repo_id
stringclasses 205
values | file_path
stringlengths 33
141
| content
stringlengths 1
307k
| __index_level_0__
int64 0
0
|
---|---|---|---|
/home/johnshepherd/drake/examples/bouncing_ball | /home/johnshepherd/drake/examples/bouncing_ball/test/bouncing_ball_test.cc | #include "drake/examples/bouncing_ball/bouncing_ball.h"
#include <memory>
#include <gtest/gtest.h>
#include "drake/systems/analysis/runge_kutta3_integrator.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/test_utilities/scalar_conversion.h"
namespace drake {
namespace examples {
namespace bouncing_ball {
namespace {
// Computes the drop time from the initial height and the gravitational
// acceleration.
double CalcDropTime(const double g, const double q0) {
DRAKE_DEMAND(g < 0.0);
DRAKE_DEMAND(q0 > 0.0);
// The time that the ball will impact the ground is:
// gt^2/2 + q0 = 0
// Solve the quadratic equation at^2 + bt + c = 0 for t.
const double a = g/2;
const double c = q0;
return std::sqrt(-c/a);
}
// Computes the closed form height and velocity at tf seconds for a bouncing
// ball starting from height q0, subject to gravitational acceleration g,
// and with coefficient of restitution e, assuming that the initial velocity
// is zero. Restitution coefficients of 0 and 1 are the only ones supported.
// Returns a pair of values, the first corresponding to the height at tf,
// the second corresponding to the velocity at tf.
std::pair<double, double> CalcClosedFormHeightAndVelocity(double g,
double e,
double q0,
double tf) {
const double drop_time = CalcDropTime(g, q0);
// Handle the cases appropriately.
if (e == 0.0) {
// TODO(edrumwri): Test these cases when we can handle the Zeno's Paradox
// problem.
if (tf < drop_time) {
// In a ballistic phase.
return std::make_pair(g*tf*tf/2 + q0, g*tf);
} else {
// Ball has hit the ground.
return std::make_pair(0.0, 0.0);
}
}
if (e == 1.0) {
// Get the number of phases that have passed.
int num_phases = static_cast<int>(std::floor(tf / drop_time));
// Get the time within the phase.
const double t = tf - num_phases*drop_time;
// Even phases mean that the ball is falling, odd phases mean that it is
// rising.
if ((num_phases & 1) == 0) {
return std::make_pair(g*t*t/2 + q0, g*t);
} else {
// Get the ball velocity at the time of impact.
const double vf = g*drop_time;
return std::make_pair(g*t*t/2 - vf*t, g*t - vf);
}
}
throw std::logic_error("Invalid restitution coefficient!");
}
class BouncingBallTest : public ::testing::Test {
protected:
void SetUp() override {
dut_ = std::make_unique<BouncingBall<double>>();
context_ = dut_->CreateDefaultContext();
output_ = dut_->AllocateOutput();
derivatives_ = dut_->AllocateTimeDerivatives();
}
systems::VectorBase<double>& continuous_state() {
return context_->get_mutable_continuous_state_vector();
}
const systems::VectorBase<double>& generalized_position() {
return context_->get_continuous_state().get_generalized_position();
}
const systems::VectorBase<double>& generalized_velocity() {
return context_->get_continuous_state().get_generalized_velocity();
}
std::unique_ptr<BouncingBall<double>> dut_; //< The device under test.
std::unique_ptr<systems::Context<double>> context_;
std::unique_ptr<systems::SystemOutput<double>> output_;
std::unique_ptr<systems::ContinuousState<double>> derivatives_;
};
GTEST_TEST(BouncingBall, AutoDiff) {
BouncingBall<AutoDiffXd> ad_plant;
}
TEST_F(BouncingBallTest, Transmogrification) {
ASSERT_TRUE(systems::is_autodiffxd_convertible(*dut_));
ASSERT_TRUE(systems::is_symbolic_convertible(*dut_));
}
TEST_F(BouncingBallTest, Topology) {
ASSERT_EQ(0, dut_->num_input_ports());
ASSERT_EQ(1, dut_->num_output_ports());
const auto& output_port = dut_->get_output_port(0);
EXPECT_EQ(systems::kVectorValued, output_port.get_data_type());
}
TEST_F(BouncingBallTest, Output) {
// Grab a pointer to where the CalcOutput results will be saved.
const auto result = output_->get_vector_data(0);
// Initial state and output.
dut_->CalcOutput(*context_, output_.get());
EXPECT_EQ(10.0, result->GetAtIndex(0));
EXPECT_EQ(0.0, result->GetAtIndex(1));
// New state just propagates through.
continuous_state().SetAtIndex(0, 1.0);
continuous_state().SetAtIndex(1, 2.0);
dut_->CalcOutput(*context_, output_.get());
EXPECT_EQ(1.0, result->GetAtIndex(0));
EXPECT_EQ(2.0, result->GetAtIndex(1));
}
TEST_F(BouncingBallTest, Derivatives) {
// Grab a pointer to where the EvalTimeDerivatives results will be saved.
const auto& result = derivatives_->get_mutable_vector();
// Evaluate time derivatives.
dut_->CalcTimeDerivatives(*context_, derivatives_.get());
EXPECT_EQ(0.0, result.GetAtIndex(0));
EXPECT_EQ(-9.81, result.GetAtIndex(1));
// Test at non-zero velocity.
continuous_state().SetAtIndex(1, 5.3);
dut_->CalcTimeDerivatives(*context_, derivatives_.get());
EXPECT_EQ(5.3, result.GetAtIndex(0));
EXPECT_EQ(-9.81, result.GetAtIndex(1));
}
TEST_F(BouncingBallTest, Accessors) {
// Evaluate accessors specific to the second-order system.
EXPECT_EQ(10.0, generalized_position().GetAtIndex(0));
EXPECT_EQ(0, generalized_velocity().GetAtIndex(0));
}
TEST_F(BouncingBallTest, Simulate) {
// Small errors from time-of-impact isolation tolerances propagate for this
// particular instance of the problem (with restitution coefficient of 1).
// Drake's integrators control local (truncation) rather than global
// (i.e., solution to the initial value problem) error. This means that the
// number of digits of precision obtained will not be equal to the digits of
// precision requested (via the accuracy setting) for longer running times
// than t_final = 10.0.
const double t_final = 10.0;
const double q0 = 1.0;
const double v0 = 0.0;
const double accuracy = 1e-4;
// Prepare to integrate.
// TODO(edrumwri): Update the code below when accuracy is settable purely
// from the context.
drake::systems::Simulator<double> simulator(*dut_, std::move(context_));
simulator.reset_integrator<systems::RungeKutta3Integrator<double>>();
simulator.get_mutable_context().SetAccuracy(accuracy);
simulator.get_mutable_integrator().request_initial_step_size_target(1e-3);
simulator.get_mutable_integrator().set_target_accuracy(accuracy);
// Note: The bouncing ball's witness function is triggered when the ball's
// height is positive then non-positive. As shown below, the maximum step size
// is limited to avoid missing a subsequent bounce in the following situation:
// 1. Bounce detected (previous height positive, current height negative).
// 2. Impact reverses ball's downward velocity to an upward velocity.
// 3. Integrator advances time too far so that the next time the witness
// function is called, the ball's height is again negative.
// 4. Since the witness function sees two subsequent negative heights, the
// witness function is not triggered (so subsequent bounce is missed).
// This situation arises when:
// a. an integrator can simulate parabolic trajectory without error (e.g.,
// 2nd-order integrator) which means the time step can be large, or
// b. a semi-explicit 1st-order integrator has large error tolerances that
// allow for a large time step and two successive negative heights.
ASSERT_EQ(dut_->get_restitution_coef(), 1.0);
const double g = dut_->get_gravitational_acceleration();
const double drop_time = CalcDropTime(g, q0);
simulator.get_mutable_integrator().set_maximum_step_size(drop_time);
simulator.Initialize();
// Set the initial state for the bouncing ball.
systems::VectorBase<double>& xc = simulator.get_mutable_context().
get_mutable_continuous_state_vector();
xc.SetAtIndex(0, q0);
xc.SetAtIndex(1, v0);
// Integrate.
simulator.AdvanceTo(t_final);
EXPECT_EQ(simulator.get_mutable_context().get_time(), t_final);
// Check against closed form solution for the bouncing ball. We anticipate
// some small integration error.
const double tol = accuracy;
double height, velocity;
std::tie(height, velocity) = CalcClosedFormHeightAndVelocity(
dut_->get_gravitational_acceleration(),
dut_->get_restitution_coef(), q0, t_final);
EXPECT_NEAR(xc.GetAtIndex(0), height, tol);
EXPECT_NEAR(xc.GetAtIndex(1), velocity, tol);
}
} // namespace
} // namespace bouncing_ball
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d_geometry.h | #pragma once
#include "drake/geometry/scene_graph.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace rod2d {
// Expresses the Rod2D's geometry to a SceneGraph.
///
/// @system
/// name: Rod2dGeometry
/// input_ports:
/// - state
/// output_ports:
/// - geometry_pose
/// @endsystem
///
/// This class has no public constructor; instead use the AddToBuilder() static
/// method to create and add it to a DiagramBuilder directly.
class Rod2dGeometry final : public systems::LeafSystem<double> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(Rod2dGeometry);
~Rod2dGeometry() final;
/// Creates, adds, and connects a Rod2dGeometry system into the given
/// `builder`. Both the `rod2d_state_port.get_system()` and `scene_graph`
/// systems must have been added to the given `builder` already.
///
/// The `scene_graph` pointer is not retained by the %Rod2dGeometry
/// system. The return value pointer is an alias of the new %Rod2dGeometry
/// system that is owned by the `builder`.
///
/// The rod is visualized with a cylinder with the given `radius`, and
/// `length` displayed with the a default grey color.
static const Rod2dGeometry* AddToBuilder(
double radius, double length, systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& rod2d_state_port,
geometry::SceneGraph<double>* scene_graph);
private:
Rod2dGeometry(double radius, double length, geometry::SceneGraph<double>*);
void OutputGeometryPose(const systems::Context<double>&,
geometry::FramePoseVector<double>*) const;
// Geometry source identifier for this system to interact with SceneGraph.
geometry::SourceId source_id_{};
// The id for the rod's body.
geometry::FrameId frame_id_{};
};
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_binary",
"drake_cc_googletest",
"drake_cc_library",
)
package(default_visibility = ["//visibility:private"])
drake_cc_library(
name = "constraint_solver",
srcs = ["constraint_solver.cc"],
hdrs = [
"constraint_problem_data.h",
"constraint_solver.h",
],
deps = [
"//common:essential",
"//solvers:moby_lcp_solver",
],
)
drake_cc_library(
name = "rod2d_state_vector",
srcs = ["rod2d_state_vector.cc"],
hdrs = ["rod2d_state_vector.h"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_cc_binary(
name = "rod2d_sim",
srcs = ["rod2d_sim.cc"],
add_test_rule = 1,
test_rule_args = [" --sim_duration=0.01"],
deps = [
":rod2d",
":rod2d_geometry",
"//common:essential",
"//geometry:drake_visualizer",
"//systems/analysis:implicit_euler_integrator",
"//systems/analysis:runge_kutta3_integrator",
"//systems/analysis:simulator",
"//systems/framework",
"@gflags",
],
)
drake_cc_library(
name = "rod2d",
srcs = ["rod2d.cc"],
hdrs = [
"rod2d.h",
],
deps = [
":constraint_solver",
":rod2d_state_vector",
"//common:essential",
"//solvers:mathematical_program",
"//systems/framework:leaf_system",
],
)
drake_cc_library(
name = "rod2d_geometry",
srcs = ["rod2d_geometry.cc"],
hdrs = [
"rod2d_geometry.h",
],
deps = [
"//geometry:geometry_frame",
"//geometry:geometry_ids",
"//geometry:geometry_instance",
"//geometry:geometry_roles",
"//geometry:kinematics_vector",
"//geometry:rgba",
"//geometry:scene_graph",
"//math:geometric_transform",
"//systems/framework:diagram_builder",
"//systems/framework:leaf_system",
],
)
# === test/ ===
drake_cc_googletest(
name = "constraint_solver_test",
deps = [
":constraint_solver",
":rod2d",
"//solvers:moby_lcp_solver",
"//solvers:unrevised_lemke_solver",
],
)
drake_cc_googletest(
name = "rod2d_test",
deps = [
":constraint_solver",
":rod2d",
"//common:essential",
"//common/test_utilities:eigen_matrix_compare",
"//common/test_utilities:expect_no_throw",
"//systems/analysis:simulator",
],
)
add_lint_tests()
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d_sim.cc | #include <cmath>
#include <iomanip>
#include <iostream>
#include <limits>
#include <memory>
#include <gflags/gflags.h>
#include "drake/examples/rod2d/rod2d.h"
#include "drake/examples/rod2d/rod2d_geometry.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/systems/analysis/implicit_euler_integrator.h"
#include "drake/systems/analysis/runge_kutta3_integrator.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
using Rod2D = drake::examples::rod2d::Rod2D<double>;
using drake::examples::rod2d::Rod2dGeometry;
using drake::geometry::DrakeVisualizerd;
using drake::geometry::SceneGraph;
using drake::systems::Context;
using drake::systems::DiagramBuilder;
using drake::systems::ImplicitEulerIntegrator;
using drake::systems::RungeKutta3Integrator;
using drake::systems::Simulator;
// Simulation parameters.
DEFINE_string(system_type, "discretized",
"Type of rod system, valid values are "
"'discretized','continuous'");
DEFINE_double(dt, 1e-2, "Integration step size");
DEFINE_double(rod_radius, 5e-2, "Radius of the rod (for visualization only)");
DEFINE_double(sim_duration, 10, "Simulation duration in virtual seconds");
DEFINE_double(accuracy, 1e-5,
"Requested simulation accuracy (ignored for discretized system)");
int main(int argc, char* argv[]) {
// Parse any flags.
gflags::ParseCommandLineFlags(&argc, &argv, true);
DiagramBuilder<double> builder;
// Create the rod and add it to the diagram.
Rod2D* rod;
if (FLAGS_system_type == "discretized") {
rod = builder.template AddSystem<Rod2D>(Rod2D::SystemType::kDiscretized,
FLAGS_dt);
} else if (FLAGS_system_type == "continuous") {
rod =
builder.template AddSystem<Rod2D>(Rod2D::SystemType::kContinuous, 0.0);
} else {
std::cerr << "Invalid simulation type '" << FLAGS_system_type
<< "'; note that types are case sensitive." << std::endl;
return -1;
}
auto& scene_graph = *builder.AddSystem<SceneGraph<double>>();
Rod2dGeometry::AddToBuilder(FLAGS_rod_radius, rod->get_rod_half_length() * 2,
&builder, rod->state_output(), &scene_graph);
DrakeVisualizerd::AddToBuilder(&builder, scene_graph);
// Set the names of the systems.
rod->set_name("rod");
auto diagram = builder.Build();
// Make no external forces act on the rod.
auto context = diagram->CreateDefaultContext();
Context<double>& rod_context =
diagram->GetMutableSubsystemContext(*rod, context.get());
const Eigen::Vector3d ext_input(0, 0, 0);
rod->get_input_port(0).FixValue(&rod_context, ext_input);
// Set up the integrator.
Simulator<double> simulator(*diagram, std::move(context));
if (FLAGS_system_type == "continuous") {
simulator.reset_integrator<ImplicitEulerIntegrator<double>>();
} else {
simulator.reset_integrator<RungeKutta3Integrator<double>>();
}
simulator.get_mutable_integrator().set_target_accuracy(FLAGS_accuracy);
simulator.get_mutable_integrator().set_maximum_step_size(FLAGS_dt);
// Start simulating.
simulator.set_target_realtime_rate(1.0);
while (simulator.get_context().get_time() < FLAGS_sim_duration) {
const double t = simulator.get_context().get_time();
simulator.AdvanceTo(std::min(t + 1, FLAGS_sim_duration));
}
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d.h | #pragma once
#include <memory>
#include <utility>
#include <vector>
#include "drake/examples/rod2d/constraint_problem_data.h"
#include "drake/examples/rod2d/constraint_solver.h"
#include "drake/examples/rod2d/rod2d_state_vector.h"
#include "drake/solvers/moby_lcp_solver.h"
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace rod2d {
// TODO(edrumwri): Track energy and add a test to check it.
/** Dynamical system representation of a rod contacting a half-space in
two dimensions.
<h3>Notation</h3>
In the discussion below and in code comments, we will use the 2D analog of our
standard multibody notation as described in detail here:
@ref multibody_notation.
<!-- https://drake.mit.edu/doxygen_cxx/group__multibody__notation.html -->
For a quick summary and translation to 2D:
- When we combine rotational and translational quantities into a single
quantity in 3D, we call those "spatial" quantities. In 2D those combined
quantities are actually planar, but we will continue to refer to them as
"spatial" to keep the notation analogous and promote easy extension of 2D
pedagogical examples to 3D.
- We use capital letters to represent bodies and coordinate frames. Frame F has
an origin point Fo, and a basis formed by orthogonal unit vector axes Fx and
Fy, with an implicit `Fz=Fx × Fy` always pointing out of the screen for a 2D
system. The inertial frame World is W, and the rod frame is R.
- We also use capitals to represent points, and we allow a frame name F to be
used where a point is expected to represent its origin Fo.
- We use `p_CD` to represent the position vector from point C to point D. Note
that if A and B are frames, `p_AB` means `p_AoBo`.
- If we need to be explicit about the expressed-in frame F for any quantity, we
add the suffix `_F` to its symbol. So the position vector from C to D,
expressed in W, is `p_CD_W`.
- R_AB is the rotation matrix giving frame B's orientation in frame A.
- X_AB is the transformation matrix giving frame B's pose in frame A, combining
both a rotation and a translation; this is conventionally called a
"transform". A transform is a spatial quantity.
In 2D, with frames A and B the above quantities are (conceptually) matrices
with the indicated dimensions: <pre>
p_AB = Bo-Ao = |x| R_AB=| cθ -sθ | X_AB=| R_AB p_AB |
|y|₂ₓ₁ | sθ cθ |₂ₓ₂ | 0 0 1 |₃ₓ₃
</pre>
where x,y are B's Cartesian coordinates in the A frame, and θ is the
counterclockwise angle from Ax to Bx, measured about Az (and Bz). In practice,
2D rotations are represented just by the scalar angle θ, and 2D transforms are
represented by (x,y,θ).
We use v for translational velocity of a point and w (ω) for rotational
velocity of a frame. The symbols are:
- `v_AP` is point P's velocity in frame A, expressed in frame A if no
other frame is given as a suffix.
- `w_AB` is frame B's angular velocity in frame A, expressed in frame A
if no other frame is given as a suffix.
- `V_AB` is frame B's spatial velocity in A, meaning `v_ABo` and `w_AB`.
These quantities are conceptually: <pre>
v_AB = |vx| w_AB=|0| V_AB=| w_AB |
|vy| |0| | v_AB |₆ₓ₁
| 0|₃ₓ₁ |ω|₃ₓ₁
</pre>
but in 2D we represent translational velocity with just (vx,vy), angular
velocity with just the scalar w=ω=@f$\dot{\theta}@f$ (that is, d/dt θ), and
spatial velocity as (vx,vy,ω).
Forces f and torques τ are represented similarly:
- `f_P` is an in-plane force applied to a point P fixed to some rigid body.
- `t_A` is an in-plane torque applied to frame A (meaning it is about Az).
- `F_A` is a spatial force including both `f_Ao` and `t_A`.
The above symbols can be suffixed with an expressed-in frame if the frame is
not already obvious, so `F_A_W` is a spatial force applied to frame A (at Ao)
but expressed in W. These quantities are conceptually: <pre>
f_A = |fx| t_A=|0| F_A=| t_A |
|fy| |0| | f_A |₆ₓ₁
| 0|₃ₓ₁ |τ|₃ₓ₁
</pre>
but in 2D we represent translational force with just (fx,fy), torque with just
the scalar t=τ, and spatial force as (fx,fy,τ).
<h3>The 2D rod model</h3>
The rod's coordinate frame R is placed at the rod's center point Ro, which
is also its center of mass Rcm. R's planar pose is given by a planar
transform X_WR=(x,y,θ). When X_WR=0 (identity transform), R is coincident
with the World frame W, and aligned horizontally as shown: <pre>
+Wy +Ry
| |
| |<---- h ----->
| ==============|==============
Wo*-----> +Wx Rl* Ro*-----> +Rx *Rr
=============================
World frame Rod R, θ=0
</pre>
θ is the angle between Rx and Wx, measured using the right hand rule about
Wz (out of the screen), that is, counterclockwise. The rod has half-length
h, and "left" and "right" endpoints `Rl=Ro-h*Rx` and `Rr=Ro+h*Rx` at which
it can contact the halfspace whose surface is at Wy=0.
This system can be simulated using one of three models:
- continuously, using a compliant contact model (the rod is rigid, but contact
between the rod and the half-space is modeled as compliant) simulated using
ordinary differential equations (ODEs),
- a fully rigid model simulated with piecewise differential algebraic
equations (DAEs), and
- a fully rigid model simulated as a discrete system using a first-order
discretization approach.
The rod state is initialized to the configuration that corresponds to the
Painlevé Paradox problem, described in [Stewart 2000]. The paradox consists
of a rod contacting a planar surface *without impact* and subject to sliding
Coulomb friction. The problem is well known to correspond to an
*inconsistent rigid contact configuration*, where impulsive forces are
necessary to resolve the problem.
Inputs: planar force (two-dimensional) and torque (scalar), which are
arbitrary "external" forces (expressed in the world frame) applied
at the center-of-mass of the rod.
States: planar position (state indices 0 and 1) and orientation (state
index 2), and planar linear velocity (state indices 3 and 4) and
scalar angular velocity (state index 5) in units of m, radians,
m/s, and rad/s, respectively. Orientation is measured counter-
clockwise with respect to the x-axis.
Outputs: Output Port 0 corresponds to the state vector.
- [Stewart, 2000] D. Stewart, "Rigid-Body Dynamics with Friction and
Impact". SIAM Rev., 42(1), 3-39, 2000.
@system
name: Rod2D
input_ports:
- u0
output_ports:
- state_output
@endsystem
@tparam_double_only
*/
template <typename T>
class Rod2D : public systems::LeafSystem<T> {
public:
~Rod2D() override {}
/// System model and approach for simulating the system.
enum class SystemType {
/// For modeling the system using rigid contact, Coulomb friction, and
/// hybrid mode variables and simulating the system through piecewise
/// solutions of differential algebraic equations.
kPiecewiseDAE,
/// For modeling the system using either rigid or compliant contact,
/// Coulomb friction, and a first-order time discretization (which can
/// be applied to simulating the system without an integrator).
kDiscretized,
/// For modeling the system using compliant contact, Coulomb friction,
/// and ordinary differential equations and simulating the system
/// through standard algorithms for solving initial value problems.
kContinuous
};
/// Constructor for the 2D rod system using the piecewise DAE (differential
/// algebraic equation) based approach, the discretization approach, or the
/// continuous ordinary differential equation based approach.
/// @param dt The integration step size. This step size cannot be reset
/// after construction.
/// @throws std::exception if @p dt is not positive and system_type is
/// kDiscretized or @p dt is not zero and system_type is
/// kPiecewiseDAE or kContinuous.
explicit Rod2D(SystemType system_type, double dt);
static const Rod2dStateVector<T>& get_state(
const systems::ContinuousState<T>& cstate) {
return dynamic_cast<const Rod2dStateVector<T>&>(cstate.get_vector());
}
static Rod2dStateVector<T>& get_mutable_state(
systems::ContinuousState<T>* cstate) {
return dynamic_cast<Rod2dStateVector<T>&>(cstate->get_mutable_vector());
}
static const Rod2dStateVector<T>& get_state(
const systems::Context<T>& context) {
return dynamic_cast<const Rod2dStateVector<T>&>(
context.get_continuous_state_vector());
}
static Rod2dStateVector<T>& get_mutable_state(systems::Context<T>* context) {
return dynamic_cast<Rod2dStateVector<T>&>(
context->get_mutable_continuous_state_vector());
}
/// Transforms dissipation (α) to damping, given a characteristic
// deformation.
double TransformDissipationToDampingAboutDeformation(
double characteristic_deformation) const {
// Equation (16) from [Hunt 1975], yields b = 3/2 * α * k * x. We can
// assume that the stiffness and dissipation are determined for a small
// deformation (x). Put another way, we determine the damping coefficient
// for a harmonic oscillator from linearizing the dissipation factor about
// the characteristic deformation: the system will behave like a harmonic
// oscillator oscillating about x = `characteristic_deformation` meters.
return dissipation_ * 1.5 * stiffness_ * characteristic_deformation *
half_length_;
}
/// Transforms damping (b) to dissipation (α) , given a characteristic
/// deformation.
double TransformDampingToDissipationAboutDeformation(
double characteristic_deformation, double b) const {
// See documentation for TransformDissipationToDampingAboutDeformation()
// for explanation of this formula.
return b / (1.5 * stiffness_ * characteristic_deformation * half_length_);
}
/// Gets the constraint force mixing parameter (CFM, used for discretized
/// systems only), which should lie in the interval [0, infinity].
double get_cfm() const {
return 1.0 /
(stiffness_ * dt_ + TransformDissipationToDampingAboutDeformation(
kCharacteristicDeformation));
}
/// Gets the error reduction parameter (ERP, used for discretized
/// systems only), which should lie in the interval [0, 1].
double get_erp() const {
return dt_ * stiffness_ /
(stiffness_ * dt_ + TransformDissipationToDampingAboutDeformation(
kCharacteristicDeformation));
}
/// Gets the generalized position of the rod, given a Context. The first two
/// components represent the location of the rod's center-of-mass, expressed
/// in the global frame. The third component represents the orientation of
/// the rod, measured counter-clockwise with respect to the x-axis.
Vector3<T> GetRodConfig(const systems::Context<T>& context) const {
return context.get_state()
.get_continuous_state()
.get_generalized_position()
.CopyToVector();
}
/// Gets the generalized velocity of the rod, given a Context. The first
/// two components represent the translational velocities of the
/// center-of-mass. The third component represents the angular velocity of
/// the rod.
Vector3<T> GetRodVelocity(const systems::Context<T>& context) const {
return context.get_state()
.get_continuous_state()
.get_generalized_velocity()
.CopyToVector();
}
/// Gets the acceleration (with respect to the positive y-axis) due to
/// gravity (i.e., this number should generally be negative).
double get_gravitational_acceleration() const { return g_; }
/// Sets the acceleration (with respect to the positive y-axis) due to
/// gravity (i.e., this number should generally be negative).
void set_gravitational_acceleration(double g) { g_ = g; }
/// Gets the coefficient of dynamic (sliding) Coulomb friction.
double get_mu_coulomb() const { return mu_; }
/// Sets the coefficient of dynamic (sliding) Coulomb friction.
void set_mu_coulomb(double mu) { mu_ = mu; }
/// Gets the mass of the rod.
double get_rod_mass() const { return mass_; }
/// Sets the mass of the rod.
void set_rod_mass(double mass) { mass_ = mass; }
/// Gets the half-length h of the rod.
double get_rod_half_length() const { return half_length_; }
/// Sets the half-length h of the rod.
void set_rod_half_length(double half_length) { half_length_ = half_length; }
/// Gets the rod moment of inertia.
double get_rod_moment_of_inertia() const { return J_; }
/// Sets the rod moment of inertia.
void set_rod_moment_of_inertia(double J) { J_ = J; }
/// Get compliant contact normal stiffness in N/m.
double get_stiffness() const { return stiffness_; }
/// Set compliant contact normal stiffness in N/m (>= 0).
void set_stiffness(double stiffness) {
DRAKE_DEMAND(stiffness >= 0);
stiffness_ = stiffness;
}
/// Get compliant contact normal dissipation in 1/velocity (s/m).
double get_dissipation() const { return dissipation_; }
/// Set compliant contact normal dissipation in 1/velocity (s/m, >= 0).
void set_dissipation(double dissipation) {
DRAKE_DEMAND(dissipation >= 0);
dissipation_ = dissipation;
}
/// Sets stiffness and dissipation for the rod from cfm and erp values (used
/// for discretized system implementations).
void SetStiffnessAndDissipation(double cfm, double erp) {
// These values were determined by solving the equations:
// cfm = 1 / (dt * stiffness + damping)
// erp = dt * stiffness / (dt * stiffness + damping)
// for k and b.
const double k = erp / (cfm * dt_);
const double b = (1 - erp) / cfm;
set_stiffness(k);
set_dissipation(TransformDampingToDissipationAboutDeformation(
kCharacteristicDeformation, b));
}
/// Get compliant contact static friction (stiction) coefficient `μ_s`.
double get_mu_static() const { return mu_s_; }
/// Set contact stiction coefficient (>= mu_coulomb). This has no
/// effect if the rod model is discretized.
void set_mu_static(double mu_static) {
DRAKE_DEMAND(mu_static >= mu_);
mu_s_ = mu_static;
}
/// Get the stiction speed tolerance (m/s).
double get_stiction_speed_tolerance() const { return v_stick_tol_; }
/// Set the stiction speed tolerance (m/s). This is the maximum slip
/// speed that we are willing to consider as sticking. For a given normal
/// force N this is the speed at which the friction force will be largest,
/// at `μ_s*N` where `μ_s` is the static coefficient of friction. This has no
/// effect if the rod model is not compliant.
void set_stiction_speed_tolerance(double v_stick_tol) {
DRAKE_DEMAND(v_stick_tol > 0);
v_stick_tol_ = v_stick_tol;
}
/// Gets the rotation matrix that transforms velocities from a sliding
/// contact frame to the global frame.
/// @param xaxis_velocity The velocity of the rod at the point of contact,
/// projected along the +x-axis.
/// @returns a 2x2 orthogonal matrix with first column set to the contact
/// normal, which is +y ([0 1]) and second column set to the
/// direction of sliding motion, ±x (±[1 0]). Both directions are
/// expressed in the global frame.
/// @note Aborts if @p xaxis_velocity is zero.
Matrix2<T> GetSlidingContactFrameToWorldTransform(
const T& xaxis_velocity) const;
/// Gets the rotation matrix that transforms velocities from a non-sliding
/// contact frame to the global frame. Note: all such non-sliding frames are
/// identical for this example.
/// @returns a 2x2 orthogonal matrix with first column set to the contact
/// normal, which is +y ([0 1]) and second column set to the
/// contact tangent +x ([1 0]). Both directions are expressed in
/// the global frame.
Matrix2<T> GetNonSlidingContactFrameToWorldTransform() const;
/// Checks whether the system is in an impacting state, meaning that the
/// relative velocity along the contact normal between the rod and the
/// halfspace is such that the rod will begin interpenetrating the halfspace
/// at any time Δt in the future (i.e., Δt > 0). If the context does not
/// correspond to a configuration where the rod and halfspace are contacting,
/// this method returns `false`.
bool IsImpacting(const systems::Context<T>& context) const;
/// Gets the integration step size for the discretized system.
/// @returns 0 if this is a DAE-based system.
double get_integration_step_size() const { return dt_; }
/// Gets the model and simulation type for this system.
SystemType get_system_type() const { return system_type_; }
/// Return net contact forces as a spatial force F_Ro_W=(fx,fy,τ) where
/// translational force f_Ro_W=(fx,fy) is applied at the rod origin Ro,
/// and torque t_R=τ is the moment due to the contact forces actually being
/// applied elsewhere. The returned spatial force may be the resultant of
/// multiple active contact points. Only valid for simulation type
/// kContinuous.
Vector3<T> CalcCompliantContactForces(
const systems::Context<T>& context) const;
/// Gets the number of witness functions for the system active in the system
/// for a given state (using @p context).
int DetermineNumWitnessFunctions(const systems::Context<T>& context) const;
/// Returns the state of this rod.
const systems::OutputPort<T>& state_output() const {
return *state_output_port_;
}
/// Utility method for determining the World frame location of one of three
/// points on the rod whose origin is Ro. Let r be the half-length of the rod.
/// Define point P = Ro+k*r where k = { -1, 0, 1 }. This returns p_WP.
/// @param x The horizontal location of the rod center of mass (expressed in
/// the world frame).
/// @param y The vertical location of the rod center of mass (expressed in
/// the world frame).
/// @param k The rod endpoint (k=+1 indicates the rod "right" endpoint,
/// k=-1 indicates the rod "left" endpoint, and k=0 indicates the
/// rod origin; each of these are described in the primary class
/// documentation.
/// @param ctheta cos(theta), where θ is the orientation of the rod (as
/// described in the primary class documentation).
/// @param stheta sin(theta), where θ is the orientation of the rod (as
/// described in the class documentation).
/// @param half_rod_len Half the length of the rod.
/// @returns p_WP, the designated point on the rod, expressed in the world
/// frame.
static Vector2<T> CalcRodEndpoint(const T& x, const T& y, int k,
const T& ctheta, const T& stheta,
double half_rod_len);
/// Given a location p_WC of a point C in the World frame, define the point Rc
/// on the rod that is coincident with C, and report Rc's World frame velocity
/// v_WRc. We're given p_WRo=(x,y) and V_WRo = (v_WRo,w_WR) =
/// (xdot,ydot,thetadot).
/// @param p_WRo The center-of-mass of the rod, expressed in the world frame.
/// @param v_WRo The translational velocity of the rod, expressed in the
/// world frame.
/// @param w_WR The angular velocity of the rod.
/// @param p_WC The location of a point on the rod.
/// @returns The translational velocity of p_WC, expressed in the world frame.
static Vector2<T> CalcCoincidentRodPointVelocity(
const Vector2<T>& p_WRo, const Vector2<T>& v_WRo,
const T& w_WR, // aka thetadot
const Vector2<T>& p_WC);
/// Gets the point(s) of contact for the 2D rod.
/// @p context The context storing the current configuration and velocity of
/// the rod.
/// @p points Contains the contact points (those rod endpoints touching or
/// lying within the ground halfspace) on return. This function
/// aborts if @p points is null or @p points is non-empty.
void GetContactPoints(const systems::Context<T>& context,
std::vector<Vector2<T>>* points) const;
/// Gets the tangent velocities for all contact points.
/// @p context The context storing the current configuration and velocity of
/// the rod.
/// @p points The set of context points.
/// @p vels Contains the velocities (measured along the x-axis) on return.
/// This function aborts if @p vels is null. @p vels will be resized
/// appropriately (to the same number of elements as @p points) on
/// return.
void GetContactPointsTangentVelocities(const systems::Context<T>& context,
const std::vector<Vector2<T>>& points,
std::vector<T>* vels) const;
/// Initializes the contact data for the rod, given a set of contact points.
/// Aborts if data is null or if `points.size() != tangent_vels.size()`.
/// @param points a vector of contact points, expressed in the world frame.
/// @param tangent_vels a vector of tangent velocities at the contact points,
/// measured along the positive x-axis.
/// @param[out] data the rigid contact problem data.
void CalcConstraintProblemData(const systems::Context<T>& context,
const std::vector<Vector2<T>>& points,
const std::vector<T>& tangent_vels,
ConstraintAccelProblemData<T>* data) const;
/// Initializes the impacting contact data for the rod, given a set of contact
/// points. Aborts if data is null.
/// @param points a vector of contact points, expressed in the world frame.
/// @param[out] data the rigid impact problem data.
void CalcImpactProblemData(const systems::Context<T>& context,
const std::vector<Vector2<T>>& points,
ConstraintVelProblemData<T>* data) const;
private:
friend class Rod2DDAETest;
friend class Rod2DDAETest_RigidContactProblemDataBallistic_Test;
Vector3<T> GetJacobianRow(const systems::Context<T>& context,
const Vector2<T>& p, const Vector2<T>& dir) const;
Vector3<T> GetJacobianDotRow(const systems::Context<T>& context,
const Vector2<T>& p,
const Vector2<T>& dir) const;
static Matrix2<T> GetRotationMatrixDerivative(T theta, T thetadot);
Matrix3<T> GetInertiaMatrix() const;
T GetSlidingVelocityTolerance() const;
MatrixX<T> solve_inertia(const MatrixX<T>& B) const;
int get_k(const systems::Context<T>& context) const;
void DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const override;
void CalcDiscreteUpdate(const systems::Context<T>& context,
systems::DiscreteValues<T>* discrete_state) const;
void SetDefaultState(const systems::Context<T>& context,
systems::State<T>* state) const override;
Vector3<T> ComputeExternalForces(const systems::Context<T>& context) const;
Matrix3<T> GetInverseInertiaMatrix() const;
void CalcTwoContactNoSlidingForces(const systems::Context<T>& context,
Vector2<T>* fN, Vector2<T>* fF) const;
void CalcTwoContactSlidingForces(const systems::Context<T>& context,
Vector2<T>* fN, Vector2<T>* fF) const;
Vector2<T> CalcStickingImpactImpulse(
const systems::Context<T>& context) const;
Vector2<T> CalcFConeImpactImpulse(const systems::Context<T>& context) const;
void CalcAccelerationsCompliantContactAndBallistic(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const;
// 2D cross product returns a scalar. This is the z component of the 3D
// cross product [ax ay 0] × [bx by 0]; the x,y components are zero.
static T cross2(const Vector2<T>& a, const Vector2<T>& b) {
return a[0] * b[1] - b[0] * a[1];
}
// Calculates the cross product [0 0 ω] × [rx ry 0] and returns the first
// two elements of the result (the z component is zero).
static Vector2<T> w_cross_r(const T& w, const Vector2<T>& r) {
return w * Vector2<T>(-r[1], r[0]);
}
// Quintic step function approximation used by Stribeck friction model.
static T step5(const T& x);
// Friction model used in the continuous model.
static T CalcMuStribeck(const T& us, const T& ud, const T& v);
// The constraint solver.
ConstraintSolver<T> solver_;
// Solves linear complementarity problems for the discretized system.
solvers::MobyLCPSolver<T> lcp_;
// The system type, unable to be changed after object construction.
const SystemType system_type_;
// TODO(edrumwri,sherm1) Document these defaults once they stabilize.
double dt_{0.}; // Step-size for the discretization approach.
double mass_{1.}; // The mass of the rod (kg).
double half_length_{1.}; // The length of the rod (m).
double mu_{1000.}; // The (dynamic) coefficient of friction.
double g_{-9.81}; // The acceleration due to gravity (in y direction).
double J_{1.}; // The moment of the inertia of the rod.
// Compliant contact parameters.
double stiffness_{10000}; // Normal stiffness of the ground plane (N/m).
double dissipation_{1}; // Dissipation factor in 1/velocity (s/m).
double mu_s_{mu_}; // Static coefficient of friction (>= mu).
double v_stick_tol_{1e-5}; // Slip speed below which the compliant model
// considers the rod to be in stiction.
// Characteristic deformation is 1mm for a 1m (unit length) rod half-length.
double kCharacteristicDeformation{1e-3};
// Output ports.
const systems::OutputPort<T>* state_output_port_{nullptr};
};
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/README.md | # Multibody Dynamics Constraints
This documentation describes `ConstraintSolver`, a prototype solver for mixed
linear complementarity problems (MLCPs). In particular, problems corresponding
to rigid multi-body systems with bilateral and/or unilateral constraints as well
as polyhedral approximations of friction cone constraints. Although very feature
complete, we found out that this solver performs very badly for real robotic
applications. The reason being that even though there is good theory on the
existence and uniqueness of MLCP solutions, these problems are often badly
ill-conditioned numerically, leading to catastrophic failures in practice.
Moreover, we found out that performance does not scale well with the number of
constraints. This lack of robustness and performance is the reason why you find
this implementation within an example and not as part of a supported main Drake
feature.
`ConstraintSolver` helps solve computational dynamics problems with
algebraic constraints, like differential algebraic equations:<pre>
ẋ = f(x, t, λ)
0 = g(x, t, λ)
</pre>
where `g()` corresponds to one or more constraint equations and λ
is typically interpretable as "forces" that enforce the constraints.
`ConstraintSolver` permits solving initial value problems subject to such
constraints and does so in a manner very different from "smoothing methods"
(also known as "penalty methods"). Smoothing methods have traditionally required
significant computation to maintain `g = 0` to high accuracy (and typically
yielding what is known in ODE and DAE literature as a "computationally stiff
system").
We also provide the core components of an efficient first-order integration
scheme for a system with both compliant and rigid unilateral constraints. Such
a system arises in many contact problems, where the correct modeling parameters
would yield a computationally stiff system. The integration scheme is
low-accuracy but very stable for mechanical systems, even when the algebraic
variables (i.e., the constraint forces) are computed to low accuracy.
This discussion will provide necessary background material in:
- [Constraint types](#constraint_types)
- [Constraint stabilization](#constraint_stabilization)
- [Constraint Jacobians](#constraint_Jacobians)
- [Contact surface constraints](#contact_surface_constraints)
A prodigious number of variables will be referenced throughout the discussion on
constraints. Variables common to both acceleration-level constraints and
velocity-level constraints will be covered in [Variable
definitions](#constraint_variable_defs). References for this discussion will be
provided in [References](#constraint_references).
## <a id="constraint_types"></a>Constraint types
Constraints can be categorized as either bilateral ("two-sided" constraints,
e.g., g(q) = 0) or unilateral ("one-sided" constraints, e.g., g(q) ≥ 0).
Although the former can be realized through the latter using a pair of
inequality constraints, g(q) ≥ 0 and -g(q) ≥ 0; the constraint problem structure
distinguishes the two types to maximize computational efficiency in the solution
algorithms. It is assumed throughout this documentation that g(.) is a vector
function. In general, the constraint functions do not maintain consistent units:
the units of the iᵗʰ constraint function are not necessarily equivalent to the
units of the jᵗʰ dimension of g(.).
Constraints may be posed at the position level:<pre>
gₚ(t;q)
</pre>
at the velocity level:<pre>
gᵥ(t,q;v)
</pre>
or at the acceleration level:<pre>
gₐ(t,q,v;v̇)
</pre>
Additionally, constraints posed at any level can impose constraints on the
constraint forces, λ. For example, the position-level constraint:<pre>
gₚ(t,q;λ)
</pre>
could be used to enforce q ≥ 0, λ ≥ 0, and qλ = 0, a triplet of conditions
useful for, e.g., ensuring that a joint range-of-motion limit is respected
(q ≥ 0), guaranteeing that no constraint force is applied if the constraint
is not active (q > 0 implies λ = 0), and guaranteeing that the constraint force
can only push the connected links apart at the joint rather than act to "glue"
them together (λ > 0).
Note the semicolon in all of the above definitions of g() separates general
constraint dependencies (e.g., t,q,v in gₐ) from variables that must be
determined using the constraints (e.g., v̇ in gₐ).
<h4>Constraints with velocity-level unknowns</h4>
This documentation distinguishes
between equations that are posed at the position level but are
differentiated once with respect to time (i.e., to reduce the Differential
Algebraic Equation or Differential Complementarity Problem index [[Ascher
1998]](#Ascher1998)):<pre>
d/dt gₚ(t; q) = ġₚ(t, q; v)
</pre>
vs. equations that **must** be posed at the velocity-level
(i.e., nonholonomic constraints):<pre>
gᵥ(t, q; v).
</pre>
Both cases yield a constraint with velocity-level unknowns, thereby allowing the
constraint to be used in a velocity-level constraint formulation (e.g., an
Index-2 DAE or Index-2 DCP). *Only the former constraint may "drift" from zero
over time*, due to truncation and discretization errors, unless corrected. See
[Constraint stabilization](#constraint_stabilization) for further information.
<h4>Constraints with force and acceleration-level unknowns</h4>
A bilateral constraint equation with force and acceleration-level unknowns will
take the form:
<pre>
g̈ₚ(t,q,v;v̇) = 0
</pre>
if gₚ(.) has been differentiated twice with respect to time (for DAE/DCP index
reduction), or<pre>
ġᵥ(t,q,v;v̇) = 0
</pre>
if gᵥ(.) is nonholonomic and has been differentiated once with respect to time
(again, for DAE/DCP index reduction). As noted elsewhere, constraints can also
incorporate terms dependent on constraint forces, like:<pre>
g̈ₚ + λ = 0
</pre>
Making the constraint type above more general by permitting unknowns over λ
allows constraints to readily express, e.g., sliding Coulomb friction
constraints (of the form fₜ = μ⋅fₙ).
<h4>Complementarity conditions</h4>
Each unilateral constraint comprises a triplet of equations. For example:<pre>
gₐ(t,q,v;v̇,λ) ≥ 0
λ ≥ 0
gₐ(t,q,v;v̇,λ)⋅λ = 0
</pre>
which we will typically write in the common shorthand notation:<pre>
0 ≤ gₐ ⊥ λ ≥ 0
</pre>
Interpreting this triplet of constraint equations, two conditions become
apparent: (1) when the constraint is inactive (gₐ > 0), the constraint force
must be zero (λ = 0) and (2) the constraint force can only act in one
direction (λ ≥ 0). This triplet is known as a *complementarity constraint*.
## <a id="constraint_stabilization"></a>Constraint stabilization
Both truncation and rounding errors can prevent constraints from being
exactly satisfied. For example, consider the bilateral holonomic constraint
equation gₚ(t,q) = 0. Even if
gₚ(t₀,q(t₀)) = ġₚ(t₀,q(t₀),v(t₀)) = g̈ₚ(t₀,q(t₀),v(t₀),v̇(t₀)) = 0, it is often
true that gₚ(t₁,q(t₁)) will be nonzero for sufficiently large Δt = t₁ - t₀.
One way to address this constraint "drift" is through *dynamic stabilization*.
In particular, holonomic unilateral constraints that have been differentiated
twice with respect to time can be modified from:<pre>
0 ≤ g̈ₚ ⊥ λ ≥ 0
</pre>
to:<pre>
0 ≤ g̈ₚ + 2αġₚ + β²gₚ ⊥ λ ≥ 0
</pre>
and holonomic bilateral constraints can be modified from:<pre>
g̈ₚ = 0
</pre>
to:<pre>
g̈ₚ + 2αġₚ + β²gₚ = 0
</pre>
for non-negative scalar α and real β (α and β can also represent diagonal
matrices for greater generality). α and β, which both have units of 1/sec
(i.e., the reciprocal of unit time) are described more fully in
[[Baumgarte 1972]](#Baumgarte1972). The use of α and β above make correcting
position-level (gₚ) and velocity-level (gₚ̇) holonomic constraint errors
analogous to the dynamic problem of stabilizing a damped harmonic oscillator.
Given that analogy, 2α is the viscous damping coefficient and β² the stiffness
coefficient. These two coefficients make predicting the motion of the oscillator
challenging to interpret, so one typically converts them to undamped angular
frequency (ω₀) and damping ratio (ζ) via the following equations:<pre>
ω₀² = β²
ζ = α/β
</pre>
To eliminate constraint errors as quickly as possible, one strategy used in
commercial software uses ζ=1, implying *critical damping*, and undamped angular
frequency ω₀ that is high enough to correct errors rapidly but low enough to
avoid computational stiffness. Picking that parameter is considered to be more
art than science (see [[Ascher 1995]](#Ascher1995)). Given desired ω₀ and ζ,
α and β are set using the equations above.
