AF_COIP = 0x14
AF_DATAKIT = 0x9
AF_DECnet = 0xc
AF_DLI = 0xd
AF_E164 = 0x1c
AF_ECMA = 0x8
AF_HYLINK = 0xf
AF_IEEE80211 = 0x25
AF_IMPLINK = 0x3
AF_INET = 0x2
AF_INET6 = 0x1e
AF_IPX = 0x17
AF_ISDN = 0x1c
AF_ISO = 0x7
AF_LAT = 0xe
AF_LINK = 0x12
AF_LOCAL = 0x1
AF_MAX = 0x28
AF_NATM = 0x1f
AF_NDRV = 0x1b
AF_NETBIOS = 0x21
AF_NS = 0x6
AF_OSI = 0x7
AF_PPP = 0x22
AF_PUP = 0x4
AF_RESERVED_36 = 0x24
AF_ROUTE = 0x11
AF_SIP = 0x18
AF_SNA = 0xb
AF_SYSTEM = 0x20
AF_UNIX = 0x1
AF_UNSPEC = 0x0
AF_UTUN = 0x26
ALTWERASE = 0x200
ATTR_BIT_MAP_COUNT = 0x5
ATTR_CMN_ACCESSMASK = 0x20000
ATTR_CMN_ACCTIME = 0x1000
ATTR_CMN_ADDEDTIME = 0x10000000
ATTR_CMN_BKUPTIME = 0x2000
ATTR_CMN_CHGTIME = 0x800
ATTR_CMN_CRTIME = 0x200
ATTR_CMN_DATA_PROTECT_FLAGS = 0x40000000
ATTR_CMN_DEVID = 0x2
ATTR_CMN_DOCUMENT_ID = 0x100000
ATTR_CMN_ERROR = 0x20000000
ATTR_CMN_EXTENDED_SECURITY = 0x400000
ATTR_CMN_FILEID = 0x2000000
ATTR_CMN_FLAGS = 0x40000
ATTR_CMN_FNDRINFO = 0x4000
ATTR_CMN_FSID = 0x4
ATTR_CMN_FULLPATH = 0x8000000
ATTR_CMN_GEN_COUNT = 0x80000
ATTR_CMN_GRPID = 0x10000
ATTR_CMN_GRPUUID = 0x1000000
ATTR_CMN_MODTIME = 0x400
ATTR_CMN_NAME = 0x1
ATTR_CMN_NAMEDATTRCOUNT = 0x80000
ATTR_CMN_NAMEDATTRLIST = 0x100000
ATTR_CMN_OBJID = 0x20
ATTR_CMN_OBJPERMANENTID = 0x40
ATTR_CMN_OBJTAG = 0x10
ATTR_CMN_OBJTYPE = 0x8
ATTR_CMN_OWNERID = 0x8000
ATTR_CMN_PARENTID = 0x4000000
ATTR_CMN_PAROBJID = 0x80
ATTR_CMN_RETURNED_ATTRS = 0x80000000
ATTR_CMN_SCRIPT = 0x100
ATTR_CMN_SETMASK = 0x41c7ff00
ATTR_CMN_USERACCESS = 0x200000
ATTR_CMN_UUID = 0x800000
ATTR_CMN_VALIDMASK = 0xffffffff
ATTR_CMN_VOLSETMASK = 0x6700
ATTR_FILE_ALLOCSIZE = 0x4
ATTR_FILE_CLUMPSIZE = 0x10
ATTR_FILE_DATAALLOCSIZE = 0x400
ATTR_FILE_DATAEXTENTS = 0x800
ATTR_FILE_DATALENGTH = 0x200
ATTR_FILE_DEVTYPE = 0x20
ATTR_FILE_FILETYPE = 0x40
ATTR_FILE_FORKCOUNT = 0x80
ATTR_FILE_FORKLIST = 0x100
ATTR_FILE_IOBLOCKSIZE = 0x8
ATTR_FILE_LINKCOUNT = 0x1
ATTR_FILE_RSRCALLOCSIZE = 0x2000
ATTR_FILE_RSRCEXTENTS = 0x4000
ATTR_FILE_RSRCLENGTH = 0x1000
ATTR_FILE_SETMASK = 0x20
ATTR_FILE_TOTALSIZE = 0x2
ATTR_FILE_VALIDMASK = 0x37ff
ATTR_VOL_ALLOCATIONCLUMP = 0x40
ATTR_VOL_ATTRIBUTES = 0x40000000
ATTR_VOL_CAPABILITIES = 0x20000
ATTR_VOL_DIRCOUNT = 0x400
ATTR_VOL_ENCODINGSUSED = 0x10000
ATTR_VOL_FILECOUNT = 0x200
ATTR_VOL_FSTYPE = 0x1
ATTR_VOL_INFO = 0x80000000
ATTR_VOL_IOBLOCKSIZE = 0x80
ATTR_VOL_MAXOBJCOUNT = 0x800
ATTR_VOL_MINALLOCATION = 0x20
ATTR_VOL_MOUNTEDDEVICE = 0x8000
ATTR_VOL_MOUNTFLAGS = 0x4000
ATTR_VOL_MOUNTPOINT = 0x1000
ATTR_VOL_NAME = 0x2000
ATTR_VOL_OBJCOUNT = 0x100
ATTR_VOL_QUOTA_SIZE = 0x10000000
ATTR_VOL_RESERVED_SIZE = 0x20000000
ATTR_VOL_SETMASK = 0x80002000
ATTR_VOL_SIGNATURE = 0x2
ATTR_VOL_SIZE = 0x4
ATTR_VOL_SPACEAVAIL = 0x10
ATTR_VOL_SPACEFREE = 0x8
ATTR_VOL_UUID = 0x40000
ATTR_VOL_VALIDMASK = 0xf007ffff
B0 = 0x0
B110 = 0x6e
B115200 = 0x1c200
B1200 = 0x4b0
B134 = 0x86
B14400 = 0x3840
B150 = 0x96
B1800 = 0x708
B19200 = 0x4b00
B200 = 0xc8
B230400 = 0x38400
B2400 = 0x960
B28800 = 0x7080
B300 = 0x12c
B38400 = 0x9600
B4800 = 0x12c0
B50 = 0x32
B57600 = 0xe100
B600 = 0x258
B7200 = 0x1c20
B75 = 0x4b
B76800 = 0x12c00
B9600 = 0x2580
BIOCFLUSH = 0x20004268
BIOCGBLEN = 0x40044266
BIOCGDLT = 0x4004426a
BIOCGDLTLIST = 0xc00c4279
BIOCGETIF = 0x4020426b
BIOCGHDRCMPLT = 0x40044274
BIOCGRSIG = 0x40044272
BIOCGRTIMEOUT = 0x4008426e
BIOCGSEESENT = 0x40044276
BIOCGSTATS = 0x4008426f
BIOCIMMEDIATE = 0x80044270
BIOCPROMISC = 0x20004269
BIOCSBLEN = 0xc0044266
BIOCSDLT = 0x80044278
BIOCSETF = 0x80084267
BIOCSETFNR = 0x8008427e
BIOCSETIF = 0x8020426c
BIOCSHDRCMPLT = 0x80044275
BIOCSRSIG = 0x80044273
BIOCSRTIMEOUT = 0x8008426d
BIOCSSEESENT = 0x80044277
BIOCVERSION = 0x40044271
BPF_A = 0x10
BPF_ABS = 0x20
BPF_ADD = 0x0
BPF_ALIGNMENT = 0x4
BPF_ALU = 0x4
BPF_AND = 0x50
BPF_B = 0x10
BPF_DIV = 0x30
BPF_H = 0x8
BPF_IMM = 0x0
BPF_IND = 0x40
BPF_JA = 0x0
BPF_JEQ = 0x10
BPF_JGE = 0x30
BPF_JGT = 0x20
BPF_JMP = 0x5
BPF_JSET = 0x40
BPF_K = 0x0
BPF_LD = 0x0
BPF_LDX = 0x1
BPF_LEN = 0x80
BPF_LSH = 0x60
BPF_MAJOR_VERSION = 0x1
BPF_MAXBUFSIZE = 0x80000
BPF_MAXINSNS = 0x200
BPF_MEM = 0x60
BPF_MEMWORDS = 0x10
BPF_MINBUFSIZE = 0x20
BPF_MINOR_VERSION = 0x1
BPF_MISC = 0x7
BPF_MSH = 0xa0
BPF_MUL = 0x20
BPF_NEG = 0x80
BPF_OR = 0x40
BPF_RELEASE = 0x30bb6
BPF_RET = 0x6
BPF_RSH = 0x70
BPF_ST = 0x2
BPF_STX = 0x3
BPF_SUB = 0x10
BPF_TAX = 0x0
BPF_TXA = 0x80
BPF_W = 0x0
BPF_X = 0x8
BRKINT = 0x2
BS0 = 0x0
BS1 = 0x8000
BSDLY = 0x8000
CFLUSH = 0xf
CLOCAL = 0x8000
CLOCK_MONOTONIC = 0x6
CLOCK_MONOTONIC_RAW = 0x4
CLOCK_MONOTONIC_RAW_APPROX = 0x5
CLOCK_PROCESS_CPUTIME_ID = 0xc
CLOCK_REALTIME = 0x0
CLOCK_THREAD_CPUTIME_ID = 0x10
CLOCK_UPTIME_RAW = 0x8
CLOCK_UPTIME_RAW_APPROX = 0x9
CR0 = 0x0
CR1 = 0x1000
CR2 = 0x2000
CR3 = 0x3000
CRDLY = 0x3000
CREAD = 0x800
CRTSCTS = 0x30000
CS5 = 0x0
CS6 = 0x100
CS7 = 0x200
CS8 = 0x300
CSIZE = 0x300
CSTART = 0x11
CSTATUS = 0x14
CSTOP = 0x13
CSTOPB = 0x400
CSUSP = 0x1a
CTL_HW = 0x6
CTL_KERN = 0x1
CTL_MAXNAME = 0xc
CTL_NET = 0x4
DLT_A429 = 0xb8
DLT_A653_ICM = 0xb9
DLT_AIRONET_HEADER = 0x78
DLT_AOS = 0xde
DLT_APPLE_IP_OVER_IEEE1394 = 0x8a
DLT_ARCNET = 0x7
DLT_ARCNET_LINUX = 0x81
DLT_ATM_CLIP = 0x13
DLT_ATM_RFC1483 = 0xb
DLT_AURORA = 0x7e
DLT_AX25 = 0x3
DLT_AX25_KISS = 0xca
DLT_BACNET_MS_TP = 0xa5
DLT_BLUETOOTH_HCI_H4 = 0xbb
DLT_BLUETOOTH_HCI_H4_WITH_PHDR = 0xc9
DLT_CAN20B = 0xbe
DLT_CAN_SOCKETCAN = 0xe3
DLT_CHAOS = 0x5
DLT_CHDLC = 0x68
DLT_CISCO_IOS = 0x76
DLT_C_HDLC = 0x68
DLT_C_HDLC_WITH_DIR = 0xcd
DLT_DBUS = 0xe7
DLT_DECT = 0xdd
DLT_DOCSIS = 0x8f
DLT_DVB_CI = 0xeb
DLT_ECONET = 0x73
DLT_EN10MB = 0x1
DLT_EN3MB = 0x2
DLT_ENC = 0x6d
DLT_ERF = 0xc5
DLT_ERF_ETH = 0xaf
DLT_ERF_POS = 0xb0
DLT_FC_2 = 0xe0
DLT_FC_2_WITH_FRAME_DELIMS = 0xe1
DLT_FDDI = 0xa
DLT_FLEXRAY = 0xd2
DLT_FRELAY = 0x6b
DLT_FRELAY_WITH_DIR = 0xce
DLT_GCOM_SERIAL = 0xad
DLT_GCOM_T1E1 = 0xac
DLT_GPF_F = 0xab
DLT_GPF_T = 0xaa
DLT_GPRS_LLC = 0xa9
DLT_GSMTAP_ABIS = 0xda
DLT_GSMTAP_UM = 0xd9
DLT_HHDLC = 0x79
DLT_IBM_SN = 0x92
DLT_IBM_SP = 0x91
DLT_IEEE802 = 0x6
DLT_IEEE802_11 = 0x69
DLT_IEEE802_11_RADIO = 0x7f
DLT_IEEE802_11_RADIO_AVS = 0xa3
DLT_IEEE802_15_4 = 0xc3
DLT_IEEE802_15_4_LINUX = 0xbf
DLT_IEEE802_15_4_NOFCS = 0xe6
DLT_IEEE802_15_4_NONASK_PHY = 0xd7
DLT_IEEE802_16_MAC_CPS = 0xbc
DLT_IEEE802_16_MAC_CPS_RADIO = 0xc1
DLT_IPFILTER = 0x74
DLT_IPMB = 0xc7
DLT_IPMB_LINUX = 0xd1
DLT_IPNET = 0xe2
DLT_IPOIB = 0xf2
DLT_IPV4 = 0xe4
DLT_IPV6 = 0xe5
DLT_IP_OVER_FC = 0x7a
DLT_JUNIPER_ATM1 = 0x89
DLT_JUNIPER_ATM2 = 0x87
DLT_JUNIPER_ATM_CEMIC = 0xee
DLT_JUNIPER_CHDLC = 0xb5
DLT_JUNIPER_ES = 0x84
DLT_JUNIPER_ETHER = 0xb2
DLT_JUNIPER_FIBRECHANNEL = 0xea
DLT_JUNIPER_FRELAY = 0xb4
DLT_JUNIPER_GGSN = 0x85
DLT_JUNIPER_ISM = 0xc2
DLT_JUNIPER_MFR = 0x86
DLT_JUNIPER_MLFR = 0x83
DLT_JUNIPER_MLPPP = 0x82
DLT_JUNIPER_MONITOR = 0xa4
DLT_JUNIPER_PIC_PEER = 0xae
DLT_JUNIPER_PPP = 0xb3
DLT_JUNIPER_PPPOE = 0xa7
DLT_JUNIPER_PPPOE_ATM = 0xa8
DLT_JUNIPER_SERVICES = 0x88
DLT_JUNIPER_SRX_E2E = 0xe9
DLT_JUNIPER_ST = 0xc8
DLT_JUNIPER_VP = 0xb7
DLT_JUNIPER_VS = 0xe8
DLT_LAPB_WITH_DIR = 0xcf
DLT_LAPD = 0xcb
DLT_LIN = 0xd4
DLT_LINUX_EVDEV = 0xd8
DLT_LINUX_IRDA = 0x90
DLT_LINUX_LAPD = 0xb1
DLT_LINUX_PPP_WITHDIRECTION = 0xa6
DLT_LINUX_SLL = 0x71
DLT_LOOP = 0x6c
DLT_LTALK = 0x72
DLT_MATCHING_MAX = 0xf5
DLT_MATCHING_MIN = 0x68
DLT_MFR = 0xb6
DLT_MOST = 0xd3
DLT_MPEG_2_TS = 0xf3
DLT_MPLS = 0xdb
DLT_MTP2 = 0x8c
DLT_MTP2_WITH_PHDR = 0x8b
DLT_MTP3 = 0x8d
DLT_MUX27010 = 0xec
DLT_NETANALYZER = 0xf0
DLT_NETANALYZER_TRANSPARENT = 0xf1
DLT_NFC_LLCP = 0xf5
DLT_NFLOG = 0xef
DLT_NG40 = 0xf4
DLT_NULL = 0x0
DLT_PCI_EXP = 0x7d
DLT_PFLOG = 0x75
DLT_PFSYNC = 0x12
DLT_PPI = 0xc0
DLT_PPP = 0x9
DLT_PPP_BSDOS = 0x10
DLT_PPP_ETHER = 0x33
DLT_PPP_PPPD = 0xa6
DLT_PPP_SERIAL = 0x32
DLT_PPP_WITH_DIR = 0xcc
DLT_PPP_WITH_DIRECTION = 0xa6
DLT_PRISM_HEADER = 0x77
DLT_PRONET = 0x4
DLT_RAIF1 = 0xc6
DLT_RAW = 0xc
DLT_RIO = 0x7c
DLT_SCCP = 0x8e
DLT_SITA = 0xc4
DLT_SLIP = 0x8
DLT_SLIP_BSDOS = 0xf
DLT_STANAG_5066_D_PDU = 0xed
DLT_SUNATM = 0x7b
DLT_SYMANTEC_FIREWALL = 0x63
DLT_TZSP = 0x80
DLT_USB = 0xba
DLT_USB_LINUX = 0xbd
DLT_USB_LINUX_MMAPPED = 0xdc
DLT_USER0 = 0x93
DLT_USER1 = 0x94
DLT_USER10 = 0x9d
DLT_USER11 = 0x9e
DLT_USER12 = 0x9f
DLT_USER13 = 0xa0
DLT_USER14 = 0xa1
DLT_USER15 = 0xa2
DLT_USER2 = 0x95
DLT_USER3 = 0x96
DLT_USER4 = 0x97
DLT_USER5 = 0x98
DLT_USER6 = 0x99
DLT_USER7 = 0x9a
DLT_USER8 = 0x9b
DLT_USER9 = 0x9c
DLT_WIHART = 0xdf
DLT_X2E_SERIAL = 0xd5
DLT_X2E_XORAYA = 0xd6
DT_BLK = 0x6
DT_CHR = 0x2
DT_DIR = 0x4
DT_FIFO = 0x1
DT_LNK = 0xa
DT_REG = 0x8
DT_SOCK = 0xc
DT_UNKNOWN = 0x0
DT_WHT = 0xe
ECHO = 0x8
ECHOCTL = 0x40
ECHOE = 0x2
ECHOK = 0x4
ECHOKE = 0x1
ECHONL = 0x10
ECHOPRT = 0x20
EVFILT_AIO = -0x3
EVFILT_EXCEPT = -0xf
EVFILT_FS = -0x9
EVFILT_MACHPORT = -0x8
EVFILT_PROC = -0x5
EVFILT_READ = -0x1
EVFILT_SIGNAL = -0x6
EVFILT_SYSCOUNT = 0xf
EVFILT_THREADMARKER = 0xf
EVFILT_TIMER = -0x7
EVFILT_USER = -0xa
EVFILT_VM = -0xc
EVFILT_VNODE = -0x4
EVFILT_WRITE = -0x2
EV_ADD = 0x1
EV_CLEAR = 0x20
EV_DELETE = 0x2
EV_DISABLE = 0x8
EV_DISPATCH = 0x80
EV_DISPATCH2 = 0x180
EV_ENABLE = 0x4
EV_EOF = 0x8000
EV_ERROR = 0x4000
EV_FLAG0 = 0x1000
EV_FLAG1 = 0x2000
EV_ONESHOT = 0x10
EV_OOBAND = 0x2000
EV_POLL = 0x1000
EV_RECEIPT = 0x40
EV_SYSFLAGS = 0xf000
EV_UDATA_SPECIFIC = 0x100
EV_VANISHED = 0x200
EXTA = 0x4b00
EXTB = 0x9600
EXTPROC = 0x800
FD_CLOEXEC = 0x1
FD_SETSIZE = 0x400
FF0 = 0x0
FF1 = 0x4000
FFDLY = 0x4000
FLUSHO = 0x800000
FSOPT_ATTR_CMN_EXTENDED = 0x20
FSOPT_NOFOLLOW = 0x1
FSOPT_NOINMEMUPDATE = 0x2
FSOPT_PACK_INVAL_ATTRS = 0x8
FSOPT_REPORT_FULLSIZE = 0x4
F_ADDFILESIGS = 0x3d
F_ADDFILESIGS_FOR_DYLD_SIM = 0x53
F_ADDFILESIGS_RETURN = 0x61
F_ADDSIGS = 0x3b
F_ALLOCATEALL = 0x4
F_ALLOCATECONTIG = 0x2
F_BARRIERFSYNC = 0x55
F_CHECK_LV = 0x62
F_CHKCLEAN = 0x29
F_DUPFD = 0x0
F_DUPFD_CLOEXEC = 0x43
F_FINDSIGS = 0x4e
F_FLUSH_DATA = 0x28
F_FREEZE_FS = 0x35
F_FULLFSYNC = 0x33
F_GETCODEDIR = 0x48
F_GETFD = 0x1
F_GETFL = 0x3
F_GETLK = 0x7
F_GETLKPID = 0x42
F_GETNOSIGPIPE = 0x4a
F_GETOWN = 0x5
F_GETPATH = 0x32
F_GETPATH_MTMINFO = 0x47
F_GETPROTECTIONCLASS = 0x3f
F_GETPROTECTIONLEVEL = 0x4d
F_GLOBAL_NOCACHE = 0x37
F_LOG2PHYS = 0x31
F_LOG2PHYS_EXT = 0x41
F_NOCACHE = 0x30
F_NODIRECT = 0x3e
F_OK = 0x0
F_PATHPKG_CHECK = 0x34
F_PEOFPOSMODE = 0x3
F_PREALLOCATE = 0x2a
F_PUNCHHOLE = 0x63
F_RDADVISE = 0x2c
F_RDAHEAD = 0x2d
F_RDLCK = 0x1
F_SETBACKINGSTORE = 0x46
F_SETFD = 0x2
F_SETFL = 0x4
F_SETLK = 0x8
F_SETLKW = 0x9
F_SETLKWTIMEOUT = 0xa
F_SETNOSIGPIPE = 0x49
F_SETOWN = 0x6
F_SETPROTECTIONCLASS = 0x40
F_SETSIZE = 0x2b
F_SINGLE_WRITER = 0x4c
F_THAW_FS = 0x36
F_TRANSCODEKEY = 0x4b
F_TRIM_ACTIVE_FILE = 0x64
F_UNLCK = 0x2
F_VOLPOSMODE = 0x4
F_WRLCK = 0x3
HUPCL = 0x4000
HW_MACHINE = 0x1
ICANON = 0x100
ICMP6_FILTER = 0x12
ICRNL = 0x100
IEXTEN = 0x400
IFF_ALLMULTI = 0x200
IFF_ALTPHYS = 0x4000
IFF_BROADCAST = 0x2
IFF_DEBUG = 0x4
IFF_LINK0 = 0x1000
IFF_LINK1 = 0x2000
IFF_LINK2 = 0x4000
IFF_LOOPBACK = 0x8
IFF_MULTICAST = 0x8000
IFF_NOARP = 0x80
IFF_NOTRAILERS = 0x20
IFF_OACTIVE = 0x400
IFF_POINTOPOINT = 0x10
IFF_PROMISC = 0x100
IFF_RUNNING = 0x40
IFF_SIMPLEX = 0x800
IFF_UP = 0x1
IFNAMSIZ = 0x10
IFT_1822 = 0x2
IFT_AAL5 = 0x31
IFT_ARCNET = 0x23
IFT_ARCNETPLUS = 0x24
IFT_ATM = 0x25
IFT_BRIDGE = 0xd1
IFT_CARP = 0xf8
IFT_CELLULAR = 0xff
IFT_CEPT = 0x13
IFT_DS3 = 0x1e
IFT_ENC = 0xf4
IFT_EON = 0x19
IFT_ETHER = 0x6
IFT_FAITH = 0x38
IFT_FDDI = 0xf
IFT_FRELAY = 0x20
IFT_FRELAYDCE = 0x2c
IFT_GIF = 0x37
IFT_HDH1822 = 0x3
IFT_HIPPI = 0x2f
IFT_HSSI = 0x2e
IFT_HY = 0xe
IFT_IEEE1394 = 0x90
IFT_IEEE8023ADLAG = 0x88
IFT_ISDNBASIC = 0x14
IFT_ISDNPRIMARY = 0x15
IFT_ISO88022LLC = 0x29
IFT_ISO88023 = 0x7
IFT_ISO88024 = 0x8
IFT_ISO88025 = 0x9
IFT_ISO88026 = 0xa
IFT_L2VLAN = 0x87
IFT_LAPB = 0x10
IFT_LOCALTALK = 0x2a
IFT_LOOP = 0x18
IFT_MIOX25 = 0x26
IFT_MODEM = 0x30
IFT_NSIP = 0x1b
IFT_OTHER = 0x1
IFT_P10 = 0xc
IFT_P80 = 0xd
IFT_PARA = 0x22
IFT_PDP = 0xff
IFT_PFLOG = 0xf5
IFT_PFSYNC = 0xf6
IFT_PKTAP = 0xfe
IFT_PPP = 0x17
IFT_PROPMUX = 0x36
IFT_PROPVIRTUAL = 0x35
IFT_PTPSERIAL = 0x16
IFT_RS232 = 0x21
IFT_SDLC = 0x11
IFT_SIP = 0x1f
IFT_SLIP = 0x1c
IFT_SMDSDXI = 0x2b
IFT_SMDSICIP = 0x34
IFT_SONET = 0x27
IFT_SONETPATH = 0x32
IFT_SONETVT = 0x33
IFT_STARLAN = 0xb
IFT_STF = 0x39
IFT_T1 = 0x12
IFT_ULTRA = 0x1d
IFT_V35 = 0x2d
IFT_X25 = 0x5
IFT_X25DDN = 0x4
IFT_X25PLE = 0x28
IFT_XETHER = 0x1a
IGNBRK = 0x1
IGNCR = 0x80
IGNPAR = 0x4
IMAXBEL = 0x2000
INLCR = 0x40
INPCK = 0x10
IN_CLASSA_HOST = 0xffffff
IN_CLASSA_MAX = 0x80
IN_CLASSA_NET = 0xff000000
IN_CLASSA_NSHIFT = 0x18
IN_CLASSB_HOST = 0xffff
IN_CLASSB_MAX = 0x10000
IN_CLASSB_NET = 0xffff0000
IN_CLASSB_NSHIFT = 0x10
IN_CLASSC_HOST = 0xff
IN_CLASSC_NET = 0xffffff00
IN_CLASSC_NSHIFT = 0x8
IN_CLASSD_HOST = 0xfffffff
IN_CLASSD_NET = 0xf0000000
IN_CLASSD_NSHIFT = 0x1c
IN_LINKLOCALNETNUM = 0xa9fe0000
IN_LOOPBACKNET = 0x7f
IPPROTO_3PC = 0x22
IPPROTO_ADFS = 0x44
IPPROTO_AH = 0x33
IPPROTO_AHIP = 0x3d
IPPROTO_APES = 0x63
IPPROTO_ARGUS = 0xd
IPPROTO_AX25 = 0x5d
IPPROTO_BHA = 0x31
IPPROTO_BLT = 0x1e
IPPROTO_BRSATMON = 0x4c
IPPROTO_CFTP = 0x3e
IPPROTO_CHAOS = 0x10
IPPROTO_CMTP = 0x26
IPPROTO_CPHB = 0x49
IPPROTO_CPNX = 0x48
IPPROTO_DDP = 0x25
IPPROTO_DGP = 0x56
IPPROTO_DIVERT = 0xfe
IPPROTO_DONE = 0x101
IPPROTO_DSTOPTS = 0x3c
IPPROTO_EGP = 0x8
IPPROTO_EMCON = 0xe
IPPROTO_ENCAP = 0x62
IPPROTO_EON = 0x50
IPPROTO_ESP = 0x32
IPPROTO_ETHERIP = 0x61
IPPROTO_FRAGMENT = 0x2c
IPPROTO_GGP = 0x3
IPPROTO_GMTP = 0x64
IPPROTO_GRE = 0x2f
IPPROTO_HELLO = 0x3f
IPPROTO_HMP = 0x14
IPPROTO_HOPOPTS = 0x0
IPPROTO_ICMP = 0x1
IPPROTO_ICMPV6 = 0x3a
IPPROTO_IDP = 0x16
IPPROTO_IDPR = 0x23
IPPROTO_IDRP = 0x2d
IPPROTO_IGMP = 0x2
IPPROTO_IGP = 0x55
IPPROTO_IGRP = 0x58
IPPROTO_IL = 0x28
IPPROTO_INLSP = 0x34
IPPROTO_INP = 0x20
IPPROTO_IP = 0x0
IPPROTO_IPCOMP = 0x6c
IPPROTO_IPCV = 0x47
IPPROTO_IPEIP = 0x5e
IPPROTO_IPIP = 0x4
IPPROTO_IPPC = 0x43
IPPROTO_IPV4 = 0x4
IPPROTO_IPV6 = 0x29
IPPROTO_IRTP = 0x1c
IPPROTO_KRYPTOLAN = 0x41
IPPROTO_LARP = 0x5b
IPPROTO_LEAF1 = 0x19
IPPROTO_LEAF2 = 0x1a
IPPROTO_MAX = 0x100
IPPROTO_MAXID = 0x34
IPPROTO_MEAS = 0x13
IPPROTO_MHRP = 0x30
IPPROTO_MICP = 0x5f
IPPROTO_MTP = 0x5c
IPPROTO_MUX = 0x12
IPPROTO_ND = 0x4d
IPPROTO_NHRP = 0x36
IPPROTO_NONE = 0x3b
IPPROTO_NSP = 0x1f
IPPROTO_NVPII = 0xb
IPPROTO_OSPFIGP = 0x59
IPPROTO_PGM = 0x71
IPPROTO_PIGP = 0x9
IPPROTO_PIM = 0x67
IPPROTO_PRM = 0x15
IPPROTO_PUP = 0xc
IPPROTO_PVP = 0x4b
IPPROTO_RAW = 0xff
IPPROTO_RCCMON = 0xa
IPPROTO_RDP = 0x1b
IPPROTO_ROUTING = 0x2b
IPPROTO_RSVP = 0x2e
IPPROTO_RVD = 0x42
IPPROTO_SATEXPAK = 0x40
IPPROTO_SATMON = 0x45
IPPROTO_SCCSP = 0x60
IPPROTO_SCTP = 0x84
IPPROTO_SDRP = 0x2a
IPPROTO_SEP = 0x21
IPPROTO_SRPC = 0x5a
IPPROTO_ST = 0x7
IPPROTO_SVMTP = 0x52
IPPROTO_SWIPE = 0x35
IPPROTO_TCF = 0x57
IPPROTO_TCP = 0x6
IPPROTO_TP = 0x1d
IPPROTO_TPXX = 0x27
IPPROTO_TRUNK1 = 0x17
IPPROTO_TRUNK2 = 0x18
IPPROTO_TTP = 0x54
IPPROTO_UDP = 0x11
IPPROTO_VINES = 0x53
IPPROTO_VISA = 0x46
IPPROTO_VMTP = 0x51
IPPROTO_WBEXPAK = 0x4f
IPPROTO_WBMON = 0x4e
IPPROTO_WSN = 0x4a
IPPROTO_XNET = 0xf
IPPROTO_XTP = 0x24
IPV6_2292DSTOPTS = 0x17
IPV6_2292HOPLIMIT = 0x14
IPV6_2292HOPOPTS = 0x16
IPV6_2292NEXTHOP = 0x15
IPV6_2292PKTINFO = 0x13
IPV6_2292PKTOPTIONS = 0x19
IPV6_2292RTHDR = 0x18
IPV6_BINDV6ONLY = 0x1b
IPV6_BOUND_IF = 0x7d
IPV6_CHECKSUM = 0x1a
IPV6_DEFAULT_MULTICAST_HOPS = 0x1
IPV6_DEFAULT_MULTICAST_LOOP = 0x1
IPV6_DEFHLIM = 0x40
IPV6_FAITH = 0x1d
IPV6_FLOWINFO_MASK = 0xffffff0f
IPV6_FLOWLABEL_MASK = 0xffff0f00
IPV6_FLOW_ECN_MASK = 0x300
IPV6_FRAGTTL = 0x3c
IPV6_FW_ADD = 0x1e
IPV6_FW_DEL = 0x1f
IPV6_FW_FLUSH = 0x20
IPV6_FW_GET = 0x22
IPV6_FW_ZERO = 0x21
IPV6_HLIMDEC = 0x1
IPV6_IPSEC_POLICY = 0x1c
IPV6_JOIN_GROUP = 0xc
IPV6_LEAVE_GROUP = 0xd
IPV6_MAXHLIM = 0xff
IPV6_MAXOPTHDR = 0x800
IPV6_MAXPACKET = 0xffff
IPV6_MAX_GROUP_SRC_FILTER = 0x200
IPV6_MAX_MEMBERSHIPS = 0xfff
IPV6_MAX_SOCK_SRC_FILTER = 0x80
IPV6_MIN_MEMBERSHIPS = 0x1f
IPV6_MMTU = 0x500
IPV6_MULTICAST_HOPS = 0xa
IPV6_MULTICAST_IF = 0x9
IPV6_MULTICAST_LOOP = 0xb
IPV6_PORTRANGE = 0xe
IPV6_PORTRANGE_DEFAULT = 0x0
IPV6_PORTRANGE_HIGH = 0x1
IPV6_PORTRANGE_LOW = 0x2
IPV6_RECVTCLASS = 0x23
IPV6_RTHDR_LOOSE = 0x0
IPV6_RTHDR_STRICT = 0x1
IPV6_RTHDR_TYPE_0 = 0x0
IPV6_SOCKOPT_RESERVED1 = 0x3
IPV6_TCLASS = 0x24
IPV6_UNICAST_HOPS = 0x4
IPV6_V6ONLY = 0x1b
IPV6_VERSION = 0x60
IPV6_VERSION_MASK = 0xf0
IP_ADD_MEMBERSHIP = 0xc
IP_ADD_SOURCE_MEMBERSHIP = 0x46
IP_BLOCK_SOURCE = 0x48
IP_BOUND_IF = 0x19
IP_DEFAULT_MULTICAST_LOOP = 0x1
IP_DEFAULT_MULTICAST_TTL = 0x1
IP_DF = 0x4000
IP_DROP_MEMBERSHIP = 0xd
IP_DROP_SOURCE_MEMBERSHIP = 0x47
IP_DUMMYNET_CONFIGURE = 0x3c
IP_DUMMYNET_DEL = 0x3d
IP_DUMMYNET_FLUSH = 0x3e
IP_DUMMYNET_GET = 0x40
IP_FAITH = 0x16
IP_FW_ADD = 0x28
IP_FW_DEL = 0x29
IP_FW_FLUSH = 0x2a
IP_FW_GET = 0x2c
IP_FW_RESETLOG = 0x2d
IP_FW_ZERO = 0x2b
IP_HDRINCL = 0x2
IP_IPSEC_POLICY = 0x15
IP_MAXPACKET = 0xffff
IP_MAX_GROUP_SRC_FILTER = 0x200
IP_MAX_MEMBERSHIPS = 0xfff
IP_MAX_SOCK_MUTE_FILTER = 0x80
IP_MAX_SOCK_SRC_FILTER = 0x80
IP_MF = 0x2000
IP_MIN_MEMBERSHIPS = 0x1f
IP_MSFILTER = 0x4a
IP_MSS = 0x240
IP_MULTICAST_IF = 0x9
IP_MULTICAST_IFINDEX = 0x42
IP_MULTICAST_LOOP = 0xb
IP_MULTICAST_TTL = 0xa
IP_MULTICAST_VIF = 0xe
IP_NAT__XXX = 0x37
IP_OFFMASK = 0x1fff
IP_OLD_FW_ADD = 0x32
IP_OLD_FW_DEL = 0x33
IP_OLD_FW_FLUSH = 0x34
IP_OLD_FW_GET = 0x36
IP_OLD_FW_RESETLOG = 0x38
IP_OLD_FW_ZERO = 0x35
IP_OPTIONS = 0x1
IP_PKTINFO = 0x1a
IP_PORTRANGE = 0x13
IP_PORTRANGE_DEFAULT = 0x0
IP_PORTRANGE_HIGH = 0x1
IP_PORTRANGE_LOW = 0x2
IP_RECVDSTADDR = 0x7
IP_RECVIF = 0x14
IP_RECVOPTS = 0x5
IP_RECVPKTINFO = 0x1a
IP_RECVRETOPTS = 0x6
IP_RECVTOS = 0x1b
IP_RECVTTL = 0x18
IP_RETOPTS = 0x8
IP_RF = 0x8000
IP_RSVP_OFF = 0x10
IP_RSVP_ON = 0xf
IP_RSVP_VIF_OFF = 0x12
IP_RSVP_VIF_ON = 0x11
IP_STRIPHDR = 0x17
IP_TOS = 0x3
IP_TRAFFIC_MGT_BACKGROUND = 0x41
IP_TTL = 0x4
IP_UNBLOCK_SOURCE = 0x49
ISIG = 0x80
ISTRIP = 0x20
IUTF8 = 0x4000
IXANY = 0x800
IXOFF = 0x400
IXON = 0x200
KERN_HOSTNAME = 0xa
KERN_OSRELEASE = 0x2
KERN_OSTYPE = 0x1
KERN_VERSION = 0x4
LOCK_EX = 0x2
LOCK_NB = 0x4
LOCK_SH = 0x1
LOCK_UN = 0x8
MADV_CAN_REUSE = 0x9
MADV_DONTNEED = 0x4
MADV_FREE = 0x5
MADV_FREE_REUSABLE = 0x7
MADV_FREE_REUSE = 0x8
MADV_NORMAL = 0x0
MADV_PAGEOUT = 0xa
MADV_RANDOM = 0x1
MADV_SEQUENTIAL = 0x2
MADV_WILLNEED = 0x3
MADV_ZERO_WIRED_PAGES = 0x6
MAP_ANON = 0x1000
MAP_ANONYMOUS = 0x1000
MAP_COPY = 0x2
MAP_FILE = 0x0
MAP_FIXED = 0x10
MAP_HASSEMAPHORE = 0x200
MAP_JIT = 0x800
MAP_NOCACHE = 0x400
MAP_NOEXTEND = 0x100
MAP_NORESERVE = 0x40
MAP_PRIVATE = 0x2
MAP_RENAME = 0x20
MAP_RESERVED0080 = 0x80
MAP_RESILIENT_CODESIGN = 0x2000
MAP_RESILIENT_MEDIA = 0x4000
MAP_SHARED = 0x1
MCL_CURRENT = 0x1
MCL_FUTURE = 0x2
MNT_ASYNC = 0x40
MNT_AUTOMOUNTED = 0x400000
MNT_CMDFLAGS = 0xf0000
MNT_CPROTECT = 0x80
MNT_DEFWRITE = 0x2000000
MNT_DONTBROWSE = 0x100000
MNT_DOVOLFS = 0x8000
MNT_DWAIT = 0x4
MNT_EXPORTED = 0x100
MNT_FORCE = 0x80000
MNT_IGNORE_OWNERSHIP = 0x200000
MNT_JOURNALED = 0x800000
MNT_LOCAL = 0x1000
MNT_MULTILABEL = 0x4000000
MNT_NOATIME = 0x10000000
MNT_NOBLOCK = 0x20000
MNT_NODEV = 0x10
MNT_NOEXEC = 0x4
MNT_NOSUID = 0x8
MNT_NOUSERXATTR = 0x1000000
MNT_NOWAIT = 0x2
MNT_QUARANTINE = 0x400
MNT_QUOTA = 0x2000
MNT_RDONLY = 0x1
MNT_RELOAD = 0x40000
MNT_ROOTFS = 0x4000
MNT_SYNCHRONOUS = 0x2
MNT_UNION = 0x20
MNT_UNKNOWNPERMISSIONS = 0x200000
MNT_UPDATE = 0x10000
MNT_VISFLAGMASK = 0x17f0f5ff
MNT_WAIT = 0x1
MSG_CTRUNC = 0x20
MSG_DONTROUTE = 0x4
MSG_DONTWAIT = 0x80
MSG_EOF = 0x100
MSG_EOR = 0x8
MSG_FLUSH = 0x400
MSG_HAVEMORE = 0x2000
MSG_HOLD = 0x800
MSG_NEEDSA = 0x10000
MSG_OOB = 0x1
MSG_PEEK = 0x2
MSG_RCVMORE = 0x4000
MSG_SEND = 0x1000
MSG_TRUNC = 0x10
MSG_WAITALL = 0x40
MSG_WAITSTREAM = 0x200
MS_ASYNC = 0x1
MS_DEACTIVATE = 0x8
MS_INVALIDATE = 0x2
MS_KILLPAGES = 0x4
MS_SYNC = 0x10
NAME_MAX = 0xff
NET_RT_DUMP = 0x1
NET_RT_DUMP2 = 0x7
NET_RT_FLAGS = 0x2
NET_RT_IFLIST = 0x3
NET_RT_IFLIST2 = 0x6
NET_RT_MAXID = 0xa
NET_RT_STAT = 0x4
NET_RT_TRASH = 0x5
NFDBITS = 0x20
NL0 = 0x0
NL1 = 0x100
NL2 = 0x200
NL3 = 0x300
NLDLY = 0x300
NOFLSH = 0x80000000
NOKERNINFO = 0x2000000
NOTE_ABSOLUTE = 0x8
NOTE_ATTRIB = 0x8
NOTE_BACKGROUND = 0x40
NOTE_CHILD = 0x4
NOTE_CRITICAL = 0x20
NOTE_DELETE = 0x1
NOTE_EXEC = 0x20000000
NOTE_EXIT = 0x80000000
NOTE_EXITSTATUS = 0x4000000
NOTE_EXIT_CSERROR = 0x40000
NOTE_EXIT_DECRYPTFAIL = 0x10000
NOTE_EXIT_DETAIL = 0x2000000
NOTE_EXIT_DETAIL_MASK = 0x70000
NOTE_EXIT_MEMORY = 0x20000
NOTE_EXIT_REPARENTED = 0x80000
NOTE_EXTEND = 0x4
NOTE_FFAND = 0x40000000
NOTE_FFCOPY = 0xc0000000
NOTE_FFCTRLMASK = 0xc0000000
NOTE_FFLAGSMASK = 0xffffff
NOTE_FFNOP = 0x0
NOTE_FFOR = 0x80000000
NOTE_FORK = 0x40000000
NOTE_FUNLOCK = 0x100
NOTE_LEEWAY = 0x10
NOTE_LINK = 0x10
NOTE_LOWAT = 0x1
NOTE_MACH_CONTINUOUS_TIME = 0x80
NOTE_NONE = 0x80
NOTE_NSECONDS = 0x4
NOTE_OOB = 0x2
NOTE_PCTRLMASK = -0x100000
NOTE_PDATAMASK = 0xfffff
NOTE_REAP = 0x10000000
NOTE_RENAME = 0x20
NOTE_REVOKE = 0x40
NOTE_SECONDS = 0x1
NOTE_SIGNAL = 0x8000000
NOTE_TRACK = 0x1
NOTE_TRACKERR = 0x2
NOTE_TRIGGER = 0x1000000
NOTE_USECONDS = 0x2
NOTE_VM_ERROR = 0x10000000
NOTE_VM_PRESSURE = 0x80000000
NOTE_VM_PRESSURE_SUDDEN_TERMINATE = 0x20000000
NOTE_VM_PRESSURE_TERMINATE = 0x40000000
NOTE_WRITE = 0x2
OCRNL = 0x10
OFDEL = 0x20000
OFILL = 0x80
ONLCR = 0x2
ONLRET = 0x40
ONOCR = 0x20
ONOEOT = 0x8
OPOST = 0x1
OXTABS = 0x4
O_ACCMODE = 0x3
O_ALERT = 0x20000000
O_APPEND = 0x8
O_ASYNC = 0x40
O_CLOEXEC = 0x1000000
O_CREAT = 0x200
O_DIRECTORY = 0x100000
O_DP_GETRAWENCRYPTED = 0x1
O_DP_GETRAWUNENCRYPTED = 0x2
O_DSYNC = 0x400000
O_EVTONLY = 0x8000
O_EXCL = 0x800
O_EXLOCK = 0x20
O_FSYNC = 0x80
O_NDELAY = 0x4
O_NOCTTY = 0x20000
O_NOFOLLOW = 0x100
O_NONBLOCK = 0x4
O_POPUP = 0x80000000
O_RDONLY = 0x0
O_RDWR = 0x2
O_SHLOCK = 0x10
O_SYMLINK = 0x200000
O_SYNC = 0x80
O_TRUNC = 0x400
O_WRONLY = 0x1
PARENB = 0x1000
PARMRK = 0x8
PARODD = 0x2000
PENDIN = 0x20000000
PRIO_PGRP = 0x1
PRIO_PROCESS = 0x0
PRIO_USER = 0x2
PROT_EXEC = 0x4
PROT_NONE = 0x0
PROT_READ = 0x1
PROT_WRITE = 0x2
PT_ATTACH = 0xa
PT_ATTACHEXC = 0xe
PT_CONTINUE = 0x7
PT_DENY_ATTACH = 0x1f
PT_DETACH = 0xb
PT_FIRSTMACH = 0x20
PT_FORCEQUOTA = 0x1e
PT_KILL = 0x8
PT_READ_D = 0x2
PT_READ_I = 0x1
PT_READ_U = 0x3
PT_SIGEXC = 0xc
PT_STEP = 0x9
PT_THUPDATE = 0xd
PT_TRACE_ME = 0x0
PT_WRITE_D = 0x5
PT_WRITE_I = 0x4
PT_WRITE_U = 0x6
RLIMIT_AS = 0x5
RLIMIT_CORE = 0x4
RLIMIT_CPU = 0x0
RLIMIT_CPU_USAGE_MONITOR = 0x2
RLIMIT_DATA = 0x2
RLIMIT_FSIZE = 0x1
RLIMIT_MEMLOCK = 0x6
RLIMIT_NOFILE = 0x8
RLIMIT_NPROC = 0x7
RLIMIT_RSS = 0x5
RLIMIT_STACK = 0x3
RLIM_INFINITY = 0x7fffffffffffffff
RTAX_AUTHOR = 0x6
RTAX_BRD = 0x7
RTAX_DST = 0x0
RTAX_GATEWAY = 0x1
RTAX_GENMASK = 0x3
RTAX_IFA = 0x5
RTAX_IFP = 0x4
RTAX_MAX = 0x8
RTAX_NETMASK = 0x2
RTA_AUTHOR = 0x40
RTA_BRD = 0x80
RTA_DST = 0x1
RTA_GATEWAY = 0x2
RTA_GENMASK = 0x8
RTA_IFA = 0x20
RTA_IFP = 0x10
RTA_NETMASK = 0x4
RTF_BLACKHOLE = 0x1000
RTF_BROADCAST = 0x400000
RTF_CLONING = 0x100
RTF_CONDEMNED = 0x2000000
RTF_DELCLONE = 0x80
RTF_DONE = 0x40
RTF_DYNAMIC = 0x10
RTF_GATEWAY = 0x2
RTF_HOST = 0x4
RTF_IFREF = 0x4000000
RTF_IFSCOPE = 0x1000000
RTF_LLINFO = 0x400
RTF_LOCAL = 0x200000
RTF_MODIFIED = 0x20
RTF_MULTICAST = 0x800000
RTF_NOIFREF = 0x2000
RTF_PINNED = 0x100000
RTF_PRCLONING = 0x10000
RTF_PROTO1 = 0x8000
RTF_PROTO2 = 0x4000
RTF_PROTO3 = 0x40000
RTF_PROXY = 0x8000000
RTF_REJECT = 0x8
RTF_ROUTER = 0x10000000
RTF_STATIC = 0x800
RTF_UP = 0x1
RTF_WASCLONED = 0x20000
RTF_XRESOLVE = 0x200
RTM_ADD = 0x1
RTM_CHANGE = 0x3
RTM_DELADDR = 0xd
RTM_DELETE = 0x2
RTM_DELMADDR = 0x10
RTM_GET = 0x4
RTM_GET2 = 0x14
RTM_IFINFO = 0xe
RTM_IFINFO2 = 0x12
RTM_LOCK = 0x8
RTM_LOSING = 0x5
RTM_MISS = 0x7
RTM_NEWADDR = 0xc
RTM_NEWMADDR = 0xf
RTM_NEWMADDR2 = 0x13
RTM_OLDADD = 0x9
RTM_OLDDEL = 0xa
RTM_REDIRECT = 0x6
RTM_RESOLVE = 0xb
RTM_RTTUNIT = 0xf4240
RTM_VERSION = 0x5
RTV_EXPIRE = 0x4
RTV_HOPCOUNT = 0x2
RTV_MTU = 0x1
RTV_RPIPE = 0x8
RTV_RTT = 0x40
RTV_RTTVAR = 0x80
RTV_SPIPE = 0x10
RTV_SSTHRESH = 0x20
RUSAGE_CHILDREN = -0x1
RUSAGE_SELF = 0x0
SCM_CREDS = 0x3
SCM_RIGHTS = 0x1
SCM_TIMESTAMP = 0x2
SCM_TIMESTAMP_MONOTONIC = 0x4
SHUT_RD = 0x0
SHUT_RDWR = 0x2
SHUT_WR = 0x1
SIOCADDMULTI = 0x80206931
SIOCAIFADDR = 0x8040691a
SIOCARPIPLL = 0xc0206928
SIOCATMARK = 0x40047307
SIOCAUTOADDR = 0xc0206926
SIOCAUTONETMASK = 0x80206927
SIOCDELMULTI = 0x80206932
SIOCDIFADDR = 0x80206919
SIOCDIFPHYADDR = 0x80206941
SIOCGDRVSPEC = 0xc01c697b
SIOCGETVLAN = 0xc020697f
SIOCGHIWAT = 0x40047301
SIOCGIFADDR = 0xc0206921
SIOCGIFALTMTU = 0xc0206948
SIOCGIFASYNCMAP = 0xc020697c
SIOCGIFBOND = 0xc0206947
SIOCGIFBRDADDR = 0xc0206923
SIOCGIFCAP = 0xc020695b
SIOCGIFCONF = 0xc0086924
SIOCGIFDEVMTU = 0xc0206944
SIOCGIFDSTADDR = 0xc0206922
SIOCGIFFLAGS = 0xc0206911
SIOCGIFGENERIC = 0xc020693a
SIOCGIFKPI = 0xc0206987
SIOCGIFMAC = 0xc0206982
SIOCGIFMEDIA = 0xc0286938
SIOCGIFMETRIC = 0xc0206917
SIOCGIFMTU = 0xc0206933
SIOCGIFNETMASK = 0xc0206925
SIOCGIFPDSTADDR = 0xc0206940
SIOCGIFPHYS = 0xc0206935
SIOCGIFPSRCADDR = 0xc020693f
SIOCGIFSTATUS = 0xc331693d
SIOCGIFVLAN = 0xc020697f
SIOCGIFWAKEFLAGS = 0xc0206988
SIOCGLOWAT = 0x40047303
SIOCGPGRP = 0x40047309
SIOCIFCREATE = 0xc0206978
SIOCIFCREATE2 = 0xc020697a
SIOCIFDESTROY = 0x80206979
SIOCIFGCLONERS = 0xc00c6981
SIOCRSLVMULTI = 0xc008693b
SIOCSDRVSPEC = 0x801c697b
SIOCSETVLAN = 0x8020697e
SIOCSHIWAT = 0x80047300
SIOCSIFADDR = 0x8020690c
SIOCSIFALTMTU = 0x80206945
SIOCSIFASYNCMAP = 0x8020697d
SIOCSIFBOND = 0x80206946
SIOCSIFBRDADDR = 0x80206913
SIOCSIFCAP = 0x8020695a
SIOCSIFDSTADDR = 0x8020690e
SIOCSIFFLAGS = 0x80206910
SIOCSIFGENERIC = 0x80206939
SIOCSIFKPI = 0x80206986
SIOCSIFLLADDR = 0x8020693c
SIOCSIFMAC = 0x80206983
SIOCSIFMEDIA = 0xc0206937
SIOCSIFMETRIC = 0x80206918
SIOCSIFMTU = 0x80206934
SIOCSIFNETMASK = 0x80206916
SIOCSIFPHYADDR = 0x8040693e
SIOCSIFPHYS = 0x80206936
SIOCSIFVLAN = 0x8020697e
SIOCSLOWAT = 0x80047302
SIOCSPGRP = 0x80047308
SOCK_DGRAM = 0x2
SOCK_MAXADDRLEN = 0xff
SOCK_RAW = 0x3
SOCK_RDM = 0x4
SOCK_SEQPACKET = 0x5
SOCK_STREAM = 0x1
SOL_SOCKET = 0xffff
SOMAXCONN = 0x80
SO_ACCEPTCONN = 0x2
SO_BROADCAST = 0x20
SO_DEBUG = 0x1
SO_DONTROUTE = 0x10
SO_DONTTRUNC = 0x2000
SO_ERROR = 0x1007
SO_KEEPALIVE = 0x8
SO_LABEL = 0x1010
SO_LINGER = 0x80
SO_LINGER_SEC = 0x1080
SO_NETSVC_MARKING_LEVEL = 0x1119
SO_NET_SERVICE_TYPE = 0x1116
SO_NKE = 0x1021
SO_NOADDRERR = 0x1023
SO_NOSIGPIPE = 0x1022
SO_NOTIFYCONFLICT = 0x1026
SO_NP_EXTENSIONS = 0x1083
SO_NREAD = 0x1020
SO_NUMRCVPKT = 0x1112
SO_NWRITE = 0x1024
SO_OOBINLINE = 0x100
SO_PEERLABEL = 0x1011
SO_RANDOMPORT = 0x1082
SO_RCVBUF = 0x1002
SO_RCVLOWAT = 0x1004
SO_RCVTIMEO = 0x1006
SO_REUSEADDR = 0x4
SO_REUSEPORT = 0x200
SO_REUSESHAREUID = 0x1025
SO_SNDBUF = 0x1001
SO_SNDLOWAT = 0x1003
SO_SNDTIMEO = 0x1005
SO_TIMESTAMP = 0x400
SO_TIMESTAMP_MONOTONIC = 0x800
SO_TYPE = 0x1008
SO_UPCALLCLOSEWAIT = 0x1027
SO_USELOOPBACK = 0x40
SO_WANTMORE = 0x4000
SO_WANTOOBFLAG = 0x8000
S_IEXEC = 0x40
S_IFBLK = 0x6000
S_IFCHR = 0x2000
S_IFDIR = 0x4000
S_IFIFO = 0x1000
S_IFLNK = 0xa000
S_IFMT = 0xf000
S_IFREG = 0x8000
S_IFSOCK = 0xc000
S_IFWHT = 0xe000
S_IREAD = 0x100
S_IRGRP = 0x20
S_IROTH = 0x4
S_IRUSR = 0x100
S_IRWXG = 0x38
S_IRWXO = 0x7
S_IRWXU = 0x1c0
S_ISGID = 0x400
S_ISTXT = 0x200
S_ISUID = 0x800
S_ISVTX = 0x200
S_IWGRP = 0x10
S_IWOTH = 0x2
S_IWRITE = 0x80
S_IWUSR = 0x80
S_IXGRP = 0x8
S_IXOTH = 0x1
S_IXUSR = 0x40
TAB0 = 0x0
TAB1 = 0x400
TAB2 = 0x800
TAB3 = 0x4
TABDLY = 0xc04
TCIFLUSH = 0x1
TCIOFF = 0x3
TCIOFLUSH = 0x3
TCION = 0x4
TCOFLUSH = 0x2
TCOOFF = 0x1
TCOON = 0x2
TCP_CONNECTIONTIMEOUT = 0x20
TCP_CONNECTION_INFO = 0x106
TCP_ENABLE_ECN = 0x104
TCP_FASTOPEN = 0x105
TCP_KEEPALIVE = 0x10
TCP_KEEPCNT = 0x102
TCP_KEEPINTVL = 0x101
TCP_MAXHLEN = 0x3c
TCP_MAXOLEN = 0x28
TCP_MAXSEG = 0x2
TCP_MAXWIN = 0xffff
TCP_MAX_SACK = 0x4
TCP_MAX_WINSHIFT = 0xe
TCP_MINMSS = 0xd8
TCP_MSS = 0x200
TCP_NODELAY = 0x1
TCP_NOOPT = 0x8
TCP_NOPUSH = 0x4
TCP_NOTSENT_LOWAT = 0x201
TCP_RXT_CONNDROPTIME = 0x80
TCP_RXT_FINDROP = 0x100
TCP_SENDMOREACKS = 0x103
TCSAFLUSH = 0x2
TIOCCBRK = 0x2000747a
TIOCCDTR = 0x20007478
TIOCCONS = 0x80047462
TIOCDCDTIMESTAMP = 0x40087458
TIOCDRAIN = 0x2000745e
TIOCDSIMICROCODE = 0x20007455
TIOCEXCL = 0x2000740d
TIOCEXT = 0x80047460
TIOCFLUSH = 0x80047410
TIOCGDRAINWAIT = 0x40047456
TIOCGETA = 0x402c7413
TIOCGETD = 0x4004741a
TIOCGPGRP = 0x40047477
TIOCGWINSZ = 0x40087468
TIOCIXOFF = 0x20007480
TIOCIXON = 0x20007481
TIOCMBIC = 0x8004746b
TIOCMBIS = 0x8004746c
TIOCMGDTRWAIT = 0x4004745a
TIOCMGET = 0x4004746a
TIOCMODG = 0x40047403
TIOCMODS = 0x80047404
TIOCMSDTRWAIT = 0x8004745b
TIOCMSET = 0x8004746d
TIOCM_CAR = 0x40
TIOCM_CD = 0x40
TIOCM_CTS = 0x20
TIOCM_DSR = 0x100
TIOCM_DTR = 0x2
TIOCM_LE = 0x1
TIOCM_RI = 0x80
TIOCM_RNG = 0x80
TIOCM_RTS = 0x4
TIOCM_SR = 0x10
TIOCM_ST = 0x8
TIOCNOTTY = 0x20007471
TIOCNXCL = 0x2000740e
TIOCOUTQ = 0x40047473
TIOCPKT = 0x80047470
TIOCPKT_DATA = 0x0
TIOCPKT_DOSTOP = 0x20
TIOCPKT_FLUSHREAD = 0x1
TIOCPKT_FLUSHWRITE = 0x2
TIOCPKT_IOCTL = 0x40
TIOCPKT_NOSTOP = 0x10
TIOCPKT_START = 0x8
TIOCPKT_STOP = 0x4
TIOCPTYGNAME = 0x40807453
TIOCPTYGRANT = 0x20007454
TIOCPTYUNLK = 0x20007452
TIOCREMOTE = 0x80047469
TIOCSBRK = 0x2000747b
TIOCSCONS = 0x20007463
TIOCSCTTY = 0x20007461
TIOCSDRAINWAIT = 0x80047457
TIOCSDTR = 0x20007479
TIOCSETA = 0x802c7414
TIOCSETAF = 0x802c7416
TIOCSETAW = 0x802c7415
TIOCSETD = 0x8004741b
TIOCSIG = 0x2000745f
TIOCSPGRP = 0x80047476
TIOCSTART = 0x2000746e
TIOCSTAT = 0x20007465
TIOCSTI = 0x80017472
TIOCSTOP = 0x2000746f
TIOCSWINSZ = 0x80087467
TIOCTIMESTAMP = 0x40087459
TIOCUCNTL = 0x80047466
TOSTOP = 0x400000
VDISCARD = 0xf
VDSUSP = 0xb
VEOF = 0x0
VEOL = 0x1
VEOL2 = 0x2
VERASE = 0x3
VINTR = 0x8
VKILL = 0x5
VLNEXT = 0xe
VMIN = 0x10
VM_LOADAVG = 0x2
VM_MACHFACTOR = 0x4
VM_MAXID = 0x6
VM_METER = 0x1
VM_SWAPUSAGE = 0x5
VQUIT = 0x9
VREPRINT = 0x6
VSTART = 0xc
VSTATUS = 0x12
VSTOP = 0xd
VSUSP = 0xa
VT0 = 0x0
VT1 = 0x10000
VTDLY = 0x10000
VTIME = 0x11
VWERASE = 0x4
WCONTINUED = 0x10
WCOREFLAG = 0x80
WEXITED = 0x4
WNOHANG = 0x1
WNOWAIT = 0x20
WORDSIZE = 0x20
WSTOPPED = 0x8
WUNTRACED = 0x2
XATTR_CREATE = 0x2
XATTR_NODEFAULT = 0x10
XATTR_NOFOLLOW = 0x1
XATTR_NOSECURITY = 0x8
XATTR_REPLACE = 0x4
XATTR_SHOWCOMPRESSION = 0x20
)
// Errors
const (
E2BIG = syscall.Errno(0x7)
EACCES = syscall.Errno(0xd)
EADDRINUSE = syscall.Errno(0x30)
EADDRNOTAVAIL = syscall.Errno(0x31)
EAFNOSUPPORT = syscall.Errno(0x2f)
EAGAIN = syscall.Errno(0x23)
EALREADY = syscall.Errno(0x25)
EAUTH = syscall.Errno(0x50)
EBADARCH = syscall.Errno(0x56)
EBADEXEC = syscall.Errno(0x55)
EBADF = syscall.Errno(0x9)
EBADMACHO = syscall.Errno(0x58)
EBADMSG = syscall.Errno(0x5e)
EBADRPC = syscall.Errno(0x48)
EBUSY = syscall.Errno(0x10)
ECANCELED = syscall.Errno(0x59)
ECHILD = syscall.Errno(0xa)
ECONNABORTED = syscall.Errno(0x35)
ECONNREFUSED = syscall.Errno(0x3d)
ECONNRESET = syscall.Errno(0x36)
EDEADLK = syscall.Errno(0xb)
EDESTADDRREQ = syscall.Errno(0x27)
EDEVERR = syscall.Errno(0x53)
EDOM = syscall.Errno(0x21)
EDQUOT = syscall.Errno(0x45)
EEXIST = syscall.Errno(0x11)
EFAULT = syscall.Errno(0xe)
EFBIG = syscall.Errno(0x1b)
EFTYPE = syscall.Errno(0x4f)
EHOSTDOWN = syscall.Errno(0x40)
EHOSTUNREACH = syscall.Errno(0x41)
EIDRM = syscall.Errno(0x5a)
EILSEQ = syscall.Errno(0x5c)
EINPROGRESS = syscall.Errno(0x24)
EINTR = syscall.Errno(0x4)
EINVAL = syscall.Errno(0x16)
EIO = syscall.Errno(0x5)
EISCONN = syscall.Errno(0x38)
EISDIR = syscall.Errno(0x15)
ELAST = syscall.Errno(0x6a)
ELOOP = syscall.Errno(0x3e)
EMFILE = syscall.Errno(0x18)
EMLINK = syscall.Errno(0x1f)
EMSGSIZE = syscall.Errno(0x28)
EMULTIHOP = syscall.Errno(0x5f)
ENAMETOOLONG = syscall.Errno(0x3f)
ENEEDAUTH = syscall.Errno(0x51)
ENETDOWN = syscall.Errno(0x32)
ENETRESET = syscall.Errno(0x34)
ENETUNREACH = syscall.Errno(0x33)
ENFILE = syscall.Errno(0x17)
ENOATTR = syscall.Errno(0x5d)
ENOBUFS = syscall.Errno(0x37)
ENODATA = syscall.Errno(0x60)
ENODEV = syscall.Errno(0x13)
ENOENT = syscall.Errno(0x2)
ENOEXEC = syscall.Errno(0x8)
ENOLCK = syscall.Errno(0x4d)
ENOLINK = syscall.Errno(0x61)
ENOMEM = syscall.Errno(0xc)
ENOMSG = syscall.Errno(0x5b)
ENOPOLICY = syscall.Errno(0x67)
ENOPROTOOPT = syscall.Errno(0x2a)
ENOSPC = syscall.Errno(0x1c)
ENOSR = syscall.Errno(0x62)
ENOSTR = syscall.Errno(0x63)
ENOSYS = syscall.Errno(0x4e)
ENOTBLK = syscall.Errno(0xf)
ENOTCONN = syscall.Errno(0x39)
ENOTDIR = syscall.Errno(0x14)
ENOTEMPTY = syscall.Errno(0x42)
ENOTRECOVERABLE = syscall.Errno(0x68)
ENOTSOCK = syscall.Errno(0x26)
ENOTSUP = syscall.Errno(0x2d)
ENOTTY = syscall.Errno(0x19)
ENXIO = syscall.Errno(0x6)
EOPNOTSUPP = syscall.Errno(0x66)
EOVERFLOW = syscall.Errno(0x54)
EOWNERDEAD = syscall.Errno(0x69)
EPERM = syscall.Errno(0x1)
EPFNOSUPPORT = syscall.Errno(0x2e)
EPIPE = syscall.Errno(0x20)
EPROCLIM = syscall.Errno(0x43)
EPROCUNAVAIL = syscall.Errno(0x4c)
EPROGMISMATCH = syscall.Errno(0x4b)
EPROGUNAVAIL = syscall.Errno(0x4a)
EPROTO = syscall.Errno(0x64)
EPROTONOSUPPORT = syscall.Errno(0x2b)
EPROTOTYPE = syscall.Errno(0x29)
EPWROFF = syscall.Errno(0x52)
EQFULL = syscall.Errno(0x6a)
ERANGE = syscall.Errno(0x22)
EREMOTE = syscall.Errno(0x47)
EROFS = syscall.Errno(0x1e)
ERPCMISMATCH = syscall.Errno(0x49)
ESHLIBVERS = syscall.Errno(0x57)
ESHUTDOWN = syscall.Errno(0x3a)
ESOCKTNOSUPPORT = syscall.Errno(0x2c)
ESPIPE = syscall.Errno(0x1d)
ESRCH = syscall.Errno(0x3)
ESTALE = syscall.Errno(0x46)
ETIME = syscall.Errno(0x65)
ETIMEDOUT = syscall.Errno(0x3c)
ETOOMANYREFS = syscall.Errno(0x3b)
ETXTBSY = syscall.Errno(0x1a)
EUSERS = syscall.Errno(0x44)
EWOULDBLOCK = syscall.Errno(0x23)
EXDEV = syscall.Errno(0x12)
)
// Signals
const (
SIGABRT = syscall.Signal(0x6)
SIGALRM = syscall.Signal(0xe)
SIGBUS = syscall.Signal(0xa)
SIGCHLD = syscall.Signal(0x14)
SIGCONT = syscall.Signal(0x13)
SIGEMT = syscall.Signal(0x7)
SIGFPE = syscall.Signal(0x8)
SIGHUP = syscall.Signal(0x1)
SIGILL = syscall.Signal(0x4)
SIGINFO = syscall.Signal(0x1d)
SIGINT = syscall.Signal(0x2)
SIGIO = syscall.Signal(0x17)
SIGIOT = syscall.Signal(0x6)
SIGKILL = syscall.Signal(0x9)
SIGPIPE = syscall.Signal(0xd)
SIGPROF = syscall.Signal(0x1b)
SIGQUIT = syscall.Signal(0x3)
SIGSEGV = syscall.Signal(0xb)
SIGSTOP = syscall.Signal(0x11)
SIGSYS = syscall.Signal(0xc)
SIGTERM = syscall.Signal(0xf)
SIGTRAP = syscall.Signal(0x5)
SIGTSTP = syscall.Signal(0x12)
SIGTTIN = syscall.Signal(0x15)
SIGTTOU = syscall.Signal(0x16)
SIGURG = syscall.Signal(0x10)
SIGUSR1 = syscall.Signal(0x1e)
SIGUSR2 = syscall.Signal(0x1f)
SIGVTALRM = syscall.Signal(0x1a)
SIGWINCH = syscall.Signal(0x1c)
SIGXCPU = syscall.Signal(0x18)
SIGXFSZ = syscall.Signal(0x19)
)
// Error table
var errorList = [...]struct {
num syscall.Errno
name string
desc string
}{
{1, "EPERM", "operation not permitted"},
{2, "ENOENT", "no such file or directory"},
{3, "ESRCH", "no such process"},
{4, "EINTR", "interrupted system call"},
{5, "EIO", "input/output error"},
{6, "ENXIO", "device not configured"},
{7, "E2BIG", "argument list too long"},
{8, "ENOEXEC", "exec format error"},
{9, "EBADF", "bad file descriptor"},
{10, "ECHILD", "no child processes"},
{11, "EDEADLK", "resource deadlock avoided"},
{12, "ENOMEM", "cannot allocate memory"},
{13, "EACCES", "permission denied"},
{14, "EFAULT", "bad address"},
{15, "ENOTBLK", "block device required"},
{16, "EBUSY", "resource busy"},
{17, "EEXIST", "file exists"},
{18, "EXDEV", "cross-device link"},
{19, "ENODEV", "operation not supported by device"},
{20, "ENOTDIR", "not a directory"},
{21, "EISDIR", "is a directory"},
{22, "EINVAL", "invalid argument"},
{23, "ENFILE", "too many open files in system"},
{24, "EMFILE", "too many open files"},
{25, "ENOTTY", "inappropriate ioctl for device"},
{26, "ETXTBSY", "text file busy"},
{27, "EFBIG", "file too large"},
{28, "ENOSPC", "no space left on device"},
{29, "ESPIPE", "illegal seek"},
{30, "EROFS", "read-only file system"},
{31, "EMLINK", "too many links"},
{32, "EPIPE", "broken pipe"},
{33, "EDOM", "numerical argument out of domain"},
{34, "ERANGE", "result too large"},
{35, "EAGAIN", "resource temporarily unavailable"},
{36, "EINPROGRESS", "operation now in progress"},
{37, "EALREADY", "operation already in progress"},
{38, "ENOTSOCK", "socket operation on non-socket"},
{39, "EDESTADDRREQ", "destination address required"},
{40, "EMSGSIZE", "message too long"},
{41, "EPROTOTYPE", "protocol wrong type for socket"},
{42, "ENOPROTOOPT", "protocol not available"},
{43, "EPROTONOSUPPORT", "protocol not supported"},
{44, "ESOCKTNOSUPPORT", "socket type not supported"},
{45, "ENOTSUP", "operation not supported"},
{46, "EPFNOSUPPORT", "protocol family not supported"},
{47, "EAFNOSUPPORT", "address family not supported by protocol family"},
{48, "EADDRINUSE", "address already in use"},
{49, "EADDRNOTAVAIL", "can't assign requested address"},
{50, "ENETDOWN", "network is down"},
{51, "ENETUNREACH", "network is unreachable"},
{52, "ENETRESET", "network dropped connection on reset"},
{53, "ECONNABORTED", "software caused connection abort"},
{54, "ECONNRESET", "connection reset by peer"},
{55, "ENOBUFS", "no buffer space available"},
{56, "EISCONN", "socket is already connected"},
{57, "ENOTCONN", "socket is not connected"},
{58, "ESHUTDOWN", "can't send after socket shutdown"},
{59, "ETOOMANYREFS", "too many references: can't splice"},
{60, "ETIMEDOUT", "operation timed out"},
{61, "ECONNREFUSED", "connection refused"},
{62, "ELOOP", "too many levels of symbolic links"},
{63, "ENAMETOOLONG", "file name too long"},
{64, "EHOSTDOWN", "host is down"},
{65, "EHOSTUNREACH", "no route to host"},
{66, "ENOTEMPTY", "directory not empty"},
{67, "EPROCLIM", "too many processes"},
{68, "EUSERS", "too many users"},
{69, "EDQUOT", "disc quota exceeded"},
{70, "ESTALE", "stale NFS file handle"},
{71, "EREMOTE", "too many levels of remote in path"},
{72, "EBADRPC", "RPC struct is bad"},
{73, "ERPCMISMATCH", "RPC version wrong"},
{74, "EPROGUNAVAIL", "RPC prog. not avail"},
{75, "EPROGMISMATCH", "program version wrong"},
{76, "EPROCUNAVAIL", "bad procedure for program"},
{77, "ENOLCK", "no locks available"},
{78, "ENOSYS", "function not implemented"},
{79, "EFTYPE", "inappropriate file type or format"},
{80, "EAUTH", "authentication error"},
{81, "ENEEDAUTH", "need authenticator"},
{82, "EPWROFF", "device power is off"},
{83, "EDEVERR", "device error"},
{84, "EOVERFLOW", "value too large to be stored in data type"},
{85, "EBADEXEC", "bad executable (or shared library)"},
{86, "EBADARCH", "bad CPU type in executable"},
{87, "ESHLIBVERS", "shared library version mismatch"},
{88, "EBADMACHO", "malformed Mach-o file"},
{89, "ECANCELED", "operation canceled"},
{90, "EIDRM", "identifier removed"},
{91, "ENOMSG", "no message of desired type"},
{92, "EILSEQ", "illegal byte sequence"},
{93, "ENOATTR", "attribute not found"},
{94, "EBADMSG", "bad message"},
{95, "EMULTIHOP", "EMULTIHOP (Reserved)"},
{96, "ENODATA", "no message available on STREAM"},
{97, "ENOLINK", "ENOLINK (Reserved)"},
{98, "ENOSR", "no STREAM resources"},
{99, "ENOSTR", "not a STREAM"},
{100, "EPROTO", "protocol error"},
{101, "ETIME", "STREAM ioctl timeout"},
{102, "EOPNOTSUPP", "operation not supported on socket"},
{103, "ENOPOLICY", "policy not found"},
{104, "ENOTRECOVERABLE", "state not recoverable"},
{105, "EOWNERDEAD", "previous owner died"},
{106, "EQFULL", "interface output queue is full"},
}
// Signal table
var signalList = [...]struct {
num syscall.Signal
name string
desc string
}{
{1, "SIGHUP", "hangup"},
{2, "SIGINT", "interrupt"},
{3, "SIGQUIT", "quit"},
{4, "SIGILL", "illegal instruction"},
{5, "SIGTRAP", "trace/BPT trap"},
{6, "SIGABRT", "abort trap"},
{7, "SIGEMT", "EMT trap"},
{8, "SIGFPE", "floating point exception"},
{9, "SIGKILL", "killed"},
{10, "SIGBUS", "bus error"},
{11, "SIGSEGV", "segmentation fault"},
{12, "SIGSYS", "bad system call"},
{13, "SIGPIPE", "broken pipe"},
{14, "SIGALRM", "alarm clock"},
{15, "SIGTERM", "terminated"},
{16, "SIGURG", "urgent I/O condition"},
{17, "SIGSTOP", "suspended (signal)"},
{18, "SIGTSTP", "suspended"},
{19, "SIGCONT", "continued"},
{20, "SIGCHLD", "child exited"},
{21, "SIGTTIN", "stopped (tty input)"},
{22, "SIGTTOU", "stopped (tty output)"},
{23, "SIGIO", "I/O possible"},
{24, "SIGXCPU", "cputime limit exceeded"},
{25, "SIGXFSZ", "filesize limit exceeded"},
{26, "SIGVTALRM", "virtual timer expired"},
{27, "SIGPROF", "profiling timer expired"},
{28, "SIGWINCH", "window size changes"},
{29, "SIGINFO", "information request"},
{30, "SIGUSR1", "user defined signal 1"},
{31, "SIGUSR2", "user defined signal 2"},
}
| {
"pile_set_name": "Github"
} |
/* Copyright 2012 Yaqiang Wang,
* [email protected]
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or (at
* your option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser
* General Public License for more details.