## <a id="constraint_Jacobians"></a>Constraint Jacobian matrices
Much of the problem data necessary to account for constraints in dynamical
systems refers to particular Jacobian matrices. These Jacobian matrices arise
through the time derivatives of the constraint equations, e.g.:<pre>
ġₚ = ∂gₚ/∂q⋅q̇
</pre>
where we assume that gₚ above is a function only of position (not time) for
simplicity. The constraint solver currently operates on generalized velocities,
requiring us to leverage the relationship:<pre>
q̇ = N(q)⋅v
</pre>
using the left-invertible matrix N(q) between the time derivative of generalized
coordinates and generalized velocities. This yields
the requisite form:<pre>
ġₚ = ∂gₚ/∂q⋅N(q)⋅v
</pre>
Fortunately, adding new constraints defined in the form `gₚ(t,q)` does not
require considering this distinction using the operator paradigm (see, e.g.,
`N_mult` in `ConstraintAccelProblemData` and `ConstraintVelProblemData`). Since
the Jacobians are described completely by the equation `ġₚ = ∂gₚ/∂q⋅N(q)⋅v +
∂c/∂t`, one can simply evaluate `ġₚ - ∂g/∂t` for a given `v`; no Jacobian
matrix need be formed explicitly.
## <a id="contact_surface_constraints"></a>Contact surface constraints
Consider two points pᵢ and pⱼ on rigid bodies i and j, respectively, and
assume that at a certain configuration of the two bodies, ᶜq, the two
points are coincident at a single location in space,
p(ᶜq). To constrain the motion of pᵢ and pⱼ to the contact
surface as the bodies move, one can introduce the constraint
g(q) = n(q)ᵀ(pᵢ(q) - pⱼ(q)), where n(q) is the common surface normal
expressed in the world frame. gₚ(q) is a unilateral constraint, meaning that
complementarity constraints are necessary (see [Constraint
types](#constraint_types)):
<pre>
0 ≤ gₚ ⊥ λ ≥ 0
</pre>
<h4>Differentiating gₚ(.) with respect to time</h4>
As usual, gₚ(.) must be differentiated (with respect to time) once to use the
contact constraint in velocity-level constraint formulation or twice to use
it in a force/acceleration-level formulation. Differentiating gₚ(q) once with
respect to time yields:<pre>
ġₚ(q,v) = nᵀ(ṗᵢ - ṗⱼ) + ṅᵀ(pᵢ - pⱼ);
</pre>
one more differentiation with respect to time yields:<pre>
g̈ₚ(q,v,v̇) = nᵀ(p̈ᵢ - p̈ⱼ) + 2ṅᵀ(ṗᵢ - ṗⱼ) + n̈ᵀ(pᵢ - pⱼ).
</pre>
The non-negativity condition on the constraint force magnitudes (λ ≥ 0)
keeps the contact force along the contact normal compressive, as is consistent
with a non-adhesive contact model.
A more substantial discussion on the kinematics of contact can be found in
[[Pfeiffer 1996]](#Pfeiffer1996), Ch. 4.

**Figure 1:** Illustration of the interpretation of non-interpenetration
constraints when two boxes are interpenetrating (right). The boxes prior to
contact are shown at left, and are shown in the middle figure at the initial
time of contact; the surface normal n̂ is shown in this figure as well. The
bodies interpenetrate over time as the constraint becomes violated (e.g., by
constraint drift). Nevertheless, n̂ is tracked over time from its initial
direction (and definition relative to the blue body). σ represents the signed
distance the bodies must be translated along n̂ so that they are osculating
(kissing).
<h4>Constraint softening</h4>
The non-interpenetration constraint can be softened by adding a term to the time
derivative of the equation:<pre>
0 ≤ ġₚ ⊥ λ ≥ 0
</pre>
resulting in:<pre>
0 ≤ ġₚ + γλ ⊥ λ ≥ 0
</pre>
for γ ≥ 0.
<h4>Constraint stabilization</h4>
As discussed in [Constraint stabilization](#constraint_stabilization), the drift
induced by solving an Index-0 DAE or DCP (that has been reduced from an Index-3
DAE or DCP) can be mitigated through one of several strategies, one of which is
Baumgarte Stabilization. The typical application of Baumgarte Stabilization will
use the second time derivative of the complementarity condition:
<pre>
0 ≤ g̈ₚ ⊥ λ ≥ 0
</pre>
Baumgarte Stabilization would be layered on top of this equation, resulting in:
<pre>
0 ≤ g̈ₚ + 2αġₚ + β²gₚ ⊥ λ ≥ 0
</pre>
<h4>Effects of constraint softening and stabilization on
constraint problem data</h4>
- Constraint regularization/softening is effected through `gammaN`
- Constraint stabilization is effected through `kN`
- Note that neither Baumgarte Stabilization nor constraint
regularization/softening affects the definition of g(.)'s Jacobian
operators. That is, `N_mult` and either `N_minus_muQ_transpose_mult` (for
`ConstraintAccelProblemData`) or `N_transpose_mult` (for
`ConstraintVelProblemData`)
## <a id="constraint_variable_defs"></a>Variable definitions
- `nb` The number of bilateral constraint equations (nb ≥ 0)
- `nk` The number of edges in a polygonal approximation to a friction
cone (nk ≥ 4 for contacts between three-dimensional bodies, nk = 2 for
contacts between two-dimensional bodies). Note that nk = 2nr (where
nr is defined immediately below).
- `nr` *Half* the number of edges in a polygonal approximation to a
friction cone. (nr ≥ 2 for contacts between three-dimensional bodies,
nr = 1 for contacts between two-dimensional bodies).
- `nc` The number of contact surface constraint equations.
- `nck` The total number of edges in the polygonal approximations to the
nc friction cones corresponding to the nc point contacts. Note that
nck = 2ncr (where ncr is defined immediately below).
- `ncr` *Half* the total number of edges in the polygonal approximations to the
nc friction cones corresponding to the nc point contacts.
- `nv` The dimension of the system generalized velocity / force.
- `nq` The dimension of the system generalized coordinates.
- `v` The system's generalized velocity vector (of dimension nv), which is a
linear transformation of the time derivative of the system's
generalized coordinates.
- `q` The generalized coordinate vector of the system (of dimension nq).
- `t` The system time variable (a non-negative real number).
- `nu` The number of "generic" (non-contact related) unilateral constraint
equations.
- `α` A non-negative, real valued scalar used to correct the time derivative
of position constraint errors (i.e., "stabilize" the constraints) via
an error feedback process (Baumgarte Stabilization).
- `β` A non-negative, real valued scalar used to correct position constraint
errors via the same error feedback process (Baumgarte
Stabilization) that uses α.
- `γ` Non-negative, real valued scalar used to regularize constraints.
## <a id="constraint_references"></a>References
Sources referenced within the multibody constraint documentation.
<ul>
<li id="Anitescu1997"> [Anitescu 1997] M. Anitescu and F. Potra. Formulating
Dynamic Multi-Rigid-Body Contact Problems with Friction as Solvable Linear
Complementarity Problems. Nonlinear Dynamics, 14, pp. 231-247. 1997.</li>
<li id="Ascher1995"> [Ascher 1995] U. Ascher, H. Chin, L. Petzold, and
S. Reich. Stabilization of constrained mechanical systems with DAEs and
invariant manifolds. J. Mech. Struct. Machines, 23, pp. 135-158. 1995.</li>
<li id="Ascher1998"> [Ascher 1998] U. Ascher and L. Petzold. Computer Methods
for Ordinary Differential Equations and Differential Algebraic Equations.
SIAM, Philadelphia. 1998.</li>
<li id="Baumgarte1972"> [Baumgarte 1972] J. Baumgarte. Stabilization of
constraints and integrals of motion in dynamical systems. Comp. Math. Appl.
Mech. Engr., 1, pp. 1-16. 1972.</li>
<li id="Catto2011"> [Catto 2011] E. Catto. Soft Constraints: Reinventing the
Spring. Game Developers Conference presentation, 2011.</li>
<li id="Cottle1992"> [Cottle 1992] R. Cottle, J-S. Pang, and R. Stone. The
Linear Complementarity Problem. Academic Press, Boston. 1992.</li>
<li id="Hairer1996"> [Hairer 1996] E. Hairer and G. Wanner. Solving ordinary
differential equations II: stiff and differential algebraic problems, 2nd ed.
Springer-Verlag, Berlin. 1996.</li>
<li id="Lacoursiere2007"> [Lacoursiere 2007] C. Lacoursière. Ghosts and
Machines: Regularized Variational Methods for Interactive Simulations of
Multibodies with Dry Frictional Contacts. Umeå University. 2007.</li>
<li id="Pfeiffer1996"> [Pfeiffer 1996] F. Pfeiffer and C. Glocker. Multibody
Dynamics with Unilateral Contacts, John Wiley & Sons, New York. 1996.</li>
<li id="Sciavicco2000"> [Sciavicco 2000] L. Sciavicco and B. Siciliano.
Modeling and Control of Robot Manipulators, 2nd ed. Springer-Verlag, London.
2000.</li>
</ul>
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d_geometry.cc | #include "drake/examples/rod2d/rod2d_geometry.h"
#include <memory>
#include "drake/geometry/geometry_frame.h"
#include "drake/geometry/geometry_ids.h"
#include "drake/geometry/geometry_instance.h"
#include "drake/geometry/geometry_roles.h"
#include "drake/geometry/kinematics_vector.h"
#include "drake/geometry/rgba.h"
#include "drake/math/rigid_transform.h"
#include "drake/math/rotation_matrix.h"
namespace drake {
namespace examples {
namespace rod2d {
using Eigen::Vector3d;
using math::RigidTransformd;
using math::RotationMatrixd;
const Rod2dGeometry* Rod2dGeometry::AddToBuilder(
double radius, double length, systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& rod2d_state_port,
geometry::SceneGraph<double>* scene_graph) {
DRAKE_THROW_UNLESS(builder != nullptr);
DRAKE_THROW_UNLESS(scene_graph != nullptr);
auto rod2d_geometry = builder->AddSystem(std::unique_ptr<Rod2dGeometry>(
new Rod2dGeometry(radius, length, scene_graph)));
builder->Connect(rod2d_state_port, rod2d_geometry->get_input_port());
builder->Connect(
rod2d_geometry->get_output_port(),
scene_graph->get_source_pose_port(rod2d_geometry->source_id_));
return rod2d_geometry;
}
Rod2dGeometry::Rod2dGeometry(double radius, double length,
geometry::SceneGraph<double>* scene_graph) {
DRAKE_THROW_UNLESS(scene_graph != nullptr);
source_id_ = scene_graph->RegisterSource("rod2d");
frame_id_ =
scene_graph->RegisterFrame(source_id_, geometry::GeometryFrame("rod2d"));
geometry::GeometryId geometry = scene_graph->RegisterGeometry(
source_id_, frame_id_,
std::make_unique<geometry::GeometryInstance>(
RigidTransformd{},
std::make_unique<geometry::Cylinder>(radius, length), "rod2d"));
geometry::IllustrationProperties illus_props;
illus_props.AddProperty("phong", "diffuse", geometry::Rgba(0.7, 0.7, 0.7, 1));
scene_graph->AssignRole(source_id_, geometry, illus_props);
// Only uses the qs of the state (x, y, θ).
this->DeclareVectorInputPort("state", 6);
this->DeclareAbstractOutputPort("geometry_pose",
&Rod2dGeometry::OutputGeometryPose);
}
Rod2dGeometry::~Rod2dGeometry() = default;
void Rod2dGeometry::OutputGeometryPose(
const systems::Context<double>& context,
geometry::FramePoseVector<double>* poses) const {
DRAKE_DEMAND(frame_id_.is_valid());
const auto& state = get_input_port().Eval(context);
// Converts the configuration of the rod to a pose in ℜ³. The 2D system lies
// on the x-y plane with +y up. In 3D, we place it on the y = 0 plane with +z
// up. This is compatible with the fact that a drake cylinder has its axis
// aligned with the z-axis.
math::RigidTransformd pose(RotationMatrixd::MakeYRotation(state(2) + M_PI_2),
Vector3d(state(0), 0, state(1)));
*poses = {{frame_id_, pose}};
}
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d_state_vector.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace rod2d {
/// Describes the row indices of a Rod2dStateVector.
struct Rod2dStateVectorIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 6;
// The index of each individual coordinate.
static const int kX = 0;
static const int kY = 1;
static const int kTheta = 2;
static const int kXdot = 3;
static const int kYdot = 4;
static const int kThetadot = 5;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `Rod2dStateVectorIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class Rod2dStateVector final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef Rod2dStateVectorIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c x defaults to 0.0 m.
/// @arg @c y defaults to 0.0 m.
/// @arg @c theta defaults to 0.0 rad.
/// @arg @c xdot defaults to 0.0 m/s.
/// @arg @c ydot defaults to 0.0 m/s.
/// @arg @c thetadot defaults to 0.0 rad/s.
Rod2dStateVector() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_x(0.0);
this->set_y(0.0);
this->set_theta(0.0);
this->set_xdot(0.0);
this->set_ydot(0.0);
this->set_thetadot(0.0);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
Rod2dStateVector(const Rod2dStateVector& other)
: drake::systems::BasicVector<T>(other.values()) {}
Rod2dStateVector(Rod2dStateVector&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
Rod2dStateVector& operator=(const Rod2dStateVector& other) {
this->values() = other.values();
return *this;
}
Rod2dStateVector& operator=(Rod2dStateVector&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_x(symbolic::Variable("x"));
this->set_y(symbolic::Variable("y"));
this->set_theta(symbolic::Variable("theta"));
this->set_xdot(symbolic::Variable("xdot"));
this->set_ydot(symbolic::Variable("ydot"));
this->set_thetadot(symbolic::Variable("thetadot"));
}
[[nodiscard]] Rod2dStateVector<T>* DoClone() const final {
return new Rod2dStateVector;
}
/// @name Getters and Setters
//@{
/// Horizontal location of the center-of-mass.
/// @note @c x is expressed in units of m.
const T& x() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kX);
}
/// Setter that matches x().
void set_x(const T& x) {
ThrowIfEmpty();
this->SetAtIndex(K::kX, x);
}
/// Fluent setter that matches x().
/// Returns a copy of `this` with x set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_x(const T& x) const {
Rod2dStateVector<T> result(*this);
result.set_x(x);
return result;
}
/// Vertical location of the center-of-mass.
/// @note @c y is expressed in units of m.
const T& y() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kY);
}
/// Setter that matches y().
void set_y(const T& y) {
ThrowIfEmpty();
this->SetAtIndex(K::kY, y);
}
/// Fluent setter that matches y().
/// Returns a copy of `this` with y set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_y(const T& y) const {
Rod2dStateVector<T> result(*this);
result.set_y(y);
return result;
}
/// Angle of the rod (measured counter-clockwise).
/// @note @c theta is expressed in units of rad.
const T& theta() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta);
}
/// Setter that matches theta().
void set_theta(const T& theta) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta, theta);
}
/// Fluent setter that matches theta().
/// Returns a copy of `this` with theta set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_theta(const T& theta) const {
Rod2dStateVector<T> result(*this);
result.set_theta(theta);
return result;
}
/// Velocity of the horizontal location of the center-of-mass.
/// @note @c xdot is expressed in units of m/s.
const T& xdot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kXdot);
}
/// Setter that matches xdot().
void set_xdot(const T& xdot) {
ThrowIfEmpty();
this->SetAtIndex(K::kXdot, xdot);
}
/// Fluent setter that matches xdot().
/// Returns a copy of `this` with xdot set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_xdot(const T& xdot) const {
Rod2dStateVector<T> result(*this);
result.set_xdot(xdot);
return result;
}
/// Velocity of the vertical location of the center-of-mass.
/// @note @c ydot is expressed in units of m/s.
const T& ydot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kYdot);
}
/// Setter that matches ydot().
void set_ydot(const T& ydot) {
ThrowIfEmpty();
this->SetAtIndex(K::kYdot, ydot);
}
/// Fluent setter that matches ydot().
/// Returns a copy of `this` with ydot set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_ydot(const T& ydot) const {
Rod2dStateVector<T> result(*this);
result.set_ydot(ydot);
return result;
}
/// Angular velocity of the rod.
/// @note @c thetadot is expressed in units of rad/s.
const T& thetadot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kThetadot);
}
/// Setter that matches thetadot().
void set_thetadot(const T& thetadot) {
ThrowIfEmpty();
this->SetAtIndex(K::kThetadot, thetadot);
}
/// Fluent setter that matches thetadot().
/// Returns a copy of `this` with thetadot set to a new value.
[[nodiscard]] Rod2dStateVector<T> with_thetadot(const T& thetadot) const {
Rod2dStateVector<T> result(*this);
result.set_thetadot(thetadot);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& x_ref = this->GetAtIndex(K::kX);
a->Visit(drake::MakeNameValue("x", &x_ref));
T& y_ref = this->GetAtIndex(K::kY);
a->Visit(drake::MakeNameValue("y", &y_ref));
T& theta_ref = this->GetAtIndex(K::kTheta);
a->Visit(drake::MakeNameValue("theta", &theta_ref));
T& xdot_ref = this->GetAtIndex(K::kXdot);
a->Visit(drake::MakeNameValue("xdot", &xdot_ref));
T& ydot_ref = this->GetAtIndex(K::kYdot);
a->Visit(drake::MakeNameValue("ydot", &ydot_ref));
T& thetadot_ref = this->GetAtIndex(K::kThetadot);
a->Visit(drake::MakeNameValue("thetadot", &thetadot_ref));
}
/// See Rod2dStateVectorIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return Rod2dStateVectorIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(x());
result = result && !isnan(y());
result = result && !isnan(theta());
result = result && !isnan(xdot());
result = result && !isnan(ydot());
result = result && !isnan(thetadot());
return result;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The Rod2dStateVector vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d.cc | #include "drake/examples/rod2d/rod2d.h"
#include <algorithm>
#include <limits>
#include <memory>
#include <stdexcept>
#include <utility>
#include <vector>
#include "drake/common/drake_assert.h"
#include "drake/systems/framework/basic_vector.h"
// TODO(edrumwri,sherm1) This code is currently written out mostly in scalars
// but should be done in 2D vectors instead to make it more compact, easier to
// understand, and easier to relate to our 3D code.
namespace drake {
namespace examples {
namespace rod2d {
template <typename T>
Rod2D<T>::Rod2D(SystemType system_type, double dt)
: system_type_(system_type), dt_(dt) {
// Verify that the simulation approach is either piecewise DAE or
// compliant ODE.
if (system_type == SystemType::kDiscretized) {
if (dt <= 0.0)
throw std::logic_error(
"Discretization approach must be constructed using"
" strictly positive step size.");
// Discretization approach requires three position variables and
// three velocity variables, all discrete, and periodic update.
this->DeclarePeriodicDiscreteUpdateEvent(dt, 0.0,
&Rod2D<T>::CalcDiscreteUpdate);
auto state_index = this->DeclareDiscreteState(6);
state_output_port_ =
&this->DeclareStateOutputPort("state_output", state_index);
} else {
if (dt != 0)
throw std::logic_error(
"Piecewise DAE and compliant approaches must be "
"constructed using zero step size.");
// Both piecewise DAE and compliant approach require six continuous
// variables.
auto state_index = this->DeclareContinuousState(Rod2dStateVector<T>(), 3, 3,
0 /* q, v, z */);
state_output_port_ =
&this->DeclareStateOutputPort("state_output", state_index);
}
// TODO(edrumwri): When type is SystemType::kPiecewiseDAE, allocate the
// abstract mode variables.
this->DeclareInputPort(systems::kUseDefaultName, systems::kVectorValued, 3);
}
// Computes the external forces on the rod.
// @returns a three dimensional vector corresponding to the forces acting at
// the center-of-mass of the rod (first two components) and the
// last corresponding to the torque acting on the rod (final
// component). All forces and torques are expressed in the world
// frame.
template <class T>
Vector3<T> Rod2D<T>::ComputeExternalForces(
const systems::Context<T>& context) const {
// Compute the external forces (expressed in the world frame).
const int port_index = 0;
const VectorX<T>& input = this->get_input_port(port_index).Eval(context);
const Vector3<T> fgrav(0, mass_ * get_gravitational_acceleration(), 0);
const Vector3<T> fapplied = input.segment(0, 3);
return fgrav + fapplied;
}
template <class T>
int Rod2D<T>::DetermineNumWitnessFunctions(const systems::Context<T>&) const {
// No witness functions if this is not a piecewise DAE model.
if (system_type_ != SystemType::kPiecewiseDAE) return 0;
// TODO(edrumwri): Flesh out this stub.
throw std::domain_error("Rod2D<T>::DetermineNumWitnessFunctions is stubbed");
}
/// Gets the integer variable 'k' used to determine the point of contact
/// indicated by the current mode.
/// @throws std::exception if this is a discretized system (implying that
/// modes are unused).
/// @returns the value -1 to indicate the bottom of the rod (when theta = pi/2),
/// +1 to indicate the top of the rod (when theta = pi/2), or 0 to
/// indicate the mode where both endpoints of the rod are contacting
/// the halfspace.
template <class T>
int Rod2D<T>::get_k(const systems::Context<T>& context) const {
if (system_type_ != SystemType::kPiecewiseDAE)
throw std::logic_error("'k' is only valid for piecewise DAE approach.");
const int k = context.template get_abstract_state<int>(1);
DRAKE_DEMAND(std::abs(k) <= 1);
return k;
}
template <class T>
Vector2<T> Rod2D<T>::CalcRodEndpoint(const T& x, const T& y, int k,
const T& ctheta, const T& stheta,
double half_rod_len) {
const T cx = x + k * ctheta * half_rod_len;
const T cy = y + k * stheta * half_rod_len;
return Vector2<T>(cx, cy);
}
template <class T>
Vector2<T> Rod2D<T>::CalcCoincidentRodPointVelocity(
const Vector2<T>& p_WRo, const Vector2<T>& v_WRo,
const T& w_WR, // aka thetadot
const Vector2<T>& p_WC) {
const Vector2<T> p_RoC_W = p_WC - p_WRo; // Vector from R origin to C, in W.
const Vector2<T> v_WRc = v_WRo + w_cross_r(w_WR, p_RoC_W);
return v_WRc;
}
/// Solves MX = B for X, where M is the generalized inertia matrix of the rod
/// and is defined in the following manner:<pre>
/// M = | m 0 0 |
/// | 0 m 0 |
/// | 0 0 J | </pre>
/// where `m` is the mass of the rod and `J` is its moment of inertia.
template <class T>
MatrixX<T> Rod2D<T>::solve_inertia(const MatrixX<T>& B) const {
const T inv_mass = 1.0 / get_rod_mass();
const T inv_J = 1.0 / get_rod_moment_of_inertia();
Matrix3<T> iM;
// clang-format off
iM << inv_mass, 0, 0,
0, inv_mass, 0,
0, 0, inv_J;
// clang-format on
return iM * B;
}
/// The velocity tolerance for sliding.
template <class T>
T Rod2D<T>::GetSlidingVelocityTolerance() const {
// TODO(edrumwri): Change this coarse tolerance, which is only guaranteed to
// be reasonable for rod locations near the world origin and rod velocities
// of unit magnitude, to a computed tolerance that can account for these
// assumptions being violated.
return 100 * std::numeric_limits<double>::epsilon();
}
// Gets the time derivative of a 2D rotation matrix.
template <class T>
Matrix2<T> Rod2D<T>::GetRotationMatrixDerivative(T theta, T thetadot) {
using std::cos;
using std::sin;
const T cth = cos(theta), sth = sin(theta);
Matrix2<T> Rdot;
Rdot << -sth, -cth, cth, -sth;
return Rdot * thetadot;
}
template <class T>
void Rod2D<T>::GetContactPoints(const systems::Context<T>& context,
std::vector<Vector2<T>>* points) const {
using std::cos;
using std::sin;
DRAKE_DEMAND(points != nullptr);
DRAKE_DEMAND(points->empty());
const Vector3<T> q = GetRodConfig(context);
const T& x = q[0];
const T& y = q[1];
T cth = cos(q[2]), sth = sin(q[2]);
// Get the two rod endpoint locations.
const T half_len = get_rod_half_length();
const Vector2<T> pa = CalcRodEndpoint(x, y, -1, cth, sth, half_len);
const Vector2<T> pb = CalcRodEndpoint(x, y, +1, cth, sth, half_len);
// If an endpoint touches the ground, add it as a contact point.
if (pa[1] <= 0) points->push_back(pa);
if (pb[1] <= 0) points->push_back(pb);
}
template <class T>
void Rod2D<T>::GetContactPointsTangentVelocities(
const systems::Context<T>& context, const std::vector<Vector2<T>>& points,
std::vector<T>* vels) const {
DRAKE_DEMAND(vels != nullptr);
const Vector3<T> q = GetRodConfig(context);
const Vector3<T> v = GetRodVelocity(context);
// Get necessary quantities.
const Vector2<T> p_WRo = q.segment(0, 2);
const Vector2<T> v_WRo = v.segment(0, 2);
const T& w_WR = v[2];
vels->resize(points.size());
for (size_t i = 0; i < points.size(); ++i) {
(*vels)[i] =
CalcCoincidentRodPointVelocity(p_WRo, v_WRo, w_WR, points[i])[0];
}
}
// Gets the row of a contact Jacobian matrix, given a point of contact, @p p,
// and projection direction (unit vector), @p dir. Aborts if @p dir is not a
// unit vector.
template <class T>
Vector3<T> Rod2D<T>::GetJacobianRow(const systems::Context<T>& context,
const Vector2<T>& p,
const Vector2<T>& dir) const {
using std::abs;
const double eps = 10 * std::numeric_limits<double>::epsilon();
DRAKE_DEMAND(abs(dir.norm() - 1) < eps);
// Get rod configuration variables.
const Vector3<T> q = GetRodConfig(context);
// Compute cross product of the moment arm (expressed in the world frame)
// and the direction.
const Vector2<T> r(p[0] - q[0], p[1] - q[1]);
return Vector3<T>(dir[0], dir[1], cross2(r, dir));
}
// Gets the time derivative of a row of a contact Jacobian matrix, given a
// point of contact, @p p, and projection direction (unit vector), @p dir.
// Aborts if @p dir is not a unit vector.
template <class T>
Vector3<T> Rod2D<T>::GetJacobianDotRow(const systems::Context<T>& context,
const Vector2<T>& p,
const Vector2<T>& dir) const {
using std::abs;
const double eps = 10 * std::numeric_limits<double>::epsilon();
DRAKE_DEMAND(abs(dir.norm() - 1) < eps);
// Get rod state variables.
const Vector3<T> q = GetRodConfig(context);
const Vector3<T> v = GetRodVelocity(context);
// Get the transformation of vectors from the rod frame to the
// world frame and its time derivative.
const T& theta = q[2];
Eigen::Rotation2D<T> R(theta);
// Get the vector from the rod center-of-mass to the contact point,
// expressed in the rod frame.
const Vector2<T> x = q.segment(0, 2);
const Vector2<T> u = R.inverse() * (p - x);
// Compute the translational velocity of the contact point.
const Vector2<T> xdot = v.segment(0, 2);
const T& thetadot = v[2];
const Matrix2<T> Rdot = GetRotationMatrixDerivative(theta, thetadot);
const Vector2<T> pdot = xdot + Rdot * u;
// Compute cross product of the time derivative of the moment arm (expressed
// in the world frame) and the direction.
const Vector2<T> rdot(pdot[0] - v[0], pdot[1] - v[1]);
return Vector3<T>(0, 0, cross2(rdot, dir));
}
template <class T>
Matrix2<T> Rod2D<T>::GetSlidingContactFrameToWorldTransform(
const T& xaxis_velocity) const {
// Note: the contact normal for the rod with the horizontal plane always
// points along the world +y axis; the sliding tangent vector points along
// either the +/-x (world) axis. The << operator populates the matrix row by
// row, so
// R_WC = | 0 ±1 |
// | 1 0 |
// indicating that the contact normal direction (the +x axis in the contact
// frame) is +y in the world frame and the contact tangent direction (more
// precisely, the direction of sliding, the +y axis in the contact frame) is
// ±x in the world frame.
DRAKE_DEMAND(xaxis_velocity != 0);
Matrix2<T> R_WC;
// R_WC's elements match how they appear lexically: one row per line.
// clang-format off
R_WC << 0, ((xaxis_velocity > 0) ? 1 : -1),
1, 0;
// clang-format on
return R_WC;
}
template <class T>
Matrix2<T> Rod2D<T>::GetNonSlidingContactFrameToWorldTransform() const {
// Note: the contact normal for the rod with the horizontal plane always
// points along the world +y axis; the non-sliding tangent vector always
// points along the world +x axis. The << operator populates the matrix row by
// row, so
// R_WC = | 0 1 |
// | 1 0 |
// indicating that the contact normal direction is (the +x axis in the contact
// frame) is +y in the world frame and the contact tangent direction (the +y
// axis in the contact frame) is +x in the world frame.
Matrix2<T> R_WC;
// R_WC's elements match how they appear lexically: one row per line.
// clang-format off
R_WC << 0, 1,
1, 0;
// clang-format on
return R_WC;
}
template <class T>
void Rod2D<T>::CalcConstraintProblemData(
const systems::Context<T>& context, const std::vector<Vector2<T>>& points,
const std::vector<T>& tangent_vels,
ConstraintAccelProblemData<T>* data) const {
using std::abs;
DRAKE_DEMAND(data != nullptr);
DRAKE_DEMAND(points.size() == tangent_vels.size());
// Set the inertia solver.
data->solve_inertia = [this](const MatrixX<T>& m) {
return solve_inertia(m);
};
// The normal and tangent spanning direction are unique.
const Matrix2<T> R_wc = GetNonSlidingContactFrameToWorldTransform();
const Vector2<T> contact_normal = R_wc.col(0);
const Vector2<T> contact_tangent = R_wc.col(1);
// Verify contact normal and tangent directions are as we expect.
DRAKE_ASSERT(abs(contact_normal.dot(Vector2<T>(0, 1)) - 1) <
std::numeric_limits<double>::epsilon());
DRAKE_ASSERT(abs(contact_tangent.dot(Vector2<T>(1, 0)) - 1) <
std::numeric_limits<double>::epsilon());
// Get the set of contact points.
const int nc = points.size();
// Get the set of tangent velocities.
const T sliding_vel_tol = GetSlidingVelocityTolerance();
// Set sliding and non-sliding contacts.
std::vector<int>& non_sliding_contacts = data->non_sliding_contacts;
std::vector<int>& sliding_contacts = data->sliding_contacts;
non_sliding_contacts.clear();
sliding_contacts.clear();
for (int i = 0; i < nc; ++i) {
if (abs(tangent_vels[i]) < sliding_vel_tol) {
non_sliding_contacts.push_back(i);
} else {
sliding_contacts.push_back(i);
}
}
// Designate sliding and non-sliding contacts.
const int num_sliding = sliding_contacts.size();
const int num_non_sliding = non_sliding_contacts.size();
// Set sliding and non-sliding friction coefficients.
data->mu_sliding.setOnes(num_sliding) *= get_mu_coulomb();
data->mu_non_sliding.setOnes(num_non_sliding) *= get_mu_static();
// Set spanning friction cone directions (set to unity, because rod is 2D).
data->r.resize(num_non_sliding);
for (int i = 0; i < num_non_sliding; ++i) data->r[i] = 1;
// Form the normal contact Jacobian (N).
const int ngc = 3; // Number of generalized coordinates / velocities.
MatrixX<T> N(nc, ngc);
for (int i = 0; i < nc; ++i)
N.row(i) = GetJacobianRow(context, points[i], contact_normal);
data->N_mult = [N](const VectorX<T>& w) -> VectorX<T> {
return N * w;
};
// Form Ndot (time derivative of N) and compute Ndot * v.
MatrixX<T> Ndot(nc, ngc);
for (int i = 0; i < nc; ++i)
Ndot.row(i) = GetJacobianDotRow(context, points[i], contact_normal);
const Vector3<T> v = GetRodVelocity(context);
data->kN = Ndot * v;
// Form the tangent directions contact Jacobian (F), its time derivative
// (Fdot), and compute Fdot * v.
const int nr = std::accumulate(data->r.begin(), data->r.end(), 0);
MatrixX<T> F = MatrixX<T>::Zero(nr, ngc);
MatrixX<T> Fdot = MatrixX<T>::Zero(nr, ngc);
for (int i = 0; i < static_cast<int>(non_sliding_contacts.size()); ++i) {
const int contact_index = non_sliding_contacts[i];
F.row(i) = GetJacobianRow(context, points[contact_index], contact_tangent);
Fdot.row(i) =
GetJacobianDotRow(context, points[contact_index], contact_tangent);
}
data->kF = Fdot * v;
data->F_mult = [F](const VectorX<T>& w) -> VectorX<T> {
return F * w;
};
data->F_transpose_mult = [F](const VectorX<T>& w) -> VectorX<T> {
return F.transpose() * w;
};
// Form N - mu*Q (Q is sliding contact direction Jacobian).
MatrixX<T> N_minus_mu_Q = N;
Vector3<T> Qrow;
for (int i = 0; i < static_cast<int>(sliding_contacts.size()); ++i) {
const int contact_index = sliding_contacts[i];
Matrix2<T> sliding_contract_frame =
GetSlidingContactFrameToWorldTransform(tangent_vels[contact_index]);
const auto& sliding_dir = sliding_contract_frame.col(1);
Qrow = GetJacobianRow(context, points[contact_index], sliding_dir);
N_minus_mu_Q.row(contact_index) -= data->mu_sliding[i] * Qrow;
}
data->N_minus_muQ_transpose_mult =
[N_minus_mu_Q](const VectorX<T>& w) -> VectorX<T> {
return N_minus_mu_Q.transpose() * w;
};
data->kL.resize(0);
// Set external force vector.
data->tau = ComputeExternalForces(context);
}
template <class T>
void Rod2D<T>::CalcImpactProblemData(const systems::Context<T>& context,
const std::vector<Vector2<T>>& points,
ConstraintVelProblemData<T>* data) const {
using std::abs;
DRAKE_DEMAND(data != nullptr);
// Setup the generalized inertia matrix.
Matrix3<T> M;
// clang-format off
M << mass_, 0, 0,
0, mass_, 0,
0, 0, J_;
// clang-format on
// Get the generalized velocity.
data->Mv =
M *
context.get_continuous_state().get_generalized_velocity().CopyToVector();
// Set the inertia solver.
data->solve_inertia = [this](const MatrixX<T>& m) {
return solve_inertia(m);
};
// The normal and tangent spanning direction are unique for the rod undergoing
// impact (i.e., unlike with non-impacting rigid contact equations, the
// frame does not change depending on sliding velocity direction).
const Matrix2<T> non_sliding_contact_frame =
GetNonSlidingContactFrameToWorldTransform();
const Vector2<T> contact_normal = non_sliding_contact_frame.col(0);
const Vector2<T> contact_tan = non_sliding_contact_frame.col(1);
// Get the set of contact points.
const int num_contacts = points.size();
// Set sliding and non-sliding friction coefficients.
data->mu.setOnes(num_contacts) *= get_mu_coulomb();
// Set spanning friction cone directions (set to unity, because rod is 2D).
data->r.resize(num_contacts);
for (int i = 0; i < num_contacts; ++i) data->r[i] = 1;
// Form the normal contact Jacobian (N).
const int num_generalized_coordinates = 3;
MatrixX<T> N(num_contacts, num_generalized_coordinates);
for (int i = 0; i < num_contacts; ++i)
N.row(i) = GetJacobianRow(context, points[i], contact_normal);
data->N_mult = [N](const VectorX<T>& w) -> VectorX<T> {
return N * w;
};
data->N_transpose_mult = [N](const VectorX<T>& w) -> VectorX<T> {
return N.transpose() * w;
};
data->kN.setZero(num_contacts);
data->gammaN.setZero(num_contacts);
// Form the tangent directions contact Jacobian (F).
const int nr = std::accumulate(data->r.begin(), data->r.end(), 0);
MatrixX<T> F(nr, num_generalized_coordinates);
for (int i = 0; i < num_contacts; ++i) {
F.row(i) = GetJacobianRow(context, points[i], contact_tan);
}
data->F_mult = [F](const VectorX<T>& w) -> VectorX<T> {
return F * w;
};
data->F_transpose_mult = [F](const VectorX<T>& w) -> VectorX<T> {
return F.transpose() * w;
};
data->kF.setZero(nr);
data->gammaF.setZero(nr);
data->gammaE.setZero(num_contacts);
data->kL.resize(0);
data->gammaL.resize(0);
}
/// Integrates the Rod 2D example forward in time using a
/// half-explicit discretization scheme.
template <class T>
void Rod2D<T>::CalcDiscreteUpdate(
const systems::Context<T>& context,
systems::DiscreteValues<T>* discrete_state) const {
using std::cos;
using std::sin;
// Determine ERP and CFM.
const double erp = get_erp();
const double cfm = get_cfm();
// Get the necessary state variables.
const systems::BasicVector<T>& state = context.get_discrete_state(0);
const auto& q = state.value().template segment<3>(0);
Vector3<T> v = state.value().template segment<3>(3);
const T& x = q(0);
const T& y = q(1);
const T& theta = q(2);
// Construct the problem data.
const int ngc = 3; // Number of generalized coords / velocities.
ConstraintVelProblemData<T> problem_data(ngc);
// Two contact points, corresponding to the two rod endpoints, are always
// used, regardless of whether any part of the rod is in contact with the
// halfspace. This practice is standard in discretization approaches with
// constraint stabilization. See:
// M. Anitescu and G. Hart. A Constraint-Stabilized Time-Stepping Approach
// for Rigid Multibody Dynamics with Joints, Contact, and Friction. Intl.
// J. for Numerical Methods in Engr., 60(14), 2004.
const int nc = 2;
// Find left and right end point locations.
const T stheta = sin(theta), ctheta = cos(theta);
const Vector2<T> left =
CalcRodEndpoint(x, y, -1, ctheta, stheta, half_length_);
const Vector2<T> right =
CalcRodEndpoint(x, y, 1, ctheta, stheta, half_length_);
// Set up the contact normal and tangent (friction) direction Jacobian
// matrices. These take the form:
// | 0 1 n1 | | 1 0 f1 |
// N = | 0 1 n2 | F = | 1 0 f2 |
// where n1, n2/f1, f2 are the moment arm induced by applying the
// force at the given contact point along the normal/tangent direction.
Eigen::Matrix<T, nc, ngc> N, F;
N(0, 0) = N(1, 0) = 0;
N(0, 1) = N(1, 1) = 1;
N(0, 2) = (left[0] - x);
N(1, 2) = (right[0] - x);
F(0, 0) = F(1, 0) = 1;
F(0, 1) = F(1, 1) = 0;
F(0, 2) = -(left[1] - y);
F(1, 2) = -(right[1] - y);
// Set the number of tangent directions.
problem_data.r = {1, 1};
// Get the total number of tangent directions.
const int nr =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
// Set the coefficients of friction.
problem_data.mu.setOnes(nc) *= get_mu_coulomb();
// Set up the operators.
problem_data.N_mult = [&N](const VectorX<T>& vv) -> VectorX<T> {
return N * vv;
};
problem_data.N_transpose_mult = [&N](const VectorX<T>& vv) -> VectorX<T> {
return N.transpose() * vv;
};
problem_data.F_transpose_mult = [&F](const VectorX<T>& vv) -> VectorX<T> {
return F.transpose() * vv;
};
problem_data.F_mult = [&F](const VectorX<T>& vv) -> VectorX<T> {
return F * vv;
};
problem_data.solve_inertia = [this](const MatrixX<T>& mm) -> MatrixX<T> {
return GetInverseInertiaMatrix() * mm;
};
// Update the generalized velocity vector with discretized external forces
// (expressed in the world frame).
const Vector3<T> fext = ComputeExternalForces(context);
v += dt_ * problem_data.solve_inertia(fext);
problem_data.Mv = GetInertiaMatrix() * v;
// Set stabilization parameters.
problem_data.kN.resize(nc);
problem_data.kN[0] = erp * left[1] / dt_;
problem_data.kN[1] = erp * right[1] / dt_;
problem_data.kF.setZero(nr);
// Set regularization parameters.
problem_data.gammaN.setOnes(nc) *= cfm;
problem_data.gammaF.setOnes(nr) *= cfm;
problem_data.gammaE.setOnes(nc) *= cfm;
// Solve the constraint problem.
VectorX<T> cf;
solver_.SolveImpactProblem(problem_data, &cf);
// Compute the updated velocity.
VectorX<T> delta_v;
solver_.ComputeGeneralizedVelocityChange(problem_data, cf, &delta_v);
// Compute the new velocity. Note that external forces have already been
// incorporated into v.
VectorX<T> vplus = v + delta_v;
// Compute the new position using an "explicit" update.
VectorX<T> qplus = q + vplus * dt_;
// Set the new discrete state.
Eigen::VectorBlock<VectorX<T>> new_state =
discrete_state->get_mutable_value(0);
new_state.segment(0, 3) = qplus;
new_state.segment(3, 3) = vplus;
}
// Computes the impulses such that the vertical velocity at the contact point
// is zero and the frictional impulse lies exactly on the friction cone.
// These equations were determined by issuing the
// following commands in Mathematica:
//
// cx[t_] := x[t] + k*Cos[theta[t]]*(r/2)
// cy[t_] := y[t] + k*Sin[theta[t]]*(r/2)
// Solve[{mass*delta_xdot == fF,
// mass*delta_ydot == fN,
// J*delta_thetadot == (cx[t] - x)*fN - (cy - y)*fF,
// 0 == (D[y[t], t] + delta_ydot) +
// k*(r/2) *Cos[theta[t]]*(D[theta[t], t] + delta_thetadot),
// fF == mu*fN *-sgn_cxdot},
// {delta_xdot, delta_ydot, delta_thetadot, fN, fF}]
// where theta is the counter-clockwise angle the rod makes with the x-axis,
// fN and fF are contact normal and frictional forces; delta_xdot,
// delta_ydot, and delta_thetadot represent the changes in velocity,
// r is the length of the rod, sgn_xdot is the sign of the tangent
// velocity (pre-impact), and (hopefully) all other variables are
// self-explanatory.
//
// The first two equations above are the formula
// for the location of the point of contact. The next two equations
// describe the relationship between the horizontal/vertical change in
// momenta at the center of mass of the rod and the frictional/normal
// contact impulses. The fifth equation yields the moment from
// the contact impulses. The sixth equation specifies that the post-impact
// velocity in the vertical direction be zero. The last equation corresponds
// to the relationship between normal and frictional impulses (dictated by the
// Coulomb friction model).
// @returns a Vector2, with the first element corresponding to the impulse in
// the normal direction (positive y-axis) and the second element
// corresponding to the impulse in the tangential direction (positive
// x-axis).
// TODO(@edrumwri) This return vector is backwards -- should be x,y not y,x!
template <class T>
Vector2<T> Rod2D<T>::CalcFConeImpactImpulse(
const systems::Context<T>& context) const {
using std::abs;
// Get the necessary parts of the state.
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
const T& x = state.GetAtIndex(0);
const T& y = state.GetAtIndex(1);
const T& theta = state.GetAtIndex(2);
const T& xdot = state.GetAtIndex(3);
const T& ydot = state.GetAtIndex(4);
const T& thetadot = state.GetAtIndex(5);
// The two points of the rod are located at (x,y) + R(theta)*[0,r/2] and
// (x,y) + R(theta)*[0,-r/2], where
// R(theta) = | cos(theta) -sin(theta) |
// | sin(theta) cos(theta) |
// and r is designated as the rod length. Thus, the heights of
// the rod endpoints are y + sin(theta)*r/2 and y - sin(theta)*r/2,
// or, y + k*sin(theta)*r/2, where k = +/-1.
const T ctheta = cos(theta), stheta = sin(theta);
const int k = (stheta > 0) ? -1 : 1;
const T cy = y + k * stheta * half_length_;
const double mu = mu_;
const double J = J_;
const double mass = mass_;
const double r = 2 * half_length_;
// Compute the impulses.
const T cxdot = xdot - k * stheta * half_length_ * thetadot;
const int sgn_cxdot = (cxdot > 0) ? 1 : -1;
const T fN = (J * mass * (-(r * k * ctheta * thetadot) / 2 - ydot)) /
(J + (r * k * mass * mu * (-y + cy) * ctheta * sgn_cxdot) / 2 -
(r * k * mass * ctheta * (x - (r * k * ctheta) / 2 - x)) / 2);
const T fF = -sgn_cxdot * mu * fN;
// Verify normal force is non-negative.
DRAKE_DEMAND(fN >= 0);
return Vector2<T>(fN, fF);
}
// Computes the impulses such that the velocity at the contact point is zero.
// These equations were determined by issuing the following commands in
// Mathematica:
//
// cx[t_] := x[t] + k*Cos[theta[t]]*(r/2)
// cy[t_] := y[t] + k*Sin[theta[t]]*(r/2)
// Solve[{mass*delta_xdot == fF, mass*delta_ydot == fN,
// J*delta_thetadot == (cx[t] - x)*fN - (cy - y)*fF,
// 0 == (D[y[t], t] + delta_ydot) +
// k*(r/2) *Cos[theta[t]]*(D[theta[t], t] + delta_thetadot),
// 0 == (D[x[t], t] + delta_xdot) +
// k*(r/2)*-Cos[theta[t]]*(D[theta[t], t] + delta_thetadot)},
// {delta_xdot, delta_ydot, delta_thetadot, fN, fF}]
// which computes the change in velocity and frictional (fF) and normal (fN)
// impulses necessary to bring the system to rest at the point of contact,
// 'r' is the rod length, theta is the counter-clockwise angle measured
// with respect to the x-axis; delta_xdot, delta_ydot, and delta_thetadot
// are the changes in velocity.
//
// The first two equations above are the formula
// for the location of the point of contact. The next two equations
// describe the relationship between the horizontal/vertical change in
// momenta at the center of mass of the rod and the frictional/normal
// contact impulses. The fifth equation yields the moment from
// the contact impulses. The sixth and seventh equations specify that the
// post-impact velocity in the horizontal and vertical directions at the
// point of contact be zero.
// @returns a Vector2, with the first element corresponding to the impulse in
// the normal direction (positive y-axis) and the second element
// corresponding to the impulse in the tangential direction (positive
// x-axis).
// TODO(@edrumwri) This return vector is backwards -- should be x,y not y,x!
template <class T>
Vector2<T> Rod2D<T>::CalcStickingImpactImpulse(
const systems::Context<T>& context) const {
// Get the necessary parts of the state.
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
const T& x = state.GetAtIndex(0);
const T& y = state.GetAtIndex(1);
const T& theta = state.GetAtIndex(2);
const T& xdot = state.GetAtIndex(3);
const T& ydot = state.GetAtIndex(4);
const T& thetadot = state.GetAtIndex(5);
// The two points of the rod are located at (x,y) + R(theta)*[0,r/2] and
// (x,y) + R(theta)*[0,-r/2], where
// R(theta) = | cos(theta) -sin(theta) |
// | sin(theta) cos(theta) |
// and r is designated as the rod length. Thus, the heights of
// the rod endpoints are y + sin(theta)*l/2 and y - sin(theta)*l/2,
// or, y + k*sin(theta)*l/2, where k = +/-1.
const T ctheta = cos(theta), stheta = sin(theta);
const int k = (stheta > 0) ? -1 : 1;
const T cy = y + k * stheta * half_length_;
// Compute the impulses.
const double r = 2 * half_length_;
const double mass = mass_;
const double J = J_;
const T fN =
(2 * (-(r * J * k * mass * ctheta * thetadot) +
r * k * mass * mass * y * ctheta * xdot -
r * k * mass * mass * cy * ctheta * xdot - 2 * J * mass * ydot +
r * k * mass * mass * y * ctheta * ydot -
r * k * mass * mass * cy * ctheta * ydot)) /
(4 * J - 2 * r * k * mass * x * ctheta - 2 * r * k * mass * y * ctheta +
2 * r * k * mass * cy * ctheta + r * r * k * k * mass * ctheta * ctheta +
2 * r * k * mass * ctheta * x);
const T fF = -(
(mass * (-2 * r * J * k * ctheta * thetadot + 4 * J * xdot -
2 * r * k * mass * x * ctheta * xdot +
r * r * k * k * mass * ctheta * ctheta * xdot +
2 * r * k * mass * ctheta * x * xdot -
2 * r * k * mass * x * ctheta * ydot +
r * r * k * k * mass * ctheta * ctheta * ydot +
2 * r * k * mass * ctheta * x * ydot)) /
(4 * J - 2 * r * k * mass * x * ctheta - 2 * r * k * mass * y * ctheta +
2 * r * k * mass * cy * ctheta + r * r * k * k * mass * ctheta * ctheta +
2 * r * k * mass * ctheta * x));
// Verify that normal impulse is non-negative.
DRAKE_DEMAND(fN > 0.0);
return Vector2<T>(fN, fF);
}
// Returns the generalized inertia matrix computed about the
// center of mass of the rod and expressed in the world frame.
template <class T>
Matrix3<T> Rod2D<T>::GetInertiaMatrix() const {
Matrix3<T> M;
// clang-format off
M << mass_, 0, 0,
0, mass_, 0,
0, 0, J_;
// clang-format on
return M;
}
// Returns the inverse of the generalized inertia matrix computed about the
// center of mass of the rod and expressed in the world frame.
template <class T>
Matrix3<T> Rod2D<T>::GetInverseInertiaMatrix() const {
Matrix3<T> M_inv;
// clang-format off
M_inv << 1.0 / mass_, 0, 0,
0, 1.0 / mass_, 0,
0, 0, 1.0 / J_;
// clang-format on
return M_inv;
}
// This is a smooth approximation to a step function. Input x goes from 0 to 1;
// output goes 0 to 1 but smoothed with an S-shaped quintic with first and
// second derivatives zero at both ends.
template <class T>
T Rod2D<T>::step5(const T& x) {
DRAKE_ASSERT(0 <= x && x <= 1);
const T x3 = x * x * x;
return x3 * (10 + x * (6 * x - 15)); // 10x^3-15x^4+6x^5
}
// This function models Stribeck dry friction as a C² continuous quintic spline
// with 3 segments that is used to calculate an effective coefficient of
// friction mu_stribeck. That is the composite coefficient of friction that we
// use to scale the normal force to produce the friction force.
//
// We are given the friction coefficients mu_s (static) and mu_d (dynamic), and
// a dimensionless slip speed s>=0, then calculate:
// mu_stribeck =
// (a) s=0..1: smooth interpolation from 0 to mu_s
// (b) s=1..3: smooth interpolation from mu_s down to mu_d (Stribeck)
// (c) s=3..Inf mu_d
//
// s must be non-dimensionalized by taking the actual slip speed and dividing by
// the stiction slip velocity tolerance.
//
// mu_stribeck is zero at s=0 with 1st deriv (slope) zero and 2nd deriv
// (curvature) 0. At large speeds s>>0 the value is mu_d, again with zero slope
// and curvature. That makes mu_stribeck(s) C² continuous for all s>=0.
//
// The resulting curve looks like this:
//
// mu_s ***
// * *
// * *
// * *
// mu_d * * * * * * slope, curvature = 0 at Inf
// *
// *
// *
// 0 * * slope, curvature = 0 at 0
//
// | | |
// s=0 1 3
//
template <class T>
T Rod2D<T>::CalcMuStribeck(const T& mu_s, const T& mu_d, const T& s) {
DRAKE_ASSERT(mu_s >= 0 && mu_d >= 0 && s >= 0);
T mu_stribeck;
if (s >= 3)
mu_stribeck = mu_d; // sliding
else if (s >= 1)
mu_stribeck = mu_s - (mu_s - mu_d) * step5((s - 1) / 2); // Stribeck
else
mu_stribeck = mu_s * step5(s); // 0 <= s < 1 (stiction)
return mu_stribeck;
}
// Finds the locations of the two rod endpoints and generates contact forces at
// either or both end points (depending on contact condition) using a linear
// stiffness model, Hunt and Crossley normal dissipation, and a Stribeck
// friction model. No forces are applied anywhere else along the rod. The force
// is returned as the spatial force F_Ro_W (described in Rod2D class comments),
// represented as (fx,fy,τ).
//
// Note that we are attributing all of the compliance to the *halfspace*, not
// the *rod*, so that forces will be applied to the same points of the rod as is
// done in the rigid contact models. That makes comparison of the models easier.
//
// For each end point, let h be the penetration depth (in the -y direction) and
// v the slip speed along x. Then
// fK = k*h normal force due to stiffness
// fD = fK*d*hdot normal force due to dissipation
// fN = max(fK + fD, 0) total normal force (>= 0)
// fF = -mu(|v|)*fN*sign(v) friction force
// f = fN + fF total force
//
// Here mu(v) is a Stribeck function that is zero at zero slip speed, and
// reaches a maximum mu_s at the stiction speed tolerance. mu_s is the
// static coefficient of friction.
//
// Note: we return the spatial force in a Vector3 but it is not a vector
// quantity.
template <class T>
Vector3<T> Rod2D<T>::CalcCompliantContactForces(
const systems::Context<T>& context) const {
// Depends on continuous state being available.
DRAKE_DEMAND(system_type_ == SystemType::kContinuous);
using std::abs;
using std::max;
// Get the necessary parts of the state.
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
const Vector2<T> p_WRo(state.GetAtIndex(0), state.GetAtIndex(1));
const T theta = state.GetAtIndex(2);
const Vector2<T> v_WRo(state.GetAtIndex(3), state.GetAtIndex(4));
const T w_WR(state.GetAtIndex(5));
const T ctheta = cos(theta), stheta = sin(theta);
// Find left and right end point locations.
Vector2<T> p_WP[2] = {
CalcRodEndpoint(p_WRo[0], p_WRo[1], -1, ctheta, stheta, half_length_),
CalcRodEndpoint(p_WRo[0], p_WRo[1], 1, ctheta, stheta, half_length_)};
// Calculate the net spatial force to apply at rod origin from the individual
// contact forces at the endpoints.
Vector3<T> F_Ro_W(0, 0, 0); // Accumulate contact forces here.
for (int i = 0; i < 2; ++i) {
const Vector2<T>& p_WC = p_WP[i]; // Get contact point C location in World.
// Calculate penetration depth h along -y; negative means separated.
const T h = -p_WC[1];
if (h > 0) { // This point is in contact.
const Vector2<T> v_WRc = // Velocity of rod point coincident with C.
CalcCoincidentRodPointVelocity(p_WRo, v_WRo, w_WR, p_WC);
const T hdot = -v_WRc[1]; // Penetration rate in -y.
const T v = v_WRc[0]; // Slip velocity in +x.
const int sign_v = v < 0 ? -1 : 1;
const T fK = get_stiffness() * h; // See method comment above.
const T fD = fK * get_dissipation() * hdot;
const T fN = max(fK + fD, T(0));
const T mu = CalcMuStribeck(get_mu_static(), get_mu_coulomb(),
abs(v) / get_stiction_speed_tolerance());
const T fF = -mu * fN * T(sign_v);
// Find the point Rc of the rod that is coincident with the contact point
// C, measured from Ro but expressed in W.
const Vector2<T> p_RRc_W = p_WC - p_WRo;
const Vector2<T> f_Rc(fF, fN); // The force to apply at Rc.
const T t_R = cross2(p_RRc_W, f_Rc); // τ=r X f.
F_Ro_W += Vector3<T>(fF, fN, t_R);
}
}
return F_Ro_W; // A 2-vector & scalar, not really a 3-vector.
}
template <class T>
bool Rod2D<T>::IsImpacting(const systems::Context<T>& context) const {
using std::cos;
using std::sin;
// Note: we do not consider modes here.
// TODO(edrumwri): Handle two-point contacts.
// Get state data necessary to compute the point of contact.
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
const T& y = state.GetAtIndex(1);
const T& theta = state.GetAtIndex(2);
const T& ydot = state.GetAtIndex(4);
const T& thetadot = state.GetAtIndex(5);
// Get the height of the lower rod endpoint.
const T ctheta = cos(theta), stheta = sin(theta);
const int k = (stheta > 0) ? -1 : (stheta < 0) ? 1 : 0;
const T cy = y + k * stheta * half_length_;
// If rod endpoint is not touching, there is no impact.
if (cy >= 10 * std::numeric_limits<double>::epsilon()) return false;
// Compute the velocity at the point of contact.
const T cydot = ydot + k * ctheta * half_length_ * thetadot;
// Verify that the rod is not impacting.
return (cydot < -10 * std::numeric_limits<double>::epsilon());
}
// Computes the accelerations of the rod center of mass for the rod, both
// in compliant contact and contact-free.
template <typename T>
void Rod2D<T>::CalcAccelerationsCompliantContactAndBallistic(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const {
// Obtain the structure we need to write into (ds=d/dt state).
systems::VectorBase<T>& ds = derivatives->get_mutable_vector();
// Get external applied force (a spatial force at Ro, in W).
const auto Fext_Ro_W = ComputeExternalForces(context);
// Calculate contact forces (also spatial force at Ro, in W).
const Vector3<T> Fc_Ro_W = CalcCompliantContactForces(context);
const Vector3<T> F_Ro_W = Fc_Ro_W + Fext_Ro_W; // Total force.
// Second three derivative components are acceleration due to gravity,
// contact forces, and non-gravitational, non-contact external forces.
ds.SetAtIndex(3, F_Ro_W[0] / mass_);
ds.SetAtIndex(4, F_Ro_W[1] / mass_);
ds.SetAtIndex(5, F_Ro_W[2] / J_);
}
template <typename T>
void Rod2D<T>::DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const {
using std::abs;
using std::cos;
using std::sin;
// Don't compute any derivatives if this is the discretized system.
if (system_type_ == SystemType::kDiscretized) {
DRAKE_ASSERT(derivatives->size() == 0);
return;
}
// Get the necessary parts of the state.
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
const T& xdot = state.GetAtIndex(3);
const T& ydot = state.GetAtIndex(4);
const T& thetadot = state.GetAtIndex(5);
// Obtain the structure we need to write into.
systems::VectorBase<T>& f = derivatives->get_mutable_vector();
// First three derivative components are xdot, ydot, thetadot.
f.SetAtIndex(0, xdot);
f.SetAtIndex(1, ydot);
f.SetAtIndex(2, thetadot);
// Compute the velocity derivatives (accelerations).
if (system_type_ == SystemType::kContinuous) {
return CalcAccelerationsCompliantContactAndBallistic(context, derivatives);
} else {
// TODO(edrumwri): Implement the piecewise DAE approach.
throw std::domain_error(
"Rod2D<T>::DoCalcTimeDerivatives: piecewise DAE isn't implemented yet");
}
}
/// Sets the rod to a 45 degree angle with the halfspace and positions the rod
/// such that it and the halfspace are touching at exactly one point of contact.
template <typename T>
void Rod2D<T>::SetDefaultState(const systems::Context<T>&,
systems::State<T>* state) const {
using std::sin;
using std::sqrt;
// Initial state corresponds to an inconsistent configuration for piecewise
// DAE.
const double half_len = get_rod_half_length();
VectorX<T> x0(6);
const double r22 = sqrt(2) / 2;
x0 << half_len * r22, half_len * r22, M_PI / 4.0, -1, 0, 0; // Initial state.
if (system_type_ == SystemType::kDiscretized) {
state->get_mutable_discrete_state().get_mutable_vector(0).SetFromVector(x0);
} else {
// Continuous variables.
state->get_mutable_continuous_state().SetFromVector(x0);
// Set abstract variables for piecewise DAE approach.
if (system_type_ == SystemType::kPiecewiseDAE) {
// TODO(edrumwri): Indicate that the rod is in the single contact
// sliding mode.
// TODO(edrumwri): Determine and set the point of contact.
}
}
}
template class Rod2D<double>;
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/rod2d_state_vector.cc | #include "drake/examples/rod2d/rod2d_state_vector.h"
namespace drake {
namespace examples {
namespace rod2d {
const int Rod2dStateVectorIndices::kNumCoordinates;
const int Rod2dStateVectorIndices::kX;
const int Rod2dStateVectorIndices::kY;
const int Rod2dStateVectorIndices::kTheta;
const int Rod2dStateVectorIndices::kXdot;
const int Rod2dStateVectorIndices::kYdot;
const int Rod2dStateVectorIndices::kThetadot;
const std::vector<std::string>& Rod2dStateVectorIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"x",
"y",
"theta",
"xdot",
"ydot",
"thetadot",
});
return coordinates.access();
}
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/constraint_problem_data.h | #pragma once
#include <vector>
#include <Eigen/Core>
#include "drake/common/eigen_types.h"
namespace drake {
namespace examples {
namespace rod2d {
/// Structure for holding constraint data for computing forces due to
/// constraints and the resulting multibody accelerations.
///
/// The Newton-Euler equations (essentially F = ma) coupled with constraints
/// on the positional coordinates g(q) yields an Index-3 DAE
/// (see [Hairer 1996]), and generally makes initial value problems hard to
/// solve, computationally speaking; coupling the Newton-Euler equations with
/// the second time derivative of such constraint equations (i.e., g̈(q,v,v̇))
/// yields a far more manageable Index-1 DAE, again with regard to computation.
/// This structure stores problem data for computing dynamics under such
/// constraints and others (nonholonomic constraints, Coulomb friction
/// constraints, etc.)
///
/// <h3>Definition of variables specific to this class</h3>
/// (See "Variable definitions" in the local README) for the more general set of
/// definitions).
///
/// - ns ∈ ℕ The number of contacts at which sliding is occurring. Note
/// that nc = ns + nns, where nc is the number of points of contact.
/// - nns ∈ ℕ The number of contacts at which sliding is not occurring. Note
/// that nc = ns + nns.
template <class T>
struct ConstraintAccelProblemData {
/// Constructs acceleration problem data for a system with a @p gv_dim
/// dimensional generalized velocity.
explicit ConstraintAccelProblemData(int gv_dim) {
// Set default for non-transpose operators- returns an empty vector.
auto zero_fn = [](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>(0);
};
N_mult = zero_fn;
F_mult = zero_fn;
L_mult = zero_fn;
G_mult = zero_fn;
// Set default for transpose operators - returns the appropriately sized
// zero vector.
auto zero_gv_dim_fn = [gv_dim](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>::Zero(gv_dim);
};
N_minus_muQ_transpose_mult = zero_gv_dim_fn;
F_transpose_mult = zero_gv_dim_fn;
L_transpose_mult = zero_gv_dim_fn;
G_transpose_mult = zero_gv_dim_fn;
}
/// Flag for whether the complementarity problem solver should be used to
/// solve this particular problem instance. If every constraint in the problem
/// data is active, using the linear system solver
/// (`use_complementarity_problem_solver=false`) will yield a solution much
/// more quickly. If it is unknown whether every constraint is active, the
/// complementarity problem solver should be used; otherwise, the inequality
/// constraints embedded in the problem data may not be satisfied. The safe
/// (and slower) value of `true` is the default.
bool use_complementarity_problem_solver{true};
/// The indices of the sliding contacts (those contacts at which there is
/// non-zero relative velocity between bodies in the plane tangent to the
/// point of contact), out of the set of all contact indices (0...nc-1).
/// This vector must be in sorted order.
std::vector<int> sliding_contacts;
/// The indices of the non-sliding contacts (those contacts at which there
/// is zero relative velocity between bodies in the plane tangent to the
/// point of contact), out of the set of all contact indices (0...nc-1).
/// This vector must be in sorted order.
std::vector<int> non_sliding_contacts;
/// The number of spanning vectors in the contact tangents (used to linearize
/// the friction cone) at the n *non-sliding* contact points. For contact
/// problems in two dimensions, each element of r will be one. For contact
/// problems in three dimensions, a friction pyramid (for example), for a
/// contact point i will have rᵢ = 2. [Anitescu 1997] define k such vectors
/// and require that, for each vector w in the spanning set, -w also exists
/// in the spanning set. The RigidContactAccelProblemData structure expects
/// that the contact solving mechanism negates the spanning vectors so `r` =
/// k/2 spanning vectors will correspond to a k-edge polygon friction cone
/// approximation.
std::vector<int> r;
/// Coefficients of friction for the ns = nc - nns sliding contacts (where
/// `nns` is the number of non-sliding contacts). The size of this vector
/// should be equal to `sliding_contacts.size()`.
VectorX<T> mu_sliding;
/// Coefficients of friction for the nns = nc - ns non-sliding contacts (where
/// `ns` is the number of sliding contacts). The size of this vector should be
/// equal to `non_sliding_contacts.size()`.
VectorX<T> mu_non_sliding;
/// @name Data for bilateral constraints at the acceleration level
/// Problem data for bilateral constraints of functions of system
/// acceleration, where the constraint can be formulated as:<pre>
/// 0 = G(q)⋅v̇ + kᴳ(t,q,v)
/// </pre>
/// which implies the constraint definition g(t,q,v,v̇) ≡ G(q)⋅v̇ + kᴳ(t,q,v).
/// G is defined as the ℝⁿᵇˣⁿᵛ Jacobian matrix that transforms generalized
/// velocities (v ∈ ℝⁿᵛ) into the time derivatives of b bilateral constraint
/// functions. The class of constraint functions naturally includes holonomic
/// constraints, which are constraints posable as g(t,q). Such holonomic
/// constraints must be twice differentiated with respect to time to yield
/// an acceleration-level formulation (i.e., g̈(t, q, v, v̇), for the
/// aforementioned definition of g(t,q)). That differentiation yields
/// g̈ = G⋅v̇ + dG/dt⋅v, which is consistent with the constraint class under
/// the definition kᴳ(t,q,v) ≡ dG/dt⋅v. An example such (holonomic) constraint
/// function is the transmission (gearing) constraint below:<pre>
/// 0 = v̇ᵢ - rv̇ⱼ
/// </pre>
/// which can be read as the acceleration at joint i (v̇ᵢ) must equal to `r`
/// times the acceleration at joint j (v̇ⱼ); `r` is thus the gear ratio.
/// In this example, the corresponding holonomic constraint function is
/// g(q) ≡ qᵢ - rqⱼ, yielding ̈g(q, v, v̇) = v̇ᵢ - rv̇ⱼ.
/// @{
/// An operator that performs the multiplication G⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> G_mult;
/// An operator that performs the multiplication Gᵀ⋅f where f ∈ ℝⁿᵇ are the
/// magnitudes of the constraint forces. The default operator returns a
/// zero vector of dimension equal to that of the generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> G_transpose_mult;
/// This ℝⁿᵇ vector is the vector kᴳ(t,q,v) defined above.
VectorX<T> kG;
/// @}
/// @name Data for constraints on accelerations along the contact normal
/// Problem data for constraining the acceleration of two bodies projected
/// along the contact surface normal, for n point contacts.
/// These data center around two Jacobian matrices, N and Q. N is the ℝⁿᶜˣⁿᵛ
/// Jacobian matrix that transforms generalized velocities (v ∈ ℝⁿᵛ) into
/// velocities projected along the contact normals at the nc point contacts.
/// Q ∈ ℝⁿᶜˣⁿᵛ is the Jacobian matrix that transforms generalized velocities
/// (nv is the dimension of generalized velocity) into velocities projected
/// along the directions of sliding at the ns *sliding* contact points (rows
/// of Q that correspond to non-sliding contacts should be zero). Finally,
/// the Jacobian matrix N allows formulating the non-interpenetration
/// constraint (a constraint imposed at the velocity level) as:<pre>
/// 0 ≤ N(q)⋅v̇ + kᴺ(t,q,v) ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint g̈(q,v,v̇) ≡ N(q)⋅v̇ + kᴺ(t,q,v) is
/// coupled to a force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(Nv̇ + kᴺ(t,q,v)) = 0, meaning that the constraint can apply no force
/// if it is inactive (i.e., if g̈(q,v,v̇) is strictly greater than zero).
/// Note that differentiating the original constraint ġ(t,q,v) ≡ Nv (i.e.,
/// the constraint posed at the velocity level) once with
/// respect to time, such that all constraints are imposed at the
/// acceleration level, yields: <pre>
/// g̈(t,q,v,v̇) = N(q) v̇ + dN/dt(q,v) v
/// </pre>
/// Thus, the constraint at the acceleration level can be realized by setting
/// kᴺ(t,q,v) = dN/dt(q,v)⋅v. If there is pre-existing constraint error (e.g.,
/// if N(q)⋅v < 0), the kᴺ term can be used to "stabilize" this error.
/// For example, one could set `kᴺ(t,q,v) = dN/dt(q,v)⋅v + α⋅N(q)⋅v`, for
/// α ≥ 0.
/// </pre>
/// @{
/// An operator that performs the multiplication N⋅v.
/// The default operator returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> N_mult;
/// An operator that performs the multiplication (Nᵀ - μQᵀ)⋅f, where μ is a
/// diagonal matrix with nonzero entries corresponding to the coefficients of
/// friction at the s sliding contact points, and (Nᵀ - μQᵀ) transforms forces
/// (f ∈ ℝⁿᶜ) applied along the contact normals at the nc point contacts into
/// generalized forces. The default operator returns a zero vector of
/// dimension equal to that of the generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> N_minus_muQ_transpose_mult;
/// This ℝⁿᶜ vector is the vector kᴺ(t,q,v) defined above.
VectorX<T> kN;
/// @}
/// @name Data for non-sliding contact friction constraints
/// Problem data for constraining the tangential acceleration of two bodies
/// projected along the contact surface tangents, for nc point contacts.
/// These data center around the Jacobian matrix, F ∈ ℝⁿⁿʳˣⁿᵛ, that
/// transforms generalized velocities (v ∈ ℝⁿᵛ) into velocities projected
/// along the nr vectors that span the contact tangents at the nns
/// *non-sliding* point contacts (these nns * nr vectors are denoted `nnr`,
/// like "total number of nr", for brevity). For contact problems in two
/// dimensions, nr will be one and nnr would equal nns. For friction pyramids
/// at each contact in three dimensions, nr would be two and nnr would equal
/// 2nns. While the definition of nnr above indicates that every one of the
/// nns non-sliding contacts uses the same "nr", the code imposes no such
/// requirement. Finally, the Jacobian matrix F allows formulating the
/// non-sliding friction force constraints as:<pre>
/// 0 ≤ F(q)⋅v̇ + kᶠ(t,q,v) + λe ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint g̈(t,q,v,v̇) ≡ F(q)⋅v̇ + kᶠ(t,q,v) is
/// coupled to a force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(Fv̇ + kᴺ(t,q,v) + λe) = 0: the constraint can apply no
/// force if it is inactive (i.e., if g̈(t,q,v,v̇) is strictly greater than
/// zero). The presence of the λe term is taken directly from [Anitescu 1997],
/// where e is a vector of ones and zeros and λ corresponds roughly to the
/// tangential acceleration at the contacts. The interested reader should
/// refer to [Anitescu 1997] for a more thorough explanation of this
/// constraint; the full constraint equation is presented only to elucidate
/// the purpose of the kᶠ term. Analogously to the case of kᴺ, kᶠ should be
/// set to dF/dt(q,v)⋅v; also analogously, kᶠ can be used to perform
/// constraint stabilization.
/// @{
/// An operator that performs the multiplication F⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> F_mult;
/// An operator that performs the multiplication Fᵀ⋅f, where f ∈ ℝⁿⁿˢʳ
/// corresponds to frictional force magnitudes. The default operator returns
/// a zero vector of dimension equal to that of the generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> F_transpose_mult;
/// This ℝⁿⁿˢʳ vector is the vector kᶠ(t,q,v) defined above.
VectorX<T> kF;
/// @}
/// @name Data for unilateral constraints at the acceleration level
/// Problem data for unilateral constraints of functions of system
/// acceleration, where the constraint can be formulated as:<pre>
/// 0 ≤ L(q)⋅v̇ + kᴸ(t,q,v) ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint g(q,v,v̇) ≡ L(q)⋅v̇ + kᴸ(t,q,v) is coupled
/// to a force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(L⋅v̇ + kᴸ(t,q,v)) = 0, meaning that the constraint can apply no force
/// if it is inactive (i.e., if g(q,v,v̇) is strictly greater than zero). L
/// is defined as the ℝⁿᵘˣⁿᵛ Jacobian matrix that transforms generalized
/// velocities (v ∈ ℝⁿᵛ) into the time derivatives of nu unilateral constraint
/// functions. The class of constraint functions naturally includes holonomic
/// constraints, which are constraints posable as g(t,q). Such holonomic
/// constraints must be twice differentiated with respect to time to yield
/// an acceleration-level formulation (i.e., g̈(q, v, v̇, t), for the
/// aforementioned definition of g(t,q)). That differentiation yields
/// g̈ = L⋅v̇ + dL/dt⋅v, which is consistent with the constraint class under
/// the definition kᴸ(t,q,v) ≡ dL/dt⋅v. An example such holonomic constraint
/// function is a joint acceleration limit:<pre>
/// 0 ≤ -v̇ⱼ + r ⊥ fᶜⱼ ≥ 0
/// </pre>
/// which can be read as the acceleration at joint j (v̇ⱼ) must be no larger
/// than r, the force must be applied to limit the acceleration at the joint,
/// and the limiting force cannot be applied if the acceleration at the
/// joint is not at the limit (i.e., v̇ⱼ < r). In this example, the
/// corresponding holonomic constraint function is g(t,q) ≡ -qⱼ + rt²,
/// yielding ̈g(q, v, v̇) = -v̇ⱼ + r.
/// @{
/// An operator that performs the multiplication L⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> L_mult;
/// An operator that performs the multiplication Lᵀ⋅f where f ∈ ℝⁿᵘ are the
/// magnitudes of the constraint forces. The default operator returns a
/// zero vector of dimension equal to that of the generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> L_transpose_mult;
/// This ℝⁿᵘ vector is the vector kᴸ(t,q,v) defined above.
VectorX<T> kL;
/// @}
/// The ℝⁿᵛ vector tau, the generalized external force vector that
/// comprises gravitational, centrifugal, Coriolis, actuator, etc. forces
/// applied to the rigid body system at q. m is the dimension of the
/// generalized force, which is also equal to the dimension of the
/// generalized velocity.
VectorX<T> tau;
/// A function for solving the equation MX = B for matrix X, given input
/// matrix B, where M is the generalized inertia matrix for the rigid body
/// system.
std::function<MatrixX<T>(const MatrixX<T>&)> solve_inertia;
};
/// Structure for holding constraint data for computing constraint forces
/// at the velocity-level (i.e., impact problems).
template <class T>
struct ConstraintVelProblemData {
/// Constructs velocity problem data for a system with a @p gv_dim dimensional
/// generalized velocity.
explicit ConstraintVelProblemData(int gv_dim) { Reinitialize(gv_dim); }
/// Reinitializes the constraint problem data using the specified dimension
/// of the generalized velocities.
void Reinitialize(int gv_dim) {
// Set default for non-transpose operators- returns an empty vector.
auto zero_fn = [](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>(0);
};
N_mult = zero_fn;
F_mult = zero_fn;
L_mult = zero_fn;
G_mult = zero_fn;
// Set default for transpose operators - returns the appropriately sized
// zero vector.
auto zero_gv_dim_fn = [gv_dim](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>::Zero(gv_dim);
};
N_transpose_mult = zero_gv_dim_fn;
F_transpose_mult = zero_gv_dim_fn;
L_transpose_mult = zero_gv_dim_fn;
G_transpose_mult = zero_gv_dim_fn;
}
/// The number of spanning vectors in the contact tangents (used to linearize
/// the friction cone) at the nc contact points. For contact
/// problems in two dimensions, each element of r will be one. For contact
/// problems in three dimensions, a friction pyramid (for example), for a
/// contact point i will have rᵢ = 2. [Anitescu 1997] define k such vectors
/// and require that, for each vector w in the spanning set, -w also exists
/// in the spanning set. The RigidContactVelProblemData structure expects
/// that the contact solving mechanism negates the spanning vectors so `r` =
/// k/2 spanning vectors will correspond to a k-edge polygon friction cone
/// approximation.
std::vector<int> r;
/// Coefficients of friction for the nc contacts. This problem specification
/// does not distinguish between static and dynamic friction coefficients.
VectorX<T> mu;
/// @name Data for bilateral constraints at the velocity level
/// Problem data for bilateral constraints of functions of system
/// velocity, where the constraint can be formulated as:<pre>
/// 0 = G(q)⋅v + kᴳ(t,q)
/// </pre>
/// which implies the constraint definition g(t,q,v) ≡ G(q)⋅v + kᴳ(t,q). G
/// is defined as the ℝⁿᵇˣⁿᵛ Jacobian matrix that transforms generalized
/// velocities (v ∈ ℝⁿᵛ) into the time derivatives of nb bilateral constraint
/// functions. The class of constraint functions naturally includes holonomic
/// constraints, which are constraints posable as g(t,q). Such holonomic
/// constraints must be differentiated with respect to time to yield
/// a velocity-level formulation (i.e., ġ(t, q, v), for the
/// aforementioned definition of g(t,q)). That differentiation yields
/// ġ = G⋅v, which is consistent with the constraint class under
/// the definition kᴳ(t,q) ≡ 0. An example such holonomic constraint
/// function is the transmission (gearing) constraint below:<pre>
/// 0 = vᵢ - rvⱼ
/// </pre>
/// which can be read as the velocity at joint i (vᵢ) must equal to `r`
/// times the velocity at joint j (vⱼ); `r` is thus the gear ratio.
/// In this example, the corresponding holonomic constraint function is
/// g(q) ≡ qᵢ - rqⱼ, yielding ġ(q, v) = vᵢ - rvⱼ.
/// @{
/// An operator that performs the multiplication G⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> G_mult;
/// An operator that performs the multiplication Gᵀ⋅f where f ∈ ℝⁿᵇ are the
/// magnitudes of the constraint forces. The default operator returns a
/// zero vector of dimension equal to that of the generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> G_transpose_mult;
/// This ℝⁿᵇ vector is the vector kᴳ(t,q) defined above.
VectorX<T> kG;
/// @}
/// @name Data for constraints on velocities along the contact normal
/// Problem data for constraining the velocity of two bodies projected
/// along the contact surface normal, for n point contacts.
/// These data center around the Jacobian matrix N, the ℝⁿᶜˣⁿᵛ
/// Jacobian matrix that transforms generalized velocities (v ∈ ℝⁿᵛ) into
/// velocities projected along the contact normals at the n point contacts.
/// Constraint error (φ < 0, where φ is the signed distance between two
/// bodies) can be incorporated into the constraint solution process (and
/// thereby reduced) through setting the `kN` term to something other than its
/// nonzero value (typically `kN = αφ`, where `α ≥ 0`). The resulting
/// constraint on the motion will be: <pre>
/// 0 ≤ N(q) v + kᴺ(t,q) ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint ċ(q,v) ≡ N(q)⋅v + kᴺ(t,q) is coupled
/// to an impulsive force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(Nv + kᴺ(t,q)) = 0, meaning that the constraint can apply no force
/// if it is inactive (i.e., if ġ(q,v) is strictly greater than zero).
/// @{
/// An operator that performs the multiplication N⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> N_mult;
/// An operator that performs the multiplication Nᵀ⋅f, where f ∈ ℝⁿᶜ are the
/// the magnitudes of the impulsive forces applied along the contact normals
/// at the nc point contacts. The default operator returns a zero vector of
/// dimension equal to that of the generalized velocities (which should be
/// identical to the dimension of the generalized forces).
std::function<VectorX<T>(const VectorX<T>&)> N_transpose_mult;
/// This ℝⁿᶜ vector is the vector kᴺ(t,q,v) defined above.
VectorX<T> kN;
/// This ℝⁿᶜ vector represents the diagonal matrix γᴺ.
VectorX<T> gammaN;
/// @}
/// @name Data for constraints on contact friction
/// Problem data for constraining the tangential velocity of two bodies
/// projected along the contact surface tangents, for n point contacts.
/// These data center around the Jacobian matrix, F ∈ ℝⁿⁿʳˣⁿᵛ, that
/// transforms generalized velocities (v ∈ ℝⁿᵛ) into velocities projected
/// along the nr vectors that span the contact tangents at the nc
/// point contacts (these nc * nr vectors are denoted nnr for brevity). For
/// contact problems in two dimensions, nr will be one and nnr would equal nc.
/// For a friction pyramid at each point contact in three dimensions, nr
/// would be two and nnr would equation 2nc. While the definition of the
/// dimension of the Jacobian matrix above indicates that every one of the nc
/// contacts uses the same "nr", the code imposes no such requirement.
/// Constraint error (F⋅v < 0) can be reduced through the constraint solution
/// process by setting the `kF` term to something other than its default zero
/// value. The resulting constraint on the motion will
/// be:<pre>
/// 0 ≤ F(q)⋅v + kᴺ(t,q) + eλ ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint ġ(q,v) ≡ F(q)⋅v + kᶠ(t,q) + eλ is coupled
/// to an impulsive force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(Fv + kᶠ(t,q) + eλ) = 0, meaning that the constraint can apply no force
/// if it is inactive (i.e., if ġ(q,v) is strictly greater than zero).
/// The presence of the λe term is taken directly from [Anitescu 1997],
/// where e is a vector of ones and zeros and λ corresponds roughly to the
/// tangential acceleration at the contacts. The interested reader should
/// refer to [Anitescu 1997] for a more thorough explanation of this
/// constraint; the full constraint equation is presented only to elucidate
/// the purpose of the kᶠ term.
/// @{
/// An operator that performs the multiplication F⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> F_mult;
/// An operator that performs the multiplication Fᵀ⋅f, where f ∈ ℝⁿᶜʳ
/// corresponds to frictional impulsive force magnitudes. The default
/// operator returns a zero vector of dimension equal to that of the
/// generalized forces.
std::function<VectorX<T>(const VectorX<T>&)> F_transpose_mult;
/// This ℝⁿᶜʳ vector is the vector kᶠ(t,q,v) defined above.
VectorX<T> kF;
/// This ℝⁿᶜʳ vector represents the diagonal matrix γᶠ.
VectorX<T> gammaF;
/// This ℝⁿᶜ vector represents the diagonal matrix γᴱ.
VectorX<T> gammaE;
/// @}
/// @name Data for unilateral constraints at the velocity level
/// Problem data for unilateral constraints of functions of system
/// velocity, where the constraint can be formulated as:<pre>
/// 0 ≤ L(q)⋅v + kᴸ(t,q) ⊥ fᶜ ≥ 0
/// </pre>
/// which means that the constraint ċ(q,v) ≡ L(q)⋅v + kᴸ(t,q) is coupled
/// to an impulsive force constraint (fᶜ ≥ 0) and a complementarity constraint
/// fᶜ⋅(L⋅v + kᴸ(t,q)) = 0, meaning that the constraint can apply no force
/// if it is inactive (i.e., if ġ(q,v) is strictly greater than zero). L
/// is defined as the ℝⁿᵘˣⁿᵛ Jacobian matrix that transforms generalized
/// velocities (v ∈ ℝⁿᵛ) into the time derivatives of nu unilateral constraint
/// functions. The class of constraint functions naturally includes holonomic
/// constraints, which are constraints posable as g(q, t). Such holonomic
/// constraints must be differentiated with respect to time to yield
/// a velocity-level formulation (i.e., ġ(q, v, t), for the aforementioned
/// definition of g(q, t)). That differentiation yields ġ = L⋅v, which is
/// consistent with the constraint class under the definition kᴸ(t,q) ≡ 0. An
/// example such holonomic constraint function is a joint velocity limit:<pre>
/// 0 ≤ -vⱼ + r ⊥ fᶜⱼ ≥ 0
/// </pre>
/// which can be read as the velocity at joint j (vⱼ) must be no larger than
/// r, the impulsive force must be applied to limit the acceleration at the
/// joint, and the limiting force cannot be applied if the velocity at the
/// joint is not at the limit (i.e., vⱼ < r). In this example, the constraint
/// function is g(t,q) ≡ qⱼ + rt, yielding ġ(q, v) = -vⱼ + r.
/// @{
/// An operator that performs the multiplication L⋅v. The default operator
/// returns an empty vector.
std::function<VectorX<T>(const VectorX<T>&)> L_mult;
/// An operator that performs the multiplication Lᵀ⋅f where f ∈ ℝᵗ are the
/// magnitudes of the impulsive constraint forces. The default operator
/// returns a zero vector of dimension equal to that of the generalized
/// forces.
std::function<VectorX<T>(const VectorX<T>&)> L_transpose_mult;
/// This ℝⁿᵘ vector is the vector kᴸ(t,q) defined above.
VectorX<T> kL;
/// This ℝⁿᵘ vector represents the diagonal matrix γᴸ.
VectorX<T> gammaL;
/// @}
/// The ℝⁿᵛ generalized momentum immediately before any impulsive
/// forces (from impact) are applied.
VectorX<T> Mv;
/// A function for solving the equation MX = B for matrix X, given input
/// matrix B, where M is the generalized inertia matrix for the rigid body
/// system.
std::function<MatrixX<T>(const MatrixX<T>&)> solve_inertia;
};
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/constraint_solver.cc | #include "drake/examples/rod2d/constraint_solver.h"
namespace drake {
namespace examples {
namespace rod2d {
template class ConstraintSolver<double>;
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rod2d/constraint_solver.h | #pragma once
#include <algorithm>
#include <limits>
#include <memory>
#include <numeric>
#include <utility>
#include <vector>
#include "drake/common/fmt_eigen.h"
#include "drake/common/text_logging.h"
#include "drake/examples/rod2d/constraint_problem_data.h"
#include "drake/solvers/moby_lcp_solver.h"
namespace drake {
namespace examples {
namespace rod2d {
/// Solves constraint problems for constraint forces. Specifically, given
/// problem data corresponding to a rigid or multi-body system constrained
/// bilaterally and/or unilaterally and acted upon by friction, this class
/// computes the constraint forces.
///
/// This problem can be formulated as a mixed linear complementarity problem
/// (MLCP)- for 2D problems with Coulomb friction or 3D problems without Coulomb
/// friction- or a mixed complementarity problem (for 3D problems with
/// Coulomb friction). We use a polygonal approximation (of selectable accuracy)
/// to the friction cone, which yields a MLCP in all cases.
///
/// Existing algorithms for solving MLCPs, which are based upon algorithms for
/// solving "pure" linear complementarity problems (LCPs), solve smaller classes
/// of problems than the corresponding LCP versions. For example, Lemke's
/// Algorithm, which is provably able to solve the impacting problems covered by
/// this class, can solve LCPs with copositive matrices [Cottle 1992] but MLCPs
/// with only positive semi-definite matrices (the latter is a strict subset of
/// the former) [Sargent 1978].
///
/// Rather than using one of these MLCP algorithms, we instead transform the
/// problem into a pure LCP by first solving for the bilateral constraint
/// forces. This method yields an implication of which the user should be aware.
/// Bilateral constraint forces are computed before unilateral constraint
/// forces: the constraint forces will not be evenly distributed between
/// bilateral and unilateral constraints (assuming such a distribution were even
/// possible).
///
/// For the normal case of unilateral constraints admitting degrees of
/// freedom, the solution methods in this class support "softening" of the
/// constraints, as described in [Lacoursiere 2007] via the constraint force
/// mixing (CFM) and error reduction parameter (ERP) parameters that are now
/// ubiquitous in game multi-body dynamics simulation libraries.
///
/// - [Cottle 1992] R. W. Cottle, J.-S. Pang, and R. E. Stone. The Linear
/// Complementarity Problem. SIAM Classics in Applied
/// Mathematics, 1992.
/// - [Judice 1992] J. J. Judice, J. Machado, and A. Faustino. An extension of
/// the Lemke's method for the solution of a generalized
/// linear complementarity problem. In System Modeling and
/// Optimization (Lecture Notes in Control and Information
/// Sciences), Springer-Verlag, 1992.
/// - [Lacoursiere 2007] C. Lacoursiere. Ghosts and Machines: Regularized
/// Variational Methods for Interactive Simulations of
/// Multibodies with Dry Frictional Contacts.
/// Ph. D. thesis (Umea University), 2007.
/// - [Sargent 1978] R. W. H. Sargent. An efficient implementation of the Lemke
/// Algorithm and its extension to deal with upper and lower
/// bounds. Mathematical Programming Study, 7, 1978.
///
/// @tparam_double_only
template <typename T>
class ConstraintSolver {
public:
ConstraintSolver() = default;
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(ConstraintSolver)
/// Structure used to convert a mixed linear complementarity problem to a
/// pure linear complementarity problem (by solving for free variables).
struct MlcpToLcpData {
/// Decomposition of the Delassus matrix GM⁻¹Gᵀ, where G is the bilateral
/// constraint matrix and M is the system generalized inertia matrix.
Eigen::CompleteOrthogonalDecomposition<MatrixX<T>> delassus_QTZ;
/// A function pointer for solving linear systems using MLCP "A" matrix
/// (see @ref Velocity-level-MLCPs).
std::function<MatrixX<T>(const MatrixX<T>&)> A_solve;
/// A function pointer for solving linear systems using only the upper left
/// block of A⁺ in the MLCP (see @ref Velocity-level-MLCPs), where A⁺ is
/// a singularity-robust pseudo-inverse of A, toward exploiting operations
/// with zero blocks. For example:<pre>
/// A⁺ | b |
/// | 0 |</pre> and<pre>
/// A⁺ | B |
/// | 0 |
/// </pre>
/// where `b ∈ ℝⁿᵛ` is an arbitrary vector of dimension equal to the
/// generalized velocities and `B ∈ ℝⁿᵛˣᵐ` is an arbitrary matrix with
/// row dimension equal to the dimension of the generalized velocities and
/// arbitrary number of columns (denoted `m` here).
std::function<MatrixX<T>(const MatrixX<T>&)> fast_A_solve;
};
// TODO(edrumwri): Describe conditions under which it is safe to replace
// A⁻¹ by a pseudo-inverse.
// TODO(edrumwri): Constraint solver needs to use monogram notation throughout
// to make it consistent with the rest of Drake's multibody dynamics
// documentation (see Issue #9080).
/// @name Velocity-level constraint problems formulated as MLCPs.
/// @anchor Velocity-level-MLCPs
/// Constraint problems can be posed as mixed linear complementarity problems
/// (MLCP), which are problems that take the form:<pre>
/// (a) Au + X₁y + a = 0
/// (b) X₂u + X₃y + x₄ ≥ 0
/// (c) y ≥ 0
/// (d) vᵀ(x₄ + X₂u + X₃y) = 0
/// </pre>
/// where `u` are "free" variables, `y` are constrained variables, `A`, `X₁`,
/// `X₂`, and `X₃` are given matrices (we label only the most important
/// variables without subscripts to make them stand out) and `a` and `x₄` are
/// given vectors. If `A` is nonsingular, `u` can be solved for:<pre>
/// (e) u = -A⁻¹ (a + X₁y)
/// </pre>
/// allowing the mixed LCP to be converted to a "pure" LCP `(qq, MM)` by:<pre>
/// (f) qq = x₄ - X₂A⁻¹a
/// (g) MM = X₃ - X₂A⁻¹X₁
/// </pre>
/// This pure LCP can then be solved for `y` such that:<pre>
/// (h) MMv + qq ≥ 0
/// (i) y ≥ 0
/// (j) yᵀ(MMv + qq) = 0
/// </pre>
/// and this value for `y` can be substituted into (e) to obtain the value
/// for `u`.
///
/// <h3>An MLCP-based impact model:</h3>
/// Consider the following problem formulation of a multibody dynamics
/// impact model (taken from [Anitescu 1997]). In a simulator, one could use
/// this model when a collision is detected in order to compute an
/// instantaneous change in velocity.
/// <pre>
/// (1) | M -Gᵀ -Nᵀ -Dᵀ 0 -Lᵀ | | v⁺ | + |-Mv⁻ | = | 0 |
/// | G 0 0 0 0 0 | | fG | + | kᴳ | = | 0 |
/// | N 0 0 0 0 0 | | fN | + | kᴺ | = | x₅ |
/// | D 0 0 0 E 0 | | fD | + | kᴰ | = | x₆ |
/// | 0 0 μ -Eᵀ 0 0 | | λ | + | 0 | = | x₇ |
/// | L 0 0 0 0 0 | | fL | + | kᴸ | = | x₈ |
/// (2) 0 ≤ fN ⊥ x₅ ≥ 0
/// (3) 0 ≤ fD ⊥ x₆ ≥ 0
/// (4) 0 ≤ λ ⊥ x₇ ≥ 0
/// (5) 0 ≤ fL ⊥ x₈ ≥ 0
/// </pre>
/// Here, the velocity variables
/// v⁻ ∈ ℝⁿᵛ, v ∈ ℝⁿᵛ⁺ correspond to the velocity of the system before and
/// after impulses are applied, respectively. More details will be forthcoming
/// but key variables are `M ∈ ℝⁿᵛˣⁿᵛ`, the generalized inertia matrix;
/// `G ∈ ℝⁿᵇˣⁿᵛ`, `N ∈ ℝⁿᶜˣⁿᵛ`, `D ∈ ℝⁿᶜᵏˣⁿᵛ`, and `L ∈ ℝⁿᵘˣⁿᵛ` correspond to
/// Jacobian matrices for various constraints (joints, contact, friction,
/// generic unilateral constraints, respectively); `μ ∈ ℝⁿᶜˣⁿᶜ` is a diagonal
/// matrix comprised of Coulomb friction coefficients; `E ∈ ℝⁿᶜᵏˣⁿᶜ` is a
/// binary matrix used to linearize the friction cone (necessary to make
/// this into a *linear* complementarity problem); `fG ∈ ℝⁿᵇ`, `fN ∈ ℝⁿᶜ`,
/// `fD ∈ ℝⁿᶜᵏ`, and `fL ∈ ℝⁿᵘ` are constraint impulses; `λ ∈ ℝⁿᶜ`, `x₅`,
/// `x₆`, `x₇`, and `x₈` can be viewed as mathematical programming "slack"
/// variables; and `kᴳ ∈ ℝⁿᵇ`, `kᴺ ∈ ℝⁿᶜ`, `kᴰ ∈ ℝⁿᶜᵏ`, `kᴸ ∈ ℝⁿᵘ`
/// allow customizing the problem to, e.g., correct constraint violations and
/// simulate restitution. See "Variable definitions" in the local README for
/// complete definitions of `nv`, `nc`, `nb`, etc.