*/
package org.meteoinfo.data.mapdata.geotiff;
import java.util.HashMap;
/**
*
* @author yaqiang
*/
public class Tag implements Comparable {
// <editor-fold desc="Variables">
private static HashMap map = new HashMap();
public static final Tag NewSubfileType = new Tag("NewSubfileType", 254);
public static final Tag ImageWidth = new Tag("ImageWidth", 256);
public static final Tag ImageLength = new Tag("ImageLength", 257);
public static final Tag BitsPerSample = new Tag("BitsPerSample", 258);
public static final Tag Compression = new Tag("Compression", 259);
public static final Tag PhotometricInterpretation = new Tag("PhotometricInterpretation", 262);
public static final Tag FillOrder = new Tag("FillOrder", 266);
public static final Tag DocumentName = new Tag("DocumentName", 269);
public static final Tag ImageDescription = new Tag("ImageDescription", 270);
public static final Tag StripOffsets = new Tag("StripOffsets", 273);
public static final Tag Orientation = new Tag("Orientation", 274);
public static final Tag SamplesPerPixel = new Tag("SamplesPerPixel", 277);
public static final Tag RowsPerStrip = new Tag("RowsPerStrip", 278);
public static final Tag StripByteCounts = new Tag("StripByteCounts", 279);
public static final Tag XResolution = new Tag("XResolution", 282);
public static final Tag YResolution = new Tag("YResolution", 283);
public static final Tag PlanarConfiguration = new Tag("PlanarConfiguration", 284);
public static final Tag ResolutionUnit = new Tag("ResolutionUnit", 296);
public static final Tag PageNumber = new Tag("PageNumber", 297);
public static final Tag Software = new Tag("Software", 305);
public static final Tag ColorMap = new Tag("ColorMap", 320);
public static final Tag TileWidth = new Tag("TileWidth", 322);
public static final Tag TileLength = new Tag("TileLength", 323);
public static final Tag TileOffsets = new Tag("TileOffsets", 324);
public static final Tag TileByteCounts = new Tag("TileByteCounts", 325);
public static final Tag SampleFormat = new Tag("SampleFormat", 339);
public static final Tag SMinSampleValue = new Tag("SMinSampleValue", 340);
public static final Tag SMaxSampleValue = new Tag("SMaxSampleValue", 341);
public static final Tag ModelPixelScaleTag = new Tag("ModelPixelScaleTag", 33550);
public static final Tag IntergraphMatrixTag = new Tag("IntergraphMatrixTag", 33920);
public static final Tag ModelTiepointTag = new Tag("ModelTiepointTag", 33922);
public static final Tag ModelTransformationTag = new Tag("ModelTransformationTag", 34264);
public static final Tag GeoKeyDirectoryTag = new Tag("GeoKeyDirectoryTag", 34735);
public static final Tag GeoDoubleParamsTag = new Tag("GeoDoubleParamsTag", 34736);
public static final Tag GeoAsciiParamsTag = new Tag("GeoAsciiParamsTag", 34737);
public static final Tag GDALNoData = new Tag("GDALNoDataTag", 42113);
private String name;
private int code;
// </editor-fold>
// <editor-fold desc="Constructor">
static Tag get(int code) {
return (Tag) map.get(new Integer(code));
}
private Tag(String name, int code) {
this.name = name;
this.code = code;
map.put(new Integer(code), this);
}
Tag(int code) {
this.code = code;
}
// </editor-fold>
// <editor-fold desc="Get Set Methods">
/**
* Get name
*
* @return Name
*/
public String getName() {
return this.name;
}
/**
* Get code
*
* @return Code
*/
public int getCode() {
return this.code;
}
// </editor-fold>
// <editor-fold desc="Methods">
/**
* To string
*
* @return String
*/
@Override
public String toString() {
return this.code + " (" + this.name + ")";
}
/**
* Compare to
*
* @param o Object
* @return Int
*/
@Override
public int compareTo(Object o) {
return this.code - ((Tag) o).getCode();
}
// </editor-fold>
}
| {
"pile_set_name": "Github"
} |
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
using System;
using System.Collections.Generic;
using Aliyun.Acs.Core.Transform;
using Aliyun.Acs.Iot.Model.V20180120;
namespace Aliyun.Acs.Iot.Transform.V20180120
{
public class CreateProductTagsResponseUnmarshaller
{
public static CreateProductTagsResponse Unmarshall(UnmarshallerContext context)
{
CreateProductTagsResponse createProductTagsResponse = new CreateProductTagsResponse();
createProductTagsResponse.HttpResponse = context.HttpResponse;
createProductTagsResponse.RequestId = context.StringValue("CreateProductTags.RequestId");
createProductTagsResponse.Success = context.BooleanValue("CreateProductTags.Success");
createProductTagsResponse.ErrorMessage = context.StringValue("CreateProductTags.ErrorMessage");
createProductTagsResponse.Code = context.StringValue("CreateProductTags.Code");
List<CreateProductTagsResponse.CreateProductTags_ProductTag> createProductTagsResponse_invalidProductTags = new List<CreateProductTagsResponse.CreateProductTags_ProductTag>();
for (int i = 0; i < context.Length("CreateProductTags.InvalidProductTags.Length"); i++) {
CreateProductTagsResponse.CreateProductTags_ProductTag productTag = new CreateProductTagsResponse.CreateProductTags_ProductTag();
productTag.TagKey = context.StringValue("CreateProductTags.InvalidProductTags["+ i +"].TagKey");
productTag.TagValue = context.StringValue("CreateProductTags.InvalidProductTags["+ i +"].TagValue");
createProductTagsResponse_invalidProductTags.Add(productTag);
}
createProductTagsResponse.InvalidProductTags = createProductTagsResponse_invalidProductTags;
return createProductTagsResponse;
}
}
}
| {
"pile_set_name": "Github"
} |
/**
* Marlin 3D Printer Firmware
* Copyright (c) 2020 MarlinFirmware [https://github.com/MarlinFirmware/Marlin]
*
* Based on Sprinter and grbl.
* Copyright (c) 2011 Camiel Gubbels / Erik van der Zalm
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <https://www.gnu.org/licenses/>.
*
*/
#include "../../inc/MarlinConfigPre.h"
#if HAS_UI_320x240
#include "ui_320x240.h"
#include "../ultralcd.h"
#include "../menu/menu.h"
#include "../../libs/numtostr.h"
#include "../../sd/cardreader.h"
#include "../../module/temperature.h"
#include "../../module/printcounter.h"
#include "../../module/planner.h"
#include "../../module/motion.h"
#if DISABLED(LCD_PROGRESS_BAR) && BOTH(FILAMENT_LCD_DISPLAY, SDSUPPORT)
#include "../../feature/filwidth.h"
#include "../../gcode/parser.h"
#endif
#if ENABLED(AUTO_BED_LEVELING_UBL)
#include "../../feature/bedlevel/bedlevel.h"
#endif
#if !HAS_LCD_MENU
#error "Seriously? High resolution TFT screen without menu?"
#endif
static bool draw_menu_navigation = false;
void MarlinUI::tft_idle() {
#if ENABLED(TOUCH_SCREEN)
if (draw_menu_navigation) {
add_control(48, 206, PAGE_UP, imgPageUp, encoderTopLine > 0);
add_control(240, 206, PAGE_DOWN, imgPageDown, encoderTopLine + LCD_HEIGHT < screen_items);
add_control(144, 206, BACK, imgBack);
draw_menu_navigation = false;
}
#endif
tft.queue.async();
TERN_(TOUCH_SCREEN, touch.idle());
}
void MarlinUI::init_lcd() {
tft.init();
tft.set_font(MENU_FONT_NAME);
#ifdef SYMBOLS_FONT_NAME
tft.add_glyphs(SYMBOLS_FONT_NAME);
#endif
TERN_(TOUCH_SCREEN, touch.init());
clear_lcd();
}
bool MarlinUI::detected() { return true; }
void MarlinUI::clear_lcd() {
#if ENABLED(TOUCH_SCREEN)
touch.reset();
draw_menu_navigation = false;
#endif
tft.queue.reset();
tft.fill(0, 0, TFT_WIDTH, TFT_HEIGHT, COLOR_BACKGROUND);
}
#if ENABLED(SHOW_BOOTSCREEN)
#ifndef BOOTSCREEN_TIMEOUT
#define BOOTSCREEN_TIMEOUT 1500
#endif
void MarlinUI::show_bootscreen() {
tft.queue.reset();
tft.canvas(0, 0, TFT_WIDTH, TFT_HEIGHT);
tft.add_image(0, 0, imgBootScreen); // MarlinLogo320x240x16
#ifdef WEBSITE_URL
tft.add_text(4, 188, COLOR_WEBSITE_URL, WEBSITE_URL);
#endif
tft.queue.sync();
safe_delay(BOOTSCREEN_TIMEOUT);
clear_lcd();
}
#endif // SHOW_BOOTSCREEN
void MarlinUI::draw_kill_screen() {
tft.queue.reset();
tft.fill(0, 0, TFT_WIDTH, TFT_HEIGHT, COLOR_KILL_SCREEN_BG);
tft.canvas(0, 60, TFT_WIDTH, 20);
tft.set_background(COLOR_KILL_SCREEN_BG);
tft_string.set(status_message);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), 0, COLOR_KILL_SCREEN_TEXT, tft_string);
tft.canvas(0, 120, TFT_WIDTH, 20);
tft.set_background(COLOR_KILL_SCREEN_BG);
tft_string.set(GET_TEXT(MSG_HALTED));
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), 0, COLOR_KILL_SCREEN_TEXT, tft_string);
tft.canvas(0, 160, TFT_WIDTH, 20);
tft.set_background(COLOR_KILL_SCREEN_BG);
tft_string.set(GET_TEXT(MSG_PLEASE_RESET));
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), 0, COLOR_KILL_SCREEN_TEXT, tft_string);
tft.queue.sync();
}
void draw_heater_status(uint16_t x, uint16_t y, const int8_t Heater) {
MarlinImage image = imgHotEnd;
uint16_t Color;
float currentTemperature, targetTemperature;
if (Heater >= 0) { // HotEnd
currentTemperature = thermalManager.degHotend(Heater);
targetTemperature = thermalManager.degTargetHotend(Heater);
}
#if HAS_HEATED_BED
else if (Heater == H_BED) {
currentTemperature = thermalManager.degBed();
targetTemperature = thermalManager.degTargetBed();
}
#endif // HAS_HEATED_BED
#if HAS_TEMP_CHAMBER
else if (Heater == H_CHAMBER) {
currentTemperature = thermalManager.degChamber();
#if HAS_HEATED_CHAMBER
targetTemperature = thermalManager.degTargetChamber();
#else
targetTemperature = ABSOLUTE_ZERO;
#endif
}
#endif // HAS_TEMP_CHAMBER
else return;
TERN_(TOUCH_SCREEN, if (targetTemperature >= 0) touch.add_control(HEATER, x, y, 64, 100, Heater));
tft.canvas(x, y, 64, 100);
tft.set_background(COLOR_BACKGROUND);
Color = currentTemperature < 0 ? COLOR_INACTIVE : COLOR_COLD;
if (Heater >= 0) { // HotEnd
if (currentTemperature >= 50) Color = COLOR_HOTEND;
}
#if HAS_HEATED_BED
else if (Heater == H_BED) {
if (currentTemperature >= 50) Color = COLOR_HEATED_BED;
image = targetTemperature > 0 ? imgBedHeated : imgBed;
}
#endif // HAS_HEATED_BED
#if HAS_TEMP_CHAMBER
else if (Heater == H_CHAMBER) {
if (currentTemperature >= 50) Color = COLOR_CHAMBER;
image = targetTemperature > 0 ? imgChamberHeated : imgChamber;
}
#endif // HAS_TEMP_CHAMBER
tft.add_image(0, 18, image, Color);
tft_string.set((uint8_t *)i16tostr3rj(currentTemperature + 0.5));
tft_string.add(LCD_STR_DEGREE);
tft_string.trim();
tft.add_text(tft_string.center(64) + 2, 72, Color, tft_string);
if (targetTemperature >= 0) {
tft_string.set((uint8_t *)i16tostr3rj(targetTemperature + 0.5));
tft_string.add(LCD_STR_DEGREE);
tft_string.trim();
tft.add_text(tft_string.center(64) + 2, 8, Color, tft_string);
}
}
void draw_fan_status(uint16_t x, uint16_t y, const bool blink) {
TERN_(TOUCH_SCREEN, touch.add_control(FAN, x, y, 64, 100));
tft.canvas(x, y, 64, 100);
tft.set_background(COLOR_BACKGROUND);
uint8_t fanSpeed = thermalManager.fan_speed[0];
MarlinImage image;
if (fanSpeed >= 127)
image = blink ? imgFanFast1 : imgFanFast0;
else if (fanSpeed > 0)
image = blink ? imgFanSlow1 : imgFanSlow0;
else
image = imgFanIdle;
tft.add_image(0, 10, image, COLOR_FAN);
tft_string.set((uint8_t *)ui8tostr4pctrj(thermalManager.fan_speed[0]));
tft_string.trim();
tft.add_text(tft_string.center(64) + 6, 72, COLOR_FAN, tft_string);
}
void MarlinUI::draw_status_screen() {
const bool blink = get_blink();
TERN_(TOUCH_SCREEN, touch.clear());
// heaters and fan
uint16_t i, x, y = POS_Y;
for (i = 0 ; i < ITEMS_COUNT; i++) {
x = (320 / ITEMS_COUNT - 64) / 2 + (320 * i / ITEMS_COUNT);
switch (i) {
#ifdef ITEM_E0
case ITEM_E0: draw_heater_status(x, y, H_E0); break;
#endif
#ifdef ITEM_E1
case ITEM_E1: draw_heater_status(x, y, H_E1); break;
#endif
#ifdef ITEM_E2
case ITEM_E2: draw_heater_status(x, y, H_E2); break;
#endif
#ifdef ITEM_BED
case ITEM_BED: draw_heater_status(x, y, H_BED); break;
#endif
#ifdef ITEM_CHAMBER
case ITEM_CHAMBER: draw_heater_status(x, y, H_CHAMBER); break;
#endif
#ifdef ITEM_FAN
case ITEM_FAN: draw_fan_status(x, y, blink); break;
#endif
}
}
// coordinates
tft.canvas(4, 103, 312, 24);
tft.set_background(COLOR_BACKGROUND);
tft.add_rectangle(0, 0, 312, 24, COLOR_AXIS_HOMED);
uint16_t color;
uint16_t offset;
bool is_homed;
tft.add_text( 10, 3, COLOR_AXIS_HOMED , "X");
tft.add_text(127, 3, COLOR_AXIS_HOMED , "Y");
tft.add_text(219, 3, COLOR_AXIS_HOMED , "Z");
is_homed = TEST(axis_homed, X_AXIS);
tft_string.set(blink & !is_homed ? "?" : ftostr4sign(LOGICAL_X_POSITION(current_position.x)));
tft.add_text( 68 - tft_string.width(), 3, is_homed ? COLOR_AXIS_HOMED : COLOR_AXIS_NOT_HOMED, tft_string);
is_homed = TEST(axis_homed, Y_AXIS);
tft_string.set(blink & !is_homed ? "?" : ftostr4sign(LOGICAL_Y_POSITION(current_position.y)));
tft.add_text(185 - tft_string.width(), 3, is_homed ? COLOR_AXIS_HOMED : COLOR_AXIS_NOT_HOMED, tft_string);
is_homed = TEST(axis_homed, Z_AXIS);
if (blink & !is_homed) {
tft_string.set("?");
offset = 25; // ".00"
}
else {
const float z = LOGICAL_Z_POSITION(current_position.z);
tft_string.set(ftostr52sp((int16_t)z));
tft_string.rtrim();
offset = tft_string.width();
tft_string.set(ftostr52sp(z));
offset += 25 - tft_string.width();
}
tft.add_text(301 - tft_string.width() - offset, 3, is_homed ? COLOR_AXIS_HOMED : COLOR_AXIS_NOT_HOMED, tft_string);
// feed rate
tft.canvas(70, 136, 80, 32);
tft.set_background(COLOR_BACKGROUND);
color = feedrate_percentage == 100 ? COLOR_RATE_100 : COLOR_RATE_ALTERED;
tft.add_image(0, 0, imgFeedRate, color);
tft_string.set(i16tostr3rj(feedrate_percentage));
tft_string.add('%');
tft.add_text(32, 6, color , tft_string);
TERN_(TOUCH_SCREEN, touch.add_control(FEEDRATE, 70, 136, 80, 32));
// flow rate
tft.canvas(170, 136, 80, 32);
tft.set_background(COLOR_BACKGROUND);
color = planner.flow_percentage[0] == 100 ? COLOR_RATE_100 : COLOR_RATE_ALTERED;
tft.add_image(0, 0, imgFlowRate, color);
tft_string.set(i16tostr3rj(planner.flow_percentage[active_extruder]));
tft_string.add('%');
tft.add_text(32, 6, color , tft_string);
TERN_(TOUCH_SCREEN, touch.add_control(FLOWRATE, 170, 136, 80, 32, active_extruder));
// print duration
char buffer[14];
duration_t elapsed = print_job_timer.duration();
elapsed.toDigital(buffer);
tft.canvas(96, 176, 128, 20);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(buffer);
tft.add_text(tft_string.center(128), 0, COLOR_PRINT_TIME, tft_string);
// progress bar
const uint8_t progress = ui.get_progress_percent();
tft.canvas(4, 198, 312, 9);
tft.set_background(COLOR_PROGRESS_BG);
tft.add_rectangle(0, 0, 312, 9, COLOR_PROGRESS_FRAME);
if (progress)
tft.add_bar(1, 1, (310 * progress) / 100, 7, COLOR_PROGRESS_BAR);
// status message
tft.canvas(0, 216, 320, 20);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(status_message);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), 0, COLOR_STATUS_MESSAGE, tft_string);
#if ENABLED(TOUCH_SCREEN)
add_control(256, 130, menu_main, imgSettings);
TERN_(SDSUPPORT, add_control(0, 130, menu_media, imgSD, card.isMounted() && !printingIsActive(), COLOR_CONTROL_ENABLED, card.isMounted() && printingIsActive() ? COLOR_BUSY : COLOR_CONTROL_DISABLED));
#endif
}
// Draw a static item with no left-right margin required. Centered by default.
void MenuItem_static::draw(const uint8_t row, PGM_P const pstr, const uint8_t style/*=SS_DEFAULT*/, const char * const vstr/*=nullptr*/) {
menu_item(row);
tft_string.set(pstr, itemIndex, itemString);
if (vstr)
tft_string.add(vstr);
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_YELLOW, tft_string);
}
// Draw a generic menu item with pre_char (if selected) and post_char
void MenuItemBase::_draw(const bool sel, const uint8_t row, PGM_P const pstr, const char pre_char, const char post_char) {
menu_item(row, sel);
uint8_t *string = (uint8_t *)pstr;
MarlinImage image = noImage;
switch (*string) {
case 0x01: image = imgRefresh; break; // LCD_STR_REFRESH
case 0x02: image = imgDirectory; break; // LCD_STR_FOLDER
}
uint8_t offset = MENU_TEXT_X_OFFSET;
if (image != noImage) {
string++;
offset = 32;
tft.add_image(0, 0, image, COLOR_MENU_TEXT, sel ? COLOR_SELECTION_BG : COLOR_BACKGROUND);
}
tft_string.set(string, itemIndex, itemString);
tft.add_text(offset, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
}
// Draw a menu item with a (potentially) editable value
void MenuEditItemBase::draw(const bool sel, const uint8_t row, PGM_P const pstr, const char* const data, const bool pgm) {
menu_item(row, sel);
tft_string.set(pstr, itemIndex, itemString);
tft.add_text(MENU_TEXT_X_OFFSET, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
if (data) {
tft_string.set(data);
tft.add_text(TFT_WIDTH - MENU_TEXT_X_OFFSET - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
}
}
// Low-level draw_edit_screen can be used to draw an edit screen from anyplace
void MenuEditItemBase::draw_edit_screen(PGM_P const pstr, const char* const value/*=nullptr*/) {
ui.encoder_direction_normal();
TERN_(TOUCH_SCREEN, touch.clear());
uint16_t line = 1;
menu_line(line++);
tft_string.set(pstr, itemIndex, itemString);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
TERN_(AUTO_BED_LEVELING_UBL, if (ui.external_control) line++); // ftostr52() will overwrite *value so *value has to be displayed first
menu_line(line);
tft_string.set(value);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
#if ENABLED(AUTO_BED_LEVELING_UBL)
if (ui.external_control) {
menu_line(line - 1);
tft_string.set(X_LBL);
tft.add_text(52, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
tft_string.set(ftostr52(LOGICAL_X_POSITION(current_position.x)));
tft_string.trim();
tft.add_text(144 - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
tft_string.set(Y_LBL);
tft.add_text(176, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
tft_string.set(ftostr52(LOGICAL_X_POSITION(current_position.y)));
tft_string.trim();
tft.add_text(268 - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
}
#endif
extern screenFunc_t _manual_move_func_ptr;
if (ui.currentScreen != _manual_move_func_ptr && !ui.external_control) {
#define SLIDER_LENGHT 224
#define SLIDER_Y_POSITION 140
tft.canvas((TFT_WIDTH - SLIDER_LENGHT) / 2, SLIDER_Y_POSITION, SLIDER_LENGHT, 16);
tft.set_background(COLOR_BACKGROUND);
int16_t position = (SLIDER_LENGHT - 2) * ui.encoderPosition / maxEditValue;
tft.add_bar(0, 7, 1, 2, ui.encoderPosition == 0 ? COLOR_SLIDER_INACTIVE : COLOR_SLIDER);
tft.add_bar(1, 6, position, 4, COLOR_SLIDER);
tft.add_bar(position + 1, 6, SLIDER_LENGHT - 2 - position, 4, COLOR_SLIDER_INACTIVE);
tft.add_bar(SLIDER_LENGHT - 1, 7, 1, 2, int32_t(ui.encoderPosition) == maxEditValue ? COLOR_SLIDER : COLOR_SLIDER_INACTIVE);
#if ENABLED(TOUCH_SCREEN)
tft.add_image((SLIDER_LENGHT - 8) * ui.encoderPosition / maxEditValue, 0, imgSlider, COLOR_SLIDER);
touch.add_control(SLIDER, (TFT_WIDTH - SLIDER_LENGHT) / 2, SLIDER_Y_POSITION - 8, SLIDER_LENGHT, 32, maxEditValue);
#endif
}
#if ENABLED(TOUCH_SCREEN)
add_control(32, 176, DECREASE, imgDecrease);
add_control(224, 176, INCREASE, imgIncrease);
add_control(128, 176, CLICK, imgConfirm);
#endif
}
// The Select Screen presents a prompt and two "buttons"
void MenuItem_confirm::draw_select_screen(PGM_P const yes, PGM_P const no, const bool yesno, PGM_P const pref, const char * const string/*=nullptr*/, PGM_P const suff/*=nullptr*/) {
uint16_t line = 1;
if (string == NULL) line++;
menu_line(line++);
tft_string.set(pref);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
if (string) {
menu_line(line++);
tft_string.set(string);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
}
if (suff) {
menu_line(line);
tft_string.set(suff);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
}
#if ENABLED(TOUCH_SCREEN)
add_control(48, 176, CANCEL, imgCancel, true, yesno ? HALF(COLOR_CONTROL_CANCEL) : COLOR_CONTROL_CANCEL);
add_control(208, 176, CONFIRM, imgConfirm, true, yesno ? COLOR_CONTROL_CONFIRM : HALF(COLOR_CONTROL_CONFIRM));
#endif
}
#if ENABLED(SDSUPPORT)
void MenuItem_sdbase::draw(const bool sel, const uint8_t row, PGM_P const, CardReader &theCard, const bool isDir) {
menu_item(row, sel);
if (isDir)
tft.add_image(0, 0, imgDirectory, COLOR_MENU_TEXT, sel ? COLOR_SELECTION_BG : COLOR_BACKGROUND);
tft.add_text(32, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, theCard.longest_filename());
}
#endif
#if ENABLED(ADVANCED_PAUSE_FEATURE)
void MarlinUI::draw_hotend_status(const uint8_t row, const uint8_t extruder) {
#if ENABLED(TOUCH_SCREEN)
touch.clear();
draw_menu_navigation = false;
touch.add_control(RESUME_CONTINUE , 0, 0, 320, 240);
#endif
menu_line(row);
tft_string.set(GET_TEXT(MSG_FILAMENT_CHANGE_NOZZLE));
tft_string.add('E');
tft_string.add((char)('1' + extruder));
tft_string.add(' ');
tft_string.add(i16tostr3rj(thermalManager.degHotend(extruder)));
tft_string.add(LCD_STR_DEGREE);
tft_string.add(" / ");
tft_string.add(i16tostr3rj(thermalManager.degTargetHotend(extruder)));
tft_string.add(LCD_STR_DEGREE);
tft_string.trim();
tft.add_text(tft_string.center(TFT_WIDTH), MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
}
#endif // ADVANCED_PAUSE_FEATURE
#if ENABLED(AUTO_BED_LEVELING_UBL)
#define GRID_OFFSET_X 8
#define GRID_OFFSET_Y 8
#define GRID_WIDTH 144
#define GRID_HEIGHT 144
#define CONTROL_OFFSET 8
void MarlinUI::ubl_plot(const uint8_t x_plot, const uint8_t y_plot) {
tft.canvas(GRID_OFFSET_X, GRID_OFFSET_Y, GRID_WIDTH, GRID_HEIGHT);
tft.set_background(COLOR_BACKGROUND);
tft.add_rectangle(0, 0, GRID_WIDTH, GRID_HEIGHT, COLOR_WHITE);
for (uint16_t x = 0; x < GRID_MAX_POINTS_X ; x++)
for (uint16_t y = 0; y < GRID_MAX_POINTS_Y ; y++)
if (position_is_reachable({ ubl.mesh_index_to_xpos(x), ubl.mesh_index_to_ypos(y) }))
tft.add_bar(1 + (x * 2 + 1) * (GRID_WIDTH - 4) / GRID_MAX_POINTS_X / 2, GRID_HEIGHT - 3 - ((y * 2 + 1) * (GRID_HEIGHT - 4) / GRID_MAX_POINTS_Y / 2), 2, 2, COLOR_UBL);
tft.add_rectangle((x_plot * 2 + 1) * (GRID_WIDTH - 4) / GRID_MAX_POINTS_X / 2 - 1, GRID_HEIGHT - 5 - ((y_plot * 2 + 1) * (GRID_HEIGHT - 4) / GRID_MAX_POINTS_Y / 2), 6, 6, COLOR_UBL);
const xy_pos_t pos = { ubl.mesh_index_to_xpos(x_plot), ubl.mesh_index_to_ypos(y_plot) },
lpos = pos.asLogical();
tft.canvas(216, GRID_OFFSET_Y + (GRID_HEIGHT - 32) / 2 - 32, 96, 32);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(X_LBL);
tft.add_text(0, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
tft_string.set(ftostr52(lpos.x));
tft_string.trim();
tft.add_text(96 - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
tft.canvas(216, GRID_OFFSET_Y + (GRID_HEIGHT - 32) / 2, 96, 32);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(Y_LBL);
tft.add_text(0, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
tft_string.set(ftostr52(lpos.y));
tft_string.trim();
tft.add_text(96 - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
tft.canvas(216, GRID_OFFSET_Y + (GRID_HEIGHT - 32) / 2 + 32, 96, 32);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(Z_LBL);
tft.add_text(0, MENU_TEXT_Y_OFFSET, COLOR_MENU_TEXT, tft_string);
tft_string.set(isnan(ubl.z_values[x_plot][y_plot]) ? "-----" : ftostr43sign(ubl.z_values[x_plot][y_plot]));
tft_string.trim();
tft.add_text(96 - tft_string.width(), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
tft.canvas(GRID_OFFSET_X + (GRID_WIDTH - 32) / 2, GRID_OFFSET_Y + GRID_HEIGHT + CONTROL_OFFSET - 1, 32, 32);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(ui8tostr3rj(x_plot));
tft_string.trim();
tft.add_text(tft_string.center(32), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
tft.canvas(GRID_OFFSET_X + GRID_WIDTH + CONTROL_OFFSET, GRID_OFFSET_Y + (GRID_HEIGHT - 27) / 2, 32, 32);
tft.set_background(COLOR_BACKGROUND);
tft_string.set(ui8tostr3rj(y_plot));
tft_string.trim();
tft.add_text(tft_string.center(32), MENU_TEXT_Y_OFFSET, COLOR_MENU_VALUE, tft_string);
#if ENABLED(TOUCH_SCREEN)
touch.clear();
draw_menu_navigation = false;
add_control(GRID_OFFSET_X + GRID_WIDTH + CONTROL_OFFSET, GRID_OFFSET_Y + CONTROL_OFFSET, UBL, ENCODER_STEPS_PER_MENU_ITEM * GRID_MAX_POINTS_X, imgUp);
add_control(GRID_OFFSET_X + GRID_WIDTH + CONTROL_OFFSET, GRID_OFFSET_Y + GRID_HEIGHT - CONTROL_OFFSET - 32, UBL, - ENCODER_STEPS_PER_MENU_ITEM * GRID_MAX_POINTS_X, imgDown);
add_control(GRID_OFFSET_X + CONTROL_OFFSET, GRID_OFFSET_Y + GRID_HEIGHT + CONTROL_OFFSET, UBL, - ENCODER_STEPS_PER_MENU_ITEM, imgLeft);
add_control(GRID_OFFSET_X + GRID_WIDTH - CONTROL_OFFSET - 32, GRID_OFFSET_Y + GRID_HEIGHT + CONTROL_OFFSET, UBL, ENCODER_STEPS_PER_MENU_ITEM, imgRight);
add_control(224, GRID_OFFSET_Y + GRID_HEIGHT + CONTROL_OFFSET, CLICK, imgLeveling);
add_control(144, 206, BACK, imgBack);
#endif
}
#endif // AUTO_BED_LEVELING_UBL
#if ENABLED(TOUCH_SCREEN_CALIBRATION)
void MarlinUI::touch_calibration() {
static uint16_t x, y;
calibrationState calibration_stage = touch.get_calibration_state();
if (calibration_stage == CALIBRATION_NONE) {
defer_status_screen(true);
clear_lcd();
calibration_stage = touch.calibration_start();
}
else {
tft.canvas(x - 15, y - 15, 31, 31);
tft.set_background(COLOR_BACKGROUND);
}
x = 20; y = 20;
touch.clear();
if (calibration_stage < CALIBRATION_SUCCESS) {
switch(calibration_stage) {
case CALIBRATION_POINT_1: tft_string.set("Top Left"); break;
case CALIBRATION_POINT_2: y = TFT_HEIGHT - 21; tft_string.set("Bottom Left"); break;
case CALIBRATION_POINT_3: x = TFT_WIDTH - 21; tft_string.set("Top Right"); break;
case CALIBRATION_POINT_4: x = TFT_WIDTH - 21; y = TFT_HEIGHT - 21; tft_string.set("Bottom Right"); break;
default: break;
}
tft.canvas(x - 15, y - 15, 31, 31);
tft.set_background(COLOR_BACKGROUND);
tft.add_bar(0, 15, 31, 1, COLOR_TOUCH_CALIBRATION);
tft.add_bar(15, 0, 1, 31, COLOR_TOUCH_CALIBRATION);
touch.add_control(CALIBRATE, 0, 0, TFT_WIDTH, TFT_HEIGHT, uint32_t(x) << 16 | uint32_t(y));
}
else {
tft_string.set(calibration_stage == CALIBRATION_SUCCESS ? "Calibration Completed" : "Calibration Failed");
defer_status_screen(false);
touch.calibration_end();
touch.add_control(BACK, 0, 0, TFT_WIDTH, TFT_HEIGHT);
}
tft.canvas(0, (TFT_HEIGHT - tft_string.font_height()) >> 1, TFT_WIDTH, tft_string.font_height());
tft.set_background(COLOR_BACKGROUND);
tft.add_text(tft_string.center(TFT_WIDTH), 0, COLOR_MENU_TEXT, tft_string);
}
#endif // TOUCH_SCREEN_CALIBRATION
void menu_line(const uint8_t row, uint16_t color) {
tft.canvas(0, 2 + 34 * row, TFT_WIDTH, 32);
tft.set_background(color);
}
void menu_pause_option();
void menu_item(const uint8_t row, bool sel ) {
#if ENABLED(TOUCH_SCREEN)
if (row == 0) {
touch.clear();
draw_menu_navigation = TERN(ADVANCED_PAUSE_FEATURE, ui.currentScreen != menu_pause_option, true);
}
#endif
menu_line(row, sel ? COLOR_SELECTION_BG : COLOR_BACKGROUND);
TERN_(TOUCH_SCREEN, touch.add_control(sel ? CLICK : MENU_ITEM, 0, 2 + 34 * row, 320, 32, encoderTopLine + row));
}
#endif // HAS_UI_320x240
| {
"pile_set_name": "Github"
} |
testConstructorMessageCause(org.apache.commons.math.FunctionEvaluationExceptionTest)
testConstructorMessage(org.apache.commons.math.FunctionEvaluationExceptionTest)
testConstructor(org.apache.commons.math.FunctionEvaluationExceptionTest)
testConstructorCause(org.apache.commons.math.MathConfigurationExceptionTest)
testConstructorMessageCause(org.apache.commons.math.MathConfigurationExceptionTest)
testConstructorMessage(org.apache.commons.math.MathConfigurationExceptionTest)
testConstructor(org.apache.commons.math.MathConfigurationExceptionTest)
testSerialization(org.apache.commons.math.MathExceptionTest)
testConstructorCause(org.apache.commons.math.MathExceptionTest)
testConstructorMessageCause(org.apache.commons.math.MathExceptionTest)
testConstructorMessage(org.apache.commons.math.MathExceptionTest)
testPrintStackTrace(org.apache.commons.math.MathExceptionTest)
testConstructor(org.apache.commons.math.MathExceptionTest)
testSetMaximalIterationCount(org.apache.commons.math.analysis.BisectionSolverTest)
testSetRelativeAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testSetAbsoluteAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testSerialization(org.apache.commons.math.analysis.BisectionSolverTest)
testSetFunctionValueAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testResetRelativeAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testResetAbsoluteAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testResetMaximalIterationCount(org.apache.commons.math.analysis.BisectionSolverTest)
testResetFunctionValueAccuracy(org.apache.commons.math.analysis.BisectionSolverTest)
testQuinticZero(org.apache.commons.math.analysis.BisectionSolverTest)
testSinZero(org.apache.commons.math.analysis.BisectionSolverTest)
testBadEndpoints(org.apache.commons.math.analysis.BrentSolverTest)
testQuinticZero(org.apache.commons.math.analysis.BrentSolverTest)
testSinZero(org.apache.commons.math.analysis.BrentSolverTest)
testConstructorCause(org.apache.commons.math.analysis.ConvergenceExceptionTest)
testConstructorMessageCause(org.apache.commons.math.analysis.ConvergenceExceptionTest)
testConstructorMessage(org.apache.commons.math.analysis.ConvergenceExceptionTest)
testConstructor(org.apache.commons.math.analysis.ConvergenceExceptionTest)
testParameters(org.apache.commons.math.analysis.DividedDifferenceInterpolatorTest)
testSinFunction(org.apache.commons.math.analysis.DividedDifferenceInterpolatorTest)
testExpm1Function(org.apache.commons.math.analysis.DividedDifferenceInterpolatorTest)
testLinearFunction(org.apache.commons.math.analysis.LaguerreSolverTest)
testQuadraticFunction(org.apache.commons.math.analysis.LaguerreSolverTest)
testParameters(org.apache.commons.math.analysis.LaguerreSolverTest)
testQuinticFunction2(org.apache.commons.math.analysis.LaguerreSolverTest)
testQuinticFunction(org.apache.commons.math.analysis.LaguerreSolverTest)
testExpm1Function2(org.apache.commons.math.analysis.MullerSolverTest)
testParameters(org.apache.commons.math.analysis.MullerSolverTest)
testSinFunction(org.apache.commons.math.analysis.MullerSolverTest)
testQuinticFunction2(org.apache.commons.math.analysis.MullerSolverTest)
testExpm1Function(org.apache.commons.math.analysis.MullerSolverTest)
testSinFunction2(org.apache.commons.math.analysis.MullerSolverTest)
testQuinticFunction(org.apache.commons.math.analysis.MullerSolverTest)
testParameters(org.apache.commons.math.analysis.NevilleInterpolatorTest)
testSinFunction(org.apache.commons.math.analysis.NevilleInterpolatorTest)
testExpm1Function(org.apache.commons.math.analysis.NevilleInterpolatorTest)
testSerialization(org.apache.commons.math.analysis.NewtonSolverTest)
testQuinticZero(org.apache.commons.math.analysis.NewtonSolverTest)
testSinZero(org.apache.commons.math.analysis.NewtonSolverTest)
testLinearFunction(org.apache.commons.math.analysis.PolynomialFunctionLagrangeFormTest)
testQuadraticFunction(org.apache.commons.math.analysis.PolynomialFunctionLagrangeFormTest)
testParameters(org.apache.commons.math.analysis.PolynomialFunctionLagrangeFormTest)
testQuinticFunction(org.apache.commons.math.analysis.PolynomialFunctionLagrangeFormTest)
testLinearFunction(org.apache.commons.math.analysis.PolynomialFunctionNewtonFormTest)
testQuadraticFunction(org.apache.commons.math.analysis.PolynomialFunctionNewtonFormTest)
testParameters(org.apache.commons.math.analysis.PolynomialFunctionNewtonFormTest)
testQuinticFunction(org.apache.commons.math.analysis.PolynomialFunctionNewtonFormTest)
testQuadratic(org.apache.commons.math.analysis.PolynomialFunctionTest)
testConstants(org.apache.commons.math.analysis.PolynomialFunctionTest)
testQuintic(org.apache.commons.math.analysis.PolynomialFunctionTest)
testLinear(org.apache.commons.math.analysis.PolynomialFunctionTest)
testfirstDerivativeComparision(org.apache.commons.math.analysis.PolynomialFunctionTest)
testValues(org.apache.commons.math.analysis.PolynomialSplineFunctionTest)
testConstructor(org.apache.commons.math.analysis.PolynomialSplineFunctionTest)
testParameters(org.apache.commons.math.analysis.RiddersSolverTest)
testSinFunction(org.apache.commons.math.analysis.RiddersSolverTest)
testExpm1Function(org.apache.commons.math.analysis.RiddersSolverTest)
testQuinticFunction(org.apache.commons.math.analysis.RiddersSolverTest)
testParameters(org.apache.commons.math.analysis.RombergIntegratorTest)
testSinFunction(org.apache.commons.math.analysis.RombergIntegratorTest)
testQuinticFunction(org.apache.commons.math.analysis.RombergIntegratorTest)
testParameters(org.apache.commons.math.analysis.SimpsonIntegratorTest)
testSinFunction(org.apache.commons.math.analysis.SimpsonIntegratorTest)
testQuinticFunction(org.apache.commons.math.analysis.SimpsonIntegratorTest)
testInterpolateSin(org.apache.commons.math.analysis.SplineInterpolatorTest)
testInterpolateLinearDegenerateTwoSegment(org.apache.commons.math.analysis.SplineInterpolatorTest)
testIllegalArguments(org.apache.commons.math.analysis.SplineInterpolatorTest)
testInterpolateLinear(org.apache.commons.math.analysis.SplineInterpolatorTest)
testInterpolateLinearDegenerateThreeSegment(org.apache.commons.math.analysis.SplineInterpolatorTest)
testParameters(org.apache.commons.math.analysis.TrapezoidIntegratorTest)
testSinFunction(org.apache.commons.math.analysis.TrapezoidIntegratorTest)
testQuinticFunction(org.apache.commons.math.analysis.TrapezoidIntegratorTest)
testNewBrentSolverValid(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewBisectionSolverValid(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewBrentSolverNull(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewBisectionSolverNull(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewNewtonSolverNull(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewNewtonSolverValid(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewSecantSolverValid(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testNewSecantSolverNull(org.apache.commons.math.analysis.UnivariateRealSolverFactoryImplTest)
testSolveNoRoot(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testSolveSin(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testSolveAccuracySin(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testSolveBadParameters(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testSolveNull(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testBracketSin(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testBracketCornerSolution(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testSolveAccuracyNull(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testBadParameters(org.apache.commons.math.analysis.UnivariateRealSolverUtilsTest)
testParseNegativeImaginary(org.apache.commons.math.complex.ComplexFormatTest)
testConstructorSingleFormat(org.apache.commons.math.complex.ComplexFormatTest)
testParseSimpleWithDecimals(org.apache.commons.math.complex.ComplexFormatTest)
testZeroImaginary(org.apache.commons.math.complex.ComplexFormatTest)
testSetImaginaryFormatNull(org.apache.commons.math.complex.ComplexFormatTest)
testDifferentImaginaryChar(org.apache.commons.math.complex.ComplexFormatTest)
testFormatNumber(org.apache.commons.math.complex.ComplexFormatTest)
testFormatObject(org.apache.commons.math.complex.ComplexFormatTest)
testNan(org.apache.commons.math.complex.ComplexFormatTest)
testSimpleWithDecimalsTrunc(org.apache.commons.math.complex.ComplexFormatTest)
testSetImaginaryCharacterNull(org.apache.commons.math.complex.ComplexFormatTest)
testStaticFormatComplex(org.apache.commons.math.complex.ComplexFormatTest)
testGetRealFormat(org.apache.commons.math.complex.ComplexFormatTest)
testParseNegativeBoth(org.apache.commons.math.complex.ComplexFormatTest)
testParseNegativeReal(org.apache.commons.math.complex.ComplexFormatTest)
testGetImaginaryFormat(org.apache.commons.math.complex.ComplexFormatTest)
testParseSimpleWithDecimalsTrunc(org.apache.commons.math.complex.ComplexFormatTest)
testNegativeInfinity(org.apache.commons.math.complex.ComplexFormatTest)
testSetRealFormatNull(org.apache.commons.math.complex.ComplexFormatTest)
testPaseNegativeInfinity(org.apache.commons.math.complex.ComplexFormatTest)
testParseDifferentImaginaryChar(org.apache.commons.math.complex.ComplexFormatTest)
testSetImaginaryCharacterEmpty(org.apache.commons.math.complex.ComplexFormatTest)
testSimpleNoDecimals(org.apache.commons.math.complex.ComplexFormatTest)
testZeroReal(org.apache.commons.math.complex.ComplexFormatTest)
testNegativeBoth(org.apache.commons.math.complex.ComplexFormatTest)
testNegativeReal(org.apache.commons.math.complex.ComplexFormatTest)
testNegativeImaginary(org.apache.commons.math.complex.ComplexFormatTest)
testParseSimpleNoDecimals(org.apache.commons.math.complex.ComplexFormatTest)
testPositiveInfinity(org.apache.commons.math.complex.ComplexFormatTest)
testParseZeroReal(org.apache.commons.math.complex.ComplexFormatTest)
testParseNan(org.apache.commons.math.complex.ComplexFormatTest)
testParseZeroImaginary(org.apache.commons.math.complex.ComplexFormatTest)
testParsePositiveInfinity(org.apache.commons.math.complex.ComplexFormatTest)
testSimpleWithDecimals(org.apache.commons.math.complex.ComplexFormatTest)
testConjugateNaN(org.apache.commons.math.complex.ComplexTest)
testEqualsClass(org.apache.commons.math.complex.ComplexTest)
testAddInfinite(org.apache.commons.math.complex.ComplexTest)
testAbs(org.apache.commons.math.complex.ComplexTest)
testAdd(org.apache.commons.math.complex.ComplexTest)
testSubtract(org.apache.commons.math.complex.ComplexTest)
testDivideNaNInf(org.apache.commons.math.complex.ComplexTest)
testDivideNaN(org.apache.commons.math.complex.ComplexTest)
testEqualsRealDifference(org.apache.commons.math.complex.ComplexTest)
testNegateNaN(org.apache.commons.math.complex.ComplexTest)
testEqualsNull(org.apache.commons.math.complex.ComplexTest)
testEqualsSame(org.apache.commons.math.complex.ComplexTest)
testEqualsTrue(org.apache.commons.math.complex.ComplexTest)
testMultiplyNaN(org.apache.commons.math.complex.ComplexTest)
testConjugate(org.apache.commons.math.complex.ComplexTest)
testMultiplyNaNInf(org.apache.commons.math.complex.ComplexTest)
testEqualsImaginaryDifference(org.apache.commons.math.complex.ComplexTest)
testConstructorNaN(org.apache.commons.math.complex.ComplexTest)
testHashCode(org.apache.commons.math.complex.ComplexTest)
testAbsNaN(org.apache.commons.math.complex.ComplexTest)
testAddNaN(org.apache.commons.math.complex.ComplexTest)
testDivide(org.apache.commons.math.complex.ComplexTest)
testMultiply(org.apache.commons.math.complex.ComplexTest)
testEqualsNaN(org.apache.commons.math.complex.ComplexTest)
testDivideInfinite(org.apache.commons.math.complex.ComplexTest)
testNegate(org.apache.commons.math.complex.ComplexTest)
testConjugateInfiinite(org.apache.commons.math.complex.ComplexTest)
testSubtractNaN(org.apache.commons.math.complex.ComplexTest)
testAbsInfinite(org.apache.commons.math.complex.ComplexTest)
testConstructor(org.apache.commons.math.complex.ComplexTest)
testTanNull(org.apache.commons.math.complex.ComplexUtilsTest)
testTanhInf(org.apache.commons.math.complex.ComplexUtilsTest)
testTanhNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrt1zNull(org.apache.commons.math.complex.ComplexUtilsTest)
testlogNull(org.apache.commons.math.complex.ComplexUtilsTest)
testExpNull(org.apache.commons.math.complex.ComplexUtilsTest)
testAcosInf(org.apache.commons.math.complex.ComplexUtilsTest)
testAcosNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testCos(org.apache.commons.math.complex.ComplexUtilsTest)
testExp(org.apache.commons.math.complex.ComplexUtilsTest)
testLog(org.apache.commons.math.complex.ComplexUtilsTest)
testPow(org.apache.commons.math.complex.ComplexUtilsTest)
testSin(org.apache.commons.math.complex.ComplexUtilsTest)
testTan(org.apache.commons.math.complex.ComplexUtilsTest)
testAcos(org.apache.commons.math.complex.ComplexUtilsTest)
testAsin(org.apache.commons.math.complex.ComplexUtilsTest)
testAtan(org.apache.commons.math.complex.ComplexUtilsTest)
testCosh(org.apache.commons.math.complex.ComplexUtilsTest)
testSinh(org.apache.commons.math.complex.ComplexUtilsTest)
testTanh(org.apache.commons.math.complex.ComplexUtilsTest)
testAsinInf(org.apache.commons.math.complex.ComplexUtilsTest)
testAsinNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testAtanInf(org.apache.commons.math.complex.ComplexUtilsTest)
testAtanNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testAcosNull(org.apache.commons.math.complex.ComplexUtilsTest)
testTanhCritical(org.apache.commons.math.complex.ComplexUtilsTest)
testPowZero(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtImaginaryZero(org.apache.commons.math.complex.ComplexUtilsTest)
testPolar2ComplexInf(org.apache.commons.math.complex.ComplexUtilsTest)
testPolar2ComplexNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtRealPositive(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrt1zNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtRealNegative(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtPolar(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtNull(org.apache.commons.math.complex.ComplexUtilsTest)
testLogZero(org.apache.commons.math.complex.ComplexUtilsTest)
testAsinNull(org.apache.commons.math.complex.ComplexUtilsTest)
testTanhNull(org.apache.commons.math.complex.ComplexUtilsTest)
testTanCritical(org.apache.commons.math.complex.ComplexUtilsTest)
testCoshNull(org.apache.commons.math.complex.ComplexUtilsTest)
testPowNaNBase(org.apache.commons.math.complex.ComplexUtilsTest)
testPolar2ComplexIllegalModulus(org.apache.commons.math.complex.ComplexUtilsTest)
testCosNull(org.apache.commons.math.complex.ComplexUtilsTest)
testCoshInf(org.apache.commons.math.complex.ComplexUtilsTest)
testCoshNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testAtanNull(org.apache.commons.math.complex.ComplexUtilsTest)
testPowNaNExponent(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtRealZero(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtImaginaryNegative(org.apache.commons.math.complex.ComplexUtilsTest)
testsinhNull(org.apache.commons.math.complex.ComplexUtilsTest)
testCosInf(org.apache.commons.math.complex.ComplexUtilsTest)
testCosNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testExpInf(org.apache.commons.math.complex.ComplexUtilsTest)
testExpNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testLogInf(org.apache.commons.math.complex.ComplexUtilsTest)
testLogNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testPowInf(org.apache.commons.math.complex.ComplexUtilsTest)
testSinNull(org.apache.commons.math.complex.ComplexUtilsTest)
testSinhInf(org.apache.commons.math.complex.ComplexUtilsTest)
testSinhNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testSinInf(org.apache.commons.math.complex.ComplexUtilsTest)
testSinNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrt1z(org.apache.commons.math.complex.ComplexUtilsTest)
testTanInf(org.apache.commons.math.complex.ComplexUtilsTest)
testTanNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testPolar2Complex(org.apache.commons.math.complex.ComplexUtilsTest)
testpowNull(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtInf(org.apache.commons.math.complex.ComplexUtilsTest)
testSqrtNaN(org.apache.commons.math.complex.ComplexUtilsTest)
testParseNegativeImaginary(org.apache.commons.math.complex.FrenchComplexFormatTest)
testConstructorSingleFormat(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseSimpleWithDecimals(org.apache.commons.math.complex.FrenchComplexFormatTest)
testZeroImaginary(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSetImaginaryFormatNull(org.apache.commons.math.complex.FrenchComplexFormatTest)
testDifferentImaginaryChar(org.apache.commons.math.complex.FrenchComplexFormatTest)
testFormatNumber(org.apache.commons.math.complex.FrenchComplexFormatTest)
testFormatObject(org.apache.commons.math.complex.FrenchComplexFormatTest)
testNan(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSimpleWithDecimalsTrunc(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSetImaginaryCharacterNull(org.apache.commons.math.complex.FrenchComplexFormatTest)
testStaticFormatComplex(org.apache.commons.math.complex.FrenchComplexFormatTest)
testGetRealFormat(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseNegativeBoth(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseNegativeReal(org.apache.commons.math.complex.FrenchComplexFormatTest)
testGetImaginaryFormat(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseSimpleWithDecimalsTrunc(org.apache.commons.math.complex.FrenchComplexFormatTest)
testNegativeInfinity(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSetRealFormatNull(org.apache.commons.math.complex.FrenchComplexFormatTest)
testPaseNegativeInfinity(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseDifferentImaginaryChar(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSetImaginaryCharacterEmpty(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSimpleNoDecimals(org.apache.commons.math.complex.FrenchComplexFormatTest)
testZeroReal(org.apache.commons.math.complex.FrenchComplexFormatTest)
testNegativeBoth(org.apache.commons.math.complex.FrenchComplexFormatTest)
testNegativeReal(org.apache.commons.math.complex.FrenchComplexFormatTest)
testNegativeImaginary(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseSimpleNoDecimals(org.apache.commons.math.complex.FrenchComplexFormatTest)
testPositiveInfinity(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseZeroReal(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseNan(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParseZeroImaginary(org.apache.commons.math.complex.FrenchComplexFormatTest)
testParsePositiveInfinity(org.apache.commons.math.complex.FrenchComplexFormatTest)
testSimpleWithDecimals(org.apache.commons.math.complex.FrenchComplexFormatTest)
testDegenerate0(org.apache.commons.math.distribution.BinomialDistributionTest)
testDegenerate1(org.apache.commons.math.distribution.BinomialDistributionTest)
testDensities(org.apache.commons.math.distribution.BinomialDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.BinomialDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.BinomialDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.BinomialDistributionTest)
testScale(org.apache.commons.math.distribution.CauchyDistributionTest)
testMedian(org.apache.commons.math.distribution.CauchyDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.CauchyDistributionTest)
testSetScale(org.apache.commons.math.distribution.CauchyDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.CauchyDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.CauchyDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.CauchyDistributionTest)
testConsistency(org.apache.commons.math.distribution.CauchyDistributionTest)
testDfAccessors(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testSmallDf(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testConsistency(org.apache.commons.math.distribution.ChiSquareDistributionTest)
testWeibullDistributionZeroPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionPositivePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionPositiveNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateTDistributionPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionPositivePositiveZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateTDistributionNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testWeibullDistributionNegativePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateExponentialDistributionZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCauchyDistributionNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionNegativePositivePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCauchyDistributionZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateGammaDistributionPositiveZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateFDistributionPositiveZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionPositiveZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateGammaDistributionNegativePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testWeibullDistributionPositiveZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionZeroPositivePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateFDistributionNegativePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testWeibullDistributionPositiveNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionPositiveNegativePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateGammaDistributionPositivePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateTDistributionZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateGammaDistributionPositiveNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionNegativePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionPositiveZeroPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateChiSquareDistributionPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateExponentialDistributionPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateChiSquareDistributionNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionSmallPopulationSize(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateFDistributionPositivePositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateExponentialDistributionNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateChiSquareDistributionZero(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateFDistributionPositiveNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testHypergeometricDistributionPositivePositiveNegative(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateGammaDistributionZeroPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCreateFDistributionZeroPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionZeroPositive(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionPositiveOne(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testBinomialDistributionPositiveTwo(org.apache.commons.math.distribution.DistributionFactoryImplTest)