///
/// From the notation above in Equations (a)-(d), we can convert the MLCP
/// to a "pure" linear complementarity problem (LCP), which is easier to
/// solve, at least for active-set-type mathematical programming
/// approaches:<pre>
/// A ≡ | M -Ĝᵀ| a ≡ |-Mv⁻ | X₁ ≡ |-Nᵀ -Dᵀ 0 -Lᵀ |
/// | Ĝ 0 | | kᴳ | | 0 0 0 0 |
///
/// X₂ ≡ | N 0 | b ≡ | kᴺ | X₃ ≡ | 0 0 0 0 |
/// | D 0 | | kᴰ | | 0 0 E 0 |
/// | 0 0 | | 0 | | μ -Eᵀ 0 0 |
/// | L 0 | | kᴸ | | 0 0 0 0 |
///
/// u ≡ | v⁺ | y ≡ | fN |
/// | fG | | fD |
/// | λ |
/// | fL |
/// </pre>
/// Where applicable, ConstraintSolver computes solutions to linear equations
/// with rank-deficient `A` (e.g., `AX₅ = x₆`) using a least squares approach
/// (the complete orthogonal factorization). Now, using Equations (f) and (g)
/// and defining `C` as the nv × nv-dimensional upper left block of
/// `A⁻¹` (`nv` is the dimension of the generalized velocities) the pure
/// LCP `(qq,MM)` is defined as:<pre>
/// MM ≡ | NCNᵀ NCDᵀ 0 NCLᵀ |
/// | DCNᵀ DCDᵀ E DCLᵀ |
/// | μ -Eᵀ 0 0 |
/// | LCNᵀ LCDᵀ 0 LCLᵀ |
///
/// qq ≡ | kᴺ - |N 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |
/// | kᴰ - |D 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |
/// | 0 |
/// | kᴸ - |L 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |
/// </pre>
/// where `nb` is the number of bilateral constraint equations. The solution
/// `y` will then take the form:<pre>
/// y ≡ | fN |
/// | fD |
/// | λ |
/// | fL |
/// </pre>
/// The key variables for using the MLCP-based formulations are the matrix `A`
/// and vector `a`, as seen in documentation of MlcpToLcpData and the
/// following methods. During its operation, ConstructBaseDiscretizedTimeLcp()
/// constructs (and returns) functions for solving `AX=B`, where `B` is a
/// given matrix and `X` is an unknown matrix. UpdateDiscretizedTimeLcp()
/// computes and returns `a` during its operation.
///
/// <h3>Another use of the MLCP formulation (discretized multi-body dynamics
/// with contact and friction):</h3>
///
/// Without reconstructing the entire MLCP, we now show a very similar
/// formulation to solve the problem of discretizing
/// the multi-body dynamics equations with contact and friction. This
/// particular formulation provides several nice features: 1) the formulation
/// is semi-implicit and models compliant contact efficiently, including both
/// sticking contact and contact between very stiff surfaces; 2) all
/// constraint forces are computed in Newtons (typical "time stepping
/// methods" require considerable care to correctly compare constraint forces,
/// which are impulsive, and non-constraint forces, which are non-impulsive);
/// and 3) can be made almost symplectic by choosing a
/// representation and computational coordinate frame that minimize
/// velocity-dependent forces (thereby explaining the extreme stability of
/// software like ODE and Bullet that computes dynamics in body frames
/// (minimizing the magnitudes of velocity-dependent forces) and provides
/// the ability to disable gyroscopic forces.
///
/// The discretization problem replaces the meaning of v⁻ and v⁺ in the MLCP
/// to mean the generalized velocity at time t and the generalized velocity
/// at time t+h, respectively, for discretization quantum h (or, equivalently,
/// integration step size h). The LCP is adjusted to the form:<pre>
/// MM ≡ | hNCNᵀ+γᴺ hNCDᵀ 0 hNCLᵀ |
/// | hDCNᵀ hDCDᵀ E hDCLᵀ |
/// | μ -Eᵀ 0 0 |
/// | hLCNᵀ hLCDᵀ 0 hLCLᵀ+γᴸ |
///
/// qq ≡ | kᴺ - |N 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |
/// | kᴰ - |D 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |
/// | 0 |
/// | kᴸ - |L 0ⁿᵛ⁺ⁿᵇ|A⁻¹a |</pre>
/// where `γᴺ`, `γᴸ`, `kᴺ`, `kᴸ`, and `a` are all functions of `h`;
/// documentation that describes how to update these (and dependent) problem
/// data to attain desired constraint stiffness and dissipation is
/// forthcoming.
///
/// The procedure one uses to formulate and solve this discretization problem
/// is:
/// -# Call ConstructBaseDiscretizedTimeLcp()
/// -# Select an integration step size, dt
/// -# Compute `kᴺ' and `kᴸ` in the problem data, accounting for dt as
/// necessary
/// -# Call UpdateDiscretizedTimeLcp(), obtaining MM and qq that encode the
/// linear complementarity problem
/// -# Solve the linear complementarity problem
/// -# If LCP solved, quit.
/// -# Reduce dt and repeat the process from 3. until success.
///
/// The solution to the LCP can be used to obtain the constraint forces via
/// PopulatePackedConstraintForcesFromLcpSolution().
///
/// <h3>Obtaining the generalized constraint forces:</h3>
///
/// Given the constraint forces, which have been obtained either through
/// SolveImpactProblem() (in which case the forces are impulsive) or through
/// direct solution of the LCP corresponding to the discretized multibody
/// dynamics problem, followed by
/// PopulatePackedConstraintForcesFromLcpSolution() (in which cases the forces
/// are non-impulsive), the generalized forces/impulses due to the constraints
/// can then be acquired via ComputeGeneralizedForceFromConstraintForces().
// @{
/// Computes the base time-discretization of the system using the problem
/// data, resulting in the `MM` and `qq` described in
/// @ref Velocity-level-MLCPs; if `MM` and `qq` are modified no further, the
/// LCP corresponds to an impact problem (i.e., the multibody dynamics problem
/// would not be discretized). The data output (`mlcp_to_lcp_data`, `MM`, and
/// `qq`) can be updated using a particular time step in
/// UpdateDiscretizedTimeLcp(), resulting in a non-impulsive problem
/// formulation. In that case, the multibody dynamics equations *are*
/// discretized, as described in UpdateDiscretizedTimeLcp().
/// @note If you really do wish to solve an impact problem, you should use
/// SolveImpactProblem() instead.
/// @param problem_data the constraint problem data.
/// @param[out] mlcp_to_lcp_data a pointer to a valid MlcpToLcpData object;
/// the caller must ensure that this pointer remains valid through
/// the constraint solution process.
/// @param[out] MM a pointer to a matrix that will contain the parts of the
/// LCP matrix not dependent upon the time step on return.
/// @param[out] qq a pointer to a vector that will contain the parts of the
/// LCP vector not dependent upon the time step on return.
/// @pre `mlcp_to_lcp_data`, `MM`, and `qq` are non-null on entry.
/// @see UpdateDiscretizedTimeLcp()
static void ConstructBaseDiscretizedTimeLcp(
const ConstraintVelProblemData<T>& problem_data,
MlcpToLcpData* mlcp_to_lcp_data, MatrixX<T>* MM, VectorX<T>* qq);
/// Updates the time-discretization of the LCP initially computed in
/// ConstructBaseDiscretizedTimeLcp() using the problem data and time step
/// `h`. Solving the resulting pure LCP yields non-impulsive constraint forces
/// that can be obtained from PopulatePackedConstraintForcesFromLcpSolution().
/// @param problem_data the constraint problem data.
/// @param[out] mlcp_to_lcp_data a pointer to a valid MlcpToLcpData object;
/// the caller must ensure that this pointer remains valid through
/// the constraint solution process.
/// @param[out] a the vector corresponding to the MLCP vector `a`, on return.
/// @param[out] MM a pointer to the updated LCP matrix on return.
/// @param[out] qq a pointer to the updated LCP vector on return.
/// @pre `mlcp_to_lcp_data`, `a`, `MM`, and `qq` are non-null on entry.
/// @see ConstructBaseDiscretizedTimeLcp()
static void UpdateDiscretizedTimeLcp(
const ConstraintVelProblemData<T>& problem_data, double h,
MlcpToLcpData* mlcp_to_lcp_data, VectorX<T>* a, MatrixX<T>* MM,
VectorX<T>* qq);
/// Solves the impact problem described above.
/// @param problem_data The data used to compute the impulsive constraint
/// forces.
/// @param cf The computed impulsive forces, on return, in a packed storage
/// format. The first `nc` elements of `cf` correspond to the
/// magnitudes of the contact impulses applied along the normals of
/// the `nc` contact points. The next elements of `cf`
/// correspond to the frictional impulses along the `r` spanning
/// directions at each point of contact. The first `r`
/// values (after the initial `nc` elements) correspond to the first
/// contact, the next `r` values correspond to the second contact,
/// etc. The next `ℓ` values of `cf` correspond to the impulsive
/// forces applied to enforce unilateral constraint functions. The
/// final `b` values of `cf` correspond to the forces applied to
/// enforce generic bilateral constraints. This packed storage
/// format can be turned into more useful representations through
/// ComputeGeneralizedForceFromConstraintForces() and
/// CalcContactForcesInContactFrames(). `cf` will be resized as
/// necessary.
/// @pre Constraint data has been computed.
/// @throws std::exception if the constraint forces cannot be computed
/// (due to, e.g., the effects of roundoff error in attempting to
/// solve a complementarity problem); in such cases, it is
/// recommended to increase regularization and attempt again.
/// @throws std::exception if `cf` is null.
void SolveImpactProblem(const ConstraintVelProblemData<T>& problem_data,
VectorX<T>* cf) const;
/// Populates the packed constraint force vector from the solution to the
/// linear complementarity problem (LCP) constructed using
/// ConstructBaseDiscretizedTimeLcp() and UpdateDiscretizedTimeLcp().
/// @param problem_data the constraint problem data.
/// @param mlcp_to_lcp_data a reference to a MlcpToLcpData object.
/// @param zz the solution to the LCP resulting from
/// UpdateDiscretizedTimeLcp().
/// @param a the vector `a` output from UpdateDiscretizedTimeLcp().
/// @param dt the time step used to discretize the problem.
/// @param[out] cf the constraint forces, on return. The first `nc` elements
/// of `cf` correspond to the magnitudes of the contact forces applied
/// along the normals of the `nc` contact points. The next elements of
/// `cf` correspond to the frictional forces along the `r` spanning
/// directions at each point of contact. The first `r` values (after
/// the initial `nc` elements) correspond to the first contact, the
/// next `r` values correspond to the second contact, etc. The next
/// `ℓ` values of `cf` correspond to the impulsive forces applied to
/// enforce unilateral constraint functions. The final `b` values of
/// `cf` correspond to the forces applied to enforce generic bilateral
/// constraints. This packed storage format can be turned into more
/// useful representations through
/// ComputeGeneralizedForceFromConstraintForces() and
/// CalcContactForcesInContactFrames().
/// @pre cf is non-null.
static void PopulatePackedConstraintForcesFromLcpSolution(
const ConstraintVelProblemData<T>& problem_data,
const MlcpToLcpData& mlcp_to_lcp_data, const VectorX<T>& zz,
const VectorX<T>& a, double dt, VectorX<T>* cf);
//@}
/// Solves the appropriate constraint problem at the acceleration level.
/// @param problem_data The data used to compute the constraint forces.
/// @param cf The computed constraint forces, on return, in a packed storage
/// format. The first `nc` elements of `cf` correspond to the
/// magnitudes of the contact forces applied along the normals of
/// the `nc` contact points. The next elements of `cf`
/// correspond to the frictional forces along the `r` spanning
/// directions at each non-sliding point of contact. The first `r`
/// values (after the initial `nc` elements) correspond to the first
/// non-sliding contact, the next `r` values correspond to the
/// second non-sliding contact, etc. The next `ℓ` values of `cf`
/// correspond to the forces applied to enforce generic unilateral
/// constraints. The final `b` values of `cf` correspond to the
/// forces applied to enforce generic bilateral constraints. This
/// packed storage format can be turned into more useful
/// representations through
/// ComputeGeneralizedForceFromConstraintForces() and
/// CalcContactForcesInContactFrames(). `cf` will be resized as
/// necessary.
/// @pre Constraint data has been computed.
/// @throws std::exception if the constraint forces cannot be computed
/// (due to, e.g., an "inconsistent" rigid contact configuration).
/// @throws std::exception if `cf` is null.
void SolveConstraintProblem(const ConstraintAccelProblemData<T>& problem_data,
VectorX<T>* cf) const;
/// Computes the generalized force on the system from the constraint forces
/// given in packed storage.
/// @param problem_data The data used to compute the contact forces.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for SolveConstraintProblem.
/// @param[out] generalized_force The generalized force acting on the system
/// from the total constraint wrench is stored here, on return.
/// This method will resize `generalized_force` as necessary. The
/// indices of `generalized_force` will exactly match the indices
/// of `problem_data.f`.
/// @throws std::exception if `generalized_force` is null or `cf`
/// vector is incorrectly sized.
static void ComputeGeneralizedForceFromConstraintForces(
const ConstraintAccelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_force);
/// Computes the generalized force on the system from the constraint forces
/// given in packed storage.
/// @param problem_data The data used to compute the contact forces.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for
/// PopulatePackedConstraintForcesFromLcpSolution().
/// @param[out] generalized_force The generalized force acting on the system
/// from the total constraint wrench is stored here, on return.
/// This method will resize `generalized_force` as necessary. The
/// indices of `generalized_force` will exactly match the indices
/// of `problem_data.f`.
/// @throws std::exception if `generalized_force` is null or `cf`
/// vector is incorrectly sized.
static void ComputeGeneralizedForceFromConstraintForces(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_force);
/// Computes the system generalized acceleration due to both external forces
/// and constraint forces.
/// @param problem_data The acceleration-level constraint data.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for SolveConstraintProblem.
/// @param[out] generalized_acceleration The generalized acceleration, on
/// return.
/// @throws std::exception if @p generalized_acceleration is null or
/// @p cf vector is incorrectly sized.
static void ComputeGeneralizedAcceleration(
const ConstraintAccelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_acceleration) {
ComputeGeneralizedAccelerationFromConstraintForces(
problem_data, cf, generalized_acceleration);
(*generalized_acceleration) += problem_data.solve_inertia(problem_data.tau);
}
/// Computes a first-order approximation of generalized acceleration due
/// *only* to constraint forces.
/// @param problem_data The velocity-level constraint data.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for SolveConstraintProblem.
/// @param v The system generalized velocity at time t.
/// @param dt The discretization time constant (i.e., the "time step" for
/// simulations) used to take the system's generalized velocities
/// from time t to time t + `dt`.
/// @param[out] generalized_acceleration The generalized acceleration, on
/// return. The original will be resized (if necessary) and
/// overwritten.
/// @warning This method uses the method `problem_data.solve_inertia()` in
/// order to compute `v(t+dt)`, so the computational demands may
/// be significant.
/// @throws std::exception if `generalized_acceleration` is null or
/// `cf` vector is incorrectly sized.
/// @pre `dt` is positive.
static void ComputeGeneralizedAcceleration(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& v,
const VectorX<T>& cf, double dt, VectorX<T>* generalized_acceleration);
/// Computes the system generalized acceleration due *only* to constraint
/// forces.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for SolveConstraintProblem.
/// @throws std::exception if @p generalized_acceleration is null or
/// @p cf vector is incorrectly sized.
static void ComputeGeneralizedAccelerationFromConstraintForces(
const ConstraintAccelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_acceleration);
/// Computes the system generalized acceleration due *only* to constraint
/// forces.
/// @param cf The computed constraint forces, in the packed storage
/// format described in documentation for SolveConstraintProblem.
/// @throws std::exception if @p generalized_acceleration is null or
/// @p cf vector is incorrectly sized.
static void ComputeGeneralizedAccelerationFromConstraintForces(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_acceleration);
/// Computes the change to the system generalized velocity from constraint
/// impulses.
/// @param cf The computed constraint impulses, in the packed storage
/// format described in documentation for SolveImpactProblem.
/// @throws std::exception if `generalized_delta_v` is null or
/// `cf` vector is incorrectly sized.
static void ComputeGeneralizedVelocityChange(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_delta_v);
/// Gets the contact forces expressed in each contact frame *for 2D contact
/// problems* from the "packed" solution returned by SolveConstraintProblem().
/// @param cf the output from SolveConstraintProblem()
/// @param problem_data the problem data input to SolveConstraintProblem()
/// @param contact_frames the contact frames corresponding to the contacts.
/// The first column of each matrix should give the contact normal,
/// while the second column gives a contact tangent. For sliding
/// contacts, the contact tangent should point along the direction of
/// sliding. For non-sliding contacts, the tangent direction should be
/// that used to determine `problem_data.F`. All vectors should be
/// expressed in the global frame.
/// @param[out] contact_forces a non-null vector of a doublet of values, where
/// the iᵗʰ element represents the force along each basis
/// vector in the iᵗʰ contact frame.
/// @throws std::exception if `contact_forces` is null, if
/// `contact_forces` is not empty, if `cf` is not the
/// proper size, if the number of tangent directions is not one per
/// non-sliding contact (indicating that the contact problem might not
/// be 2D), if the number of contact frames is not equal to the number
/// of contacts, or if a contact frame does not appear to be
/// orthonormal.
/// @note On return, the contact force at the iᵗʰ contact point expressed
/// in the world frame is `contact_frames[i]` * `contact_forces[i]`.
static void CalcContactForcesInContactFrames(
const VectorX<T>& cf, const ConstraintAccelProblemData<T>& problem_data,
const std::vector<Matrix2<T>>& contact_frames,
std::vector<Vector2<T>>* contact_forces);
/// Gets the contact forces expressed in each contact frame *for 2D contact
/// problems* from a "packed" solution returned by, e.g.,
/// SolveImpactProblem(). If the constraint forces are impulsive, the contact
/// forces are impulsive (with units of Ns); similarly, if the constraint
/// forces are non-impulsive, the contact forces will be non-impulsive (with
/// units of N).
/// @param cf the constraint forces in packed format.
/// @param problem_data the problem data input to SolveImpactProblem()
/// @param contact_frames the contact frames corresponding to the contacts.
/// The first column of each matrix should give the contact normal,
/// while the second column gives a contact tangent (specifically, the
/// tangent direction used to determine `problem_data.F`). All
/// vectors should be expressed in the global frame.
/// @param[out] contact_forces a non-null vector of a doublet of values,
/// where the iᵗʰ element represents the force along
/// each basis vector in the iᵗʰ contact frame.
/// @throws std::exception if `contact_forces` is null, if
/// `contact_forces` is not empty, if `cf` is not the
/// proper size, if the number of tangent directions is not one per
/// contact (indicating that the contact problem might not be 2D), if
/// the number of contact frames is not equal to the number
/// of contacts, or if a contact frame does not appear to be
/// orthonormal.
/// @note On return, the contact force at the iᵗʰ contact point expressed
/// in the world frame is `contact_frames[i]` * `contact_forces[i]`.
static void CalcContactForcesInContactFrames(
const VectorX<T>& cf, const ConstraintVelProblemData<T>& problem_data,
const std::vector<Matrix2<T>>& contact_frames,
std::vector<Vector2<T>>* contact_forces);
private:
static void PopulatePackedConstraintForcesFromLcpSolution(
const ConstraintVelProblemData<T>& problem_data,
const MlcpToLcpData& mlcp_to_lcp_data, const VectorX<T>& zz,
const VectorX<T>& a, VectorX<T>* cf);
static void ConstructLinearEquationSolversForMlcp(
const ConstraintVelProblemData<T>& problem_data,
MlcpToLcpData* mlcp_to_lcp_data);
void FormAndSolveConstraintLcp(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, VectorX<T>* cf) const;
void FormAndSolveConstraintLinearSystem(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, VectorX<T>* cf) const;
static void CheckAccelConstraintMatrix(
const ConstraintAccelProblemData<T>& problem_data, const MatrixX<T>& MM);
static void CheckVelConstraintMatrix(
const ConstraintVelProblemData<T>& problem_data, const MatrixX<T>& MM);
// Computes a constraint space compliance matrix A⋅M⁻¹⋅Bᵀ, where A ∈ ℝᵃˣᵐ
// (realized here using an operator) and B ∈ ℝᵇˣᵐ are both Jacobian matrices
// and M⁻¹ ∈ ℝᵐˣᵐ is the inverse of the generalized inertia matrix. Note that
// mixing types of constraints is explicitly allowed. Aborts if A_iM_BT is
// not of size a × b.
static void ComputeConstraintSpaceComplianceMatrix(
std::function<VectorX<T>(const VectorX<T>&)> A_mult, int a,
const MatrixX<T>& M_inv_BT, Eigen::Ref<MatrixX<T>>);
// Computes the matrix M⁻¹⋅Gᵀ, G ∈ ℝᵐˣⁿ is a constraint Jacobian matrix
// (realized here using an operator) and M⁻¹ ∈ ℝⁿˣⁿ is the inverse of the
// generalized inertia matrix. Resizes iM_GT as necessary.
static void ComputeInverseInertiaTimesGT(
std::function<MatrixX<T>(const MatrixX<T>&)> M_inv_mult,
std::function<VectorX<T>(const VectorX<T>&)> G_transpose_mult, int m,
MatrixX<T>* iM_GT);
static void FormImpactingConstraintLcp(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& invA_a,
MatrixX<T>* MM, VectorX<T>* qq);
static void FormSustainedConstraintLcp(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& invA_a, MatrixX<T>* MM, VectorX<T>* qq);
static void FormSustainedConstraintLinearSystem(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& invA_a, MatrixX<T>* MM, VectorX<T>* qq);
template <typename ProblemData>
static void DetermineNewPartialInertiaSolveOperator(
const ProblemData* problem_data, int num_generalized_velocities,
const Eigen::CompleteOrthogonalDecomposition<MatrixX<T>>* delassus_QTZ,
std::function<MatrixX<T>(const MatrixX<T>&)>* A_solve);
template <typename ProblemData>
static void DetermineNewFullInertiaSolveOperator(
const ProblemData* problem_data, int num_generalized_velocities,
const Eigen::CompleteOrthogonalDecomposition<MatrixX<T>>* delassus_QTZ,
std::function<MatrixX<T>(const MatrixX<T>&)>* A_solve);
template <typename ProblemData>
static ProblemData* UpdateProblemDataForUnilateralConstraints(
const ProblemData& problem_data,
std::function<const MatrixX<T>(const MatrixX<T>&)> modified_inertia_solve,
int gv_dim, ProblemData* modified_problem_data);
drake::solvers::MobyLCPSolver<T> lcp_;
};
// Given a matrix A of blocks consisting of generalized inertia (M) and the
// Jacobian of bilaterals constraints (G):
// A ≡ | M -Gᵀ |
// | G 0 |
// this function sets a function pointer that computes X for X = A⁻¹ | B | and
// | 0 |
// given B for the case where X will be premultiplied by some matrix | R 0 |,
// where R is an arbitrary matrix. This odd operation is relatively common, and
// optimizing for this case allows us to skip some expensive matrix arithmetic.
// @param num_generalized_velocities The dimension of the system generalized
// velocities.
// @param problem_data The constraint problem data.
// @param delassus_QTZ The factorization of the Delassus Matrix GM⁻¹Gᵀ,
// where G is the constraint Jacobian corresponding to the
// independent constraints.
// @param[out] A_solve The operator for solving AX = B, on return.
template <typename T>
template <typename ProblemData>
void ConstraintSolver<T>::DetermineNewPartialInertiaSolveOperator(
const ProblemData* problem_data, int num_generalized_velocities,
const Eigen::CompleteOrthogonalDecomposition<MatrixX<T>>* delassus_QTZ,
std::function<MatrixX<T>(const MatrixX<T>&)>* A_solve) {
const int num_eq_constraints = problem_data->kG.size();
*A_solve = [problem_data, delassus_QTZ, num_eq_constraints,
num_generalized_velocities](const MatrixX<T>& X) -> MatrixX<T> {
// ************************************************************************
// See DetermineNewFullInertiaSolveOperator() for block inversion formula.
// ************************************************************************
// Set the result matrix.
const int C_rows = num_generalized_velocities;
const int E_cols = num_eq_constraints;
MatrixX<T> result(C_rows + E_cols, X.cols());
// Begin computation of components of C (upper left hand block of inverse
// of A): compute M⁻¹ X
const MatrixX<T> iM_X = problem_data->solve_inertia(X);
// Compute G M⁻¹ X
MatrixX<T> G_iM_X(E_cols, X.cols());
for (int i = 0; i < X.cols(); ++i)
G_iM_X.col(i) = problem_data->G_mult(iM_X.col(i));
// Compute (GM⁻¹Gᵀ)⁻¹GM⁻¹X
const MatrixX<T> Del_G_iM_X = delassus_QTZ->solve(G_iM_X);
// Compute Gᵀ(GM⁻¹Gᵀ)⁻¹GM⁻¹X
const MatrixX<T> GT_Del_G_iM_X = problem_data->G_transpose_mult(Del_G_iM_X);
// Compute M⁻¹Gᵀ(GM⁻¹Gᵀ)⁻¹GM⁻¹X
const MatrixX<T> iM_GT_Del_G_iM_X =
problem_data->solve_inertia(GT_Del_G_iM_X);
// Compute the result
result = iM_X - iM_GT_Del_G_iM_X;
return result;
};
}
// Given a matrix A of blocks consisting of generalized inertia (M) and the
// Jacobian of bilaterals constraints (G):
// A ≡ | M -Gᵀ |
// | G 0 |
// this function sets a function pointer that computes X for AX = B, given B.
// @param num_generalized_velocities The dimension of the system generalized
// velocities.
// @param problem_data The constraint problem data.
// @param delassus_QTZ The factorization of the Delassus Matrix GM⁻¹Gᵀ,
// where G is the constraint Jacobian corresponding to the
// independent constraints.
// @param[out] A_solve The operator for solving AX = B, on return.
template <typename T>
template <typename ProblemData>
void ConstraintSolver<T>::DetermineNewFullInertiaSolveOperator(
const ProblemData* problem_data, int num_generalized_velocities,
const Eigen::CompleteOrthogonalDecomposition<MatrixX<T>>* delassus_QTZ,
std::function<MatrixX<T>(const MatrixX<T>&)>* A_solve) {
// Get the number of equality constraints.
const int num_eq_constraints = problem_data->kG.size();
*A_solve = [problem_data, delassus_QTZ, num_eq_constraints,
num_generalized_velocities](const MatrixX<T>& B) -> MatrixX<T> {
// From a block matrix inversion,
// | M -Gᵀ |⁻¹ | Y | = | C E || Y | = | CY + EZ |
// | G 0 | | Z | | -Eᵀ F || Z | | -EᵀY + FZ |
// where E ≡ M⁻¹Gᵀ(GM⁻¹Gᵀ)⁻¹
// C ≡ M⁻¹ - M⁻¹Gᵀ(GM⁻¹Gᵀ)⁻¹GM⁻¹ = M⁻¹ - M⁻¹GᵀE
// -Eᵀ ≡ -(GM⁻¹Gᵀ)⁻¹GM⁻¹
// F ≡ (GM⁻¹Gᵀ)⁻¹
// B ≡ | Y |
// | Z |
// Set the result matrix (X).
const int C_rows = num_generalized_velocities;
const int E_cols = num_eq_constraints;
MatrixX<T> X(C_rows + E_cols, B.cols());
// Name the blocks of B and X.
const auto Y = B.topRows(C_rows);
const auto Z = B.bottomRows(B.rows() - C_rows);
auto X_top = X.topRows(C_rows);
auto X_bot = X.bottomRows(X.rows() - C_rows);
// 1. Begin computation of components of C.
// Compute M⁻¹ Y
const MatrixX<T> iM_Y = problem_data->solve_inertia(Y);
// Compute G M⁻¹ Y
MatrixX<T> G_iM_Y(E_cols, Y.cols());
for (int i = 0; i < Y.cols(); ++i)
G_iM_Y.col(i) = problem_data->G_mult(iM_Y.col(i));
// Compute (GM⁻¹Gᵀ)⁻¹GM⁻¹Y
const MatrixX<T> Del_G_iM_Y = delassus_QTZ->solve(G_iM_Y);
// Compute Gᵀ(GM⁻¹Gᵀ)⁻¹GM⁻¹Y
const MatrixX<T> GT_Del_G_iM_Y = problem_data->G_transpose_mult(Del_G_iM_Y);
// Compute M⁻¹Gᵀ(GM⁻¹Gᵀ)⁻¹GM⁻¹Y
const MatrixX<T> iM_GT_Del_G_iM_Y =
problem_data->solve_inertia(GT_Del_G_iM_Y);
// 2. Begin computation of components of E
// Compute (GM⁻¹Gᵀ)⁻¹Z
const MatrixX<T> Del_Z = delassus_QTZ->solve(Z);
// Compute Gᵀ(GM⁻¹Gᵀ)⁻¹Z
const MatrixX<T> GT_Del_Z = problem_data->G_transpose_mult(Del_Z);
// Compute M⁻¹Gᵀ(GM⁻¹Gᵀ)⁻¹Z = EZ
const MatrixX<T> iM_GT_Del_Z = problem_data->solve_inertia(GT_Del_Z);
// Set the top block of the result.
X_top = iM_Y - iM_GT_Del_G_iM_Y + iM_GT_Del_Z;
// Set the bottom block of the result.
X_bot = delassus_QTZ->solve(Z) - Del_G_iM_Y;
return X;
};
}
template <typename T>
template <typename ProblemData>
ProblemData* ConstraintSolver<T>::UpdateProblemDataForUnilateralConstraints(
const ProblemData& problem_data,
std::function<const MatrixX<T>(const MatrixX<T>&)> modified_inertia_solve,
int gv_dim, ProblemData* modified_problem_data) {
// Verify that the modified problem data points to something.
DRAKE_DEMAND(modified_problem_data != nullptr);
// Get the number of equality constraints.
const int num_eq_constraints = problem_data.kG.size();
// Construct a new problem data.
if (num_eq_constraints == 0) {
// Just point to the original problem data.
return const_cast<ProblemData*>(&problem_data);
} else {
// Alias the modified problem data so that we don't need to change its
// pointer.
ProblemData& new_data = *modified_problem_data;
// Copy most of the data unchanged.
new_data = problem_data;
// Construct zero functions.
auto zero_fn = [](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>(0);
};
auto zero_gv_dim_fn = [gv_dim](const VectorX<T>&) -> VectorX<T> {
return VectorX<T>::Zero(gv_dim);
};
// Remove the bilateral constraints.
new_data.kG.resize(0);
new_data.G_mult = zero_fn;
new_data.G_transpose_mult = zero_gv_dim_fn;
// Update the inertia function pointer.
new_data.solve_inertia = modified_inertia_solve;
return &new_data;
}
}
// Forms and solves the system of linear equations used to compute the
// accelerations during ODE evaluations, as an alternative to the linear
// complementarity problem formulation. If all constraints are known to be
// active, this method will provide the correct solution rapidly.
// @param problem_data The constraint data formulated at the acceleration
// level.
// @param trunc_neg_invA_a The first ngc elements of -A⁻¹a, where
// A ≡ | M Gᵀ | (M is the generalized inertia matrix and G is the
// | G 0 | Jacobian matrix for the bilateral constraints)
// and a ≡ | -τ | (τ [tau] and kG are defined in `problem_data`).
// | kG |
// @param[out] cf The unilateral constraint forces, on return, in a packed
// storage format. The first `nc` elements of `cf` correspond to
// the magnitudes of the contact forces applied along the normals
// of the `nc` contact points. The next elements of `cf`
// correspond to the frictional forces along the `r` spanning
// directions at each non-sliding point of contact. The first `r`
// values (after the initial `nc` elements) correspond to the
// first non-sliding contact, the next `r` values correspond to
// the second non-sliding contact, etc. The next `ℓ` values of
// `cf` correspond to the forces applied to enforce generic
// unilateral constraints. `cf` will be resized as necessary.
template <class T>
void ConstraintSolver<T>::FormAndSolveConstraintLinearSystem(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, VectorX<T>* cf) const {
using std::abs;
using std::max;
DRAKE_DEMAND(cf != nullptr);
// Alias problem data.
const std::vector<int>& sliding_contacts = problem_data.sliding_contacts;
const std::vector<int>& non_sliding_contacts =
problem_data.non_sliding_contacts;
// Get numbers of friction directions and types of contacts.
const int num_sliding = sliding_contacts.size();
const int num_non_sliding = non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
// Initialize contact force vector.
cf->resize(num_contacts + num_spanning_vectors + num_limits +
num_eq_constraints);
// Using Equations (f) and (g) from the comments in
// FormAndSolveConstraintLcp() and defining C as the upper left block of A⁻¹,
// the linear system is defined as MM*z + qq = 0, where:
//
// MM ≡ | NC(Nᵀ-μQᵀ) NCDᵀ NCLᵀ |
// | DC(Nᵀ-μQᵀ) DCDᵀ DCLᵀ |
// | LC(Nᵀ-μQᵀ) LCDᵀ LCLᵀ |
//
// qq ≡ | kᴺ + |N 0|A⁻¹a |
// | kᴰ + |D 0|A⁻¹a |
// | kᴸ + |L 0|A⁻¹a |
// @TODO(edrumwri): Consider checking whether or not the constraints are
// satisfied to a user-specified tolerance; a set of constraint equations that
// are dependent upon time (e.g., prescribed motion constraints) might not be
// fully satisfiable.
// Set up the linear system.
MatrixX<T> MM;
VectorX<T> qq;
FormSustainedConstraintLinearSystem(problem_data, trunc_neg_invA_a, &MM, &qq);
// Solve the linear system.
Eigen::CompleteOrthogonalDecomposition<MatrixX<T>> MM_QTZ(MM);
cf->head(qq.size()) = MM_QTZ.solve(-qq);
}
// Forms and solves the linear complementarity problem used to compute the
// accelerations during ODE evaluations, as an alternative to the linear
// system problem formulation. In contrast to
// FormAndSolveConstraintLinearSystem(), this approach does not require the
// active constraints to be known a priori. Significantly more computation is
// required, however.
// @sa FormAndSolveConstraintLinearSystem for descriptions of parameters.
template <typename T>
void ConstraintSolver<T>::FormAndSolveConstraintLcp(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, VectorX<T>* cf) const {
using std::abs;
using std::max;
DRAKE_DEMAND(cf != nullptr);
// Alias problem data.
const std::vector<int>& sliding_contacts = problem_data.sliding_contacts;
const std::vector<int>& non_sliding_contacts =
problem_data.non_sliding_contacts;
// Get numbers of friction directions and types of contacts.
const int num_sliding = sliding_contacts.size();
const int num_non_sliding = non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int nk = num_spanning_vectors * 2;
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
// Initialize contact force vector.
cf->resize(num_contacts + num_spanning_vectors + num_limits +
num_eq_constraints);
// The constraint problem is a mixed linear complementarity problem of the
// form:
// (a) Au + Xv + a = 0
// (b) Yu + Bv + b ≥ 0
// (c) v ≥ 0
// (d) vᵀ(b + Yu + Bv) = 0
// where u are "free" variables. If the matrix A is nonsingular, u can be
// solved for:
// (e) u = -A⁻¹ (a + Xv)
// allowing the mixed LCP to be converted to a "pure" LCP (qq, MM) by:
// (f) qq = b - YA⁻¹a
// (g) MM = B - YA⁻¹X
// Our mixed linear complementarity problem takes the specific form:
// (1) | M -Gᵀ -(Nᵀ-μQᵀ) -Dᵀ 0 -Lᵀ | | v̇ | + | -f | = | 0 |
// | G 0 0 0 0 0 | | fG | + | kᴳ | = | 0 |
// | N 0 0 0 0 0 | | fN | + | kᴺ | = | α |
// | D 0 0 0 E 0 | | fD | + | kᴰ | = | β |
// | 0 0 μ -Eᵀ 0 0 | | λ | + | 0 | = | γ |
// | L 0 0 0 0 0 | | fL | + | kᴸ | = | δ |
// (2) 0 ≤ fN ⊥ α ≥ 0
// (3) 0 ≤ fD ⊥ β ≥ 0
// (4) 0 ≤ λ ⊥ γ ≥ 0
// (5) 0 ≤ fL ⊥ δ ≥ 0
// --------------------------------------------------------------------------
// Converting the MLCP to a pure LCP:
// --------------------------------------------------------------------------
// From the notation above in Equations (a)-(d):
// A ≡ | M -Gᵀ| a ≡ | -f | X ≡ |-(Nᵀ-μQᵀ) -Dᵀ 0 -Lᵀ |
// | G 0 | | kᴳ | | 0 0 0 0 |
//
// Y ≡ | N 0 | b ≡ | kᴺ | B ≡ | 0 0 0 0 |
// | D 0 | | kᴰ | | 0 0 E 0 |
// | 0 0 | | 0 | | μ -Eᵀ 0 0 |
// | L 0 | | kᴸ | | 0 0 0 0 |
//
// u ≡ | v̇ | v ≡ | fN |
// | fG | | fD |
// | λ |
// | fL |
//
// Therefore, using Equations (f) and (g) and defining C as the upper left
// block of A⁻¹, the pure LCP (qq,MM) is defined as:
//
// MM ≡ | NC(Nᵀ-μQᵀ) NCDᵀ 0 NCLᵀ |
// | DC(Nᵀ-μQᵀ) DCDᵀ E DCLᵀ |
// | μ -Eᵀ 0 0 |
// | LC(Nᵀ-μQᵀ) LCDᵀ 0 LCLᵀ |
//
// qq ≡ | kᴺ + |N 0|A⁻¹a |
// | kᴰ + |D 0|A⁻¹a |
// | 0 |
// | kᴸ + |L 0|A⁻¹a |
//
// --------------------------------------------------------------------------
// Using the LCP solution to solve the MLCP.
// --------------------------------------------------------------------------
// From Equation (e) and the solution to the LCP (v), we can solve for u using
// the following equations:
// Xv + a = | -(Nᵀ-μQᵀ)fN - DᵀfD - LᵀfL - f |
// | kᴳ |
//
// TODO(edrumwri): Consider checking whether or not the constraints are
// satisfied to a user-specified tolerance; a set of constraint equations that
// are dependent upon time (e.g., prescribed motion constraints) might not be
// fully satisfiable.
// Set up the pure linear complementarity problem.
MatrixX<T> MM;
VectorX<T> qq;
FormSustainedConstraintLcp(problem_data, trunc_neg_invA_a, &MM, &qq);
// Get the zero tolerance for solving the LCP.
const T zero_tol = lcp_.ComputeZeroTolerance(MM);
// Solve the LCP and compute the values of the slack variables.
VectorX<T> zz;
bool success = lcp_.SolveLcpLemke(MM, qq, &zz, -1, zero_tol);
VectorX<T> ww = MM * zz + qq;
const double max_dot =
(zz.size() > 0) ? (zz.array() * ww.array()).abs().maxCoeff() : 0.0;
// NOTE: This LCP might not be solvable due to inconsistent configurations.
// Check the answer and throw a runtime error if it's no good.
// LCP constraints are zz ≥ 0, ww ≥ 0, zzᵀww = 0. Since the zero tolerance
// is used to check a single element for zero (within a single pivoting
// operation), we must compensate for the number of pivoting operations and
// the problem size. zzᵀww must use a looser tolerance to account for the
// num_vars multiplies.
const int num_vars = qq.size();
const int npivots = std::max(1, lcp_.get_num_pivots());
if (!success ||
(zz.size() > 0 &&
(zz.minCoeff() < -num_vars * npivots * zero_tol ||
ww.minCoeff() < -num_vars * npivots * zero_tol ||
max_dot > max(T(1), zz.maxCoeff()) * max(T(1), ww.maxCoeff()) *
num_vars * npivots * zero_tol))) {
throw std::runtime_error("Unable to solve LCP- it may be unsolvable.");
}
// Alias constraint force segments.
const auto fN = zz.segment(0, num_contacts);
const auto fD_plus = zz.segment(num_contacts, num_spanning_vectors);
const auto fD_minus =
zz.segment(num_contacts + num_spanning_vectors, num_spanning_vectors);
const auto fL = zz.segment(num_contacts + num_non_sliding + nk, num_limits);
// Get the constraint forces in the specified packed storage format.
cf->segment(0, num_contacts) = fN;
cf->segment(num_contacts, num_spanning_vectors) = fD_plus - fD_minus;
cf->segment(num_contacts + num_spanning_vectors, num_limits) = fL;
}
template <typename T>
void ConstraintSolver<T>::SolveConstraintProblem(
const ConstraintAccelProblemData<T>& problem_data, VectorX<T>* cf) const {
using std::abs;
using std::max;
if (!cf) throw std::logic_error("cf (output parameter) is null.");
// Alias problem data.
const std::vector<int>& sliding_contacts = problem_data.sliding_contacts;
const std::vector<int>& non_sliding_contacts =
problem_data.non_sliding_contacts;
// Get numbers of friction directions, types of contacts, and other data.
const int num_sliding = sliding_contacts.size();
const int num_non_sliding = non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
const int num_generalized_velocities = problem_data.tau.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
// Look for fast exit.
if (num_contacts == 0 && num_limits == 0 && num_eq_constraints == 0) {
cf->resize(0);
return;
}
// Look for a second fast exit. (We avoid this calculation if there are
// bilateral constraints because it's too hard to determine a workable
// tolerance at this point).
const VectorX<T> candidate_accel =
problem_data.solve_inertia(problem_data.tau);
const VectorX<T> N_eval =
problem_data.N_mult(candidate_accel) + problem_data.kN;
const VectorX<T> L_eval =
problem_data.L_mult(candidate_accel) + problem_data.kL;
if ((num_contacts == 0 || N_eval.minCoeff() >= 0) &&
(num_limits == 0 || L_eval.minCoeff() >= 0) &&
(num_eq_constraints == 0)) {
cf->setZero();
return;
}
// Prepare to set up the functionals to compute Ax = b, where A is the
// blocked saddle point matrix containing the generalized inertia matrix
// and the bilateral constraints *assuming there are bilateral constraints*.
// If there are no bilateral constraints, A_solve and fast_A_solve will
// simply point to the inertia solve operator.
MlcpToLcpData mlcp_to_lcp_data;
if (num_eq_constraints > 0) {
// Form the Delassus matrix for the bilateral constraints.
MatrixX<T> Del(num_eq_constraints, num_eq_constraints);
MatrixX<T> iM_GT(num_generalized_velocities, num_eq_constraints);
ComputeInverseInertiaTimesGT(problem_data.solve_inertia,
problem_data.G_transpose_mult,
num_eq_constraints, &iM_GT);
ComputeConstraintSpaceComplianceMatrix(problem_data.G_mult,
num_eq_constraints, iM_GT, Del);
// Compute the complete orthogonal factorization.
mlcp_to_lcp_data.delassus_QTZ.compute(Del);
// Determine a new "inertia" solve operator, which solves AX = B, where
// A = | M -Gᵀ |
// | G 0 |
// using a least-squares solution to accommodate rank-deficiency in G. This
// will allow transforming the mixed LCP into a pure LCP.
DetermineNewFullInertiaSolveOperator(
&problem_data, num_generalized_velocities,
&mlcp_to_lcp_data.delassus_QTZ, &mlcp_to_lcp_data.A_solve);
// Determine a new "inertia" solve operator, using only the upper left block
// of A⁻¹ (denoted C) to exploit zero blocks in common operations.
DetermineNewPartialInertiaSolveOperator(
&problem_data, num_generalized_velocities,
&mlcp_to_lcp_data.delassus_QTZ, &mlcp_to_lcp_data.fast_A_solve);
} else {
mlcp_to_lcp_data.A_solve = problem_data.solve_inertia;
mlcp_to_lcp_data.fast_A_solve = problem_data.solve_inertia;
}
// Copy the problem data and then update it to account for bilateral
// constraints.
const int gv_dim = problem_data.tau.size();
ConstraintAccelProblemData<T> modified_problem_data(gv_dim +
num_eq_constraints);
ConstraintAccelProblemData<T>* data_ptr = &modified_problem_data;
data_ptr = UpdateProblemDataForUnilateralConstraints(
problem_data, mlcp_to_lcp_data.fast_A_solve, gv_dim, data_ptr);
// Compute a and A⁻¹a.
VectorX<T> a(problem_data.tau.size() + num_eq_constraints);
a.head(problem_data.tau.size()) = -problem_data.tau;
a.tail(num_eq_constraints) = problem_data.kG;
const VectorX<T> invA_a = mlcp_to_lcp_data.A_solve(a);
const VectorX<T> trunc_neg_invA_a = -invA_a.head(problem_data.tau.size());
// Determine which problem formulation to use.
if (problem_data.use_complementarity_problem_solver) {
FormAndSolveConstraintLcp(problem_data, trunc_neg_invA_a, cf);
} else {
FormAndSolveConstraintLinearSystem(problem_data, trunc_neg_invA_a, cf);
}
// Alias constraint force segments.
const auto fN = cf->segment(0, num_contacts);
const auto fF = cf->segment(num_contacts, num_spanning_vectors);
const auto fL = cf->segment(num_contacts + num_spanning_vectors, num_limits);
// Determine the accelerations and the bilateral constraint forces.
// Au + Xv + a = 0
// Yu + Bv + b ≥ 0
// v ≥ 0
// vᵀ(b + Yu + Bv) = 0
// where u are "free" variables (corresponding to the time derivative of
// velocities concatenated with bilateral constraint forces). If the matrix A
// is nonsingular, u can be solved for:
// u = -A⁻¹ (a + Xv)
// allowing the mixed LCP to be converted to a "pure" LCP (q, M) by:
// q = b - DA⁻¹a
// M = B - DA⁻¹C
if (num_eq_constraints > 0) {
// In this case, Xv = -(Nᵀ + μQᵀ)fN - DᵀfD - LᵀfL and a = | -f |.
// | kG |
const VectorX<T> Xv = -data_ptr->N_minus_muQ_transpose_mult(fN) -
data_ptr->F_transpose_mult(fF) -
data_ptr->L_transpose_mult(fL);
VectorX<T> aug = a;
aug.head(Xv.size()) += Xv;
const VectorX<T> u = -mlcp_to_lcp_data.A_solve(aug);
auto lambda = cf->segment(num_contacts + num_spanning_vectors + num_limits,
num_eq_constraints);
lambda = u.tail(num_eq_constraints);
}
}
template <typename T>
void ConstraintSolver<T>::SolveImpactProblem(
const ConstraintVelProblemData<T>& problem_data, VectorX<T>* cf) const {
using std::abs;
using std::max;
if (!cf) throw std::logic_error("cf (output parameter) is null.");
// Get number of contacts and limits.
const int num_contacts = problem_data.mu.size();
if (static_cast<size_t>(num_contacts) != problem_data.r.size()) {
throw std::logic_error(
"Number of elements in 'r' does not match number"
"of elements in 'mu'");
}
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
// Look for fast exit.
if (num_contacts == 0 && num_limits == 0 && num_eq_constraints == 0) {
cf->resize(0);
return;
}
// Get number of tangent spanning vectors.