testCumulativeProbability2(org.apache.commons.math.distribution.ExponentialDistributionTest)
testCumulativeProbabilityExtremes(org.apache.commons.math.distribution.ExponentialDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.ExponentialDistributionTest)
testMeanAccessors(org.apache.commons.math.distribution.ExponentialDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.ExponentialDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.ExponentialDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.ExponentialDistributionTest)
testConsistency(org.apache.commons.math.distribution.ExponentialDistributionTest)
testCumulativeProbabilityExtremes(org.apache.commons.math.distribution.FDistributionTest)
testLargeDegreesOfFreedom(org.apache.commons.math.distribution.FDistributionTest)
testDfAccessors(org.apache.commons.math.distribution.FDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.FDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.FDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.FDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.FDistributionTest)
testConsistency(org.apache.commons.math.distribution.FDistributionTest)
testProbabilities(org.apache.commons.math.distribution.GammaDistributionTest)
testParameterAccessors(org.apache.commons.math.distribution.GammaDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.GammaDistributionTest)
testValues(org.apache.commons.math.distribution.GammaDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.GammaDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.GammaDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.GammaDistributionTest)
testConsistency(org.apache.commons.math.distribution.GammaDistributionTest)
testLargeValues(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testDegenerateNoFailures(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testDegenerateNoSuccesses(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testDegenerateFullSample(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testPopulationSize(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testMoreLargeValues(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testDensities(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.HypergeometricDistributionTest)
testSetStandardDeviation(org.apache.commons.math.distribution.NormalDistributionTest)
testGetStandardDeviation(org.apache.commons.math.distribution.NormalDistributionTest)
testQuantiles(org.apache.commons.math.distribution.NormalDistributionTest)
testGetMean(org.apache.commons.math.distribution.NormalDistributionTest)
testSetMean(org.apache.commons.math.distribution.NormalDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.NormalDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.NormalDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.NormalDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.NormalDistributionTest)
testConsistency(org.apache.commons.math.distribution.NormalDistributionTest)
testDegenerate0(org.apache.commons.math.distribution.PascalDistributionTest)
testDegenerate1(org.apache.commons.math.distribution.PascalDistributionTest)
testDensities(org.apache.commons.math.distribution.PascalDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.PascalDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.PascalDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.PascalDistributionTest)
testDegenerateInverseCumulativeProbability(org.apache.commons.math.distribution.PoissonDistributionTest)
testMean(org.apache.commons.math.distribution.PoissonDistributionTest)
testLargeMeanInverseCumulativeProbability(org.apache.commons.math.distribution.PoissonDistributionTest)
testNormalApproximateProbability(org.apache.commons.math.distribution.PoissonDistributionTest)
testLargeMeanCumulativeProbability(org.apache.commons.math.distribution.PoissonDistributionTest)
testDensities(org.apache.commons.math.distribution.PoissonDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.PoissonDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.PoissonDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.PoissonDistributionTest)
testCumulativeProbabilityAgaintStackOverflow(org.apache.commons.math.distribution.TDistributionTest)
testDfAccessors(org.apache.commons.math.distribution.TDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.TDistributionTest)
testSmallDf(org.apache.commons.math.distribution.TDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.TDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.TDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.TDistributionTest)
testConsistency(org.apache.commons.math.distribution.TDistributionTest)
testAlpha(org.apache.commons.math.distribution.WeibullDistributionTest)
testBeta(org.apache.commons.math.distribution.WeibullDistributionTest)
testSetBeta(org.apache.commons.math.distribution.WeibullDistributionTest)
testInverseCumulativeProbabilityExtremes(org.apache.commons.math.distribution.WeibullDistributionTest)
testSetAlpha(org.apache.commons.math.distribution.WeibullDistributionTest)
testInverseCumulativeProbabilities(org.apache.commons.math.distribution.WeibullDistributionTest)
testCumulativeProbabilities(org.apache.commons.math.distribution.WeibullDistributionTest)
testIllegalArguments(org.apache.commons.math.distribution.WeibullDistributionTest)
testConsistency(org.apache.commons.math.distribution.WeibullDistributionTest)
testNumeratorFormat(org.apache.commons.math.fraction.FractionFormatTest)
testFormatImproperNegative(org.apache.commons.math.fraction.FractionFormatTest)
testFormatImproper(org.apache.commons.math.fraction.FractionFormatTest)
testParseProper(org.apache.commons.math.fraction.FractionFormatTest)
testParseProperNegative(org.apache.commons.math.fraction.FractionFormatTest)
testParse(org.apache.commons.math.fraction.FractionFormatTest)
testWholeFormat(org.apache.commons.math.fraction.FractionFormatTest)
testFormatZero(org.apache.commons.math.fraction.FractionFormatTest)
testFormatNegative(org.apache.commons.math.fraction.FractionFormatTest)
testParseInvalidDenominator(org.apache.commons.math.fraction.FractionFormatTest)
testDenominatorFormat(org.apache.commons.math.fraction.FractionFormatTest)
testParseProperInvalidMinus(org.apache.commons.math.fraction.FractionFormatTest)
testParseInteger(org.apache.commons.math.fraction.FractionFormatTest)
testParseInvalid(org.apache.commons.math.fraction.FractionFormatTest)
testFormat(org.apache.commons.math.fraction.FractionFormatTest)
testParseNegative(org.apache.commons.math.fraction.FractionFormatTest)
testFloatValue(org.apache.commons.math.fraction.FractionTest)
testAbs(org.apache.commons.math.fraction.FractionTest)
testAdd(org.apache.commons.math.fraction.FractionTest)
testSubtract(org.apache.commons.math.fraction.FractionTest)
testReciprocal(org.apache.commons.math.fraction.FractionTest)
testGetReducedFraction(org.apache.commons.math.fraction.FractionTest)
testConstructorDouble(org.apache.commons.math.fraction.FractionTest)
testCompareTo(org.apache.commons.math.fraction.FractionTest)
testLongValue(org.apache.commons.math.fraction.FractionTest)
testIntValue(org.apache.commons.math.fraction.FractionTest)
testDivide(org.apache.commons.math.fraction.FractionTest)
testMultiply(org.apache.commons.math.fraction.FractionTest)
testEqualsAndHashCode(org.apache.commons.math.fraction.FractionTest)
testNegate(org.apache.commons.math.fraction.FractionTest)
testDoubleValue(org.apache.commons.math.fraction.FractionTest)
testConstructor(org.apache.commons.math.fraction.FractionTest)
testOperate(org.apache.commons.math.linear.BigMatrixImplTest)
testAddFail(org.apache.commons.math.linear.BigMatrixImplTest)
testAdd(org.apache.commons.math.linear.BigMatrixImplTest)
testScalarAdd(org.apache.commons.math.linear.BigMatrixImplTest)
testSolve(org.apache.commons.math.linear.BigMatrixImplTest)
testTrace(org.apache.commons.math.linear.BigMatrixImplTest)
testNorm(org.apache.commons.math.linear.BigMatrixImplTest)
testToString(org.apache.commons.math.linear.BigMatrixImplTest)
testIsSingular(org.apache.commons.math.linear.BigMatrixImplTest)
testConstructors(org.apache.commons.math.linear.BigMatrixImplTest)
testPlusMinus(org.apache.commons.math.linear.BigMatrixImplTest)
testDeterminant(org.apache.commons.math.linear.BigMatrixImplTest)
testMultiply2(org.apache.commons.math.linear.BigMatrixImplTest)
testDimensions(org.apache.commons.math.linear.BigMatrixImplTest)
testSubMatrix(org.apache.commons.math.linear.BigMatrixImplTest)
testPremultiplyVector(org.apache.commons.math.linear.BigMatrixImplTest)
testCopyFunctions(org.apache.commons.math.linear.BigMatrixImplTest)
testLUDecomposition(org.apache.commons.math.linear.BigMatrixImplTest)
testGetVectors(org.apache.commons.math.linear.BigMatrixImplTest)
testGetColumnMatrix(org.apache.commons.math.linear.BigMatrixImplTest)
testMultiply(org.apache.commons.math.linear.BigMatrixImplTest)
testEqualsAndHashCode(org.apache.commons.math.linear.BigMatrixImplTest)
testInverse(org.apache.commons.math.linear.BigMatrixImplTest)
testTranspose(org.apache.commons.math.linear.BigMatrixImplTest)
testPremultiply(org.apache.commons.math.linear.BigMatrixImplTest)
testGetRowMatrix(org.apache.commons.math.linear.BigMatrixImplTest)
testSetSubMatrix(org.apache.commons.math.linear.BigMatrixImplTest)
testConstructorMessage(org.apache.commons.math.linear.InvalidMatrixExceptionTest)
testConstructor(org.apache.commons.math.linear.InvalidMatrixExceptionTest)
testConstructorMessage(org.apache.commons.math.linear.MatrixIndexExceptionTest)
testConstructor(org.apache.commons.math.linear.MatrixIndexExceptionTest)
testCreateRealMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateRowRealMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateBigMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateColumnBigMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateBigIdentityMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateRowBigMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateIdentityMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testCreateColumnRealMatrix(org.apache.commons.math.linear.MatrixUtilsTest)
testAEqualQR(org.apache.commons.math.linear.QRDecompositionImplTest)
testDimensions(org.apache.commons.math.linear.QRDecompositionImplTest)
testRUpperTriangular(org.apache.commons.math.linear.QRDecompositionImplTest)
testQOrthogonal(org.apache.commons.math.linear.QRDecompositionImplTest)
testOperate(org.apache.commons.math.linear.RealMatrixImplTest)
testExamples(org.apache.commons.math.linear.RealMatrixImplTest)
testGetEntry(org.apache.commons.math.linear.RealMatrixImplTest)
testAddFail(org.apache.commons.math.linear.RealMatrixImplTest)
testAdd(org.apache.commons.math.linear.RealMatrixImplTest)
testScalarAdd(org.apache.commons.math.linear.RealMatrixImplTest)
testSolve(org.apache.commons.math.linear.RealMatrixImplTest)
testTrace(org.apache.commons.math.linear.RealMatrixImplTest)
testNorm(org.apache.commons.math.linear.RealMatrixImplTest)
testToString(org.apache.commons.math.linear.RealMatrixImplTest)
testIsSingular(org.apache.commons.math.linear.RealMatrixImplTest)
testPlusMinus(org.apache.commons.math.linear.RealMatrixImplTest)
testDeterminant(org.apache.commons.math.linear.RealMatrixImplTest)
testMultiply2(org.apache.commons.math.linear.RealMatrixImplTest)
testDimensions(org.apache.commons.math.linear.RealMatrixImplTest)
testSubMatrix(org.apache.commons.math.linear.RealMatrixImplTest)
testPremultiplyVector(org.apache.commons.math.linear.RealMatrixImplTest)
testCopyFunctions(org.apache.commons.math.linear.RealMatrixImplTest)
testLUDecomposition(org.apache.commons.math.linear.RealMatrixImplTest)
testGetVectors(org.apache.commons.math.linear.RealMatrixImplTest)
testGetColumnMatrix(org.apache.commons.math.linear.RealMatrixImplTest)
testMultiply(org.apache.commons.math.linear.RealMatrixImplTest)
testEqualsAndHashCode(org.apache.commons.math.linear.RealMatrixImplTest)
testInverse(org.apache.commons.math.linear.RealMatrixImplTest)
testTranspose(org.apache.commons.math.linear.RealMatrixImplTest)
testPremultiply(org.apache.commons.math.linear.RealMatrixImplTest)
testGetRowMatrix(org.apache.commons.math.linear.RealMatrixImplTest)
testSetSubMatrix(org.apache.commons.math.linear.RealMatrixImplTest)
testRegularizedBetaPositiveNanPositive(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositivePositivePositive(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositivePositiveNegative(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositiveZeroPositive(org.apache.commons.math.special.BetaTest)
testLogBetaPositivePositive(org.apache.commons.math.special.BetaTest)
testLogBetaPositiveNegative(org.apache.commons.math.special.BetaTest)
testLogBetaPositiveNan(org.apache.commons.math.special.BetaTest)
testRegularizedBetaNegativePositivePositive(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositivePositiveNan(org.apache.commons.math.special.BetaTest)
testLogBetaZeroPositive(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositivePositiveZero(org.apache.commons.math.special.BetaTest)
testRegularizedBetaPositiveNegativePositive(org.apache.commons.math.special.BetaTest)
testLogBetaNanPositive(org.apache.commons.math.special.BetaTest)
testLogBetaNegativePositive(org.apache.commons.math.special.BetaTest)
testLogBetaPositiveZero(org.apache.commons.math.special.BetaTest)
testRegularizedBetaZeroPositivePositive(org.apache.commons.math.special.BetaTest)
testRegularizedBetaNanPositivePositive(org.apache.commons.math.special.BetaTest)
testErf1960(org.apache.commons.math.special.ErfTest)
testErf2576(org.apache.commons.math.special.ErfTest)
testErf2807(org.apache.commons.math.special.ErfTest)
testErf3291(org.apache.commons.math.special.ErfTest)
testErf0(org.apache.commons.math.special.ErfTest)
testLogGammaPositive(org.apache.commons.math.special.GammaTest)
testLogGammaNegative(org.apache.commons.math.special.GammaTest)
testRegularizedGammaPositivePositive(org.apache.commons.math.special.GammaTest)
testRegularizedGammaPositiveNegative(org.apache.commons.math.special.GammaTest)
testLogGammaNan(org.apache.commons.math.special.GammaTest)
testRegularizedGammaNanPositive(org.apache.commons.math.special.GammaTest)
testRegularizedGammaZeroPositive(org.apache.commons.math.special.GammaTest)
testLogGammaZero(org.apache.commons.math.special.GammaTest)
testRegularizedGammaNegativePositive(org.apache.commons.math.special.GammaTest)
testRegularizedGammaPositiveNan(org.apache.commons.math.special.GammaTest)
testRegularizedGammaPositiveZero(org.apache.commons.math.special.GammaTest)
testUnivariateImpl(org.apache.commons.math.stat.CertifiedDataTest)
testStoredUnivariateImpl(org.apache.commons.math.stat.CertifiedDataTest)
testEmptyTable(org.apache.commons.math.stat.FrequencyTest)
testAdd(org.apache.commons.math.stat.FrequencyTest)
testPcts(org.apache.commons.math.stat.FrequencyTest)
testToString(org.apache.commons.math.stat.FrequencyTest)
testIntegerValues(org.apache.commons.math.stat.FrequencyTest)
testCounts(org.apache.commons.math.stat.FrequencyTest)
testDifferenceStats(org.apache.commons.math.stat.StatUtilsTest)
testPercentile(org.apache.commons.math.stat.StatUtilsTest)
testArrayIndexConditions(org.apache.commons.math.stat.StatUtilsTest)
testMax(org.apache.commons.math.stat.StatUtilsTest)
testMin(org.apache.commons.math.stat.StatUtilsTest)
testStats(org.apache.commons.math.stat.StatUtilsTest)
testSumSq(org.apache.commons.math.stat.StatUtilsTest)
testMean(org.apache.commons.math.stat.StatUtilsTest)
testN0andN1Conditions(org.apache.commons.math.stat.StatUtilsTest)
testProduct(org.apache.commons.math.stat.StatUtilsTest)
testGeometricMean(org.apache.commons.math.stat.StatUtilsTest)
testVariance(org.apache.commons.math.stat.StatUtilsTest)
testSumLog(org.apache.commons.math.stat.StatUtilsTest)
testCertifiedValues(org.apache.commons.math.stat.data.LewTest)
testCertifiedValues(org.apache.commons.math.stat.data.LotteryTest)
testGetSortedValues(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testProductAndGeometricMean(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testStats(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testN0andN1Conditions(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testPercentiles(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testSkewAndKurtosis(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsImplTest)
testGetSortedValues(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testProductAndGeometricMean(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testSerialization(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testStats(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testToString(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testN0andN1Conditions(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testAddValue(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testWindowing(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testPercentiles(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testWindowSize(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testNewInstanceClassValid(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testNewInstanceClassNull(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testSkewAndKurtosis(org.apache.commons.math.stat.descriptive.DescriptiveStatisticsTest)
testInteraction(org.apache.commons.math.stat.descriptive.InteractionTest)
testProductAndGeometricMean(org.apache.commons.math.stat.descriptive.ListUnivariateImplTest)
testSerialization(org.apache.commons.math.stat.descriptive.ListUnivariateImplTest)
testStats(org.apache.commons.math.stat.descriptive.ListUnivariateImplTest)
testN0andN1Conditions(org.apache.commons.math.stat.descriptive.ListUnivariateImplTest)
testSkewAndKurtosis(org.apache.commons.math.stat.descriptive.ListUnivariateImplTest)
testProductAndGeometricMean(org.apache.commons.math.stat.descriptive.MixedListUnivariateImplTest)
testStats(org.apache.commons.math.stat.descriptive.MixedListUnivariateImplTest)
testN0andN1Conditions(org.apache.commons.math.stat.descriptive.MixedListUnivariateImplTest)
testSkewAndKurtosis(org.apache.commons.math.stat.descriptive.MixedListUnivariateImplTest)
testSerialization(org.apache.commons.math.stat.descriptive.StatisticalSummaryValuesTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.StatisticalSummaryValuesTest)
testProductAndGeometricMean(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testGetSummary(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testSerialization(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testStats(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testNaNContracts(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testN0andN1Conditions(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.SummaryStatisticsImplTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.FirstMomentTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.FourthMomentTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.GeometricMeanTest)
testNaN(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.KurtosisTest)
testSmallSamples(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.MeanTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.SecondMomentTest)
testNaN(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.SkewnessTest)
testNaN(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testPopulation(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.StandardDeviationTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.ThirdMomentTest)
testNaN(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testPopulation(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testSerialization(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testIncrementation(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testConsistency(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testEvaluation(org.apache.commons.math.stat.descriptive.moment.VarianceTest)
testNaNs(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testSerialization(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testIncrementation(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testConsistency(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testEvaluation(org.apache.commons.math.stat.descriptive.rank.MaxTest)
testEvaluation(org.apache.commons.math.stat.descriptive.rank.MedianTest)
testNaNs(org.apache.commons.math.stat.descriptive.rank.MinTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.rank.MinTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.rank.MinTest)
testSerialization(org.apache.commons.math.stat.descriptive.rank.MinTest)
testIncrementation(org.apache.commons.math.stat.descriptive.rank.MinTest)
testConsistency(org.apache.commons.math.stat.descriptive.rank.MinTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.rank.MinTest)
testEvaluation(org.apache.commons.math.stat.descriptive.rank.MinTest)
testPercentile(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testNISTExample(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testSingleton(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testSetQuantile(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
test5(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testHighPercentile(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testNullEmpty(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testEvaluation(org.apache.commons.math.stat.descriptive.rank.PercentileTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testSerialization(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testIncrementation(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testConsistency(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testEvaluation(org.apache.commons.math.stat.descriptive.summary.ProductTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testSerialization(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testIncrementation(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testConsistency(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testEvaluation(org.apache.commons.math.stat.descriptive.summary.SumLogTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testSerialization(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testIncrementation(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testConsistency(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testEvaluation(org.apache.commons.math.stat.descriptive.summary.SumSqTest)
testSpecialValues(org.apache.commons.math.stat.descriptive.summary.SumTest)
testMomentSmallSamples(org.apache.commons.math.stat.descriptive.summary.SumTest)
testSerialization(org.apache.commons.math.stat.descriptive.summary.SumTest)
testIncrementation(org.apache.commons.math.stat.descriptive.summary.SumTest)
testConsistency(org.apache.commons.math.stat.descriptive.summary.SumTest)
testEqualsAndHashCode(org.apache.commons.math.stat.descriptive.summary.SumTest)
testEvaluation(org.apache.commons.math.stat.descriptive.summary.SumTest)
testChiSquareIndependence(org.apache.commons.math.stat.inference.ChiSquareFactoryTest)
testChiSquareZeroCount(org.apache.commons.math.stat.inference.ChiSquareFactoryTest)
testChiSquareLargeTestStatistic(org.apache.commons.math.stat.inference.ChiSquareFactoryTest)
testChiSquare(org.apache.commons.math.stat.inference.ChiSquareFactoryTest)
testChiSquareIndependence(org.apache.commons.math.stat.inference.ChiSquareTestTest)
testChiSquareZeroCount(org.apache.commons.math.stat.inference.ChiSquareTestTest)
testChiSquareLargeTestStatistic(org.apache.commons.math.stat.inference.ChiSquareTestTest)
testChiSquare(org.apache.commons.math.stat.inference.ChiSquareTestTest)
testOneSampleTTest(org.apache.commons.math.stat.inference.TTestFactoryTest)
testSmallSamples(org.apache.commons.math.stat.inference.TTestFactoryTest)
testTwoSampleTHeterscedastic(org.apache.commons.math.stat.inference.TTestFactoryTest)
testOneSampleT(org.apache.commons.math.stat.inference.TTestFactoryTest)
testPaired(org.apache.commons.math.stat.inference.TTestFactoryTest)
testTwoSampleTHomoscedastic(org.apache.commons.math.stat.inference.TTestFactoryTest)
testOneSampleTTest(org.apache.commons.math.stat.inference.TTestTest)
testSmallSamples(org.apache.commons.math.stat.inference.TTestTest)
testTwoSampleTHeterscedastic(org.apache.commons.math.stat.inference.TTestTest)
testOneSampleT(org.apache.commons.math.stat.inference.TTestTest)
testPaired(org.apache.commons.math.stat.inference.TTestTest)
testTwoSampleTHomoscedastic(org.apache.commons.math.stat.inference.TTestTest)
testOneSampleTTest(org.apache.commons.math.stat.inference.TestUtilsTest)
testChiSquareIndependence(org.apache.commons.math.stat.inference.TestUtilsTest)
testChiSquareZeroCount(org.apache.commons.math.stat.inference.TestUtilsTest)
testSmallSamples(org.apache.commons.math.stat.inference.TestUtilsTest)
testTwoSampleTHeterscedastic(org.apache.commons.math.stat.inference.TestUtilsTest)
testChiSquareLargeTestStatistic(org.apache.commons.math.stat.inference.TestUtilsTest)
testChiSquare(org.apache.commons.math.stat.inference.TestUtilsTest)
testOneSampleT(org.apache.commons.math.stat.inference.TestUtilsTest)
testPaired(org.apache.commons.math.stat.inference.TestUtilsTest)
testTwoSampleTHomoscedastic(org.apache.commons.math.stat.inference.TestUtilsTest)
testClear(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testCorr(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testNaNs(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testPerfect(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testSSENonNegative(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testPerfectNegative(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testNorris(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testInference(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testRandom(org.apache.commons.math.stat.regression.SimpleRegressionTest)
testAdHocData(org.apache.commons.math.transform.FastCosineTransformerTest)
testParameters(org.apache.commons.math.transform.FastCosineTransformerTest)
testSinFunction(org.apache.commons.math.transform.FastCosineTransformerTest)
testAdHocData(org.apache.commons.math.transform.FastFourierTransformerTest)
testParameters(org.apache.commons.math.transform.FastFourierTransformerTest)
testSinFunction(org.apache.commons.math.transform.FastFourierTransformerTest)
testAdHocData(org.apache.commons.math.transform.FastSineTransformerTest)
testParameters(org.apache.commons.math.transform.FastSineTransformerTest)
testSinFunction(org.apache.commons.math.transform.FastSineTransformerTest)
testGoldenRation(org.apache.commons.math.util.ContinuedFractionTest)
testTransformDouble(org.apache.commons.math.util.DefaultTransformerTest)
testTransformBigDecimal(org.apache.commons.math.util.DefaultTransformerTest)
testTransformObject(org.apache.commons.math.util.DefaultTransformerTest)
testTransformString(org.apache.commons.math.util.DefaultTransformerTest)
testTransformInteger(org.apache.commons.math.util.DefaultTransformerTest)
testTransformNull(org.apache.commons.math.util.DefaultTransformerTest)
testBinomialCoefficient(org.apache.commons.math.util.MathUtilsTest)
testFactorialFail(org.apache.commons.math.util.MathUtilsTest)
testIndicatorDouble(org.apache.commons.math.util.MathUtilsTest)
testRoundDouble(org.apache.commons.math.util.MathUtilsTest)
testIndicatorInt(org.apache.commons.math.util.MathUtilsTest)
testGcd(org.apache.commons.math.util.MathUtilsTest)
testLcm(org.apache.commons.math.util.MathUtilsTest)
testCosh(org.apache.commons.math.util.MathUtilsTest)
testHash(org.apache.commons.math.util.MathUtilsTest)
testSinh(org.apache.commons.math.util.MathUtilsTest)
testRoundFloat(org.apache.commons.math.util.MathUtilsTest)
testFactorial(org.apache.commons.math.util.MathUtilsTest)
testSubAndCheck(org.apache.commons.math.util.MathUtilsTest)
testBinomialCoefficientFail(org.apache.commons.math.util.MathUtilsTest)
testCoshNaN(org.apache.commons.math.util.MathUtilsTest)
testSubAndCheckErrorMessage(org.apache.commons.math.util.MathUtilsTest)
test0Choose0(org.apache.commons.math.util.MathUtilsTest)
testMulAndCheck(org.apache.commons.math.util.MathUtilsTest)
testSignByte(org.apache.commons.math.util.MathUtilsTest)
testSignLong(org.apache.commons.math.util.MathUtilsTest)
testEquals(org.apache.commons.math.util.MathUtilsTest)
testIndicatorFloat(org.apache.commons.math.util.MathUtilsTest)
testIndicatorShort(org.apache.commons.math.util.MathUtilsTest)
testIndicatorByte(org.apache.commons.math.util.MathUtilsTest)
testIndicatorLong(org.apache.commons.math.util.MathUtilsTest)
testSignDouble(org.apache.commons.math.util.MathUtilsTest)
testAddAndCheck(org.apache.commons.math.util.MathUtilsTest)
testSignInt(org.apache.commons.math.util.MathUtilsTest)
testSinhNaN(org.apache.commons.math.util.MathUtilsTest)
testSignFloat(org.apache.commons.math.util.MathUtilsTest)
testSignShort(org.apache.commons.math.util.MathUtilsTest)
testNextAfter(org.apache.commons.math.util.MathUtilsTest)
testNextAfterSpecialCases(org.apache.commons.math.util.MathUtilsTest)
testAdd1000(org.apache.commons.math.util.ResizableDoubleArrayTest)
testConstructors(org.apache.commons.math.util.ResizableDoubleArrayTest)
testAddElementRolling(org.apache.commons.math.util.ResizableDoubleArrayTest)
testWithInitialCapacityAndExpansionFactor(org.apache.commons.math.util.ResizableDoubleArrayTest)
testSetNumberOfElements(org.apache.commons.math.util.ResizableDoubleArrayTest)
testWithInitialCapacity(org.apache.commons.math.util.ResizableDoubleArrayTest)
testDiscard(org.apache.commons.math.util.ResizableDoubleArrayTest)
testMutators(org.apache.commons.math.util.ResizableDoubleArrayTest)
testSetElementArbitraryExpansion(org.apache.commons.math.util.ResizableDoubleArrayTest)
testGetValues(org.apache.commons.math.util.ResizableDoubleArrayTest)
testMinMax(org.apache.commons.math.util.ResizableDoubleArrayTest)
testClear(org.apache.commons.math.util.TransformerMapTest)
testContainsTransformer(org.apache.commons.math.util.TransformerMapTest)
testTransformers(org.apache.commons.math.util.TransformerMapTest)
testPutTransformer(org.apache.commons.math.util.TransformerMapTest)
testContainsClass(org.apache.commons.math.util.TransformerMapTest)
testClasses(org.apache.commons.math.util.TransformerMapTest)
testRemoveTransformer(org.apache.commons.math.util.TransformerMapTest)
| {
"pile_set_name": "Github"
} |
/***************************************************************************/
/* */
/* ftlcdfil.h */
/* */
/* FreeType API for color filtering of subpixel bitmap glyphs */
/* (specification). */
/* */
/* Copyright 2006-2018 by */
/* David Turner, Robert Wilhelm, and Werner Lemberg. */
/* */
/* This file is part of the FreeType project, and may only be used, */
/* modified, and distributed under the terms of the FreeType project */
/* license, LICENSE.TXT. By continuing to use, modify, or distribute */
/* this file you indicate that you have read the license and */
/* understand and accept it fully. */
/* */
/***************************************************************************/
#ifndef FTLCDFIL_H_
#define FTLCDFIL_H_
#include <ft2build.h>
#include FT_FREETYPE_H
#include FT_PARAMETER_TAGS_H
#ifdef FREETYPE_H
#error "freetype.h of FreeType 1 has been loaded!"
#error "Please fix the directory search order for header files"
#error "so that freetype.h of FreeType 2 is found first."
#endif
FT_BEGIN_HEADER
/***************************************************************************
*
* @section:
* lcd_filtering
*
* @title:
* LCD Filtering
*
* @abstract:
* Reduce color fringes of subpixel-rendered bitmaps.
*
* @description:
* Should you #define FT_CONFIG_OPTION_SUBPIXEL_RENDERING in your
* `ftoption.h', which enables patented ClearType-style rendering,
* the LCD-optimized glyph bitmaps should be filtered to reduce color
* fringes inherent to this technology. The default FreeType LCD
* rendering uses different technology, and API described below,
* although available, does nothing.
*
* ClearType-style LCD rendering exploits the color-striped structure of
* LCD pixels, increasing the available resolution in the direction of
* the stripe (usually horizontal RGB) by a factor of~3. Since these
* subpixels are color pixels, using them unfiltered creates severe
* color fringes. Use the @FT_Library_SetLcdFilter API to specify a
* low-pass filter, which is then applied to subpixel-rendered bitmaps
* generated through @FT_Render_Glyph. The filter sacrifices some of
* the higher resolution to reduce color fringes, making the glyph image
* slightly blurrier. Positional improvements will remain.
*
* A filter should have two properties:
*
* 1) It should be normalized, meaning the sum of the 5~components
* should be 256 (0x100). It is possible to go above or under this
* target sum, however: going under means tossing out contrast, going
* over means invoking clamping and thereby non-linearities that
* increase contrast somewhat at the expense of greater distortion
* and color-fringing. Contrast is better enhanced through stem
* darkening.
*
* 2) It should be color-balanced, meaning a filter `{~a, b, c, b, a~}'
* where a~+ b~=~c. It distributes the computed coverage for one
* subpixel to all subpixels equally, sacrificing some won resolution
* but drastically reducing color-fringing. Positioning improvements
* remain! Note that color-fringing can only really be minimized
* when using a color-balanced filter and alpha-blending the glyph
* onto a surface in linear space; see @FT_Render_Glyph.
*
* Regarding the form, a filter can be a `boxy' filter or a `beveled'
* filter. Boxy filters are sharper but are less forgiving of non-ideal
* gamma curves of a screen (viewing angles!), beveled filters are
* fuzzier but more tolerant.
*
* Examples:
*
* - [0x10 0x40 0x70 0x40 0x10] is beveled and neither balanced nor
* normalized.
*
* - [0x1A 0x33 0x4D 0x33 0x1A] is beveled and balanced but not
* normalized.
*
* - [0x19 0x33 0x66 0x4c 0x19] is beveled and normalized but not
* balanced.
*
* - [0x00 0x4c 0x66 0x4c 0x00] is boxily beveled and normalized but not
* balanced.
*
* - [0x00 0x55 0x56 0x55 0x00] is boxy, normalized, and almost
* balanced.
*
* - [0x08 0x4D 0x56 0x4D 0x08] is beveled, normalized and, almost
* balanced.
*
* The filter affects glyph bitmaps rendered through @FT_Render_Glyph,
* @FT_Load_Glyph, and @FT_Load_Char. It does _not_ affect the output
* of @FT_Outline_Render and @FT_Outline_Get_Bitmap.
*
* If this feature is activated, the dimensions of LCD glyph bitmaps are
* either wider or taller than the dimensions of the corresponding
* outline with regard to the pixel grid. For example, for
* @FT_RENDER_MODE_LCD, the filter adds 3~subpixels to the left, and
* 3~subpixels to the right. The bitmap offset values are adjusted
* accordingly, so clients shouldn't need to modify their layout and
* glyph positioning code when enabling the filter.
*
* It is important to understand that linear alpha blending and gamma
* correction is critical for correctly rendering glyphs onto surfaces
* without artifacts and even more critical when subpixel rendering is
* involved.
*
* Each of the 3~alpha values (subpixels) is independently used to blend
* one color channel. That is, red alpha blends the red channel of the
* text color with the red channel of the background pixel. The
* distribution of density values by the color-balanced filter assumes
* alpha blending is done in linear space; only then color artifacts
* cancel out.
*/
/****************************************************************************
*
* @enum:
* FT_LcdFilter
*
* @description:
* A list of values to identify various types of LCD filters.
*
* @values:
* FT_LCD_FILTER_NONE ::
* Do not perform filtering. When used with subpixel rendering, this
* results in sometimes severe color fringes.
*
* FT_LCD_FILTER_DEFAULT ::
* The default filter reduces color fringes considerably, at the cost
* of a slight blurriness in the output.
*
* It is a beveled, normalized, and color-balanced five-tap filter
* that is more forgiving to screens with non-ideal gamma curves and
* viewing angles. Note that while color-fringing is reduced, it can
* only be minimized by using linear alpha blending and gamma
* correction to render glyphs onto surfaces. The default filter
* weights are [0x08 0x4D 0x56 0x4D 0x08].
*
* FT_LCD_FILTER_LIGHT ::
* The light filter is a variant that is sharper at the cost of
* slightly more color fringes than the default one.
*
* It is a boxy, normalized, and color-balanced three-tap filter that
* is less forgiving to screens with non-ideal gamma curves and
* viewing angles. This filter works best when the rendering system
* uses linear alpha blending and gamma correction to render glyphs
* onto surfaces. The light filter weights are
* [0x00 0x55 0x56 0x55 0x00].
*
* FT_LCD_FILTER_LEGACY ::
* This filter corresponds to the original libXft color filter. It
* provides high contrast output but can exhibit really bad color
* fringes if glyphs are not extremely well hinted to the pixel grid.
* In other words, it only works well if the TrueType bytecode
* interpreter is enabled *and* high-quality hinted fonts are used.
*
* This filter is only provided for comparison purposes, and might be
* disabled or stay unsupported in the future.
*
* FT_LCD_FILTER_LEGACY1 ::
* For historical reasons, the FontConfig library returns a different
* enumeration value for legacy LCD filtering. To make code work that
* (incorrectly) forwards FontConfig's enumeration value to
* @FT_Library_SetLcdFilter without proper mapping, it is thus easiest
* to have another enumeration value, which is completely equal to
* `FT_LCD_FILTER_LEGACY'.
*
* @since:
* 2.3.0 (`FT_LCD_FILTER_LEGACY1' since 2.6.2)
*/
typedef enum FT_LcdFilter_
{
FT_LCD_FILTER_NONE = 0,
FT_LCD_FILTER_DEFAULT = 1,
FT_LCD_FILTER_LIGHT = 2,
FT_LCD_FILTER_LEGACY1 = 3,
FT_LCD_FILTER_LEGACY = 16,
FT_LCD_FILTER_MAX /* do not remove */
} FT_LcdFilter;
/**************************************************************************
*
* @func:
* FT_Library_SetLcdFilter
*
* @description:
* This function is used to apply color filtering to LCD decimated
* bitmaps, like the ones used when calling @FT_Render_Glyph with
* @FT_RENDER_MODE_LCD or @FT_RENDER_MODE_LCD_V.
*
* @input:
* library ::
* A handle to the target library instance.
*
* filter ::
* The filter type.
*
* You can use @FT_LCD_FILTER_NONE here to disable this feature, or
* @FT_LCD_FILTER_DEFAULT to use a default filter that should work
* well on most LCD screens.
*
* @return:
* FreeType error code. 0~means success.
*
* @note:
* This feature is always disabled by default. Clients must make an
* explicit call to this function with a `filter' value other than
* @FT_LCD_FILTER_NONE in order to enable it.
*
* Due to *PATENTS* covering subpixel rendering, this function doesn't
* do anything except returning `FT_Err_Unimplemented_Feature' if the
* configuration macro FT_CONFIG_OPTION_SUBPIXEL_RENDERING is not
* defined in your build of the library, which should correspond to all
* default builds of FreeType.
*
* @since:
* 2.3.0
*/
FT_EXPORT( FT_Error )
FT_Library_SetLcdFilter( FT_Library library,
FT_LcdFilter filter );
/**************************************************************************
*
* @func:
* FT_Library_SetLcdFilterWeights
*
* @description:
* This function can be used to enable LCD filter with custom weights,
* instead of using presets in @FT_Library_SetLcdFilter.
*
* @input:
* library ::
* A handle to the target library instance.
*
* weights ::
* A pointer to an array; the function copies the first five bytes and
* uses them to specify the filter weights.
*
* @return:
* FreeType error code. 0~means success.
*
* @note:
* Due to *PATENTS* covering subpixel rendering, this function doesn't
* do anything except returning `FT_Err_Unimplemented_Feature' if the
* configuration macro FT_CONFIG_OPTION_SUBPIXEL_RENDERING is not
* defined in your build of the library, which should correspond to all
* default builds of FreeType.
*
* LCD filter weights can also be set per face using @FT_Face_Properties
* with @FT_PARAM_TAG_LCD_FILTER_WEIGHTS.
*
* @since:
* 2.4.0
*/
FT_EXPORT( FT_Error )
FT_Library_SetLcdFilterWeights( FT_Library library,
unsigned char *weights );
/*
* @type:
* FT_LcdFiveTapFilter
*
* @description:
* A typedef for passing the five LCD filter weights to
* @FT_Face_Properties within an @FT_Parameter structure.
*
* @since:
* 2.8
*
*/
#define FT_LCD_FILTER_FIVE_TAPS 5
typedef FT_Byte FT_LcdFiveTapFilter[FT_LCD_FILTER_FIVE_TAPS];
/* */
FT_END_HEADER
#endif /* FTLCDFIL_H_ */
/* END */
| {
"pile_set_name": "Github"
} |
<?php
$lang['L_NOFTPPOSSIBLE']="Es stehen keine FTP-Funktionen zur Verfügung!";
$lang['L_INFO_LOCATION']="Du befindest dich auf ";
$lang['L_INFO_DATABASES']="Folgende Datenbank(en) befinden sich auf dem MySql-Server:";
$lang['L_INFO_NODB']="Datenbank existiert nicht";
$lang['L_INFO_DBDETAIL']="Detail-Information von Datenbank ";
$lang['L_INFO_DBEMPTY']="Die Datenbank ist leer!";
$lang['L_INFO_RECORDS']="Datensätze";
$lang['L_INFO_SIZE']="Größe";
$lang['L_INFO_LASTUPDATE']="letztes Update";
$lang['L_INFO_SUM']="insgesamt";
$lang['L_INFO_OPTIMIZED']="optimiert";
$lang['L_OPTIMIZE_DATABASES']="Tabellen optimieren";
$lang['L_CHECK_TABLES']="Tabellen überprüfen";
$lang['L_CLEAR_DATABASE']="Datenbank leeren";
$lang['L_DELETE_DATABASE']="Datenbank löschen";
$lang['L_INFO_CLEARED']="wurde geleert";
$lang['L_INFO_DELETED']="wurde gelöscht";
$lang['L_INFO_EMPTYDB1']="Soll die Datenbank";
$lang['L_INFO_EMPTYDB2']=" wirklich geleert werden? (Achtung! Alle Daten gehen unwiderruflich verloren)";
$lang['L_INFO_KILLDB']=" wirklich gelöscht werden? (Achtung! Alle Daten gehen unwiderruflich verloren)";
$lang['L_PROCESSKILL1']="Es wird versucht, Prozess ";
$lang['L_PROCESSKILL2']="zu beenden.";
$lang['L_PROCESSKILL3']="Es wird seit ";
$lang['L_PROCESSKILL4']=" Sekunde(n) versucht, Prozess ";
$lang['L_HTACC_CREATE']="Verzeichnisschutz erstellen";
$lang['L_ENCRYPTION_TYPE']="Verschlüsselungsart";
$lang['L_HTACC_CRYPT']="Crypt maximal 8 Zeichen (Linux und Unix-Systeme)";
$lang['L_HTACC_MD5']="MD5 (Linux und Unix-Systeme)";
$lang['L_HTACC_NO_ENCRYPTION']="unverschlüsselt (Windows)";
$lang['L_HTACCESS8']="Es besteht bereits ein Verzeichnisschutz. Wenn Du einen neuen erstellst, wird der alte überschrieben!";
$lang['L_HTACC_NO_USERNAME']="Du musst einen Namen eingeben!";
$lang['L_PASSWORDS_UNEQUAL']="Die Passwörter sind nicht identisch oder leer!";
$lang['L_HTACC_CONFIRM_DELETE']="Soll der Verzeichnisschutz jetzt erstellt werden?";
$lang['L_HTACC_CREATED']="Der Verzeichnisschutz wurde erstellt.";
$lang['L_HTACC_CONTENT']="Inhalt der Datei";
$lang['L_HTACC_CREATE_ERROR']="Es ist ein Fehler bei der Erstellung des Verzeichnisschutzes aufgetreten!<br>Bitte erzeuge die Dateien manuell mit folgendem Inhalt";
$lang['L_HTACC_PROPOSED']="Dringend empfohlen";
$lang['L_HTACC_EDIT']=".htaccess editieren";
$lang['L_HTACCESS18']=".htaccess erstellen in ";
$lang['L_HTACCESS19']="Neu laden ";
$lang['L_HTACCESS20']="Skript ausführen";
$lang['L_HTACCESS21']="Handler zufügen";
$lang['L_HTACCESS22']="Ausführbar machen";
$lang['L_HTACCESS23']="Verzeichnis-Listing";
$lang['L_HTACCESS24']="Error-Dokument";
$lang['L_HTACCESS25']="Rewrite aktivieren";
$lang['L_HTACCESS26']="Deny / Allow";
$lang['L_HTACCESS27']="Redirect";
$lang['L_HTACCESS28']="Error-Log";
$lang['L_HTACCESS29']="weitere Beispiele und Dokumentation";
$lang['L_HTACCESS30']="Provider";
$lang['L_HTACCESS31']="allgemein";
$lang['L_HTACCESS32']="Achtung! Die .htaccess hat eine direkte Auswirkung auf den Browser.<br>Bei falscher Anwendung sind die Seiten nicht mehr erreichbar.";
$lang['L_PHPBUG']="Bug in zlib! Keine Kompression möglich!";
$lang['L_DISABLEDFUNCTIONS']="Abgeschaltete Funktionen";
$lang['L_NOGZPOSSIBLE']="Da zlib nicht installiert ist, stehen keine GZip-Funktionen zur Verfügung!";
$lang['L_DELETE_HTACCESS']="Verzeichnisschutz entfernen (.htaccess löschen)";
$lang['L_WRONG_RIGHTS']="Die Datei oder das Verzeichnis '%s' ist für mich nicht beschreibbar.<br>
Entweder hat sie/es den falschen Besitzer (Owner) oder die falschen Rechte (Chmod).<br>
Bitte setze die richtigen Attribute mit Deinem FTP-Programm. <br>
Die Datei oder das Verzeichnis benötigt die Rechte %s.<br>";
$lang['L_CANT_CREATE_DIR']="Ich konntes das Verzeichnis '%s' nicht erstellen.
Bitte erstelle es mit Deinem FTP-Programm.";
$lang['L_TABLE_TYPE']="Typ";
$lang['L_CHECK']="prüfen";
$lang['L_HTACC_SHA1']="SHA1 (alle Systeme)";
$lang['L_OS']="Betriebssystem";
$lang['L_MSD_VERSION']="MySQLDumper-Version";
$lang['L_MYSQL_VERSION']="MySQL-Version";
$lang['L_PHP_VERSION']="PHP-Version";
$lang['L_MAX_EXECUTION_TIME']="Maximale Ausführungszeit";
$lang['L_PHP_EXTENSIONS']="PHP-Erweiterungen";
$lang['L_MEMORY']="Speicher";
$lang['L_FILE_MISSING']="konnte Datei nicht finden";
?> | {
"pile_set_name": "Github"
} |
/* (No Commment) */
"CFBundleName" = "餐厅";
| {
"pile_set_name": "Github"
} |
## run-all-benchmarks.pkg
# Compiled by:
# src/app/benchmarks/benchmarks.lib
stipulate
package bj = benchmark_junk; # benchmark_junk is from src/app/benchmarks/benchmark-junk.pkg
herein
package run_all_benchmarks {
#
fun run_all_benchmarks ()
=
{
r = ([]: List( bj::Benchmark_Result ));
# Linux times seem accurate to about 4ms
# so I tweak these to run about 400ms each
# to give us times accurate to roughly +-1%:
r = tagged_int_loop::run_benchmark 200000000 ! r; # tagged_int_loop is from src/app/benchmarks/tagged-int-loop.pkg
r = one_word_int_loop::run_benchmark 200000000 ! r; # one_word_int_loop is from src/app/benchmarks/one-word-int-loop.pkg
r = tagged_int_loops::run_benchmark 200 ! r; # tagged_int_loops is from src/app/benchmarks/tagged-int-loops.pkg
r = one_word_int_loops::run_benchmark 200 ! r; # one_word_int_loops is from src/app/benchmarks/one-word-int-loops.pkg
r = tagged_int_loop_with_overflow_trapping::run_benchmark 200000000 ! r; # tagged_int_loop is from src/app/benchmarks/tagged-int-loop.pkg
r = one_word_int_loop_with_overflow_trapping::run_benchmark 200000000 ! r; # one_word_int_loop is from src/app/benchmarks/one-word-int-loop.pkg
r = tagged_int_loops_with_overflow_trapping::run_benchmark 200 ! r; # tagged_int_loops is from src/app/benchmarks/tagged-int-loops.pkg
r = one_word_int_loops_with_overflow_trapping::run_benchmark 200 ! r; # one_word_int_loops is from src/app/benchmarks/one-word-int-loops.pkg
r = tagged_int_shellsort::run_benchmark 15000000 ! r; # tagged_int_shellsort is from src/app/benchmarks/tagged-int-shellsort.pkg
r = tagged_int_shellsort_no_bounds_checking::run_benchmark 15000000 ! r; # tagged_int_shellsort_no_bounds_checking is from src/app/benchmarks/tagged-int-shellsort.pkg
bj::summarize_all_benchmarks (reverse r);
};
my _ =
run_all_benchmarks ();
};
end;
| {
"pile_set_name": "Github"
} |
// Copyright 2016 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build amd64,linux
// +build !gccgo
package unix
import "syscall"
//go:noescape
func gettimeofday(tv *Timeval) (err syscall.Errno)
| {
"pile_set_name": "Github"
} |
{% load static %}
<script src="{% static 'fiber/js/markitup-1.1.10/jquery.markitup.js' %}" type="text/javascript"></script>
<script src="{% static 'fiber/js/markitup-1.1.10/sets/textile_fiber/set.js' %}" type="text/javascript"></script>
<script src="{% static 'fiber/js/fiber.markitup.js' %}" type="text/javascript"></script>
| {
"pile_set_name": "Github"
} |
jsToolBar.strings = {};
jsToolBar.strings['Strong'] = 'Gras';
jsToolBar.strings['Italic'] = 'Italique';
jsToolBar.strings['Underline'] = 'Souligné';
jsToolBar.strings['Deleted'] = 'Rayé';
jsToolBar.strings['Code'] = 'Code en ligne';
jsToolBar.strings['Heading 1'] = 'Titre niveau 1';
jsToolBar.strings['Heading 2'] = 'Titre niveau 2';
jsToolBar.strings['Heading 3'] = 'Titre niveau 3';
jsToolBar.strings['Unordered list'] = 'Liste à puces';
jsToolBar.strings['Ordered list'] = 'Liste numérotée';
jsToolBar.strings['Quote'] = 'Citer';
jsToolBar.strings['Unquote'] = 'Supprimer citation';
jsToolBar.strings['Preformatted text'] = 'Texte préformaté';
jsToolBar.strings['Wiki link'] = 'Lien vers une page Wiki';
jsToolBar.strings['Image'] = 'Image';
| {
"pile_set_name": "Github"
} |
// Copyright (c) 2006 Foundation for Research and Technology-Hellas (Greece).
// All rights reserved.
//
// This file is part of CGAL (www.cgal.org).
// You can redistribute it and/or modify it under the terms of the GNU
// General Public License as published by the Free Software Foundation,
// either version 3 of the License, or (at your option) any later version.
//
// Licensees holding a valid commercial license may use this file in
// accordance with the commercial license agreement provided with the software.
//
// This file is provided AS IS with NO WARRANTY OF ANY KIND, INCLUDING THE
// WARRANTY OF DESIGN, MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE.