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
// Determine the pre-impact velocity.
const VectorX<T> v = problem_data.solve_inertia(problem_data.Mv);
// If no impact and no bilateral constraints, do not apply the impact model.
// (We avoid this calculation if there are bilateral constraints because it's
// too hard to determine a workable tolerance at this point).
const VectorX<T> N_eval = problem_data.N_mult(v) + problem_data.kN;
const VectorX<T> L_eval = problem_data.L_mult(v) + problem_data.kL;
if ((num_contacts == 0 || N_eval.minCoeff() >= 0) &&
(num_limits == 0 || L_eval.minCoeff() >= 0) &&
(num_eq_constraints == 0)) {
cf->setZero(num_contacts + num_spanning_vectors + num_limits);
return;
}
// Initialize contact force vector.
cf->resize(num_contacts + num_spanning_vectors + num_limits +
num_eq_constraints);
// Construct the operators required to "factor out" the bilateral constraints
// through conversion of a mixed linear complementarity problem into a "pure"
// linear complementarity problem. See
// ConstructLinearEquationSolversForMlcp() for more information.
MlcpToLcpData mlcp_to_lcp_data;
ConstructLinearEquationSolversForMlcp(problem_data, &mlcp_to_lcp_data);
// Copy the problem data and then update it to account for bilateral
// constraints.
const int gv_dim = problem_data.Mv.size();
ConstraintVelProblemData<T> modified_problem_data(gv_dim +
num_eq_constraints);
ConstraintVelProblemData<T>* data_ptr = &modified_problem_data;
data_ptr = UpdateProblemDataForUnilateralConstraints(
problem_data, mlcp_to_lcp_data.fast_A_solve, gv_dim, data_ptr);
// Compute a and A⁻¹a.
const VectorX<T>& Mv = problem_data.Mv;
VectorX<T> a(Mv.size() + num_eq_constraints);
a.head(Mv.size()) = -Mv;
a.tail(num_eq_constraints) = problem_data.kG;
const VectorX<T> invA_a = mlcp_to_lcp_data.A_solve(a);
const VectorX<T> trunc_neg_invA_a = -invA_a.head(Mv.size());
// Construct the linear complementarity problem.
MatrixX<T> MM;
VectorX<T> qq;
FormImpactingConstraintLcp(problem_data, trunc_neg_invA_a, &MM, &qq);
// Get the tolerance for zero used by the LCP solver.
const T zero_tol = lcp_.ComputeZeroTolerance(MM);
// Solve the LCP and compute the values of the slack variables.
VectorX<T> zz;
bool success = lcp_.SolveLcpLemke(MM, qq, &zz, -1, zero_tol);
VectorX<T> ww = MM * zz + qq;
const T max_dot =
(zz.size() > 0) ? (zz.array() * ww.array()).abs().maxCoeff() : 0.0;
// Check the answer and solve using progressive regularization if necessary.
const int num_vars = qq.size();
const int npivots = std::max(lcp_.get_num_pivots(), 1);
if (!success ||
(zz.size() > 0 &&
(zz.minCoeff() < -num_vars * npivots * zero_tol ||
ww.minCoeff() < -num_vars * npivots * zero_tol ||
max_dot > max(T(1), zz.maxCoeff()) * max(T(1), ww.maxCoeff()) *
num_vars * npivots * zero_tol))) {
// Report difficulty
DRAKE_LOGGER_DEBUG(
"Unable to solve impacting problem LCP without "
"progressive regularization");
DRAKE_LOGGER_DEBUG("zero tolerance for z/w: {}",
num_vars * npivots * zero_tol);
DRAKE_LOGGER_DEBUG("Solver reports success? {}", success);
DRAKE_LOGGER_DEBUG("minimum z: {}", zz.minCoeff());
DRAKE_LOGGER_DEBUG("minimum w: {}", ww.minCoeff());
DRAKE_LOGGER_DEBUG("zero tolerance for <z,w>: {}",
max(T(1), zz.maxCoeff()) * max(T(1), ww.maxCoeff()) *
num_vars * npivots * zero_tol);
DRAKE_LOGGER_DEBUG("z'w: {}", max_dot);
// Use progressive regularization to solve.
const int min_exp = -16; // Minimum regularization factor: 1e-16.
const unsigned step_exp = 1; // Regularization progressively increases by a
// factor of ten.
const int max_exp = 1; // Maximum regularization: 1e1.
const double piv_tol = -1; // Make solver compute the pivot tolerance.
if (!lcp_.SolveLcpLemkeRegularized(MM, qq, &zz, min_exp, step_exp, max_exp,
piv_tol, zero_tol)) {
throw std::runtime_error("Progressively regularized LCP solve failed.");
} else {
ww = MM * zz + qq;
DRAKE_LOGGER_DEBUG("minimum z: {}", zz.minCoeff());
DRAKE_LOGGER_DEBUG("minimum w: {}", ww.minCoeff());
DRAKE_LOGGER_DEBUG("z'w: ", (zz.array() * ww.array()).abs().maxCoeff());
}
}
// Construct the packed force vector.
PopulatePackedConstraintForcesFromLcpSolution(problem_data, mlcp_to_lcp_data,
zz, a, cf);
}
template <typename T>
void ConstraintSolver<T>::ConstructLinearEquationSolversForMlcp(
const ConstraintVelProblemData<T>& problem_data,
MlcpToLcpData* mlcp_to_lcp_data) {
// --------------------------------------------------------------------------
// Using the LCP solution to solve the MLCP.
// --------------------------------------------------------------------------
// From Equation (e) and the solution to the LCP (v), we can solve for u using
// the following equations:
// Xv + a = | -NᵀfN - DᵀfD - LᵀfL - Mv |
// | kᴳ |
//
// Prepare to set up the functionals to compute Ax = b, where A is the
// blocked saddle point matrix containing the generalized inertia matrix
// and the bilateral constraints *assuming there are bilateral constraints*.
// If there are no bilateral constraints, A_solve and fast_A_solve will
// simply point to the inertia solve operator.
// Form the Delassus matrix for the bilateral constraints.
const int num_generalized_velocities = problem_data.Mv.size();
const int num_eq_constraints = problem_data.kG.size();
if (num_eq_constraints > 0) {
MatrixX<T> Del(num_eq_constraints, num_eq_constraints);
MatrixX<T> iM_GT(num_generalized_velocities, num_eq_constraints);
ComputeInverseInertiaTimesGT(problem_data.solve_inertia,
problem_data.G_transpose_mult,
num_eq_constraints, &iM_GT);
ComputeConstraintSpaceComplianceMatrix(problem_data.G_mult,
num_eq_constraints, iM_GT, Del);
// Compute the complete orthogonal factorization.
mlcp_to_lcp_data->delassus_QTZ.compute(Del);
// Determine a new "inertia" solve operator, which solves AX = B, where
// A = | M -Gᵀ |
// | G 0 |
// using the newly reduced set of constraints. This will allow transforming
// the mixed LCP into a pure LCP.
DetermineNewFullInertiaSolveOperator(
&problem_data, num_generalized_velocities,
&mlcp_to_lcp_data->delassus_QTZ, &mlcp_to_lcp_data->A_solve);
// Determine a new "inertia" solve operator, using only the upper left block
// of A⁻¹ to exploit zeros in common operations.
DetermineNewPartialInertiaSolveOperator(
&problem_data, num_generalized_velocities,
&mlcp_to_lcp_data->delassus_QTZ, &mlcp_to_lcp_data->fast_A_solve);
} else {
mlcp_to_lcp_data->A_solve = problem_data.solve_inertia;
mlcp_to_lcp_data->fast_A_solve = problem_data.solve_inertia;
}
}
// Populates the packed constraint force vector from the solution to the
// linear complementarity problem (LCP).
// @param problem_data the constraint problem data.
// @param a reference to a MlcpToLcpData object.
// @param a the vector `a` output from UpdateDiscretizedTimeLcp().
// @param[out] cf the constraint forces, on return.
// @pre cf is non-null.
template <typename T>
void ConstraintSolver<T>::PopulatePackedConstraintForcesFromLcpSolution(
const ConstraintVelProblemData<T>& problem_data,
const MlcpToLcpData& mlcp_to_lcp_data, const VectorX<T>& zz,
const VectorX<T>& a, VectorX<T>* cf) {
PopulatePackedConstraintForcesFromLcpSolution(problem_data, mlcp_to_lcp_data,
zz, a, 1.0, cf);
}
template <typename T>
void ConstraintSolver<T>::PopulatePackedConstraintForcesFromLcpSolution(
const ConstraintVelProblemData<T>& problem_data,
const MlcpToLcpData& mlcp_to_lcp_data, const VectorX<T>& zz,
const VectorX<T>& a, double dt, VectorX<T>* cf) {
// Resize the force vector.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
cf->resize(num_contacts + num_spanning_vectors + num_limits +
num_eq_constraints);
// Quit early if zz is empty.
if (zz.size() == 0) {
cf->setZero();
if (num_eq_constraints > 0) {
const VectorX<T> u = -mlcp_to_lcp_data.A_solve(a);
auto lambda = cf->segment(
num_contacts + num_spanning_vectors + num_limits, num_eq_constraints);
// Transform the impulsive forces to non-impulsive forces.
lambda = u.tail(num_eq_constraints) / dt;
DRAKE_LOGGER_DEBUG("Bilateral constraint forces/impulses: {}",
fmt_eigen(lambda.transpose()));
}
return;
}
// Alias constraint force segments.
const auto fN = zz.segment(0, num_contacts);
const auto fD_plus = zz.segment(num_contacts, num_spanning_vectors);
const auto fD_minus =
zz.segment(num_contacts + num_spanning_vectors, num_spanning_vectors);
const auto fL =
zz.segment(num_contacts * 2 + num_spanning_vectors * 2, num_limits);
const auto fF = cf->segment(num_contacts, num_spanning_vectors);
// Get the constraint forces in the specified packed storage format.
cf->segment(0, num_contacts) = fN;
cf->segment(num_contacts, num_spanning_vectors) = fD_plus - fD_minus;
cf->segment(num_contacts + num_spanning_vectors, num_limits) = fL;
DRAKE_LOGGER_DEBUG("Normal contact forces/impulses: {}",
fmt_eigen(fN.transpose()));
DRAKE_LOGGER_DEBUG("Frictional contact forces/impulses: {}",
fmt_eigen((fD_plus - fD_minus).transpose()));
DRAKE_LOGGER_DEBUG(
"Generic unilateral constraint "
"forces/impulses: {}",
fmt_eigen(fL.transpose()));
// Determine the new velocity and the bilateral constraint forces/
// impulses.
// Au + Xv + a = 0
// Yu + Bv + b ≥ 0
// v ≥ 0
// vᵀ(b + Yu + Bv) = 0
// where u are "free" variables (corresponding to new velocities
// concatenated with bilateral constraint forces/impulses). If
// the matrix A is nonsingular, u can be solved for:
// u = -A⁻¹ (a + Xv)
// allowing the mixed LCP to be converted to a "pure" LCP (q, M) by:
// q = b - DA⁻¹a
// M = B - DA⁻¹C
if (num_eq_constraints > 0) {
// In this case, Xv = -NᵀfN - DᵀfD -LᵀfL and a = | -Mv(t) |.
// | kG |
// First, make the forces impulsive.
const VectorX<T> Xv = (-problem_data.N_transpose_mult(fN) -
problem_data.F_transpose_mult(fF) -
problem_data.L_transpose_mult(fL)) *
dt;
VectorX<T> aug = a;
aug.head(Xv.size()) += Xv;
const VectorX<T> u = -mlcp_to_lcp_data.A_solve(aug);
auto lambda = cf->segment(num_contacts + num_spanning_vectors + num_limits,
num_eq_constraints);
// Transform the impulsive forces back to non-impulsive forces.
lambda = u.tail(num_eq_constraints) / dt;
DRAKE_LOGGER_DEBUG("Bilateral constraint forces/impulses: {}",
fmt_eigen(lambda.transpose()));
}
}
template <typename T>
void ConstraintSolver<T>::UpdateDiscretizedTimeLcp(
const ConstraintVelProblemData<T>& problem_data, double dt,
MlcpToLcpData* mlcp_to_lcp_data, VectorX<T>* a, MatrixX<T>* MM,
VectorX<T>* qq) {
DRAKE_DEMAND(MM != nullptr);
DRAKE_DEMAND(qq != nullptr);
DRAKE_DEMAND(a != nullptr);
// Look for early exit.
if (qq->rows() == 0) return;
// Recompute the linear equation solvers, if necessary.
if (problem_data.kG.size() > 0) {
ConstructLinearEquationSolversForMlcp(problem_data, mlcp_to_lcp_data);
}
// Compute a and A⁻¹a.
const int num_eq_constraints = problem_data.kG.size();
const VectorX<T>& Mv = problem_data.Mv;
a->resize(Mv.size() + num_eq_constraints);
a->head(Mv.size()) = -Mv;
a->tail(num_eq_constraints) = problem_data.kG;
const VectorX<T> invA_a = mlcp_to_lcp_data->A_solve(*a);
const VectorX<T> trunc_neg_invA_a = -invA_a.head(Mv.size());
// Look for quick exit.
if (qq->rows() == 0) return;
// Get numbers of contacts.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Alias these variables for more readable construction of MM and qq.
const int nc = num_contacts;
const int nr = num_spanning_vectors;
const int nk = nr * 2;
const int nl = num_limits;
// Alias operators to make accessing them less clunky.
const auto N = problem_data.N_mult;
const auto F = problem_data.F_mult;
const auto L = problem_data.L_mult;
// Verify that all gamma vectors are either empty or non-negative.
const VectorX<T>& gammaN = problem_data.gammaN;
const VectorX<T>& gammaF = problem_data.gammaF;
const VectorX<T>& gammaE = problem_data.gammaE;
const VectorX<T>& gammaL = problem_data.gammaL;
DRAKE_DEMAND(gammaN.size() == 0 || gammaN.minCoeff() >= 0);
DRAKE_DEMAND(gammaF.size() == 0 || gammaF.minCoeff() >= 0);
DRAKE_DEMAND(gammaE.size() == 0 || gammaE.minCoeff() >= 0);
DRAKE_DEMAND(gammaL.size() == 0 || gammaL.minCoeff() >= 0);
// Scale the Delassus matrices, which are all but the third row (block) and
// third column (block) of the following matrix.
// N⋅M⁻¹⋅Nᵀ N⋅M⁻¹⋅Dᵀ 0 N⋅M⁻¹⋅Lᵀ
// D⋅M⁻¹⋅Nᵀ D⋅M⁻¹⋅Dᵀ E D⋅M⁻¹⋅Lᵀ
// μ -Eᵀ 0 0
// L⋅M⁻¹⋅Nᵀ L⋅M⁻¹⋅Dᵀ 0 L⋅M⁻¹⋅Lᵀ
// where D = | F |
// | -F |
const int nr2 = nr * 2;
MM->topLeftCorner(nc + nr2, nc + nr2) *= dt;
MM->bottomLeftCorner(nl, nc + nr2) *= dt;
MM->topRightCorner(nc + nr2, nl) *= dt;
MM->bottomRightCorner(nl, nl) *= dt;
// Regularize the LCP matrix.
MM->topLeftCorner(nc, nc) += Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaN);
MM->block(nc, nc, nr, nr) += Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaF);
MM->block(nc + nr, nc + nr, nr, nr) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaF);
MM->block(nc + nk, nc + nk, nc, nc) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaE);
MM->block(nc * 2 + nk, nc * 2 + nk, nl, nl) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaL);
// Update qq.
qq->segment(0, nc) = N(trunc_neg_invA_a) + problem_data.kN;
qq->segment(nc, nr) = F(trunc_neg_invA_a) + problem_data.kF;
qq->segment(nc + nr, nr) = -qq->segment(nc, nr);
qq->segment(nc + nk, nc).setZero();
qq->segment(nc * 2 + nk, num_limits) = L(trunc_neg_invA_a) + problem_data.kL;
}
template <typename T>
void ConstraintSolver<T>::ConstructBaseDiscretizedTimeLcp(
const ConstraintVelProblemData<T>& problem_data,
MlcpToLcpData* mlcp_to_lcp_data, MatrixX<T>* MM, VectorX<T>* qq) {
DRAKE_DEMAND(MM != nullptr);
DRAKE_DEMAND(qq != nullptr);
DRAKE_DEMAND(mlcp_to_lcp_data != nullptr);
// Get number of contacts and limits.
const int num_contacts = problem_data.mu.size();
if (static_cast<size_t>(num_contacts) != problem_data.r.size()) {
throw std::logic_error(
"Number of elements in 'r' does not match number"
"of elements in 'mu'");
}
const int num_limits = problem_data.kL.size();
const int num_eq_constraints = problem_data.kG.size();
// Look for fast exit.
if (num_contacts == 0 && num_limits == 0 && num_eq_constraints == 0) {
MM->resize(0, 0);
qq->resize(0);
return;
}
// If no impact and no bilateral constraints, construct an empty matrix
// and vector. (We avoid this possible shortcut if there are bilateral
// constraints because it's too hard to determine a workable tolerance at
// this point).
const VectorX<T> v = problem_data.solve_inertia(problem_data.Mv);
const VectorX<T> N_eval = problem_data.N_mult(v) + problem_data.kN;
const VectorX<T> L_eval = problem_data.L_mult(v) + problem_data.kL;
if ((num_contacts == 0 || N_eval.minCoeff() >= 0) &&
(num_limits == 0 || L_eval.minCoeff() >= 0) &&
(num_eq_constraints == 0)) {
MM->resize(0, 0);
qq->resize(0);
return;
}
// Determine the "A" and fast "A" solution operators, which allow us to
// solve the mixed linear complementarity problem by first solving a "pure"
// linear complementarity problem. See @ref Velocity-level-MLCPs in
// Doxygen documentation (above).
ConstructLinearEquationSolversForMlcp(problem_data, mlcp_to_lcp_data);
// Allocate storage for a.
VectorX<T> a(problem_data.Mv.size() + num_eq_constraints);
// Compute a and A⁻¹a.
const VectorX<T>& Mv = problem_data.Mv;
a.head(Mv.size()) = -Mv;
a.tail(num_eq_constraints) = problem_data.kG;
const VectorX<T> invA_a = mlcp_to_lcp_data->A_solve(a);
const VectorX<T> trunc_neg_invA_a = -invA_a.head(Mv.size());
// Set up the linear complementarity problem.
FormImpactingConstraintLcp(problem_data, trunc_neg_invA_a, MM, qq);
}
template <class T>
void ConstraintSolver<T>::ComputeConstraintSpaceComplianceMatrix(
std::function<VectorX<T>(const VectorX<T>&)> A_mult, int a,
const MatrixX<T>& iM_BT, Eigen::Ref<MatrixX<T>> A_iM_BT) {
const int b = iM_BT.cols();
DRAKE_DEMAND(A_iM_BT.rows() == a && A_iM_BT.cols() == b);
// Look for fast exit.
if (a == 0 || b == 0) return;
VectorX<T> iM_bT; // Intermediate result vector.
for (int i = 0; i < b; ++i) {
iM_bT = iM_BT.col(i);
A_iM_BT.col(i) = A_mult(iM_bT);
}
}
template <class T>
void ConstraintSolver<T>::ComputeInverseInertiaTimesGT(
std::function<MatrixX<T>(const MatrixX<T>&)> M_inv_mult,
std::function<VectorX<T>(const VectorX<T>&)> G_transpose_mult, int m,
MatrixX<T>* iM_GT) {
DRAKE_DEMAND(iM_GT != nullptr);
DRAKE_DEMAND(iM_GT->cols() == m);
VectorX<T> basis(m); // Basis vector.
VectorX<T> gT; // Intermediate result vector.
// Look for fast exit.
if (m == 0) return;
for (int i = 0; i < m; ++i) {
// Get the i'th column of G.
basis.setZero();
basis[i] = 1;
gT = G_transpose_mult(basis);
iM_GT->col(i) = M_inv_mult(gT);
}
}
// Checks the validity of the constraint matrix. This operation is relatively
// expensive and should only be called in debug mode. Nevertheless, it's
// useful to debug untested constraint Jacobian operators.
template <class T>
void ConstraintSolver<T>::CheckAccelConstraintMatrix(
const ConstraintAccelProblemData<T>& problem_data, const MatrixX<T>& MM) {
// Get numbers of types of contacts.
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Alias operators and vectors to make accessing them less clunky.
auto FT = problem_data.F_transpose_mult;
auto L = problem_data.L_mult;
auto iM = problem_data.solve_inertia;
// Alias these variables for more readable construction of MM and qq.
const int ngv = problem_data.tau.size(); // generalized velocity dimension.
const int nr = num_spanning_vectors;
const int nk = nr * 2;
const int nl = num_limits;
const int num_sliding = problem_data.sliding_contacts.size();
const int num_non_sliding = problem_data.non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
// Get the block of M that was set through a transposition operation.
Eigen::Ref<const MatrixX<T>> L_iM_FT =
MM.block(num_contacts + nk + num_non_sliding, num_contacts, nl, nr);
// Compute the block from scratch.
MatrixX<T> L_iM_FT_true(nl, nr);
MatrixX<T> iM_FT(ngv, nr);
ComputeInverseInertiaTimesGT(iM, FT, nr, &iM_FT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_FT, L_iM_FT_true);
// Determine the zero tolerance.
const double zero_tol =
std::numeric_limits<double>::epsilon() * MM.norm() * MM.rows();
// Check that the blocks are nearly equal.
DRAKE_ASSERT((L_iM_FT - L_iM_FT_true).norm() < zero_tol);
}
// Forms the linear system matrix and vector, which is used to determine the
// constraint forces.
template <class T>
void ConstraintSolver<T>::FormSustainedConstraintLinearSystem(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, MatrixX<T>* MM, VectorX<T>* qq) {
DRAKE_DEMAND(MM != nullptr);
DRAKE_DEMAND(qq != nullptr);
// Get numbers of types of contacts.
const int num_sliding = problem_data.sliding_contacts.size();
const int num_non_sliding = problem_data.non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Problem matrices and vectors are mildly adapted from:
// M. Anitescu and F. Potra. Formulating Dynamic Multi-Rigid Body Contact
// Problems as Solvable Linear Complementarity Problems. Nonlinear Dynamics,
// 14, 1997.
// Alias operators and vectors to make accessing them less clunky.
auto N = problem_data.N_mult;
auto F = problem_data.F_mult;
auto FT = problem_data.F_transpose_mult;
auto L = problem_data.L_mult;
auto LT = problem_data.L_transpose_mult;
auto iM = problem_data.solve_inertia;
const VectorX<T>& kN = problem_data.kN;
const VectorX<T>& kF = problem_data.kF;
const VectorX<T>& kL = problem_data.kL;
// Alias these variables for more readable construction of MM and qq.
const int ngv = problem_data.tau.size(); // generalized velocity dimension.
const int nc = num_contacts;
const int nr = num_spanning_vectors;
const int nl = num_limits;
const int num_vars = nc + nr + nl;
// Precompute some matrices that will be reused repeatedly.
MatrixX<T> iM_NT_minus_muQT(ngv, nc), iM_FT(ngv, nr), iM_LT(ngv, nl);
ComputeInverseInertiaTimesGT(iM, problem_data.N_minus_muQ_transpose_mult, nc,
&iM_NT_minus_muQT);
ComputeInverseInertiaTimesGT(iM, FT, nr, &iM_FT);
ComputeInverseInertiaTimesGT(iM, LT, nl, &iM_LT);
// Name the blocks of the matrix, which takes the form:
// N⋅M⁻¹⋅(Nᵀ - μₛQᵀ) N⋅M⁻¹⋅Fᵀ N⋅M⁻¹⋅Lᵀ
// F⋅M⁻¹⋅(Nᵀ - μₛQᵀ) F⋅M⁻¹⋅Fᵀ D⋅M⁻¹⋅Lᵀ
// L⋅M⁻¹⋅(Nᵀ - μₛQᵀ) L⋅M⁻¹⋅Fᵀ L⋅M⁻¹⋅Lᵀ
MM->resize(num_vars, num_vars);
Eigen::Ref<MatrixX<T>> N_iM_NT_minus_muQT = MM->block(0, 0, nc, nc);
Eigen::Ref<MatrixX<T>> N_iM_FT = MM->block(0, nc, nc, nr);
Eigen::Ref<MatrixX<T>> N_iM_LT = MM->block(0, nc + nr, nc, nl);
Eigen::Ref<MatrixX<T>> F_iM_NT_minus_muQT = MM->block(nc, 0, nr, nc);
Eigen::Ref<MatrixX<T>> F_iM_FT = MM->block(nc, nc, nr, nr);
Eigen::Ref<MatrixX<T>> F_iM_LT = MM->block(nc, nc + nr, nr, nl);
Eigen::Ref<MatrixX<T>> L_iM_NT_minus_muQT = MM->block(nc + nr, 0, nl, nc);
Eigen::Ref<MatrixX<T>> L_iM_FT = MM->block(nc + nr, nc, nl, nr);
Eigen::Ref<MatrixX<T>> L_iM_LT = MM->block(nc + nr, nc + nr, nl, nl);
// Compute the blocks.
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_NT_minus_muQT,
N_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_FT, N_iM_FT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_LT, N_iM_LT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_NT_minus_muQT,
F_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_FT, F_iM_FT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_LT, F_iM_LT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_NT_minus_muQT,
L_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_LT, L_iM_LT);
L_iM_FT = F_iM_LT.transpose().eval();
// Construct the vector:
// N⋅A⁻¹⋅a + kN
// F⋅A⁻¹⋅a + kD
// L⋅A⁻¹⋅a + kL
qq->resize(num_vars, 1);
qq->segment(0, nc) = N(trunc_neg_invA_a) + kN;
qq->segment(nc, nr) = F(trunc_neg_invA_a) + kF;
qq->segment(nc + nr, num_limits) = L(trunc_neg_invA_a) + kL;
}
// Forms the LCP matrix and vector, which is used to determine the constraint
// forces (and can also be used to determine the active set of constraints at
// the acceleration-level).
template <class T>
void ConstraintSolver<T>::FormSustainedConstraintLcp(
const ConstraintAccelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, MatrixX<T>* MM, VectorX<T>* qq) {
DRAKE_DEMAND(MM != nullptr);
DRAKE_DEMAND(qq != nullptr);
// Get numbers of types of contacts.
const int num_sliding = problem_data.sliding_contacts.size();
const int num_non_sliding = problem_data.non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Problem matrices and vectors are mildly adapted from:
// M. Anitescu and F. Potra. Formulating Dynamic Multi-Rigid Body Contact
// Problems as Solvable Linear Complementarity Problems. Nonlinear Dynamics,
// 14, 1997.
// Alias operators and vectors to make accessing them less clunky.
auto N = problem_data.N_mult;
auto F = problem_data.F_mult;
auto FT = problem_data.F_transpose_mult;
auto L = problem_data.L_mult;
auto LT = problem_data.L_transpose_mult;
auto iM = problem_data.solve_inertia;
const VectorX<T>& kN = problem_data.kN;
const VectorX<T>& kF = problem_data.kF;
const VectorX<T>& kL = problem_data.kL;
const VectorX<T>& mu_non_sliding = problem_data.mu_non_sliding;
// Construct a matrix similar to E in [Anitescu 1997]. This matrix will be
// used to specify the constraints (adapted from [Anitescu 1997] Eqn 2.7):
// 0 ≤ μₙₛ fNᵢ - eᵀ fF ⊥ λᵢ ≥ 0 and
// 0 ≤ e λᵢ + F dv/dt + dF/dt v ⊥ fF ≥ 0,
// where scalar λᵢ can roughly be interpreted as the remaining tangential
// acceleration at non-sliding contact i after frictional forces have been
// applied and e is a vector of ones (i.e., a segment of the appropriate
// column of E). Note that this matrix differs from the exact definition of
// E in [Anitescu 1997] to reflect the different layout of the LCP matrix
// from [Anitescu 1997] (the latter puts all spanning directions corresponding
// to a contact in one block; we put half of the spanning directions
// corresponding to a contact into one block and the other half into another
// block).
MatrixX<T> E = MatrixX<T>::Zero(num_spanning_vectors, num_non_sliding);
for (int i = 0, j = 0; i < num_non_sliding; ++i) {
E.col(i).segment(j, problem_data.r[i]).setOnes();
j += problem_data.r[i];
}
// Alias these variables for more readable construction of MM and qq.
const int ngv = problem_data.tau.size(); // generalized velocity dimension.
const int nc = num_contacts;
const int nr = num_spanning_vectors;
const int nk = nr * 2;
const int nl = num_limits;
const int num_vars = nc + nk + num_non_sliding + nl;
// Precompute some matrices that will be reused repeatedly.
MatrixX<T> iM_NT_minus_muQT(ngv, nc), iM_FT(ngv, nr), iM_LT(ngv, nl);
ComputeInverseInertiaTimesGT(iM, problem_data.N_minus_muQ_transpose_mult, nc,
&iM_NT_minus_muQT);
ComputeInverseInertiaTimesGT(iM, FT, nr, &iM_FT);
ComputeInverseInertiaTimesGT(iM, LT, nl, &iM_LT);
// Prepare blocks of the LCP matrix, which takes the form:
// N⋅M⁻¹⋅(Nᵀ - μₛQᵀ) N⋅M⁻¹⋅Dᵀ 0 N⋅M⁻¹⋅Lᵀ
// D⋅M⁻¹⋅(Nᵀ - μₛQᵀ) D⋅M⁻¹⋅Dᵀ E D⋅M⁻¹⋅Lᵀ
// μ -Eᵀ 0 0
// L⋅M⁻¹⋅(Nᵀ - μₛQᵀ) L⋅M⁻¹⋅Dᵀ 0 L⋅M⁻¹⋅Lᵀ
// where D = | F |
// | -F |
MM->resize(num_vars, num_vars);
Eigen::Ref<MatrixX<T>> N_iM_NT_minus_muQT = MM->block(0, 0, nc, nc);
Eigen::Ref<MatrixX<T>> N_iM_FT = MM->block(0, nc, nc, nr);
Eigen::Ref<MatrixX<T>> N_iM_LT =
MM->block(0, nc + nk + num_non_sliding, nc, nl);
Eigen::Ref<MatrixX<T>> F_iM_NT_minus_muQT = MM->block(nc, 0, nr, nc);
Eigen::Ref<MatrixX<T>> F_iM_FT = MM->block(nc, nc, nr, nr);
Eigen::Ref<MatrixX<T>> F_iM_LT =
MM->block(nc, nc + nk + num_non_sliding, nr, nl);
Eigen::Ref<MatrixX<T>> L_iM_NT_minus_muQT =
MM->block(nc + nk + num_non_sliding, 0, nl, nc);
Eigen::Ref<MatrixX<T>> L_iM_LT =
MM->block(nc + nk + num_non_sliding, nc + nk + num_non_sliding, nl, nl);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_NT_minus_muQT,
N_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_FT, N_iM_FT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_LT, N_iM_LT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_NT_minus_muQT,
F_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_FT, F_iM_FT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_LT, F_iM_LT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_NT_minus_muQT,
L_iM_NT_minus_muQT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_LT, L_iM_LT);
// Construct the LCP matrix. First do the "normal contact direction" rows.
MM->block(0, nc + nr, nc, nr) = -MM->block(0, nc, nc, nr);
MM->block(0, nc + nk, nc, num_non_sliding).setZero();
// Now construct the un-negated tangent contact direction rows.
MM->block(nc, nc + nr, num_spanning_vectors, nr) =
-MM->block(nc, nc, nr, num_spanning_vectors);
MM->block(nc, nc + nk, num_spanning_vectors, num_non_sliding) = E;
// Now construct the negated tangent contact direction rows. These negated
// tangent contact directions allow the LCP to compute forces applied along
// the negative x-axis. E will have to be reset to un-negate it.
MM->block(nc + nr, 0, nr, MM->cols()) = -MM->block(nc, 0, nr, MM->cols());
MM->block(nc + nr, nc + nk, num_spanning_vectors, num_non_sliding) = E;
// Construct the next block, which provides the friction "cone" constraint.
const std::vector<int>& ns_contacts = problem_data.non_sliding_contacts;
MM->block(nc + nk, 0, num_non_sliding, nc).setZero();
for (int i = 0; static_cast<size_t>(i) < ns_contacts.size(); ++i)
(*MM)(nc + nk + i, ns_contacts[i]) = mu_non_sliding[i];
MM->block(nc + nk, nc, num_non_sliding, num_spanning_vectors) =
-E.transpose();
MM->block(nc + nk, nc + num_spanning_vectors, num_non_sliding,
num_spanning_vectors) = -E.transpose();
MM->block(nc + nk, nc + nk, num_non_sliding, num_non_sliding + nl).setZero();
// Construct the last row block, which provides the generic unilateral
// constraints.
MM->block(nc + nk + num_non_sliding, 0, nl, nc + nk + num_non_sliding) =
MM->block(0, nc + nk + num_non_sliding, nc + nk + num_non_sliding, nl)
.transpose()
.eval();
// Check the transposed blocks of the LCP matrix.
DRAKE_ASSERT_VOID(CheckAccelConstraintMatrix(problem_data, *MM));
// Construct the LCP vector:
// N⋅A⁻¹⋅a + kN
// D⋅A⁻¹⋅a + kD
// 0
// L⋅A⁻¹⋅a + kL
// where, as above, D is defined as [F -F] (and kD is defined as [kF -kF].
qq->resize(num_vars, 1);
qq->segment(0, nc) = N(trunc_neg_invA_a) + kN;
qq->segment(nc, nr) = F(trunc_neg_invA_a) + kF;
qq->segment(nc + nr, nr) = -qq->segment(nc, nr);
qq->segment(nc + nk, num_non_sliding).setZero();
qq->segment(nc + nk + num_non_sliding, num_limits) = L(trunc_neg_invA_a) + kL;
}
template <class T>
void ConstraintSolver<T>::CheckVelConstraintMatrix(
const ConstraintVelProblemData<T>& problem_data, const MatrixX<T>& MM) {
// Get numbers of contacts.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Alias operators and vectors to make accessing them less clunky.
const auto N = problem_data.N_mult;
const auto NT = problem_data.N_transpose_mult;
const auto F = problem_data.F_mult;
const auto FT = problem_data.F_transpose_mult;
const auto L = problem_data.L_mult;
const auto LT = problem_data.L_transpose_mult;
auto iM = problem_data.solve_inertia;
// Alias these variables for more readable construction of MM and qq.
const int ngv = problem_data.Mv.size(); // generalized velocity dimension.
const int nr = num_spanning_vectors;
const int nk = nr * 2;
const int nl = num_limits;
// Get blocks of M that were set through a transposition operation.
Eigen::Ref<const MatrixX<T>> F_iM_NT =
MM.block(num_contacts, 0, nr, num_contacts);
Eigen::Ref<const MatrixX<T>> L_iM_NT =
MM.block(num_contacts * 2 + nk, 0, nl, num_contacts);
Eigen::Ref<const MatrixX<T>> L_iM_FT =
MM.block(num_contacts * 2 + nk, num_contacts, nl, nr);
// Compute the blocks from scratch.
MatrixX<T> F_iM_NT_true(nr, num_contacts), L_iM_NT_true(nl, num_contacts);
MatrixX<T> L_iM_FT_true(nl, nr);
MatrixX<T> iM_NT(ngv, num_contacts), iM_FT(ngv, nr);
ComputeInverseInertiaTimesGT(iM, NT, num_contacts, &iM_NT);
ComputeInverseInertiaTimesGT(iM, FT, nr, &iM_FT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_NT, F_iM_NT_true);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_NT, L_iM_NT_true);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_FT, L_iM_FT_true);
// Determine the zero tolerance.
const double zero_tol =
std::numeric_limits<double>::epsilon() * MM.norm() * MM.rows();
// Check that the blocks are nearly equal. Note: these tests are necessary
// because Eigen does not correctly compute the norm of an empty matrix.
DRAKE_ASSERT(F_iM_NT.rows() == 0 || F_iM_NT.cols() == 0 ||
(F_iM_NT - F_iM_NT_true).norm() < zero_tol);
DRAKE_ASSERT(L_iM_NT.rows() == 0 || L_iM_NT.cols() == 0 ||
(L_iM_NT - L_iM_NT_true).norm() < zero_tol);
DRAKE_ASSERT(L_iM_FT.rows() == 0 || L_iM_FT.cols() == 0 ||
(L_iM_FT - L_iM_FT_true).norm() < zero_tol);
}
// Forms the LCP matrix and vector, which is used to determine the collisional
// impulses.
template <class T>
void ConstraintSolver<T>::FormImpactingConstraintLcp(
const ConstraintVelProblemData<T>& problem_data,
const VectorX<T>& trunc_neg_invA_a, MatrixX<T>* MM, VectorX<T>* qq) {
DRAKE_DEMAND(MM != nullptr);
DRAKE_DEMAND(qq != nullptr);
// Get numbers of contacts.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
// Problem matrices and vectors are nearly identical to:
// M. Anitescu and F. Potra. Formulating Dynamic Multi-Rigid Body Contact
// Problems as Solvable Linear Complementarity Problems. Nonlinear Dynamics,
// 14, 1997.
// Alias operators and vectors to make accessing them less clunky.
const auto N = problem_data.N_mult;
const auto NT = problem_data.N_transpose_mult;
const auto F = problem_data.F_mult;
const auto FT = problem_data.F_transpose_mult;
const auto L = problem_data.L_mult;
const auto LT = problem_data.L_transpose_mult;
auto iM = problem_data.solve_inertia;
const VectorX<T>& mu = problem_data.mu;
const VectorX<T>& gammaN = problem_data.gammaN;
const VectorX<T>& gammaF = problem_data.gammaF;
const VectorX<T>& gammaE = problem_data.gammaE;
const VectorX<T>& gammaL = problem_data.gammaL;
// Construct the matrix E in [Anitscu 1997]. This matrix will be used to
// specify the constraints:
// 0 ≤ μ⋅fN - E⋅fF ⊥ λ ≥ 0 and
// 0 ≤ e⋅λ + F⋅v ⊥ fF ≥ 0,
// where λ can roughly be interpreted as the remaining tangential velocity
// at the impacting contacts after frictional impulses have been applied and
// e is a vector of ones (i.e., a segment of the appropriate column of E).
// Note that this matrix differs from the exact definition of E in
// [Anitescu 1997] to reflect the different layout of the LCP matrix from
// [Anitescu 1997] (the latter puts all spanning directions corresponding
// to a contact in one block; we put half of the spanning directions
// corresponding to a contact into one block and the other half into another
// block).
MatrixX<T> E = MatrixX<T>::Zero(num_spanning_vectors, num_contacts);
for (int i = 0, j = 0; i < num_contacts; ++i) {
E.col(i).segment(j, problem_data.r[i]).setOnes();
j += problem_data.r[i];
}
// Alias these variables for more readable construction of MM and qq.
const int ngv = problem_data.Mv.size(); // generalized velocity dimension.
const int nc = num_contacts;
const int nr = num_spanning_vectors;
const int nk = nr * 2;
const int nl = num_limits;
// Precompute some matrices that will be reused repeatedly.
MatrixX<T> iM_NT(ngv, nc), iM_FT(ngv, nr), iM_LT(ngv, nl);
ComputeInverseInertiaTimesGT(iM, NT, nc, &iM_NT);
ComputeInverseInertiaTimesGT(iM, FT, nr, &iM_FT);
ComputeInverseInertiaTimesGT(iM, LT, nl, &iM_LT);
// Prepare blocks of the LCP matrix, which takes the form:
// N⋅M⁻¹⋅Nᵀ N⋅M⁻¹⋅Dᵀ 0 N⋅M⁻¹⋅Lᵀ
// D⋅M⁻¹⋅Nᵀ D⋅M⁻¹⋅Dᵀ E D⋅M⁻¹⋅Lᵀ
// μ -Eᵀ 0 0
// L⋅M⁻¹⋅Nᵀ L⋅M⁻¹⋅Dᵀ 0 L⋅M⁻¹⋅Lᵀ
// where D = | F |
// | -F |
const int num_vars = nc * 2 + nk + num_limits;
MM->resize(num_vars, num_vars);
Eigen::Ref<MatrixX<T>> N_iM_NT = MM->block(0, 0, nc, nc);
Eigen::Ref<MatrixX<T>> N_iM_FT = MM->block(0, nc, nc, nr);
Eigen::Ref<MatrixX<T>> N_iM_LT = MM->block(0, nc * 2 + nk, nc, nl);
Eigen::Ref<MatrixX<T>> F_iM_FT = MM->block(nc, nc, nr, nr);
Eigen::Ref<MatrixX<T>> F_iM_LT = MM->block(nc, nc * 2 + nk, nr, nl);
Eigen::Ref<MatrixX<T>> L_iM_LT = MM->block(nc * 2 + nk, nc * 2 + nk, nl, nl);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_NT, N_iM_NT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_FT, N_iM_FT);
ComputeConstraintSpaceComplianceMatrix(N, nc, iM_LT, N_iM_LT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_FT, F_iM_FT);
ComputeConstraintSpaceComplianceMatrix(F, nr, iM_LT, F_iM_LT);
ComputeConstraintSpaceComplianceMatrix(L, nl, iM_LT, L_iM_LT);
// Construct the LCP matrix. First do the "normal contact direction" rows:
MM->block(0, nc + nr, nc, nr) = -MM->block(0, nc, nc, nr);
MM->block(0, nc + nk, nc, nc).setZero();
// Now construct the un-negated tangent contact direction rows.
MM->block(nc, 0, nr, nc) = MM->block(0, nc, nc, nr).transpose().eval();
MM->block(nc, nc + nr, nr, nr) = -MM->block(nc, nc, nr, nr);
MM->block(nc, nc + nk, num_spanning_vectors, nc) = E;
// Now construct the negated tangent contact direction rows. These negated
// tangent contact directions allow the LCP to compute forces applied along
// the negative x-axis. E will have to be reset to un-negate it.
MM->block(nc + nr, 0, nr, MM->cols()) = -MM->block(nc, 0, nr, MM->cols());
MM->block(nc + nr, nc + nk, num_spanning_vectors, nc) = E;
// Construct the next two row blocks, which provide the friction "cone"
// constraint.
MM->block(nc + nk, 0, nc, nc) = Eigen::DiagonalMatrix<T, Eigen::Dynamic>(mu);
MM->block(nc + nk, nc, nc, num_spanning_vectors) = -E.transpose();
MM->block(nc + nk, nc + num_spanning_vectors, nc, num_spanning_vectors) =
-E.transpose();
MM->block(nc + nk, nc + nk, nc, nc + nl).setZero();
// Construct the last row block, which provides the generic unilateral
// constraints.
MM->block(nc * 2 + nk, 0, nl, nc * 2 + nk) =
MM->block(0, nc * 2 + nk, nc * 2 + nk, nl).transpose().eval();
// Check the transposed blocks of the LCP matrix.
DRAKE_ASSERT_VOID(CheckVelConstraintMatrix(problem_data, *MM));
// Verify that all gamma vectors are either empty or non-negative.
DRAKE_DEMAND(gammaN.size() == 0 || gammaN.minCoeff() >= 0);
DRAKE_DEMAND(gammaF.size() == 0 || gammaF.minCoeff() >= 0);
DRAKE_DEMAND(gammaE.size() == 0 || gammaE.minCoeff() >= 0);
DRAKE_DEMAND(gammaL.size() == 0 || gammaL.minCoeff() >= 0);
// Regularize the LCP matrix.
MM->topLeftCorner(nc, nc) += Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaN);
MM->block(nc, nc, nr, nr) += Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaF);
MM->block(nc + nr, nc + nr, nr, nr) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaF);
MM->block(nc + nk, nc + nk, nc, nc) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaE);
MM->block(nc * 2 + nk, nc * 2 + nk, nl, nl) +=
Eigen::DiagonalMatrix<T, Eigen::Dynamic>(gammaL);
// Construct the LCP vector:
// NA⁻¹a + kN
// DA⁻¹a + kD
// 0
// LA⁻¹a + kL
// where, as above, D is defined as [F -F] (and kD = [kF -kF]).
qq->resize(num_vars, 1);
qq->segment(0, nc) = N(trunc_neg_invA_a) + problem_data.kN;
qq->segment(nc, nr) = F(trunc_neg_invA_a) + problem_data.kF;
qq->segment(nc + nr, nr) = -qq->segment(nc, nr);
qq->segment(nc + nk, nc).setZero();
qq->segment(nc * 2 + nk, num_limits) = L(trunc_neg_invA_a) + problem_data.kL;
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedForceFromConstraintForces(
const ConstraintAccelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_force) {
if (!generalized_force)
throw std::logic_error("generalized_force vector is null.");
// Get numbers of types of contacts.
const int num_sliding = problem_data.sliding_contacts.size();
const int num_non_sliding = problem_data.non_sliding_contacts.size();
const int num_contacts = num_sliding + num_non_sliding;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_bilat_constraints = problem_data.kG.size();
// Verify cf is the correct size.
const int num_vars =
num_contacts + num_spanning_vectors + num_limits + num_bilat_constraints;
if (cf.size() != num_vars) {
throw std::logic_error(
"cf (constraint force) parameter incorrectly"
"sized.");
}
/// Get the normal and non-sliding contact forces.
const Eigen::Ref<const VectorX<T>> f_normal = cf.segment(0, num_contacts);
const Eigen::Ref<const VectorX<T>> f_non_sliding_frictional =
cf.segment(num_contacts, num_spanning_vectors);
/// Get the limit forces.
const Eigen::Ref<const VectorX<T>> f_limit =
cf.segment(num_contacts + num_spanning_vectors, num_limits);
// Get the bilateral constraint forces.
const Eigen::Ref<const VectorX<T>> f_bilat = cf.segment(
num_contacts + num_spanning_vectors + num_limits, num_bilat_constraints);
/// Compute the generalized force.
*generalized_force = problem_data.N_minus_muQ_transpose_mult(f_normal) +
problem_data.F_transpose_mult(f_non_sliding_frictional) +
problem_data.L_transpose_mult(f_limit) +
problem_data.G_transpose_mult(f_bilat);
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedForceFromConstraintForces(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_force) {
if (!generalized_force)
throw std::logic_error("generalized_force vector is null.");
// Look for fast exit.
if (cf.size() == 0) {
generalized_force->setZero(problem_data.Mv.size(), 1);
return;
}
// Get number of contacts.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_bilat_constraints = problem_data.kG.size();
// Verify cf is the correct size.
const int num_vars =
num_contacts + num_spanning_vectors + num_limits + num_bilat_constraints;
if (num_vars != cf.size()) {
throw std::logic_error(
"Unexpected packed constraint force vector"
" dimension.");
}
/// Get the normal and tangential contact forces.
const Eigen::Ref<const VectorX<T>> f_normal = cf.segment(0, num_contacts);
const Eigen::Ref<const VectorX<T>> f_frictional =
cf.segment(num_contacts, num_spanning_vectors);
/// Get the limit forces.
const Eigen::Ref<const VectorX<T>> f_limit =
cf.segment(num_contacts + num_spanning_vectors, num_limits);
// Get the bilateral constraint forces.
const Eigen::Ref<const VectorX<T>> f_bilat = cf.segment(
num_contacts + num_spanning_vectors + num_limits, num_bilat_constraints);
/// Compute the generalized forces.