//
// $URL$
// $Id$
//
//
// Author(s) : Menelaos Karavelas <[email protected]>
#ifndef CGAL_VORONOI_DIAGRAM_2_VALIDITY_TESTERS_H
#define CGAL_VORONOI_DIAGRAM_2_VALIDITY_TESTERS_H 1
#include <CGAL/license/Voronoi_diagram_2.h>
#include <CGAL/Voronoi_diagram_2/basic.h>
#include <algorithm>
#include <CGAL/Triangulation_utils_2.h>
#include <CGAL/Voronoi_diagram_2/Finder_classes.h>
namespace CGAL {
namespace VoronoiDiagram_2 { namespace Internal {
//=========================================================================
//=========================================================================
template<class VDA, class Base_it>
class Edge_validity_tester
{
// tests whether a halfedge has as face a face with zero area.
private:
const VDA* vda_;
private:
typedef Triangulation_cw_ccw_2 CW_CCW_2;
// Base_it is essentially VDA::Edges_iterator_base
typedef Base_it Edges_iterator_base;
typedef typename VDA::Halfedge_handle Halfedge_handle;
typedef typename VDA::Delaunay_graph::Vertex_handle Delaunay_vertex_handle;
public:
Edge_validity_tester(const VDA* vda = NULL) : vda_(vda) {}
bool operator()(const Edges_iterator_base& eit) const {
CGAL_assertion( !vda_->edge_rejector()(vda_->dual(), eit->dual()) );
int cw_i = CW_CCW_2::cw( eit->dual().second );
CGAL_assertion_code( int ccw_i = CW_CCW_2::ccw( eit->dual().second ); )
CGAL_assertion_code(Delaunay_vertex_handle v_ccw_i = eit->dual().first->vertex(ccw_i);)
CGAL_assertion( !vda_->face_rejector()(vda_->dual(), v_ccw_i) );
Delaunay_vertex_handle v_cw_i = eit->dual().first->vertex(cw_i);
if ( !vda_->face_rejector()(vda_->dual(), v_cw_i) ) {
return false;
}
Halfedge_handle he(eit);
Halfedge_handle he_opp = eit->opposite();
CGAL_assertion( he_opp->opposite() == he );
return he->face()->dual() < he_opp->face()->dual();
}
};
//=========================================================================
//=========================================================================
template<class VDA>
class Vertex_validity_tester
{
private:
const VDA* vda_;
private:
typedef typename VDA::Delaunay_graph::Face_handle Delaunay_face_handle;
typedef typename VDA::Delaunay_graph::Finite_faces_iterator
Delaunay_faces_iterator;
public:
Vertex_validity_tester(const VDA* vda = NULL) : vda_(vda) {}
bool operator()(const Delaunay_faces_iterator& fit) const {
Delaunay_face_handle f(fit);
Delaunay_face_handle fvalid = Find_valid_vertex<VDA>()(vda_,f);
return f != fvalid;
}
};
//=========================================================================
//=========================================================================
} } //namespace VoronoiDiagram_2::Internal
} //namespace CGAL
#endif // CGAL_VORONOI_DIAGRAM_2_VALIDITY_TESTERS_H
| {
"pile_set_name": "Github"
} |
# Copyright (c) 2003-2020, CKSource - Frederico Knabben. All rights reserved.
#
# !!! IMPORTANT !!!
#
# Before you edit this file, please keep in mind that contributing to the project
# translations is possible ONLY via the Transifex online service.
#
# To submit your translations, visit https://www.transifex.com/ckeditor/ckeditor5.
#
# To learn more, check out the official contributor's guide:
# https://ckeditor.com/docs/ckeditor5/latest/framework/guides/contributing/contributing.html
#
msgid ""
msgstr ""
"Language-Team: Lithuanian (https://www.transifex.com/ckeditor/teams/11143/lt/)\n"
"Language: lt\n"
"Plural-Forms: nplurals=4; plural=(n % 10 == 1 && (n % 100 > 19 || n % 100 < 11) ? 0 : (n % 10 >= 2 && n % 10 <=9) && (n % 100 > 19 || n % 100 < 11) ? 1 : n % 1 != 0 ? 2: 3);\n"
msgctxt "Toolbar button tooltip for inserting an image or file via a CKFinder file browser."
msgid "Insert image or file"
msgstr "Įterpti vaizdą ar failą"
msgctxt "Error message displayed when inserting a resized version of an image failed."
msgid "Could not obtain resized image URL."
msgstr "Nepavyko gauti pakeisto dydžio paveiksliuko URL."
msgctxt "Title of a notification displayed when inserting a resized version of an image failed."
msgid "Selecting resized image failed"
msgstr "Nepavyko pasirinkti pakeisto vaizdo"
msgctxt "Error message displayed when an image cannot be inserted at the current position."
msgid "Could not insert image at the current position."
msgstr "Nepavyko įterpti vaizdo į dabartinę vietą."
msgctxt "Title of a notification displayed when an image cannot be inserted at the current position."
msgid "Inserting image failed"
msgstr "Nepavyko įterpti vaizdo"
| {
"pile_set_name": "Github"
} |
1
00:00:32,332 --> 00:00:37,337
♪♪~
2
00:00:37,337 --> 00:00:45,245
♪♪~
3
00:00:45,245 --> 00:00:49,349
(カメラのシャッター音)
4
00:00:49,349 --> 00:00:53,336
(小宮山志保)財布は現金のみ。
カード類 免許証 一切なし。
5
00:00:53,336 --> 00:00:56,373
(村瀬健吾)身元のわかる所持品は
一切なしか…。
6
00:00:56,373 --> 00:00:59,426
名無しの死体ってわけね。
7
00:00:59,426 --> 00:01:01,426
(村瀬)ああ。
8
00:01:03,363 --> 00:01:05,348
(浅輪直樹)
あれ? どうしたんですか?
9
00:01:05,348 --> 00:01:07,417
(加納倫太郎)プルタブ開いてるのに
全然飲んでない。
10
00:01:07,417 --> 00:01:09,417
本当ですね。
11
00:01:10,503 --> 00:01:12,503
どうして飲まなかったんだろう?
12
00:01:13,306 --> 00:01:17,360
(青柳 靖)
昨日 男の声を聞いたんですね?
13
00:01:17,360 --> 00:01:20,380
ええ そうなんです。
夕方6時過ぎに→
14
00:01:20,380 --> 00:01:24,351
犬の散歩に出たんですけど
この下の道に来た時に…。
15
00:01:24,351 --> 00:01:26,353
≪返してくれよ!
16
00:01:26,353 --> 00:01:28,338
(青柳)「返してくれ」か…。
17
00:01:28,338 --> 00:01:31,441
(早瀬川真澄)死因は
頭部を強打した事による脳挫傷。
18
00:01:31,441 --> 00:01:34,311
死亡推定時刻は
昨日の午後6時前後。
19
00:01:34,311 --> 00:01:37,330
ちょうど主婦が
男の声を聞いた時刻に一致するな。
20
00:01:37,330 --> 00:01:39,366
お疲れさまです。
あ お疲れさまです。
21
00:01:39,366 --> 00:01:43,353
現場の缶コーヒーの
分析結果が出ました。
22
00:01:43,353 --> 00:01:46,356
コーヒーの中から 致死量の
スリーパーが検出されました。
23
00:01:46,356 --> 00:01:48,358
ん? スリーパー?
24
00:01:48,358 --> 00:01:51,394
スリーパーは粉末状の合成麻薬。
25
00:01:51,394 --> 00:01:54,347
多量に摂取すると
心拍や血圧が低下して→
26
00:01:54,347 --> 00:01:56,299
眠るように死ねるって言われてる。
27
00:01:56,299 --> 00:01:59,336
最近 北欧経由で入ってきた
新しい麻薬で→
28
00:01:59,336 --> 00:02:02,372
まだ それほど
出回ってないはずなんだけど。
29
00:02:02,372 --> 00:02:05,342
浅輪君
缶コーヒーに付着してた指紋は?
30
00:02:05,342 --> 00:02:07,444
被害者の指紋と一致しました。
31
00:02:07,444 --> 00:02:10,444
あと 気になる事があるんです。
(志保)何?
32
00:02:12,365 --> 00:02:15,368
これと同じような
スリーパー入りの缶コーヒーを飲んで→
33
00:02:15,368 --> 00:02:17,320
死亡した遺体が
すでに3体発見されてます。
34
00:02:17,320 --> 00:02:21,408
えっ!?
所持品は現金入りの財布のみ。
35
00:02:21,408 --> 00:02:23,408
3体とも 身元がわからなかった。
36
00:02:24,344 --> 00:02:27,314
(青柳)けど なんで 3人とも
身元がわかんねえのかな?
37
00:02:27,314 --> 00:02:32,352
そうね。 身なりを見る限り
普通の生活者って感じなのにね。
38
00:02:32,352 --> 00:02:34,337
(矢沢英明)
まず最初に発見されたのが→
39
00:02:34,337 --> 00:02:38,341
Aさん。
発見されたのは4月2日 朝。
40
00:02:38,341 --> 00:02:41,244
死亡推定時刻は
前日の午後6時前後になります。
41
00:02:41,244 --> 00:02:44,364
場所 夕日の丘緑地。
42
00:02:44,364 --> 00:02:46,366
見た目
気持ちよく寝てるようにしか→
43
00:02:46,366 --> 00:02:50,353
見えないですね。
次に発見されたのがBさん。
44
00:02:50,353 --> 00:02:53,323
発見された日が5月11日 朝。
45
00:02:53,323 --> 00:02:57,360
死亡推定時刻
前夜の午後10時前後。
46
00:02:57,360 --> 00:03:00,363
場所 あすか台丘陵 展望広場。
47
00:03:00,363 --> 00:03:03,450
知ってる ここ!
星 すっごいきれいに見えるのよ。
48
00:03:03,450 --> 00:03:06,450
星? 星とか見るのか? 小宮山君。
49
00:03:07,253 --> 00:03:09,272
(志保)悪い?
(村瀬)いや…。
50
00:03:09,272 --> 00:03:11,341
矢沢 次。
51
00:03:11,341 --> 00:03:14,327
で 3番目に発見されたのが
Cさん。
52
00:03:14,327 --> 00:03:17,347
発見されたのが5月28日 朝。
53
00:03:17,347 --> 00:03:21,368
死亡推定時刻
前夜の午後11時前後。
54
00:03:21,368 --> 00:03:24,354
場所 花園自然公園。
55
00:03:24,354 --> 00:03:28,358
あら~ 3人とも
景色のいいとこで死んだのね。
56
00:03:28,358 --> 00:03:30,443
この手だけどさ…。
(志保)手?
57
00:03:30,443 --> 00:03:33,443
うん。
なんか握ってるように見えない?
58
00:03:34,364 --> 00:03:36,366
ああ そう言われたら そうですね。
59
00:03:36,366 --> 00:03:41,354
この手も この手も この手も。
60
00:03:41,354 --> 00:03:44,357
確かに3人とも
何か握ってたように見えますね。
61
00:03:44,357 --> 00:03:47,360
(青柳)う~ん でも
その握ってた何かは→
62
00:03:47,360 --> 00:03:49,396
どこにいったのよ?
いや 少なくともさ→
63
00:03:49,396 --> 00:03:51,348
この3人が死んだ時に→
64
00:03:51,348 --> 00:03:53,366
誰か そばにいた事だけは
確かだよね。
65
00:03:53,366 --> 00:03:55,352
えっ?
なんで そんな事わかるんですか?
66
00:03:55,352 --> 00:03:57,387
だって スリーパーって→
67
00:03:57,387 --> 00:03:59,422
粉末状の合成麻薬って
先生 言ってたじゃない。
68
00:03:59,422 --> 00:04:04,344
だとしたら 1人で缶に入れて
飲んで 死んだんだとしたら→
69
00:04:04,344 --> 00:04:06,363
その包み紙っていうか
パッケージが→
70
00:04:06,363 --> 00:04:08,348
残ってなきゃいけない。
けど 報告書には→
71
00:04:08,348 --> 00:04:12,385
その記載が どこにもない。
つまり 現場には他に誰かいて→
72
00:04:12,385 --> 00:04:14,320
そのスリーパーの
パッケージなりと→
73
00:04:14,320 --> 00:04:16,256
握られてたものを
持ち去ったって事かな。
74
00:04:16,256 --> 00:04:20,360
いやいやいや ちょっと待て。
今回の事件は 犯行時に→
75
00:04:20,360 --> 00:04:22,362
「返してくれ」っていう
男の声が聞かれてる。
76
00:04:22,362 --> 00:04:25,331
もしかして この3人は
犯人に だまされて→
77
00:04:25,331 --> 00:04:27,350
スリーパー入り缶コーヒーを
飲まされ→
78
00:04:27,350 --> 00:04:30,387
手に握っていた何かを奪われた。
79
00:04:30,387 --> 00:04:33,440
ところが今回は
事態に気づいた被害者が→
80
00:04:33,440 --> 00:04:37,343
缶コーヒーを飲まずに抵抗して
殺害された。
81
00:04:37,343 --> 00:04:40,346
この一連のスリーパー関連事件は
連続強盗殺人の可能性がある。
82
00:04:40,346 --> 00:04:43,333
浅輪と係長は その3人を含めた
被害者の身元捜査。
83
00:04:43,333 --> 00:04:45,402
青柳さんたちは
昨日の事件の地取り。
84
00:04:45,402 --> 00:04:47,387
我々は
スリーパーの出どころを洗う。
85
00:04:47,387 --> 00:04:49,387
行くぞ 小宮山君。
(志保)はい!
86
00:04:50,373 --> 00:04:52,342
なんだよ!
87
00:04:52,342 --> 00:04:54,344
なんで あのヒラ刑事が
指示出してんだよ。
88
00:04:54,344 --> 00:04:56,429
あれ? 行かないんですか?
89
00:04:56,429 --> 00:04:58,429
なんすか!?
90
00:04:59,532 --> 00:05:01,532
やる事があるんだぜ。
91
00:05:02,335 --> 00:05:04,354
(青柳)ポチッと。
(矢沢)なんすか? これ。
92
00:05:04,354 --> 00:05:07,357
(青柳)昨日の現場付近の
防犯カメラの映像。
93
00:05:07,357 --> 00:05:10,410
えっ それ
みんなに隠してたんですか?
94
00:05:10,410 --> 00:05:12,295
みんなにって…
いや 誰にも聞かれてないもの。
95
00:05:12,295 --> 00:05:14,364
うわっ もう意味がわからない。
96
00:05:14,364 --> 00:05:18,384
(青柳)あ 被害者みっけ。
(矢沢)あ…。
97
00:05:18,384 --> 00:05:20,353
(青柳)3時50分か。
98
00:05:20,353 --> 00:05:22,338
(矢沢)
死亡推定時刻は6時前後だから→
99
00:05:22,338 --> 00:05:24,457
被害者 2時間あまり
現場にいたって事になりますね。
100
00:05:24,457 --> 00:05:27,457
うん。
2時間 何してたんだろうな?
101
00:05:28,344 --> 00:05:31,414
(青柳)おっ 現場へ向かう女子高生
かわいい。
102
00:05:31,414 --> 00:05:33,466
もう… そんなの関係ないでしょ。
103
00:05:33,466 --> 00:05:36,466
犯行時刻前後見せてくださいよ
早く。
104
00:05:37,454 --> 00:05:40,454
(青柳)このおばちゃん
事情聴取した人。
105
00:05:41,341 --> 00:05:43,409
あっ。
106
00:05:43,409 --> 00:05:45,409
さっきの女子高生…。
107
00:05:50,366 --> 00:05:53,303
(真澄)残念ながら
特に手術の痕もないし→
108
00:05:53,303 --> 00:05:56,389
職業に結びつくような
身体的な特徴もない。
109
00:05:56,389 --> 00:05:58,341
そうですか…。
110
00:05:58,341 --> 00:06:01,361
身元の確認 難航しそうね。
111
00:06:01,361 --> 00:06:06,349
うん…。 自殺として処理された
前の3人も当たってみますよ。
112
00:06:06,349 --> 00:06:09,252
発見された時の服なんかも
保管されてるはずですからね。
113
00:06:09,252 --> 00:06:13,339
先生。 前の3人の右手の写真って
ないですかね?
114
00:06:13,339 --> 00:06:15,325
右手の写真ですか?
115
00:06:15,325 --> 00:06:18,344
ええ。 3人とも
何か握ってたようなんですが→
116
00:06:18,344 --> 00:06:21,431
手のアップの写真があったら
何かわかるかもしれないと思って。
117
00:06:21,431 --> 00:06:23,431
わかりました 探してみます。
118
00:06:24,467 --> 00:06:27,467
あ これだ。 ありました。
119
00:06:29,422 --> 00:06:35,422
これが 最初に発見されたAさんが
着てた服ですね。
120
00:06:36,296 --> 00:06:38,448
なんか 量販店に売ってる
普通の服みたい。
121
00:06:38,448 --> 00:06:41,448
うーん 確かに そうですね。
122
00:06:42,335 --> 00:06:44,304
あ なんだ? これ。
123
00:06:44,304 --> 00:06:46,339
それ
クリーニング屋のタグじゃない?
124
00:06:46,339 --> 00:06:49,325
あ そうですよ。
ああ これ 上着脱いだ時とかに→
125
00:06:49,325 --> 00:06:51,377
ここに
まだ くっついたままになってて→
126
00:06:51,377 --> 00:06:53,329
慌てて引きちぎって
ズボンの中に入れるって→
127
00:06:53,329 --> 00:06:55,448
これ 僕も よくやるんですよ。
128
00:06:55,448 --> 00:06:57,448
あ これがあれば 身元わかるかも。
129
00:06:59,302 --> 00:07:01,304
(志保)あれが江木圭一ね。
(村瀬)ああ。
130
00:07:01,304 --> 00:07:04,374
以前 麻薬の不法所持で
挙げられた事がある。
131
00:07:04,374 --> 00:07:08,361
戸倉組の末端だが
スリーパーの売人という噂だ。
132
00:07:08,361 --> 00:07:10,513
随分 金回りよさそうね。
133
00:07:10,513 --> 00:07:14,513
フッ ひょっとしたら アガリを
ピンハネしてるのかもしれんな。
134
00:07:17,353 --> 00:07:20,356
(志保)ちょっと 持ち物
見せてもらっていいですか?
135
00:07:20,356 --> 00:07:22,342
(江木圭一)ああっ! 離せ!
136
00:07:22,342 --> 00:07:24,394
うわっ! おい てめえ!
137
00:07:24,394 --> 00:07:27,347
オラッ! ああっ…。
大丈夫ですか?
138
00:07:27,347 --> 00:07:30,350
(志保)これ あなたの
セカンドバッグに入っていた→
139
00:07:30,350 --> 00:07:32,335
スリーパー。
140
00:07:32,335 --> 00:07:35,405
あなたが これを売っていた相手を
全員言ってもらうわよ。
141
00:07:35,405 --> 00:07:38,341
(江木)俺は
誰にも売ったりしてませんよ。
142
00:07:38,341 --> 00:07:40,360
嘘をつくな。
本当っすよ。
143
00:07:40,360 --> 00:07:43,313
それは
俺が使うために持ってたんすよ。
144
00:07:43,313 --> 00:07:46,399
それとも そいつを人に売ったって
証拠でもあるんすか?
145
00:07:46,399 --> 00:07:52,338
♪♪~
146
00:07:52,338 --> 00:07:54,440
(志保)この人たちに見覚えは?
147
00:07:54,440 --> 00:07:57,440
(江木)いやぁ 見た事ないっすね。
148
00:07:59,429 --> 00:08:01,429
じゃあ この人は?
149
00:08:02,448 --> 00:08:04,448
知らないなぁ。
150
00:08:08,354 --> 00:08:10,323
(田辺瑞恵)警視庁?
151
00:08:10,323 --> 00:08:13,443
常盤木アズサさんは ごたい…
ございた… ご在宅でしょうか?
152
00:08:13,443 --> 00:08:15,443
ご在宅でしょうか?
153
00:08:16,446 --> 00:08:18,446
(青柳)ご在宅でしょうか?
ああ 今 言える…。
154
00:08:21,367 --> 00:08:23,436
(瑞恵)お嬢様…。
155
00:08:23,436 --> 00:08:26,436
(青柳)ちょっと
話を聞きたいだけなんで。
156
00:08:30,259 --> 00:08:32,345
(矢沢)ご家族の方は?
157
00:08:32,345 --> 00:08:34,347
(常盤木アズサ)
父も母も 今は演奏旅行で→
158
00:08:34,347 --> 00:08:36,316
ヨーロッパを回っています。
159
00:08:36,316 --> 00:08:39,402
(矢沢)ああ ご両親とも
音楽家ですもんね。
160
00:08:39,402 --> 00:08:41,337
(青柳)おい 写真。
(矢沢)はい。
161
00:08:41,337 --> 00:08:44,290
(青柳)こんな写真 ごめんね。
(矢沢)いつの間に…。
162
00:08:44,290 --> 00:08:46,376
(青柳)この人 会った事あるかな?
163
00:08:46,376 --> 00:08:48,411
ありません。
(青柳)じゃあ 昨日の→
164
00:08:48,411 --> 00:08:51,447
午後4時から6時ぐらいまで
どこにいたかな?
165
00:08:51,447 --> 00:08:54,447
答えなければならない理由は
なんですか?
166
00:08:55,251 --> 00:08:57,437
写真。
え?
167
00:08:57,437 --> 00:08:59,437
持ってるじゃないですか。
168
00:09:00,406 --> 00:09:05,445
(青柳)昨日 この階段の先の神社で
人が殺されたんだけど。
169
00:09:05,445 --> 00:09:07,445
ニュースでも言ってたでしょ?
170
00:09:08,464 --> 00:09:11,464
制服で学校を調べたんですね。
171
00:09:15,338 --> 00:09:17,440
(アズサ)学校がわかれば→
172
00:09:17,440 --> 00:09:20,440
生徒の名前と住所は
すぐにわかりますよね。
173
00:09:23,312 --> 00:09:27,300
(矢沢)あのさ 何か
見たり聞いたりした事があれば→
174
00:09:27,300 --> 00:09:29,369
教えて…。
(青柳)神社の方向に行ってから→
175
00:09:29,369 --> 00:09:31,337
戻ってくるまで2時間あまり→
176
00:09:31,337 --> 00:09:33,356
何してたの?
散歩してました。
177
00:09:33,356 --> 00:09:36,359
へえ~ わざわざ あそこまで
電車で行って 散歩したの?
178
00:09:36,359 --> 00:09:38,327
ええ。
179
00:09:38,327 --> 00:09:40,430
おう 写真。
もういいっすよ。
180
00:09:40,430 --> 00:09:43,430
帰りだけ走ってるのは
なんでかな?
181
00:09:45,351 --> 00:09:48,304
気がついたら 思っていたより
時間が経っていたので→
182
00:09:48,304 --> 00:09:50,440
急いだんです。
183
00:09:50,440 --> 00:09:55,440
質問が それだけなら
もう帰ってもらえますか?
184
00:09:57,430 --> 00:09:59,430
はい。
185
00:10:06,439 --> 00:10:08,439
大人なめんなよ。
186
00:10:14,280 --> 00:10:16,349
(店主)このタグ うちのですわ。
本当ですか?
187
00:10:16,349 --> 00:10:19,352
じゃあ このタグから
クリーニングに出した人物って→
188
00:10:19,352 --> 00:10:21,387
特定出来ませんかね?
ああ わかりました。
189
00:10:21,387 --> 00:10:23,356
ちょっと待ってくださいね。
はい お願いします。
190
00:10:23,356 --> 00:10:27,360
ええ… 3の546の…。
191
00:10:27,360 --> 00:10:30,329
ああ ありました。
192
00:10:30,329 --> 00:10:32,381
中谷実さんですね。
193
00:10:32,381 --> 00:10:36,302
(田村)この寮にいた中谷実さんに
間違いないです。
194
00:10:36,302 --> 00:10:39,322
工場の期間工だったんですが
いきなり いなくなっちゃって。
195
00:10:39,322 --> 00:10:42,358
いなくなっちゃったって
いつ頃ですか?
196
00:10:42,358 --> 00:10:45,344
4月の初め頃でしたかね。
197
00:10:45,344 --> 00:10:47,346
その中谷さんと
親しくしてた方って→
198
00:10:47,346 --> 00:10:50,299
どなたか いらっしゃいます?
さあ…。
199
00:10:50,299 --> 00:10:53,269
あまり人と付き合うタイプじゃ
ありませんでしたからね。
200
00:10:53,269 --> 00:10:55,288
部屋 拝見していいですか?
いや ちょっと… すいません!
201
00:10:55,288 --> 00:10:58,391
ちょっと待って。
あ ちょっと待ってください。
202
00:10:58,391 --> 00:11:01,444
もう新しい人が入っちゃってて…。
203
00:11:01,444 --> 00:11:05,348
え じゃあ 中谷さんの荷物って
ここには ないんですか?
204
00:11:05,348 --> 00:11:09,302
中谷さんは両親を亡くしてて
兄弟もないしで…。
205
00:11:09,302 --> 00:11:13,406
仕方ないんで 管理人の私が
処分させてもらいました。
206
00:11:13,406 --> 00:11:15,341
じゃあ 荷物はないって事ですね?
207
00:11:15,341 --> 00:11:18,344
き…
決まってるじゃないですか…。
208
00:11:18,344 --> 00:11:26,319
♪♪~
209
00:11:26,319 --> 00:11:28,321
何 これ? 中谷さんの?
210
00:11:28,321 --> 00:11:30,456
ダメだよ 人のもの
勝手に自分のものにしちゃ。
211
00:11:30,456 --> 00:11:33,456
逮捕するよ。
すみません…。
212
00:11:34,260 --> 00:11:37,280
これが管理人がネコババしてた
パソコンってわけね。
213
00:11:37,280 --> 00:11:39,365
うん。
(村瀬)その男は一体→
214
00:11:39,365 --> 00:11:41,334
人のパソコンで何やってたんだ?
215
00:11:41,334 --> 00:11:44,337
無料のオンラインゲームを
楽しんでたそうです。
216
00:11:44,337 --> 00:11:47,340
悲しいほどケチくせえ奴だな。
217
00:11:47,340 --> 00:11:49,358
コーヒー飲む?
はい。
218
00:11:49,358 --> 00:11:51,360
で ちょっと
これ 見てもらっていいですか?
219
00:11:51,360 --> 00:11:54,430
これ 失踪前の中谷さんが
頻繁に訪れていたサイトです。
220
00:11:54,430 --> 00:11:56,349
(志保)
「Heart&Voice」?
221
00:11:56,349 --> 00:11:59,352
色んな事があって 生きるのが
つらくなった人たちが→
222
00:11:59,352 --> 00:12:01,337
ここに
自分の思いを書き込むんですよ。
223
00:12:01,337 --> 00:12:05,341
(村瀬)それにしても これ
すごい数の書き込みだな。
224
00:12:05,341 --> 00:12:07,260
こんなに大勢 死にたがってんの?
225
00:12:07,260 --> 00:12:11,347
日本の年間自殺者数は
3万人を超えてますからね。
226
00:12:11,347 --> 00:12:13,349
先進国でも
常にトップクラスですよ。
227
00:12:13,349 --> 00:12:15,351
僕のコーヒー。
自分で淹れろ。
228
00:12:15,351 --> 00:12:17,370
えっ…。
このサイトには→
229
00:12:17,370 --> 00:12:19,338
会員専用ルームっていうのが
あるんですね。
230
00:12:19,338 --> 00:12:22,341
これは 自分が置かれている状況を
お互いに話し合う事で→
231
00:12:22,341 --> 00:12:25,294
もう一度
生きる希望を見いだすっていうか→
232
00:12:25,294 --> 00:12:27,346
まあ いわば アメリカ型の→
233
00:12:27,346 --> 00:12:29,348
セラピーサイトみたいな
もんなんですよ。
234
00:12:29,348 --> 00:12:32,451
ちょっと 僕もコーヒー…。
なるほどねぇ。
235
00:12:32,451 --> 00:12:36,451
で その会員専用ルームが これね。
236
00:12:38,257 --> 00:12:40,359
ああ それ
僕もやってみたんですけど→
237
00:12:40,359 --> 00:12:42,345
会員になる手続きが
むちゃくちゃ面倒なんですよ。
238
00:12:42,345 --> 00:12:44,330
メールアドレスとか書いて
入力すれば→
239
00:12:44,330 --> 00:12:47,366
IDが送られてくるんじゃない?
ああ それが違うんですよ。
240
00:12:47,366 --> 00:12:49,252
ここのサイトのログインIDは→
241
00:12:49,252 --> 00:12:52,288
本人限定の受け取り郵便が
送られてくるんですね。
242
00:12:52,288 --> 00:12:55,291
それって 本人が
身分証明書を提示しないと→
243
00:12:55,291 --> 00:12:57,343
受け取れないってやつだよね。
244
00:12:57,343 --> 00:12:59,328
ネットバンクとかで
身分確認のために使ってる。
245
00:12:59,328 --> 00:13:01,330
そうです そうです そうです。
246
00:13:01,330 --> 00:13:03,366
しかし なんで そんな厳重に
チェックする必要があるんだ?
247
00:13:03,366 --> 00:13:06,369
それはですね
身元確認を厳しく行う事で→
248
00:13:06,369 --> 00:13:09,322
相手が なりすましや
冷やかしでない事を保証し→
249
00:13:09,322 --> 00:13:11,324
深い信頼…。
「深い信頼関係の中で→
250
00:13:11,324 --> 00:13:14,343
生きる希望を回復する場を
実現する」
251
00:13:14,343 --> 00:13:17,330
これ 僕 覚えたんですよ。
まあ あの 会員専用っていっても→
252
00:13:17,330 --> 00:13:19,398
みんなハンドルネーム
使ってるんですけどね。
253
00:13:19,398 --> 00:13:21,367
あのさぁ…。
はい?
254
00:13:21,367 --> 00:13:24,420
このハンドルネーム
ジェミニっていう人だけどさ…。
255
00:13:24,420 --> 00:13:26,420
ジェミニ?
256
00:13:27,506 --> 00:13:31,506
本当 頻繁に書き込んでるよね。
257
00:13:32,361 --> 00:13:34,447
本当ですね。
258
00:13:34,447 --> 00:13:38,447
誰の質問にも
懇切丁寧に答えてる。
259
00:13:40,252 --> 00:13:43,289
よし とにかく
一応 そのサイトの管理人を→
260
00:13:43,289 --> 00:13:45,358
サイバー犯罪対策課に
調べてもらおう。
261
00:13:45,358 --> 00:13:47,343
なんで また ヒラ刑事のお前が
仕切ってんだよ?
262
00:13:47,343 --> 00:13:49,395
ヒラ…?
ああ そうそう。 もう一つ→
263
00:13:49,395 --> 00:13:52,365
気になる事があったんですよ。
(青柳)なんだ? ヒラ刑事その4。
264
00:13:52,365 --> 00:13:54,367
えっと… その4?
(青柳)うん。
265
00:13:54,367 --> 00:13:57,353
寮の管理人さんが
中谷さんの部屋を片付けてる時に→
266
00:13:57,353 --> 00:14:00,506
なくなってるものが
あったんですって。
267
00:14:00,506 --> 00:14:03,506
中谷さん 確か 熱帯魚を
飼ってたはずなんですがね。
268
00:14:04,343 --> 00:14:08,297
あ…。
え? なんですか?
269
00:14:08,297 --> 00:14:10,333
熱帯魚… 熱帯魚。
あっ!
270
00:14:10,333 --> 00:14:12,401
え 何やってんの?
いや 主任たちは→
271
00:14:12,401 --> 00:14:14,437
ちょっと用事を思い出したので
出かけてくる。
272
00:14:14,437 --> 00:14:17,437
え? ちょっと…。
273
00:14:19,442 --> 00:14:21,442
(矢沢)あああ…。
(青柳)ごめんなさい!
274
00:14:23,245 --> 00:14:26,449
私 何かした?
ああ いや… ごめんね。
275
00:14:26,449 --> 00:14:29,449
気にしないで。 いつもの事だから。
あ そう。
276
00:14:31,370 --> 00:14:35,324
頼まれてた右手の写真です。
ああ ありがとう。
277
00:14:35,324 --> 00:14:38,444
最近 お疲れなんじゃないですか?
うん ちょっと。
278
00:14:38,444 --> 00:14:41,444
右手 出してください。
279
00:14:47,303 --> 00:14:49,372
元気注入しておきましたんで。
280
00:14:49,372 --> 00:14:51,372
ラブ注入の方がいいんですけど。
281
00:14:55,444 --> 00:14:59,444
それは おいおい。 失礼します。
282
00:15:01,350 --> 00:15:04,303
えーっと?
えーっと えっと えっと…。
283
00:15:04,303 --> 00:15:07,390
なんの話してたっけ?
ああ あれだ…。
284
00:15:07,390 --> 00:15:09,341
中谷さんですよ。
それだ それ。
285
00:15:09,341 --> 00:15:12,361
そうそうそう。 それはそうとね
この中谷実さんが→
286
00:15:12,361 --> 00:15:15,331
このサイトに
頻繁に訪れていたって事は→
287
00:15:15,331 --> 00:15:18,417
やっぱり 彼は自殺だったのかな?
288
00:15:18,417 --> 00:15:22,304
仮に強盗殺人だったとして
中谷さんみたいな人から→
289
00:15:22,304 --> 00:15:24,373
一体 何を奪い取るって
いうんですかね?
290
00:15:24,373 --> 00:15:26,442
(村瀬)「みたいな人」って
失礼だろ お前 それ。
291
00:15:26,442 --> 00:15:28,442
たとえ どん底にいるからって。
292
00:15:29,528 --> 00:15:31,528
(三沢成明)お待たせ致しました。
293
00:15:36,435 --> 00:15:38,435
(三沢)ありがとうございました。
294
00:15:43,309 --> 00:15:46,345
浅輪君 ちょっと見て。
はい?
295
00:15:46,345 --> 00:15:48,347
ん?
ここ→
296
00:15:48,347 --> 00:15:52,451
なんか 固いものを押しつけられた
痕みたいじゃない?
297
00:15:52,451 --> 00:15:56,451
ここも ここも ここも。
298
00:15:57,406 --> 00:16:01,406
本当だ。 なんでしょうね?
299
00:18:25,254 --> 00:18:28,274
4月の初めに
宅配便で届いたんですよね?
300
00:18:28,274 --> 00:18:31,327
(青柳)何便ですか?
ハヤブサ。
301
00:18:31,327 --> 00:18:35,281
(矢沢)4月の初め頃
北芝3丁目の18の→
302
00:18:35,281 --> 00:18:37,333
常盤木アズサさん宛てに→
303
00:18:37,333 --> 00:18:39,335
熱帯魚が
送られてるはずなんですけど。
304
00:18:39,335 --> 00:18:42,338
ああ 送り主は
中谷実さんとなってますね。
305
00:18:42,338 --> 00:18:45,424
4月の2日に配達されてます。
4月2日。
306
00:18:45,424 --> 00:18:47,424
どうもありがとうございました。
どうも。
307
00:18:48,310 --> 00:18:51,263
中谷実は死亡する3日前に→
308
00:18:51,263 --> 00:18:53,299
常盤木アズサに
熱帯魚を送っていると。
309
00:18:53,299 --> 00:18:56,352
これで2人は繋がったな。
310
00:18:56,352 --> 00:18:59,438
でも なんで
熱帯魚だったんですかね?
311
00:18:59,438 --> 00:19:03,438
(青柳)それは…
常盤木アズサを洗えばわかるだろ。
312
00:19:05,311 --> 00:19:08,314
ねえ ちょっと聞いて。
おかしな事になってる。
313
00:19:08,314 --> 00:19:10,299
ん? どうした?
314
00:19:10,299 --> 00:19:13,485
中谷実さんの
死亡手続きをしようとしたら→
315
00:19:13,485 --> 00:19:15,485
中谷さんの住民票が
動かされてるの。
316
00:19:16,322 --> 00:19:18,290
住民票が動かされてる?
317
00:19:18,290 --> 00:19:21,310
しかも 彼が死亡したあとによ。
318
00:19:21,310 --> 00:19:24,380
中谷さんは現在 港区北麻布に
暮らしてる事になってる。
319
00:19:24,380 --> 00:19:27,333
(村瀬)どういう事だ? それ…。
320
00:19:27,333 --> 00:19:41,313
♪♪~
321
00:19:41,313 --> 00:19:44,283
中谷実の部屋に
出入りしている人を見てる人は→
322
00:19:44,283 --> 00:19:46,418
誰もいないわ。
そうか…。
323
00:19:46,418 --> 00:19:49,418
実際は
誰も住んでないんじゃないかしら。
324
00:19:50,322 --> 00:19:53,275
しかし 住所があれば
郵便物が届く。
325
00:19:53,275 --> 00:19:55,411
何? これ。
326
00:19:55,411 --> 00:19:57,411
(村瀬)城西銀行の督促状。
327
00:19:58,230 --> 00:20:00,316
(青柳)田辺さんでしたっけ?
(瑞恵)はい。
328
00:20:00,316 --> 00:20:02,301
(青柳)こちらには いつ頃から?
329
00:20:02,301 --> 00:20:04,336
最初に来たのは→
330
00:20:04,336 --> 00:20:07,406
お二人がお生まれになって
すぐの頃でしたので→
331
00:20:07,406 --> 00:20:09,325
かれこれ16年になります。
332
00:20:09,325 --> 00:20:11,360
お二人?
ええ。
333
00:20:11,360 --> 00:20:17,360
アズミ様という
二卵性の双子の弟さんです。
334
00:20:22,254 --> 00:20:24,273
(矢沢)双子…。
335
00:20:24,273 --> 00:20:26,325
あ。
え? 何?
336
00:20:26,325 --> 00:20:29,294
ジェミニですよ。
例のサイトに熱心に書き込んでた。
337
00:20:29,294 --> 00:20:31,263
ジェミニって
双子座の事なんです。
338
00:20:31,263 --> 00:20:33,399
え そうなの?
ええ。
339
00:20:33,399 --> 00:20:36,268
へえ~。
…え? えっ そのアズミ君は?
340
00:20:36,268 --> 00:20:39,288
今年の1月に亡くなりました。
341
00:20:39,288 --> 00:20:42,424
亡くなったって
ご病気か何かですか?
342
00:20:42,424 --> 00:20:45,424
いえ… 自殺です。
343
00:20:47,396 --> 00:20:49,396
自殺?
344
00:20:50,482 --> 00:20:53,482
右側がアズミ様です。
345
00:20:56,321 --> 00:20:59,258
双子の弟が自殺ですか?
(青柳)うん。
346
00:20:59,258 --> 00:21:01,360
(志保)
ハンドルネーム ジェミニって→
347
00:21:01,360 --> 00:21:03,395
ひょっとしたら
そのアズサさんじゃないの?
348
00:21:03,395 --> 00:21:05,314
彼女 中谷さんとも
知り合いだったし。
349
00:21:05,314 --> 00:21:08,283
(青柳)なあ。
あなたは このような重要人物の→
350
00:21:08,283 --> 00:21:11,353
情報の全てを 今の今まで
我々に隠してたんですか?
351
00:21:11,353 --> 00:21:14,273
いや 隠してたわけじゃない。
ちゃんと報告したじゃないか。
352
00:21:14,273 --> 00:21:17,292
そっちこそ どこ行ってたんだよ?
ああ よくぞ聞いてくれました。
353
00:21:17,292 --> 00:21:20,345
城西銀行に行ってたんですよ。
(青柳)ふーん。
354
00:21:20,345 --> 00:21:25,317
この中谷実は死亡後 城西銀行から
相当額の住宅融資を受けてます。
355
00:21:25,317 --> 00:21:27,252
(青柳)死んでから家建てて
どうすんだよ。
356
00:21:27,252 --> 00:21:29,321
普通 墓だろ。 なあ?
いや お墓だって→
357
00:21:29,321 --> 00:21:31,340
死んだ人には建てられません。
あ そりゃそうだ。
358
00:21:31,340 --> 00:21:34,326
(村瀬)頭の血の巡りの
悪い人たちのために→
359
00:21:34,326 --> 00:21:37,329
順序立てて説明しましょうか。
ふーん。
360
00:21:37,329 --> 00:21:39,314
(2人)浅輪 ちゃんと聞いとけ。
いや 俺じゃないでしょ。
361
00:21:39,314 --> 00:21:44,253
中谷実さんの死後
何者かが彼の住民票を港区へ移動。
362
00:21:44,253 --> 00:21:47,322
そこで中谷実さんの戸籍を元に→
363
00:21:47,322 --> 00:21:50,342
商社役員の中谷実が
作り上げられたわけですよ。
364
00:21:50,342 --> 00:21:52,327
実際の手口としては→
365
00:21:52,327 --> 00:21:57,349
まず 商社で在籍証明と
源泉徴収票を偽造する。
366
00:21:57,349 --> 00:22:01,320
それを港区役所に提出して
課税証明書を手に入れた。
367
00:22:01,320 --> 00:22:04,323
そうして
書類を一切整えたうえで→
368
00:22:04,323 --> 00:22:07,326
中谷実を名乗る別人が銀行に赴き→
369
00:22:07,326 --> 00:22:09,394
多額の融資を引き出したってわけ。
370
00:22:09,394 --> 00:22:12,314
で いざ
返済する段になってみれば→
371
00:22:12,314 --> 00:22:15,317
返済すべき 当の中谷実は
どこにも存在しない。
372
00:22:15,317 --> 00:22:18,320
つまり
中谷実を利用した融資詐欺。
373
00:22:18,320 --> 00:22:21,406
(志保)そういう事。 城西銀行は
なんにも知らないで→
374
00:22:21,406 --> 00:22:25,310
せっせと死んだ人間に
督促状を送っていたわけ。
375
00:22:25,310 --> 00:22:28,280
頭取も真っ青で すぐに
二課に被害届を出すそうよ。
376
00:22:28,280 --> 00:22:30,349
(村瀬のせき払い)
377
00:22:30,349 --> 00:22:32,384
で ここからは
私の推測なんですが→
378
00:22:32,384 --> 00:22:36,321
この4人の被害者を出した
一連のスリーパー事件→
379
00:22:36,321 --> 00:22:38,290
これ そもそもの目的は…。
(青柳)彼らの戸籍を→
380
00:22:38,290 --> 00:22:40,309
手に入れる事が
目的だったんだろうな。
381
00:22:40,309 --> 00:22:42,327
ええ。 それ なんで あなたが今…。
382
00:22:42,327 --> 00:22:44,379
確かに そう考えると 身元が
わからなくなっていた事にも→
383
00:22:44,379 --> 00:22:46,315
合点が…。
合点がいくね。
384
00:22:46,315 --> 00:22:48,267
いきますね 合点がいきますね。
合点がいくね。
385
00:22:48,267 --> 00:22:51,353
遺体の身元が公になれば
詐欺が即座に ばれてしまう。
386
00:22:51,353 --> 00:22:54,406
だから 名無しの遺体にしとく
必要があったってわけ。
387
00:22:54,406 --> 00:22:57,326
でも そうなってくると
常盤木アズサという少女は→
388
00:22:57,326 --> 00:23:00,279
どう この事件に
関わってきたんですかね?
389
00:23:00,279 --> 00:23:03,365
彼女は 中谷実さんと
繋がりがあっただけでなく→
390
00:23:03,365 --> 00:23:06,268
今朝の事件で
被害者が殺された時にも→
391
00:23:06,268 --> 00:23:08,287
現場の近くにいたわけですよね。
392
00:23:08,287 --> 00:23:10,422
これ 偶然とは思えないでしょ。
393
00:23:10,422 --> 00:23:13,422
(電話)
394
00:23:14,343 --> 00:23:16,328
はい 9係。
「サイバー犯罪課です」
395
00:23:16,328 --> 00:23:19,331
「“ハート・アンド・ボイス”の
サイト管理者がわかりました」
396
00:23:19,331 --> 00:23:21,316
「三沢成明 53歳」
397
00:23:21,316 --> 00:23:24,386
「住まいは 港区西赤坂3の5の9」
398
00:23:24,386 --> 00:23:27,256
「メグレスという宝石店の
セールスチーフです」
399
00:23:27,256 --> 00:23:29,408
了解です。
ありがとうございました。
400
00:23:29,408 --> 00:23:31,408
三沢成明か…。
401
00:23:32,494 --> 00:23:34,494
メグレス…?
402
00:23:35,297 --> 00:23:37,299
(志保)メグレスって ほら
あの江木の立ち寄った店よ!
403
00:23:37,299 --> 00:23:39,318
ああっ!
あの江木の→
404
00:23:39,318 --> 00:23:41,353
アドレス帳のリスト!
おっ… ちょっと待て。
405
00:23:41,353 --> 00:23:44,323
あれ? どこにあるんだよ おい!
406
00:23:44,323 --> 00:23:47,326
あった。
え? そっちかよ!
407
00:23:47,326 --> 00:23:50,329
三沢 三沢…。
(村瀬)三沢…。
408
00:23:50,329 --> 00:23:52,447
(村瀬・志保)あっ!
(村瀬)三沢成明…。
409
00:23:52,447 --> 00:23:55,447
(チャイム)
410
00:23:56,318 --> 00:23:58,387
こんばんは。
411
00:23:58,387 --> 00:24:02,387
とりあえず 麻薬の不法所持容疑で
ガサ入れでーす。
412
00:24:05,394 --> 00:24:07,394
はい 失礼しまーす。
413
00:24:09,414 --> 00:24:11,414
おお~。
414
00:24:12,301 --> 00:24:17,339
(志保)うわっ。
えーと… 私 こっち。
415
00:24:17,339 --> 00:24:23,328
♪♪~(オーディオの音楽)
416
00:24:23,328 --> 00:24:35,274
♪♪~
417
00:24:35,274 --> 00:24:37,309
発見。
(村瀬)なんだ?
418
00:24:37,309 --> 00:24:39,278
スリーパーよ。
419
00:24:39,278 --> 00:24:47,269
♪♪~
420
00:24:47,269 --> 00:24:49,304
青柳さん。
あ?
421
00:24:49,304 --> 00:24:51,373
青柳さん!
あ!?
422
00:24:51,373 --> 00:24:53,373
これ…。
423
00:24:58,430 --> 00:25:01,430
「前川俊夫」…。
424
00:25:02,451 --> 00:25:05,451
この写真 今朝の被害者だ。
425
00:25:08,323 --> 00:25:11,259
三沢成明 まずは…。
426
00:25:11,259 --> 00:25:16,415
まずは 麻薬…
麻薬取締法違反で逮捕だ!
427
00:25:16,415 --> 00:25:19,301
だから うるせえから
止めろっつってんだよ!
428
00:25:19,301 --> 00:25:21,286
♪♪~(オーディオの音楽)
429
00:25:21,286 --> 00:25:23,422
♪♪~(大音量の音楽)
アーッ!
430
00:25:23,422 --> 00:25:25,422
それ ボリューム!
431
00:25:27,409 --> 00:25:29,409
何が『死と乙女』だよ。
432
00:25:31,480 --> 00:25:35,480
おい 三沢 昨日の
午後4時から6時 どこにいた?
433
00:25:36,284 --> 00:25:39,237
その時間なら 店にいましたよ。
434
00:25:39,237 --> 00:25:43,358
(村瀬)殺害された前川俊夫さんの
保険証や戸籍謄本を→
435
00:25:43,358 --> 00:25:47,429
なぜ あなたが持っていたのか
説明してもらえますか。
436
00:25:47,429 --> 00:25:52,429
彼の生前
本人から譲り受けました。
437
00:25:53,301 --> 00:25:57,339
そういったものを他人に譲ると
譲った本人は→
438
00:25:57,339 --> 00:26:00,375
その後 生きていくのが
大変 困難になるとは→
439
00:26:00,375 --> 00:26:02,327
考えませんでしたか?
440
00:26:02,327 --> 00:26:05,263
うん… 場合によってはね。
441
00:26:05,263 --> 00:26:08,300
場合によっては…。
442
00:26:08,300 --> 00:26:12,337
あなたの運営するウェブサイト
「ハート・アンド・ボイス」。
443
00:26:12,337 --> 00:26:16,324
あそこには 自殺を考えてる人間が
随分 大勢集まってますね。
444
00:26:16,324 --> 00:26:18,260
しかも 入会するには→
445
00:26:18,260 --> 00:26:21,329
厳重な本人確認が
必要とされている。
446
00:26:21,329 --> 00:26:24,282
セラピーには
信頼関係が必要なんですよ。
447
00:26:24,282 --> 00:26:26,318
あなたは あのサイトを利用して→
448
00:26:26,318 --> 00:26:29,371
ある条件に合う人間を
探してたんじゃないんですか?
449
00:26:29,371 --> 00:26:32,257
「失踪しても 誰も捜さない人間」
450
00:26:32,257 --> 00:26:35,293
「言い換えれば
犯罪に使うには もってこいの→
451
00:26:35,293 --> 00:26:37,329
条件のいい戸籍の持ち主」
452
00:26:37,329 --> 00:26:39,364
「その手の戸籍は
ちょっと手を加えれば→
453
00:26:39,364 --> 00:26:42,334
いくらでも
経歴を塗り替える事が出来る」
454
00:26:42,334 --> 00:26:45,320
「本人が
身元不明で死亡していれば→
455
00:26:45,320 --> 00:26:48,306
本人の口から事が露見する
恐れもない」
456
00:26:48,306 --> 00:26:50,425
(三沢)「とんだ言いがかりですね」
457
00:26:50,425 --> 00:26:54,425
(村瀬)あなたのサイトの
会員だった 中谷実さん。
458
00:26:59,401 --> 00:27:03,401
彼の戸籍は
住宅融資詐欺に利用されてました。
459
00:27:04,339 --> 00:27:06,308
そうですか。
460
00:27:06,308 --> 00:27:08,326
あなた自身は無関係だと?
461
00:27:08,326 --> 00:27:11,363
ええ。 彼の戸籍が…。
462
00:27:11,363 --> 00:27:15,383
「欲しいという人がいたので
差し上げました」
463
00:27:15,383 --> 00:27:18,383
三沢は
戸籍ブローカーってわけですか。
464
00:27:20,338 --> 00:27:23,325
(村瀬)あなたは
条件に合う自殺志願者から→
465
00:27:23,325 --> 00:27:26,328
戸籍を手に入れ
代わりにスリーパーを与えた。
466
00:27:26,328 --> 00:27:29,381
そして その戸籍を売って
利益を得ていたわけだ。
467
00:27:29,381 --> 00:27:32,334
まるで見てきたような
おっしゃりようだ。
468
00:27:32,334 --> 00:27:35,420
ところが その
うまくいっていたビジネスに→
469
00:27:35,420 --> 00:27:37,420
問題が起こった。
470
00:27:38,423 --> 00:27:41,326
(村瀬)前川俊夫さんは
自殺してくれなかった。
471
00:27:41,326 --> 00:27:47,466
人間 誰しも自分の望む死に方で
死ねるとは限りませんねぇ。
472
00:27:47,466 --> 00:27:49,466
≪(ノック)
473
00:27:51,419 --> 00:27:53,419
あ~ チキショー。
474
00:27:56,341 --> 00:27:59,427
三沢のアリバイ
成立しちゃってました。
475
00:27:59,427 --> 00:28:02,427
(矢沢)店の防犯カメラに
映っちゃってました。
476
00:28:04,399 --> 00:28:07,399
無論 私は
誰も殺しちゃいませんよ。
477
00:28:11,373 --> 00:28:19,373
♪♪~
478
00:30:36,301 --> 00:30:44,409
♪♪~
479
00:30:44,409 --> 00:30:48,313
(志保)常盤木アズサという女の子
ご存じですね?
480
00:30:48,313 --> 00:30:52,334
あなたのパソコンに入っていた
会員名簿に→
481
00:30:52,334 --> 00:30:55,320
彼女の名前がありました。
482
00:30:55,320 --> 00:30:59,424
常盤木アズサ
ハンドルネーム ジェミニ。
483
00:30:59,424 --> 00:31:02,424
ええ 知ってますよ。
484
00:31:03,294 --> 00:31:06,314
(志保)
彼女 随分熱心な会員ですね。
485
00:31:06,314 --> 00:31:11,319
(三沢)彼女は
他人の全てを受け入れるんです。
486
00:31:11,319 --> 00:31:16,324
頑張れとも言わず
励ますでもなく→
487
00:31:16,324 --> 00:31:21,379
ただ 共感を示し
相手の話を聞き続ける。
488
00:31:21,379 --> 00:31:25,379
何時間でも どんな相手の話でも。
489
00:31:27,318 --> 00:31:30,321
いささか
常軌を逸した情熱ですが…。
490
00:31:30,321 --> 00:31:32,273
(矢沢)三沢の話は事実です。
491
00:31:32,273 --> 00:31:36,344
彼女 1人終われば また次
って感じで 話を聞いてて→
492
00:31:36,344 --> 00:31:39,297
何日も一睡もしてない事が
あるようです。
493
00:31:39,297 --> 00:31:43,334
彼女は自分の事も顧みずに→
494
00:31:43,334 --> 00:31:47,222
死を望む人の気持ちを
受け入れようとした。
495
00:31:47,222 --> 00:31:52,327
あなた その彼女の気持ちを
利用したんじゃありませんか?
496
00:31:52,327 --> 00:31:55,330
どういう事ですか?
497
00:31:55,330 --> 00:31:58,333
あなたは死を望む人間に
こう持ちかけた。
498
00:31:58,333 --> 00:32:03,405
戸籍を譲る意思があれば
ジェミニがスリーパーを届けると。
499
00:32:03,405 --> 00:32:06,405
彼女はバロック真珠のような子だ。
500
00:32:08,293 --> 00:32:11,396
(三沢)美しいが ゆがんでいる。
501
00:32:11,396 --> 00:32:15,396
彼女は 人の死に
とりつかれているんです。
502
00:32:16,317 --> 00:32:20,321
アズサさんと 亡くなった
彼女の双子の弟 アズミ君の事→
503
00:32:20,321 --> 00:32:22,323
教えて頂けますか?
504
00:32:22,323 --> 00:32:26,294
それが
アズサさんの役に立つんですか?
505
00:32:26,294 --> 00:32:29,264
はい。
(瑞恵)わかりました。
506
00:32:29,264 --> 00:32:32,350
(瑞恵)アズサ様とアズミ様は→
507
00:32:32,350 --> 00:32:35,420
ご両親が 演奏旅行で
ご不在がちでしたので→
508
00:32:35,420 --> 00:32:38,323
お小さい時から
ほとんどの時間を→
509
00:32:38,323 --> 00:32:42,293
お二人っきりで
過ごしていたんです。
510
00:32:42,293 --> 00:32:45,380
まるで 言葉で喋らなくても→
511
00:32:45,380 --> 00:32:48,316
お互いに
気持ちが通じているような→
512
00:32:48,316 --> 00:32:51,319
本当に仲のいい双子でした。
513
00:32:51,319 --> 00:32:59,327
♪♪~
514
00:32:59,327 --> 00:33:03,281
アズミ君って どんな少年でした?
515
00:33:03,281 --> 00:33:06,367
(瑞恵)アズミ様は
とても おとなしい性格で→
516
00:33:06,367 --> 00:33:10,321
ペーパーグライダーを飛ばすのが
好きでした。
517
00:33:10,321 --> 00:33:13,324
アズミ様にとって
しっかりしたアズサ様は→
518
00:33:13,324 --> 00:33:15,393
姉であると同時に→
519
00:33:15,393 --> 00:33:19,393
どこか 母親のような
存在でもあったようです。
520
00:33:21,332 --> 00:33:24,319
アズミ君の自殺の原因は?
521
00:33:24,319 --> 00:33:26,354
(瑞恵)わかりません。
522
00:33:26,354 --> 00:33:33,328
ただ このまま生きていく事に
不安と疑問を持ったらしく→
523
00:33:33,328 --> 00:33:36,331
深く悩んでいらっしゃいました。
524
00:33:36,331 --> 00:33:39,317
そんなアズミ様を アズサ様は→
525
00:33:39,317 --> 00:33:42,220
頑張りなさいと
励まし続けていたんです。
526
00:33:42,220 --> 00:33:46,291
でも その日の昼頃→
527
00:33:46,291 --> 00:33:48,276
ビルの屋上に向かうアズミ様を→
528
00:33:48,276 --> 00:33:51,329
近くに住む子供が
見ていたそうです。
529
00:33:51,329 --> 00:34:01,306
♪♪~
530
00:34:01,306 --> 00:34:06,344
(瑞恵)純粋なアズミ様は
苦しめば苦しむほど→
531
00:34:06,344 --> 00:34:10,331
心のバランスを
崩されていたようです。
532
00:34:10,331 --> 00:34:13,334
そして…。
533
00:34:13,334 --> 00:34:33,404
♪♪~
534
00:34:33,404 --> 00:34:35,404
アズミ…?
535
00:34:41,329 --> 00:34:45,300
アズミが…。
536
00:34:45,300 --> 00:34:47,352
飛んだ…。
537
00:34:47,352 --> 00:35:11,292
♪♪~
538
00:35:11,292 --> 00:35:14,362
(瑞恵)アズミ様を失った
アズサ様は→
539
00:35:14,362 --> 00:35:17,315
まるで
心が壊れてしまったみたいに→
540
00:35:17,315 --> 00:35:20,318
涙を流す事すら出来なかった。
541
00:35:20,318 --> 00:35:31,279
♪♪~
542
00:35:31,279 --> 00:35:35,333
(瑞恵)何か
事件に巻き込まれているのなら→
543
00:35:35,333 --> 00:35:39,454
どうか アズサ様を
助けて差し上げてください。
544
00:35:39,454 --> 00:35:43,454
あの… アズサさんの部屋
拝見出来ますか?
545
00:35:44,392 --> 00:35:46,392
あっ 青柳さん
これ 読んでください!
546
00:35:48,313 --> 00:35:50,315
(青柳)「では ノーバディさん→
547
00:35:50,315 --> 00:35:54,435
午後3時…
アクアリバーテラスに行きます」
548
00:35:54,435 --> 00:35:56,435
彼女 今日も届けに…!
549
00:35:58,323 --> 00:36:00,325
(青柳)三沢! てめえ どれだけ→
550
00:36:00,325 --> 00:36:02,277
あの子を利用すりゃ
気が済むんだよ!
551
00:36:02,277 --> 00:36:04,345
(矢沢)青柳さん!
(村瀬)何やってんだ!? あんた!
552
00:36:04,345 --> 00:36:07,215
こいつ また 彼女に
スリーパー届けに行かせたんだよ。
553
00:36:07,215 --> 00:36:11,319
私は彼女に 何一つ
強制した覚えはありませんよ。
554
00:36:11,319 --> 00:36:14,405
なんだ この野郎…!
(矢沢)青柳さん!
555
00:36:14,405 --> 00:36:17,405
今は彼女を捜す方が先でしょう。
556
00:39:04,292 --> 00:39:06,327
あんた ここで
女の子を待ってるの?
557
00:39:06,327 --> 00:39:09,447
え…?
558
00:39:09,447 --> 00:39:11,447
警察だ。
559
00:39:12,400 --> 00:39:15,386
ジェミニに会ったのか?
560
00:39:15,386 --> 00:39:19,386
僕が来た時
これがベンチに置かれてました。
561
00:39:21,342 --> 00:39:24,345
スリーパーは?
え…?
562
00:39:24,345 --> 00:39:28,316
彼女があんたに届けるはずだった
スリーパーは!?