*generalized_force = problem_data.N_transpose_mult(f_normal) +
problem_data.F_transpose_mult(f_frictional) +
problem_data.L_transpose_mult(f_limit) +
problem_data.G_transpose_mult(f_bilat);
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedAcceleration(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& v,
const VectorX<T>& cf, double dt, VectorX<T>* generalized_acceleration) {
DRAKE_DEMAND(dt > 0);
// Keep from allocating storage by reusing `generalized_acceleration`; at
// first, it will hold the generalized force from constraint forces.
ComputeGeneralizedForceFromConstraintForces(problem_data, cf,
generalized_acceleration);
// Using a first-order approximation to velocity, the new velocity is:
// v(t+dt) = v(t) + dt * ga
// = inv(M) * (M * v(t) + dt * gf)
// where ga is the generalized acceleration and gf is the generalized force.
// Note: we have no way to break apart the Mv term. But, we can instead
// compute v(t+dt) and then solve for the acceleration.
const VectorX<T> vplus = problem_data.solve_inertia(
problem_data.Mv + dt * (*generalized_acceleration));
*generalized_acceleration = (vplus - v) / dt;
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedAccelerationFromConstraintForces(
const ConstraintAccelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_acceleration) {
if (!generalized_acceleration)
throw std::logic_error("generalized_acceleration vector is null.");
VectorX<T> generalized_force;
ComputeGeneralizedForceFromConstraintForces(problem_data, cf,
&generalized_force);
*generalized_acceleration = problem_data.solve_inertia(generalized_force);
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedAccelerationFromConstraintForces(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_acceleration) {
if (!generalized_acceleration)
throw std::logic_error("generalized_acceleration vector is null.");
VectorX<T> generalized_force;
ComputeGeneralizedForceFromConstraintForces(problem_data, cf,
&generalized_force);
*generalized_acceleration = problem_data.solve_inertia(generalized_force);
}
template <class T>
void ConstraintSolver<T>::ComputeGeneralizedVelocityChange(
const ConstraintVelProblemData<T>& problem_data, const VectorX<T>& cf,
VectorX<T>* generalized_delta_v) {
if (!generalized_delta_v)
throw std::logic_error("generalized_delta_v vector is null.");
VectorX<T> generalized_impulse;
ComputeGeneralizedForceFromConstraintForces(problem_data, cf,
&generalized_impulse);
*generalized_delta_v = problem_data.solve_inertia(generalized_impulse);
}
template <class T>
void ConstraintSolver<T>::CalcContactForcesInContactFrames(
const VectorX<T>& cf, const ConstraintAccelProblemData<T>& problem_data,
const std::vector<Matrix2<T>>& contact_frames,
std::vector<Vector2<T>>* contact_forces) {
using std::abs;
// Loose tolerance for unit vectors and orthogonality.
const double loose_eps = std::sqrt(std::numeric_limits<double>::epsilon());
// Verify that contact_forces is non-null and is empty.
if (!contact_forces)
throw std::logic_error("Vector of contact forces is null.");
if (!contact_forces->empty())
throw std::logic_error("Vector of contact forces is not empty.");
// Verify that cf is the correct size.
const int num_non_sliding_contacts = problem_data.non_sliding_contacts.size();
const int num_contacts =
problem_data.sliding_contacts.size() + num_non_sliding_contacts;
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_bilat_constraints = problem_data.kG.size();
const int num_vars =
num_contacts + num_spanning_vectors + num_limits + num_bilat_constraints;
if (num_vars != cf.size()) {
throw std::logic_error(
"Unexpected packed constraint force vector "
"dimension.");
}
// Verify that the problem is indeed two-dimensional.
if (num_spanning_vectors != num_non_sliding_contacts) {
throw std::logic_error(
"Problem data 'r' indicates contact problem is not "
"two-dimensional");
}
// Verify that the correct number of contact frames has been specified.
if (contact_frames.size() != static_cast<size_t>(num_contacts)) {
throw std::logic_error(
"Number of contact frames does not match number of "
"contacts.");
}
// Verify that sliding contact indices are sorted.
DRAKE_ASSERT(std::is_sorted(problem_data.sliding_contacts.begin(),
problem_data.sliding_contacts.end()));
// Resize the force vector.
contact_forces->resize(contact_frames.size());
// Set the forces.
for (int i = 0, sliding_index = 0, non_sliding_index = 0; i < num_contacts;
++i) {
// Alias the force.
Vector2<T>& contact_force_i = (*contact_forces)[i];
// Get the contact normal and tangent.
const Vector2<T> contact_normal = contact_frames[i].col(0);
const Vector2<T> contact_tangent = contact_frames[i].col(1);
// Verify that each direction is of unit length.
if (abs(contact_normal.norm() - 1) > loose_eps)
throw std::runtime_error("Contact normal apparently not unit length.");
if (abs(contact_tangent.norm() - 1) > loose_eps)
throw std::runtime_error("Contact tangent apparently not unit length.");
// Verify that the two directions are orthogonal.
if (abs(contact_normal.dot(contact_tangent)) > loose_eps) {
throw std::logic_error(fmt::format(
"Contact normal ({}) and contact tangent ({}) insufficiently "
"orthogonal.",
fmt_eigen(contact_normal.transpose()),
fmt_eigen(contact_tangent.transpose())));
}
// Initialize the contact force expressed in the global frame.
Vector2<T> f0(0, 0);
// Add in the contact normal.
f0 += contact_normal * cf[i];
// Determine whether the contact is sliding.
const bool is_sliding =
std::binary_search(problem_data.sliding_contacts.begin(),
problem_data.sliding_contacts.end(), i);
// Subtract/add the tangential force in the world frame.
if (is_sliding) {
f0 -= contact_tangent * cf[i] * problem_data.mu_sliding[sliding_index++];
} else {
f0 += contact_tangent * cf[num_contacts + non_sliding_index++];
}
// Compute the contact force in the contact frame.
contact_force_i = contact_frames[i].transpose() * f0;
}
}
template <class T>
void ConstraintSolver<T>::CalcContactForcesInContactFrames(
const VectorX<T>& cf, const ConstraintVelProblemData<T>& problem_data,
const std::vector<Matrix2<T>>& contact_frames,
std::vector<Vector2<T>>* contact_forces) {
using std::abs;
// Loose tolerance for unit vectors and orthogonality.
const double loose_eps = std::sqrt(std::numeric_limits<double>::epsilon());
// Verify that contact_forces is non-null and is empty.
if (!contact_forces)
throw std::logic_error("Vector of contact forces is null.");
if (!contact_forces->empty())
throw std::logic_error("Vector of contact forces is not empty.");
// Verify that cf is the correct size.
const int num_contacts = problem_data.mu.size();
const int num_spanning_vectors =
std::accumulate(problem_data.r.begin(), problem_data.r.end(), 0);
const int num_limits = problem_data.kL.size();
const int num_bilat_constraints = problem_data.kG.size();
const int num_vars =
num_contacts + num_spanning_vectors + num_limits + num_bilat_constraints;
if (num_vars != cf.size()) {
throw std::logic_error(
"Unexpected packed constraint force vector "
"dimension.");
}
// Verify that the problem is indeed two-dimensional.
if (num_spanning_vectors != num_contacts) {
throw std::logic_error(
"Problem data 'r' indicates contact problem is not "
"two-dimensional");
}
// Verify that the correct number of contact frames has been specified.
if (contact_frames.size() != static_cast<size_t>(num_contacts)) {
throw std::logic_error(
"Number of contact frames does not match number of "
"contacts.");
}
// Resize the force vector.
contact_forces->resize(contact_frames.size());
// Set the forces.
for (int i = 0, tangent_index = 0; i < num_contacts; ++i) {
// Alias the force.
Vector2<T>& contact_force_i = (*contact_forces)[i];
// Get the contact normal and tangent.
const Vector2<T> contact_normal = contact_frames[i].col(0);
const Vector2<T> contact_tangent = contact_frames[i].col(1);
// Verify that each direction is of unit length.
if (abs(contact_normal.norm() - 1) > loose_eps)
throw std::runtime_error("Contact normal apparently not unit length.");
if (abs(contact_tangent.norm() - 1) > loose_eps)
throw std::runtime_error("Contact tangent apparently not unit length.");
// Verify that the two directions are orthogonal.
if (abs(contact_normal.dot(contact_tangent)) > loose_eps) {
throw std::logic_error(fmt::format(
"Contact normal ({}) and contact tangent ({}) insufficiently "
"orthogonal.",
fmt_eigen(contact_normal.transpose()),
fmt_eigen(contact_tangent.transpose())));
}
// Compute the contact force expressed in the global frame.
Vector2<T> j0 = contact_normal * cf[i] +
contact_tangent * cf[num_contacts + tangent_index++];
// Compute the contact force in the contact frame.
contact_force_i = contact_frames[i].transpose() * j0;
}
}
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/rod2d | /home/johnshepherd/drake/examples/rod2d/test/rod2d_test.cc | #include "drake/examples/rod2d/rod2d.h"
#include <memory>
#include <gtest/gtest.h>
#include "drake/common/test_utilities/eigen_matrix_compare.h"
#include "drake/common/test_utilities/expect_no_throw.h"
#include "drake/examples/rod2d/constraint_problem_data.h"
#include "drake/examples/rod2d/constraint_solver.h"
#include "drake/systems/analysis/simulator.h"
using drake::systems::AbstractValues;
using drake::systems::BasicVector;
using drake::systems::Context;
using drake::systems::ContinuousState;
using drake::systems::Simulator;
using drake::systems::State;
using drake::systems::SystemOutput;
using drake::systems::VectorBase;
using Eigen::Vector2d;
using Eigen::Vector3d;
using Eigen::VectorXd;
namespace drake {
namespace examples {
namespace rod2d {
/// Class for testing the Rod2D example using a piecewise DAE
/// approach.
class Rod2DDAETest : public ::testing::Test {
protected:
void SetUp() override {
dut_ = std::make_unique<Rod2D<double>>(
Rod2D<double>::SystemType::kPiecewiseDAE, 0.0);
context_ = dut_->CreateDefaultContext();
output_ = dut_->AllocateOutput();
derivatives_ = dut_->AllocateTimeDerivatives();
// Use a non-unit mass.
dut_->set_rod_mass(2.0);
// Set cfm to be very small, so that the complementarity problems are
// well conditioned but the system is still nearly perfectly rigid. erp is
// to be set to 0.2 (a reasonable default).
const double cfm = 100 * std::numeric_limits<double>::epsilon();
const double erp = 0.2;
dut_->SetStiffnessAndDissipation(cfm, erp);
// Set a zero input force (this is the default).
const Vector3<double> ext_input(0, 0, 0);
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
}
std::unique_ptr<State<double>> CloneState() const {
return context_->CloneState();
}
VectorBase<double>& continuous_state() {
return context_->get_mutable_continuous_state_vector();
}
// Sets a secondary initial Painlevé configuration.
void SetSecondInitialConfig() {
// Set the configuration to an inconsistent (Painlevé) type state with
// the rod at a 135 degree counter-clockwise angle with respect to the
// x-axis. The rod in [Stewart, 2000] is at a 45 degree counter-clockwise
// angle with respect to the x-axis.
// * [Stewart, 2000] D. Stewart, "Rigid-Body Dynamics with Friction and
// Impact". SIAM Rev., 42(1), 3-39, 2000.
using std::sqrt;
const double half_len = dut_->get_rod_half_length();
const double r22 = std::sqrt(2) / 2;
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = -half_len * r22;
xc[1] = half_len * r22;
xc[2] = 3 * M_PI / 4.0;
xc[3] = 1.0;
xc[4] = 0.0;
xc[5] = 0.0;
// TODO(edrumwri): Put the rod into the single contact sliding mode.
}
// Sets the rod to a state that corresponds to ballistic motion.
void SetBallisticState() {
const double half_len = dut_->get_rod_half_length();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0;
xc[1] = 10 * half_len;
xc[2] = M_PI_2;
xc[3] = 1.0;
xc[4] = 2.0;
xc[5] = 3.0;
// TODO(edrumwri): Set the mode to ballistic.
}
// Sets the rod to an interpenetrating configuration without modifying the
// velocity or any mode variables.
void SetInterpenetratingConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = -1.0; // com vertical position
xc[2] = 0.0; // rod rotation
}
// Sets the rod to a resting horizontal configuration without modifying the
// mode variables.
void SetRestingHorizontalConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = 0.0; // com vertical position
xc[2] = 0.0; // rod rotation
xc[3] = xc[4] = xc[5] = 0.0; // velocity variables
}
// Sets the rod to a resting vertical configuration without modifying the
// mode variables.
void SetRestingVerticalConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0; // com horizontal position
xc[1] = dut_->get_rod_half_length(); // com vertical position
xc[2] = M_PI_2; // rod rotation
xc[3] = xc[4] = xc[5] = 0.0; // velocity variables
}
// Sets the contact mode such that the left endpoint is contacting. The caller
// sets whether or not the contact should be considered to be sliding.
void SetLeftEndpointContacting(State<double>* state, bool sliding) {
// TODO(edrumwri): Implement this.
}
// Sets the rod to an arbitrary impacting state.
void SetImpactingState() {
// This state is identical to that obtained from SetSecondInitialConfig()
// but with the vertical component of velocity set such that the state
// corresponds to an impact.
SetSecondInitialConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[4] = -1.0; // com horizontal velocity
// TODO(edrumwri): Set the rod mode to indicate that it is in the single
// contact sliding mode.
}
// Computes rigid impact data.
void CalcRigidImpactVelProblemData(ConstraintVelProblemData<double>* data) {
// Get the points of contact.
std::vector<Vector2d> contacts;
dut_->GetContactPoints(*context_, &contacts);
// Compute the problem data.
dut_->CalcImpactProblemData(*context_, contacts, data);
}
// Computes rigid contact data.
void CalcConstraintAccelProblemData(
ConstraintAccelProblemData<double>* data) {
// TODO(edrumwri): Compute the rigid contact data.
}
// Models an impact.
void ModelImpact() {
ConstraintVelProblemData<double> data(3 /* ngc */);
CalcRigidImpactVelProblemData(&data);
VectorX<double> cf;
contact_solver_.SolveImpactProblem(data, &cf);
// Get the update to the generalized velocity.
VectorX<double> delta_v;
contact_solver_.ComputeGeneralizedVelocityChange(data, cf, &delta_v);
// Update the velocity part of the state.
context_->get_mutable_continuous_state()
.get_mutable_generalized_velocity()
.SetFromVector(data.solve_inertia(data.Mv) + delta_v);
}
// Gets the number of generalized coordinates for the rod.
int get_rod_num_coordinates() { return 3; }
// Gets the output dimension of a Jacobian multiplication operator.
int GetOperatorDim(std::function<VectorX<double>(const VectorX<double>&)> J) {
return J(VectorX<double>(get_rod_num_coordinates())).size();
}
// Checks the consistency of a transpose operator.
void CheckTransOperatorDim(
std::function<VectorX<double>(const VectorX<double>&)> JT,
int num_constraints) {
EXPECT_EQ(JT(VectorX<double>(num_constraints)).size(),
get_rod_num_coordinates());
}
// Checks consistency of rigid contact problem data.
void CheckProblemConsistency(const ConstraintAccelProblemData<double>& data,
int num_contacts) {
// TODO(edrumwri): Stop short-circuiting this after piecewise DAE
// implementation repaired.
return;
EXPECT_EQ(num_contacts,
data.sliding_contacts.size() + data.non_sliding_contacts.size());
EXPECT_EQ(GetOperatorDim(data.N_mult), num_contacts);
CheckTransOperatorDim(data.N_minus_muQ_transpose_mult, num_contacts);
EXPECT_EQ(GetOperatorDim(data.L_mult), data.kL.size());
CheckTransOperatorDim(data.L_transpose_mult, data.kL.size());
EXPECT_EQ(data.tau.size(), get_rod_num_coordinates());
EXPECT_EQ(data.kN.size(), num_contacts);
EXPECT_EQ(data.kF.size(), data.non_sliding_contacts.size());
EXPECT_EQ(data.mu_non_sliding.size(), data.non_sliding_contacts.size());
EXPECT_EQ(data.mu_sliding.size(), data.sliding_contacts.size());
EXPECT_EQ(data.r.size(), data.non_sliding_contacts.size());
EXPECT_TRUE(data.solve_inertia);
EXPECT_TRUE(std::is_sorted(data.sliding_contacts.begin(),
data.sliding_contacts.end()));
EXPECT_TRUE(std::is_sorted(data.non_sliding_contacts.begin(),
data.non_sliding_contacts.end()));
// Only true because this problem is 2D.
EXPECT_EQ(GetOperatorDim(data.F_mult), data.non_sliding_contacts.size());
CheckTransOperatorDim(data.F_transpose_mult,
data.non_sliding_contacts.size());
}
// Checks consistency of rigid impact problem data.
void CheckProblemConsistency(const ConstraintVelProblemData<double>& data,
int num_contacts) {
EXPECT_EQ(num_contacts, data.mu.size());
EXPECT_EQ(num_contacts, data.r.size());
EXPECT_EQ(GetOperatorDim(data.N_mult), num_contacts);
CheckTransOperatorDim(data.N_transpose_mult, num_contacts);
EXPECT_EQ(data.kN.size(), num_contacts);
EXPECT_EQ(data.Mv.size(), get_rod_num_coordinates());
EXPECT_TRUE(data.solve_inertia);
EXPECT_EQ(GetOperatorDim(data.F_mult), num_contacts);
CheckTransOperatorDim(data.F_transpose_mult, num_contacts);
EXPECT_EQ(data.kF.size(), num_contacts);
EXPECT_EQ(GetOperatorDim(data.L_mult), data.kL.size());
CheckTransOperatorDim(data.L_transpose_mult, data.kL.size());
}
std::unique_ptr<Rod2D<double>> dut_; //< The device under test.
std::unique_ptr<Context<double>> context_;
std::unique_ptr<SystemOutput<double>> output_;
std::unique_ptr<ContinuousState<double>> derivatives_;
ConstraintSolver<double> contact_solver_;
};
// Verifies that the state vector functions throw no exceptions.
TEST_F(Rod2DDAETest, NamedStateVectorsNoThrow) {
DRAKE_EXPECT_NO_THROW(Rod2D<double>::get_mutable_state(context_.get()));
DRAKE_EXPECT_NO_THROW(Rod2D<double>::get_state(*context_));
DRAKE_EXPECT_NO_THROW(
Rod2D<double>::get_state(context_->get_continuous_state()));
DRAKE_EXPECT_NO_THROW(Rod2D<double>::get_mutable_state(
&context_->get_mutable_continuous_state()));
}
// Tests that named state vector components are at expected indices.
TEST_F(Rod2DDAETest, ExpectedIndices) {
// Set the state.
Rod2dStateVector<double>& state =
Rod2D<double>::get_mutable_state(context_.get());
state.set_x(1.0);
state.set_y(2.0);
state.set_theta(3.0);
state.set_xdot(5.0);
state.set_ydot(7.0);
state.set_thetadot(11.0);
// Check the indices.
const VectorBase<double>& x = context_->get_continuous_state_vector();
EXPECT_EQ(state.x(), x[0]);
EXPECT_EQ(state.y(), x[1]);
EXPECT_EQ(state.theta(), x[2]);
EXPECT_EQ(state.xdot(), x[3]);
EXPECT_EQ(state.ydot(), x[4]);
EXPECT_EQ(state.thetadot(), x[5]);
}
// Checks that the output port represents the state.
TEST_F(Rod2DDAETest, Output) {
const ContinuousState<double>& xc = context_->get_continuous_state();
std::unique_ptr<SystemOutput<double>> output = dut_->AllocateOutput();
dut_->CalcOutput(*context_, output.get());
for (int i = 0; i < xc.size(); ++i)
EXPECT_EQ(xc[i], output->get_vector_data(0)->value()(i));
}
// Verifies that setting dut to an impacting state actually results in an
// impacting state.
TEST_F(Rod2DDAETest, ImpactingState) {
// TODO(edrumwri): Set this state to impacting.
// TODO(edrumwri): Verify that the rod system thinks that it is impacting.
}
// Tests parameter getting and setting.
TEST_F(Rod2DDAETest, Parameters) {
// Set parameters to non-default values.
const double g = -1.0;
const double mass = 0.125;
const double mu = 0.5;
const double h = 0.03125;
const double J = 0.25;
dut_->set_gravitational_acceleration(g);
dut_->set_rod_mass(mass);
dut_->set_mu_coulomb(mu);
dut_->set_rod_half_length(h);
dut_->set_rod_moment_of_inertia(J);
EXPECT_EQ(dut_->get_gravitational_acceleration(), g);
EXPECT_EQ(dut_->get_rod_mass(), mass);
EXPECT_EQ(dut_->get_mu_coulomb(), mu);
EXPECT_EQ(dut_->get_rod_half_length(), h);
EXPECT_EQ(dut_->get_rod_moment_of_inertia(), J);
}
// Verify that impact handling works as expected.
TEST_F(Rod2DDAETest, ImpactWorks) {
// Cause the initial state to be impacting, with center of mass directly
// over the point of contact.
const double half_len = dut_->get_rod_half_length();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0;
xc[1] = half_len;
xc[2] = M_PI_2;
xc[3] = 0.0;
xc[4] = -1.0;
xc[5] = 0.0;
// TODO(edrumwri): Verify that the rod is in an impacting state.
// TODO(edrumwri): Model the impact (as fully inelastic), updating the state.
// TODO(edrumwri): Verify that the state has been modified such that the body
// is no longer in an impacting state and the configuration has not been
// modified.
}
// Verify that derivatives match what we expect from a non-inconsistent,
// ballistic configuration.
TEST_F(Rod2DDAETest, ConsistentDerivativesBallistic) {
// Set the initial state to ballistic motion.
SetBallisticState();
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify that the derivatives match what we expect for this
// non-inconsistent ballistic system.
}
// Verify that derivatives match what we expect from a non-inconsistent
// contacting configuration.
TEST_F(Rod2DDAETest, ConsistentDerivativesContacting) {
// Set the initial state to sustained contact with zero tangential velocity
// at the point of contact.
const double half_len = dut_->get_rod_half_length();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0;
xc[1] = half_len;
xc[2] = M_PI_2;
xc[3] = 0.0;
xc[4] = 0.0;
xc[5] = 0.0;
SetLeftEndpointContacting(&context_->get_mutable_state(), false);
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify that derivatives match what we expect from a
// non-inconsistent contacting configuration. In this case, there is no
// initial sliding, velocity and the rod is oriented vertically, so we expect
// no sliding to begin to occur.
// TODO(edrumwri): Set the coefficient of friction to zero, update the
// sliding velocity, and try again. Derivatives should be exactly the same
// because no frictional force can be applied.
xc[3] = -1.0;
SetLeftEndpointContacting(&context_->get_mutable_state(), true);
dut_->set_mu_coulomb(0.0);
// TODO(edrumwri): Calculate and check derivatives here.
// TODO(edrumwri): Add a large upward force and ensure that the rod
// accelerates upward.
const double fup = 100.0;
const Vector3<double> ext_input(0, fup, 0);
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
// TODO(edrumwri): Calculate and check derivatives here.
}
// Verify that derivatives match what we expect from a sticking contact
// configuration.
TEST_F(Rod2DDAETest, DerivativesContactingAndSticking) {
// Set the initial state to sustained contact with zero tangential velocity
// at the point of contact and the rod being straight up.
const double half_len = dut_->get_rod_half_length();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0;
xc[1] = half_len;
xc[2] = M_PI_2;
xc[3] = 0.0;
xc[4] = 0.0;
xc[5] = 0.0;
// TODO(edrumwri): Set the mode variable appropriately.
// Set a constant horizontal input force, as if applied at the bottom of
// the rod.
const double f_x = 1.0;
const double f_y = -1.0;
const Vector3<double> ext_input(f_x, f_y, f_x * dut_->get_rod_half_length());
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
// Set the coefficient of friction such that the contact forces are right
// on the edge of the friction cone. Determine the predicted normal force
// (this simple formula is dependent upon the upright rod configuration).
const double mu_stick =
f_x /
(dut_->get_rod_mass() * -dut_->get_gravitational_acceleration() - f_y);
dut_->set_mu_coulomb(mu_stick);
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify that derivatives match what we expect: sticking
// should continue.
// Set the coefficient of friction to 99.9% of the sticking value and then
// verify that the contact state transitions from a sticking one to a
// non-sticking one.
const double mu_slide = 0.999 * mu_stick;
dut_->set_mu_coulomb(mu_slide);
// TODO(edrumwri): Calculate and check derivatives here.
// Set the coefficient of friction to zero and try again.
dut_->set_mu_coulomb(0.0);
// TODO(edrumwri): Calculate and check derivatives here.
}
// Verify that the (non-impacting) Painlevé configuration does not result in a
// state change.
TEST_F(Rod2DDAETest, ImpactNoChange) {
// TODO(edrumwri): Verify not impacting at initial state.
// Get the continuous state.
const VectorX<double> xc_old =
context_->get_continuous_state().get_vector().CopyToVector();
// Model the impact and get the continuous state out.
// TODO(edrumwri): Model the impact here.
const VectorX<double> xc =
context_->get_continuous_state().get_vector().CopyToVector();
// TODO(edrumwri): Verify the continuous state did not change.
}
// Verify that applying the impact model to an impacting configuration results
// in a non-impacting configuration. This test exercises the model for the case
// where impulses that yield tangential sticking lie within the friction cone.
TEST_F(Rod2DDAETest, InfFrictionImpactThenNoImpact) {
// Cause the initial state to be impacting.
SetImpactingState();
// Set the coefficient of friction to infinite.
dut_->set_mu_coulomb(std::numeric_limits<double>::infinity());
// Handle the impact and copy the result to the context.
std::unique_ptr<State<double>> new_state = CloneState();
// TODO(edrumwri): Model the impact.
context_->get_mutable_state().SetFrom(*new_state);
// TODO(edrumwri): Verify the state is no longer an impacting one.
// TODO(edrumwri): Attempt to model one more impact- and verify that there is
// no change in the continuous state.
}
// Verify that applying an impact model to an impacting state results in a
// non-impacting state. This test exercises the model for the case
// where impulses that yield tangential sticking lie outside the friction cone.
TEST_F(Rod2DDAETest, NoFrictionImpactThenNoImpact) {
// Set the initial state to be impacting.
SetImpactingState();
// Set the coefficient of friction to zero.
dut_->set_mu_coulomb(0.0);
// Model the impact and copy the result to the context.
std::unique_ptr<State<double>> new_state = CloneState();
// TODO(edrumwri): Model the impact here.
context_->get_mutable_state().SetFrom(*new_state);
// TODO(edrumwri): Verify that the state does not denote impact.
// Do one more impact- there should now be no change.
// TODO(edrumwri): Model the impact here.
// TODO(edrumwri): Verify that there was no further change from the second
// impact.
}
// Verify that no exceptions thrown for a non-sliding configuration.
TEST_F(Rod2DDAETest, NoSliding) {
const double half_len = dut_->get_rod_half_length();
const double r22 = std::sqrt(2) / 2;
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Set the coefficient of friction to zero (triggering the case on the
// edge of the friction cone).
dut_->set_mu_coulomb(0.0);
// This configuration has no sliding velocity.
xc[0] = -half_len * r22;
xc[1] = half_len * r22;
xc[2] = 3 * M_PI / 4.0;
xc[3] = 0.0;
xc[4] = 0.0;
xc[5] = 0.0;
SetLeftEndpointContacting(&context_->get_mutable_state(), false);
// TODO(edrumwri): Verify no impact.
// TODO(edrumwri): Verify no exceptions thrown when calculating derivatives.
// Set the coefficient of friction to infinite.
dut_->set_mu_static(std::numeric_limits<double>::infinity());
dut_->set_mu_coulomb(std::numeric_limits<double>::infinity());
// No exceptions should be thrown.
// TODO(edrumwri): Verify no exceptions thrown when calculating derivatives.
}
// Test multiple (two-point) contact configurations.
TEST_F(Rod2DDAETest, MultiPoint) {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Set the rod to a horizontal, two-contact configuration.
xc[0] = 0;
xc[1] = 0;
xc[2] = 0;
// Set the velocity on the rod such that it is moving horizontally.
xc[3] = 1.0;
// TODO(edrumwri): Verify no impact.
// Set the coefficient of friction to zero.
dut_->set_mu_coulomb(0.0);
// Compute the derivatives and verify that the linear and angular acceleration
// are approximately zero.
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify derivatives(3:5) are all approximately zero.
// Set the coefficient of friction to "very large".
const double large = 100.0;
dut_->set_mu_coulomb(large);
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify derivatives(3) is approximately (scaled by the
// magnitude of large) equal to the graviational acceleration.
// TODO(edrumwri): Verify derivatives(4:5) are all approximately zero.
// Set the rod velocity to zero.
xc[3] = 0.0;
// TODO(edrumwri): Verify no impact.
// Set a constant force pushing the rod.
const double fX = 1.0;
const Vector3<double> ext_input(fX, 0, 0);
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
// Verify that the linear and angular acceleration are still zero.
// TODO(edrumwri): Calculate the derivatives and verify derivatives(3:5) are
// all approximately zero.
// Set the coefficient of friction to zero. Now the force should result
// in the rod being pushed to the right.
dut_->set_mu_coulomb(0.0);
// TODO(edrumwri): Calculate the derivatives.
// TODO(edrumwri): Verify derivatives(3) is approximately equal to the
// fX divided by the rod mass.
// TODO(edrumwri): Verify derivatives(4:5) are all approximately zero.
}
// Verify that the Painlevé configuration does not correspond to an impacting
// state.
TEST_F(Rod2DDAETest, ImpactNoChange2) {
SetSecondInitialConfig();
// TODO(edrumwri): Model the impact.
// TODO(edrumwri): Verify the state has not changed.
}
// Verify that applying the impact model to an impacting state results
// in a non-impacting state.
TEST_F(Rod2DDAETest, InfFrictionImpactThenNoImpact2) {
// Set writable state.
std::unique_ptr<State<double>> new_state = CloneState();
// Cause the initial state to be impacting.
SetImpactingState();
// Set the coefficient of friction to infinite.
dut_->set_mu_coulomb(std::numeric_limits<double>::infinity());
// Model the impact and copy the result to the context.
// TODO(edrumwri): Model the impact.
context_->get_mutable_state().SetFrom(*new_state);
// TODO(edrumwri): Verify the state no longer corresponds to an impact.
// Model one more impact- there should now be no change.
// TODO(edrumwri): Model the impact.
// TODO(edrumwri): Verify the state has not changed.
}
// Verify that applying the impact model to an impacting state results in a
// non-impacting state.
TEST_F(Rod2DDAETest, NoFrictionImpactThenNoImpact2) {
// Set writable state.
std::unique_ptr<State<double>> new_state = CloneState();
// Cause the initial state to be impacting.
SetImpactingState();
// TODO(edrumwri):
// Set the coefficient of friction to zero.
dut_->set_mu_coulomb(0.0);
// Model the impact and copy the result to the context.
// TODO(edrumwri): Model the impact.
context_->get_mutable_state().SetFrom(*new_state);
// TODO(edrumwri): Verify that the state does not correspond to an impact.
// Do one more impact- there should now be no change.
// TODO(edrumwri): Model the impact.
// TODO(edrumwri): Verify the state has not changed.
}
// Verifies that rod in a ballistic state does not correspond to an impact.
TEST_F(Rod2DDAETest, BallisticNoImpact) {
// Cause the initial state to be impacting.
SetImpactingState();
// Move the rod upward vertically so that it is no longer impacting.
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[1] += 10.0;
// TODO(edrumwri): Verify that no impact occurs.
EXPECT_FALSE(dut_->IsImpacting(*context_));
}
// Verifies that the rigid contact problem data has reasonable values when the
// rod is in a ballistic state.
TEST_F(Rod2DDAETest, RigidContactProblemDataBallistic) {
SetBallisticState();
// Compute the problem data.
ConstraintAccelProblemData<double> data(get_rod_num_coordinates());
CalcConstraintAccelProblemData(&data);
// Verify that the data has reasonable values.
const int num_contacts = 0;
CheckProblemConsistency(data, num_contacts);
}
// Verifies that the rigid contact problem data has reasonable values when the
// rod is in a two-contact, at-rest configuration.
TEST_F(Rod2DDAETest, RigidContactProblemDataHorizontalResting) {
// Set the rod to a resting horizontal configuration.
SetRestingHorizontalConfig();
// Compute the problem data.
ConstraintAccelProblemData<double> data(3 /* gen. vel. dim */);
CalcConstraintAccelProblemData(&data);
const int num_contacts = 2;
CheckProblemConsistency(data, num_contacts);
// TODO(edrumwri): Verify that both contacts are not sliding.
}
// Verifies that the rigid contact problem data has reasonable values when the
// rod is in a two-contact, sliding configuration.
TEST_F(Rod2DDAETest, RigidContactProblemDataHorizontalSliding) {
// Set the rod to a sliding horizontal configuration.
SetRestingHorizontalConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = 1.0; // horizontal velocity of the rod center-of-mass.
// Compute the problem data.
ConstraintAccelProblemData<double> data(3 /* gen. vel. dim */);
CalcConstraintAccelProblemData(&data);
const int num_contacts = 2;
CheckProblemConsistency(data, num_contacts);
// TODO(edrumwri): Verify that both contacts are sliding.
}
// Verifies that the rigid contact problem data has reasonable values when the
// rod is in a single-contact, at-rest configuration.
TEST_F(Rod2DDAETest, RigidContactProblemDataVerticalResting) {
// Set the rod to a resting vertical configuration.
SetRestingVerticalConfig();
// Compute the problem data.
ConstraintAccelProblemData<double> data(3 /* gen. vel. dim */);
CalcConstraintAccelProblemData(&data);
const int num_contacts = 1;
CheckProblemConsistency(data, num_contacts);
// TODO(edrumwri): Verify that contact is not sliding.
// TODO(edrumwri): Most tests are necessary to better stress the validity of
// N, N - μQ, F, dN/dt⋅v, and dF/dt⋅v.
// TODO(edrumwri): Verify that N has no angular component.
}
// Verifies that the rigid contact problem data has reasonable values when the
// rod is in a two-contact, sliding configuration.
TEST_F(Rod2DDAETest, RigidContactProblemDataVerticalSliding) {
// Set the rod to a sliding vertical configuration.
SetRestingVerticalConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = 1.0;
// Compute the problem data.
ConstraintAccelProblemData<double> data(3 /* gen. vel. dim */);
CalcConstraintAccelProblemData(&data);
const int num_contacts = 1;
CheckProblemConsistency(data, num_contacts);
// TODO(edrumwri): Verify that contact is sliding.
}
/// Class for testing the Rod 2D example using a first order time
/// stepping approach.
class Rod2DDiscretizedTest : public ::testing::Test {
protected:
void SetUp() override {
const double dt = 1e-2;
dut_ = std::make_unique<Rod2D<double>>(
Rod2D<double>::SystemType::kDiscretized, dt);
context_ = dut_->CreateDefaultContext();
output_ = dut_->AllocateOutput();
// Use a non-unit mass.
dut_->set_rod_mass(2.0);
// Set a zero input force (this is the default).
const Vector3<double> ext_input(0, 0, 0);
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
}
BasicVector<double>& mutable_discrete_state() {
return context_->get_mutable_discrete_state(0);
}
// Sets a secondary initial Rod2D configuration.
void SetSecondInitialConfig() {
// Set the configuration to an inconsistent (Painlevé) type state with
// the rod at a 135 degree counter-clockwise angle with respect to the
// x-axis. The rod in [Stewart, 2000] is at a 45 degree counter-clockwise
// angle with respect to the x-axis.
// * [Stewart, 2000] D. Stewart, "Rigid-Body Dynamics with Friction and
// Impact". SIAM Rev., 42(1), 3-39, 2000.
using std::sqrt;
const double half_len = dut_->get_rod_half_length();
const double r22 = std::sqrt(2) / 2;
auto xd = mutable_discrete_state().get_mutable_value();
xd[0] = -half_len * r22;
xd[1] = half_len * r22;
xd[2] = 3 * M_PI / 4.0;
xd[3] = 1.0;
xd[4] = 0.0;
xd[5] = 0.0;
}
std::unique_ptr<Rod2D<double>> dut_; //< The device under test.
std::unique_ptr<Context<double>> context_;
std::unique_ptr<SystemOutput<double>> output_;
std::unique_ptr<ContinuousState<double>> derivatives_;
};
/// Verify that Rod 2D system eventually goes to rest using the
/// first-order discretization approach (this tests expected meta behavior).
TEST_F(Rod2DDiscretizedTest, RodGoesToRest) {
// Set the initial state to an inconsistent configuration.
SetSecondInitialConfig();
// Init the simulator.
Simulator<double> simulator(*dut_, std::move(context_));
// Integrate forward to a point where the rod should be at rest.
const double t_final = 10;
simulator.AdvanceTo(t_final);
// Get angular orientation and velocity.
const VectorXd& xd = simulator.get_context().get_discrete_state(0).value();
const double theta = xd(2);
const double theta_dot = xd(5);
// After sufficiently long, theta should be 0 or M_PI and the velocity
// should be nearly zero.
EXPECT_TRUE(std::fabs(theta) < 1e-6 || std::fabs(theta - M_PI) < 1e-6);
EXPECT_NEAR(theta_dot, 0.0, 1e-6);
}
// Validates the number of witness functions is determined correctly.
TEST_F(Rod2DDiscretizedTest, NumWitnessFunctions) {
EXPECT_EQ(dut_->DetermineNumWitnessFunctions(*context_), 0);
}
// This test checks to see whether a single semi-explicit step of the piecewise
// DAE based Rod2D system is equivalent to a single step of the semi-explicit
// time stepping based system.
GTEST_TEST(Rod2DCrossValidationTest, OneStepSolutionSliding) {
// Create two Rod2D systems.
const double dt = 1e-1;
Rod2D<double> ts(Rod2D<double>::SystemType::kDiscretized, dt);
Rod2D<double> pdae(Rod2D<double>::SystemType::kPiecewiseDAE, 0.0);
// Set the coefficient of friction to a small value for both.
const double mu = 0.01;
ts.set_mu_coulomb(mu);
pdae.set_mu_coulomb(mu);
// Set "one step" constraint stabilization (not generally recommended, but
// works for a single step) and small regularization.
const double cfm = std::numeric_limits<double>::epsilon();
const double erp = 1.0;
ts.SetStiffnessAndDissipation(cfm, erp);
// Create contexts for both.
std::unique_ptr<Context<double>> context_ts = ts.CreateDefaultContext();
std::unique_ptr<Context<double>> context_pdae = pdae.CreateDefaultContext();
// Set zero input forces for both.
Vector3<double> fext(0, 0, 0);
ts.get_input_port(0).FixValue(context_ts.get(), fext);
pdae.get_input_port(0).FixValue(context_pdae.get(), fext);
// Init the simulator for the discretized (time stepping) system.
Simulator<double> simulator_ts(ts, std::move(context_ts));
// Integrate forward by a single *large* dt. Note that the update rate
// is set by the time stepping system, so stepping to dt should yield
// exactly one step.
simulator_ts.AdvanceTo(dt);
EXPECT_EQ(simulator_ts.get_num_discrete_updates(), 1);
// Manually integrate the continuous state forward for the piecewise DAE
// based approach.
std::unique_ptr<ContinuousState<double>> f = pdae.AllocateTimeDerivatives();
// TODO(edrumwri): Calculate the derivatives here.
auto& xc = context_pdae->get_mutable_continuous_state_vector();
xc.SetAtIndex(3, xc.GetAtIndex(3) + dt * ((*f)[3]));
xc.SetAtIndex(4, xc.GetAtIndex(4) + dt * ((*f)[4]));
xc.SetAtIndex(5, xc.GetAtIndex(5) + dt * ((*f)[5]));
xc.SetAtIndex(0, xc.GetAtIndex(0) + dt * xc.GetAtIndex(3));
xc.SetAtIndex(1, xc.GetAtIndex(1) + dt * xc.GetAtIndex(4));
xc.SetAtIndex(2, xc.GetAtIndex(2) + dt * xc.GetAtIndex(5));
// TODO(edrumwri): Check that the solution is nearly identical.
// TODO(edrumwri): Introduce more extensive tests that cross-validate the
// time-stepping based approach against the piecewise DAE-based approach for
// the case of sliding contacts at multiple points.
}
// This test checks to see whether a single semi-explicit step of the piecewise
// DAE based Rod2D system is equivalent to a single step of the semi-explicit
// time stepping based system for a sticking contact scenario.
GTEST_TEST(Rod2DCrossValidationTest, OneStepSolutionSticking) {
// Create two Rod2D systems.
const double dt = 1e-1;
Rod2D<double> ts(Rod2D<double>::SystemType::kDiscretized, dt);
Rod2D<double> pdae(Rod2D<double>::SystemType::kPiecewiseDAE, 0.0);
// Set the coefficient of friction to a large value for both.
const double mu = 100.0;
ts.set_mu_coulomb(mu);
pdae.set_mu_coulomb(mu);
// Set "one step" constraint stabilization (not generally recommended, but
// works for a single step) and small regularization.
const double cfm = std::numeric_limits<double>::epsilon();
const double erp = 1.0;
ts.SetStiffnessAndDissipation(cfm, erp);
// Create contexts for both.
std::unique_ptr<Context<double>> context_ts = ts.CreateDefaultContext();
std::unique_ptr<Context<double>> context_pdae = pdae.CreateDefaultContext();
// This configuration has no sliding velocity.
const double half_len = pdae.get_rod_half_length();
ContinuousState<double>& xc = context_pdae->get_mutable_continuous_state();
auto xd = context_ts->get_mutable_discrete_state(0).get_mutable_value();
xc[0] = xd[0] = 0.0;
xc[1] = xd[1] = half_len;
xc[2] = xd[2] = M_PI_2;
xc[3] = xd[3] = 0.0;
xc[4] = xd[4] = 0.0;
xc[5] = xd[5] = 0.0;
// Set constant input forces for both.
const double x = 1.0;
Vector3<double> fext(x, 0, x * ts.get_rod_half_length());
ts.get_input_port(0).FixValue(context_ts.get(), fext);
pdae.get_input_port(0).FixValue(context_pdae.get(), fext);
// Init the simulator for the time stepping system.
Simulator<double> simulator_ts(ts, std::move(context_ts));
// Integrate forward by a single *large* dt. Note that the update rate
// is set by the discretized system, so stepping to dt should yield
// exactly one step.
simulator_ts.AdvanceTo(dt);
EXPECT_EQ(simulator_ts.get_num_discrete_updates(), 1);
// TODO(edrumwri): Manually integrate the continuous state forward for the
// piecewise DAE based approach.
std::unique_ptr<ContinuousState<double>> f = pdae.AllocateTimeDerivatives();
// TODO(edrumwri): Compute derivatives here.
xc[3] += +dt * ((*f)[3]);
xc[4] += +dt * ((*f)[4]);
xc[5] += +dt * ((*f)[5]);
xc[0] += +dt * xc[3];
xc[1] += +dt * xc[4];
xc[2] += +dt * xc[5];
// TODO(edrumwri): Check that the solution is nearly identical.
}
/// Class for testing the Rod 2D example using compliant contact
/// thus permitting integration as an ODE.
class Rod2DContinuousTest : public ::testing::Test {
protected:
void SetUp() override {
dut_ = std::make_unique<Rod2D<double>>(
Rod2D<double>::SystemType::kContinuous, 0.0);
context_ = dut_->CreateDefaultContext();
output_ = dut_->AllocateOutput();
derivatives_ = dut_->AllocateTimeDerivatives();
// Use a non-unit mass.
dut_->set_rod_mass(2.0);
// Using default compliant contact parameters.
// Set a zero input force (this is the default).
const Vector3<double> ext_input(0, 0, 0);
dut_->get_input_port(0).FixValue(context_.get(), ext_input);
}
// Calculate time derivatives using the context member and writing to
// the derivatives member.
void CalcTimeDerivatives() {
dut_->CalcTimeDerivatives(*context_, derivatives_.get());
}
std::unique_ptr<State<double>> CloneState() const {
return context_->CloneState();
}
// Return the state x,y,θ,xdot,ydot,θdot as a Vector6.
Vector6d get_state() const {
const ContinuousState<double>& xc = context_->get_continuous_state();
return Vector6d(xc.CopyToVector());
}
// Return d/dt state xdot,ydot,θdot,xddot,yddot,θddot as a Vector6.
Vector6d get_state_dot() const {
const ContinuousState<double>& xcd = *derivatives_;
return Vector6d(xcd.CopyToVector());
}
// Sets the planar pose in the context.
void set_pose(double x, double y, double theta) {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = x;
xc[1] = y;
xc[2] = theta;
}
// Sets the planar velocity in the context.
void set_velocity(double xdot, double ydot, double thetadot) {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = xdot;
xc[4] = ydot;
xc[5] = thetadot;
}
// Returns planar pose derivative (should be planar velocities xdot,
// ydot, thetadot).
Vector3d get_pose_dot() const {
const ContinuousState<double>& xcd = *derivatives_;
return Vector3d(xcd[0], xcd[1], xcd[2]);
}
// Returns planar acceleration (xddot, yddot, thetaddot).
Vector3d get_accelerations() const {
const ContinuousState<double>& xcd = *derivatives_;
return Vector3d(xcd[3], xcd[4], xcd[5]);
}
// Sets the rod to a state that corresponds to ballistic motion.
void SetBallisticState() {
const double half_len = dut_->get_rod_half_length();
set_pose(0, 10 * half_len, M_PI_2);
set_velocity(1, 2, 3);
}
// Sets the rod to a perfectly vertical position in which either the left or
// right endpoint is contacting, or a perfectly horizontal position in which
// both endpoints are contacting. In all cases the penetration depth into the
// halfplane is set to 1 cm, and the rod velocity is set to zero.
// k=-1,0,1 -> left, both, right.
void SetContactingState(int k) {
DRAKE_DEMAND(-1 <= k && k <= 1);
const double half_len = dut_->get_rod_half_length();
const double penetration = 0.01; // 1 cm
set_pose(0, std::abs(k) * half_len - penetration, -k * M_PI_2);
set_velocity(0, 0, 0);
}
std::unique_ptr<Rod2D<double>> dut_; //< The device under test.
std::unique_ptr<Context<double>> context_;
std::unique_ptr<SystemOutput<double>> output_;
std::unique_ptr<ContinuousState<double>> derivatives_;
};
/// Verify that the compliant contact resists penetration.