563
00:39:28,316 --> 00:39:32,303
僕が来た時には メモだけ…。
564
00:39:32,303 --> 00:39:35,323
彼女 スリーパーを持って
どこかに…。
565
00:39:35,323 --> 00:39:37,308
自殺すんなよ。
566
00:39:37,308 --> 00:39:48,419
♪♪~
567
00:39:48,419 --> 00:39:52,419
あれ? 係長 これ…。
568
00:39:58,346 --> 00:40:00,331
(加納の声)「警察の方へ」
569
00:40:00,331 --> 00:40:03,434
「一昨日 神社で
男の人を殺したのは私です」
570
00:40:03,434 --> 00:40:07,434
「罪を償います。 常盤木アズサ」
571
00:40:09,440 --> 00:40:11,440
浅輪です。
572
00:40:14,328 --> 00:40:16,330
わかった。
573
00:40:16,330 --> 00:40:20,384
死なせねえよ。
もしかして 彼女…。
574
00:40:20,384 --> 00:40:28,326
♪♪~
575
00:40:28,326 --> 00:40:31,329
(志保)どこに行ったか
心当たりはないの?
576
00:40:31,329 --> 00:40:34,332
彼女 スリーパーを持ってるのよ!
577
00:40:34,332 --> 00:40:40,304
追い詰められた人間にとって
死は一番の安らぎですよ。
578
00:40:40,304 --> 00:40:45,443
お前 彼女を自殺させるつもりで
スリーパーを…!
579
00:40:45,443 --> 00:40:56,443
♪♪~
580
00:41:07,331 --> 00:41:30,354
♪♪~
581
00:41:30,354 --> 00:41:32,406
おい 矢沢… なんとかしろ。
582
00:41:32,406 --> 00:41:34,406
何 びびってるんですか。
583
00:41:37,328 --> 00:41:40,431
(青柳)どうせ死ぬなら
スリーパーにしたらどうだ!
584
00:41:40,431 --> 00:41:42,431
(矢沢)えっ!?
585
00:41:43,467 --> 00:41:47,467
(青柳)少なくとも あれだ
痛くねえぞ。
586
00:41:51,359 --> 00:41:56,464
最初は そうしようと
思ったんですけど→
587
00:41:56,464 --> 00:41:58,464
それじゃあ ダメなんです。
588
00:42:00,434 --> 00:42:04,434
眠るように死んだんじゃ
罰にならない。
589
00:42:06,290 --> 00:42:08,342
私は人殺しだから。
590
00:42:08,342 --> 00:42:11,412
(青柳)おととい
スリーパーを届けに行った先で→
591
00:42:11,412 --> 00:42:13,412
何があった?
592
00:42:16,334 --> 00:42:20,438
人殺しっつうのは
黙って死んじゃダメなんだよ!
593
00:42:20,438 --> 00:42:23,438
どうせ死ぬんだったら
喋ってから死ね。
594
00:42:31,415 --> 00:42:39,415
私… スリーパーを渡して
あの人の話を聞いたんです。
595
00:42:41,342 --> 00:42:45,396
(アズサの声)
つらかった事や色んな事。
596
00:42:45,396 --> 00:42:48,396
そのあと…。
597
00:42:49,350 --> 00:42:53,337
(前川俊夫)
今日は よしてもいいかな。
598
00:42:53,337 --> 00:42:56,440
なんか 延々
話 聞いてもらったあとで→
599
00:42:56,440 --> 00:42:58,440
悪いんだけど…。
600
00:43:01,312 --> 00:43:07,385
いいえ 全然そんな事ないです。
601
00:43:07,385 --> 00:43:10,354
俺の一生って→
602
00:43:10,354 --> 00:43:15,443
人にだまされっぱなしの
つまらない人生だったけど→
603
00:43:15,443 --> 00:43:21,443
いざ死ぬとなると
やっぱ 迷うよね。
604
00:43:25,336 --> 00:43:31,342
あの… 渡したもの
返してもらえるんだよね?
605
00:43:31,342 --> 00:43:34,245
渡したものって
なんの事ですか?
606
00:43:34,245 --> 00:43:37,264
えっ? 何言ってんの?
607
00:43:37,264 --> 00:43:41,352
戸籍だよ。
戸籍…?
608
00:43:41,352 --> 00:43:45,372
管理人が指定した私書箱に
ちゃんと送ったじゃないか。
609
00:43:45,372 --> 00:43:47,341
届いたから
あんたが来たんだろ?
610
00:43:47,341 --> 00:43:49,343
戸籍情報の類を送ったら→
611
00:43:49,343 --> 00:43:52,346
ジェミニがスリーパーを届ける
って約束じゃないか!
612
00:43:52,346 --> 00:43:55,316
約束って どういう事です?
613
00:43:55,316 --> 00:43:58,335
ふざけるなよ…。
614
00:43:58,335 --> 00:44:02,339
何も知らずに スリーパーだけ
運んできたっていうのか!?
615
00:44:02,339 --> 00:44:06,360
私 本当に
戸籍の事なんて何も…!
616
00:44:06,360 --> 00:44:09,280
いい加減にしろよ。
617
00:44:09,280 --> 00:44:12,333
あんたまで 俺をだますのか!?
618
00:44:12,333 --> 00:44:15,436
返せよ! 返してくれよ!
619
00:44:15,436 --> 00:44:17,436
返せよ…!
620
00:44:21,342 --> 00:44:24,395
うっ… あぁー!
621
00:44:24,395 --> 00:44:28,395
ぐわっ! あぁ…。
622
00:44:32,319 --> 00:44:35,289
あの人 死んでしまった…。
623
00:44:35,289 --> 00:44:38,476
君のせいじゃない!
君は身を守ろうとしただけだ。
624
00:44:38,476 --> 00:44:40,476
なんで こんな事 始めたんだ?
625
00:44:44,348 --> 00:44:47,351
1人で死ぬのは寂しすぎる。
626
00:44:47,351 --> 00:44:50,321
三沢に そう言われたのか?
(アズサ)違う。
627
00:44:50,321 --> 00:44:52,323
私が本当に そう思ったから。
628
00:44:52,323 --> 00:44:55,392
だから こんなふうに
自分を罰してきたのか?
629
00:44:55,392 --> 00:44:58,392
アズミ君が死んでから ずっと。
630
00:45:01,432 --> 00:45:03,432
そうなのか?
631
00:45:10,307 --> 00:45:18,399
私… アズミの気持ちを
わかろうとしなかった。
632
00:45:18,399 --> 00:45:23,437
アズミに
強くなってもらいたくて…。
633
00:45:23,437 --> 00:45:29,437
あの子の苦しみを
理解してあげられなかった。
634
00:45:32,363 --> 00:45:37,434
本当は 頑張れなんて
言っちゃいけなかったんです。
635
00:45:37,434 --> 00:45:43,434
アズミは つらいとも苦しいとも
言わなくなって…。
636
00:45:55,452 --> 00:45:58,452
青柳さんでしたっけ?
637
00:46:00,391 --> 00:46:06,391
あの日 アズミは
お昼頃に ここに来たんです。
638
00:46:08,315 --> 00:46:11,285
それから 日が傾いて→
639
00:46:11,285 --> 00:46:16,407
夕焼けになって
すっかり暗くなるまで→
640
00:46:16,407 --> 00:46:19,410
あの子は ひとりぼっちで→
641
00:46:19,410 --> 00:46:22,410
何時間も ここで空を見てた。
642
00:46:25,416 --> 00:46:32,416
私 あの子を
寂しい気持ちのまま…。
643
00:46:36,443 --> 00:46:39,443
ひとりぼっちで
逝かせてしまったんです。
644
00:46:41,432 --> 00:46:44,432
私がいたのに…。
645
00:46:46,437 --> 00:46:49,437
私がいたのに…!
646
00:46:52,426 --> 00:46:55,426
あの子を
1人で逝かせてしまった…。
647
00:46:56,497 --> 00:46:58,497
ダメだ。
648
00:47:01,452 --> 00:47:04,452
アズミ…。
649
00:47:06,423 --> 00:47:11,423
私も飛んで そばに行くね。
650
00:47:14,431 --> 00:47:18,431
飛ぶ前に教えて!
係長…。
651
00:47:20,337 --> 00:47:23,290
スリーパー飲んで
亡くなった人→
652
00:47:23,290 --> 00:47:27,328
死ぬ前に なんか
持ってたようなんだけど。
653
00:47:27,328 --> 00:47:29,346
(矢沢)今 そこ?
654
00:47:29,346 --> 00:47:33,283
3人とも 右手の甲の この辺に→
655
00:47:33,283 --> 00:47:37,471
硬いものを押しつけたような
痕があった。
656
00:47:37,471 --> 00:47:40,471
君 時計 内側にしてるよね?
657
00:47:45,295 --> 00:47:50,334
彼らが握ってたのは
君の手なんじゃないのかな。
658
00:47:50,334 --> 00:48:07,351
♪♪~
659
00:48:07,351 --> 00:48:09,303
君は 死んでいく人の手を→
660
00:48:09,303 --> 00:48:12,339
握っていてあげたんじゃ
ないのかな?
661
00:48:12,339 --> 00:48:15,409
ひとりぼっちで
寂しい気持ちのまま→
662
00:48:15,409 --> 00:48:18,409
逝かせたくない。
そう思って。
663
00:48:19,329 --> 00:48:24,334
そばに座って
ずっと手を握ってあげてた。
664
00:48:24,334 --> 00:48:28,305
自死を願う人に 君 ずっと
一生懸命 寄り添おうとした。
665
00:48:28,305 --> 00:48:32,326
熱帯魚をかわいがってた
中谷さんからは→
666
00:48:32,326 --> 00:48:34,344
熱帯魚を預かり→
667
00:48:34,344 --> 00:48:37,331
きれいな夕日の中で死にたい
っていう人がいたら→
668
00:48:37,331 --> 00:48:40,434
そういう場所を探してあげて→
669
00:48:40,434 --> 00:48:45,434
最後の最後まで
手を握ってあげてた。
670
00:48:49,326 --> 00:48:54,348
他に 何もしてあげられないから。
671
00:48:54,348 --> 00:48:59,436
違う。 君 たくさんの事をした。
672
00:48:59,436 --> 00:49:03,436
それに 君が
気がついてないだけだ。
673
00:49:04,341 --> 00:49:08,362
いつの間にか あのサイトから
いなくなってた人たち いたよね。
674
00:49:08,362 --> 00:49:10,397
覚えてるかな?
675
00:49:10,397 --> 00:49:15,352
上司から嫌がらせ受けて
死にたいって言ってた男の人。
676
00:49:15,352 --> 00:49:18,338
あの人 ふるさとに帰って→
677
00:49:18,338 --> 00:49:21,358
今 立派に
運送会社で働いてるんだよ。
678
00:49:21,358 --> 00:49:23,410
君にありがとうって言ってた。
679
00:49:23,410 --> 00:49:27,331
嘘!?
嘘じゃない。 これ見て。
680
00:49:27,331 --> 00:49:30,384
三沢のパソコンにあった
あのサイトの会員名簿。
681
00:49:30,384 --> 00:49:33,384
これで連絡とって
確認したんだよ。
682
00:49:34,421 --> 00:49:36,340
長年連れ添った奥さん 亡くして→
683
00:49:36,340 --> 00:49:40,310
1人で生きがいがないって
言ってた人 いたよね。
684
00:49:40,310 --> 00:49:44,448
あの人は
地域のボランティアに参加して→
685
00:49:44,448 --> 00:49:48,448
児童館で
子供たちに遊びを教えてる。
686
00:49:49,286 --> 00:49:53,340
君が ずっと
話を聞いてあげたおかげで→
687
00:49:53,340 --> 00:49:57,494
生き直そうと
歩き始めた人たちがいる。
688
00:49:57,494 --> 00:50:13,494
♪♪~
689
00:50:16,296 --> 00:50:18,432
あっ…。
690
00:50:18,432 --> 00:50:22,432
(泣き声)
691
00:50:24,338 --> 00:50:27,341
大人 怖がらせんなよ。
692
00:50:27,341 --> 00:50:31,345
(泣き声)
(青柳)よしよし…。
693
00:50:31,345 --> 00:50:38,352
(泣き声)
694
00:50:38,352 --> 00:50:43,340
常盤木アズサ 確保。
矢沢が確保。
695
00:50:43,340 --> 00:50:46,426
保護 保護です。
696
00:50:46,426 --> 00:50:55,426
♪♪~
697
00:50:58,438 --> 00:51:03,438
常盤木アズサさん
無事 保護されたわよ。
698
00:51:07,297 --> 00:51:10,417
人を死に追いやるのも 人なら→
699
00:51:10,417 --> 00:51:13,417
人を救えるのも 人なのよ。
700
00:51:16,340 --> 00:51:20,310
彼女が色んな事
証言してくれるだろうな。
701
00:51:20,310 --> 00:51:23,330
さあ あんたにも そろそろ→
702
00:51:23,330 --> 00:51:27,434
洗いざらい
全部 喋ってもらおうか。
703
00:51:27,434 --> 00:51:32,434
まず手始めに
戸籍を誰に いくらで売ったとか。
704
00:51:38,295 --> 00:51:42,349
(アナウンサー)「大手銀行が 相次いで
多額の融資金をだまし取られた→
705
00:51:42,349 --> 00:51:46,403
住宅融資詐欺事件で
警視庁捜査二課は…」
706
00:51:46,403 --> 00:51:49,339
二課に
特大の恩を売ってやったぞ。
707
00:51:49,339 --> 00:51:53,327
ハハハ… まあ 調べてきたのは
私と小宮山君ですがね。
708
00:51:53,327 --> 00:51:57,397
ちっちぇえな~!
誰が調べたとかって…。
709
00:51:57,397 --> 00:51:59,233
なんすか? それ。
自分の手柄って…。
710
00:51:59,233 --> 00:52:02,336
いつも 自分が…!
まあまあ… 2人とも!
711
00:52:02,336 --> 00:52:05,339
より小さく見えますよ。
(村瀬)より小さ…!?
712
00:52:05,339 --> 00:52:08,342
小宮山君 そうめん食ってないで
言ってやれよ 君も。
713
00:52:08,342 --> 00:52:13,347
いや~ 夏のそうめんは最高だね。
ねえ この のど越しが最高!
714
00:52:13,347 --> 00:52:17,284
そうめんってさ
びっくり水がポイントなんだよね。
715
00:52:17,284 --> 00:52:19,353
なんですか? びっくり水って。
716
00:52:19,353 --> 00:52:22,422
あれ? 差し水の事
びっくり水って言わない?
717
00:52:22,422 --> 00:52:25,422
(浅輪・志保)言わないよね~。
| {
"pile_set_name": "Github"
} |
/*
* CDDL HEADER START
*
* The contents of this file are subject to the terms of the
* Common Development and Distribution License, Version 1.0 only
* (the "License"). You may not use this file except in compliance
* with the License.
*
* You can obtain a copy of the license at usr/src/OPENSOLARIS.LICENSE
* or http://www.opensolaris.org/os/licensing.
* See the License for the specific language governing permissions
* and limitations under the License.
*
* When distributing Covered Code, include this CDDL HEADER in each
* file and include the License file at usr/src/OPENSOLARIS.LICENSE.
* If applicable, add the following below this CDDL HEADER, with the
* fields enclosed by brackets "[]" replaced with your own identifying
* information: Portions Copyright [yyyy] [name of copyright owner]
*
* CDDL HEADER END
*/
/*
* Copyright 2008 Sun Microsystems, Inc. All rights reserved.
* Use is subject to license terms.
*/
#ifndef _LIBSPL_LIBDEVINFO_H
#define _LIBSPL_LIBDEVINFO_H
#endif /* _LIBSPL_LIBDEVINFO_H */
| {
"pile_set_name": "Github"
} |
; Joomla! Project
; Copyright (C) 2005 - 2017 Open Source Matters. All rights reserved.
; License GNU General Public License version 2 or later; see LICENSE.txt, see LICENSE.php
; Note : All ini files need to be saved as UTF-8
COM_POSTINSTALL="Post-installation Messages"
COM_POSTINSTALL_BTN_HIDE="Hide this message"
COM_POSTINSTALL_BTN_RESET="Reset Messages"
COM_POSTINSTALL_CONFIGURATION="Post-installation Messages: Options"
COM_POSTINSTALL_LBL_MESSAGES="Post-installation and Upgrade Messages"
COM_POSTINSTALL_LBL_NOMESSAGES_DESC="You have read all the messages."
COM_POSTINSTALL_LBL_NOMESSAGES_TITLE="No Messages"
COM_POSTINSTALL_LBL_RELEASENEWS="Release news <a href="_QQ_"https://www.joomla.org/announcements/release-news.html"_QQ_">from the Joomla! Project</a>"
COM_POSTINSTALL_LBL_SINCEVERSION="Since version %s"
COM_POSTINSTALL_MESSAGES_FOR="Showing messages for"
COM_POSTINSTALL_MESSAGES_TITLE="Post-installation Messages for %s"
COM_POSTINSTALL_XML_DESCRIPTION="Displays post-installation and post-upgrade messages for Joomla and its extensions."
| {
"pile_set_name": "Github"
} |
// Copyright 2008 The Closure Library Authors. All Rights Reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS-IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/**
* @fileoverview An alternative custom button renderer that uses even more CSS
* voodoo than the default implementation to render custom buttons with fake
* rounded corners and dimensionality (via a subtle flat shadow on the bottom
* half of the button) without the use of images.
*
* Based on the Custom Buttons 3.1 visual specification, see
* http://go/custombuttons
*
* @author [email protected] (Emil A Eklund)
* @see ../demos/imagelessbutton.html
*/
goog.provide('goog.ui.ImagelessButtonRenderer');
goog.require('goog.dom.classes');
goog.require('goog.ui.Button');
goog.require('goog.ui.ControlContent');
goog.require('goog.ui.CustomButtonRenderer');
goog.require('goog.ui.INLINE_BLOCK_CLASSNAME');
goog.require('goog.ui.registry');
/**
* Custom renderer for {@link goog.ui.Button}s. Imageless buttons can contain
* almost arbitrary HTML content, will flow like inline elements, but can be
* styled like block-level elements.
*
* @constructor
* @extends {goog.ui.CustomButtonRenderer}
*/
goog.ui.ImagelessButtonRenderer = function() {
goog.ui.CustomButtonRenderer.call(this);
};
goog.inherits(goog.ui.ImagelessButtonRenderer, goog.ui.CustomButtonRenderer);
/**
* The singleton instance of this renderer class.
* @type {goog.ui.ImagelessButtonRenderer?}
* @private
*/
goog.ui.ImagelessButtonRenderer.instance_ = null;
goog.addSingletonGetter(goog.ui.ImagelessButtonRenderer);
/**
* Default CSS class to be applied to the root element of components rendered
* by this renderer.
* @type {string}
*/
goog.ui.ImagelessButtonRenderer.CSS_CLASS =
goog.getCssName('goog-imageless-button');
/**
* Returns the button's contents wrapped in the following DOM structure:
* <div class="goog-inline-block goog-imageless-button">
* <div class="goog-inline-block goog-imageless-button-outer-box">
* <div class="goog-imageless-button-inner-box">
* <div class="goog-imageless-button-pos-box">
* <div class="goog-imageless-button-top-shadow"> </div>
* <div class="goog-imageless-button-content">Contents...</div>
* </div>
* </div>
* </div>
* </div>
* @override
*/
goog.ui.ImagelessButtonRenderer.prototype.createDom;
/** @override */
goog.ui.ImagelessButtonRenderer.prototype.getContentElement = function(
element) {
return /** @type {Element} */ (element && element.firstChild &&
element.firstChild.firstChild &&
element.firstChild.firstChild.firstChild.lastChild);
};
/**
* Takes a text caption or existing DOM structure, and returns the content
* wrapped in a pseudo-rounded-corner box. Creates the following DOM structure:
* <div class="goog-inline-block goog-imageless-button-outer-box">
* <div class="goog-inline-block goog-imageless-button-inner-box">
* <div class="goog-imageless-button-pos">
* <div class="goog-imageless-button-top-shadow"> </div>
* <div class="goog-imageless-button-content">Contents...</div>
* </div>
* </div>
* </div>
* Used by both {@link #createDom} and {@link #decorate}. To be overridden
* by subclasses.
* @param {goog.ui.ControlContent} content Text caption or DOM structure to wrap
* in a box.
* @param {goog.dom.DomHelper} dom DOM helper, used for document interaction.
* @return {Element} Pseudo-rounded-corner box containing the content.
* @override
*/
goog.ui.ImagelessButtonRenderer.prototype.createButton = function(content,
dom) {
var baseClass = this.getCssClass();
var inlineBlock = goog.ui.INLINE_BLOCK_CLASSNAME + ' ';
return dom.createDom('div',
inlineBlock + goog.getCssName(baseClass, 'outer-box'),
dom.createDom('div',
inlineBlock + goog.getCssName(baseClass, 'inner-box'),
dom.createDom('div', goog.getCssName(baseClass, 'pos'),
dom.createDom('div', goog.getCssName(baseClass, 'top-shadow'),
'\u00A0'),
dom.createDom('div', goog.getCssName(baseClass, 'content'),
content))));
};
/**
* Check if the button's element has a box structure.
* @param {goog.ui.Button} button Button instance whose structure is being
* checked.
* @param {Element} element Element of the button.
* @return {boolean} Whether the element has a box structure.
* @protected
* @override
*/
goog.ui.ImagelessButtonRenderer.prototype.hasBoxStructure = function(
button, element) {
var outer = button.getDomHelper().getFirstElementChild(element);
var outerClassName = goog.getCssName(this.getCssClass(), 'outer-box');
if (outer && goog.dom.classes.has(outer, outerClassName)) {
var inner = button.getDomHelper().getFirstElementChild(outer);
var innerClassName = goog.getCssName(this.getCssClass(), 'inner-box');
if (inner && goog.dom.classes.has(inner, innerClassName)) {
var pos = button.getDomHelper().getFirstElementChild(inner);
var posClassName = goog.getCssName(this.getCssClass(), 'pos');
if (pos && goog.dom.classes.has(pos, posClassName)) {
var shadow = button.getDomHelper().getFirstElementChild(pos);
var shadowClassName = goog.getCssName(
this.getCssClass(), 'top-shadow');
if (shadow && goog.dom.classes.has(shadow, shadowClassName)) {
var content = button.getDomHelper().getNextElementSibling(shadow);
var contentClassName = goog.getCssName(
this.getCssClass(), 'content');
if (content && goog.dom.classes.has(content, contentClassName)) {
// We have a proper box structure.
return true;
}
}
}
}
}
return false;
};
/**
* Returns the CSS class to be applied to the root element of components
* rendered using this renderer.
* @return {string} Renderer-specific CSS class.
* @override
*/
goog.ui.ImagelessButtonRenderer.prototype.getCssClass = function() {
return goog.ui.ImagelessButtonRenderer.CSS_CLASS;
};
// Register a decorator factory function for goog.ui.ImagelessButtonRenderer.
goog.ui.registry.setDecoratorByClassName(
goog.ui.ImagelessButtonRenderer.CSS_CLASS,
function() {
return new goog.ui.Button(null,
goog.ui.ImagelessButtonRenderer.getInstance());
});
// Register a decorator factory function for toggle buttons using the
// goog.ui.ImagelessButtonRenderer.
goog.ui.registry.setDecoratorByClassName(
goog.getCssName('goog-imageless-toggle-button'),
function() {
var button = new goog.ui.Button(null,
goog.ui.ImagelessButtonRenderer.getInstance());
button.setSupportedState(goog.ui.Component.State.CHECKED, true);
return button;
});
| {
"pile_set_name": "Github"
} |
//
// LMJCalendarViewController.m
// PLMMPRJK
//
// Created by HuXuPeng on 2017/5/7.
// Copyright © 2017年 GoMePrjk. All rights reserved.
//
#import "LMJCalendarViewController.h"
#import "LMJEventTool.h"
@interface LMJCalendarViewController ()
@end
@implementation LMJCalendarViewController
- (void)viewDidLoad {
[super viewDidLoad];
LMJWeak(self);
LMJEventModel *eventModel = [[LMJEventModel alloc] init];
// @property (nonatomic, strong) NSString *title; //标题
// @property (nonatomic, strong) NSString *location; //地点
//@"yyyy-MM-dd HH:mm"
// @property (nonatomic, strong) NSString *startDateStr; //开始时间
// @property (nonatomic, strong) NSString *endDateStr; //结束时间
// @property (nonatomic, assign) BOOL allDay; //是否全天
// @property (nonatomic, strong) NSString *notes; //备注
// if (alarmStr.length == 0) {
// alarmTime = 0;
// } else if ([alarmStr isEqualToString:@"不提醒"]) {
// alarmTime = 0;
// } else if ([alarmStr isEqualToString:@"1分钟前"]) {
// alarmTime = 60.0 * -1.0f;
// } else if ([alarmStr isEqualToString:@"10分钟前"]) {
// alarmTime = 60.0 * -10.f;
// } else if ([alarmStr isEqualToString:@"30分钟前"]) {
// alarmTime = 60.0 * -30.f;
// } else if ([alarmStr isEqualToString:@"1小时前"]) {
// alarmTime = 60.0 * -60.f;
// } else if ([alarmStr isEqualToString:@"1天前"]) {
// alarmTime = 60.0 * - 60.f * 24;
// @property (nonatomic, strong) NSString *alarmStr; //提醒
eventModel.title = @"eventModel标题";
eventModel.location = @"BeiJing";
eventModel.startDateStr = @"2018-04-05 19:10";
eventModel.endDateStr = @"2018-04-05 20:10";
eventModel.allDay = YES;
eventModel.notes = @"eventModel备注";
eventModel.alarmStr = @"1小时前";
self.addItem([LMJWordItem itemWithTitle:@"事件标题: " subTitle:@"" itemOperation:nil])
.addItem([LMJWordItem itemWithTitle:@"增加日历事件" subTitle: nil itemOperation:^(NSIndexPath *indexPath) {
[[LMJEventTool sharedEventTool] createEventWithEventModel:eventModel];
[weakself.view makeToast:@"增加了"];
}])
.addItem([LMJWordItem itemWithTitle:@"查找" subTitle: nil itemOperation:^(NSIndexPath *indexPath) {
EKEvent *event = [[LMJEventTool sharedEventTool] getEventWithEKEventModel:eventModel];
weakself.sections.firstObject.items.firstObject.subTitle = event.title;
[weakself.tableView reloadRow:0 inSection:0 withRowAnimation:0];
}])
.addItem([LMJWordItem itemWithTitle:@"删除" subTitle:nil itemOperation:^(NSIndexPath *indexPath) {
BOOL isDeleted = [[LMJEventTool sharedEventTool] deleteEvent:eventModel];
if (isDeleted) {
[weakself.view makeToast:@"删除成功"];
weakself.sections.firstObject.items.firstObject.subTitle = nil;
[weakself.tableView reloadRow:0 inSection:0 withRowAnimation:0];
}else {
[weakself.view makeToast:@"删除失败"];
}
}]);
}
#pragma mark - LMJNavUIBaseViewControllerDataSource
/** 导航条左边的按钮 */
- (UIImage *)lmjNavigationBarLeftButtonImage:(UIButton *)leftButton navigationBar:(LMJNavigationBar *)navigationBar
{
[leftButton setImage:[UIImage imageNamed:@"NavgationBar_white_back"] forState:UIControlStateHighlighted];
return [UIImage imageNamed:@"NavgationBar_blue_back"];
}
#pragma mark - LMJNavUIBaseViewControllerDelegate
/** 左边的按钮的点击 */
-(void)leftButtonEvent:(UIButton *)sender navigationBar:(LMJNavigationBar *)navigationBar
{
[self.navigationController popViewControllerAnimated:YES];
}
@end
| {
"pile_set_name": "Github"
} |
using System;
using System.Text;
using System.Collections.Generic;
using System.IO;
using System.Windows.Forms;
using DotSpatial.Positioning;
namespace Diagnostics
{
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
}
} | {
"pile_set_name": "Github"
} |
<!DOCTYPE html>
<!-- this file is auto-generated. DO NOT EDIT.
/*
** Copyright (c) 2012 The Khronos Group Inc.
**
** Permission is hereby granted, free of charge, to any person obtaining a
** copy of this software and/or associated documentation files (the
** "Materials"), to deal in the Materials without restriction, including
** without limitation the rights to use, copy, modify, merge, publish,
** distribute, sublicense, and/or sell copies of the Materials, and to
** permit persons to whom the Materials are furnished to do so, subject to
** the following conditions:
**
** The above copyright notice and this permission notice shall be included
** in all copies or substantial portions of the Materials.
**
** THE MATERIALS ARE PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
** EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
** MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
** IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
** CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
** TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
** MATERIALS OR THE USE OR OTHER DEALINGS IN THE MATERIALS.
*/
-->
<html>
<head>
<meta charset="utf-8">
<title>WebGL GLSL conformance test: operators_001_to_008.html</title>
<link rel="stylesheet" href="../../../../resources/js-test-style.css" />
<link rel="stylesheet" href="../../../resources/ogles-tests.css" />
<script src="../../../../resources/js-test-pre.js"></script>
<script src="../../../resources/webgl-test.js"></script>
<script src="../../../resources/webgl-test-utils.js"></script>
<script src="../../ogles-utils.js"></script>
</head>
<body>
<canvas id="example" width="500" height="500" style="width: 16px; height: 16px;"></canvas>
<div id="description"></div>
<div id="console"></div>
</body>
<script>
"use strict";
OpenGLESTestRunner.run({
"tests": [
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "../default/default.vert",
"fragmentShader": "postfixincrement_frag.frag"
},
"name": "postfixincrement_frag.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "postfixincrement_vert.vert",
"fragmentShader": "../default/default.frag"
},
"name": "postfixincrement_vert.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "../default/default.vert",
"fragmentShader": "postfixdecrement_frag.frag"
},
"name": "postfixdecrement_frag.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "postfixdecrement_vert.vert",
"fragmentShader": "../default/default.frag"
},
"name": "postfixdecrement_vert.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "../default/default.vert",
"fragmentShader": "prefixincrement_frag.frag"
},
"name": "prefixincrement_frag.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "prefixincrement_vert.vert",
"fragmentShader": "../default/default.frag"
},
"name": "prefixincrement_vert.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "../default/default.vert",
"fragmentShader": "prefixdecrement_frag.frag"
},
"name": "prefixdecrement_frag.test.html",
"pattern": "compare"
},
{
"referenceProgram": {
"vertexShader": "../default/default.vert",
"uniforms": {
"result": {
"count": 1,
"type": "uniform4fv",
"value": [
1.0,
1.0,
1.0,
1.0
]
}
},
"fragmentShader": "../default/expected.frag"
},
"model": null,
"testProgram": {
"vertexShader": "prefixdecrement_vert.vert",
"fragmentShader": "../default/default.frag"
},
"name": "prefixdecrement_vert.test.html",
"pattern": "compare"
}
]
});
var successfullyParsed = true;
</script>
</html>
| {
"pile_set_name": "Github"
} |
--[[
文件:classical-ai.lua
主题:经典战术
]]--
--[[
PART 00:常用工具函数
]]--
--判断卡牌的花色是否相符
function MatchSuit(card, suit_table)
if #suit_table > 0 then
local cardsuit = card:getSuit()
for _,suit in pairs(suit_table) do
if cardsuit == suit then
return true
end
end
end
return false
end
--判断卡牌的类型是否相符
function MatchType(card, type_table)
if type(suit_table) == "string" then
type_table = type_table:split("|")
end
if #type_table > 0 then
for _,cardtype in pairs(type_table) do
if card:isKindOf(cardtype) then
return true
end
end
end
return false
end
--[[
PART 01:3V3经典战术
内容:黄金一波流、绝情阵
]]--
--黄金一波流--
--相关信息
sgs.GoldenWaveDetail = {
KurouActor = {}, --苦肉执行者
YijiActor = {}, --遗计执行者
JijiuActor = {}, --急救执行者
EruptSignal = {}, --起爆信号(五谷丰登中,急救执行者得到的红色牌)
}
--判断是否使用黄金一波流
function GoldenWaveStart(self)
local huanggai = self.player
local room = huanggai:getRoom()
sgs.GoldenWaveDetail.EruptSignal = {}
if huanggai:hasSkill("kurou") then
local guojia, huatuo
if #self.friends_noself > 1 then
for _,friend in pairs(self.friends_noself) do
if friend:hasSkill("yiji") then
guojia = friend
elseif friend:hasSkill("jijiu") then
huatuo = friend
else
room:setPlayerMark(friend, "GWF_Forbidden", 1)
end
end
end
if guojia and huatuo then
sgs.GoldenWaveDetail.KurouActor = {huanggai:objectName()}
sgs.GoldenWaveDetail.YijiActor = {guojia:objectName()}
sgs.GoldenWaveDetail.JijiuActor = {huatuo:objectName()}
room:setPlayerMark(huanggai, "GoldenWaveFlow", 1)
room:setPlayerMark(guojia, "GoldenWaveFlow", 1)
room:setPlayerMark(huatuo, "GoldenWaveFlow", 1)
return true
else
sgs.GoldenWaveDetail.KurouActor = {}
sgs.GoldenWaveDetail.YijiActor = {}
sgs.GoldenWaveDetail.JijiuActor = {}
room:setPlayerMark(huanggai, "GWF_Forbidden", 1)
return false
end
end
room:setPlayerMark(huanggai, "GWF_Forbidden", 1)
return false
end
--黄金苦肉
function GWFKurouTurnUse(self)
local huanggai = self.player
local released = sgs.GoldenWaveDetail.EruptSignal["Released"]
if released then
if self.getHp() > 1 then
return sgs.Card_Parse("@KurouCard=.")
end
else
return sgs.Card_Parse("@KurouCard=.")
end
end
--黄金遗计
function GWFYijiAsk(player, card_ids)
local guojia = self.player
local released = sgs.GoldenWaveDetail.EruptSignal["Released"]
local huanggai = sgs.GoldenWaveDetail.KurouActor[1]
local huatuo = sgs.GoldenWaveDetail.JijiuActor[1]
if released then
for _,id in ipairs(card_ids) do
return huanggai, id
end
else
for _,id in ipairs(card_ids) do
local card = sgs.Sanguosha:getCard(id)
if MatchType(card, "Crossbow|AOE|Duel") then
return huanggai, id
elseif card:isRed() and huatuo:isAlive() then
return huatuo, id
else
return huanggai, id
end
end
end
end
--黄金急救
function GWFJijiuSignal(card, player, card_place)
local huatuo = player
if #EruptSignal > 0 then
if card:getId() == EruptSignal[1] then
local cards = player:getCards("he")
for _,id in sgs.qlist(cards) do
if id ~= EruptSignal[1] then
local acard = sgs.Sanguosha:getCard(id)
if acard:isRed() then
return false
end
end
end
sgs.GoldenWaveDetail.EruptSignal["Released"] = card:getId()
end
end
return true
end
--命苦的郭嘉(未完成)
--绝情阵
--相关信息
sgs.RuthlessDetail = {
JianxiongActor = {}, --奸雄执行者
TianxiangActor = {}, --天香执行者
YijiActor = {}, --遗计执行者
}
--判断是否使用绝情阵
function RuthlessStart(self)
local caocao = self.player
local room = caocao:getRoom()
if caocao:hasSkill("jianxiong") then
local xiaoqiao, guojia
if #self.friends_noself > 1 then
for _,friend in pairs(self.friends_noself) do
if friend:hasSkill("yiji") then
guojia = friend
elseif friend:hasSkill("tianxiang") then
xiaoqiao = friend
else
room:setPlayerMark(friend, "RL_Forbidden", 1)
end
end
end
if xiaoqiao and guojia then
sgs.RuthlessDetail.JianxiongActor = {caocao:objectName()}
sgs.RuthlessDetail.TianxiangActor = {xiaoqiao:objectName()}
sgs.RuthlessDetail.YijiActor = {guojia:objectName()}
room:setPlayerMark(caocao, "Ruthless", 1)
room:setPlayerMark(guojia, "Ruthless", 1)
room:setPlayerMark(xiaoqiao, "Ruthless", 1)
return true
else
sgs.RuthlessDetail.JianxiongActor = {}
sgs.RuthlessDetail.TianxiangActor = {}
sgs.RuthlessDetail.YijiActor = {}
room:setPlayerMark(caocao, "RL_Forbidden", 1)
return false
end
end
room:setPlayerMark(caocao, "RL_Forbidden", 1)
return false
end
--绝情天香
function RLTianxiangSkillUse(self, data)
local xiaoqiao = self.player
local caocao = sgs.RuthlessDetail.JianxiongActor[1]
local damage = data
if damage then
local aoe = damage.card
if aoe and aoe:isKindOf("AOE") then
local handcards = self.player:getCards("h")
handcards = sgs.QList2Table(handcards)
self:sortByUseValue(handcards, true)
for _, id in ipairs(handcards) do
local suit = card:getSuit()
if suit == sgs.Card_Heart or (xiaoqiao:hasSkill("hongyan") and suit == sgs.Card_Spade) then
return "@TianxiangCard="..id.."->"..caocao:objectName()
end
end
end
end
end
--绝情遗计
function RLYijiAsk(player, card_ids)
local guojia = self.player
local caocao = sgs.RuthlessDetail.JianxiongActor[1]
local xiaoqiao = sgs.RuthlessDetail.TianxiangActor[1]
for _,id in ipairs(card_ids) do
local card = sgs.Sanguosha:getCard(id)
if MatchType(card, "Crossbow|AOE|Duel|ExNihilo|Peach") then
return caocao, id
else
local hearts = {sgs.Card_Heart, sgs.Card_Spade}
if MatchSuit(card, hearts) and xiaoqiao:isAlive() then
return xiaoqiao, id
else
return caocao, id
end
end
end
end
--[[
PART 02:KOF经典战术
内容:苦肉一波带、控底爆发
]]--
--苦肉一波带--
--判断是否使用苦肉一波带
function KOFKurouStart(self)
local others = self.room:getOtherPlayers(self.player)
if others:length() == 1 then
local enemy = others:first()
if self:hasSkills("fankui|guixin|fenyong|zhichi|jilei", enemy) then
self:speak("不行,这家伙不好对付,慢苦为妙。")
self.room:setPlayerMark(self.player, "KKR_Forbidden", 1)
return false
end
self:speak("看我大苦肉一波带走!")
self.room:setPlayerMark(self.player, "KOFKurouRush", 1)
return true
end
return false
end
--一波苦肉
function KOFKurouTurnUse(self)
local huanggai = self.player
if huanggai:getHp() > 1 then
return sgs.Card_Parse("@KurouCard=.")
end
if self:getCardsNum("Analeptic") + self:getCardsNum("Peach") > 0 then
return sgs.Card_Parse("@KurouCard=.")
end
end
--控底爆发--
--相关信息
sgs.KOFControlType = {} --起爆卡牌的类型
sgs.KOFControlSuit = {} --起爆卡牌的花色
sgs.KOFControlResult = {} --控底结果
sgs.KOFControlDetail = { --爆发详细信息
EruptSkill = {}, --待爆发技能名
MaxInterval = {}, --爆发时可容忍的两起爆卡牌间间隔
ControlFinished = {} --爆发结束标志
}
--判断是否使用控底爆发战术
function KOFControlStart(player)
local room = player:getRoom()
if player:hasSkill("guanxing") or player:hasSkill("super_guanxing") then
local tag = player:getTag("1v1Arrange")
if tag then
local followList = tag:toStringList()
if followList then
if #followList > 0 then
local follow = 1
for _,name in ipairs(followList) do
local general = sgs.Sanguosha:getGeneral(name)
local flag = false
if general:hasSkill("luoshen") then
sgs.KOFControlSuit = {sgs.Card_Spade, sgs.Card_Club}
sgs.KOFControlDetail.EruptSkill = {"luoshen"}
sgs.KOFControlDetail.MaxInterval = {0}
sgs.KOFControlDetail.ControlFinished = {false}
flag = true
elseif general:hasSkill("jizhi") then
sgs.KOFControlType = {"TrickCard"}
sgs.KOFControlDetail.EruptSkill = {"jizhi"}
sgs.KOFControlDetail.MaxInterval = {1}
sgs.KOFControlDetail.ControlFinished = {false}
elseif general:hasSkill("xiaoji") then
sgs.KOFControlType = {"EquipCard"}
sgs.KOFControlDetail.EruptSkill = {"xiaoji"}
sgs.KOFControlDetail.MaxInterval = {2}
sgs.KOFControlDetail.ControlFinished = {false}
elseif general:hasSkill("guhuo") then
sgs.KOFControlSuit = {sgs.Card_Heart}
sgs.KOFControlDetail.EruptSkill = {"guhuo"}
sgs.KOFControlDetail.MaxInterval = {1}
sgs.KOFControlDetail.ControlFinished = {false}
elseif general:hasSkill("caizhaoji_hujia") then
sgs.KOFControlSuit = {sgs.Card_Heart, sgs.Card_Diamond}
sgs.KOFControlDetail.EruptSkill = {"caizhaoji_hujia"}
sgs.KOFControlDetail.MaxInterval = {0}
sgs.KOFControlDetail.ControlFinished = {false}
flag = true
end
if #sgs.KOFControlType > 0 or #sgs.KOFControlSuit > 0 then
room:setPlayerMark(player, "KOFControl", follow)
if flag then
room:setPlayerMark(player, "StrictControl", 1)
end
return true
end
follow = follow + 1
end
end
end
end
end
room:setPlayerMark(player, "KFC_Forbidden", 1)
return false
end
--执行控底观星
function KOFGuanxing(self, cards)
local up = {}
local bottom = {}
local strict = self.player:getMark("StrictControl") > 0
for _,id in pairs(cards) do
local card = sgs.Sanguosha:getCard(id)
if MatchSuit(card, sgs.KOFControlSuit) or MatchType(card, sgs.KOFControlType) then --相符
if card:isKindOf("Peach") then --相符,但是桃子
if self:isWeak() then --相符、桃子、虚弱
table.insert(up, id)
else --相符、桃子、不虚弱
table.insert(bottom, id)
table.insert(sgs.KOFControlResult, id)
self.room:setPlayerMark(self.player, "KOFInterval", 0)
end
else --相符、不是桃子
table.insert(bottom, id)
table.insert(sgs.KOFControlResult, id)
self.room:setPlayerMark(self.player, "KOFInterval", 0)
end
elseif strict then --不相符,严格
table.insert(up, id)
elseif card:isKindOf("Crossbow") then --不相符、不严格、诸葛连弩
table.insert(bottom, id)
table.insert(sgs.KOFControlResult, id)
local marks = self.player:getMark("KOFInterval")
self.room:setPlayerMark(self.player, "KOFInterval", marks+1)
else --不相符、不严格、不为诸葛连弩
local marks = self.player:getMark("KOFInterval")
local maxInterval = sgs.KOFControlDetail.MaxInterval[1]
if maxInterval and marks < maxInterval then --不相符、不严格、不为诸葛连弩、间隔较小
local value = sgs.ai_use_value[card:objectName()]
if value and value > 4 then --不相符、不严格、不为诸葛连弩、间隔较小、使用价值高
table.insert(bottom, id)
table.insert(sgs.KOFControlResult, id)
self.room:setPlayerMark(self.player, "KOFInterval", marks+1)
else --不相符、不严格、不为诸葛连弩、间隔较小、使用价值低
table.insert(up, id)
end
else --不相符、不严格、不为诸葛连弩、间隔较大
table.insert(up, id)
end
end
end
return up, bottom
end
--判断中间武将是否需要让路(待完善)
function KOFNeedDeath(player)
return false
end
--[[
PART X:控制总部
]]--
sgs.classical_func = {}
function Tactic(skillname, self, data)
local func = sgs.classical_func[skillname]
if func then
return func(self, data)
end
end
--观星
sgs.classical_func["guanxing"] = function(self, up_only)
if not up_only then
if self.player:getMark("KFC_Forbidden") == 0 then
if self.player:getMark("KFC_Control") > 0 then
return KOFGuanxing
end
if KOFControlStart(self.player) then
return KOFGuanxing
end
end
end
end
--苦肉
sgs.classical_func["kurou"] = function(self, data)
local mode = string.lower(self.room:getMode())
if mode == "02_1v1" then
if self.player:getMark("KOFKurouRush") > 0 then
return KOFKurouTurnUse
elseif self.player:getMark("KKR_Forbidden") == 0 then
if KOFKurouStart(self) then
return KOFKurouTurnUse
end
end
elseif mode == "06_3v3" then
if self.player:getMark("GoldenWaveFlow") > 0 then
return GWFKurouTurnUse
elseif self.player:getMark("GWF_Forbidden") == 0 then
if GoldenWaveStart(self) then
return GWFKurouTurnUse
end
end
end
end
--遗计
sgs.classical_func["yiji"] = function(self, data)
local mode = string.lower(self.room:getMode())
if mode == "06_3v3" then
if self.player:getMark("GoldenWaveFlow") > 0 then
return GWFYijiAsk
elseif self.player:getMark("Ruthless") > 0 then
return RLYijiAsk
end
end
end
--急救
sgs.classical_func["jijiu"] = function(self, data)
local mode = string.lower(self.room:getMode())
if mode == "06_3v3" then
if self.player:getMark("GoldenWaveFlow") > 0 then
return GWFJijiuSignal
end
end
end
--天香
sgs.classical_func["tianxiang"] = function(self, data)
local mode = string.lower(self.room:getMode())
if mode == "06_3v3" then
if self.player:getMark("Ruthless") > 0 then
return RLTianxiangSkillUse
end
end
end | {
"pile_set_name": "Github"
} |
//
// detail/winrt_ssocket_service.hpp
// ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
//
// Copyright (c) 2003-2015 Christopher M. Kohlhoff (chris at kohlhoff dot com)
//
// Distributed under the Boost Software License, Version 1.0. (See accompanying
// file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
//
#ifndef ASIO_DETAIL_WINRT_SSOCKET_SERVICE_HPP
#define ASIO_DETAIL_WINRT_SSOCKET_SERVICE_HPP
#if defined(_MSC_VER) && (_MSC_VER >= 1200)
# pragma once
#endif // defined(_MSC_VER) && (_MSC_VER >= 1200)
#include "asio/detail/config.hpp"
#if defined(ASIO_WINDOWS_RUNTIME)
#include "asio/error.hpp"
#include "asio/io_service.hpp"
#include "asio/detail/addressof.hpp"
#include "asio/detail/winrt_socket_connect_op.hpp"
#include "asio/detail/winrt_ssocket_service_base.hpp"
#include "asio/detail/winrt_utils.hpp"
#include "asio/detail/push_options.hpp"
namespace clmdep_asio {
namespace detail {
template <typename Protocol>
class winrt_ssocket_service :
public winrt_ssocket_service_base
{
public:
// The protocol type.
typedef Protocol protocol_type;
// The endpoint type.
typedef typename Protocol::endpoint endpoint_type;
// The native type of a socket.
typedef Windows::Networking::Sockets::StreamSocket^ native_handle_type;
// The implementation type of the socket.
struct implementation_type : base_implementation_type
{
// Default constructor.
implementation_type()
: base_implementation_type(),
protocol_(endpoint_type().protocol())
{
}
// The protocol associated with the socket.
protocol_type protocol_;
};
// Constructor.
winrt_ssocket_service(clmdep_asio::io_service& io_service)
: winrt_ssocket_service_base(io_service)
{
}
// Move-construct a new socket implementation.
void move_construct(implementation_type& impl,
implementation_type& other_impl)
{
this->base_move_construct(impl, other_impl);
impl.protocol_ = other_impl.protocol_;
other_impl.protocol_ = endpoint_type().protocol();
}
// Move-assign from another socket implementation.
void move_assign(implementation_type& impl,
winrt_ssocket_service& other_service,
implementation_type& other_impl)
{
this->base_move_assign(impl, other_service, other_impl);
impl.protocol_ = other_impl.protocol_;
other_impl.protocol_ = endpoint_type().protocol();
}
// Move-construct a new socket implementation from another protocol type.
template <typename Protocol1>
void converting_move_construct(implementation_type& impl,
typename winrt_ssocket_service<
Protocol1>::implementation_type& other_impl)
{
this->base_move_construct(impl, other_impl);
impl.protocol_ = protocol_type(other_impl.protocol_);
other_impl.protocol_ = typename Protocol1::endpoint().protocol();
}
// Open a new socket implementation.
clmdep_asio::error_code open(implementation_type& impl,
const protocol_type& protocol, clmdep_asio::error_code& ec)
{
if (is_open(impl))
{
ec = clmdep_asio::error::already_open;
return ec;
}
try
{
impl.socket_ = ref new Windows::Networking::Sockets::StreamSocket;
impl.protocol_ = protocol;
ec = clmdep_asio::error_code();
}
catch (Platform::Exception^ e)
{
ec = clmdep_asio::error_code(e->HResult,
clmdep_asio::system_category());
}
return ec;
}
// Assign a native socket to a socket implementation.
clmdep_asio::error_code assign(implementation_type& impl,
const protocol_type& protocol, const native_handle_type& native_socket,
clmdep_asio::error_code& ec)
{
if (is_open(impl))
{
ec = clmdep_asio::error::already_open;
return ec;
}
impl.socket_ = native_socket;
impl.protocol_ = protocol;
ec = clmdep_asio::error_code();
return ec;
}
// Bind the socket to the specified local endpoint.
clmdep_asio::error_code bind(implementation_type&,
const endpoint_type&, clmdep_asio::error_code& ec)
{
ec = clmdep_asio::error::operation_not_supported;
return ec;
}
// Get the local endpoint.
endpoint_type local_endpoint(const implementation_type& impl,
clmdep_asio::error_code& ec) const
{
endpoint_type endpoint;
endpoint.resize(do_get_endpoint(impl, true,
endpoint.data(), endpoint.size(), ec));
return endpoint;
}
// Get the remote endpoint.
endpoint_type remote_endpoint(const implementation_type& impl,
clmdep_asio::error_code& ec) const
{
endpoint_type endpoint;
endpoint.resize(do_get_endpoint(impl, false,
endpoint.data(), endpoint.size(), ec));
return endpoint;
}
// Set a socket option.
template <typename Option>
clmdep_asio::error_code set_option(implementation_type& impl,
const Option& option, clmdep_asio::error_code& ec)
{
return do_set_option(impl, option.level(impl.protocol_),
option.name(impl.protocol_), option.data(impl.protocol_),
option.size(impl.protocol_), ec);
}
// Get a socket option.
template <typename Option>
clmdep_asio::error_code get_option(const implementation_type& impl,
Option& option, clmdep_asio::error_code& ec) const
{
std::size_t size = option.size(impl.protocol_);
do_get_option(impl, option.level(impl.protocol_),
option.name(impl.protocol_),
option.data(impl.protocol_), &size, ec);
if (!ec)
option.resize(impl.protocol_, size);
return ec;
}
// Connect the socket to the specified endpoint.
clmdep_asio::error_code connect(implementation_type& impl,
const endpoint_type& peer_endpoint, clmdep_asio::error_code& ec)
{
return do_connect(impl, peer_endpoint.data(), ec);
}
// Start an asynchronous connect.
template <typename Handler>
void async_connect(implementation_type& impl,
const endpoint_type& peer_endpoint, Handler& handler)
{
bool is_continuation =
clmdep_asio_handler_cont_helpers::is_continuation(handler);
// Allocate and construct an operation to wrap the handler.
typedef winrt_socket_connect_op<Handler> op;
typename op::ptr p = { clmdep_asio::detail::addressof(handler),
clmdep_asio_handler_alloc_helpers::allocate(
sizeof(op), handler), 0 };
p.p = new (p.v) op(handler);
ASIO_HANDLER_CREATION((p.p, "socket", &impl, "async_connect"));
start_connect_op(impl, peer_endpoint.data(), p.p, is_continuation);
p.v = p.p = 0;
}
};
} // namespace detail
} // namespace clmdep_asio
#include "asio/detail/pop_options.hpp"
#endif // defined(ASIO_WINDOWS_RUNTIME)
#endif // ASIO_DETAIL_WINRT_SSOCKET_SERVICE_HPP
| {
"pile_set_name": "Github"
} |
{
"jsonSchemaSemanticVersion": "1.0.0",
"imports": [
{
"corpusPath": "cdm:/foundations.1.1.cdm.json"
},
{
"corpusPath": "/core/operationsCommon/Common.1.0.cdm.json",
"moniker": "base_Common"
},
{
"corpusPath": "/core/operationsCommon/DataEntityView.1.0.cdm.json",
"moniker": "base_DataEntityView"
},
{
"corpusPath": "/core/operationsCommon/Tables/SupplyChain/ProductInformationManagement/Main/InventTable.1.0.cdm.json"
},
{
"corpusPath": "/core/operationsCommon/Tables/SupplyChain/ProcurementAndSourcing/WorksheetHeader/PurchTable.1.0.cdm.json"
},
{
"corpusPath": "/core/operationsCommon/Tables/SupplyChain/ProcurementAndSourcing/Transaction/VendPackingSlipJour.1.0.cdm.json"
},
{
"corpusPath": "/core/operationsCommon/Tables/SupplyChain/ProcurementAndSourcing/Transaction/VendPackingSlipTrans.1.0.cdm.json"
},
{
"corpusPath": "/core/operationsCommon/Tables/Finance/Ledger/Main/CompanyInfo.1.0.cdm.json"
}
],
"definitions": [
{
"entityName": "PurchPackingSlipTmp",
"extendsEntity": "base_Common/Common",
"exhibitsTraits": [
{
"traitReference": "is.CDM.entityVersion",
"arguments": [
{
"name": "versionNumber",
"value": "1.0"
}
]
}
],
"hasAttributes": [
{
"name": "ExternalItemNum",
"dataType": "ExternalItemId",
"isNullable": true,
"description": ""
},
{
"name": "InventDimPrint",
"dataType": "FreeTxt",
"isNullable": true,
"description": ""
},
{
"name": "InventDimProduct",
"dataType": "InventDimPrint",
"isNullable": true,
"description": ""
},
{
"name": "ItemId",
"dataType": "ItemId",
"isNullable": true,
"description": ""
},
{
"name": "JournalRecId",
"dataType": "VendPackingSlipJourRecId",
"description": ""
},
{
"name": "Name",
"dataType": "ItemFreeTxt",
"isNullable": true,
"description": ""
},
{
"name": "Ordered",
"dataType": "PurchQty",
"isNullable": true,
"description": ""
},
{
"name": "PackingSlipId",
"dataType": "PackingSlipId",
"isNullable": true,
"description": ""
},
{
"name": "pdsCWQty",
"dataType": "PdsCWInventQty",
"isNullable": true,
"description": ""
},
{
"name": "pdsCWStr",
"dataType": "String255",
"isNullable": true,
"description": ""
},
{
"name": "pdsCWUnitId",
"dataType": "PdsCWUnitId",
"isNullable": true,
"description": ""
},
{
"name": "PurchId",
"dataType": "PurchIdBase",
"isNullable": true,
"description": ""
},
{
"name": "PurchUnitTxt",
"dataType": "UnitOfMeasureReportingText",
"isNullable": true,
"description": ""
},
{
"name": "Qty",
"dataType": "PurchDeliveredQty",
"isNullable": true,
"description": ""
},
{
"name": "Remain",
"dataType": "PurchQty",
"isNullable": true,
"description": ""
},
{
"name": "ValueMST",
"dataType": "AmountMST",
"isNullable": true,
"displayName": "Value",
"description": ""
},
{
"name": "VendPackingSlipTrans",
"dataType": "VendPackingSlipTransRecId",
"description": ""
},
{
"name": "DataAreaId",
"dataType": "string",
"isReadOnly": true
},
{
"entity": {
"entityReference": "InventTable"
},
"name": "Relationship_InventTableRelationship",
"resolutionGuidance": {
"entityByReference": {
"allowReference": true
}
}
},
{
"entity": {
"entityReference": "PurchTable"
},
"name": "Relationship_PurchTableRelationship",
"resolutionGuidance": {
"entityByReference": {
"allowReference": true
}
}
},
{
"entity": {
"entityReference": "VendPackingSlipJour"
},
"name": "Relationship_VendPackingSlipJourRelationship",
"resolutionGuidance": {
"entityByReference": {
"allowReference": true
}
}
},
{
"entity": {
"entityReference": "VendPackingSlipTrans"
},
"name": "Relationship_VendPackingSlipTransRelationship",
"resolutionGuidance": {
"entityByReference": {
"allowReference": true
}
}
},
{
"entity": {
"entityReference": "CompanyInfo"
},
"name": "Relationship_CompanyRelationship",
"resolutionGuidance": {
"entityByReference": {
"allowReference": true
}
}
}
],
"displayName": "Show packing slip"
},
{
"dataTypeName": "ExternalItemId",
"extendsDataType": "string"
},
{
"dataTypeName": "FreeTxt",
"extendsDataType": "string"
},
{
"dataTypeName": "InventDimPrint",
"extendsDataType": "string"
},
{
"dataTypeName": "ItemId",
"extendsDataType": "string"
},
{
"dataTypeName": "VendPackingSlipJourRecId",
"extendsDataType": "bigInteger"
},
{
"dataTypeName": "ItemFreeTxt",
"extendsDataType": "string"
},
{
"dataTypeName": "PurchQty",
"extendsDataType": "decimal"
},
{
"dataTypeName": "PackingSlipId",
"extendsDataType": "string"
},
{
"dataTypeName": "PdsCWInventQty",
"extendsDataType": "decimal"
},
{
"dataTypeName": "String255",
"extendsDataType": "string"
},
{
"dataTypeName": "PdsCWUnitId",
"extendsDataType": "string"
},
{
"dataTypeName": "PurchIdBase",
"extendsDataType": "string"
},
{
"dataTypeName": "UnitOfMeasureReportingText",
"extendsDataType": "string"
},
{
"dataTypeName": "PurchDeliveredQty",
"extendsDataType": "decimal"
},
{
"dataTypeName": "AmountMST",
"extendsDataType": "decimal"
},
{
"dataTypeName": "VendPackingSlipTransRecId",
"extendsDataType": "bigInteger"
}
]
} | {
"pile_set_name": "Github"
} |
require 'tzinfo/timezone_definition'
module TZInfo
module Definitions
module America
module Halifax
include TimezoneDefinition
timezone 'America/Halifax' do |tz|
tz.offset :o0, -15264, 0, :LMT
tz.offset :o1, -14400, 0, :AST
tz.offset :o2, -14400, 3600, :ADT
tz.offset :o3, -14400, 3600, :AWT
tz.offset :o4, -14400, 3600, :APT
tz.transition 1902, 6, :o1, 724774703, 300
tz.transition 1916, 4, :o2, 7262864, 3
tz.transition 1916, 10, :o1, 19369101, 8
tz.transition 1918, 4, :o2, 9686791, 4
tz.transition 1918, 10, :o1, 58125545, 24
tz.transition 1920, 5, :o2, 7267361, 3
tz.transition 1920, 8, :o1, 19380525, 8
tz.transition 1921, 5, :o2, 7268447, 3
tz.transition 1921, 9, :o1, 19383501, 8
tz.transition 1922, 4, :o2, 7269524, 3
tz.transition 1922, 9, :o1, 19386421, 8
tz.transition 1923, 5, :o2, 7270637, 3
tz.transition 1923, 9, :o1, 19389333, 8
tz.transition 1924, 5, :o2, 7271729, 3
tz.transition 1924, 9, :o1, 19392349, 8
tz.transition 1925, 5, :o2, 7272821, 3
tz.transition 1925, 9, :o1, 19395373, 8
tz.transition 1926, 5, :o2, 7273955, 3
tz.transition 1926, 9, :o1, 19398173, 8
tz.transition 1927, 5, :o2, 7275005, 3
tz.transition 1927, 9, :o1, 19401197, 8
tz.transition 1928, 5, :o2, 7276139, 3
tz.transition 1928, 9, :o1, 19403989, 8
tz.transition 1929, 5, :o2, 7277231, 3
tz.transition 1929, 9, :o1, 19406861, 8
tz.transition 1930, 5, :o2, 7278323, 3
tz.transition 1930, 9, :o1, 19409877, 8
tz.transition 1931, 5, :o2, 7279415, 3
tz.transition 1931, 9, :o1, 19412901, 8
tz.transition 1932, 5, :o2, 7280486, 3
tz.transition 1932, 9, :o1, 19415813, 8
tz.transition 1933, 4, :o2, 7281578, 3
tz.transition 1933, 10, :o1, 19418781, 8
tz.transition 1934, 5, :o2, 7282733, 3
tz.transition 1934, 9, :o1, 19421573, 8
tz.transition 1935, 6, :o2, 7283867, 3
tz.transition 1935, 9, :o1, 19424605, 8
tz.transition 1936, 6, :o2, 7284962, 3
tz.transition 1936, 9, :o1, 19427405, 8
tz.transition 1937, 5, :o2, 7285967, 3
tz.transition 1937, 9, :o1, 19430429, 8
tz.transition 1938, 5, :o2, 7287059, 3
tz.transition 1938, 9, :o1, 19433341, 8
tz.transition 1939, 5, :o2, 7288235, 3
tz.transition 1939, 9, :o1, 19436253, 8
tz.transition 1940, 5, :o2, 7289264, 3
tz.transition 1940, 9, :o1, 19439221, 8
tz.transition 1941, 5, :o2, 7290356, 3
tz.transition 1941, 9, :o1, 19442133, 8
tz.transition 1942, 2, :o3, 9721599, 4
tz.transition 1945, 8, :o4, 58360379, 24
tz.transition 1945, 9, :o1, 58361489, 24
tz.transition 1946, 4, :o2, 9727755, 4
tz.transition 1946, 9, :o1, 58370225, 24
tz.transition 1947, 4, :o2, 9729211, 4
tz.transition 1947, 9, :o1, 58378961, 24
tz.transition 1948, 4, :o2, 9730667, 4
tz.transition 1948, 9, :o1, 58387697, 24
tz.transition 1949, 4, :o2, 9732123, 4
tz.transition 1949, 9, :o1, 58396433, 24
tz.transition 1951, 4, :o2, 9735063, 4
tz.transition 1951, 9, :o1, 58414073, 24
tz.transition 1952, 4, :o2, 9736519, 4
tz.transition 1952, 9, :o1, 58422809, 24
tz.transition 1953, 4, :o2, 9737975, 4
tz.transition 1953, 9, :o1, 58431545, 24
tz.transition 1954, 4, :o2, 9739431, 4
tz.transition 1954, 9, :o1, 58440281, 24
tz.transition 1956, 4, :o2, 9742371, 4
tz.transition 1956, 9, :o1, 58457921, 24
tz.transition 1957, 4, :o2, 9743827, 4
tz.transition 1957, 9, :o1, 58466657, 24
tz.transition 1958, 4, :o2, 9745283, 4
tz.transition 1958, 9, :o1, 58475393, 24
tz.transition 1959, 4, :o2, 9746739, 4
tz.transition 1959, 9, :o1, 58484129, 24
tz.transition 1962, 4, :o2, 9751135, 4
tz.transition 1962, 10, :o1, 58511177, 24
tz.transition 1963, 4, :o2, 9752591, 4
tz.transition 1963, 10, :o1, 58519913, 24
tz.transition 1964, 4, :o2, 9754047, 4
tz.transition 1964, 10, :o1, 58528649, 24
tz.transition 1965, 4, :o2, 9755503, 4
tz.transition 1965, 10, :o1, 58537553, 24
tz.transition 1966, 4, :o2, 9756959, 4
tz.transition 1966, 10, :o1, 58546289, 24
tz.transition 1967, 4, :o2, 9758443, 4
tz.transition 1967, 10, :o1, 58555025, 24
tz.transition 1968, 4, :o2, 9759899, 4
tz.transition 1968, 10, :o1, 58563761, 24
tz.transition 1969, 4, :o2, 9761355, 4
tz.transition 1969, 10, :o1, 58572497, 24
tz.transition 1970, 4, :o2, 9957600
tz.transition 1970, 10, :o1, 25678800
tz.transition 1971, 4, :o2, 41407200
tz.transition 1971, 10, :o1, 57733200
tz.transition 1972, 4, :o2, 73461600
tz.transition 1972, 10, :o1, 89182800
tz.transition 1973, 4, :o2, 104911200
tz.transition 1973, 10, :o1, 120632400
tz.transition 1974, 4, :o2, 136360800
tz.transition 1974, 10, :o1, 152082000
tz.transition 1975, 4, :o2, 167810400
tz.transition 1975, 10, :o1, 183531600
tz.transition 1976, 4, :o2, 199260000
tz.transition 1976, 10, :o1, 215586000
tz.transition 1977, 4, :o2, 230709600
tz.transition 1977, 10, :o1, 247035600
tz.transition 1978, 4, :o2, 262764000
tz.transition 1978, 10, :o1, 278485200
tz.transition 1979, 4, :o2, 294213600
tz.transition 1979, 10, :o1, 309934800
tz.transition 1980, 4, :o2, 325663200
tz.transition 1980, 10, :o1, 341384400
tz.transition 1981, 4, :o2, 357112800
tz.transition 1981, 10, :o1, 372834000
tz.transition 1982, 4, :o2, 388562400
tz.transition 1982, 10, :o1, 404888400
tz.transition 1983, 4, :o2, 420012000
tz.transition 1983, 10, :o1, 436338000
tz.transition 1984, 4, :o2, 452066400
tz.transition 1984, 10, :o1, 467787600
tz.transition 1985, 4, :o2, 483516000
tz.transition 1985, 10, :o1, 499237200
tz.transition 1986, 4, :o2, 514965600
tz.transition 1986, 10, :o1, 530686800
tz.transition 1987, 4, :o2, 544600800
tz.transition 1987, 10, :o1, 562136400
tz.transition 1988, 4, :o2, 576050400
tz.transition 1988, 10, :o1, 594190800
tz.transition 1989, 4, :o2, 607500000
tz.transition 1989, 10, :o1, 625640400
tz.transition 1990, 4, :o2, 638949600
tz.transition 1990, 10, :o1, 657090000
tz.transition 1991, 4, :o2, 671004000
tz.transition 1991, 10, :o1, 688539600
tz.transition 1992, 4, :o2, 702453600
tz.transition 1992, 10, :o1, 719989200
tz.transition 1993, 4, :o2, 733903200
tz.transition 1993, 10, :o1, 752043600
tz.transition 1994, 4, :o2, 765352800
tz.transition 1994, 10, :o1, 783493200
tz.transition 1995, 4, :o2, 796802400
tz.transition 1995, 10, :o1, 814942800
tz.transition 1996, 4, :o2, 828856800
tz.transition 1996, 10, :o1, 846392400
tz.transition 1997, 4, :o2, 860306400
tz.transition 1997, 10, :o1, 877842000
tz.transition 1998, 4, :o2, 891756000
tz.transition 1998, 10, :o1, 909291600
tz.transition 1999, 4, :o2, 923205600
tz.transition 1999, 10, :o1, 941346000
tz.transition 2000, 4, :o2, 954655200
tz.transition 2000, 10, :o1, 972795600
tz.transition 2001, 4, :o2, 986104800
tz.transition 2001, 10, :o1, 1004245200
tz.transition 2002, 4, :o2, 1018159200
tz.transition 2002, 10, :o1, 1035694800
tz.transition 2003, 4, :o2, 1049608800
tz.transition 2003, 10, :o1, 1067144400
tz.transition 2004, 4, :o2, 1081058400
tz.transition 2004, 10, :o1, 1099198800
tz.transition 2005, 4, :o2, 1112508000
tz.transition 2005, 10, :o1, 1130648400
tz.transition 2006, 4, :o2, 1143957600
tz.transition 2006, 10, :o1, 1162098000
tz.transition 2007, 3, :o2, 1173592800
tz.transition 2007, 11, :o1, 1194152400
tz.transition 2008, 3, :o2, 1205042400
tz.transition 2008, 11, :o1, 1225602000
tz.transition 2009, 3, :o2, 1236492000
tz.transition 2009, 11, :o1, 1257051600
tz.transition 2010, 3, :o2, 1268546400
tz.transition 2010, 11, :o1, 1289106000
tz.transition 2011, 3, :o2, 1299996000
tz.transition 2011, 11, :o1, 1320555600
tz.transition 2012, 3, :o2, 1331445600
tz.transition 2012, 11, :o1, 1352005200
tz.transition 2013, 3, :o2, 1362895200
tz.transition 2013, 11, :o1, 1383454800
tz.transition 2014, 3, :o2, 1394344800
tz.transition 2014, 11, :o1, 1414904400
tz.transition 2015, 3, :o2, 1425794400
tz.transition 2015, 11, :o1, 1446354000
tz.transition 2016, 3, :o2, 1457848800
tz.transition 2016, 11, :o1, 1478408400
tz.transition 2017, 3, :o2, 1489298400
tz.transition 2017, 11, :o1, 1509858000
tz.transition 2018, 3, :o2, 1520748000
tz.transition 2018, 11, :o1, 1541307600
tz.transition 2019, 3, :o2, 1552197600
tz.transition 2019, 11, :o1, 1572757200
tz.transition 2020, 3, :o2, 1583647200
tz.transition 2020, 11, :o1, 1604206800
tz.transition 2021, 3, :o2, 1615701600
tz.transition 2021, 11, :o1, 1636261200
tz.transition 2022, 3, :o2, 1647151200
tz.transition 2022, 11, :o1, 1667710800
tz.transition 2023, 3, :o2, 1678600800
tz.transition 2023, 11, :o1, 1699160400
tz.transition 2024, 3, :o2, 1710050400
tz.transition 2024, 11, :o1, 1730610000
tz.transition 2025, 3, :o2, 1741500000
tz.transition 2025, 11, :o1, 1762059600
tz.transition 2026, 3, :o2, 1772949600
tz.transition 2026, 11, :o1, 1793509200
tz.transition 2027, 3, :o2, 1805004000
tz.transition 2027, 11, :o1, 1825563600
tz.transition 2028, 3, :o2, 1836453600
tz.transition 2028, 11, :o1, 1857013200
tz.transition 2029, 3, :o2, 1867903200
tz.transition 2029, 11, :o1, 1888462800
tz.transition 2030, 3, :o2, 1899352800
tz.transition 2030, 11, :o1, 1919912400
tz.transition 2031, 3, :o2, 1930802400
tz.transition 2031, 11, :o1, 1951362000
tz.transition 2032, 3, :o2, 1962856800
tz.transition 2032, 11, :o1, 1983416400
tz.transition 2033, 3, :o2, 1994306400
tz.transition 2033, 11, :o1, 2014866000
tz.transition 2034, 3, :o2, 2025756000
tz.transition 2034, 11, :o1, 2046315600
tz.transition 2035, 3, :o2, 2057205600
tz.transition 2035, 11, :o1, 2077765200
tz.transition 2036, 3, :o2, 2088655200
tz.transition 2036, 11, :o1, 2109214800
tz.transition 2037, 3, :o2, 2120104800
tz.transition 2037, 11, :o1, 2140664400
tz.transition 2038, 3, :o2, 9861987, 4
tz.transition 2038, 11, :o1, 59177633, 24
tz.transition 2039, 3, :o2, 9863443, 4
tz.transition 2039, 11, :o1, 59186369, 24
tz.transition 2040, 3, :o2, 9864899, 4
tz.transition 2040, 11, :o1, 59195105, 24
tz.transition 2041, 3, :o2, 9866355, 4
tz.transition 2041, 11, :o1, 59203841, 24
tz.transition 2042, 3, :o2, 9867811, 4
tz.transition 2042, 11, :o1, 59212577, 24
tz.transition 2043, 3, :o2, 9869267, 4
tz.transition 2043, 11, :o1, 59221313, 24
tz.transition 2044, 3, :o2, 9870751, 4
tz.transition 2044, 11, :o1, 59230217, 24
tz.transition 2045, 3, :o2, 9872207, 4
tz.transition 2045, 11, :o1, 59238953, 24
tz.transition 2046, 3, :o2, 9873663, 4
tz.transition 2046, 11, :o1, 59247689, 24
tz.transition 2047, 3, :o2, 9875119, 4
tz.transition 2047, 11, :o1, 59256425, 24
tz.transition 2048, 3, :o2, 9876575, 4
tz.transition 2048, 11, :o1, 59265161, 24
tz.transition 2049, 3, :o2, 9878059, 4
tz.transition 2049, 11, :o1, 59274065, 24
tz.transition 2050, 3, :o2, 9879515, 4
tz.transition 2050, 11, :o1, 59282801, 24
end
end
end
end
end
| {
"pile_set_name": "Github"
} |
%verify "executed"
%include "arm-vfp/funop.S" {"instr":"ftosizs s1, s0"}
| {
"pile_set_name": "Github"
} |
Title: Wine 0.9.41 发布
Date: 2007-07-14 08:02
Author: toy
Category: Apps
Slug: wine-0941-released
[Wine](http://www.winehq.org/) 于昨日获得了小幅更新,发布了新的 0.9.41
版。这个版本不仅实现了一些新的改进,而且也修订了许多
bug。目前,仅有该版本的源码包可用,适用于常见 Linux
发行版的二进制包还需稍作等待。
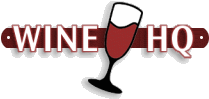
据悉,该版本的 Wine 主要包括下列改进:
- 实现了许多 gdiplus 函数
- 更为完整的 pdh.dll 实现
- 支持 MSI 远程调用
- 在 crypt32.dll 中提供了消息支持
现在,你可以[获取 Wine 0.9.41
的源码包](http://prdownloads.sourceforge.net/wine/wine-0.9.41.tar.bz2)自行编译。当然,你也可以等候官方提供预编译的二进制包。
| {
"pile_set_name": "Github"
} |
// Copyright 2015 Dolphin Emulator Project
// Licensed under GPLv2+
// Refer to the license.txt file included.
#pragma once
#include <memory>
#include <optional>
#include <vector>
#include "Common/CommonTypes.h"
class PointerWrap;
namespace DiscIO
{
struct Partition;
}
namespace DVDInterface
{
enum class ReplyType : u32;
}
namespace DiscIO
{
enum class Platform;
class Volume;
} // namespace DiscIO
namespace IOS::ES
{
class TMDReader;
class TicketReader;
} // namespace IOS::ES
namespace DVDThread
{
void Start();
void Stop();
void DoState(PointerWrap& p);
void SetDisc(std::unique_ptr<DiscIO::Volume> disc);
bool HasDisc();
bool IsEncryptedAndHashed();
DiscIO::Platform GetDiscType();
u64 PartitionOffsetToRawOffset(u64 offset, const DiscIO::Partition& partition);
IOS::ES::TMDReader GetTMD(const DiscIO::Partition& partition);
IOS::ES::TicketReader GetTicket(const DiscIO::Partition& partition);
bool IsInsertedDiscRunning();
// This function returns true and calls SConfig::SetRunningGameMetadata(Volume&, Partition&)
// if both of the following conditions are true:
// - A disc is inserted
// - The title_id argument doesn't contain a value, or its value matches the disc's title ID
bool UpdateRunningGameMetadata(const DiscIO::Partition& partition,
std::optional<u64> title_id = {});
void StartRead(u64 dvd_offset, u32 length, const DiscIO::Partition& partition,
DVDInterface::ReplyType reply_type, s64 ticks_until_completion);
void StartReadToEmulatedRAM(u32 output_address, u64 dvd_offset, u32 length,
const DiscIO::Partition& partition, DVDInterface::ReplyType reply_type,
s64 ticks_until_completion);
} // namespace DVDThread
| {
"pile_set_name": "Github"
} |
/*
* Copyright (c) 2003, 2011, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package java.lang.instrument;
/*
* Copyright 2003 Wily Technology, Inc.
*/
/**
* This class serves as a parameter block to the <code>Instrumentation.redefineClasses</code> method.
* Serves to bind the <code>Class</code> that needs redefining together with the new class file bytes.
*
* @see java.lang.instrument.Instrumentation#redefineClasses
* @since 1.5
*/
public final class ClassDefinition {
/**
* The class to redefine
*/
private final Class<?> mClass;
/**
* The replacement class file bytes
*/
private final byte[] mClassFile;
/**
* Creates a new <code>ClassDefinition</code> binding using the supplied
* class and class file bytes. Does not copy the supplied buffer, just captures a reference to it.
*
* @param theClass the <code>Class</code> that needs redefining
* @param theClassFile the new class file bytes
*
* @throws java.lang.NullPointerException if the supplied class or array is <code>null</code>.
*/
public
ClassDefinition( Class<?> theClass,
byte[] theClassFile) {
if (theClass == null || theClassFile == null) {
throw new NullPointerException();
}
mClass = theClass;
mClassFile = theClassFile;
}
/**
* Returns the class.
*
* @return the <code>Class</code> object referred to.
*/
public Class<?>
getDefinitionClass() {
return mClass;
}
/**
* Returns the array of bytes that contains the new class file.
*
* @return the class file bytes.
*/
public byte[]
getDefinitionClassFile() {
return mClassFile;
}
}
| {
"pile_set_name": "Github"
} |
{{#with tryGetTargetReflectionDeep}}
{{#ifCond ../name '===' name}}
Re-exports <a href="{{relativeURL url}}">{{name}}</a>
{{else if flags.isExported}}
Renames and re-exports <a href="{{relativeURL url}}">{{name}}</a>
{{else}}
Renames and exports <a href="{{relativeURL url}}">{{name}}</a>
{{/ifCond}}
{{else}}
Re-exports {{name}}
{{/with}}
| {
"pile_set_name": "Github"
} |
import random
import multiaddr
import pytest
from libp2p.peer.id import ID
from libp2p.peer.peerinfo import InvalidAddrError, PeerInfo, info_from_p2p_addr
ALPHABETS = "123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxyz"
VALID_MULTI_ADDR_STR = "/ip4/127.0.0.1/tcp/8000/p2p/3YgLAeMKSAPcGqZkAt8mREqhQXmJT8SN8VCMN4T6ih4GNX9wvK8mWJnWZ1qA2mLdCQ" # noqa: E501
def test_init_():
random_addrs = [random.randint(0, 255) for r in range(4)]
random_id_string = ""
for _ in range(10):
random_id_string += random.SystemRandom().choice(ALPHABETS)
peer_id = ID(random_id_string.encode())
peer_info = PeerInfo(peer_id, random_addrs)
assert peer_info.peer_id == peer_id
assert peer_info.addrs == random_addrs
@pytest.mark.parametrize(
"addr",
(
pytest.param(multiaddr.Multiaddr("/"), id="empty multiaddr"),
pytest.param(
multiaddr.Multiaddr("/ip4/127.0.0.1"),
id="multiaddr without peer_id(p2p protocol)",
),
),
)
def test_info_from_p2p_addr_invalid(addr):
with pytest.raises(InvalidAddrError):
info_from_p2p_addr(addr)
def test_info_from_p2p_addr_valid():
m_addr = multiaddr.Multiaddr(VALID_MULTI_ADDR_STR)
info = info_from_p2p_addr(m_addr)
assert (
info.peer_id.pretty()
== "3YgLAeMKSAPcGqZkAt8mREqhQXmJT8SN8VCMN4T6ih4GNX9wvK8mWJnWZ1qA2mLdCQ"
)
assert len(info.addrs) == 1
assert str(info.addrs[0]) == "/ip4/127.0.0.1/tcp/8000"
| {
"pile_set_name": "Github"
} |
/*
* Copyright (C) 2014 Freescale Semiconductor
*
* SPDX-License-Identifier: GPL-2.0+
*/
#ifndef __FSL_DPAA_FD_H
#define __FSL_DPAA_FD_H
/* Place-holder for FDs, we represent it via the simplest form that we need for
* now. Different overlays may be needed to support different options, etc. (It
* is impractical to define One True Struct, because the resulting encoding
* routines (lots of read-modify-writes) would be worst-case performance whether
* or not circumstances required them.) */
struct dpaa_fd {
union {
u32 words[8];
struct dpaa_fd_simple {
u32 addr_lo;
u32 addr_hi;
u32 len;
/* offset in the MS 16 bits, BPID in the LS 16 bits */
u32 bpid_offset;
u32 frc; /* frame context */
/* "err", "va", "cbmt", "asal", [...] */
u32 ctrl;
/* flow context */
u32 flc_lo;
u32 flc_hi;
} simple;
};
};
enum dpaa_fd_format {
dpaa_fd_single = 0,
dpaa_fd_list,
dpaa_fd_sg
};
static inline u64 ldpaa_fd_get_addr(const struct dpaa_fd *fd)
{
return (u64)((((uint64_t)fd->simple.addr_hi) << 32)
+ fd->simple.addr_lo);
}
static inline void ldpaa_fd_set_addr(struct dpaa_fd *fd, u64 addr)
{
fd->simple.addr_hi = upper_32_bits(addr);
fd->simple.addr_lo = lower_32_bits(addr);
}
static inline u32 ldpaa_fd_get_len(const struct dpaa_fd *fd)
{
return fd->simple.len;
}
static inline void ldpaa_fd_set_len(struct dpaa_fd *fd, u32 len)
{
fd->simple.len = len;
}
static inline uint16_t ldpaa_fd_get_offset(const struct dpaa_fd *fd)
{
return (uint16_t)(fd->simple.bpid_offset >> 16) & 0x0FFF;
}
static inline void ldpaa_fd_set_offset(struct dpaa_fd *fd, uint16_t offset)
{
fd->simple.bpid_offset &= 0xF000FFFF;
fd->simple.bpid_offset |= (u32)offset << 16;
}
static inline uint16_t ldpaa_fd_get_bpid(const struct dpaa_fd *fd)
{
return (uint16_t)(fd->simple.bpid_offset & 0xFFFF);
}
static inline void ldpaa_fd_set_bpid(struct dpaa_fd *fd, uint16_t bpid)
{
fd->simple.bpid_offset &= 0xFFFF0000;
fd->simple.bpid_offset |= (u32)bpid;
}
/* When frames are dequeued, the FDs show up inside "dequeue" result structures
* (if at all, not all dequeue results contain valid FDs). This structure type
* is intentionally defined without internal detail, and the only reason it
* isn't declared opaquely (without size) is to allow the user to provide
* suitably-sized (and aligned) memory for these entries. */
struct ldpaa_dq {
uint32_t dont_manipulate_directly[16];
};
/* Parsing frame dequeue results */
#define LDPAA_DQ_STAT_FQEMPTY 0x80
#define LDPAA_DQ_STAT_HELDACTIVE 0x40
#define LDPAA_DQ_STAT_FORCEELIGIBLE 0x20
#define LDPAA_DQ_STAT_VALIDFRAME 0x10
#define LDPAA_DQ_STAT_ODPVALID 0x04
#define LDPAA_DQ_STAT_VOLATILE 0x02
#define LDPAA_DQ_STAT_EXPIRED 0x01
uint32_t ldpaa_dq_flags(const struct ldpaa_dq *);
static inline int ldpaa_dq_is_pull(const struct ldpaa_dq *dq)
{
return (int)(ldpaa_dq_flags(dq) & LDPAA_DQ_STAT_VOLATILE);
}
static inline int ldpaa_dq_is_pull_complete(
const struct ldpaa_dq *dq)
{
return (int)(ldpaa_dq_flags(dq) & LDPAA_DQ_STAT_EXPIRED);
}
/* seqnum/odpid are valid only if VALIDFRAME and ODPVALID flags are TRUE */
uint16_t ldpaa_dq_seqnum(const struct ldpaa_dq *);
uint16_t ldpaa_dq_odpid(const struct ldpaa_dq *);
uint32_t ldpaa_dq_fqid(const struct ldpaa_dq *);
uint32_t ldpaa_dq_byte_count(const struct ldpaa_dq *);
uint32_t ldpaa_dq_frame_count(const struct ldpaa_dq *);
uint32_t ldpaa_dq_fqd_ctx_hi(const struct ldpaa_dq *);
uint32_t ldpaa_dq_fqd_ctx_lo(const struct ldpaa_dq *);
/* get the Frame Descriptor */
const struct dpaa_fd *ldpaa_dq_fd(const struct ldpaa_dq *);
#endif /* __FSL_DPAA_FD_H */
| {
"pile_set_name": "Github"
} |
##
##
| {
"pile_set_name": "Github"
} |
// Copyright 2018 The Go Authors. All rights reserved.
// Use of this source code is governed by a BSD-style
// license that can be found in the LICENSE file.
// +build aix
// +build ppc
package unix
//sysnb Getrlimit(resource int, rlim *Rlimit) (err error) = getrlimit64
//sysnb Setrlimit(resource int, rlim *Rlimit) (err error) = setrlimit64
//sys Seek(fd int, offset int64, whence int) (off int64, err error) = lseek64
//sys mmap(addr uintptr, length uintptr, prot int, flags int, fd int, offset int64) (xaddr uintptr, err error)
func setTimespec(sec, nsec int64) Timespec {
return Timespec{Sec: int32(sec), Nsec: int32(nsec)}
}
func setTimeval(sec, usec int64) Timeval {
return Timeval{Sec: int32(sec), Usec: int32(usec)}
}
func (iov *Iovec) SetLen(length int) {
iov.Len = uint32(length)
}
func (msghdr *Msghdr) SetControllen(length int) {
msghdr.Controllen = uint32(length)
}
func (msghdr *Msghdr) SetIovlen(length int) {
msghdr.Iovlen = int32(length)
}
func (cmsg *Cmsghdr) SetLen(length int) {
cmsg.Len = uint32(length)
}
func Fstat(fd int, stat *Stat_t) error {
return fstat(fd, stat)
}
func Fstatat(dirfd int, path string, stat *Stat_t, flags int) error {
return fstatat(dirfd, path, stat, flags)
}
func Lstat(path string, stat *Stat_t) error {
return lstat(path, stat)
}
func Stat(path string, statptr *Stat_t) error {
return stat(path, statptr)
}
| {
"pile_set_name": "Github"
} |
StrCpy $MUI_FINISHPAGE_SHOWREADME_TEXT_STRING "Vis versjonsmerknader"
StrCpy $ConfirmEndProcess_MESSAGEBOX_TEXT "Fant ${APPLICATION_EXECUTABLE}-prosess(er) som må stoppes.$\nVil du at installasjonsprogrammet skal stoppe dem for deg?"
StrCpy $ConfirmEndProcess_KILLING_PROCESSES_TEXT "Terminerer ${APPLICATION_EXECUTABLE}-prosesser."
StrCpy $ConfirmEndProcess_KILL_NOT_FOUND_TEXT "Fant ikke prosess som skulle termineres!"
StrCpy $PageReinstall_NEW_Field_1 "En eldre versjon av ${APPLICATION_NAME} er installert på systemet ditt. Det anbefales at du avinstallerer den versjonen før installering av ny versjon. Velg hva du vil gjøre og klikk Neste for å fortsette."
StrCpy $PageReinstall_NEW_Field_2 "Avinstaller før installering"
StrCpy $PageReinstall_NEW_Field_3 "Ikke avinstaller"
StrCpy $PageReinstall_NEW_MUI_HEADER_TEXT_TITLE "Allerede installert"
StrCpy $PageReinstall_NEW_MUI_HEADER_TEXT_SUBTITLE "Velg hvordan du vil installere ${APPLICATION_NAME}."
StrCpy $PageReinstall_OLD_Field_1 "En nyere versjon av ${APPLICATION_NAME} er allerede installert! Det anbefales ikke at du installerer en eldre versjon. Hvis du virkelig ønsker å installere denne eldre versjonen, er det bedre å avinstallere gjeldende versjon først. Velg hva du vil gjøre og klikk Neste for å fortsette."
StrCpy $PageReinstall_SAME_Field_1 "${APPLICATION_NAME} ${VERSION} er installert allerede.$\n$\nVelg hva du ønsker å gjøre og klikk Neste for å fortsette."
StrCpy $PageReinstall_SAME_Field_2 "Legg til/installer komponenter på nytt"
StrCpy $PageReinstall_SAME_Field_3 "Avinstaller ${APPLICATION_NAME}"
StrCpy $UNINSTALLER_APPDATA_TITLE "Avinstaller ${APPLICATION_NAME}"
StrCpy $PageReinstall_SAME_MUI_HEADER_TEXT_SUBTITLE "Velg hva slags vedlikehold som skal utføres."
StrCpy $SEC_APPLICATION_DETAILS "Installerer ${APPLICATION_NAME} grunnleggende."
StrCpy $OPTION_SECTION_SC_SHELL_EXT_SECTION "Integrering med Windows Utforsker"
StrCpy $OPTION_SECTION_SC_SHELL_EXT_DetailPrint "Installerer integrering med Windows Utforsker"
StrCpy $OPTION_SECTION_SC_START_MENU_SECTION "Snarvei i Start-menyen"
StrCpy $OPTION_SECTION_SC_START_MENU_DetailPrint "Legger til snarvei for ${APPLICATION_NAME} i Start-menyen."
StrCpy $OPTION_SECTION_SC_DESKTOP_SECTION "Snarvei på skrivebordet"
StrCpy $OPTION_SECTION_SC_DESKTOP_DetailPrint "Oppretter snarveier på skrivebordet"
StrCpy $OPTION_SECTION_SC_QUICK_LAUNCH_SECTION "Snarvei i Hurtigstart"
StrCpy $OPTION_SECTION_SC_QUICK_LAUNCH_DetailPrint "Oppretter snarvei i Hurtigstart"
StrCpy $OPTION_SECTION_SC_APPLICATION_Desc "${APPLICATION_NAME} grunnleggende."
StrCpy $OPTION_SECTION_SC_START_MENU_Desc "${APPLICATION_NAME}-snarvei."
StrCpy $OPTION_SECTION_SC_DESKTOP_Desc "Skrivebordssnarvei for ${APPLICATION_NAME}."
StrCpy $OPTION_SECTION_SC_QUICK_LAUNCH_Desc "Hurtigstart-snarvei for ${APPLICATION_NAME}."
StrCpy $UNINSTALLER_FILE_Detail "Skriver Avinstallasjonsprogram."
StrCpy $UNINSTALLER_REGISTRY_Detail "Skriver registernøkler for installasjonsprogrammet"
StrCpy $UNINSTALLER_FINISHED_Detail "Ferdig"
StrCpy $UNINSTALL_MESSAGEBOX "Det ser ikke ut som ${APPLICATION_NAME} er installert i mappe '$INSTDIR'.$\n$\nFortsett likevel (ikke anbefalt)?"
StrCpy $UNINSTALL_ABORT "Avinstallering avbrutt av bruker"
StrCpy $INIT_NO_QUICK_LAUNCH "Hurtigstart-snarvei (I/T)"
StrCpy $INIT_NO_DESKTOP "Snarvei på skrivebordet (skriver over eksisterende)"
StrCpy $UAC_ERROR_ELEVATE "Klarte ikke å heve tilgangsnivå. Feil: "
StrCpy $UAC_INSTALLER_REQUIRE_ADMIN "Dette installasjonsprogrammet krever administrasjonstilgang. Prøv igjen"
StrCpy $INIT_INSTALLER_RUNNING "Installasjonsprogrammet kjører allerede."
StrCpy $UAC_UNINSTALLER_REQUIRE_ADMIN "Avinstallasjonsprogrammet krever administrasjonstilgang. Prøv igjen"
StrCpy $UAC_ERROR_LOGON_SERVICE "Påloggingstjenesten kjører ikke, avbryter!"
StrCpy $INIT_UNINSTALLER_RUNNING "Avinstallasjonsprogrammet kjører allerede."
StrCpy $SectionGroup_Shortcuts "Snarveier"
| {
"pile_set_name": "Github"
} |
<a class="{% if not nav_item.is_link %}reference internal{% endif %}{% if nav_item.active%} current{%endif%}" href="{% if not nav_item.is_section %}{{ nav_item.url|url }}{% else %}#{% endif %}">{{ nav_item.title }}</a>
{%- set navlevel = navlevel + 1 %}
{%- if navlevel <= config.theme.navigation_depth
and ((nav_item.is_page and nav_item.toc.items
and (not config.theme.titles_only
and (nav_item == page or not config.theme.collapse_navigation)))
or (nav_item.is_section and nav_item.children)) %}
<ul{% if nav_item.active %} class="current"{% endif %}>
{%- if nav_item.is_page %}
{#- Skip first level of toc which is page title. #}
{%- set toc_item = nav_item.toc.items[0] %}
{%- include 'toc.html' %}
{%- elif nav_item.is_section %}
{%- for nav_item in nav_item.children %}
<li class="toctree-l{{ navlevel }}{% if nav_item.active%} current{%endif%}">
{%- include 'nav.html' %}
</li>
{%- endfor %}
{%- endif %}
</ul>
{%- endif %}
{%- set navlevel = navlevel - 1 %}
| {
"pile_set_name": "Github"
} |
94e2c25a08522071ca4d2314ddb2a4a1 *tests/data/fate/vsynth_lena-ffvhuff444p16.avi
26360720 tests/data/fate/vsynth_lena-ffvhuff444p16.avi
05ccd9a38f9726030b3099c0c99d3a13 *tests/data/fate/vsynth_lena-ffvhuff444p16.out.rawvideo
stddev: 0.45 PSNR: 55.06 MAXDIFF: 7 bytes: 7603200/ 7603200
| {
"pile_set_name": "Github"
} |
# /* **************************************************************************
# * *
# * (C) Copyright Edward Diener 2014. *
# * Distributed under the Boost Software License, Version 1.0. (See *
# * accompanying file LICENSE_1_0.txt or copy at *
# * http://www.boost.org/LICENSE_1_0.txt) *
# * *
# ************************************************************************** */
#
# /* See http://www.boost.org for most recent version. */
#
# ifndef BOOST_PREPROCESSOR_ARRAY_DETAIL_GET_DATA_HPP
# define BOOST_PREPROCESSOR_ARRAY_DETAIL_GET_DATA_HPP
#
# include <boost/preprocessor/config/config.hpp>
# include <boost/preprocessor/tuple/rem.hpp>
# include <boost/preprocessor/control/if.hpp>
# include <boost/preprocessor/control/iif.hpp>
# include <boost/preprocessor/facilities/is_1.hpp>
#
# /* BOOST_PP_ARRAY_DETAIL_GET_DATA */
#
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_NONE(size, data)
# if BOOST_PP_VARIADICS && !(BOOST_PP_VARIADICS_MSVC && _MSC_VER <= 1400)
# if BOOST_PP_VARIADICS_MSVC
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY_VC_DEFAULT(size, data) BOOST_PP_TUPLE_REM(size) data
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY_VC_CAT(size, data) BOOST_PP_TUPLE_REM_CAT(size) data
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY(size, data) \
BOOST_PP_IIF \
( \
BOOST_PP_IS_1(size), \
BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY_VC_CAT, \
BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY_VC_DEFAULT \
) \
(size,data) \
/**/
# else
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY(size, data) BOOST_PP_TUPLE_REM(size) data
# endif
# else
# define BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY(size, data) BOOST_PP_TUPLE_REM(size) data
# endif
# define BOOST_PP_ARRAY_DETAIL_GET_DATA(size, data) \
BOOST_PP_IF \
( \
size, \
BOOST_PP_ARRAY_DETAIL_GET_DATA_ANY, \
BOOST_PP_ARRAY_DETAIL_GET_DATA_NONE \
) \
(size,data) \
/**/
#
# endif /* BOOST_PREPROCESSOR_ARRAY_DETAIL_GET_DATA_HPP */
| {
"pile_set_name": "Github"
} |
////////////////////////////////////////////////////////////////////////////////
// Copyright (c) 2007-2016 Hartmut Kaiser
// Copyright (c) 2008-2009 Chirag Dekate, Anshul Tandon
// Copyright (c) 2012-2013 Thomas Heller
//
// SPDX-License-Identifier: BSL-1.0
// Distributed under the Boost Software License, Version 1.0. (See accompanying
// file LICENSE_1_0.txt or copy at http://www.boost.org/LICENSE_1_0.txt)
////////////////////////////////////////////////////////////////////////////////
#pragma once
#include <hpx/config.hpp>
#include <hpx/assert.hpp>
#include <hpx/modules/errors.hpp>
#include <hpx/topology/cpu_mask.hpp>
#include <boost/variant.hpp>
#include <cstddef>
#include <cstdint>
#include <limits>
#include <string>
#include <utility>
#include <vector>
namespace hpx { namespace threads {
namespace detail {
typedef std::vector<std::int64_t> bounds_type;
enum distribution_type
{
compact = 0x01,
scatter = 0x02,
balanced = 0x04,
numa_balanced = 0x08
};
struct spec_type
{
enum type
{
unknown,
thread,
socket,
numanode,
core,
pu
};
HPX_CORE_EXPORT static char const* type_name(type t);
static std::int64_t all_entities()
{
return (std::numeric_limits<std::int64_t>::min)();
}
spec_type(type t = unknown, std::int64_t min = all_entities(),
std::int64_t max = all_entities())
: type_(t)
, index_bounds_()
{
if (t != unknown)
{
if (max == 0 || max == all_entities())
{
// one or all entities
index_bounds_.push_back(min);
}
else if (min != all_entities())
{
// all entities between min and -max, or just min,max
HPX_ASSERT(min >= 0);
index_bounds_.push_back(min);
index_bounds_.push_back(max);
}
}
}
bool operator==(spec_type const& rhs) const
{
if (type_ != rhs.type_ ||
index_bounds_.size() != rhs.index_bounds_.size())
return false;
for (std::size_t i = 0; i < index_bounds_.size(); ++i)
{
if (index_bounds_[i] != rhs.index_bounds_[i])
return false;
}
return true;
}
type type_;
bounds_type index_bounds_;
};
typedef std::vector<spec_type> mapping_type;
typedef std::pair<spec_type, mapping_type> full_mapping_type;
typedef std::vector<full_mapping_type> mappings_spec_type;
typedef boost::variant<distribution_type, mappings_spec_type>
mappings_type;
HPX_CORE_EXPORT bounds_type extract_bounds(
spec_type const& m, std::size_t default_last, error_code& ec);
HPX_CORE_EXPORT void parse_mappings(std::string const& spec,
mappings_type& mappings, error_code& ec = throws);
} // namespace detail
HPX_CORE_EXPORT void parse_affinity_options(std::string const& spec,
std::vector<mask_type>& affinities, std::size_t used_cores,
std::size_t max_cores, std::size_t num_threads,
std::vector<std::size_t>& num_pus, bool use_process_mask,
error_code& ec = throws);
// backwards compatibility helper
inline void parse_affinity_options(std::string const& spec,
std::vector<mask_type>& affinities, error_code& ec = throws)
{
std::vector<std::size_t> num_pus;
parse_affinity_options(
spec, affinities, 1, 1, affinities.size(), num_pus, false, ec);
}
}} // namespace hpx::threads
| {
"pile_set_name": "Github"
} |
@extends('errors/layout')
@section('title') 403 Forbidden @endsection
@section('content')
<h1><i class="fa fa-ban red"></i> 403 Forbidden</h1>
<p class="lead">抱歉!您可能被禁止访问该页面,如有问题请联系管理员。</p>
<p>
<a class="btn btn-default btn-lg" href="{{ route('website.index') }}"><span class="green">返回首页</span></a>
</p>
@endsection
| {
"pile_set_name": "Github"
} |
{
"path": "../../../notebooks/minio_setup.ipynb"
}
| {
"pile_set_name": "Github"
} |
/*
* stree.c
*
* Copyright 2010 Alexander Petukhov <devel(at)apetukhov.ru>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301, USA.
*/
/*
* Contains function to manipulate stack trace tree view.
*/
#include <stdlib.h>
#include <string.h>
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
#include <geanyplugin.h>
#include "stree.h"
#include "breakpoints.h"
#include "utils.h"
#include "debug_module.h"
#include "pixbuf.h"
#include "cell_renderers/cellrendererframeicon.h"
/* Tree view columns */
enum
{
S_FRAME, /* the frame if it's a frame, or NULL if it's a thread */
S_THREAD_ID,
S_ACTIVE,
S_N_COLUMNS
};
/* active thread and frame */
static glong active_thread_id = 0;
static int active_frame_index = 0;
/* callbacks */
static select_frame_cb select_frame = NULL;
static select_thread_cb select_thread = NULL;
static move_to_line_cb move_to_line = NULL;
/* tree view, model and store handles */
static GtkWidget *tree = NULL;
static GtkTreeModel *model = NULL;
static GtkTreeStore *store = NULL;
static GtkTreeViewColumn *column_filepath = NULL;
static GtkTreeViewColumn *column_address = NULL;
/* cell renderer for a frame arrow */
static GtkCellRenderer *renderer_arrow = NULL;
static GType frame_get_type (void);
G_DEFINE_BOXED_TYPE(frame, frame, frame_ref, frame_unref)
#define STREE_TYPE_FRAME (frame_get_type ())
/* finds the iter for thread @thread_id */
static gboolean find_thread_iter (gint thread_id, GtkTreeIter *iter)
{
gboolean found = FALSE;
if (gtk_tree_model_get_iter_first(model, iter))
{
do
{
gint existing_thread_id;
gtk_tree_model_get(model, iter, S_THREAD_ID, &existing_thread_id, -1);
if (existing_thread_id == thread_id)
found = TRUE;
}
while (! found && gtk_tree_model_iter_next(model, iter));
}
return found;
}
/*
* frame arrow clicked callback
*/
static void on_frame_arrow_clicked(CellRendererFrameIcon *cell_renderer, gchar *path, gpointer user_data)
{
GtkTreePath *new_active_frame = gtk_tree_path_new_from_string (path);
if (gtk_tree_path_get_indices(new_active_frame)[1] != active_frame_index)
{
GtkTreeIter thread_iter;
GtkTreeIter iter;
GtkTreePath *old_active_frame;
find_thread_iter (active_thread_id, &thread_iter);
old_active_frame = gtk_tree_model_get_path (model, &thread_iter);
gtk_tree_path_append_index(old_active_frame, active_frame_index);
gtk_tree_model_get_iter(model, &iter, old_active_frame);
gtk_tree_store_set (store, &iter, S_ACTIVE, FALSE, -1);
active_frame_index = gtk_tree_path_get_indices(new_active_frame)[1];
select_frame(active_frame_index);
gtk_tree_model_get_iter(model, &iter, new_active_frame);
gtk_tree_store_set (store, &iter, S_ACTIVE, TRUE, -1);
gtk_tree_path_free(old_active_frame);
}
gtk_tree_path_free(new_active_frame);
}
/*
* shows a tooltip for a file name
*/
static gboolean on_query_tooltip(GtkWidget *widget, gint x, gint y, gboolean keyboard_mode, GtkTooltip *tooltip, gpointer user_data)
{
GtkTreePath *tpath = NULL;
GtkTreeViewColumn *column = NULL;
gboolean show = FALSE;
int bx, by;
gtk_tree_view_convert_widget_to_bin_window_coords(GTK_TREE_VIEW(widget), x, y, &bx, &by);
if (gtk_tree_view_get_path_at_pos(GTK_TREE_VIEW(widget), bx, by, &tpath, &column, NULL, NULL))
{
if (2 == gtk_tree_path_get_depth(tpath))
{
gint start_pos, width;
gtk_tree_view_column_cell_get_position(column, renderer_arrow, &start_pos, &width);
if (column == column_filepath)
{
frame *f;
GtkTreeIter iter;
gtk_tree_model_get_iter(model, &iter, tpath);
gtk_tree_model_get(model, &iter, S_FRAME, &f, -1);
gtk_tooltip_set_text(tooltip, f->file);
gtk_tree_view_set_tooltip_row(GTK_TREE_VIEW(widget), tooltip, tpath);
show = TRUE;
frame_unref(f);
}
else if (column == column_address && bx >= start_pos && bx < start_pos + width)
{
gtk_tooltip_set_text(tooltip, gtk_tree_path_get_indices(tpath)[1] == active_frame_index ? _("Active frame") : _("Click an arrow to switch to a frame"));
gtk_tree_view_set_tooltip_row(GTK_TREE_VIEW(widget), tooltip, tpath);
show = TRUE;
}
}
gtk_tree_path_free(tpath);
}
return show;
}
/*
* shows arrow icon for the frame rows, hides renderer for a thread ones
*/
static void on_render_arrow(GtkTreeViewColumn *tree_column, GtkCellRenderer *cell, GtkTreeModel *tree_model,
GtkTreeIter *iter, gpointer data)
{
GtkTreePath *tpath = gtk_tree_model_get_path(model, iter);
g_object_set(cell, "visible", 1 != gtk_tree_path_get_depth(tpath), NULL);
gtk_tree_path_free(tpath);
}
/*
* empty line renderer text for thread row
*/
static void on_render_line(GtkTreeViewColumn *tree_column, GtkCellRenderer *cell, GtkTreeModel *tree_model,
GtkTreeIter *iter, gpointer data)
{
frame *f;
gtk_tree_model_get (model, iter, S_FRAME, &f, -1);
if (! f)
g_object_set(cell, "text", "", NULL);
else
{
GValue value = G_VALUE_INIT;
g_value_init(&value, G_TYPE_INT);
g_value_set_int (&value, f->line);
g_object_set_property (G_OBJECT (cell), "text", &value);
g_value_unset (&value);
frame_unref (f);
}
}
/*
* shows only the file name instead of a full path
*/
static void on_render_filename(GtkTreeViewColumn *tree_column, GtkCellRenderer *cell, GtkTreeModel *tree_model,
GtkTreeIter *iter, gpointer data)
{
frame *f;
gtk_tree_model_get(model, iter, S_FRAME, &f, -1);
if (! f)
g_object_set(cell, "text", "", NULL);
else
{
gchar *name;
name = f->file ? g_path_get_basename(f->file) : NULL;
g_object_set(cell, "text", name ? name : f->file, NULL);
g_free(name);
frame_unref (f);
}
}
/*
* Handles same tree row click to open frame position
*/
static gboolean on_msgwin_button_press(GtkWidget *widget, GdkEventButton *event, gpointer user_data)
{
if (event->type == GDK_BUTTON_PRESS)
{
GtkTreePath *pressed_path = NULL;
GtkTreeViewColumn *column = NULL;
if (gtk_tree_view_get_path_at_pos(GTK_TREE_VIEW(tree), (int)event->x, (int)event->y, &pressed_path, &column, NULL, NULL))
{
if (2 == gtk_tree_path_get_depth(pressed_path))
{
GtkTreePath *selected_path;
gtk_tree_view_get_cursor(GTK_TREE_VIEW(tree), &selected_path, NULL);
if (selected_path && !gtk_tree_path_compare(pressed_path, selected_path))
{
frame *f;
GtkTreeIter iter;
gtk_tree_model_get_iter (
model,
&iter,
pressed_path);
gtk_tree_model_get (model, &iter, S_FRAME, &f, -1);
/* check if file name is not empty and we have source files for the frame */
if (f->have_source)
{
move_to_line(f->file, f->line);
}
frame_unref(f);
}
if (selected_path)
gtk_tree_path_free(selected_path);
}
gtk_tree_path_free(pressed_path);
}
}
return FALSE;
}
/*
* Tree view cursor changed callback
*/
static void on_cursor_changed(GtkTreeView *treeview, gpointer user_data)
{
GtkTreePath *path;
GtkTreeIter iter;
frame *f;
int thread_id;
gtk_tree_view_get_cursor(treeview, &path, NULL);
if (! path)
return;
gtk_tree_model_get_iter (model, &iter, path);
gtk_tree_model_get (model, &iter,
S_FRAME, &f, S_THREAD_ID, &thread_id, -1);
if (f) /* frame */
{
/* check if file name is not empty and we have source files for the frame */
if (f->have_source)
{
move_to_line(f->file, f->line);
}
frame_unref(f);
}
else /* thread */
{
if (thread_id != active_thread_id)
select_thread(thread_id);
}
gtk_tree_path_free(path);
}
static void on_render_function (GtkTreeViewColumn *tree_column, GtkCellRenderer *cell,
GtkTreeModel *tree_model, GtkTreeIter *iter, gpointer data)
{
frame *f;
gtk_tree_model_get (tree_model, iter, S_FRAME, &f, -1);
if (! f)
g_object_set (cell, "text", "", NULL);
else
{
g_object_set (cell, "text", f->function, NULL);
frame_unref (f);
}
}
static void on_render_address (GtkTreeViewColumn *tree_column, GtkCellRenderer *cell,
GtkTreeModel *tree_model, GtkTreeIter *iter, gpointer data)
{
frame *f;
gtk_tree_model_get (tree_model, iter, S_FRAME, &f, -1);
if (f)
{
g_object_set (cell, "text", f->address, NULL);
frame_unref (f);
}
else
{
gint thread_id;
gchar *thread_label;
gtk_tree_model_get (model, iter, S_THREAD_ID, &thread_id, -1);
thread_label = g_strdup_printf(_("Thread %i"), thread_id);
g_object_set (cell, "text", thread_label, NULL);
g_free(thread_label);
}
}
/*
* inits stack trace tree
*/
GtkWidget* stree_init(move_to_line_cb ml, select_thread_cb st, select_frame_cb sf)
{
GtkTreeViewColumn *column;
GtkCellRenderer *renderer;
move_to_line = ml;
select_thread = st;
select_frame = sf;
/* create tree view */
store = gtk_tree_store_new (
S_N_COLUMNS,
STREE_TYPE_FRAME, /* frame */
G_TYPE_INT /* thread ID */,
G_TYPE_BOOLEAN /* active */);
model = GTK_TREE_MODEL(store);
tree = gtk_tree_view_new_with_model (GTK_TREE_MODEL(store));
g_object_unref(store);
/* set tree view properties */
gtk_tree_view_set_headers_visible(GTK_TREE_VIEW(tree), 1);
gtk_widget_set_has_tooltip(tree, TRUE);
gtk_tree_view_set_show_expanders(GTK_TREE_VIEW(tree), FALSE);
/* connect signals */
g_signal_connect(G_OBJECT(tree), "cursor-changed", G_CALLBACK (on_cursor_changed), NULL);
/* for clicking on already selected frame */
g_signal_connect(G_OBJECT(tree), "button-press-event", G_CALLBACK(on_msgwin_button_press), NULL);
g_signal_connect(G_OBJECT(tree), "query-tooltip", G_CALLBACK (on_query_tooltip), NULL);
/* creating columns */
/* address */
column_address = column = gtk_tree_view_column_new();
gtk_tree_view_column_set_title(column, _("Address"));
renderer_arrow = cell_renderer_frame_icon_new ();
g_object_set(renderer_arrow, "pixbuf_active", (gpointer)frame_current_pixbuf, NULL);
g_object_set(renderer_arrow, "pixbuf_highlighted", (gpointer)frame_pixbuf, NULL);
gtk_tree_view_column_pack_start(column, renderer_arrow, TRUE);
gtk_tree_view_column_set_attributes(column, renderer_arrow, "active_frame", S_ACTIVE, NULL);
gtk_tree_view_column_set_cell_data_func(column, renderer_arrow, on_render_arrow, NULL, NULL);
g_signal_connect (G_OBJECT(renderer_arrow), "clicked", G_CALLBACK(on_frame_arrow_clicked), NULL);
renderer = gtk_cell_renderer_text_new ();
gtk_tree_view_column_pack_start(column, renderer, TRUE);
gtk_tree_view_column_set_cell_data_func(column, renderer, on_render_address, NULL, NULL);
gtk_tree_view_append_column (GTK_TREE_VIEW (tree), column);
/* function */
renderer = gtk_cell_renderer_text_new ();
column = gtk_tree_view_column_new_with_attributes (_("Function"), renderer, NULL);
gtk_tree_view_column_set_cell_data_func(column, renderer, on_render_function, NULL, NULL);
gtk_tree_view_column_set_resizable (column, TRUE);
gtk_tree_view_append_column (GTK_TREE_VIEW (tree), column);
/* file */
renderer = gtk_cell_renderer_text_new ();
column_filepath = column = gtk_tree_view_column_new_with_attributes (_("File"), renderer, NULL);
gtk_tree_view_column_set_resizable (column, TRUE);
gtk_tree_view_append_column (GTK_TREE_VIEW (tree), column);
gtk_tree_view_column_set_cell_data_func(column, renderer, on_render_filename, NULL, NULL);
/* line */
renderer = gtk_cell_renderer_text_new ();
column = gtk_tree_view_column_new_with_attributes (_("Line"), renderer, NULL);
gtk_tree_view_column_set_cell_data_func(column, renderer, on_render_line, NULL, NULL);
gtk_tree_view_column_set_resizable (column, TRUE);
gtk_tree_view_append_column (GTK_TREE_VIEW (tree), column);
/* Last invisible column */
column = gtk_tree_view_column_new ();
gtk_tree_view_append_column (GTK_TREE_VIEW (tree), column);
return tree;
}
/*
* add frames to the tree view
*/
void stree_add(GList *frames)
{
GtkTreeIter thread_iter;
GList *item;
g_object_ref (model);
gtk_tree_view_set_model (GTK_TREE_VIEW (tree), NULL);
find_thread_iter (active_thread_id, &thread_iter);
/* prepending is a *lot* faster than appending, so prepend with a reversed data set */
for (item = g_list_last (frames); item; item = item->prev)
{
gtk_tree_store_insert_with_values (store, NULL, &thread_iter, 0,
S_FRAME, item->data, -1);
}
gtk_tree_view_set_model (GTK_TREE_VIEW (tree), model);
g_object_unref (model);
}
/*
* clear tree view completely
*/
void stree_clear(void)
{
gtk_tree_store_clear(store);
}
/*
* select first frame in the stack
*/
void stree_select_first_frame(gboolean make_active)
{
GtkTreeIter thread_iter, frame_iter;
gtk_tree_view_expand_all(GTK_TREE_VIEW(tree));
if (find_thread_iter (active_thread_id, &thread_iter) &&
gtk_tree_model_iter_children(model, &frame_iter, &thread_iter))
{
GtkTreePath* path;
if (make_active)
{
gtk_tree_store_set (store, &frame_iter, S_ACTIVE, TRUE, -1);
active_frame_index = 0;
}
path = gtk_tree_model_get_path(model, &frame_iter);
gtk_tree_view_set_cursor(GTK_TREE_VIEW(tree), path, NULL, FALSE);
gtk_tree_path_free(path);
}
}
/*
* called on plugin exit to free module data
*/
void stree_destroy(void)
{
}
/*
* add new thread to the tree view
*/
void stree_add_thread(int thread_id)
{
GtkTreeIter thread_iter, new_thread_iter;
if (gtk_tree_model_get_iter_first(model, &thread_iter))
{
GtkTreeIter *consecutive = NULL;
do
{
int existing_thread_id;
gtk_tree_model_get(model, &thread_iter, S_THREAD_ID, &existing_thread_id, -1);
if (existing_thread_id > thread_id)
{
consecutive = &thread_iter;
break;
}
}
while(gtk_tree_model_iter_next(model, &thread_iter));
if(consecutive)
{
gtk_tree_store_prepend(store, &new_thread_iter, consecutive);
}
else
{
gtk_tree_store_append(store, &new_thread_iter, NULL);
}
}
else
{
gtk_tree_store_append (store, &new_thread_iter, NULL);
}
gtk_tree_store_set (store, &new_thread_iter,
S_FRAME, NULL,
S_THREAD_ID, thread_id,
-1);
}
/*
* remove a thread from the tree view
*/
void stree_remove_thread(int thread_id)
{
GtkTreeIter iter;
if (find_thread_iter (thread_id, &iter))
gtk_tree_store_remove(store, &iter);
}
/*
* remove all frames
*/
void stree_remove_frames(void)
{
GtkTreeIter child;
GtkTreeIter thread_iter;
if (find_thread_iter (active_thread_id, &thread_iter) &&
gtk_tree_model_iter_children(model, &child, &thread_iter))
{
while(gtk_tree_store_remove(GTK_TREE_STORE(model), &child))
;
}
}
/*
* set current thread id
*/
void stree_set_active_thread_id(int thread_id)
{
active_thread_id = thread_id;
}
| {
"pile_set_name": "Github"
} |
// Boost.Units - A C++ library for zero-overhead dimensional analysis and
// unit/quantity manipulation and conversion
//
// Copyright (C) 2003-2008 Matthias Christian Schabel
// Copyright (C) 2008 Steven Watanabe
//
// Distributed under the Boost Software License, Version 1.0. (See
// accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
#ifndef BOOST_UNITS_ACCELERATION_DERIVED_DIMENSION_HPP
#define BOOST_UNITS_ACCELERATION_DERIVED_DIMENSION_HPP
#include <boost/units/derived_dimension.hpp>
#include <boost/units/physical_dimensions/length.hpp>
#include <boost/units/physical_dimensions/time.hpp>
namespace boost {
namespace units {
/// derived dimension for acceleration : L T^-2
typedef derived_dimension<length_base_dimension,1,
time_base_dimension,-2>::type acceleration_dimension;
} // namespace units
} // namespace boost
#endif // BOOST_UNITS_ACCELERATION_DERIVED_DIMENSION_HPP
| {
"pile_set_name": "Github"
} |
# Copyright(c) 2017 Google Inc.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may not
# use this file except in compliance with the License. You may obtain a copy of
# the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations under
# the License.
Import-Module -DisableNameChecking ..\..\..\BuildTools.psm1
try {
Push-Location
Set-Location IO.Swagger
$url = "http://localhost:7412"
$job = Run-Kestrel $url
Set-Location ../IO.SwaggerTest
$env:ASPNETCORE_URLS = $url
dotnet test --test-adapter-path:. --logger:junit 2>&1 | %{ "$_" }
} finally {
Stop-Job $job
Receive-Job $job
Remove-Job $job
Pop-Location
} | {
"pile_set_name": "Github"
} |
/*
* Copyright 2006-2009, 2017, 2020 United States Government, as represented by the
* Administrator of the National Aeronautics and Space Administration.
* All rights reserved.
*
* The NASA World Wind Java (WWJ) platform is licensed under the Apache License,
* Version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed
* under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* NASA World Wind Java (WWJ) also contains the following 3rd party Open Source
* software:
*
* Jackson Parser – Licensed under Apache 2.0
* GDAL – Licensed under MIT
* JOGL – Licensed under Berkeley Software Distribution (BSD)
* Gluegen – Licensed under Berkeley Software Distribution (BSD)
*
* A complete listing of 3rd Party software notices and licenses included in
* NASA World Wind Java (WWJ) can be found in the WorldWindJava-v2.2 3rd-party
* notices and licenses PDF found in code directory.