TEST_F(Rod2DContinuousTest, ForcesHaveRightSign) {
// We expect only roundoff errors, scaled by force magnitude (~1e-14).
const double kTightTol = 50 * std::numeric_limits<double>::epsilon();
SetContactingState(-1); // left
Vector3d F_Ro_W_left = dut_->CalcCompliantContactForces(*context_);
CalcTimeDerivatives();
Vector6d xcd = get_state_dot();
EXPECT_EQ(xcd[0], 0); // xdot, ydot, thetadot
EXPECT_EQ(xcd[1], 0);
EXPECT_EQ(xcd[2], 0);
// Total acceleration is gravity plus acceleration due to contact forces;
// extract just the contact contribution. It should point up!
const double a_contact = xcd[4] - dut_->get_gravitational_acceleration();
// Vertical acceleration is >> 1; just checking for correct sign. Rod is
// vertical so horizontal and angular accelerations are zero.
EXPECT_NEAR(xcd[3], 0, kTightTol); // no x acceleration
EXPECT_GT(a_contact, 1.); // + y acceleration
EXPECT_NEAR(xcd[5], 0, kTightTol); // no angular acceleration
// Now add some downward velocity; that should *increase* the force we
// calculated above from just penetration. We're checking that the sign is
// correct by making the penetration rate large enough to at least double
// the overall force.
set_velocity(0, -10, 0);
Vector3d F_Ro_W_ldown = dut_->CalcCompliantContactForces(*context_);
EXPECT_GT(F_Ro_W_ldown[1], 2 * F_Ro_W_left[1]); // Did it double?
// An extreme upwards velocity should be a "pull out" situation resulting
// in (exactly) zero force rather than a negative force.
set_velocity(0, 1000, 0);
Vector3d F_Ro_W_lup = dut_->CalcCompliantContactForces(*context_);
EXPECT_TRUE(F_Ro_W_lup == Vector3d::Zero());
// Sliding -x should produce a +x friction force and a positive torque;
// no effect on y force.
set_velocity(-10, 0, 0);
Vector3d F_Ro_W_nx = dut_->CalcCompliantContactForces(*context_);
EXPECT_GT(F_Ro_W_nx[0], 1.);
EXPECT_NEAR(F_Ro_W_nx[1], F_Ro_W_left[1], kTightTol);
EXPECT_GT(F_Ro_W_nx[2], 1.);
// Sliding +x should produce a -x friction force and a negative torque;
// no effect on y force.
set_velocity(10, 0, 0);
Vector3d F_Ro_W_px = dut_->CalcCompliantContactForces(*context_);
EXPECT_LT(F_Ro_W_px[0], -1.);
EXPECT_NEAR(F_Ro_W_px[1], F_Ro_W_left[1], kTightTol);
EXPECT_LT(F_Ro_W_px[2], -1.);
SetContactingState(1); // Right should behave same as left.
Vector3d F_Ro_W_right = dut_->CalcCompliantContactForces(*context_);
EXPECT_TRUE(F_Ro_W_right.isApprox(F_Ro_W_left, kTightTol));
// With both ends in contact the force should double and there should
// be zero moment.
SetContactingState(0);
Vector3d F_Ro_W_both = dut_->CalcCompliantContactForces(*context_);
EXPECT_TRUE(F_Ro_W_both.isApprox(F_Ro_W_left + F_Ro_W_right, kTightTol));
EXPECT_NEAR(F_Ro_W_both[2], 0., kTightTol);
}
// Validates the number of witness functions is determined correctly.
TEST_F(Rod2DContinuousTest, NumWitnessFunctions) {
EXPECT_EQ(dut_->DetermineNumWitnessFunctions(*context_), 0);
}
// Verifies that output ports give expected values.
GTEST_TEST(Rod2DCrossValidationTest, Outputs) {
// Create two Rod2D systems, one time stepping (i.e., discretized),
// one with continuous state.
const double dt = 1e-1;
Rod2D<double> ts(Rod2D<double>::SystemType::kDiscretized, dt);
Rod2D<double> pdae(Rod2D<double>::SystemType::kPiecewiseDAE, 0.0);
// Create contexts for both.
std::unique_ptr<Context<double>> context_ts = ts.CreateDefaultContext();
std::unique_ptr<Context<double>> context_pdae = pdae.CreateDefaultContext();
// Allocate outputs for both.
auto output_ts = ts.AllocateOutput();
auto output_pdae = pdae.AllocateOutput();
// Compute outputs.
ts.CalcOutput(*context_ts, output_ts.get());
pdae.CalcOutput(*context_pdae, output_pdae.get());
// Set port indices.
const int state_port = 0;
// Verify that state outputs are identical.
const double eq_tol = 10 * std::numeric_limits<double>::epsilon();
const VectorXd x_ts = output_ts->get_vector_data(state_port)->CopyToVector();
VectorXd x_pdae = output_pdae->get_vector_data(state_port)->CopyToVector();
EXPECT_LT((x_ts - x_pdae).lpNorm<Eigen::Infinity>(), eq_tol);
// Transform the rod and verify that pose output is as expected.
x_pdae[0] = 0;
x_pdae[1] = pdae.get_rod_half_length();
x_pdae[2] = M_PI_2;
context_pdae->SetContinuousState(x_pdae);
pdae.CalcOutput(*context_pdae, output_pdae.get());
}
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/rod2d | /home/johnshepherd/drake/examples/rod2d/test/constraint_solver_test.cc | #include "drake/examples/rod2d/constraint_solver.h"
#include <cmath>
#include <memory>
#include <gtest/gtest.h>
#include "drake/common/drake_assert.h"
#include "drake/common/ssize.h"
#include "drake/examples/rod2d/rod2d.h"
#include "drake/solvers/unrevised_lemke_solver.h"
using drake::examples::rod2d::Rod2D;
using drake::systems::BasicVector;
using drake::systems::Context;
using drake::systems::ContinuousState;
using Vector2d = Eigen::Vector2d;
namespace drake {
namespace examples {
namespace rod2d {
namespace {
class Constraint2DSolverTest : public ::testing::Test {
protected:
void SetUp() override {
rod_ = std::make_unique<Rod2D<double>>(
Rod2D<double>::SystemType::kPiecewiseDAE, 0);
context_ = rod_->CreateDefaultContext();
// Use a non-unit mass.
rod_->set_rod_mass(2.0);
// Set a zero input force (this is the default).
const Vector3<double> ext_input(0.0, 0.0, 0.0);
rod_->get_input_port(0).FixValue(context_.get(), ext_input);
// Construct the problem data for the 2D rod.
const int num_velocities = 3;
accel_data_ =
std::make_unique<ConstraintAccelProblemData<double>>(num_velocities);
vel_data_ =
std::make_unique<ConstraintVelProblemData<double>>(num_velocities);
// Set epsilon for floating point tolerance testing; due to rounding error
// in the LCP solver, this is approximately the tightest tolerance with
// which the tests will still pass.
eps_ = 100 * std::numeric_limits<double>::epsilon();
}
// Zero tolerance for results depending on LCP solve (< 0 indicates not set).
double eps_{-1};
ConstraintSolver<double> solver_;
std::unique_ptr<Rod2D<double>> rod_;
std::unique_ptr<Context<double>> context_;
std::unique_ptr<ConstraintAccelProblemData<double>> accel_data_;
std::unique_ptr<ConstraintVelProblemData<double>> vel_data_;
const bool kForceAppliedToLeft = false;
const bool kForceAppliedToRight = true;
const bool kSlideLeft = false;
const bool kSlideRight = true;
const bool kLinearSystemSolver = false;
const bool kLCPSolver = true;
// Gets the frame for a sliding contact.
Matrix2<double> GetSlidingContactFrameToWorldTransform(
double xaxis_velocity) const {
const double dir = (xaxis_velocity > 0) ? 1 : -1;
const Matrix2<double> R =
rod_->GetSlidingContactFrameToWorldTransform(xaxis_velocity);
// Verify that the x-axis of the contact frame, which corresponds to
// the contact normal, points along the world y-axis, and the y-axis
// of the contact frame, which corresponds to the contact sliding direction
// vector, along the x-axis or negative x-axis.
EXPECT_LT(std::fabs(R.col(0).dot(Vector2<double>::UnitY()) - 1.0),
std::numeric_limits<double>::epsilon());
EXPECT_LT(std::fabs(R.col(1).dot(Vector2<double>::UnitX()) - dir),
std::numeric_limits<double>::epsilon());
return R;
}
// Gets the frame for a non-sliding contact.
Matrix2<double> GetNonSlidingContactFrameToWorldTransform() const {
const Matrix2<double> R = rod_->GetNonSlidingContactFrameToWorldTransform();
// Verify that the x-axis of the contact frame, which corresponds to
// the contact normal, points along the world y-axis, and the y-axis
// of the contact frame, which corresponds to a contact tangent
// vector, along the world x-axis.
EXPECT_LT(std::fabs(R.col(0).dot(Vector2<double>::UnitY()) - 1.0),
std::numeric_limits<double>::epsilon());
EXPECT_LT(std::fabs(R.col(1).dot(Vector2<double>::UnitX()) - 1.0),
std::numeric_limits<double>::epsilon());
return R;
}
// Sets the rod to a resting horizontal configuration without modifying the
// rod's mode variables.
void SetRodToRestingHorizontalConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = 0.0; // com vertical position
xc[2] = 0.0; // rod rotation
xc[3] = xc[4] = xc[5] = 0.0; // velocity variables
}
// Sets the rod to an upward moving horizontal configuration without modifying
// the rod's mode variables.
void SetRodToUpwardMovingHorizontalConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = 0.0; // com vertical position
xc[2] = 0.0; // rod rotation
xc[3] = 0.0; // no horizontal velocity.
xc[4] = 1.0; // upward velocity.
xc[5] = 0.0; // no angular velocity.
}
// Sets the rod to an impacting, sliding velocity with the rod
// configured to lie upon its side and without modifying the rod's mode
// variables.
void SetRodToSlidingImpactingHorizontalConfig(bool sliding_to_right) {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = 0.0; // com vertical position
xc[2] = 0.0; // rod rotation
xc[3] = (sliding_to_right) ? 1 : -1; // sliding horizontal velocity.
xc[4] = -1.0; // impacting velocity.
xc[5] = 0.0; // no angular velocity.
}
// Sets the rod to a sliding velocity with the rod configured to impact
// vertically and without modifying the rod's mode variables.
void SetRodToSlidingImpactingVerticalConfig(bool sliding_to_right) {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
// Configuration has the rod on its side.
xc[0] = 0.0; // com horizontal position
xc[1] = 0.0; // com vertical position
xc[2] = M_PI_2; // rod rotation
xc[3] = (sliding_to_right) ? 1 : -1; // sliding horizontal velocity.
xc[4] = -1.0; // impacting velocity.
xc[5] = 0.0; // no angular velocity.
}
// Sets the rod to a resting vertical configuration without modifying the
// mode variables.
void SetRodToRestingVerticalConfig() {
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0; // com horizontal position
xc[1] = rod_->get_rod_half_length(); // com vertical position
xc[2] = M_PI_2; // rod rotation
xc[3] = xc[4] = xc[5] = 0.0; // velocity variables
}
// Computes rigid contact data, duplicating contact points and friction
// directions, as specified.
// @param contact_points_dup the number of times (>= 0) each contact point
// should be duplicated in the contact data.
// @param friction_directions_dup the number of times (>= 0) that each
// friction basis direction should be duplicated. Since the 2D tests
// only use one friction direction, duplication ensures that the
// algorithms are able to handle duplicated directions without error.
void CalcConstraintAccelProblemData(ConstraintAccelProblemData<double>* data,
int contact_points_dup,
int friction_directions_dup) {
DRAKE_DEMAND(contact_points_dup >= 0);
DRAKE_DEMAND(friction_directions_dup >= 0);
// Reset constraint acceleration data.
const int num_velocities = 3;
*data = ConstraintAccelProblemData<double>(num_velocities);
// Get the points of contact from Rod2D.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
// Duplicate the contact points.
std::vector<Vector2d> contacts_dup;
for (int i = 0; i < ssize(contacts); ++i) {
for (int j = 0; j < contact_points_dup; ++j)
contacts_dup.push_back(contacts[i]);
}
contacts.insert(contacts.end(), contacts_dup.begin(), contacts_dup.end());
// Get the contact tangent velocities.
rod_->GetContactPointsTangentVelocities(*context_, contacts, &tangent_vels);
// Compute the problem data.
rod_->CalcConstraintProblemData(*context_, contacts, tangent_vels, data);
// Get old F.
const int num_fdir = std::accumulate(data->r.begin(), data->r.end(), 0);
const int ngc = get_rod_num_coordinates();
MatrixX<double> Fold = MatrixX<double>::Zero(num_fdir, ngc);
for (int j = 0; j < ngc; ++j)
Fold.col(j) = data->F_mult(VectorX<double>::Unit(ngc, j));
// Determine new F by duplicating each row the specified number of times.
const int new_friction_directions = friction_directions_dup + 1;
MatrixX<double> F(
new_friction_directions * data->non_sliding_contacts.size(), ngc);
for (int i = 0, k = 0; i < Fold.rows(); ++i)
for (int j = 0; j < new_friction_directions; ++j)
F.row(k++) = Fold.row(i);
// Redetermine F_mult and F_transpose_mult operator lambdas.
data->F_mult = [F](const VectorX<double>& w) -> VectorX<double> {
return F * w;
};
data->F_transpose_mult = [F](const VectorX<double>& w) -> VectorX<double> {
return F.transpose() * w;
};
// Update r with the new friction directions.
for (int i = 0; i < ssize(data->r); ++i)
data->r[i] = new_friction_directions;
// Resize kF (recall the vector always is zero for this 2D problem), gammaF,
// and gammaE.
data->kF.setZero(data->non_sliding_contacts.size() *
new_friction_directions);
// Add in empty rows to G, by default, allowing us to verify that no
// constraint forces are added (and that solution method is robust to
// unnecessary constraints).
data->G_mult = [](const VectorX<double>& w) -> VectorX<double> {
return VectorX<double>::Zero(1);
};
data->G_transpose_mult =
[ngc](const VectorX<double>& w) -> VectorX<double> {
return VectorX<double>::Zero(ngc);
};
data->kG.setZero(0);
// Check the consistency of the data.
CheckProblemConsistency(*data, contacts.size());
}
// Computes velocity data for solving acceleration problems. This allows us
// to compare the first-order discretized solutions (with rigid contact) and
// force/velocity constraints against the force/acceleration solutions using
// force/acceleration constraints.
// @param contact_points_dup the number of times (>= 0) each contact point
// should be duplicated in the contact data.
// @param friction_directions_dup the number of times (>= 0) that each
// friction basis direction should be duplicated. Since the 2D tests
// only use one friction direction, duplication ensures that the
// algorithms are able to handle duplicated directions without error.
void CalcDiscreteTimeProblemData(double dt,
ConstraintVelProblemData<double>* data,
int contact_points_dup,
int friction_directions_dup) {
// Use the constraint velocity data to do most of the work.
CalcConstraintProblemDataForImpact(data, contact_points_dup,
friction_directions_dup);
// Set the normal compliance and damping to yield truly rigid contact.
const double stiffness = std::numeric_limits<double>::infinity();
const double damping = 0.0;
// Compute the softening coefficients.
const double denom = dt * stiffness + damping;
const double cfm = 1.0 / denom;
data->gammaN.setOnes() *= cfm;
// Add in gravitational forces.
const double grav_accel = rod_->get_gravitational_acceleration();
data->Mv += Vector3<double>(0, grav_accel * rod_->get_rod_mass(), 0) * dt;
}
void SolveDiscretizationProblem(
const ConstraintVelProblemData<double>& problem_data, double dt,
VectorX<double>* cf) {
DRAKE_DEMAND(cf != nullptr);
VectorX<double> qq, a;
MatrixX<double> MM;
ConstraintSolver<double>::MlcpToLcpData mlcp_to_lcp_data;
solver_.ConstructBaseDiscretizedTimeLcp(problem_data, &mlcp_to_lcp_data,
&MM, &qq);
solver_.UpdateDiscretizedTimeLcp(problem_data, dt, &mlcp_to_lcp_data, &a,
&MM, &qq);
// Determine the zero tolerance.
solvers::UnrevisedLemkeSolver<double> lcp;
const double zero_tol = lcp.ComputeZeroTolerance(MM);
// Attempt to solve the linear complementarity problem.
int num_pivots;
VectorX<double> zz;
const bool success = lcp.SolveLcpLemke(MM, qq, &zz, &num_pivots);
VectorX<double> ww = MM * zz + qq;
double max_dot =
(zz.size() > 0) ? (zz.array() * ww.array()).abs().maxCoeff() : 0.0;
// Check for success.
const int num_vars = qq.size();
ASSERT_TRUE(success);
ASSERT_TRUE(zz.size() == 0 || zz.minCoeff() > -num_vars * zero_tol);
ASSERT_TRUE(ww.size() == 0 || ww.minCoeff() > -num_vars * zero_tol);
ASSERT_TRUE(zz.size() == 0 || max_dot < std::max(1.0, zz.maxCoeff()) *
std::max(1.0, ww.maxCoeff()) *
num_vars * zero_tol);
// Compute the packed constraint forces.
solver_.PopulatePackedConstraintForcesFromLcpSolution(
problem_data, mlcp_to_lcp_data, zz, a, dt, cf);
}
// Computes rigid contact data without any duplication of contact points or
// friction directions.
void CalcConstraintAccelProblemData(
ConstraintAccelProblemData<double>* data) {
CalcConstraintAccelProblemData(data,
0, // no contacts duplicated
0); // no friction directions duplicated
}
// Computes constraint data without duplicate contacts or friction directions.
void CalcDiscreteTimeProblemData(double dt,
ConstraintVelProblemData<double>* data) {
CalcDiscreteTimeProblemData(dt, data, 0, 0);
}
// Computes rigid impacting contact data.
// @param contact_points_dup the number of times (>= 0) each contact point
// should be duplicated in the contact data.
// @param friction_directions_dup the number of times (>= 0) that each
// friction basis direction should be duplicated. Since the 2D tests
// only use one friction direction, duplication ensures that the
// algorithms are able to handle duplicated directions without error.
void CalcConstraintProblemDataForImpact(
ConstraintVelProblemData<double>* data, int contact_points_dup,
int friction_directions_dup) {
DRAKE_DEMAND(contact_points_dup >= 0);
DRAKE_DEMAND(friction_directions_dup >= 0);
// Reset constraint acceleration data.
const int num_velocities = 3;
*data = ConstraintVelProblemData<double>(num_velocities);
// Get the points of contact from Rod2D.
std::vector<Vector2d> contacts;
rod_->GetContactPoints(*context_, &contacts);
// Duplicate the contact points.
std::vector<Vector2d> contacts_dup;
for (int i = 0; i < ssize(contacts); ++i) {
for (int j = 0; j < contact_points_dup; ++j)
contacts_dup.push_back(contacts[i]);
}
contacts.insert(contacts.end(), contacts_dup.begin(), contacts_dup.end());
// Compute the problem data.
rod_->CalcImpactProblemData(*context_, contacts, data);
// Get old F.
const int num_fdir = std::accumulate(data->r.begin(), data->r.end(), 0);
const int ngc = get_rod_num_coordinates();
MatrixX<double> Fold = MatrixX<double>::Zero(num_fdir, ngc);
for (int j = 0; j < ngc; ++j)
Fold.col(j) = data->F_mult(VectorX<double>::Unit(ngc, j));
// Determine new F by duplicating each row the specified number of times.
const int new_friction_directions = friction_directions_dup + 1;
MatrixX<double> F(new_friction_directions * contacts.size(), ngc);
for (int i = 0, k = 0; i < Fold.rows(); ++i)
for (int j = 0; j < new_friction_directions; ++j)
F.row(k++) = Fold.row(i);
// Redetermine F_mult and F_transpose_mult operator lambdas.
data->F_mult = [F](const VectorX<double>& w) -> VectorX<double> {
return F * w;
};
data->F_transpose_mult = [F](const VectorX<double>& w) -> VectorX<double> {
return F.transpose() * w;
};
// Resize kF (recall the vector always is zero for this 2D problem),
// gammaF, and gammaE.
data->kF.setZero(contacts.size() * new_friction_directions);
data->gammaF.setZero(contacts.size() * new_friction_directions);
data->gammaE.setZero(contacts.size());
// Update r with the new friction directions per contact.
for (int i = 0; i < ssize(data->r); ++i)
data->r[i] = new_friction_directions;
// Add in empty rows to G, by default, allowing us to verify that no
// constraint forces are added (and that solution method is robust to
// unnecessary constraints).
data->G_mult = [](const VectorX<double>& w) -> VectorX<double> {
return VectorX<double>::Zero(1);
};
data->G_transpose_mult =
[ngc](const VectorX<double>& w) -> VectorX<double> {
return VectorX<double>::Zero(ngc);
};
data->kG.setZero(0);
// Check the consistency of the data.
CheckProblemConsistency(*data, contacts.size());
}
// Computes rigid impacting contact data with no duplication of contact points
// or friction directions.
void CalcConstraintProblemDataForImpact(
ConstraintVelProblemData<double>* data) {
CalcConstraintProblemDataForImpact(data,
0, // no contact points duplicated
0); // no friction directions duplicated
}
// Gets the number of generalized coordinates for the rod.
int get_rod_num_coordinates() const { return 3; }
// Gets the output dimension of a Jacobian multiplication operator.
int GetOperatorDim(
std::function<VectorX<double>(const VectorX<double>&)> J) const {
return J(VectorX<double>(get_rod_num_coordinates())).size();
}
// Checks the consistency of a transpose operator.
void CheckTransOperatorDim(
std::function<VectorX<double>(const VectorX<double>&)> JT,
int num_constraints) const {
EXPECT_EQ(JT(VectorX<double>(num_constraints)).size(),
get_rod_num_coordinates());
}
// Checks consistency of rigid contact problem data.
void CheckProblemConsistency(const ConstraintAccelProblemData<double>& data,
int num_contacts) const {
const int ngc = get_rod_num_coordinates();
const int num_fdir = std::accumulate(data.r.begin(), data.r.end(), 0);
EXPECT_EQ(num_contacts,
data.sliding_contacts.size() + data.non_sliding_contacts.size());
EXPECT_EQ(GetOperatorDim(data.N_mult), num_contacts);
CheckTransOperatorDim(data.N_minus_muQ_transpose_mult, num_contacts);
EXPECT_EQ(GetOperatorDim(data.F_mult), num_fdir);
CheckTransOperatorDim(data.F_transpose_mult, num_fdir);
EXPECT_EQ(GetOperatorDim(data.L_mult), data.kL.size());
CheckTransOperatorDim(data.L_transpose_mult, data.kL.size());
EXPECT_EQ(data.tau.size(), ngc);
EXPECT_EQ(data.kN.size(), num_contacts);
EXPECT_EQ(data.kF.size(), num_fdir);
EXPECT_EQ(data.mu_non_sliding.size(), data.non_sliding_contacts.size());
EXPECT_EQ(data.mu_sliding.size(), data.sliding_contacts.size());
EXPECT_EQ(data.r.size(), data.non_sliding_contacts.size());
EXPECT_TRUE(data.solve_inertia);
EXPECT_TRUE(std::is_sorted(data.sliding_contacts.begin(),
data.sliding_contacts.end()));
EXPECT_TRUE(std::is_sorted(data.non_sliding_contacts.begin(),
data.non_sliding_contacts.end()));
}
// Checks consistency of rigid impact problem data.
void CheckProblemConsistency(const ConstraintVelProblemData<double>& data,
int num_contacts) const {
const int ngc = get_rod_num_coordinates();
const int num_spanning_directions =
std::accumulate(data.r.begin(), data.r.end(), 0);
EXPECT_EQ(GetOperatorDim(data.N_mult), num_contacts);
CheckTransOperatorDim(data.N_transpose_mult, num_contacts);
EXPECT_EQ(GetOperatorDim(data.F_mult), num_spanning_directions);
CheckTransOperatorDim(data.F_transpose_mult, num_spanning_directions);
EXPECT_EQ(GetOperatorDim(data.L_mult), data.kL.size());
CheckTransOperatorDim(data.L_transpose_mult, data.kL.size());
EXPECT_EQ(data.gammaN.size(), num_contacts);
EXPECT_EQ(data.gammaF.size(), num_spanning_directions);
EXPECT_EQ(data.gammaE.size(), num_contacts);
EXPECT_EQ(data.gammaL.size(), data.kL.size());
EXPECT_EQ(data.Mv.size(), ngc);
EXPECT_EQ(data.mu.size(), num_contacts);
EXPECT_EQ(data.r.size(), num_contacts);
EXPECT_TRUE(data.solve_inertia);
// TODO(edrumwri): Relax test for kF to be zero in the future (i.e., we
// currently allow no constraint stabilization along the F direction).
EXPECT_LT(data.kF.norm(), std::numeric_limits<double>::epsilon());
}
// Tests the rod in a single-point sticking configuration, with an external
// force applied either to the right or to the left. Given sufficiently small
// force and sufficiently large friction coefficient, the contact should
// remain in stiction.
void SinglePointSticking(bool force_applied_to_right) {
// Set the contact to large friction. Note that only the static friction
// coefficient will be used since there are no sliding contacts. However,
// set_mu_static() throws an exception if it is not at least as large as
// the Coulomb friction coefficient.
rod_->set_mu_coulomb(15.0);
rod_->set_mu_static(15.0);
// Get the acceleration due to gravity.
const double grav_accel = rod_->get_gravitational_acceleration();
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = contact_dup + 1;
// Set the state of the rod to resting vertically with no velocity.
SetRodToRestingVerticalConfig();
// Construct the contact frame.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get(), contact_dup,
friction_dir_dup);
EXPECT_TRUE(accel_data_->sliding_contacts.empty());
// Add a force, acting at the point of contact, that pulls the rod
// horizontally.
const double horz_f = (force_applied_to_right) ? 100 : -100;
accel_data_->tau[0] += horz_f;
accel_data_->tau[2] += horz_f * rod_->get_rod_half_length();
// Case 1: set kN as if the bodies are not accelerating into each
// other along the contact normal and verify that no contact forces
// are applied.
{
accel_data_->kN.setOnes() *= std::fabs(grav_accel);
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that no forces are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact forces.
{
accel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces equal the horizontal force.
const int total_cone_edges = std::accumulate(
accel_data_->r.begin(), accel_data_->r.end(), 0);
double ffriction = cf.segment(n_contacts, total_cone_edges).sum();
EXPECT_NEAR(ffriction, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify the generalized acceleration of the rod is equal to zero.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_LT(ga.norm(), eps_ * cf.size());
}
// Case 3: set kN as if the bodies are accelerating twice as hard into
// each other along the contact normal.
{
accel_data_->kN.setOnes() *= -std::fabs(grav_accel);
// Recompute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces equal the horizontal force.
const int total_cone_edges = std::accumulate(
accel_data_->r.begin(), accel_data_->r.end(), 0);
double ffriction = cf.segment(n_contacts, total_cone_edges).sum();
EXPECT_NEAR(ffriction, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the generalized acceleration of the rod is equal to the
// gravitational acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_NEAR(ga.norm(), std::fabs(grav_accel), eps_ * cf.size());
}
}
}
}
// Tests the rod in a single-point sticking configuration, with an external
// force applied either to the right or to the left. Given sufficiently small
// force and sufficiently large friction coefficient, the contact should
// remain in stiction.
void SinglePointStickingDiscretized(bool force_applied_to_right) {
// Set the contact to large friction. However, set_mu_static() throws an
// exception if it is not at least as large as the Coulomb friction
// coefficient.
rod_->set_mu_coulomb(15.0);
rod_->set_mu_static(15.0);
// Get the acceleration due to gravity.
const double grav_accel = rod_->get_gravitational_acceleration();
// Set the state of the rod to resting vertically with no velocity.
SetRodToRestingVerticalConfig();
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = contact_dup + 1;
// Construct the contact frame.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Compute the problem data.
CalcDiscreteTimeProblemData(dt_, vel_data_.get(), contact_dup,
friction_dir_dup);
// Add a force applied at the point of contact that results in a torque
// at the rod center-of-mass.
const double horz_f = (force_applied_to_right) ? 100 : -100;
vel_data_->Mv +=
Vector3<double>(horz_f, 0, horz_f * rod_->get_rod_half_length()) *
dt_;
// Case 1: set kN as if the bodies are not moving into each other along
// the contact normal and verify that no contact forces are applied.
{
vel_data_->kN.setOnes() *= std::fabs(grav_accel) * dt_;
// Compute the contact forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify that no forces are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero the stabilization term and recompute the contact forces.
{
vel_data_->kN.setZero();
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces equal the horizontal force.
const int total_cone_edges =
std::accumulate(vel_data_->r.begin(), vel_data_->r.end(), 0);
double ffriction = cf.segment(n_contacts, total_cone_edges).sum();
EXPECT_NEAR(ffriction, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces),
std::logic_error);
}
// Get the rod velocity.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
// Verify the generalized acceleration of the rod is equal to zero.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_LT(ga.norm(), eps_ * cf.size());
}
// Case 3: set kN as if the bodies are accelerating twice as hard into
// each other along the contact normal.
{
vel_data_->kN.setOnes() *= -std::fabs(grav_accel) * dt_;
// Recompute the contact forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces equal the applied,
// horizontal force.
const int total_cone_edges =
std::accumulate(vel_data_->r.begin(), vel_data_->r.end(), 0);
double ffriction = cf.segment(n_contacts, total_cone_edges).sum();
EXPECT_NEAR(ffriction, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces),
std::logic_error);
}
// Get the rod velocity.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
// Verify that the generalized acceleration of the rod is equal to
// the gravitational acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_NEAR(ga.norm(), std::fabs(grav_accel), eps_ * cf.size());
}
}
}
}
// Tests the rod in a two-point sticking configuration (i.e., force should
// be applied with no resulting tangential motion), with force applied either
// to the right or to the left (force_applied_to_right = false) and using
// either the LCP solver or the linear system solver (use_lcp_solver = false).
void TwoPointSticking(bool force_applied_to_right, bool use_lcp_solver) {
// Set the contact to large friction. Note that only the static friction
// coefficient will be used since there are no sliding contacts.
rod_->set_mu_coulomb(0.0);
rod_->set_mu_static(15.0);
// Get the acceleration due to gravity.
const double grav_accel = rod_->get_gravitational_acceleration();
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = 2 * (contact_dup + 1);
// Set the state of the rod to resting on its side with no velocity.
SetRodToRestingHorizontalConfig();
// Construct the contact frames.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get(), contact_dup,
friction_dir_dup);
EXPECT_TRUE(accel_data_->sliding_contacts.empty());
// Indicate whether to use the linear system solver.
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Add a force pulling the rod horizontally.
const double horz_f = (force_applied_to_right) ? 100 : -100;
accel_data_->tau[0] += horz_f;
// Case 1: set kN as if the bodies are not accelerating into each
// other along the contact normal and verify that no contact forces
// are applied.
{
accel_data_->kN.setOnes() *= std::fabs(grav_accel);
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that no forces are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact forces.
{
accel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Do this next part *only* if the friction directions are not
// duplicated (which would cause an exception to be thrown).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frames.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), n_contacts);
// Verify that the normal forces equal the gravitational force.
// Normal forces are in the first component of each vector.
// Frictional forces are in the second component of each vector.
const double mg = rod_->get_rod_mass() *
std::fabs(rod_->get_gravitational_acceleration());
double normal_force_mag = 0;
double fric_force = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
normal_force_mag += contact_forces[i][0];
fric_force += contact_forces[i][1];
}
EXPECT_NEAR(normal_force_mag, mg, eps_ * cf.size());
// Verify that the negation of the frictional forces equal the
// horizontal forces.
EXPECT_NEAR(fric_force, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify the generalized acceleration of the rod is equal to zero.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_LT(ga.norm(), eps_ * cf.size());
}
// Case 3: set kN as if the bodies are accelerating twice as hard into
// each other along the contact normal.
{
accel_data_->kN.setOnes() *= -std::fabs(grav_accel);
// Recompute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces equal the horizontal force.
const int total_cone_edges = std::accumulate(
accel_data_->r.begin(), accel_data_->r.end(), 0);
double ffriction = cf.segment(n_contacts, total_cone_edges).sum();
EXPECT_NEAR(ffriction, -horz_f, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the generalized acceleration of the rod is equal to the
// gravitational acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_NEAR(ga.norm(), std::fabs(grav_accel), eps_ * cf.size());
}
}
}
}
// Tests the rod in a two-point non-sticking configuration that will
// transition to sliding from the external force (applied toward the right
// or the left, as specified.
void TwoPointNonSlidingToSliding(bool applied_to_right) {
// Set the contact to large friction. Note that only the static friction
// coefficient will be used since there are no sliding contacts.
const double mu_static = 0.1;
rod_->set_mu_coulomb(0.0);
rod_->set_mu_static(mu_static);
// Get the acceleration due to gravity.
const double grav_accel = rod_->get_gravitational_acceleration();
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = 2 * (1 + contact_dup);
// Set the state of the rod to resting on its side with no velocity.
SetRodToRestingHorizontalConfig();
// Construct the contact frames.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get(), contact_dup,
friction_dir_dup);
// Add a force pulling the rod horizontally.
const double horz_f = (applied_to_right) ? 100.0 : -100;
accel_data_->tau[0] += horz_f;
// Case 1: set kN as if the bodies are not accelerating into each
// other along the contact normal and verify that no contact forces
// are applied.
{
accel_data_->kN.setOnes() *= std::fabs(grav_accel);
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that no forces are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact forces.
{
accel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Get the contact forces expressed in the contact frames *only*
// if the friction directions are not duplicated (which would cause an
// exception to be thrown).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
EXPECT_TRUE(accel_data_->sliding_contacts.empty());
// Get the contact forces expressed in the contact frames.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), n_contacts);
// Verify that the frictional forces are maximized.
double fnormal = 0;
double ffrictional = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fnormal += contact_forces[i][0];
ffrictional += std::fabs(contact_forces[i][1]);
}
EXPECT_NEAR(ffrictional, mu_static * fnormal, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the horizontal acceleration is in the appropriate
// direction.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_GT((applied_to_right) ? ga[0] : -ga[0], 0);
}
// Case 3: now, set kN as if the bodies are accelerating twice as hard
// into each other along the contact normal.
{
accel_data_->kN.setOnes() *= -std::fabs(grav_accel);
// Recompute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the frictional forces are maximized.
double fnormal = 0;
double ffrictional = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fnormal += contact_forces[i][0];
ffrictional += std::fabs(contact_forces[i][1]);
}
EXPECT_NEAR(ffrictional, mu_static * fnormal, eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the vertical acceleration of the rod is equal to the
// gravitational acceleration and that the horizontal acceleration is
// in the appropriate direction.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_NEAR(ga[1], std::fabs(grav_accel), eps_ * cf.size());
EXPECT_GT((applied_to_right) ? ga[0] : -ga[0], 0);
}
}
}
}
// Tests the rod in a two-point contact configuration, without sliding
// occurring at either contact point, that will transition to sliding from the
// external force (applied toward the right or the left, as specified).
void TwoPointNonSlidingToSlidingDiscretized(bool applied_to_right) {
// Set the contact to large friction.
const double mu_static = 0.1;
rod_->set_mu_coulomb(mu_static);
rod_->set_mu_static(mu_static);
// Get the acceleration due to gravity.
const double grav_accel = rod_->get_gravitational_acceleration();
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = 2 * (1 + contact_dup);
// Set the state of the rod to resting on its side with zero velocity.
SetRodToRestingHorizontalConfig();
// Construct the contact frames.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Compute the problem data.
CalcDiscreteTimeProblemData(dt_, vel_data_.get(), contact_dup,
friction_dir_dup);
// Add a force pulling the rod horizontally.
const double horz_f = (applied_to_right) ? 100.0 : -100;
vel_data_->Mv[0] += horz_f * dt_;
// Case 1: set kN as if the bodies are not accelerating into each
// other along the contact normal and verify that no contact forces
// are applied.
{
vel_data_->kN.setOnes() *= std::fabs(grav_accel) * dt_;
// Compute the contact forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify that no forces are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact forces.
{
vel_data_->kN.setZero();
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Get the contact forces expressed in the contact frames *only*
// if the friction directions are not duplicated (which would cause an
// exception to be thrown).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frames.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), n_contacts);
// Get the expected sign of the frictional forces.
const double ff_sign = (applied_to_right) ? -1.0 : 1.0;
// Verify that the frictional forces are maximized and in the
// correct direction.
double fnormal = 0;
double ffrictional = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fnormal += contact_forces[i][0];
ffrictional += contact_forces[i][1];
EXPECT_NEAR(ffrictional * ff_sign, mu_static * fnormal,
eps_ * cf.size());
}
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the horizontal acceleration is in the appropriate
// direction.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_GT((applied_to_right) ? ga[0] : -ga[0], 0);
}
// Case 3: set kN as if the bodies are accelerating twice as hard into
// each other along the contact normal.
{
vel_data_->kN.setOnes() *= -std::fabs(grav_accel) * dt_;
// Recompute the contact forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// The primary test below is conducted when there is no friction
// direction duplication because CalcContactForcesInContactFrames()
// would throw an exception (the secondary test looks for this case).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
// Get the contact forces expressed in the contact frame.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Get the sign of the force application.
const double ff_sign = (applied_to_right) ? -1.0 : 1.0;
// Verify that the frictional forces are maximized.
double fnormal = 0;
double ffrictional = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fnormal += contact_forces[i][0];
ffrictional += contact_forces[i][1];
}
EXPECT_NEAR(ff_sign * ffrictional, mu_static * fnormal,
eps_ * cf.size());
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the vertical acceleration of the rod is equal to the
// gravitational acceleration and that the horizontal acceleration is
// in the appropriate direction.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_NEAR(ga[1], std::fabs(grav_accel), eps_ * cf.size());
EXPECT_GT((applied_to_right) ? ga[0] : -ga[0], 0);
}
}
}
}
// Tests the rod in a two-point impact, with initial velocity sliding to the
// right or left, as specified, with impulses insufficient to put the rod
// into stiction.
void TwoPointImpactNoTransitionToStiction(bool sliding_to_right) {
// Set the coefficient of friction to very small.
const double mu = 1e-4;
rod_->set_mu_coulomb(mu);
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = 2 * (contact_dup + 1);
// Construct the contact frames.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Set the configuration of the rod to lying on its side and impacting.
SetRodToSlidingImpactingHorizontalConfig(sliding_to_right);
// Get the vertical velocity of the rod.
const double vert_vel =
context_->get_continuous_state().CopyToVector()[4];
// Set the sign of the sliding direction.
const double sign = (sliding_to_right) ? 1 : -1;
// Compute the problem data.
CalcConstraintProblemDataForImpact(vel_data_.get(), contact_dup,
friction_dir_dup);
// Get the generalized velocity of the rod.
const VectorX<double> v0 = vel_data_->solve_inertia(vel_data_->Mv);
// Case 1: set kN as if the bodies are not moving toward each
// other along the contact normal and verify that no contact forces
// are applied.
{
vel_data_->kN.setOnes() *= -vert_vel;
// Compute the contact impulses.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify that no impulses are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact impulses.
{
vel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Get the sign of the force application.
const double ff_sign = (sliding_to_right) ? -1.0 : 1.0;
// Get the impact forces expressed in the contact frames *only*
// if the friction directions are not duplicated (which would cause an
// exception to be thrown).
std::vector<Vector2<double>> contact_impulses;
if (friction_dir_dup == 0) {
// Get the contact impulses expressed in the contact frames.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_impulses);
// Verify that the number of contact impulse vectors is correct.
ASSERT_EQ(contact_impulses.size(), n_contacts);
// Verify that the frictional impulses are maximized.
double jnormal = 0;
double jfrictional = 0;
for (int i = 0; i < ssize(contact_impulses); ++i) {
jnormal += contact_impulses[i][0];
jfrictional += contact_impulses[i][1];
EXPECT_NEAR(ff_sign * jfrictional, mu * jnormal, eps_);
}
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_impulses),
std::logic_error);
}
// Verify that the horizontal velocity is in the proper direction.
VectorX<double> dgv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &dgv);
EXPECT_GT(sign * v0[0] + dgv[0], 0);
}
// Case 3: set kN as if the bodies are moving twice as fast into
// each other along the contact normal.
{
vel_data_->kN.setOnes() *= vert_vel;
// Recompute the contact impulses.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Get the impact forces expressed in the contact frames if the
// friction directions are not duplicated.
std::vector<Vector2<double>> contact_impulses;
if (friction_dir_dup == 0) {
// Get the contact impulses expressed in the contact frames.
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_impulses);
// Verify that the number of contact impulse vectors is correct.
ASSERT_EQ(contact_impulses.size(), n_contacts);
// Verify that the frictional impulses are maximized.
double jnormal = 0;
double jfrictional = 0;
for (int i = 0; i < ssize(contact_impulses); ++i) {
jnormal += contact_impulses[i][0];
jfrictional += std::fabs(contact_impulses[i][1]);
}
EXPECT_NEAR(jfrictional, mu * jnormal, eps_);
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_impulses),
std::logic_error);
}
// Verify that the horizontal velocity is in the proper direction and
// that the vertical velocity is essentially reversed.
VectorX<double> dgv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &dgv);
EXPECT_GT(sign * v0[0] + dgv[0], 0);
EXPECT_NEAR(v0[1] + dgv[1], -v0[1], eps_);
}
}
}
}
// Tests the rod in a two-point impacting and sticking configuration,
// with initial velocity sliding to the right or left, as specified.
void TwoPointImpactingAndSticking(bool sliding_to_right) {
// Set the rod to large friction.
rod_->set_mu_coulomb(15.0);
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
const int n_contacts = 2 * (contact_dup + 1);
// Set the state of the rod to lying on its side with both impacting
// velocity and horizontally moving velocity.
SetRodToSlidingImpactingHorizontalConfig(sliding_to_right);
// Construct the contact frames.
std::vector<Matrix2<double>> frames;
for (int i = 0; i < n_contacts; ++i)
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Get the vertical velocity of the rod.
const double vert_vel =
context_->get_continuous_state().CopyToVector()[4];
// Compute the impact problem data.
CalcConstraintProblemDataForImpact(vel_data_.get(), contact_dup,
friction_dir_dup);
// Get the generalized velocity of the rod.
const VectorX<double> v0 = vel_data_->solve_inertia(vel_data_->Mv);
// Case 1: set kN as if the bodies are not moving toward each
// other along the contact normal and verify that no contact forces
// are applied.
{
vel_data_->kN.setOnes() *= -vert_vel;
// Compute the contact impulses.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify that no impulses are applied.
EXPECT_LT(cf.norm(), std::numeric_limits<double>::epsilon());
}
// Case 2: zero stabilization term and recompute the contact impulses.
{
vel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify that the x-axis of the contact frame, which corresponds to
// the contact normal, points along the world y-axis, and the y-axis
// of the contact frame, which corresponds to a contact tangent
// vector, points along the world x-axis.
for (int i = 0; i < ssize(frames); ++i) {
EXPECT_LT(
std::fabs(frames[i].col(0).dot(Vector2<double>::UnitY()) - 1.0),
std::numeric_limits<double>::epsilon());
EXPECT_LT(
std::fabs(frames[i].col(1).dot(Vector2<double>::UnitX()) - 1.0),
std::numeric_limits<double>::epsilon());
}
// Get the impulsive contact forces expressed in the contact frames
// *only* if the friction directions are not duplicated (which would
// cause an exception to be thrown).
std::vector<Vector2<double>> contact_forces;
if (friction_dir_dup == 0) {
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), n_contacts);
} else {
EXPECT_THROW(
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces),
std::logic_error);
}
// Verify that the generalized velocity of the rod is equal to zero.
VectorX<double> dgv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &dgv);
EXPECT_LT((v0 + dgv).norm(), eps_);
}
// Case 3: set kN as if the bodies are moving twice as fast into
// each other along the contact normal.
{
vel_data_->kN.setOnes() *= vert_vel;
// Recompute the contact impulses.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify that all components of the generalized velocity of the rod
// except that corresponding to the vertical motion are equal to zero;
// the vertical motion should oppose the initial vertical motion.
VectorX<double> dgv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &dgv);
EXPECT_LT((v0[0] + dgv[0]), eps_);
EXPECT_NEAR((v0[1] + dgv[1]), -vert_vel, eps_);
EXPECT_LT((v0[2] + dgv[2]), eps_);
}
}
}
}
// Tests the rod in a sliding configuration with sliding velocity as
// specified. If `upright` is true, then the rod makes contact at a single
// point. Otherwise, it will be on its side and make contact at two points.
// The fully upright and on-side configurations permit readily predicting
// the requisite force(s) and motion.
void Sliding(bool sliding_to_right, bool upright, bool use_lcp_solver) {
if (upright) {
SetRodToRestingVerticalConfig();
} else {
// Set the state of the rod to resting on its side w/ horizontal velocity.
SetRodToRestingHorizontalConfig();
}
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = (sliding_to_right) ? 1 : -1;
// Get the gravitational acceleration.
const double grav_accel = rod_->get_gravitational_acceleration();
// Set the coefficient of friction.
rod_->set_mu_coulomb(0.0);
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Case 1: counteract the acceleration from gravity using the kN term.
{
accel_data_->kN.setOnes() *= -grav_accel;
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that no forces were applied.
EXPECT_LT(cf.norm(), eps_);
}
// Case 2: zero out the kN term and try again.
{
accel_data_->kN.setZero();
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Get the contact tangent velocities.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts,
&tangent_vels);
// Construct the contact frame(s).
std::vector<Matrix2<double>> frames;
frames.push_back(
GetSlidingContactFrameToWorldTransform(tangent_vels.front()));
if (!upright) {
frames.push_back(
GetSlidingContactFrameToWorldTransform(tangent_vels.back()));
}
// Get the contact forces expressed in the contact frame.
std::vector<Vector2<double>> contact_forces;
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that there are no non-sliding frictional forces.
EXPECT_TRUE(accel_data_->non_sliding_contacts.empty());
const int nc = accel_data_->sliding_contacts.size();
EXPECT_EQ(cf.size(), nc);
// Verify that the normal contact forces exactly oppose gravity (the
// frictional forces should be zero).
const double mg = std::fabs(rod_->get_gravitational_acceleration()) *
rod_->get_rod_mass();
double fN = 0, fF = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fN += contact_forces[i][0];
fF += std::fabs(contact_forces[i][1]);
}
EXPECT_NEAR(fN, mg, eps_);
EXPECT_NEAR(fF, 0, eps_);
}
// Case 3: set the kN term to indicate that the rod is accelerating downward
// with twice the gravitational acceleration.
{
accel_data_->kN.setOnes() *= grav_accel;
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that the normal component of the generalized acceleration is now
// equal to the negated gravitational acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_NEAR(ga[1], -grav_accel, eps_);
}
}
// Tests the rod in a sliding configuration with sliding velocity as
// specified. If `upright` is true, then the rod makes contact at a single
// point. Otherwise, it will be on its side and make contact at two points.