*/
package gov.nasa.worldwindx.examples;
import gov.nasa.worldwind.avlist.AVKey;
import gov.nasa.worldwind.geom.*;
import gov.nasa.worldwind.globes.Globe;
import gov.nasa.worldwind.layers.RenderableLayer;
import gov.nasa.worldwind.render.*;
import gov.nasa.worldwind.util.ContourList;
import gov.nasa.worldwind.util.combine.ShapeCombiner;
/**
* Shows how to use the {@link gov.nasa.worldwind.util.combine.Combinable} interface and the {@link
* gov.nasa.worldwind.util.combine.ShapeCombiner} class to combine WorldWind surface shapes into a complex set of
* contours by using boolean operations.
* <p>
* This example creates two static SurfaceCircle instances that partially overlap and displays them in a layer named
* "Original". A ShapeCombiner is used to combine the two surface circles into a potentially complex set of contours
* using boolean operations. Three examples of such operations are given: union, intersection and difference. The result
* of each operation is displayed in a separate layer beneath the original shapes.
*
* @author dcollins
* @version $Id: ShapeCombining.java 2411 2014-10-30 21:27:00Z dcollins $
*/
public class ShapeCombining extends ApplicationTemplate
{
public static class AppFrame extends ApplicationTemplate.AppFrame
{
public AppFrame()
{
ShapeAttributes attrs = new BasicShapeAttributes();
attrs.setInteriorOpacity(0.5);
attrs.setOutlineWidth(2);
// Create two surface circles that partially overlap, and add them to a layer named "Original".
SurfaceCircle shape1 = new SurfaceCircle(attrs, LatLon.fromDegrees(50, -105), 500000);
SurfaceCircle shape2 = new SurfaceCircle(attrs, LatLon.fromDegrees(50, -100), 500000);
shape1.setValue(AVKey.DISPLAY_NAME, "Original");
shape2.setValue(AVKey.DISPLAY_NAME, "Original");
RenderableLayer originalLayer = new RenderableLayer();
originalLayer.setName("Original");
originalLayer.addRenderable(shape1);
originalLayer.addRenderable(shape2);
this.getWwd().getModel().getLayers().add(originalLayer);
// Set up a ShapeCombiner to combine the two surface circles into a potentially complex set of contours
// using boolean operations: union, intersection and difference.
Globe globe = this.getWwd().getModel().getGlobe();
double resolutionRadians = 10000 / globe.getRadius(); // 10km resolution
ShapeCombiner shapeCombiner = new ShapeCombiner(globe, resolutionRadians);
// Compute the union of the two surface circles. Display the result 10 degrees beneath the original.
ContourList union = shapeCombiner.union(shape1, shape2);
this.displayContours(union, "Union", Position.fromDegrees(-10, 0, 0));
// Compute the intersection of the two surface circles. Display the result 20 degrees beneath the original.
ContourList intersection = shapeCombiner.intersection(shape1, shape2);
this.displayContours(intersection, "Intersection", Position.fromDegrees(-20, 0, 0));
// Compute the difference of the two surface circles. Display the result 30 degrees beneath the original.
ContourList difference = shapeCombiner.difference(shape1, shape2);
this.displayContours(difference, "Difference", Position.fromDegrees(-30, 0, 0));
}
protected void displayContours(ContourList contours, String displayName, Position offset)
{
ShapeAttributes attrs = new BasicShapeAttributes();
attrs.setInteriorMaterial(Material.CYAN);
attrs.setInteriorOpacity(0.5);
attrs.setOutlineMaterial(Material.WHITE);
attrs.setOutlineWidth(2);
SurfaceMultiPolygon shape = new SurfaceMultiPolygon(attrs, contours);
shape.setValue(AVKey.DISPLAY_NAME, displayName);
shape.setPathType(AVKey.LINEAR);
shape.move(offset);
RenderableLayer layer = new RenderableLayer();
layer.setName(displayName);
layer.addRenderable(shape);
this.getWwd().getModel().getLayers().add(layer);
}
}
public static void main(String[] args)
{
start("WorldWind Shape Combining", AppFrame.class);
}
}
| {
"pile_set_name": "Github"
} |
CODE_SIGN_IDENTITY =
CONFIGURATION_BUILD_DIR = ${PODS_CONFIGURATION_BUILD_DIR}/Moya-macOS
FRAMEWORK_SEARCH_PATHS = $(inherited) "${PODS_CONFIGURATION_BUILD_DIR}/Alamofire-macOS" "${PODS_CONFIGURATION_BUILD_DIR}/ReactiveSwift-macOS" "${PODS_CONFIGURATION_BUILD_DIR}/Result-macOS" "${PODS_CONFIGURATION_BUILD_DIR}/RxAtomic-macOS" "${PODS_CONFIGURATION_BUILD_DIR}/RxSwift-macOS"
GCC_PREPROCESSOR_DEFINITIONS = $(inherited) COCOAPODS=1
OTHER_SWIFT_FLAGS = $(inherited) -D COCOAPODS
PODS_BUILD_DIR = ${BUILD_DIR}
PODS_CONFIGURATION_BUILD_DIR = ${PODS_BUILD_DIR}/$(CONFIGURATION)$(EFFECTIVE_PLATFORM_NAME)
PODS_ROOT = ${SRCROOT}
PODS_TARGET_SRCROOT = ${PODS_ROOT}/Moya
PRODUCT_BUNDLE_IDENTIFIER = org.cocoapods.${PRODUCT_NAME:rfc1034identifier}
SKIP_INSTALL = YES
| {
"pile_set_name": "Github"
} |
using UnityEngine;
using UnityEditor;
namespace Lockstep
{
[CustomPropertyDrawer(typeof(PathObjectAttribute))]
public class EditorPathObject : UnityEditor.PropertyDrawer
{
const float defaultPropertyHeight = 16f;
public override float GetPropertyHeight(SerializedProperty property, GUIContent label)
{
return defaultPropertyHeight;
}
public override void OnGUI(Rect position, SerializedProperty property, GUIContent label)
{
PathObjectAttribute Atb = attribute as PathObjectAttribute;
SerializedProperty prefabNameProp = property.FindPropertyRelative("_path");
//SerializedProperty prefabProp = property.FindPropertyRelative ("_editorPrefab");
//string lastName = prefabNameProp.stringValue;
UnityEngine.Object obj = PathObjectFactory.Load(prefabNameProp.stringValue) as UnityEngine.Object;
obj = (UnityEngine.Object)EditorGUI.ObjectField(position, label, obj, Atb.ObjectType, false);
string relativePath = "";
if (obj != null)
{
if (PathObjectUtility.TryGetPath(obj, out relativePath) == false)
{
Debug.LogErrorFormat("Object '{0}' is not detected to be in a Resources folder.", obj);
return;
}
}
prefabNameProp.stringValue = obj != null ? relativePath : "";
/*if (lastName != prefabNameProp.stringValue) {
if (PathPrefabFactory.Load (prefabNameProp.stringValue) == null) {
prefabProp.objectReferenceValue = null;
}
}*/
}
}
} | {
"pile_set_name": "Github"
} |
// Boost.Units - A C++ library for zero-overhead dimensional analysis and
// unit/quantity manipulation and conversion
//
// Copyright (C) 2003-2008 Matthias Christian Schabel
// Copyright (C) 2008 Steven Watanabe
//
// Distributed under the Boost Software License, Version 1.0. (See
// accompanying file LICENSE_1_0.txt or copy at
// http://www.boost.org/LICENSE_1_0.txt)
#ifndef BOOST_UNITS_PRESSURE_DERIVED_DIMENSION_HPP
#define BOOST_UNITS_PRESSURE_DERIVED_DIMENSION_HPP
#include <boost/units/derived_dimension.hpp>
#include <boost/units/physical_dimensions/length.hpp>
#include <boost/units/physical_dimensions/mass.hpp>
#include <boost/units/physical_dimensions/time.hpp>
namespace boost {
namespace units {
/// derived dimension for pressure : L^-1 M T^-2
typedef derived_dimension<length_base_dimension,-1,
mass_base_dimension,1,
time_base_dimension,-2>::type pressure_dimension;
} // namespace units
} // namespace boost
#endif // BOOST_UNITS_PRESSURE_DERIVED_DIMENSION_HPP
| {
"pile_set_name": "Github"
} |
{ config, pkgs, lib, ... }:
with lib;
let
cfg = config.services.jackett;
in
{
options = {
services.jackett = {
enable = mkEnableOption "Jackett";
dataDir = mkOption {
type = types.str;
default = "/var/lib/jackett/.config/Jackett";
description = "The directory where Jackett stores its data files.";
};
openFirewall = mkOption {
type = types.bool;
default = false;
description = "Open ports in the firewall for the Jackett web interface.";
};
user = mkOption {
type = types.str;
default = "jackett";
description = "User account under which Jackett runs.";
};
group = mkOption {
type = types.str;
default = "jackett";
description = "Group under which Jackett runs.";
};
package = mkOption {
type = types.package;
default = pkgs.jackett;
defaultText = "pkgs.jackett";
description = "Jackett package to use.";
};
};
};
config = mkIf cfg.enable {
systemd.tmpfiles.rules = [
"d '${cfg.dataDir}' 0700 ${cfg.user} ${cfg.group} - -"
];
systemd.services.jackett = {
description = "Jackett";
after = [ "network.target" ];
wantedBy = [ "multi-user.target" ];
serviceConfig = {
Type = "simple";
User = cfg.user;
Group = cfg.group;
ExecStart = "${cfg.package}/bin/Jackett --NoUpdates --DataFolder '${cfg.dataDir}'";
Restart = "on-failure";
};
};
networking.firewall = mkIf cfg.openFirewall {
allowedTCPPorts = [ 9117 ];
};
users.users = mkIf (cfg.user == "jackett") {
jackett = {
group = cfg.group;
home = cfg.dataDir;
uid = config.ids.uids.jackett;
};
};
users.groups = mkIf (cfg.group == "jackett") {
jackett.gid = config.ids.gids.jackett;
};
};
}
| {
"pile_set_name": "Github"
} |
; RUN: opt < %s -instcombine -S | FileCheck %s
; <rdar://problem/10803154>
; There should be no transformation.
; CHECK: %a = trunc i32 %x to i8
; CHECK: %b = icmp ne i8 %a, 0
; CHECK: %c = and i32 %x, 16711680
; CHECK: %d = icmp ne i32 %c, 0
; CHECK: %e = and i1 %b, %d
; CHECK: ret i1 %e
define i1 @f1(i32 %x) {
%a = trunc i32 %x to i8
%b = icmp ne i8 %a, 0
%c = and i32 %x, 16711680
%d = icmp ne i32 %c, 0
%e = and i1 %b, %d
ret i1 %e
}
| {
"pile_set_name": "Github"
} |
from __future__ import unicode_literals
from __future__ import print_function
from threading import Thread, RLock, Event
from datetime import datetime, timedelta
from operator import attrgetter
import logging
log = logging.getLogger("moya.runtime")
class Task(object):
"""A task scheduled for a given time"""
def __init__(self, name, callable, run_time=None, repeat=None):
self.name = name
self.callable = callable
self.run_time = run_time
self.repeat = repeat
def __unicode__(self):
if self.repeat:
return "<task '{}' repeats {}>".format(self.name, self.repeat)
else:
return "<task '{}'>".format(self.name)
__repr__ = __unicode__
def advance(self):
"""Set run time to next cycle"""
if self.repeat:
self.run_time = self.run_time + self.repeat
return True
else:
return False
def ready(self, t):
"""Check if this task is ready to be execute"""
return t >= self.run_time
def run(self):
"""Run the task in it's own thread"""
run_thread = Thread(target=self._run)
run_thread.start()
def _run(self):
try:
self.callable()
except Exception as e:
log.exception("Error in scheduled task '%s'", self.name)
class Scheduler(Thread):
"""A thread that manages scheduled / repeating tasks"""
# The granularity at which tasks can be scheduled
# Super precision is not required given that tasks are unlikely to be more than one a minute
poll_seconds = 1
def __init__(self):
super(Scheduler, self).__init__()
self.lock = RLock()
self.quit_event = Event()
self.tasks = []
self.new_tasks = []
def run(self):
while 1:
# Better than a sleep because we can interrupt it
self.quit_event.wait(self.poll_seconds)
if self.quit_event.is_set():
break
with self.lock:
self.tasks += self.new_tasks
del self.new_tasks[:]
self._check_tasks()
del self.tasks[:]
with self.lock:
del self.new_tasks[:]
def stop(self, wait=True):
"""Stop processing tasks and block till everything has quit"""
self.quit_event.set()
if wait:
self.join()
def _check_tasks(self):
if not self.tasks:
return
t = datetime.utcnow()
ready_tasks = None
self.tasks.sort(key=attrgetter("run_time"))
for i, task in enumerate(self.tasks):
if not task.ready(t):
ready_tasks = self.tasks[:i]
del self.tasks[:i]
break
else:
ready_tasks = self.tasks[:]
del self.tasks[:]
if ready_tasks:
for task in ready_tasks:
if self.quit_event.is_set():
break
task.run()
if task.advance():
self.tasks.append(task)
def add_repeat_task(self, name, callable, repeat):
"""Add a task to be invoked every `repeat` seconds"""
if isinstance(repeat, (int, long, float)):
repeat = timedelta(seconds=repeat)
run_time = datetime.utcnow() + repeat
task = Task(name, callable, run_time=run_time, repeat=repeat)
with self.lock:
self.new_tasks.append(task)
return task
if __name__ == "__main__":
import time
def hello():
print("Hello")
def world():
print("world")
scheduler = Scheduler()
print(scheduler.add_repeat_task("hello", hello, repeat=5))
print(scheduler.add_repeat_task("world", world, repeat=3))
scheduler.start()
try:
while 1:
time.sleep(1)
except:
scheduler.stop()
print("Stopped")
raise
| {
"pile_set_name": "Github"
} |
<?php
/*
* This file is part of PHPExifTool.
*
* (c) 2012 Romain Neutron <[email protected]>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace PHPExiftool\Driver\Tag\FLIR;
use JMS\Serializer\Annotation\ExclusionPolicy;
use PHPExiftool\Driver\AbstractTag;
/**
* @ExclusionPolicy("all")
*/
class DetectorBoard extends AbstractTag
{
protected $Id = 4;
protected $Name = 'DetectorBoard';
protected $FullName = 'FLIR::Parts';
protected $GroupName = 'FLIR';
protected $g0 = 'MakerNotes';
protected $g1 = 'FLIR';
protected $g2 = 'Camera';
protected $Type = 'undef';
protected $Writable = false;
protected $Description = 'Detector Board';
protected $flag_Permanent = true;
protected $Index = 5;
}
| {
"pile_set_name": "Github"
} |
//===- llvm/Assembly/PrintModulePass.h - Printing Pass ----------*- C++ -*-===//
//
// The LLVM Compiler Infrastructure
//
// This file is distributed under the University of Illinois Open Source
// License. See LICENSE.TXT for details.
//
//===----------------------------------------------------------------------===//
//
// This file defines two passes to print out a module. The PrintModulePass pass
// simply prints out the entire module when it is executed. The
// PrintFunctionPass class is designed to be pipelined with other
// FunctionPass's, and prints out the functions of the module as they are
// processed.
//
//===----------------------------------------------------------------------===//
#ifndef LLVM_ASSEMBLY_PRINTMODULEPASS_H
#define LLVM_ASSEMBLY_PRINTMODULEPASS_H
#include <string>
namespace llvm {
class FunctionPass;
class ModulePass;
class raw_ostream;
/// createPrintModulePass - Create and return a pass that writes the
/// module to the specified raw_ostream.
ModulePass *createPrintModulePass(raw_ostream *OS,
bool DeleteStream=false,
const std::string &Banner = "");
/// createPrintFunctionPass - Create and return a pass that prints
/// functions to the specified raw_ostream as they are processed.
FunctionPass *createPrintFunctionPass(const std::string &Banner,
raw_ostream *OS,
bool DeleteStream=false);
} // End llvm namespace
#endif
| {
"pile_set_name": "Github"
} |
/* xoreos - A reimplementation of BioWare's Aurora engine
*
* xoreos is the legal property of its developers, whose names
* can be found in the AUTHORS file distributed with this source
* distribution.
*
* xoreos is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 3
* of the License, or (at your option) any later version.
*
* xoreos is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with xoreos. If not, see <http://www.gnu.org/licenses/>.
*/
/** @file
* A container of The Witcher objects.
*/
#ifndef ENGINES_WITCHER_OBJECTCONTAINER_H
#define ENGINES_WITCHER_OBJECTCONTAINER_H
#include <list>
#include <map>
#include "src/common/types.h"
#include "src/aurora/nwscript/objectcontainer.h"
#include "src/engines/witcher/types.h"
namespace Engines {
namespace Witcher {
class Object;
class Module;
class Area;
class Waypoint;
class Situated;
class Placeable;
class Door;
class Creature;
class Location;
/** A class able to sort objects by distance to a target object. */
class ObjectDistanceSort {
public:
ObjectDistanceSort(const Witcher::Object &target);
bool operator()(Witcher::Object *a, Witcher::Object *b);
private:
float xt, yt, zt;
float getDistance(Witcher::Object &a);
};
class ObjectContainer : public ::Aurora::NWScript::ObjectContainer {
public:
ObjectContainer();
~ObjectContainer();
void clearObjects();
/** Add an object to this container. */
void addObject(Witcher::Object &object);
/** Remove an object from this container. */
void removeObject(Witcher::Object &object);
/** Return the first object of this type. */
::Aurora::NWScript::Object *getFirstObjectByType(ObjectType type) const;
/** Return a search context to iterate over all objects of this type. */
::Aurora::NWScript::ObjectSearch *findObjectsByType(ObjectType type) const;
static Witcher::Object *toObject(::Aurora::NWScript::Object *object);
static Module *toModule (Aurora::NWScript::Object *object);
static Area *toArea (Aurora::NWScript::Object *object);
static Waypoint *toWaypoint (Aurora::NWScript::Object *object);
static Situated *toSituated (Aurora::NWScript::Object *object);
static Placeable *toPlaceable(Aurora::NWScript::Object *object);
static Door *toDoor (Aurora::NWScript::Object *object);
static Creature *toCreature (Aurora::NWScript::Object *object);
static Creature *toPC (Aurora::NWScript::Object *object);
static Location *toLocation(Aurora::NWScript::EngineType *engineType);
private:
typedef std::list<Witcher::Object *> ObjectList;
typedef std::map<ObjectType, ObjectList> ObjectMap;
ObjectMap _objects;
};
} // End of namespace Witcher
} // End of namespace Engines
#endif // ENGINES_WITCHER_OBJECTCONTAINER_H
| {
"pile_set_name": "Github"
} |
/****************************************************************************
**
** Copyright (C) 2016 The Qt Company Ltd.
** Contact: http://www.qt.io/licensing/
**
** This file is part of the Qt Quick Controls 2 module of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:LGPL3$
** Commercial License Usage
** Licensees holding valid commercial Qt licenses may use this file in
** accordance with the commercial license agreement provided with the
** Software or, alternatively, in accordance with the terms contained in
** a written agreement between you and The Qt Company. For licensing terms
** and conditions see http://www.qt.io/terms-conditions. For further
** information use the contact form at http://www.qt.io/contact-us.
**
** GNU Lesser General Public License Usage
** Alternatively, this file may be used under the terms of the GNU Lesser
** General Public License version 3 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPLv3 included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 3 requirements
** will be met: https://www.gnu.org/licenses/lgpl.html.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 2.0 or later as published by the Free
** Software Foundation and appearing in the file LICENSE.GPL included in
** the packaging of this file. Please review the following information to
** ensure the GNU General Public License version 2.0 requirements will be
** met: http://www.gnu.org/licenses/gpl-2.0.html.
**
** $QT_END_LICENSE$
**
****************************************************************************/
import QtQuick 2.6
import QtQuick.Templates 2.0 as T
import QtQuick.Controls.Universal 2.0
T.TabButton {
id: control
implicitWidth: Math.max(background ? background.implicitWidth : 0,
contentItem.implicitWidth + leftPadding + rightPadding)
implicitHeight: Math.max(background ? background.implicitHeight : 0,
contentItem.implicitHeight + topPadding + bottomPadding)
baselineOffset: contentItem.y + contentItem.baselineOffset
padding: 12 // PivotItemMargin
contentItem: Text {
text: control.text
font: control.font
elide: Text.ElideRight
horizontalAlignment: Text.AlignHCenter
verticalAlignment: Text.AlignVCenter
opacity: control.checked || control.down ? 1.0 : 0.2
color: control.Universal.foreground
}
}
| {
"pile_set_name": "Github"
} |
{
"_args": [
[
{
"raw": "os-homedir@^1.0.0",
"scope": null,
"escapedName": "os-homedir",
"name": "os-homedir",
"rawSpec": "^1.0.0",
"spec": ">=1.0.0 <2.0.0",
"type": "range"
},
"/Users/chengwei/kafka-doc-zh/doc/zh/node_modules/tildify"
]
],
"_from": "os-homedir@>=1.0.0 <2.0.0",
"_id": "[email protected]",
"_inCache": true,
"_location": "/os-homedir",
"_nodeVersion": "6.6.0",
"_npmOperationalInternal": {
"host": "packages-16-east.internal.npmjs.com",
"tmp": "tmp/os-homedir-1.0.2.tgz_1475211519628_0.7873868853785098"
},
"_npmUser": {
"name": "sindresorhus",
"email": "[email protected]"
},
"_npmVersion": "3.10.3",
"_phantomChildren": {},
"_requested": {
"raw": "os-homedir@^1.0.0",
"scope": null,
"escapedName": "os-homedir",
"name": "os-homedir",
"rawSpec": "^1.0.0",
"spec": ">=1.0.0 <2.0.0",
"type": "range"
},
"_requiredBy": [
"/tildify"
],
"_resolved": "https://registry.npmjs.org/os-homedir/-/os-homedir-1.0.2.tgz",
"_shasum": "ffbc4988336e0e833de0c168c7ef152121aa7fb3",
"_shrinkwrap": null,
"_spec": "os-homedir@^1.0.0",
"_where": "/Users/chengwei/kafka-doc-zh/doc/zh/node_modules/tildify",
"author": {
"name": "Sindre Sorhus",
"email": "[email protected]",
"url": "sindresorhus.com"
},
"bugs": {
"url": "https://github.com/sindresorhus/os-homedir/issues"
},
"dependencies": {},
"description": "Node.js 4 `os.homedir()` ponyfill",
"devDependencies": {
"ava": "*",
"path-exists": "^2.0.0",
"xo": "^0.16.0"
},
"directories": {},
"dist": {
"shasum": "ffbc4988336e0e833de0c168c7ef152121aa7fb3",
"tarball": "https://registry.npmjs.org/os-homedir/-/os-homedir-1.0.2.tgz"
},
"engines": {
"node": ">=0.10.0"
},
"files": [
"index.js"
],
"gitHead": "b1b0ae70a5965fef7005ff6509a5dd1a78c95e36",
"homepage": "https://github.com/sindresorhus/os-homedir#readme",
"keywords": [
"builtin",
"core",
"ponyfill",
"polyfill",
"shim",
"os",
"homedir",
"home",
"dir",
"directory",
"folder",
"user",
"path"
],
"license": "MIT",
"maintainers": [
{
"name": "sindresorhus",
"email": "[email protected]"
}
],
"name": "os-homedir",
"optionalDependencies": {},
"readme": "ERROR: No README data found!",
"repository": {
"type": "git",
"url": "git+https://github.com/sindresorhus/os-homedir.git"
},
"scripts": {
"test": "xo && ava"
},
"version": "1.0.2"
}
| {
"pile_set_name": "Github"
} |
---
title: "Delete androidStoreApp"
description: "Deletes a androidStoreApp."
author: "dougeby"
localization_priority: Normal
ms.prod: "intune"
doc_type: apiPageType
---
# Delete androidStoreApp
Namespace: microsoft.graph
> **Important:** Microsoft Graph APIs under the /beta version are subject to change; production use is not supported.
> **Note:** The Microsoft Graph API for Intune requires an [active Intune license](https://go.microsoft.com/fwlink/?linkid=839381) for the tenant.
Deletes a [androidStoreApp](../resources/intune-apps-androidstoreapp.md).
## Prerequisites
One of the following permissions is required to call this API. To learn more, including how to choose permissions, see [Permissions](/graph/permissions-reference).
|Permission type|Permissions (from most to least privileged)|
|:---|:---|
|Delegated (work or school account)|DeviceManagementApps.ReadWrite.All|
|Delegated (personal Microsoft account)|Not supported.|
|Application|DeviceManagementApps.ReadWrite.All|
## HTTP Request
<!-- {
"blockType": "ignored"
}
-->
``` http
DELETE /deviceAppManagement/mobileApps/{mobileAppId}
DELETE /deviceAppManagement/mobileApps/{mobileAppId}/userStatuses/{userAppInstallStatusId}/app
DELETE /deviceAppManagement/mobileApps/{mobileAppId}/deviceStatuses/{mobileAppInstallStatusId}/app
```
## Request headers
|Header|Value|
|:---|:---|
|Authorization|Bearer <token> Required.|
|Accept|application/json|
## Request body
Do not supply a request body for this method.
## Response
If successful, this method returns a `204 No Content` response code.
## Example
### Request
Here is an example of the request.
``` http
DELETE https://graph.microsoft.com/beta/deviceAppManagement/mobileApps/{mobileAppId}
```
### Response
Here is an example of the response. Note: The response object shown here may be truncated for brevity. All of the properties will be returned from an actual call.
``` http
HTTP/1.1 204 No Content
```
| {
"pile_set_name": "Github"
} |
// +build integration
package integration
import (
"fmt"
"os"
"testing"
"github.com/apex/log"
"github.com/apex/log/handlers/cli"
"github.com/aws/aws-sdk-go/aws"
"github.com/stretchr/testify/assert"
"github.com/versent/unicreds"
)
func init() {
log.SetHandler(cli.Default)
log.SetLevel(log.DebugLevel)
}
func TestIntegrationGetSecret(t *testing.T) {
var err error
region := os.Getenv("AWS_REGION")
if region == "" {
region = "us-west-2"
}
alias := os.Getenv("UNICREDS_KEY_ALIAS")
if alias == "" {
alias = "alias/unicreds"
}
tableName := os.Getenv("UNICREDS_TABLE_NAME")
if tableName == "" {
tableName = "credential-store"
}
unicreds.SetAwsConfig(aws.String(region), nil)
encContext := unicreds.NewEncryptionContextValue()
(*encContext)["test"] = aws.String("123")
for i := 0; i < 15; i++ {
err = unicreds.PutSecret(aws.String(tableName), alias, "Integration1", fmt.Sprintf("secret%d", i), unicreds.PaddedInt(i), encContext)
if err != nil {
log.Errorf("put err: %v", err)
}
assert.Nil(t, err)
}
cred, err := unicreds.GetHighestVersionSecret(aws.String(tableName), "Integration1", encContext)
assert.Nil(t, err)
assert.Equal(t, cred.Name, "Integration1")
assert.Equal(t, cred.Secret, "secret14")
assert.NotZero(t, cred.CreatedAt)
assert.NotZero(t, cred.Version)
for i := 0; i < 15; i++ {
cred, err := unicreds.GetSecret(aws.String(tableName), "Integration1", unicreds.PaddedInt(i), encContext)
assert.Nil(t, err)
assert.Equal(t, cred.Name, "Integration1")
assert.Equal(t, cred.Secret, fmt.Sprintf("secret%d", i))
assert.NotZero(t, cred.CreatedAt)
assert.Equal(t, cred.Version, unicreds.PaddedInt(i))
}
creds, err := unicreds.GetAllSecrets(aws.String(tableName), true)
assert.Nil(t, err)
assert.Len(t, creds, 15)
// change the context and ensure this triggers an error
(*encContext)["test"] = aws.String("345")
cred, err = unicreds.GetHighestVersionSecret(aws.String(tableName), "Integration1", encContext)
assert.Error(t, err)
assert.Nil(t, cred)
err = unicreds.DeleteSecret(aws.String(tableName), "Integration1")
assert.Nil(t, err)
}
| {
"pile_set_name": "Github"
} |
<p>
<em>This section describes the status of this document at the time of its publication. Other
documents may supersede this document. A list of current
<abbr title="World Wide Web Consortium">W3C</abbr> publications and the latest revision of this
technical report can be found in the
<a href="http://www.w3.org/TR/"><abbr title="World Wide Web Consortium">W3C</abbr> technical
reports index</a> at http://www.w3.org/TR/.</em>
</p>
<p>
This document was published by the <a href="https://www.w3.org/webauthn/">Web Authentication Working Group</a>
as an Editors' Draft. This document is intended to become a W3C Recommendation.
Feedback and comments on this specification are welcome. Please use
<a href="https://github.com/w3c/webauthn/issues">Github issues</a>.
Discussions may also be found in the
<a href="http://lists.w3.org/Archives/Public/public-webauthn/">[email protected] archives</a>.
</p>
<p>
Publication as an Editors' Draft does not imply endorsement by the
<abbr title="World Wide Web Consortium">W3C</abbr> Membership. This is a draft document and may
be updated, replaced or obsoleted by other documents at any time. It is inappropriate to cite
this document as other than work in progress.
</p>
<p>
This document was produced by a group operating under the
<a href="http://www.w3.org/Consortium/Patent-Policy/">
<abbr title="World Wide Web Consortium">W3C</abbr> Patent Policy</a>.
<abbr title="World Wide Web Consortium">W3C</abbr> maintains a
<a href="https://www.w3.org/2004/01/pp-impl/87227/status" rel="disclosure">public list of any
patent disclosures</a> made in connection with the deliverables of the group; that page also
includes instructions for disclosing a patent. An individual who has actual knowledge of a
patent which the individual believes contains
<a href="http://www.w3.org/Consortium/Patent-Policy/#def-essential">Essential
Claim(s)</a> must disclose the information in accordance with
<a href="http://www.w3.org/Consortium/Patent-Policy/#sec-Disclosure">section 6 of the
<abbr title="World Wide Web Consortium">W3C</abbr> Patent Policy</a>.
</p>
<p>
This document is governed by the <a id="w3c_process_revision" href="https://www.w3.org/2020/Process-20200915/">15 September 2020 W3C Process Document</a>.
</p>
<p>[STATUSTEXT]
| {
"pile_set_name": "Github"
} |
https://github.com/easymotion/vim-easymotion.git
| {
"pile_set_name": "Github"
} |
**This airport has been automatically generated**
We have no information about VG33[*] airport other than its name, ICAO and location (US).
This airport will have to be done from scratch, which includes adding runways, taxiways, parking locations, boundaries...
Good luck if you decide to do this airport! | {
"pile_set_name": "Github"
} |
% Generated by roxygen2: do not edit by hand
% Please edit documentation in R/ncdc_datasets.r
\name{ncdc_datasets}
\alias{ncdc_datasets}
\title{Search NOAA datasets}
\usage{
ncdc_datasets(
datasetid = NULL,
datatypeid = NULL,
stationid = NULL,
locationid = NULL,
startdate = NULL,
enddate = NULL,
sortfield = NULL,
sortorder = NULL,
limit = 25,
offset = NULL,
token = NULL,
...
)
}
\arguments{
\item{datasetid}{(optional) Accepts a single valid dataset id. Data returned
will be from the dataset specified.}
\item{datatypeid}{Accepts a valid data type id or a vector or list of data
type ids. (optional)}
\item{stationid}{Accepts a valid station id or a vector or list of station
ids}
\item{locationid}{Accepts a valid location id or a vector or list of
location ids (optional)}
\item{startdate}{(optional) Accepts valid ISO formated date (yyyy-mm-dd) or date time
(YYYY-MM-DDThh:mm:ss). Data returned will have data after the specified date. The
date range must be less than 1 year.}
\item{enddate}{(optional) Accepts valid ISO formated date (yyyy-mm-dd) or date time
(YYYY-MM-DDThh:mm:ss). Data returned will have data before the specified date. The
date range must be less than 1 year.}
\item{sortfield}{The field to sort results by. Supports id, name, mindate,
maxdate, and datacoverage fields (optional)}
\item{sortorder}{Which order to sort by, asc or desc. Defaults to
asc (optional)}
\item{limit}{Defaults to 25, limits the number of results in the response.
Maximum is 1000 (optional)}
\item{offset}{Defaults to 0, used to offset the resultlist (optional)}
\item{token}{This must be a valid token token supplied to you by NCDC's
Climate Data Online access token generator. (required) See
\strong{Authentication} section below for more details.}
\item{...}{Curl options passed on to \code{\link[crul]{HttpClient}}
(optional)}
}
\value{
A data.frame for all datasets, or a list of length two, each with
a data.frame.
}
\description{
From the NOAA API docs: All of our data are in datasets. To retrieve
any data from us, you must know what dataset it is in.
}
\section{Authentication}{
Get an API key (aka, token) at \url{https://www.ncdc.noaa.gov/cdo-web/token}.
You can pass your token in as an argument or store it one of two places:
\itemize{
\item your .Rprofile file with the entry
\code{options(noaakey = "your-noaa-token")}
\item your .Renviron file with the entry
\code{NOAA_KEY=your-noaa-token}
}
See \code{\link{Startup}} for information on how to create/find your
.Rrofile and .Renviron files
}
\examples{
\dontrun{
# Get a table of all datasets
ncdc_datasets()
# Get details from a particular dataset
ncdc_datasets(datasetid='ANNUAL')
# Get datasets with Temperature at the time of observation (TOBS) data type
ncdc_datasets(datatypeid='TOBS')
## two datatypeid's
ncdc_datasets(datatypeid=c('TOBS', "ACMH"))
# Get datasets with data for a series of the same parameter arg, in this case
# stationid's
ncdc_datasets(stationid='COOP:310090')
ncdc_datasets(stationid=c('COOP:310090','COOP:310184','COOP:310212'))
# Multiple datatypeid's
ncdc_datasets(datatypeid=c('ACMC','ACMH','ACSC'))
ncdc_datasets(datasetid='ANNUAL', datatypeid=c('ACMC','ACMH','ACSC'))
ncdc_datasets(datasetid='GSOY', datatypeid=c('ACMC','ACMH','ACSC'))
# Multiple locationid's
ncdc_datasets(locationid="FIPS:30091")
ncdc_datasets(locationid=c("FIPS:30103", "FIPS:30091"))
}
}
\references{
\url{https://www.ncdc.noaa.gov/cdo-web/webservices/v2}
}
\seealso{
Other ncdc:
\code{\link{ncdc_combine}()},
\code{\link{ncdc_datacats}()},
\code{\link{ncdc_datatypes}()},
\code{\link{ncdc_locs_cats}()},
\code{\link{ncdc_locs}()},
\code{\link{ncdc_plot}()},
\code{\link{ncdc_stations}()},
\code{\link{ncdc}()}
}
\concept{ncdc}
| {
"pile_set_name": "Github"
} |
<?php
/**
* Zend Framework
*
* LICENSE
*
* This source file is subject to the new BSD license that is bundled
* with this package in the file LICENSE.txt.
* It is also available through the world-wide-web at this URL:
* http://framework.zend.com/license/new-bsd
* If you did not receive a copy of the license and are unable to
* obtain it through the world-wide-web, please send an email
* to [email protected] so we can send you a copy immediately.
*
* @category Zend
* @package Zend_XmlRpc
* @subpackage Value
* @copyright Copyright (c) 2005-2012 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
* @version $Id: BigInteger.php 24594 2012-01-05 21:27:01Z matthew $
*/
/**
* Zend_XmlRpc_Value_Integer
*/
require_once 'Zend/XmlRpc/Value/Integer.php';
/**
* @category Zend
* @package Zend_XmlRpc
* @subpackage Value
* @copyright Copyright (c) 2005-2012 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
class Zend_XmlRpc_Value_BigInteger extends Zend_XmlRpc_Value_Integer
{
/**
* @param mixed $value
*/
public function __construct($value)
{
require_once 'Zend/Crypt/Math/BigInteger.php';
$integer = new Zend_Crypt_Math_BigInteger;
$this->_value = $integer->init($value);
$this->_type = self::XMLRPC_TYPE_I8;
}
/**
* Return bigint value
*
* @return string
*/
public function getValue()
{
return $this->_value;
}
} | {
"pile_set_name": "Github"
} |
# encoding: utf-8
module ThemesForRails
class << self
def config
@config ||= ThemesForRails::Config.new
yield(@config) if block_given?
@config
end
def available_themes(&block)
Dir.glob(File.join(config.themes_path, "*"), &block)
end
alias each_theme_dir available_themes
def available_theme_names
available_themes.map {|theme| File.basename(theme) }
end
def add_themes_path_to_sass
if ThemesForRails.config.sass_is_available?
each_theme_dir do |dir|
if File.directory?(dir) # Need to get rid of the '.' and '..'
sass_dir = "#{dir}/stylesheets/sass"
css_dir = "#{dir}/stylesheets"
unless already_configured_in_sass?(sass_dir)
Sass::Plugin.add_template_location sass_dir, css_dir
end
end
end
else
raise "Sass is not available. What are you trying to do?"
end
end
def already_configured_in_sass?(sass_dir)
Sass::Plugin.template_location_array.map(&:first).include?(sass_dir)
end
end
end
require 'active_support/dependencies'
require 'themes_for_rails/interpolation'
require 'themes_for_rails/config'
require 'themes_for_rails/common_methods'
require 'themes_for_rails/url_helpers'
require 'themes_for_rails/action_view'
require 'themes_for_rails/assets_controller'
require 'themes_for_rails/action_controller'
require 'themes_for_rails/action_mailer'
require 'themes_for_rails/railtie'
require 'themes_for_rails/routes'
| {
"pile_set_name": "Github"
} |
<?php
/*
* This file is part of the Symfony package.
*
* (c) Fabien Potencier <[email protected]>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Symfony\Component\BrowserKit;
/**
* Request object.
*
* @author Fabien Potencier <[email protected]>
*
* @api
*/
class Request
{
protected $uri;
protected $method;
protected $parameters;
protected $files;
protected $cookies;
protected $server;
protected $content;
/**
* Constructor.
*
* @param string $uri The request URI
* @param string $method The HTTP method request
* @param array $parameters The request parameters
* @param array $files An array of uploaded files
* @param array $cookies An array of cookies
* @param array $server An array of server parameters
* @param string $content The raw body data
*
* @api
*/
public function __construct($uri, $method, array $parameters = array(), array $files = array(), array $cookies = array(), array $server = array(), $content = null)
{
$this->uri = $uri;
$this->method = $method;
$this->parameters = $parameters;
$this->files = $files;
$this->cookies = $cookies;
$this->server = $server;
$this->content = $content;
}
/**
* Gets the request URI.
*
* @return string The request URI
*
* @api
*/
public function getUri()
{
return $this->uri;
}
/**
* Gets the request HTTP method.
*
* @return string The request HTTP method
*
* @api
*/
public function getMethod()
{
return $this->method;
}
/**
* Gets the request parameters.
*
* @return array The request parameters
*
* @api
*/
public function getParameters()
{
return $this->parameters;
}
/**
* Gets the request server files.
*
* @return array The request files
*
* @api
*/
public function getFiles()
{
return $this->files;
}
/**
* Gets the request cookies.
*
* @return array The request cookies
*
* @api
*/
public function getCookies()
{
return $this->cookies;
}
/**
* Gets the request server parameters.
*
* @return array The request server parameters
*
* @api
*/
public function getServer()
{
return $this->server;
}
/**
* Gets the request raw body data.
*
* @return string The request raw body data.
*
* @api
*/
public function getContent()
{
return $this->content;
}
}
| {
"pile_set_name": "Github"
} |
#ifndef GRAPHICS_API_GLES3
#define NO_NATIVE_SHADOWMAP
#endif
#ifdef NO_NATIVE_SHADOWMAP
#define TEXTURE2D_SHADOW(textureName) uniform mediump sampler2D textureName
#define SAMPLE_TEXTURE2D_SHADOW(textureName, coord3) (texture2D(textureName,coord3.xy).r<coord3.z?0.0:1.0)
#define TEXTURE2D_SHADOW_PARAM(shadowMap) mediump sampler2D shadowMap
#else
#define TEXTURE2D_SHADOW(textureName) uniform mediump sampler2DShadow textureName
#define SAMPLE_TEXTURE2D_SHADOW(textureName, coord3) textureLod(textureName,coord3,0.0)
#define TEXTURE2D_SHADOW_PARAM(shadowMap) mediump sampler2DShadow shadowMap
#endif
#if defined(RECEIVESHADOW)&&defined(SHADOW)
#define CALCULATE_SHADOWS
#endif
#if defined(RECEIVESHADOW)&&defined(SHADOW_SPOT)
#define CALCULATE_SPOTSHADOWS
#endif
uniform vec4 u_ShadowBias; // x: depth bias, y: normal bias
#if defined(CALCULATE_SHADOWS)||defined(CALCULATE_SPOTSHADOWS)
#include "ShadowSampleTent.glsl"
uniform vec4 u_ShadowMapSize;
uniform vec4 u_ShadowParams; // x: shadowStrength y: ShadowSpotLightStrength
float sampleShdowMapFiltered4(TEXTURE2D_SHADOW_PARAM(shadowMap),vec3 shadowCoord,vec4 shadowMapSize)
{
float attenuation;
vec4 attenuation4;
vec2 offset=shadowMapSize.xy/2.0;
vec3 shadowCoord0=shadowCoord + vec3(-offset,0.0);
vec3 shadowCoord1=shadowCoord + vec3(offset.x,-offset.y,0.0);
vec3 shadowCoord2=shadowCoord + vec3(-offset.x,offset.y,0.0);
vec3 shadowCoord3=shadowCoord + vec3(offset,0.0);
attenuation4.x = SAMPLE_TEXTURE2D_SHADOW(shadowMap, shadowCoord0);
attenuation4.y = SAMPLE_TEXTURE2D_SHADOW(shadowMap, shadowCoord1);
attenuation4.z = SAMPLE_TEXTURE2D_SHADOW(shadowMap, shadowCoord2);
attenuation4.w = SAMPLE_TEXTURE2D_SHADOW(shadowMap, shadowCoord3);
attenuation = dot(attenuation4, vec4(0.25));
return attenuation;
}
float sampleShdowMapFiltered9(TEXTURE2D_SHADOW_PARAM(shadowMap),vec3 shadowCoord,vec4 shadowmapSize)
{
float attenuation;
float fetchesWeights[9];
vec2 fetchesUV[9];
sampleShadowComputeSamplesTent5x5(shadowmapSize, shadowCoord.xy, fetchesWeights, fetchesUV);
attenuation = fetchesWeights[0] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[0].xy, shadowCoord.z));
attenuation += fetchesWeights[1] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[1].xy, shadowCoord.z));
attenuation += fetchesWeights[2] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[2].xy, shadowCoord.z));
attenuation += fetchesWeights[3] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[3].xy, shadowCoord.z));
attenuation += fetchesWeights[4] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[4].xy, shadowCoord.z));
attenuation += fetchesWeights[5] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[5].xy, shadowCoord.z));
attenuation += fetchesWeights[6] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[6].xy, shadowCoord.z));
attenuation += fetchesWeights[7] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[7].xy, shadowCoord.z));
attenuation += fetchesWeights[8] * SAMPLE_TEXTURE2D_SHADOW(shadowMap, vec3(fetchesUV[8].xy, shadowCoord.z));
return attenuation;
}
#endif
#if defined(CALCULATE_SHADOWS)//shader中自定义的宏不可用ifdef 必须改成if defined
TEXTURE2D_SHADOW(u_ShadowMap);
uniform mat4 u_ShadowMatrices[4];
uniform vec4 u_ShadowSplitSpheres[4];// max cascade is 4
mediump int computeCascadeIndex(vec3 positionWS)
{
vec3 fromCenter0 = positionWS - u_ShadowSplitSpheres[0].xyz;
vec3 fromCenter1 = positionWS - u_ShadowSplitSpheres[1].xyz;
vec3 fromCenter2 = positionWS - u_ShadowSplitSpheres[2].xyz;
vec3 fromCenter3 = positionWS - u_ShadowSplitSpheres[3].xyz;
mediump vec4 comparison = vec4(
dot(fromCenter0, fromCenter0)<u_ShadowSplitSpheres[0].w,
dot(fromCenter1, fromCenter1)<u_ShadowSplitSpheres[1].w,
dot(fromCenter2, fromCenter2)<u_ShadowSplitSpheres[2].w,
dot(fromCenter3, fromCenter3)<u_ShadowSplitSpheres[3].w);
comparison.yzw = clamp(comparison.yzw - comparison.xyz,0.0,1.0);//keep the nearest
mediump vec4 indexCoefficient = vec4(4.0,3.0,2.0,1.0);
mediump int index = 4 - int(dot(comparison, indexCoefficient));
return index;
}
vec4 getShadowCoord(vec4 positionWS)
{
#ifdef SHADOW_CASCADE
mediump int cascadeIndex = computeCascadeIndex(positionWS.xyz);
if(cascadeIndex > 3)// out of shadow range cascadeIndex is 4.
return vec4(0.0);
#ifdef GRAPHICS_API_GLES3
return u_ShadowMatrices[cascadeIndex] * positionWS;
#else
mat4 shadowMat;
if(cascadeIndex == 0)
shadowMat = u_ShadowMatrices[0];
else if(cascadeIndex == 1)
shadowMat = u_ShadowMatrices[1];
else if(cascadeIndex == 2)
shadowMat = u_ShadowMatrices[2];
else
shadowMat = u_ShadowMatrices[3];
return shadowMat * positionWS;
#endif
#else
return u_ShadowMatrices[0] * positionWS;
#endif
}
float sampleShadowmap(vec4 shadowCoord)
{
shadowCoord.xyz /= shadowCoord.w;
float attenuation = 1.0;
if(shadowCoord.z > 0.0 && shadowCoord.z < 1.0)
{
#if defined(SHADOW_SOFT_SHADOW_HIGH)
attenuation = sampleShdowMapFiltered9(u_ShadowMap,shadowCoord.xyz,u_ShadowMapSize);
#elif defined(SHADOW_SOFT_SHADOW_LOW)
attenuation = sampleShdowMapFiltered4(u_ShadowMap,shadowCoord.xyz,u_ShadowMapSize);
#else
attenuation = SAMPLE_TEXTURE2D_SHADOW(u_ShadowMap,shadowCoord.xyz);
#endif
attenuation = mix(1.0,attenuation,u_ShadowParams.x);//shadowParams.x:shadow strength
}
return attenuation;
}
#endif
#if defined(CALCULATE_SPOTSHADOWS)//shader中自定义的宏不可用ifdef 必须改成if defined
TEXTURE2D_SHADOW(u_SpotShadowMap);
uniform mat4 u_SpotViewProjectMatrix;
float sampleSpotShadowmap(vec4 shadowCoord)
{
shadowCoord.xyz /= shadowCoord.w;
float attenuation = 1.0;
shadowCoord.xy +=1.0;
shadowCoord.xy/=2.0;
if(shadowCoord.z > 0.0 && shadowCoord.z < 1.0)
{
#if defined(SHADOW_SPOT_SOFT_SHADOW_HIGH)
attenuation = sampleShdowMapFiltered9(u_SpotShadowMap,shadowCoord.xyz,u_ShadowMapSize);
#elif defined(SHADOW_SPOT_SOFT_SHADOW_LOW)
attenuation = sampleShdowMapFiltered4(u_SpotShadowMap,shadowCoord.xyz,u_ShadowMapSize);
#else
attenuation = SAMPLE_TEXTURE2D_SHADOW(u_SpotShadowMap,shadowCoord.xyz);
#endif
attenuation = mix(1.0,attenuation,u_ShadowParams.y);//shadowParams.y:shadow strength
}
return attenuation;
}
#endif
vec3 applyShadowBias(vec3 positionWS, vec3 normalWS, vec3 lightDirection)
{
float invNdotL = 1.0 - clamp(dot(-lightDirection, normalWS),0.0,1.0);
float scale = invNdotL * u_ShadowBias.y;
// normal bias is negative since we want to apply an inset normal offset
positionWS += -lightDirection * u_ShadowBias.xxx;
positionWS += normalWS * vec3(scale);
return positionWS;
}
| {
"pile_set_name": "Github"
} |
/**
* Created with JetBrains PhpStorm.
* User: xuheng
* Date: 12-9-26
* Time: 下午12:29
* To change this template use File | Settings | File Templates.
*/
//清空上次查选的痕迹
editor.firstForSR = 0;
editor.currentRangeForSR = null;
//给tab注册切换事件
/**
* tab点击处理事件
* @param tabHeads
* @param tabBodys
* @param obj
*/
function clickHandler( tabHeads,tabBodys,obj ) {
//head样式更改
for ( var k = 0, len = tabHeads.length; k < len; k++ ) {
tabHeads[k].className = "";
}
obj.className = "focus";
//body显隐
var tabSrc = obj.getAttribute( "tabSrc" );
for ( var j = 0, length = tabBodys.length; j < length; j++ ) {
var body = tabBodys[j],
id = body.getAttribute( "id" );
if ( id != tabSrc ) {
body.style.zIndex = 1;
} else {
body.style.zIndex = 200;
}
}
}
/**
* TAB切换
* @param tabParentId tab的父节点ID或者对象本身
*/
function switchTab( tabParentId ) {
var tabElements = $G( tabParentId ).children,
tabHeads = tabElements[0].children,
tabBodys = tabElements[1].children;
for ( var i = 0, length = tabHeads.length; i < length; i++ ) {
var head = tabHeads[i];
if ( head.className === "focus" )clickHandler(tabHeads,tabBodys, head );
head.onclick = function () {
clickHandler(tabHeads,tabBodys,this);
}
}
}
//是否区分大小写
function getMatchCase(id) {
return $G(id).checked ? true : false;
}
//查找
$G("nextFindBtn").onclick = function (txt, dir, mcase) {
var findtxt = $G("findtxt").value, obj;
if (!findtxt) {
return false;
}
obj = {
searchStr:findtxt,
dir:1,
casesensitive:getMatchCase("matchCase")
};
if (!frCommond(obj)) {
alert(lang.getEnd);
}
};
$G("nextReplaceBtn").onclick = function (txt, dir, mcase) {
var findtxt = $G("findtxt1").value, obj;
if (!findtxt) {
return false;
}
obj = {
searchStr:findtxt,
dir:1,
casesensitive:getMatchCase("matchCase1")
};
frCommond(obj);
};
$G("preFindBtn").onclick = function (txt, dir, mcase) {
var findtxt = $G("findtxt").value, obj;
if (!findtxt) {
return false;
}
obj = {
searchStr:findtxt,
dir:-1,
casesensitive:getMatchCase("matchCase")
};
if (!frCommond(obj)) {
alert(lang.getStart);
}
};
$G("preReplaceBtn").onclick = function (txt, dir, mcase) {
var findtxt = $G("findtxt1").value, obj;
if (!findtxt) {
return false;
}
obj = {
searchStr:findtxt,
dir:-1,
casesensitive:getMatchCase("matchCase1")
};
frCommond(obj);
};
//替换
$G("repalceBtn").onclick = function () {
var findtxt = $G("findtxt1").value.replace(/^\s|\s$/g, ""), obj,
replacetxt = $G("replacetxt").value.replace(/^\s|\s$/g, "");
if (!findtxt) {
return false;
}
if (findtxt == replacetxt || (!getMatchCase("matchCase1") && findtxt.toLowerCase() == replacetxt.toLowerCase())) {
return false;
}
obj = {
searchStr:findtxt,
dir:1,
casesensitive:getMatchCase("matchCase1"),
replaceStr:replacetxt
};
frCommond(obj);
};
//全部替换
$G("repalceAllBtn").onclick = function () {
var findtxt = $G("findtxt1").value.replace(/^\s|\s$/g, ""), obj,
replacetxt = $G("replacetxt").value.replace(/^\s|\s$/g, "");
if (!findtxt) {
return false;
}
if (findtxt == replacetxt || (!getMatchCase("matchCase1") && findtxt.toLowerCase() == replacetxt.toLowerCase())) {
return false;
}
obj = {
searchStr:findtxt,
casesensitive:getMatchCase("matchCase1"),
replaceStr:replacetxt,
all:true
};
var num = frCommond(obj);
if (num) {
alert(lang.countMsg.replace("{#count}", num));
}
};
//执行
var frCommond = function (obj) {
return editor.execCommand("searchreplace", obj);
};
switchTab("searchtab"); | {
"pile_set_name": "Github"
} |
/*
* Callbacks prototypes for FSM
*
* Copyright (C) 1996 Universidade de Lisboa
*
* Written by Pedro Roque Marques ([email protected])
*
* This software may be used and distributed according to the terms of
* the GNU General Public License, incorporated herein by reference.
*/
#ifndef CALLBACKS_H
#define CALLBACKS_H
extern void cb_out_1(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_out_2(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_in_1(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_in_2(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_in_3(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_disc_1(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_disc_2(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_disc_3(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_notdone(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_selp_1(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
extern void cb_open(struct pcbit_dev *dev, struct pcbit_chan *chan,
struct callb_data *data);
#endif
| {
"pile_set_name": "Github"
} |
'use strict';
const runAction = require('./action');
const extend = require('node.extend');
const fs = require('fs');
const path = require('path');
const {promisify} = require('util');
const pkg = require('../package.json');
const promiseTimeout = require('p-timeout');
const puppeteer = require('puppeteer');
const semver = require('semver');
const runnerJavascriptPromises = {};
const readFile = promisify(fs.readFile);
module.exports = pa11y;
/**
* Run accessibility tests on a web page.
* @public
* @param {String} url - The URL to run tests against.
* @param {Object} [options={}] - Options to change the way tests run.
* @param {Function} [callback] - An optional callback to use instead of promises.
* @returns {Promise} Returns a promise which resolves with a results object.
*/
async function pa11y(url, options = {}, callback) {
const state = {};
let pa11yError;
let pa11yResults;
[url, options, callback] = parseArguments(url, options, callback);
try {
// Verify that the given options are valid
verifyOptions(options);
// Call the actual Pa11y test runner with
// a timeout if it takes too long
pa11yResults = await promiseTimeout(
runPa11yTest(url, options, state),
options.timeout,
`Pa11y timed out (${options.timeout}ms)`
);
} catch (error) {
// Capture error if a callback is provided, otherwise reject with error
if (callback) {
pa11yError = error;
} else {
throw error;
}
} finally {
await stateCleanup(state);
}
// Run callback if present, and resolve with pa11yResults
return callback ? callback(pa11yError, pa11yResults) : pa11yResults;
}
/**
* Parse arguments from the command-line to properly identify the url, options, and callback
* @private
* @param {String} url - The URL to run tests against.
* @param {Object} [options={}] - Options to change the way tests run.
* @param {Function} [callback] - An optional callback to use instead of promises.
* @returns {Array} the new values of url, options, and callback
*/
function parseArguments(url, options, callback) {
if (!callback && typeof options === 'function') {
callback = options;
options = {};
}
if (typeof url !== 'string') {
options = url;
url = options.url;
}
url = sanitizeUrl(url);
options = defaultOptions(options);
return [url,
options,
callback];
}
/**
* Default the passed in options using Pa11y's defaults.
* @private
* @param {Object} [options] - The options to apply defaults to.
* @returns {Object} Returns the defaulted options.
*/
function defaultOptions(options) {
options = extend({}, pa11y.defaults, options);
options.ignore = options.ignore.map(ignored => ignored.toLowerCase());
if (!options.includeNotices) {
options.ignore.push('notice');
}
if (!options.includeWarnings) {
options.ignore.push('warning');
}
return options;
}
/**
* Internal Pa11y test runner.
* @private
* @param {String} url - The URL to run tests against.
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} Returns a promise which resolves with a results object.
*/
async function runPa11yTest(url, options, state) {
options.log.info(`Running Pa11y on URL ${url}`);
await setBrowser(options, state);
await setPage(options, state);
await interceptRequests(options, state);
await gotoUrl(url, options, state);
await runActionsList(options, state);
await injectRunners(options, state);
// Launch the test runner!
options.log.debug('Running Pa11y on the page');
/* istanbul ignore next */
if (options.wait > 0) {
options.log.debug(`Waiting for ${options.wait}ms`);
}
const results = await runPa11yWithOptions(options, state);
options.log.debug(`Document title: "${results.documentTitle}"`);
await saveScreenCapture(options, state);
return results;
}
/**
* Ensures that puppeteer resources are freed and listeners removed.
* @private
* @param {Object} state - The last-known state of the test-run.
* @returns {Promise} A promise which resolves when resources are released
*/
async function stateCleanup(state) {
if (state.browser && state.autoClose) {
await state.browser.close();
} else if (state.page) {
state.page.removeListener('request', state.requestInterceptCallback);
state.page.removeListener('console', state.consoleCallback);
if (state.autoClosePage) {
await state.page.close();
}
}
}
/**
* Sets or initialises the browser.
* @private
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when resources are released
*/
async function setBrowser(options, state) {
if (options.browser) {
options.log.debug(
'Using a pre-configured Headless Chrome instance, ' +
'the `chromeLaunchConfig` option will be ignored'
);
state.browser = options.browser;
state.autoClose = false;
} else {
// Launch a Headless Chrome browser. We use a
// state object which is accessible from the
// wrapping function
options.log.debug('Launching Headless Chrome');
state.browser = await puppeteer.launch(
options.chromeLaunchConfig
);
state.autoClose = true;
}
}
/**
* Configures the browser page to be used for the test.
* @private
* @param {Object} [options] - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when the page has been configured.
*/
async function setPage(options, state) {
if (options.browser && options.page) {
state.page = options.page;
state.autoClosePage = false;
} else {
state.page = await state.browser.newPage();
state.autoClosePage = true;
}
// Listen for console logs on the page so that we can
// output them for debugging purposes
state.consoleCallback = message => {
options.log.debug(`Browser Console: ${message.text()}`);
};
state.page.on('console', state.consoleCallback);
options.log.debug('Opening URL in Headless Chrome');
if (options.userAgent) {
await state.page.setUserAgent(options.userAgent);
}
await state.page.setViewport(options.viewport);
}
/**
* Configures the browser page to intercept requests if necessary
* @private
* @param {Object} [options] - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves immediately if no listeners are necessary
* or after listener functions have been attached.
*/
async function interceptRequests(options, state) {
// Avoid to use `page.setRequestInterception` when not necessary
// because it occasionally stops page load:
// https://github.com/GoogleChrome/puppeteer/issues/3111
// https://github.com/GoogleChrome/puppeteer/issues/3121
const shouldInterceptRequests =
(options.headers && Object.keys(options.headers).length) ||
(options.method && options.method.toLowerCase() !== 'get') ||
options.postData;
if (!shouldInterceptRequests) {
return;
}
// Intercept page requests, we need to do this in order
// to set the HTTP method or post data
await state.page.setRequestInterception(true);
// Intercept requests so we can set the HTTP method
// and post data. We only want to make changes to the
// first request that's handled, which is the request
// for the page we're testing
let interceptionHandled = false;
state.requestInterceptCallback = interceptedRequest => {
const overrides = {};
if (!interceptionHandled) {
// Override the request method
options.log.debug('Setting request method');
overrides.method = options.method;
// Override the request headers (and include the user-agent)
options.log.debug('Setting request headers');
overrides.headers = {};
for (const [key, value] of Object.entries(options.headers)) {
overrides.headers[key.toLowerCase()] = value;
}
// Override the request POST data if present
if (options.postData) {
options.log.debug('Setting request POST data');
overrides.postData = options.postData;
}
interceptionHandled = true;
}
interceptedRequest.continue(overrides);
};
state.page.on('request', state.requestInterceptCallback);
}
/**
* Instructs the page to go to the provided url unless options.ignoreUrl is true
* @private
* @param {String} [url] - The URL of the page to be tested.
* @param {Object} [options] - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when the page URL has been set
*/
async function gotoUrl(url, options, state) {
// Navigate to the URL we're going to test
if (!options.ignoreUrl) {
await state.page.goto(url, {
waitUntil: 'networkidle2',
timeout: options.timeout
});
}
}
/**
* Carries out a synchronous list of actions in the page
* @private
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when all actions have completed
*/
async function runActionsList(options, state) {
if (options.actions.length) {
options.log.info('Running actions');
for (const action of options.actions) {
await runAction(state.browser, state.page, options, action);
}
options.log.info('Finished running actions');
}
}
/**
* Loads the test runners and Pa11y client-side scripts if required
* @private
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when all runners have been injected and evaluated
*/
async function injectRunners(options, state) {
// We only load these files once on the first run of Pa11y as they don't
// change between runs
if (!runnerJavascriptPromises.pa11y) {
runnerJavascriptPromises.pa11y = readFile(`${__dirname}/runner.js`, 'utf-8');
}
for (const runner of options.runners) {
if (!runnerJavascriptPromises[runner]) {
options.log.debug(`Loading runner: ${runner}`);
runnerJavascriptPromises[runner] = loadRunnerScript(runner);
}
}
// Inject the test runners
options.log.debug('Injecting Pa11y');
await state.page.evaluate(await runnerJavascriptPromises.pa11y);
for (const runner of options.runners) {
options.log.debug(`Injecting runner: ${runner}`);
const script = await runnerJavascriptPromises[runner];
await state.page.evaluate(script);
}
}
/**
* Sends a request to the page to instruct the injected pa11y script to run with the
* provided options
* @private
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves with the results of the pa11y evaluation
*/
function runPa11yWithOptions(options, state) {
/* eslint-disable no-underscore-dangle */
return state.page.evaluate(runOptions => {
return window.__pa11y.run(runOptions);
}, {
hideElements: options.hideElements,
ignore: options.ignore,
pa11yVersion: pkg.version,
rootElement: options.rootElement,
rules: options.rules,
runners: options.runners,
standard: options.standard,
wait: options.wait
});
/* eslint-enable no-underscore-dangle */
}
/**
* Generates a screen capture if required by the provided options
* @private
* @param {Object} options - Options to change the way tests run.
* @param {Object} state - The current pa11y internal state, fields will be mutated by
* this function.
* @returns {Promise} A promise which resolves when the screenshot is complete
*/
async function saveScreenCapture(options, state) {
// Generate a screen capture
if (options.screenCapture) {
options.log.info(
`Capturing screen, saving to "${options.screenCapture}"`
);
try {
await state.page.screenshot({
path: options.screenCapture,
fullPage: true
});
} catch (error) {
options.log.error(`Error capturing screen: ${error.message}`);
}
}
}
/**
* Verify that passed in options are valid.
* @private
* @param {Object} options - The options to verify.
* @returns {Undefined} Returns nothing.
* @throws {Error} Throws if options are not valid.
*/
function verifyOptions(options) {
if (!pa11y.allowedStandards.includes(options.standard)) {
throw new Error(`Standard must be one of ${pa11y.allowedStandards.join(', ')}`);
}
if (options.page && !options.browser) {
throw new Error('The page option must only be set alongside the browser option');
}
if (options.ignoreUrl && !options.page) {
throw new Error('The ignoreUrl option must only be set alongside the page option');
}
}
/**
* Sanitize a URL, ensuring it has a scheme. If the URL begins with a slash or a period,
* it will be resolved as a path against the current working directory. If the URL does
* begin with a scheme, it will be prepended with "http://".
* @private
* @param {String} url - The URL to sanitize.
* @returns {String} Returns the sanitized URL.
*/
function sanitizeUrl(url) {
if (/^\//i.test(url)) {
return `file://${url}`;
}
if (/^\./i.test(url)) {
return `file://${path.resolve(process.cwd(), url)}`;
}
if (!/^(https?|file):\/\//i.test(url)) {
return `http://${url}`;
}
return url;
}
/**
* Load a Pa11y runner module.
* @param {String} runner - The name of the runner.
* @return {Object} Returns the required module.
* TODO could this be refactored to use requireFirst (in bin/pa11y.js)
*/
function loadRunnerFile(runner) {
try {
return require(`pa11y-runner-${runner}`);
} catch (error) {}
return require(runner);
}
/**
* Assert that a Pa11y runner is compatible with a version of Pa11y.
* @param {String} runnerName - The name of the runner.
* @param {String} runnerSupportString - The runner support string (a semver range).
* @param {String} pa11yVersion - The version of Pa11y to test support for.
* @throws {Error} Throws an error if the reporter does not support the given version of Pa11y
* @returns {void}
*/
function assertReporterCompatibility(runnerName, runnerSupportString, pa11yVersion) {
if (!runnerSupportString || !semver.satisfies(pa11yVersion, runnerSupportString)) {
throw new Error([
`The installed "${runnerName}" runner does not support Pa11y ${pa11yVersion}`,
'Please update your version of Pa11y or the runner',
`Reporter Support: ${runnerSupportString}`,
`Pa11y Version: ${pa11yVersion}`
].join('\n'));
}
}
/**
* Loads a runner script
* @param {String} runner - The name of the runner.
* @throws {Error} Throws an error if the reporter does not support the given version of Pa11y
* @returns {Promise<String>} Promise
*/
async function loadRunnerScript(runner) {
const runnerModule = loadRunnerFile(runner);
let runnerBundle = '';
assertReporterCompatibility(runner, runnerModule.supports, pkg.version);
for (const runnerScript of runnerModule.scripts) {
runnerBundle += '\n\n';
runnerBundle += await readFile(runnerScript, 'utf-8');
}
return `
;${runnerBundle};
;window.__pa11y.runners['${runner}'] = ${runnerModule.run.toString()};
`;
}
/* istanbul ignore next */
const noop = () => { /* No-op */ };
/**
* Default options (excluding 'level', 'reporter', and 'threshold' which are only
* relevant when calling bin/pa11y from the CLI)
* @public
*/
pa11y.defaults = {
actions: [],
browser: null,
chromeLaunchConfig: {
ignoreHTTPSErrors: true
},
headers: {},
hideElements: null,
ignore: [],
ignoreUrl: false,
includeNotices: false,
includeWarnings: false,
log: {
debug: noop,
error: noop,
info: noop
},
method: 'GET',
postData: null,
rootElement: null,
rules: [],
runners: [
'htmlcs'
],
screenCapture: null,
standard: 'WCAG2AA',
timeout: 30000,
userAgent: `pa11y/${pkg.version}`,
viewport: {
width: 1280,
height: 1024
},
wait: 0
};
/**
* Allowed a11y standards.
* @public
*/
pa11y.allowedStandards = [
'Section508',
'WCAG2A',
'WCAG2AA',
'WCAG2AAA'
];
/**
* Alias the `isValidAction` method
*/
pa11y.isValidAction = runAction.isValidAction;
| {
"pile_set_name": "Github"
} |
--- glibc-2.3.2/configure-orig 2009-03-26 15:46:33.000000000 +0100
+++ glibc-2.3.2/configure 2009-03-26 15:49:15.000000000 +0100
@@ -3850,67 +3850,6 @@
# These programs are version sensitive.
-for ac_prog in ${ac_tool_prefix}gcc ${ac_tool_prefix}cc
-do
- # Extract the first word of "$ac_prog", so it can be a program name with args.
-set dummy $ac_prog; ac_word=$2
-echo "$as_me:$LINENO: checking for $ac_word" >&5
-echo $ECHO_N "checking for $ac_word... $ECHO_C" >&6
-if test "${ac_cv_prog_CC+set}" = set; then
- echo $ECHO_N "(cached) $ECHO_C" >&6
-else
- if test -n "$CC"; then
- ac_cv_prog_CC="$CC" # Let the user override the test.
-else
-as_save_IFS=$IFS; IFS=$PATH_SEPARATOR
-for as_dir in $PATH
-do
- IFS=$as_save_IFS
- test -z "$as_dir" && as_dir=.
- for ac_exec_ext in '' $ac_executable_extensions; do
- if $as_executable_p "$as_dir/$ac_word$ac_exec_ext"; then
- ac_cv_prog_CC="$ac_prog"
- echo "$as_me:$LINENO: found $as_dir/$ac_word$ac_exec_ext" >&5
- break 2
- fi
-done
-done
-
-fi
-fi
-CC=$ac_cv_prog_CC
-if test -n "$CC"; then
- echo "$as_me:$LINENO: result: $CC" >&5
-echo "${ECHO_T}$CC" >&6
-else
- echo "$as_me:$LINENO: result: no" >&5
-echo "${ECHO_T}no" >&6
-fi
-
- test -n "$CC" && break
-done
-
-if test -z "$CC"; then
- ac_verc_fail=yes
-else
- # Found it, now check the version.
- echo "$as_me:$LINENO: checking version of $CC" >&5
-echo $ECHO_N "checking version of $CC... $ECHO_C" >&6
- ac_prog_version=`$CC -v 2>&1 | sed -n 's/^.*version \([egcygnustpi-]*[0-9.]*\).*$/\1/p'`
- case $ac_prog_version in
- '') ac_prog_version="v. ?.??, bad"; ac_verc_fail=yes;;
- 3.[2-9]*)
- ac_prog_version="$ac_prog_version, ok"; ac_verc_fail=no;;
- *) ac_prog_version="$ac_prog_version, bad"; ac_verc_fail=yes;;
-
- esac
- echo "$as_me:$LINENO: result: $ac_prog_version" >&5
-echo "${ECHO_T}$ac_prog_version" >&6
-fi
-if test $ac_verc_fail = yes; then
- critic_missing="$critic_missing gcc"
-fi
-
for ac_prog in gnumake gmake make
do
# Extract the first word of "$ac_prog", so it can be a program name with args.
| {
"pile_set_name": "Github"
} |
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- Main Menu -->
<string name="shows">節目</string>
<string name="lists">清單</string>
<string name="movies">電影</string>
<string name="statistics">統計</string>
<string name="navigation_more">More</string>
<string name="search">搜尋</string>
<string name="preferences">設置</string>
<string name="help">說明</string>
<string name="no_more_updates">此版本的 Android 將不會收到 SeriesGuide 的任何更新。</string>
<!-- Media items -->
<string name="show_details">Details</string>
<string name="episode">集數</string>
<string name="episode_number">第 %d 集</string>
<string name="episodes">集數</string>
<string name="season">季</string>
<string name="season_number">第 %d 季</string>
<string name="specialseason">特集</string>
<string name="ratings">評分</string>
<!-- Descriptions -->
<string name="description_overview">簡介</string>
<string name="description_poster">顯示海報</string>
<string name="description_image">影集圖片</string>
<string name="description_menu_overflow">更多選項</string>
<!-- Actions -->
<string name="clear">清除</string>
<string name="discard">放棄</string>
<string name="save_selection">儲存所選項目</string>
<string name="action_display_all">顯示全部</string>
<string name="web_search">網頁搜尋</string>
<string name="add_to_homescreen">新增至主螢幕</string>
<string name="action_open">開啟</string>
<string name="sync_manually">手動同步</string>
<string name="sync_and_update">同步 & 和更新</string>
<string name="sync_and_download_all">同步& 及下載</string>
<string name="action_turn_off">關閉</string>
<string name="action_stream">Stream or purchase</string>
<!-- Search -->
<string name="clear_search_history">清除搜尋記錄</string>
<string name="search_hint">搜尋集數</string>
<!-- Status messages -->
<string name="updated">已更新到最新版本。</string>
<string name="updated_details">Details</string>
<string name="norating">無</string>
<string name="unknown">不明</string>
<string name="offline">連接到網路,然後再試一次。</string>
<string name="app_not_available">沒有應用程式可以處理此需求</string>
<string name="people_empty">什麼東西都沒有? !點擊以在試一次。</string>
<string name="database_error">無法修改資料。</string>
<string name="reinstall_info">嘗試在設置中備份,然後重新安裝應用並恢復備份。</string>
<string name="api_error_generic">無法移除節目%s。請稍後再試。</string>
<string name="api_failed">失敗:%s</string>
<string name="copy_to_clipboard">已複製至剪貼簿</string>
<!-- Sharing -->
<string name="share">分享</string>
<string name="share_show">分享節目</string>
<string name="share_episode">分享此集</string>
<string name="share_movie">分享電影</string>
<string name="addtocalendar">新增至行事曆</string>
<string name="addtocalendar_failed">無法建立行事曆事件。</string>
<string name="links">連結</string>
<!-- Comments -->
<string name="comments">評論</string>
<string name="shout_hint">輸入評論(英語,五個字以上)</string>
<string name="shout_invalid">你的評論不遵守規則 !</string>
<string name="action_post">發布</string>
<string name="isspoiler">警告爆雷</string>
<string name="no_shouts">成為第一個留言者</string>
<string name="refresh">重新整理</string>
<plurals name="replies_plural">
<item quantity="other">%d 回覆</item>
</plurals>
<!-- Metadata -->
<string name="episode_number_disk">DVD</string>
<string name="episode_firstaired">播出日期</string>
<string name="episode_firstaired_unknown">播出日期不明</string>
<string name="episode_dvdnumber">DVD集數</string>
<string name="original_release">原始版本</string>
<string name="show_genres">戲劇類別</string>
<string name="show_contentrating">節目分級</string>
<string name="show_release_times">發布時間</string>
<string name="episode_directors">導演</string>
<string name="episode_gueststars">神秘嘉賓</string>
<string name="episode_writers">編劇</string>
<string name="show_isalive">待續</string>
<string name="show_isnotalive">已完結</string>
<string name="show_isUpcoming">即將播出</string>
<string name="no_translation">%2$s沒有%1$s的翻譯說明。</string>
<string name="no_translation_title">無翻譯</string>
<string name="no_spoilers">一些細節已隱藏起來避免劇透,可前往設定關閉此功能。</string>
<string name="format_source">來源:%s</string>
<string name="format_last_edited">上次編輯於:%s</string>
<!-- Date and Time -->
<string name="today">今天</string>
<string name="now">目前</string>
<string name="daily">每日</string>
<string name="runtime_minutes">%s 分鐘</string>
<!-- Flagging -->
<string name="mark_all">全部已觀看</string>
<string name="unmark_all">全部未觀看</string>
<string name="action_watched_up_to">Watched up to here</string>
<string name="confirmation_watched_up_to">Set all episodes up to here watched?</string>
<string name="collect_all">將所有影集標示為已蒐藏</string>
<string name="uncollect_all">取消所有影集蒐藏標示</string>
<string name="action_skip">略過</string>
<string name="action_dont_skip">取消略過</string>
<string name="action_collection_add">添加到收藏</string>
<string name="action_collection_remove">從收藏移除</string>
<string name="action_watched">設為已觀看</string>
<string name="action_unwatched">設為尚未觀看</string>
<string name="state_watched">已看過</string>
<string name="state_watched_multiple_format" comment="Like watched, but includes the number of times in parentheses. Example: Watched (3)">Watched (%d)</string>
<string name="state_skipped">Skipped</string>
<string name="state_in_collection">In collection</string>
<string name="state_on_watchlist">On watchlist</string>
<string name="state_favorite">Favorite</string>
<!-- Shows -->
<string name="confirm_delete">確定要移除%s嗎?</string>
<string name="delete_error">無法移除節目。請稍後再試。</string>
<string name="delete_show">移除</string>
<string name="no_nextepisode">找無下一集</string>
<string name="context_favorite">加入最愛</string>
<string name="context_unfavorite">自最愛移除</string>
<string name="favorited">新增至我的最愛</string>
<string name="unfavorited">從我的最愛中刪除</string>
<string name="action_episode_notifications_on">開啟新劇集通知</string>
<string name="action_episode_notifications_off">開啟新劇集通知</string>
<string name="context_hide">隱藏</string>
<string name="context_unhide">取消隱藏</string>
<string name="hidden">隱藏影集</string>
<string name="unhidden">取消隱藏影集</string>
<string name="action_shows_add">增加影集</string>
<string name="action_shows_filter">影集顯示條件</string>
<string name="empty_filter">移除所有篩選</string>
<string name="action_shows_filter_favorites">我的最愛</string>
<string name="action_shows_filter_unwatched">尚未觀看</string>
<string name="action_shows_filter_upcoming">即將播出</string>
<string name="action_shows_filter_hidden">隱藏</string>
<string name="action_shows_make_all_visible">Make all hidden visible</string>
<string name="description_make_all_visible_format">Make all hidden shows (%s) visible again?</string>
<string name="action_shows_sort">排序影集</string>
<string name="action_shows_sort_title">依影集名稱</string>
<string name="action_shows_sort_latest_episode">最新一集</string>
<string name="action_shows_sort_oldest_episode">最舊一集</string>
<string name="action_shows_sort_last_watched">上一次觀看</string>
<string name="action_shows_sort_remaining">剩餘的集數</string>
<string name="action_shows_sort_favorites">喜愛影集優先</string>
<string name="context_updateshow">更新</string>
<string name="context_marknext">觀看下一集</string>
<string name="action_episodes_switch_view">Switch view</string>
<!-- History -->
<string name="user_stream">歴史紀錄</string>
<string name="recently_watched">最近觀賞</string>
<string name="friends_recently">trakt好友</string>
<string name="now_empty">沒什麼特別地事情發生,看節目去吧!</string>
<string name="now_movies_empty">沒什麼特別地事情發生,看電影去吧!</string>
<string name="user_stream_empty">Trakt上沒有任何記錄,去看一些節目並打卡吧!</string>
<string name="user_movie_stream_empty">Trakt上沒有任何記錄,去看一些電影並打卡吧!</string>
<!-- Calendar -->
<string name="upcoming">即將播出</string>
<string name="recent">最近</string>
<string name="released_today">今日播出</string>
<string name="noupcoming">沒有即將播出的集數。</string>
<string name="norecent">沒有最近的影集。</string>
<string name="calendar_settings">Calendar settings</string>
<string name="only_favorites">僅顯示喜愛影集</string>
<string name="calendar_only_collected">Only collected episodes</string>
<string name="calendar_only_premieres">Only premieres</string>
<string name="pref_infinite_scrolling">萬年曆</string>
<!-- Updating -->
<string name="update_scheduled">更新中......</string>
<string name="update_no_connection">如需更新,請連接網路</string>
<string name="update_inprogress">更新正在進行中,請稍後再試</string>
<!-- Seasons -->
<string name="season_allwatched">已觀看所有集數</string>
<plurals name="other_episodes_plural">
<item quantity="other">%d other episodes</item>
</plurals>
<plurals name="not_released_episodes_plural">
<item quantity="other">%d to be released</item>
</plurals>
<plurals name="remaining_episodes_plural">
<item quantity="other">剩餘 %d</item>
</plurals>
<!-- Add show -->
<string name="checkin_searchhint">輸入節目名稱</string>
<string name="add_empty">無此節目</string>
<string name="no_results">查無結果,請嘗試完整單字。</string>
<string name="empty_show_search">節目單中未找到</string>
<string name="action_try_again">再試一次</string>
<string name="tvdb_error_does_not_exist">TheTVDB 上不存在。稍後再試。</string>
<string name="add_all">全部加入</string>
<string name="add_multiple">所有節目將會顯示在你的清單中。</string>
<string name="add_started">%s將會顯示在你的清單中。</string>
<string name="add_error">無法新增%s</string>
<string name="add_already_exists">%s已經在你的清單當中。</string>
<string name="title_new_episodes">新劇集</string>
<string name="watched_shows">已看過</string>
<string name="shows_collection">收藏</string>
<string name="watchlist">待看清單</string>
<!-- Similar shows -->
<string name="empty_no_results">無結果</string>
<string name="title_similar_shows">類似節目</string>
<!-- Lists -->
<string name="first_list">第一個清單</string>
<string name="list_add">新增清單</string>
<string name="list_title_hint">命名您的清單</string>
<string name="list_remove">移除清單</string>
<string name="list_manage">管理清單</string>
<string name="list_item_manage">管理清單</string>
<string name="list_item_remove">從清單中移除</string>
<string name="list_empty">你可以新增整個、一季或是一集節目到清單當中。</string>
<string name="action_lists_sort">排序清單</string>
<string name="action_lists_reorder">重新排序清單</string>
<string name="error_name_already_exists">此名稱的清單已存在。</string>
<!-- Movies -->
<string name="title_discover">探索</string>
<string name="title_popular">熱門</string>
<string name="title_digital_releases">數位發行</string>
<string name="title_disc_releases">碟片版本</string>
<string name="movies_in_theatres">上映中</string>
<string name="movies_collection">收藏</string>
<string name="movies_watchlist">待看清單</string>
<string name="movies_watched">已看過</string>
<string name="watchlist_add">新增至待看清單</string>
<string name="watchlist_remove">從待看清單中移除</string>
<string name="watchlist_added">已增加至待看清單</string>
<string name="watchlist_removed">已從待看清單中移除完成</string>
<string name="movies_search_hint">輸入電影名稱</string>
<string name="movies_collection_empty">你不能將電影加入收藏中。</string>
<string name="movies_watchlist_empty">你不能將電影加入待看清單中。</string>
<string name="trailer">預告</string>
<string name="movie_cast">卡司</string>
<string name="movie_crew">工作人員</string>
<string name="action_movies_sort">電影排序</string>
<string name="action_movies_sort_title">依影集名稱</string>
<string name="action_movies_sort_release">播出日期</string>
<!-- Statistics -->
<string name="statistics_failed">無法統計資料。</string>
<string name="statistics_shows">影集</string>
<string name="statistics_episodes">集數</string>
<string name="statistics_movies">電影</string>
<string name="shows_with_next">%s 影集有新的集數</string>
<string name="shows_continuing">%s部影集仍在連載</string>
<string name="episodes_watched">%s 集已看</string>
<string name="movies_on_watchlist">%s於待看清單中。</string>
<string name="movies_watched_format">%s 集已看</string>
<plurals name="days_plural">
<item quantity="other">%d 天</item>
</plurals>
<plurals name="hours_plural">
<item quantity="other">%d 小時</item>
</plurals>
<plurals name="minutes_plural">
<item quantity="other">%d 分鐘</item>
</plurals>
<!-- Features -->
<string name="feature_supported">Supported</string>
<string name="feature_not_supported">Not supported</string>
<string name="feature_history">Personal and friend history</string>
<string name="feature_comments">Post comments</string>
<string name="feature_sync">Sync</string>
<!-- Hexagon -->
<string name="hexagon_warning">實驗性</string>
<string name="hexagon_description">SeriesGuide Cloud helps to backup and sync your shows, lists and movies</string>
<string name="hexagon_warning_trakt">While signed in with SeriesGuide Cloud some Trakt features are not available</string>
<string name="hexagon_signin">登入</string>
<string name="hexagon_signout">登出</string>
<string name="hexagon_setup_incomplete">SeriesGuide Cloud未完成設置。</string>
<string name="hexagon_setup_fail_auth">你的Google帳戶無法使用。</string>
<string name="hexagon_remove_account">移除帳戶</string>
<string name="hexagon_remove_account_success">帳戶已刪除。</string>
<string name="hexagon_remove_account_failure">無法刪除帳戶。</string>
<string name="hexagon_remove_account_confirmation">移除你的帳戶將會刪除SeriesGuide Cloud上所有節目與電影的紀錄。請確認其他裝置上的資料也一併刪除。</string>
<string name="hexagon_api_queued">發送至SeriesGuide Cloud。</string>
<string name="hexagon_signed_out">Signed out of SeriesGuide Cloud</string>
<string name="hexagon_signin_fail_format">Error with Google Sign-In (%s)</string>
<!-- trakt -->
<string name="trakt_submitqueued">正發送至trakt。</string>
<string name="checkin_success_trakt">在trakt上打卡%s</string>
<string name="checkin_canceled_success_trakt">在trakt上取消打卡。</string>
<string name="traktcheckin_cancel">覆蓋</string>
<string name="traktcheckin_inprogress">你已經在其他影集或電影打卡過了,請覆蓋前一個打卡記錄或者等待%s後再試一次。</string>
<string name="traktcheckin_wait">等待</string>
<string name="trakt_success">已發送至trakt。</string>
<string name="trakt_error_credentials">發生錯誤,請檢查您的trakt憑證並重試。</string>
<string name="trakt_error_not_exists">Trakt還無法使用,稍後再試。</string>
<string name="trakt_notice_not_exists">還不能使用trakt追蹤。</string>
<!-- Connect trakt -->
<string name="connect_trakt">連接 trakt</string>
<string name="trakt_reconnect">連結SeriesGuide與trakt</string>
<string name="trakt_reconnect_details">檢查您的 trakt 憑證</string>
<string name="waitplease">請稍候…</string>
<string name="connect">連接</string>
<string name="disconnect">中斷連接</string>
<string name="use_integrated_browser">使用內建瀏覽器</string>
<string name="show_library">顯示您的 trakt 庫</string>
<string name="about_trakt">trakt 幫助您追蹤及記錄正在看的電視節目和電影。<a href="HTTPs://trakt.tv"> 瞭解更多</a></string>
<!-- trakt ratings -->
<string name="action_rate">評分</string>
<string name="your_rating">您的評分</string>
<string name="love">太讚啦!</string>
<string name="hate">太弱了:(</string>
<string name="rating2">浪費人生</string>
<string name="rating3">糟透了</string>
<string name="rating4">雞助</string>
<string name="rating5">嗯......</string>
<string name="rating6">尚可</string>
<string name="rating7">不錯</string>
<string name="rating8">讚</string>
<string name="rating9">好愛!!</string>
<plurals name="votes">
<item quantity="other">%d 票</item>
</plurals>
<!-- Check Ins -->
<string name="checkin">打卡</string>
<string name="checkin_hint">輸入訊息(可忽略)</string>
<string name="paste_title">貼上標題</string>
<string name="pref_quick_checkin">快速打卡</string>
<string name="pref_quick_checkin_summary">免輸入打卡訊息</string>
<!-- Help -->
<string name="feedback">傳送反饋意見</string>
<string name="action_open_in_browser">於瀏覽器中開啟</string>
<!-- Settings -->
<string name="action_translate">Translate this app</string>
<string name="action_contribute_content">Translate or improve content</string>
<string name="prefs_category_advanced">進階</string>
<string name="prefs_category_about">關於本軟體</string>
<string name="pref_onlyfuture">無已播出集數</string>
<string name="pref_onlyfuturesummary">不顯示已經播出劇集</string>
<string name="pref_onlyseasoneps">隱藏特別節目</string>
<string name="pref_onlyseasonepssummary">除了季清單外不要顯示特別節目</string>
<string name="pref_exactdates">顯示確切日期</string>
<string name="pref_exactdates_summary">\"10 月 31日\" 而不是 \"3天後\"</string>
<string name="pref_nospoilers">避免劇透</string>
<string name="pref_nospoilers_summary">隱藏詳細資訊直到看過一集</string>
<string name="pref_seasonsorting">以…排序每一季</string>
<string name="pref_episodesorting">以…排序每一集</string>
<string name="pref_language">偏好的語言</string>
<string name="pref_language_fallback">Alternative language</string>
<string name="pref_updatewifionly">只有在使用Wi-Fi時載入圖片</string>
<string name="pref_updatewifionlysummary">為了減少資料量,不要透過移動或計量網路下載</string>
<string name="pref_error_reports">Report errors to app developer</string>
<plurals name="days_before_plural">
<item quantity="other">%d days before</item>
</plurals>
<plurals name="hours_before_plural">
<item quantity="other">%d hours before</item>
</plurals>
<plurals name="minutes_before_plural">
<item quantity="other">%d minutes before</item>
</plurals>
<!-- About screen -->
<string name="privacy_policy">Privacy Policy</string>
<string name="licence_tvdb">此應用程式使用基於 CC BY-NC 4.0 許可的 TheTVDB 提供的資料和圖像。</string>
<string name="licence_themoviedb">此應用程式使用 TMDb 的 API ,但未被 TMDb 認可或認證。</string>
<string name="licence_trakt">This app uses data and images by Trakt.</string>
<string name="about_open_source">SeriesGuide 是採用開放源碼軟體。</string>
<string name="licences_and_credits">憑證及信用</string>
<!-- Sort -->
<string name="sort">排序方式</string>
<string name="action_sort_ignore_articles">忽略文章</string>
<!-- Sort orders -->
<string name="sort_order_latest_first">按最新的項目排序</string>
<string name="sort_order_oldest_first">按最舊的項目排序</string>
<string name="sort_order_unwatched_first">先排未觀賞</string>
<string name="sort_order_alphabetical">A - Z</string>
<string name="sort_order_top_rated_first">最高評分</string>
<string name="sort_order_latest_first_dvd">按最新的順序排序(DVD)</string>
<string name="sort_order_oldest_first_dvd">按最舊的順序排序(DVD)</string>
<!-- Upcoming thresholds -->
<string name="pref_upcominglimit">即將撥出時間</string>
<string name="upcoming_upto_now">目前</string>
<string name="upcoming_upto_one_day">一天</string>
<string name="upcoming_upto_three_days">三天</string>
<string name="upcoming_upto_one_week">一星期</string>
<string name="upcoming_upto_two_weeks">兩星期</string>
<string name="upcoming_upto_one_month">一個月</string>
<!-- Appearance settings -->
<string name="pref_appearance">外觀</string>
<string name="pref_theme">主題</string>
<!-- Themes -->
<string name="theme_app_follow_system">Use system default</string>
<string name="theme_app_auto_battery">Set by Battery Saver</string>
<string name="theme_app_dark">Dark</string>
<string name="theme_app_light">Light</string>
<!-- Other advanced settings -->
<string name="pref_other">其他</string>
<string name="pref_autoupdatesummary">自動同步和更新 SeriesGuide 資料</string>
<string name="backup">備份/還原</string>
<string name="backup_summary">備份或還原你的影集</string>
<string name="pref_number">數字格式</string>
<string name="pref_offset">手動時間換算</string>
<string name="pref_offsetsummary">%d 小時的時差</string>
<string name="clear_cache">清除圖片暫存檔</string>
<string name="clear_cache_summary">從您的設備中刪除所有暫存的圖片</string>
<!-- Backup and Restore -->
<string name="backup_full_dump">Include descriptions, details, ratings</string>
<string name="backup_failed_file_access">無法備份。找不到或無法存取備份檔案。</string>
<string name="backup_success">備份成功。</string>
<string name="backup_failed">備份失敗。</string>
<string name="import_failed_nosd">無法還原備份。備份資料夾無法使用。</string>
<string name="import_failed_nofile">沒有可讀的備份檔案。</string>
<string name="import_success">已成功還原備份。</string>
<string name="import_failed">還原備份檔失敗。</string>
<string name="backup_button">備份</string>
<string name="import_button">還原</string>
<string name="import_warning">我明白我目前的節目,及其他清單和電影將會被取代 !</string>
<string name="no_file_selected">沒有選擇檔案</string>
<string name="action_select_file">選擇檔案</string>
<!-- Auto backup -->
<string name="pref_autobackup">自動備份</string>
<string name="pref_autobackupsummary">每週備份你的節目,清單和電影</string>
<string name="autobackup_details">These backups are unavailable if the app is installed again unless app data backup is turned on for this device (Android 6+). Create copies to preserve them otherwise.</string>
<string name="last_auto_backup">剩餘自動備份︰ %s</string>
<string name="restore_auto_backup">還原自動備份</string>
<string name="autobackup_failed">The last auto backup has failed</string>
<string name="autobackup_files_missing">為了備份,請選擇一可用備份資料夾。</string>
<string name="autobackup_create_user_copy">Create a copy after each backup</string>
<string name="autobackup_restore_available">An auto backup might be available to restore from.</string>
<!-- Welcome dialog -->
<string name="get_started">開始</string>
<string name="dismiss">解除</string>
<!-- Notifications -->
<string name="upcoming_show">%s 就要來了</string>
<string name="upcoming_episodes">新的集數來了</string>
<string name="upcoming_episodes_number">%s新集數</string>
<string name="more">%d 更多</string>
<string name="upcoming_display">選擇以顯示即將撥出的節目</string>
<string name="pref_notifications">提示信息</string>
<string name="pref_notificationssummary">當有新的集數時進行通知</string>
<string name="pref_ringtone">提示音</string>
<string name="pref_vibrate">震動</string>
<string name="pref_vibratesummary">顯示通知的同時震動</string>
<string name="pref_notifications_treshold">何時通知</string>
<string name="pref_notifications_select_shows">Select shows</string>
<string name="pref_notifications_select_shows_summary">Shows that have notifications enabled (%d)</string>
<string name="pref_notifications_hidden">Ignore hidden shows</string>
<string name="pref_notifications_hidden_summary">即使已啟用通知,也不要通知已隱藏節目</string>
<string name="pref_notification_channel_errors">錯誤</string>
<string name="pref_notifications_next_episodes_only">Only for episodes to watch next</string>
<string name="pref_notifications_next_episodes_only_summary">Only notify if the new episode is the next one to watch</string>
<string name="pref_notifications_battery_settings">Battery settings</string>
<string name="pref_notifications_battery_settings_summary">Go to Battery settings to disable battery optimizations for reliable notifications</string>
<!-- List Widget -->
<string name="hide_watched">隱藏已看過集數</string>
<string name="pref_widget_opacity">小工具背景</string>
<string name="pref_widget_type">小工具類型</string>
<string name="pref_large_font">使用大的字型</string>
<!-- Widget background options -->
<string name="widget_background_transparent">透明度</string>
<string name="widget_background_25">25%</string>
<string name="widget_background_50">50%</string>
<string name="widget_background_75">75%</string>
<string name="widget_background_opaque">透明度</string>
<!-- Google In-App Billing -->
<string name="onlyx">僅供贊助者</string>
<string name="action_upgrade">解鎖所有功能</string>
<string name="billing_action_subscribe">訂閱</string>
<string name="billing_action_manage_subscriptions">管理訂閱</string>
<string name="billing_action_pass">取得 X Pass</string>
<string name="billing_price_subscribe">每年%1$s,免費試用,可在%2$s 內隨時取消。</string>
<string name="billing_duration_format">%1$s / 年</string>
<string name="billing_sub_description">Try 30 days for free, cancel any time.</string>
<string name="billing_price_pass">一次購買</string>
<string name="upgrade_description">Every subscription unlocks all features and supports future updates. A subscription is not required to use this app.</string>
<string name="upgrade_success">謝謝贊助SeriesGuide !您可以使用的所有功能。</string>
<string name="billing_learn_more">More info and help</string>
<string name="subscription_expired">訂閱已過期</string>
<string name="subscription_expired_details">您的 SeriesGuide 訂閱已過期。</string>
<!-- Amazon In-App Billing -->
<string name="subscription_not_signed_in">登錄到你的Amazon帳戶 !</string>
<string name="subscription_unavailable">目前不可訂閱。</string>
<string name="subscription_failed">未完成購買。</string>
<string name="pass_unavailable">X Pass目前無法使用。</string>
<!-- Extensions -->
<string name="action_extensions_configure">客製化擴充套件</string>
<string name="action_extensions_add">新增擴充套件</string>
<string name="action_extensions_search">取得更多擴充套件</string>
<string name="action_extension_remove">移除</string>
<string name="extension_amazon">Amazon搜索</string>
<string name="description_extension_amazon">搜索您當地的Amazon網站</string>
<string name="pref_amazon_domain">Amazon網站</string>
<string name="extension_google_play">Google Play 搜尋</string>
<string name="description_extension_google_play">搜尋 Google Play 商店</string>
<string name="extension_youtube">YouTube 上搜尋</string>
<string name="description_extension_youtube">搜尋 YouTube 視頻</string>
<string name="description_extension_web_search">搜尋網站和圖像</string>
<!-- People -->
<string name="person_biography">個人簡介</string>
<!-- Feedback -->
<string name="feedback_question_enjoy">你喜歡 SeriesGuide 嗎?</string>
<string name="feedback_action_yes">是的!</string>
<string name="feedback_action_notreally">不太喜歡</string>
<string name="feedback_question_rate_google">到Google play上評分?</string>
<string name="feedback_question_rate_amazon">到Amazon上評分?</string>
<string name="feedback_question_feedback">告訴我如何改善它呢?</string>
<string name="feedback_action_ok">好的</string>
<string name="feedback_action_nothanks">不了,謝謝</string>
</resources>
| {
"pile_set_name": "Github"
} |
# Translation of Odoo Server.
# This file contains the translation of the following modules:
# * hr_gamification
#
# Translators:
# Martin Trigaux <[email protected]>, 2017
msgid ""
msgstr ""
"Project-Id-Version: Odoo Server 10.saas~18\n"
"Report-Msgid-Bugs-To: \n"
"POT-Creation-Date: 2017-09-20 09:53+0000\n"
"PO-Revision-Date: 2017-09-20 09:53+0000\n"
"Last-Translator: Martin Trigaux <[email protected]>, 2017\n"
"Language-Team: Kabyle (https://www.transifex.com/odoo/teams/41243/kab/)\n"
"MIME-Version: 1.0\n"
"Content-Type: text/plain; charset=UTF-8\n"
"Content-Transfer-Encoding: \n"
"Language: kab\n"
"Plural-Forms: nplurals=2; plural=(n != 1);\n"
#. module: hr_gamification
#: model_terms:ir.actions.act_window,help:hr_gamification.goals_menu_groupby_action2
msgid ""
"A goal is defined by a user and a goal type.\n"
" Goals can be created automatically by using challenges."
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,help:hr_gamification.field_hr_employee_badge_ids
msgid ""
"All employee badges, linked to the employee either directly or through the "
"user"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.actions.act_window,help:hr_gamification.challenge_list_action2
msgid ""
"Assign a list of goals to chosen users to evaluate them.\n"
" The challenge can use a period (weekly, monthly...) for automatic creation of goals.\n"
" The goals are created for the specified users or member of the group."
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_res_users_badge_ids
#: model:ir.ui.menu,name:hr_gamification.gamification_badge_menu_hr
msgid "Badges"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_hr_employee_view_form
msgid ""
"Badges are rewards of good work. Give them to people you believe deserve it."
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,help:hr_gamification.field_hr_employee_direct_badge_ids
msgid "Badges directly linked to the employee"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.view_badge_wizard_reward
msgid "Cancel"
msgstr "Sefsex"
#. module: hr_gamification
#: model:ir.actions.act_window,name:hr_gamification.challenge_list_action2
#: model:ir.ui.menu,name:hr_gamification.gamification_challenge_menu_hr
#: model:ir.ui.menu,name:hr_gamification.menu_hr_gamification
msgid "Challenges"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.actions.act_window,help:hr_gamification.challenge_list_action2
msgid "Click to create a challenge."
msgstr ""
#. module: hr_gamification
#: model_terms:ir.actions.act_window,help:hr_gamification.goals_menu_groupby_action2
msgid "Click to create a goal."
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_hr_employee_view_form
msgid "Click to grant this employee his first badge"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.view_badge_wizard_reward
msgid "Describe what they did and why it matters (will be public)"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_hr_employee_direct_badge_ids
msgid "Direct Badge"
msgstr ""
#. module: hr_gamification
#: model:ir.model,name:hr_gamification.model_hr_employee
#: model:ir.model.fields,field_description:hr_gamification.field_gamification_badge_user_employee_id
#: model:ir.model.fields,field_description:hr_gamification.field_gamification_badge_user_wizard_employee_id
msgid "Employee"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_hr_employee_badge_ids
msgid "Employee Badges"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_hr_employee_goal_ids
msgid "Employee HR Goals"
msgstr ""
#. module: hr_gamification
#: model:ir.model,name:hr_gamification.model_gamification_badge
msgid "Gamification badge"
msgstr ""
#. module: hr_gamification
#: model:ir.model,name:hr_gamification.model_gamification_badge_user
msgid "Gamification user badge"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_res_users_goal_ids
msgid "Goal"
msgstr ""
#. module: hr_gamification
#: model:ir.actions.act_window,name:hr_gamification.goals_menu_groupby_action2
#: model:ir.ui.menu,name:hr_gamification.gamification_goal_menu_hr
msgid "Goals History"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_hr_employee_view_form
msgid "Grant a Badge"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_badge_form_view
msgid "Granted"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_gamification_badge_granted_employees_count
msgid "Granted Employees Count"
msgstr ""
#. module: hr_gamification
#: model:ir.model.fields,field_description:hr_gamification.field_hr_employee_has_badges
msgid "Has Badges"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_hr_employee_view_form
msgid "Received Badges"
msgstr ""
#. module: hr_gamification
#: model:ir.actions.act_window,name:hr_gamification.action_reward_wizard
#: model_terms:ir.ui.view,arch_db:hr_gamification.view_badge_wizard_reward
msgid "Reward Employee"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.view_badge_wizard_reward
msgid "Reward Employee with"
msgstr ""
#. module: hr_gamification
#: code:addons/hr_gamification/models/gamification.py:18
#, python-format
msgid "The selected employee does not correspond to the selected user."
msgstr ""
#. module: hr_gamification
#: model:ir.model,name:hr_gamification.model_res_users
msgid "Users"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.view_badge_wizard_reward
msgid "What are you thankful for?"
msgstr ""
#. module: hr_gamification
#: code:addons/hr_gamification/wizard/gamification_badge_user_wizard.py:21
#, python-format
msgid "You can not send a badge to yourself"
msgstr ""
#. module: hr_gamification
#: code:addons/hr_gamification/wizard/gamification_badge_user_wizard.py:18
#, python-format
msgid "You can send badges only to employees linked to a user."
msgstr ""
#. module: hr_gamification
#: model:ir.model,name:hr_gamification.model_gamification_badge_user_wizard
msgid "gamification.badge.user.wizard"
msgstr ""
#. module: hr_gamification
#: model_terms:ir.ui.view,arch_db:hr_gamification.hr_hr_employee_view_form
msgid "to reward this employee for a good action"
msgstr ""
| {
"pile_set_name": "Github"
} |
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<Version>0.0.0</Version>
<Nullable>annotations</Nullable>
<GenerateFullPaths>true</GenerateFullPaths>
<TargetFramework>netcoreapp3.1</TargetFramework>
<TreatWarningsAsErrors>true</TreatWarningsAsErrors>
<DebugType Condition="'$(Configuration)'=='Release'">none</DebugType>
</PropertyGroup>
<ItemGroup>
<ProjectReference Include="..\MvcTemplate.Components\MvcTemplate.Components.csproj" />
</ItemGroup>
</Project>
| {
"pile_set_name": "Github"
} |
/*
* GDevelop Core
* Copyright 2008-present Florian Rival ([email protected]). All rights
* reserved. This project is released under the MIT License.
*/
#ifndef GDCORE_PROPERTYDESCRIPTOR
#define GDCORE_PROPERTYDESCRIPTOR
#include <vector>
#include "GDCore/String.h"
namespace gd {
class SerializerElement;
}
namespace gd {
/**
* \brief Used to describe a property shown in a property grid.
* \see gd::Object
* \see gd::EffectMetadata
*/
class GD_CORE_API PropertyDescriptor {
public:
/**
* \brief Create a property being a simple gd::String with the specified
* value.
*
* \param propertyValue The value of the property.
*/
PropertyDescriptor(gd::String propertyValue)
: currentValue(propertyValue), type("string"), label(""), hidden(false) {}
/**
* \brief Empty constructor creating an empty property to be displayed.
*/
PropertyDescriptor() : hidden(false){};
/**
* \brief Destructor
*/
virtual ~PropertyDescriptor();
/**
* \brief Change the value displayed in the property grid
*/
PropertyDescriptor& SetValue(gd::String value) {
currentValue = value;
return *this;
}
/**
* \brief Change the type of the value displayed in the property grid.
* \note The type is arbitrary and is interpreted by the class updating the
* property grid: Refer to it or to the documentation of the class which is
* returning the PropertyDescriptor to learn more about valid values for the
* type.
*/
PropertyDescriptor& SetType(gd::String type_) {
type = type_;
return *this;
}
/**
* \brief Change the label displayed in the property grid.
*/
PropertyDescriptor& SetLabel(gd::String label_) {
label = label_;
return *this;
}
/**
* \brief Change the description displayed to the user, if any.
*/
PropertyDescriptor& SetDescription(gd::String description_) {
description = description_;
return *this;
}
/**
* \brief Add an information about the property.
* \note The information are arbitrary and are interpreted by the class
* updating the property grid: Refer to it or to the documentation of the
* class which is returning the PropertyDescriptor to learn more about valid
* values for the extra information.
*/
PropertyDescriptor& AddExtraInfo(const gd::String& info) {
extraInformation.push_back(info);
return *this;
}
const gd::String& GetValue() const { return currentValue; }
const gd::String& GetType() const { return type; }
const gd::String& GetLabel() const { return label; }
const gd::String& GetDescription() const { return description; }
const std::vector<gd::String>& GetExtraInfo() const {
return extraInformation;
}
/**
* \brief Set if the property should be shown or hidden in the editor.
*/
PropertyDescriptor& SetHidden(bool enable = true) {
hidden = enable;
return *this;
}
/**
* \brief Check if the property should be shown or hidden in the editor.
*/
bool IsHidden() const { return hidden; }
/** \name Serialization
*/
///@{
/**
* \brief Serialize the PropertyDescriptor.
*/
virtual void SerializeTo(SerializerElement& element) const;
/**
* \brief Unserialize the PropertyDescriptor.
*/
virtual void UnserializeFrom(const SerializerElement& element);
/**
* \brief Serialize only the value and extra informations.
*/
virtual void SerializeValuesTo(SerializerElement& element) const;
/**
* \brief Unserialize only the value and extra informations.
*/
virtual void UnserializeValuesFrom(const SerializerElement& element);
///@}
private:
gd::String currentValue; ///< The current value to be shown.
gd::String
type; ///< The type of the property. This is arbitrary and interpreted by
///< the class responsible for updating the property grid.
gd::String label; //< The user-friendly property name
gd::String description; //< The user-friendly property description
std::vector<gd::String>
extraInformation; ///< Can be used to store for example the available
///< choices, if a property is a displayed as a combo
///< box.
bool hidden;
};
} // namespace gd
#endif
| {
"pile_set_name": "Github"
} |
sat
| {
"pile_set_name": "Github"
} |
bin.includes = feature.xml
| {
"pile_set_name": "Github"
} |
--- gpu/command_buffer/tests/gl_copy_texture_CHROMIUM_unittest.cc.orig 2019-04-08 08:32:57 UTC
+++ gpu/command_buffer/tests/gl_copy_texture_CHROMIUM_unittest.cc
@@ -562,7 +562,7 @@ class GLCopyTextureCHROMIUMES3Test : public GLCopyText
bool ShouldSkipNorm16() const {
DCHECK(!ShouldSkipTest());
-#if (defined(OS_MACOSX) || defined(OS_WIN) || defined(OS_LINUX)) && \
+#if (defined(OS_MACOSX) || defined(OS_WIN) || defined(OS_LINUX) || defined(OS_BSD)) && \
(defined(ARCH_CPU_X86) || defined(ARCH_CPU_X86_64))
// Make sure it's tested; it is safe to assume that the flag is always true
// on desktop.
| {
"pile_set_name": "Github"
} |
#ifndef ABSTRACT_H
#define ABSTRACT_H
#ifdef PROFILE
#define printf(a, b) Serial.println(b)
#endif
#if defined ARDUINO_SAM_DUE || defined ADAFRUIT_GRAND_CENTRAL_M4
#define HostOS 0x01
#endif
#ifdef CORE_TEENSY
#define HostOS 0x04
#endif
#ifdef ESP32
#define HostOS 0x05
#endif
#ifdef _STM32_DEF_
#define HostOS 0x06
#endif
/* Memory abstraction functions */
/*===============================================================================*/
bool _RamLoad(char* filename, uint16 address) {
File f;
bool result = false;
if (f = SD.open(filename, FILE_READ)) {
while (f.available())
_RamWrite(address++, f.read());
f.close();
result = true;
}
return(result);
}
/* Filesystem (disk) abstraction fuctions */
/*===============================================================================*/
File rootdir, userdir;
#define FOLDERCHAR '/'
typedef struct {
uint8 dr;
uint8 fn[8];
uint8 tp[3];
uint8 ex, s1, s2, rc;
uint8 al[16];
uint8 cr, r0, r1, r2;
} CPM_FCB;
typedef struct {
uint8 dr;
uint8 fn[8];
uint8 tp[3];
uint8 ex, s1, s2, rc;
uint8 al[16];
} CPM_DIRENTRY;
#if defined board_teensy41
static DirFat_t fileDirEntry;
#else
static dir_t fileDirEntry;
#endif
File _sys_fopen_w(uint8* filename) {
return(SD.open((char*)filename, O_CREAT | O_WRITE));
}
int _sys_fputc(uint8 ch, File& f) {
return(f.write(ch));
}
void _sys_fflush(File& f) {
f.flush();
}
void _sys_fclose(File& f) {
f.close();
}
int _sys_select(uint8* disk) {
uint8 result = FALSE;
File f;
digitalWrite(LED, HIGH ^ LEDinv);
if (f = SD.open((char*)disk, O_READ)) {
if (f.isDirectory())
result = TRUE;
f.close();
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
long _sys_filesize(uint8* filename) {
long l = -1;
File f;
digitalWrite(LED, HIGH ^ LEDinv);
if (f = SD.open((char*)filename, O_RDONLY)) {
l = f.size();
f.close();
}
digitalWrite(LED, LOW ^ LEDinv);
return(l);
}
int _sys_openfile(uint8* filename) {
File f;
int result = 0;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_READ);
if (f) {
f.dirEntry(&fileDirEntry);
f.close();
result = 1;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
int _sys_makefile(uint8* filename) {
File f;
int result = 0;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_CREAT | O_WRITE);
if (f) {
f.close();
result = 1;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
int _sys_deletefile(uint8* filename) {
digitalWrite(LED, HIGH ^ LEDinv);
return(SD.remove((char*)filename));
digitalWrite(LED, LOW ^ LEDinv);
}
int _sys_renamefile(uint8* filename, uint8* newname) {
File f;
int result = 0;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_WRITE | O_APPEND);
if (f) {
if (f.rename((char*)newname)) {
f.close();
result = 1;
}
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
#ifdef DEBUGLOG
void _sys_logbuffer(uint8* buffer) {
#ifdef CONSOLELOG
puts((char*)buffer);
#else
File f;
uint8 s = 0;
while (*(buffer + s)) // Computes buffer size
++s;
if (f = SD.open(LogName, O_CREAT | O_APPEND | O_WRITE)) {
f.write(buffer, s);
f.flush();
f.close();
}
#endif
}
#endif
bool _sys_extendfile(char* fn, unsigned long fpos)
{
uint8 result = true;
File f;
unsigned long i;
digitalWrite(LED, HIGH ^ LEDinv);
if (f = SD.open(fn, O_WRITE | O_APPEND)) {
if (fpos > f.size()) {
for (i = 0; i < f.size() - fpos; ++i) {
if (f.write((uint8)0) != 1) {
result = false;
break;
}
}
}
f.close();
} else {
result = false;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
uint8 _sys_readseq(uint8* filename, long fpos) {
uint8 result = 0xff;
File f;
uint8 bytesread;
uint8 dmabuf[BlkSZ];
uint8 i;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_READ);
if (f) {
if (f.seek(fpos)) {
for (i = 0; i < BlkSZ; ++i)
dmabuf[i] = 0x1a;
bytesread = f.read(&dmabuf[0], BlkSZ);
if (bytesread) {
for (i = 0; i < BlkSZ; ++i)
_RamWrite(dmaAddr + i, dmabuf[i]);
}
result = bytesread ? 0x00 : 0x01;
} else {
result = 0x01;
}
f.close();
} else {
result = 0x10;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
uint8 _sys_writeseq(uint8* filename, long fpos) {
uint8 result = 0xff;
File f;
digitalWrite(LED, HIGH ^ LEDinv);
if (_sys_extendfile((char*)filename, fpos))
f = SD.open((char*)filename, O_RDWR);
if (f) {
if (f.seek(fpos)) {
if (f.write(_RamSysAddr(dmaAddr), BlkSZ))
result = 0x00;
} else {
result = 0x01;
}
f.close();
} else {
result = 0x10;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
uint8 _sys_readrand(uint8* filename, long fpos) {
uint8 result = 0xff;
File f;
uint8 bytesread;
uint8 dmabuf[BlkSZ];
uint8 i;
long extSize;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_READ);
if (f) {
if (f.seek(fpos)) {
for (i = 0; i < BlkSZ; ++i)
dmabuf[i] = 0x1a;
bytesread = f.read(&dmabuf[0], BlkSZ);
if (bytesread) {
for (i = 0; i < BlkSZ; ++i)
_RamWrite(dmaAddr + i, dmabuf[i]);
}
result = bytesread ? 0x00 : 0x01;
} else {
if (fpos >= 65536L * BlkSZ) {
result = 0x06; // seek past 8MB (largest file size in CP/M)
} else {
extSize = f.size();
// round file size up to next full logical extent
extSize = ExtSZ * ((extSize / ExtSZ) + ((extSize % ExtSZ) ? 1 : 0));
if (fpos < extSize)
result = 0x01; // reading unwritten data
else
result = 0x04; // seek to unwritten extent
}
}
f.close();
} else {
result = 0x10;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
uint8 _sys_writerand(uint8* filename, long fpos) {
uint8 result = 0xff;
File f;
digitalWrite(LED, HIGH ^ LEDinv);
if (_sys_extendfile((char*)filename, fpos)) {
f = SD.open((char*)filename, O_RDWR);
}
if (f) {
if (f.seek(fpos)) {
if (f.write(_RamSysAddr(dmaAddr), BlkSZ))
result = 0x00;
} else {
result = 0x06;
}
f.close();
} else {
result = 0x10;
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
static uint8 findNextDirName[13];
static uint16 fileRecords = 0;
static uint16 fileExtents = 0;
static uint16 fileExtentsUsed = 0;
static uint16 firstFreeAllocBlock;
uint8 _findnext(uint8 isdir) {
File f;
uint8 result = 0xff;
bool isfile;
uint32 bytes;
digitalWrite(LED, HIGH ^ LEDinv);
if (allExtents && fileRecords) {
_mockupDirEntry();
result = 0;
} else {
while (f = userdir.openNextFile()) {
f.getName((char*)&findNextDirName[0], 13);
isfile = !f.isDirectory();
bytes = f.size();
f.dirEntry(&fileDirEntry);
f.close();
if (!isfile)
continue;
_HostnameToFCBname(findNextDirName, fcbname);
if (match(fcbname, pattern)) {
if (isdir) {
// account for host files that aren't multiples of the block size
// by rounding their bytes up to the next multiple of blocks
if (bytes & (BlkSZ - 1)) {
bytes = (bytes & ~(BlkSZ - 1)) + BlkSZ;
}
fileRecords = bytes / BlkSZ;
fileExtents = fileRecords / BlkEX + ((fileRecords & (BlkEX - 1)) ? 1 : 0);
fileExtentsUsed = 0;
firstFreeAllocBlock = firstBlockAfterDir;
_mockupDirEntry();
} else {
fileRecords = 0;
fileExtents = 0;
fileExtentsUsed = 0;
firstFreeAllocBlock = firstBlockAfterDir;
}
_RamWrite(tmpFCB, filename[0] - '@');
_HostnameToFCB(tmpFCB, findNextDirName);
result = 0x00;
break;
}
}
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
uint8 _findfirst(uint8 isdir) {
uint8 path[4] = { '?', FOLDERCHAR, '?', 0 };
path[0] = filename[0];
path[2] = filename[2];
if (userdir)
userdir.close();
userdir = SD.open((char*)path); // Set directory search to start from the first position
_HostnameToFCBname(filename, pattern);
fileRecords = 0;
fileExtents = 0;
fileExtentsUsed = 0;
return(_findnext(isdir));
}
uint8 _findnextallusers(uint8 isdir) {
uint8 result = 0xFF;
char dirname[13];
bool done = false;
while (!done) {
while (!userdir) {
userdir = rootdir.openNextFile();
if (!userdir) {
done = true;
break;
}
userdir.getName(dirname, sizeof dirname);
if (userdir.isDirectory() && strlen(dirname) == 1 && isxdigit(dirname[0])) {
currFindUser = dirname[0] <= '9' ? dirname[0] - '0' : toupper(dirname[0]) - 'A' + 10;
break;
}
userdir.close();
}
if (userdir) {
result = _findnext(isdir);
if (result) {
userdir.close();
} else {
done = true;
}
} else {
result = 0xFF;
done = true;
}
}
return result;
}
uint8 _findfirstallusers(uint8 isdir) {
uint8 path[2] = { '?', 0 };
path[0] = filename[0];
if (rootdir)
rootdir.close();
if (userdir)
userdir.close();
rootdir = SD.open((char*)path); // Set directory search to start from the first position
strcpy((char*)pattern, "???????????");
if (!rootdir)
return 0xFF;
fileRecords = 0;
fileExtents = 0;
fileExtentsUsed = 0;
return(_findnextallusers(isdir));
}
uint8 _Truncate(char* filename, uint8 rc) {
File f;
int result = 0;
digitalWrite(LED, HIGH ^ LEDinv);
f = SD.open((char*)filename, O_WRITE | O_APPEND);
if (f) {
if (f.truncate(rc * BlkSZ)) {
f.close();
result = 1;
}
}
digitalWrite(LED, LOW ^ LEDinv);
return(result);
}
void _MakeUserDir() {
uint8 dFolder = cDrive + 'A';
uint8 uFolder = toupper(tohex(userCode));
uint8 path[4] = { dFolder, FOLDERCHAR, uFolder, 0 };
digitalWrite(LED, HIGH ^ LEDinv);
SD.mkdir((char*)path);
digitalWrite(LED, LOW ^ LEDinv);
}
uint8 _sys_makedisk(uint8 drive) {
uint8 result = 0;
if (drive < 1 || drive>16) {
result = 0xff;
} else {
uint8 dFolder = drive + '@';
uint8 disk[2] = { dFolder, 0 };
digitalWrite(LED, HIGH ^ LEDinv);
if (!SD.mkdir((char*)disk)) {
result = 0xfe;
} else {
uint8 path[4] = { dFolder, FOLDERCHAR, '0', 0 };
SD.mkdir((char*)path);
}
digitalWrite(LED, LOW ^ LEDinv);
}
return(result);
}
/* Console abstraction functions */
/*===============================================================================*/
int _kbhit(void) {
return(Serial.available());
}
uint8 _getch(void) {
while (!Serial.available());
return(Serial.read());
}
uint8 _getche(void) {
uint8 ch = _getch();
Serial.write(ch);
return(ch);
}
void _putch(uint8 ch) {
Serial.write(ch);
}
void _clrscr(void) {
Serial.println("\e[H\e[J");
}
#endif
| {
"pile_set_name": "Github"
} |