// The fully upright and on-side configurations permit readily predicting
// the requisite force(s) and motion.
void SlidingDiscretized(bool sliding_to_right, bool upright) {
if (upright) {
SetRodToRestingVerticalConfig();
} else {
// Set the state of the rod to resting on its side w/ horizontal velocity.
SetRodToRestingHorizontalConfig();
}
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = (sliding_to_right) ? 1 : -1;
// Get the gravitational acceleration.
const double grav_accel = rod_->get_gravitational_acceleration();
// Set the coefficient of friction to zero. Note that a test that uses
// a non-zero coefficient of friction with sliding is
// SlidingPlusBilateralDiscretized().
rod_->set_mu_coulomb(0.0);
// Compute the problem data.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
CalcDiscreteTimeProblemData(dt_, vel_data_.get());
// Case 1: counteract the acceleration from gravity using the kN term.
{
vel_data_->kN.setOnes() *= -grav_accel * dt_;
// Compute the contact forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify that no forces were applied.
EXPECT_LT(cf.norm(), eps_);
}
// Case 2: zero out the kN term and try again.
{
vel_data_->kN.setZero();
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Get the contact tangent velocities.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts,
&tangent_vels);
// Construct the contact frame(s).
std::vector<Matrix2<double>> frames;
frames.push_back(
GetSlidingContactFrameToWorldTransform(tangent_vels.front()));
if (!upright) {
frames.push_back(
GetSlidingContactFrameToWorldTransform(tangent_vels.back()));
}
// Get the contact forces expressed in the contact frame.
std::vector<Vector2<double>> contact_forces;
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that the normal contact forces exactly oppose gravity (the
// frictional forces should be zero).
const double mg = std::fabs(rod_->get_gravitational_acceleration()) *
rod_->get_rod_mass();
double fN = 0, fF = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fN += contact_forces[i][0];
fF += std::fabs(contact_forces[i][1]);
}
EXPECT_NEAR(fN, mg, eps_);
EXPECT_NEAR(fF, 0, eps_);
}
// Case 3: set the kN term to indicate that the rod is accelerating downward
// with twice the gravitational acceleration.
{
vel_data_->kN.setOnes() *= grav_accel * dt_;
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify that the normal component of the generalized acceleration is now
// equal to the negated gravitational acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_NEAR(ga[1], -grav_accel, eps_);
}
}
// Tests the rod in an upright sliding configuration with sliding velocity as
// specified. The rod will be constrained to prevent rotational acceleration
// using a bilateral constraint as well.
void SlidingPlusBilateral(bool sliding_to_right, bool use_lcp_solver) {
SetRodToRestingVerticalConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = (sliding_to_right) ? 1 : -1;
// Set the coefficient of friction. A nonzero coefficient of friction should
// cause the rod to rotate.
rod_->set_mu_coulomb(0.1);
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Add in bilateral constraints on rotational motion.
accel_data_->kG.setZero(1); // No right hand side term.
accel_data_->G_mult = [](const VectorX<double>& v) -> VectorX<double> {
VectorX<double> result(1); // Only one constraint.
// Constrain the angular velocity (and hence angular acceleration) to be
// zero.
result[0] = v[2];
return result;
};
accel_data_->G_transpose_mult =
[this](const VectorX<double>& f) -> VectorX<double> {
// A force (torque) applied to the third component needs no
// transformation.
DRAKE_DEMAND(f.size() == 1);
VectorX<double> result(get_rod_num_coordinates());
result.setZero();
result[2] = f[0];
return result;
};
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Get the contact tangent velocities.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts, &tangent_vels);
// Construct the contact frame(s).
std::vector<Matrix2<double>> frames;
frames.push_back(
GetSlidingContactFrameToWorldTransform(tangent_vels.front()));
// Get the contact forces expressed in the contact frame.
std::vector<Vector2<double>> contact_forces;
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *accel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that there are no non-sliding frictional forces.
EXPECT_TRUE(accel_data_->non_sliding_contacts.empty());
const int num_contacts = accel_data_->sliding_contacts.size();
const int num_bilateral_eqns = accel_data_->kG.size();
EXPECT_EQ(cf.size(), num_contacts + num_bilateral_eqns);
// Verify that the normal contact forces exactly oppose gravity and the
// friction forces are of the appropriate size. Frictional forces must
// always be negative- it is the contact frame that can change.
const double mg = std::fabs(rod_->get_gravitational_acceleration()) *
rod_->get_rod_mass();
double fN = 0, fF = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fN += contact_forces[i][0];
fF += contact_forces[i][1];
}
EXPECT_NEAR(fN, mg, eps_);
EXPECT_LT(fF, 0);
EXPECT_NEAR(fF, -mg * rod_->get_mu_coulomb(), eps_);
// Get the generalized acceleration and verify that there is no angular
// acceleration.
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_LT(ga[2], eps_ * cf.size());
// Indicate through modification of the kG term that the system already has
// angular velocity (which violates our desire to constrain the
// orientation) and solve again.
accel_data_->kG[0] = 1.0; // Indicate a ccw angular motion..
solver_.SolveConstraintProblem(*accel_data_, &cf);
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &ga);
EXPECT_NEAR(ga[2], -accel_data_->kG[0], eps_ * cf.size());
}
// Tests the rod in an upright sliding and impacting state, with sliding
// velocity as specified. The rod will be constrained to prevent rotational
// velocity using a bilateral constraint as well.
void SlidingPlusBilateralImpact(bool sliding_to_right) {
SetRodToSlidingImpactingVerticalConfig(sliding_to_right);
// Set the coefficient of friction. A nonzero coefficient of friction should
// cause the rod to rotate.
rod_->set_mu_coulomb(0.1);
// Compute the problem data.
CalcConstraintProblemDataForImpact(vel_data_.get());
// Compute the generalized velocity.
const VectorX<double> v0 = vel_data_->solve_inertia(vel_data_->Mv);
// Add in bilateral constraints on rotational motion.
vel_data_->kG.setZero(1); // No right hand side term.
vel_data_->G_mult = [](const VectorX<double>& w) -> VectorX<double> {
VectorX<double> result(1); // Only one constraint.
// Constrain the angular velocity to be zero.
result[0] = w[2];
return result;
};
vel_data_->G_transpose_mult =
[this](const VectorX<double>& f) -> VectorX<double> {
// An impulsive force (torque) applied to the third component needs no
// transformation.
DRAKE_DEMAND(f.size() == 1);
VectorX<double> result(get_rod_num_coordinates());
result.setZero();
result[2] = f[0];
return result;
};
// Compute the impact forces.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Construct the contact frame(s).
std::vector<Matrix2<double>> frames;
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Get the contact impulses expressed in the contact frame.
std::vector<Vector2<double>> contact_forces;
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that there are no non-sliding frictional forces.
const int num_contacts = vel_data_->mu.size();
const int num_bilateral_eqns = vel_data_->kG.size();
EXPECT_EQ(cf.size(), num_contacts * 2 + num_bilateral_eqns);
// Get the pre-impact vertical momentum.
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
const double mv = rod_->get_rod_mass() * xc[4];
// Verify that the normal contact impulses exactly oppose the pre-impact
double fN = 0, fF = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fN += contact_forces[i][0];
fF += contact_forces[i][1];
}
const double sign = (sliding_to_right) ? 1 : -1;
EXPECT_NEAR(fN, -mv, eps_);
EXPECT_NEAR(fF, sign * mv * rod_->get_mu_coulomb(), eps_);
// Get the change in generalized velocity and verify that there is no
// angular velocity.
VectorX<double> gv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &gv);
EXPECT_LT((v0[2] + gv[2]), eps_ * cf.size());
// Indicate through modification of the kG term that the system already has
// angular orientation (which violates our desire to keep the rod at
// zero rotation) and solve again.
vel_data_->kG[0] = 1.0; // Indicate a ccw orientation..
solver_.SolveImpactProblem(*vel_data_, &cf);
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &gv);
EXPECT_NEAR(v0[2] + gv[2], -vel_data_->kG[0], eps_ * cf.size());
}
// Tests the rod in an upright sliding state, with sliding
// direction as specified. The rod will be constrained to prevent rotational
// acceleration using a bilateral constraint as well.
void SlidingPlusBilateralDiscretized(bool sliding_to_right) {
using std::max;
SetRodToRestingVerticalConfig();
// Set the coefficient of friction. A nonzero coefficient of friction should
// cause the rod to rotate.
rod_->set_mu_coulomb(0.1);
// Compute the problem data with no duplicated contacts or friction
// directions.
CalcDiscreteTimeProblemData(dt_, vel_data_.get());
// Update Mv to slide to the given direction and to account for
// gravitational force.
const double rod_mass = rod_->get_rod_mass();
vel_data_->Mv[0] += ((sliding_to_right) ? 1.0 : -1.0) * rod_mass;
// Add in bilateral constraints on rotational motion.
vel_data_->kG.setZero(1); // No right hand side term.
vel_data_->G_mult = [](const VectorX<double>& w) -> VectorX<double> {
VectorX<double> result(1); // Only one constraint.
// Constrain the angular velocity to be zero.
result[0] = w[2];
return result;
};
vel_data_->G_transpose_mult =
[this](const VectorX<double>& f) -> VectorX<double> {
// A force (torque) applied to the third component needs no
// transformation.
DRAKE_DEMAND(f.size() == 1);
VectorX<double> result(get_rod_num_coordinates());
result.setZero();
result[2] = f[0];
return result;
};
// Solve the discretization problem.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// cf should be 3-dimensional. First dimension is normal force (along
// global +y), second dimension is frictional force (along +x),
// third dimension is constraint force (along +theta). Since the bilateral
// constraint should prevent angular motion, the constraint force (cf[2])
// should counteract the rotation that would be induced by the frictional
// force (cf[1]).
ASSERT_EQ(cf.size(), 3);
const double zero_tol = 10 * std::numeric_limits<double>::epsilon();
EXPECT_NEAR(cf[1], -cf[2], zero_tol);
// Construct the contact frame(s).
std::vector<Matrix2<double>> frames;
frames.push_back(GetNonSlidingContactFrameToWorldTransform());
// Get the contact forces expressed in the contact frame.
std::vector<Vector2<double>> contact_forces;
ConstraintSolver<double>::CalcContactForcesInContactFrames(
cf, *vel_data_, frames, &contact_forces);
// Verify that the number of contact force vectors is correct.
ASSERT_EQ(contact_forces.size(), frames.size());
// Verify that there are no non-sliding frictional forces.
const int num_contacts = vel_data_->mu.size();
const int num_bilateral_eqns = vel_data_->kG.size();
EXPECT_EQ(cf.size(), num_contacts * 2 + num_bilateral_eqns);
// Determine the force that should be acting on the system.
const double fdown = -rod_->get_gravitational_acceleration() * rod_mass;
// Verify that the normal contact forces exactly oppose the discretized
// force.
double fN = 0, fF = 0;
for (int i = 0; i < ssize(contact_forces); ++i) {
fN += contact_forces[i][0];
fF += contact_forces[i][1];
}
const double sign = (sliding_to_right) ? 1 : -1;
EXPECT_NEAR(fN, fdown, eps_);
EXPECT_NEAR(fF, sign * -fdown * rod_->get_mu_coulomb(), eps_);
// Get the generalized acceleration from the constraint forces and verify
// that the moment is zero.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> ga;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_LT(ga[2], eps_ * cf.size());
// Indicate through modification of the kG term that the system already has
// angular orientation (which violates our desire to keep the rod at
// zero rotation) and solve again.
vel_data_->kG[0] = 1.0; // Indicate a ccw orientation..
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Compute the generalized acceleration.
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &ga);
EXPECT_NEAR(ga[2] * dt_, -vel_data_->kG[0], eps_ * cf.size());
}
// Tests the rod in a one-point sliding contact configuration with a second
// constraint that prevents horizontal acceleration. This test tests the
// interaction between contact and limit constraints.
void OnePointPlusLimit(bool use_lcp_solver) {
// Set the state of the rod to vertically-at-rest and sliding to the left.
// Set the state of the rod to resting on its side with horizontal velocity.
SetRodToRestingHorizontalConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = 1.0;
// Set the coefficient of friction to somewhat small (to limit the sliding
// force).
rod_->set_mu_coulomb(1e-1);
// Get the gravitational acceleration.
const double grav_accel = rod_->get_gravitational_acceleration();
// First, construct the acceleration-level problem data as normal to set
// inertia solver and external forces.
CalcConstraintAccelProblemData(accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Get the original N and Nᵀ - μQᵀ
const int ngc = get_rod_num_coordinates();
const int num_old_contacts = 2;
MatrixX<double> N(num_old_contacts, ngc);
MatrixX<double> N_minus_muQ_transpose(ngc, num_old_contacts);
for (int i = 0; i < num_old_contacts; ++i) {
N_minus_muQ_transpose.col(i) =
accel_data_->N_minus_muQ_transpose_mult(VectorX<double>::Unit(2, i));
}
for (int i = 0; i < ngc; ++i)
N.col(i) = accel_data_->N_mult(VectorX<double>::Unit(ngc, i));
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
accel_data_->sliding_contacts.resize(1);
accel_data_->mu_sliding.resize(1);
accel_data_->N_mult = [&N](const VectorX<double>& v) {
return N.row(0) * v;
};
accel_data_->kN.setZero(1);
accel_data_->kL.setZero(1);
accel_data_->N_minus_muQ_transpose_mult =
[&N_minus_muQ_transpose](const VectorX<double>& l) {
return N_minus_muQ_transpose.col(0) * l;
};
// Set the Jacobian entry- in this case, the limit is a lower limit on the
// second coordinate (vertical position).
const int num_limits = 1;
accel_data_->L_mult = [&N](const VectorX<double>& v) -> VectorX<double> {
return N.row(1) * v;
};
accel_data_->L_transpose_mult =
[&N](const VectorX<double>& v) -> VectorX<double> {
return N.row(1).transpose() * v;
};
accel_data_->kL.setZero(num_limits);
// Case 1: set kN and kL terms to counteract gravity, which should prevent
// any constraint forces from being applied.
{
accel_data_->kN.setOnes() *= -grav_accel;
accel_data_->kL.setOnes() *= -grav_accel;
// Compute the constraint forces and verify that none are applied.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
EXPECT_LT(cf.norm(), eps_);
}
// Case 2: reset kN and kL and recompute constraint forces.
{
accel_data_->kN.setZero();
accel_data_->kL.setZero();
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify the size of cf is as expected.
const int num_contacts = 1;
EXPECT_EQ(cf.size(), num_contacts + num_limits);
// Verify that the vertical acceleration is zero. If the cross-constraint
// term LM⁻¹(Nᵀ - μQᵀ) is not computed properly, this acceleration might
// not be zero. Note that μQᵀ will not have any effect here.
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &vdot);
EXPECT_NEAR(vdot[1], 0, eps_);
}
// Case 3: set kN and kL terms to effectively double gravity, which should
// cause the rod to accelerate upward.
{
accel_data_->kN.setOnes() *= grav_accel;
accel_data_->kL.setOnes() *= grav_accel;
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &vdot);
EXPECT_NEAR(vdot[1], -grav_accel, eps_);
}
}
// Tests the rod in a one-point sliding contact configuration with a second
// constraint that prevents horizontal acceleration. This test tests the
// interaction between contact and limit constraints.
void OnePointPlusLimitDiscretized() {
// Set the state of the rod to resting on its side with horizontal velocity.
SetRodToRestingHorizontalConfig();
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[3] = 1.0;
// Set the coefficient of friction to somewhat small (to limit the sliding
// force).
rod_->set_mu_coulomb(1e-1);
// Get the gravitational acceleration.
const double grav_accel = rod_->get_gravitational_acceleration();
// First, construct the acceleration-level problem data as normal to set
// inertia solver and external forces.
CalcDiscreteTimeProblemData(dt_, vel_data_.get());
// Get the original N
const int ngc = get_rod_num_coordinates();
const int num_old_contacts = 2;
MatrixX<double> N(num_old_contacts, ngc);
MatrixX<double> N_transpose(ngc, num_old_contacts);
for (int i = 0; i < num_old_contacts; ++i) {
N_transpose.col(i) =
vel_data_->N_transpose_mult(VectorX<double>::Unit(2, i));
}
for (int i = 0; i < ngc; ++i)
N.col(i) = vel_data_->N_mult(VectorX<double>::Unit(ngc, i));
// Get the original F.
const int num_friction_dirs = 1;
MatrixX<double> F(num_old_contacts * num_friction_dirs, ngc);
MatrixX<double> F_transpose(ngc, num_old_contacts * num_friction_dirs);
for (int i = 0; i < num_old_contacts * num_friction_dirs; ++i) {
F_transpose.col(i) = vel_data_->F_transpose_mult(
VectorX<double>::Unit(num_old_contacts * num_friction_dirs, i));
}
for (int i = 0; i < ngc; ++i)
F.col(i) = vel_data_->F_mult(VectorX<double>::Unit(ngc, i));
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
vel_data_->mu.setZero(1);
vel_data_->r = {1};
vel_data_->N_mult = [&N](const VectorX<double>& v) {
return N.row(0) * v;
};
vel_data_->F_mult = [&F](const VectorX<double>& v) {
return F.row(0) * v;
};
vel_data_->kN.setZero(1);
vel_data_->kF.setZero(1);
vel_data_->gammaN.setZero(1);
vel_data_->gammaF.setZero(1);
vel_data_->gammaE.setZero(1);
vel_data_->kL.setZero(1);
vel_data_->gammaL.setZero(1);
vel_data_->N_transpose_mult = [&N_transpose](const VectorX<double>& l) {
return N_transpose.col(0) * l;
};
vel_data_->F_transpose_mult = [&F_transpose](const VectorX<double>& l) {
return F_transpose.col(0) * l;
};
// Set the Jacobian entry- in this case, the limit is a lower limit on the
// second coordinate (vertical position).
const int num_limits = 1;
vel_data_->L_mult = [&N](const VectorX<double>& v) -> VectorX<double> {
return N.row(1) * v;
};
vel_data_->L_transpose_mult =
[&N](const VectorX<double>& v) -> VectorX<double> {
return N.row(1).transpose() * v;
};
vel_data_->kL.setZero(num_limits);
// Case 1: set kN and kL terms to counteract gravity, which should prevent
// any constraint forces from being applied.
{
vel_data_->kN.setOnes() *= -grav_accel * dt_;
vel_data_->kL.setOnes() *= -grav_accel * dt_;
// Compute the constraint forces and verify that none are applied.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
EXPECT_LT(cf.norm(), eps_);
}
// Case 2: reset kN and kL and recompute constraint forces.
{
vel_data_->kN.setZero();
vel_data_->kL.setZero();
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify the size of cf is as expected.
const int num_contacts = 1;
EXPECT_EQ(cf.size(),
num_contacts + num_friction_dirs * num_contacts + num_limits);
// Verify that the vertical acceleration is zero. If the cross-constraint
// term LM⁻¹Nᵀ is not computed properly, this acceleration might not
// be zero.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &vdot);
EXPECT_NEAR(vdot[1], 0, eps_);
}
// Case 3: set kN and kL terms to effectively double gravity, which should
// cause the rod to accelerate upward.
{
vel_data_->kN.setOnes() *= grav_accel * dt_;
vel_data_->kL.setOnes() *= grav_accel * dt_;
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &vdot);
EXPECT_NEAR(vdot[1], -grav_accel, eps_);
}
}
// Tests the rod in a two-point contact configuration with both sticking and
// sliding contacts. This test tests that the cross-term interaction between
// sliding friction forces and non-sliding friction forces constraints is
// correct.
void TwoPointContactCrossTerms(bool use_lcp_solver) {
// Set the state of the rod to resting.
SetRodToRestingHorizontalConfig();
// Set the sliding coefficient of friction to somewhat small and the static
// coefficient of friction to very large.
rod_->set_mu_coulomb(1e-1);
rod_->set_mu_static(1.0);
// First, construct the acceleration-level problem data as usual to set
// inertia solver and external forces.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts, &tangent_vels);
// Modify the tangent velocity on the left contact to effect a sliding
// contact. This modification can be imagined as the left end of the rod
// is touching a conveyor belt moving to the right.
tangent_vels[0] = 1.0;
// Compute the constraint problem data.
rod_->CalcConstraintProblemData(*context_, contacts, tangent_vels,
accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Check the consistency of the data.
CheckProblemConsistency(*accel_data_, contacts.size());
// Compute the constraint forces. Note that we increase cfm to prevent the
// occasional "failure to solve LCP" exception.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), accel_data_->sliding_contacts.size() +
accel_data_->non_sliding_contacts.size() * 2);
// Verify that the horizontal acceleration is zero (since mu_static is so
// large, meaning that the sticking friction force is able to overwhelm the
// sliding friction force. If the cross-constraint term FM⁻¹(Nᵀ - μQᵀ) is
// not computed properly, this acceleration might not be zero.
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &vdot);
EXPECT_NEAR(vdot[0], 0, eps_);
}
// Tests that the cross-term interaction between contact forces and generic
// unilateral constraints is computed correctly at the acceleration level.
void ContactLimitCrossTermAccel(bool use_lcp_solver) {
// Set the state of the rod to resting.
SetRodToRestingHorizontalConfig();
// Set the sliding coefficient of friction to zero (it won't be used) and
// the static coefficient of friction to a relatively large value.
rod_->set_mu_coulomb(0.0);
rod_->set_mu_static(1.0);
// First, construct the acceleration-level problem data as normal to set
// inertia solver and external forces.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts, &tangent_vels);
// Compute the constraint problem data.
rod_->CalcConstraintProblemData(*context_, contacts, tangent_vels,
accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Add some horizontal force.
accel_data_->tau[0] = 1.0;
// Construct the problem as a limit constraint preventing movement in the
// upward direction.
const int ngc = get_rod_num_coordinates();
const int num_generic_unilateral_constraints = 1;
accel_data_->kL.resize(num_generic_unilateral_constraints);
// Set the Jacobian entry- in this case, the limit is an upper limit on the
// second coordinate (vertical position). The constraint is: v̇₂ ≤ 0, which
// we transform to the form: -v̇₂ ≥ 0 (explaining the provenance of the minus
// sign in L).
const int num_limit_constraints = 1;
MatrixX<double> L(accel_data_->kL.size(), ngc);
L.setZero();
L(0, 1) = -1;
accel_data_->L_mult = [&L](const VectorX<double>& v) -> VectorX<double> {
return L * v;
};
accel_data_->L_transpose_mult =
[&L](const VectorX<double>& v) -> VectorX<double> {
return L.transpose() * v;
};
accel_data_->kL.setZero(num_limit_constraints);
// Check the consistency of the data.
CheckProblemConsistency(*accel_data_, contacts.size());
// Compute the constraint forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), accel_data_->non_sliding_contacts.size() * 2 + 1);
// Verify that the horizontal and vertical acceleration of the rod c.o.m.
// is zero.
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &vdot);
EXPECT_NEAR(vdot[0], 0, eps_);
EXPECT_NEAR(vdot[1], 0, eps_);
}
// Tests the rod in a two-point contacting configuration *realized through
// a configuration limit constraint*. No frictional forces are applied, so
// any velocity projections along directions other than the contact normal
// will be irrelevant.
void TwoPointAsLimit(bool use_lcp_solver) {
// Set the state of the rod to resting on its side.
SetRodToRestingHorizontalConfig();
// First, construct the acceleration-level problem data as normal to set
// inertia solver and external forces.
CalcConstraintAccelProblemData(accel_data_.get());
accel_data_->use_complementarity_problem_solver = use_lcp_solver;
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
const int ngc = get_rod_num_coordinates();
accel_data_->sliding_contacts.resize(0);
accel_data_->non_sliding_contacts.resize(0);
accel_data_->mu_sliding.resize(0);
accel_data_->mu_non_sliding.resize(0);
accel_data_->r.resize(0);
accel_data_->N_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
accel_data_->kN.resize(0);
accel_data_->F_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
accel_data_->F_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
accel_data_->kF.resize(0);
accel_data_->kL.resize(1);
accel_data_->N_minus_muQ_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
// Set the Jacobian entry- in this case, the limit is a lower limit on the
// second coordinate (vertical position).
const int num_limit_constraints = 1;
MatrixX<double> L(accel_data_->kL.size(), ngc);
L.setZero();
L(0, 1) = 1;
accel_data_->L_mult = [&L](const VectorX<double>& v) -> VectorX<double> {
return L * v;
};
accel_data_->L_transpose_mult =
[&L](const VectorX<double>& v) -> VectorX<double> {
return L.transpose() * v;
};
accel_data_->kL.setZero(num_limit_constraints);
// Compute the constraint forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), 1);
// Verify that the normal force exactly opposes gravity.
const double mg = std::fabs(rod_->get_gravitational_acceleration()) *
rod_->get_rod_mass();
EXPECT_NEAR(cf[0], mg, eps_);
// Set the Jacobian entry- in this case, the limit is an upper limit on the
// second coordinate (vertical position).
L *= -1;
// Reverse the external force (gravity) on the rod. tau was set by the
// call to Rod2D::CalcConstraintProblemData().
accel_data_->tau *= -1;
// Recompute the constraint forces, and verify that they're still equal
// to the force from gravity. Note: if the forces were to be applied to the
// rod, one will need to compute Lᵀcf[0] to obtain the generalized force;
// this is how we can handle upper and lower limits with only non-negativity
// constraints.
solver_.SolveConstraintProblem(*accel_data_, &cf);
EXPECT_EQ(cf.size(), 1);
EXPECT_NEAR(cf[0], mg, eps_);
// Verify that the vertical acceleration is zero.
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*accel_data_, cf, &vdot);
EXPECT_NEAR(vdot[1], 0, eps_);
}
// Tests the rod in a two-point contacting configuration *realized through
// a configuration limit constraint*. No frictional forces are applied, so
// any velocity projections along directions other than the contact normal
// will be irrelevant.
void TwoPointAsLimitDiscretized() {
// Set the state of the rod to resting on its side.
SetRodToRestingHorizontalConfig();
// First, construct the acceleration-level problem data as normal to set
// inertia solver and external forces.
CalcDiscreteTimeProblemData(dt_, vel_data_.get());
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
const int ngc = get_rod_num_coordinates();
vel_data_->mu.resize(0);
vel_data_->r.resize(0);
vel_data_->N_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
vel_data_->kN.resize(0);
vel_data_->gammaN.resize(0);
vel_data_->F_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
vel_data_->F_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
vel_data_->kF.resize(0);
vel_data_->gammaF.resize(0);
vel_data_->gammaE.resize(0);
vel_data_->kL.resize(1);
vel_data_->gammaL.setZero(1);
vel_data_->N_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
// Set the Jacobian entry- in this case, the limit is a lower limit on the
// second coordinate (vertical position).
const int num_limit_constraints = 1;
MatrixX<double> L(vel_data_->kL.size(), ngc);
L.setZero();
L(0, 1) = 1;
vel_data_->L_mult = [&L](const VectorX<double>& v) -> VectorX<double> {
return L * v;
};
vel_data_->L_transpose_mult =
[&L](const VectorX<double>& v) -> VectorX<double> {
return L.transpose() * v;
};
vel_data_->kL.setZero(num_limit_constraints);
// Compute the constraint forces.
VectorX<double> cf;
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), 1);
// Verify that the normal force exactly opposes gravity.
const double mg = std::fabs(rod_->get_gravitational_acceleration()) *
rod_->get_rod_mass();
EXPECT_NEAR(cf[0], mg, eps_);
// Set the Jacobian entry- in this case, the limit is an upper limit on the
// second coordinate (vertical position).
L *= -1;
// Reverse the external force (gravity) on the rod. tau was set by the
// call to Rod2D::CalcConstraintProblemData().
vel_data_->Mv *= -1;
// Recompute the constraint forces, and verify that they're still equal
// to the force from gravity. Note: if the forces were to be applied to the
// rod, one will need to compute Lᵀcf[0] to obtain the generalized force;
// this is how we can handle upper and lower limits with only non-negativity
// constraints.
SolveDiscretizationProblem(*vel_data_, dt_, &cf);
EXPECT_EQ(cf.size(), 1);
EXPECT_NEAR(cf[0], mg, eps_);
// Verify that the vertical acceleration is zero.
const VectorX<double> v0 = rod_->GetRodVelocity(*context_);
VectorX<double> vdot;
solver_.ComputeGeneralizedAcceleration(*vel_data_, v0, cf, dt_, &vdot);
EXPECT_NEAR(vdot[1], 0, eps_);
}
private:
// The time-step size for discretization. Note: if the time step is too
// large, the accuracy might be too low for the necessary effect to emerge).
// But we also want the step size to be large enough to test robustness. The
// selected value should be a good compromise.
const double dt_{1e-4};
};
// Tests the rod in single-point sticking configurations.
TEST_F(Constraint2DSolverTest, SinglePointStickingBothSigns) {
// Test sticking with applied force to the right (true) and the left (false).
SinglePointSticking(kForceAppliedToRight);
SinglePointSticking(kForceAppliedToLeft);
SinglePointStickingDiscretized(kForceAppliedToRight);
SinglePointStickingDiscretized(kForceAppliedToLeft);
}
// Tests the rod in a two-point sticking configurations.
TEST_F(Constraint2DSolverTest, TwoPointStickingSign) {
// Test sticking with applied force to the right and the left, and with both
// the LCP solver and the linear system solver.
TwoPointSticking(kForceAppliedToRight, kLCPSolver);
TwoPointSticking(kForceAppliedToLeft, kLCPSolver);
TwoPointSticking(kForceAppliedToRight, kLinearSystemSolver);
TwoPointSticking(kForceAppliedToLeft, kLinearSystemSolver);
}
// Tests the rod in two-point non-sliding configurations that will transition
// to sliding.
TEST_F(Constraint2DSolverTest, TwoPointNonSlidingToSlidingSign) {
// Test sticking with applied force to the right (true) and the left (false).
TwoPointNonSlidingToSliding(kForceAppliedToRight);
TwoPointNonSlidingToSliding(kForceAppliedToLeft);
TwoPointNonSlidingToSlidingDiscretized(kForceAppliedToRight);
TwoPointNonSlidingToSlidingDiscretized(kForceAppliedToLeft);
}
// Tests the rod in a two-point impact which is insufficient to put the rod
// into stiction, with pre-impact velocity in two directions (right = true,
// left = false).
TEST_F(Constraint2DSolverTest, TwoPointImpactNoTransitionToStictionTest) {
TwoPointImpactNoTransitionToStiction(kSlideRight);
TwoPointImpactNoTransitionToStiction(kSlideLeft);
}
// Tests the rod in a two-point impacting and sticking configuration with
// pre-impact velocity to the right (true) or left (false).
TEST_F(Constraint2DSolverTest, TwoPointImpactingAndStickingTest) {
TwoPointImpactingAndSticking(kSlideRight);
TwoPointImpactingAndSticking(kSlideLeft);
}
// Tests the rod in a two-point sliding configuration, both to the right
// and to the left and using both the LCP and linear system solvers.
TEST_F(Constraint2DSolverTest, TwoPointSlidingTest) {
Sliding(kSlideRight, false /* not upright */, kLCPSolver);
Sliding(kSlideLeft, false /* not upright */, kLCPSolver);
Sliding(kSlideRight, false /* not upright */, kLinearSystemSolver);
Sliding(kSlideLeft, false /* not upright */, kLinearSystemSolver);
SlidingDiscretized(kSlideRight, false /* not upright */);
SlidingDiscretized(kSlideLeft, false /* not upright */);
}
// Tests the rod in a single point sliding configuration, with sliding both
// to the right and to the left and using both the LCP and linear system
// solvers.
TEST_F(Constraint2DSolverTest, SinglePointSlidingTest) {
Sliding(kSlideRight, true /* upright */, kLCPSolver);
Sliding(kSlideLeft, true /* upright */, kLCPSolver);
Sliding(kSlideRight, true /* upright */, kLinearSystemSolver);
Sliding(kSlideLeft, true /* upright */, kLinearSystemSolver);
SlidingDiscretized(kSlideRight, true /* upright */);
SlidingDiscretized(kSlideLeft, true /* upright */);
}
// Tests the rod in a single point sliding configuration, with sliding both
// to the right and to the left, and with a bilateral constraint imposed, and
// using both the LCP and linear system solvers.
TEST_F(Constraint2DSolverTest, SinglePointSlidingPlusBilateralTest) {
SlidingPlusBilateral(kSlideRight, kLCPSolver);
SlidingPlusBilateral(kSlideLeft, kLCPSolver);
SlidingPlusBilateral(kSlideRight, kLinearSystemSolver);
SlidingPlusBilateral(kSlideLeft, kLinearSystemSolver);
SlidingPlusBilateralDiscretized(kSlideRight);
SlidingPlusBilateralDiscretized(kSlideLeft);
}
// Tests the rod in a single point impacting configuration, with sliding both
// to the right and to the left, and with a bilateral constraint imposed.
TEST_F(Constraint2DSolverTest, SinglePointSlidingImpactPlusBilateralTest) {
SlidingPlusBilateralImpact(kSlideRight);
SlidingPlusBilateralImpact(kSlideLeft);
}
// Tests the rod in a one-point sliding contact configuration with a second
// constraint that prevents horizontal acceleration. This test tests the
// interaction between contact and limit constraints using both the LCP solver
// and the linear system solver.
TEST_F(Constraint2DSolverTest, OnePointPlusLimitTest) {
OnePointPlusLimit(kLCPSolver);
OnePointPlusLimit(kLinearSystemSolver);
OnePointPlusLimitDiscretized();
}
// Tests the rod in a two-point contact configuration with both sticking and
// sliding contacts and using both the LCP and linear system solvers. This test
// tests that the cross-term interaction between sliding friction forces and
// non-sliding friction forces constraints is correct.
TEST_F(Constraint2DSolverTest, TwoPointContactCrossTermsTest) {
TwoPointContactCrossTerms(kLCPSolver);
TwoPointContactCrossTerms(kLinearSystemSolver);
}
// Tests that the cross-term interaction between contact forces and generic
// unilateral constraints is computed correctly at the acceleration level. Tests
// both the LCP solver and the linear system solver.
TEST_F(Constraint2DSolverTest, ContactLimitCrossTermAccelTest) {
ContactLimitCrossTermAccel(kLCPSolver);
ContactLimitCrossTermAccel(kLinearSystemSolver);
}
// Tests the rod in a two-point contacting configuration *realized through
// a configuration limit constraint* using both the LCP and linear system
// solvers. No frictional forces are applied, so any velocity projections along
// directions other than the contact normal will be irrelevant.
TEST_F(Constraint2DSolverTest, TwoPointAsLimitTest) {
TwoPointAsLimit(kLCPSolver);
TwoPointAsLimit(kLinearSystemSolver);
TwoPointAsLimitDiscretized();
}
// Tests the rod in a two-point configuration, in a situation where a force
// pulls the rod upward (and no contact forces should be applied).
TEST_F(Constraint2DSolverTest, TwoPointPulledUpward) {
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
// Set the state of the rod to resting on its side with no velocity.
SetRodToRestingHorizontalConfig();
// Compute the problem data.
CalcConstraintAccelProblemData(accel_data_.get(), contact_dup,
friction_dir_dup);
// Add a force pulling the rod upward.
accel_data_->tau[1] += 100.0;
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveConstraintProblem(*accel_data_, &cf);
// Verify that the contact forces are zero.
EXPECT_LT(cf.norm(), eps_);
}
}
}
// Tests the rod in a two-point configuration, in a situation where the rod
// is moving upward, so no impulsive forces should be applied.
TEST_F(Constraint2DSolverTest, NoImpactImpliesNoImpulses) {
// Duplicate contact points up to two times and the friction directions up
// to three times.
for (int contact_dup = 0; contact_dup < 3; ++contact_dup) {
for (int friction_dir_dup = 0; friction_dir_dup < 4; ++friction_dir_dup) {
// Set the state of the rod to resting on its side with upward velocity.
SetRodToUpwardMovingHorizontalConfig();
// Compute the problem data.
CalcConstraintProblemDataForImpact(vel_data_.get(), contact_dup,
friction_dir_dup);
// Compute the contact forces.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify that the impact forces are zero.
EXPECT_LT(cf.norm(), eps_);
}
}
}
// Tests that the cross-term interaction between contact forces and generic
// unilateral constraints is computed correctly at the velocity level.
TEST_F(Constraint2DSolverTest, ContactLimitCrossTermVel) {
// Set the state of the rod to resting.
SetRodToSlidingImpactingHorizontalConfig(true);
// Set the coefficient of friction (mu_static won't be used in this problem,
// but an exception will be thrown if mu_coulomb is greater than mu_static)
// to a relatively large number.
rod_->set_mu_coulomb(1.0);
rod_->set_mu_static(1.0);
// First, construct the velocity-level problem data as normal to set
// inertia solver and external forces.
std::vector<Vector2d> contacts;
std::vector<double> tangent_vels;
rod_->GetContactPoints(*context_, &contacts);
rod_->GetContactPointsTangentVelocities(*context_, contacts, &tangent_vels);
// Compute the constraint problem data.
rod_->CalcImpactProblemData(*context_, contacts, vel_data_.get());
// Add in some horizontal velocity to test the transition to stiction too.
vel_data_->Mv[0] = 1.0;
const VectorX<double> v0 = vel_data_->solve_inertia(vel_data_->Mv);
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
const int ngc = get_rod_num_coordinates();
const int num_generic_unilateral_constraints = 1;
vel_data_->kL.resize(num_generic_unilateral_constraints);
vel_data_->gammaL.setZero(num_generic_unilateral_constraints);
// Set the Jacobian entry- in this case, the limit is an upper limit on the
// second coordinate (vertical position). The constraint is: v₂ ≤ 0, which
// we transform to the form: -v₂ ≥ 0 (explaining the provenance of the minus
// sign in L).
const int num_limit_constraints = 1;
MatrixX<double> L(vel_data_->kL.size(), ngc);
L.setZero();
L(0, 1) = -1;
vel_data_->L_mult = [&L](const VectorX<double>& vv) -> VectorX<double> {
return L * vv;
};
vel_data_->L_transpose_mult =
[&L](const VectorX<double>& vv) -> VectorX<double> {
return L.transpose() * vv;
};
vel_data_->kL.setZero(num_limit_constraints);
// Check the consistency of the data.
CheckProblemConsistency(*vel_data_, contacts.size());
// Compute the constraint forces. Note that we increase cfm to prevent the
// occasional "failure to solve LCP" exception.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), vel_data_->mu.size() * 2 + 1);
// Verify that the horizontal velocity is unchanged and that the vertical
// velocity is zero.
VectorX<double> dv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &dv);
EXPECT_NEAR(v0[0] + dv[0], 0, eps_);
EXPECT_NEAR(v0[1] + dv[1], 0, eps_);
}
// Tests the rod in a two-point configuration *realized through a configuration
// limit constraint*, velocity-level version. No frictional forces are applied,
// so any velocity projections along directions other than the contact normal
// will be irrelevant.
TEST_F(Constraint2DSolverTest, TwoPointImpactAsLimit) {
// Set the state of the rod to impacting on its side.
SetRodToSlidingImpactingHorizontalConfig(true /* moving to the right */);
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
const double vert_vel = xc[4];
// First, construct the velocity-level problem data as normal to set
// inertia solver and external forces.
CalcConstraintProblemDataForImpact(vel_data_.get());
// Compute v.
VectorX<double> v0 = vel_data_->solve_inertia(vel_data_->Mv);
// Construct the problem as a limit constraint preventing movement in the
// downward direction.
const int ngc = get_rod_num_coordinates();
vel_data_->mu.resize(0);
vel_data_->r.resize(0);
vel_data_->N_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
vel_data_->N_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
vel_data_->kN.resize(0);
vel_data_->gammaN.resize(0);
vel_data_->F_mult = [](const VectorX<double>&) {
return VectorX<double>(0);
};
vel_data_->F_transpose_mult = [ngc](const VectorX<double>&) {
return VectorX<double>::Zero(ngc);
};
vel_data_->kF.resize(0);
vel_data_->gammaF.resize(0);
vel_data_->gammaE.resize(0);
// Set the Jacobian entry- in this case, the limit is a lower limit on the
// second coordinate (vertical position).
const int num_limits = 1;
MatrixX<double> L(num_limits, ngc);
L.setZero();
L(0, 1) = 1;
vel_data_->L_mult = [&L](const VectorX<double>& w) -> VectorX<double> {
return L * w;
};
vel_data_->L_transpose_mult =
[&L](const VectorX<double>& w) -> VectorX<double> {
return L.transpose() * w;
};
vel_data_->kL.setZero(num_limits);
vel_data_->gammaL.setZero(num_limits);
// Compute the constraint impulses.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Verify the size of cf is as expected.
EXPECT_EQ(cf.size(), 1);
// Verify that the normal force exactly opposes the momentum.
const double mv = std::fabs(vert_vel) * rod_->get_rod_mass();
EXPECT_NEAR(cf[0], mv, eps_);
// Set the Jacobian entry- in this case, the limit is an upper limit on the
// second coordinate (vertical position).
L *= -1;
// Reverse the velocity on the rod, which was set by the call to
// Rod2D::CalcImpactProblemData().
vel_data_->Mv *= -1;
v0 *= -1;
// Recompute the constraint impulses, and verify that they're still equal
// to the momentum. Note: if the impulses were to be applied to the
// rod, one will need to compute Lᵀcf[0] to obtain the generalized impulse;
// this is how we can handle upper and lower limits with only non-negativity
// constraints.
solver_.SolveImpactProblem(*vel_data_, &cf);
EXPECT_EQ(cf.size(), 1);
EXPECT_NEAR(cf[0], mv, eps_);
// Verify that the vertical velocity is zero.
VectorX<double> vnew;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &vnew);
EXPECT_NEAR(v0[1] + vnew[1], 0, eps_);
// Now test whether constraint stabilization works by trying to get the rod to
// move downward as fast as it's currently moving upward
// (according to vel_data_->v). Note that Lv is negative, indicating "error"
// to be corrected (as desired in this test).
vel_data_->kL = L * v0;
// Recompute the constraint impulses, and verify that they're now equal to
// twice the momentum.
solver_.SolveImpactProblem(*vel_data_, &cf);
EXPECT_EQ(cf.size(), 1);
EXPECT_NEAR(cf[0], mv * 2, eps_);
}
// Tests that a purely bilaterally constrained problem is handled correctly.
TEST_F(Constraint2DSolverTest, BilateralOnly) {
// Set the rod to a ballistic state.
ContinuousState<double>& xc = context_->get_mutable_continuous_state();
xc[0] = 0.0; // com horizontal position.
xc[1] = 10.0; // com vertical position.
xc[2] = 0.0; // rod rotation.
xc[3] = 0.0; // no horizontal velocity.
xc[4] = 0.0; // upward velocity.
xc[5] = 0.0; // no angular velocity.
// Compute the problem data.
CalcConstraintProblemDataForImpact(vel_data_.get());
// Compute the generalized velocity.
const VectorX<double> v = vel_data_->solve_inertia(vel_data_->Mv);
// Add in bilateral constraints on rotational motion.
vel_data_->G_mult = [](const VectorX<double>& w) -> VectorX<double> {
VectorX<double> result(1); // Only one constraint.
result[0] = w[2]; // Constrain the angular velocity to be zero.
return result;
};
vel_data_->G_transpose_mult =
[this](const VectorX<double>& f) -> VectorX<double> {
// An impulsive force (torque) applied to the third component needs no
// transformation.
DRAKE_DEMAND(f.size() == 1);
VectorX<double> result(get_rod_num_coordinates());
result.setZero();
result[2] = f[0];
return result;
};
// Indicate through construction of the kG term that the system already has
// angular orientation (which violates our desire to keep the rod at
// zero rotation).
vel_data_->kG.resize(1);
vel_data_->kG[0] = 1.0; // Indicate a ccw orientation.
// Compute the impact forces.
VectorX<double> cf;
solver_.SolveImpactProblem(*vel_data_, &cf);
// Get the change in generalized velocity and verify that the angular
// velocity has changed counter-clockwise.
VectorX<double> gv;
solver_.ComputeGeneralizedVelocityChange(*vel_data_, cf, &gv);
EXPECT_NEAR(v[2] + gv[2], -vel_data_->kG[0], eps_ * cf.size());
}
} // namespace
} // namespace rod2d
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/metrics.py | import numpy as np
def _wrap_to(value, low, high):
assert low < high
return ((value - low) % (high - low)) + low
def deviation_from_upright_equilibrium(x):
return np.array([
# The upright equilibrium theta0 is pi; rotate this angle accordingly.
_wrap_to(x[0], 0, 2 * np.pi) - np.pi,
_wrap_to(x[1], -np.pi, np.pi),
x[2],
x[3],
])
def final_state_cost(x_tape):
"""
Returns the L2-norm of the deviation from the upright equilibrium at the
final state in `x_tape`.
"""
return np.linalg.norm(deviation_from_upright_equilibrium(x_tape[:, -1]))
def ensemble_cost(x_tapes):
"""
Returns the total cost for the trajectories in `x_tapes`.
"""
per_trajectory_costs = [final_state_cost(x_tape) for x_tape in x_tapes]
# Return the L1-norm of the per-trajectory-costs, scaled by the number of
# trajectories.
return np.linalg.norm(per_trajectory_costs, 1) / len(x_tapes)
def is_success(x_tape):
"""
Returns true if the final state in `x_tape` is close to the upright
equilibrium.
"""
return final_state_cost(x_tape) < 1e-3
def success_rate(x_tapes):
"""
Given a list of x-tapes, returns the fraction of the total for which
`is_success()` returns True.
"""
return np.sum([is_success(x_tape) for x_tape in x_tapes]) / len(x_tapes)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_input.cc | #include "drake/examples/acrobot/acrobot_input.h"
namespace drake {
namespace examples {
namespace acrobot {
const int AcrobotInputIndices::kNumCoordinates;
const int AcrobotInputIndices::kTau;
const std::vector<std::string>& AcrobotInputIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"tau",
});
return coordinates.access();
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_params.cc | #include "drake/examples/acrobot/acrobot_params.h"
namespace drake {
namespace examples {
namespace acrobot {
const int AcrobotParamsIndices::kNumCoordinates;
const int AcrobotParamsIndices::kM1;
const int AcrobotParamsIndices::kM2;
const int AcrobotParamsIndices::kL1;
const int AcrobotParamsIndices::kL2;
const int AcrobotParamsIndices::kLc1;
const int AcrobotParamsIndices::kLc2;
const int AcrobotParamsIndices::kIc1;
const int AcrobotParamsIndices::kIc2;
const int AcrobotParamsIndices::kB1;
const int AcrobotParamsIndices::kB2;
const int AcrobotParamsIndices::kGravity;
const std::vector<std::string>& AcrobotParamsIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"m1",
"m2",
"l1",
"l2",
"lc1",
"lc2",
"Ic1",
"Ic2",
"b1",
"b2",
"gravity",
});
return coordinates.access();
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/run_plant_w_lcm.cc | /*
* A simulated acrobot plant that communicates to its controller through LCM,
* implemented by the following Diagram system:
*
* LcmSubscriberSystem -->
* AcrobotCommandReceiver -->
* AcrobotPlant -->
* AcrobotStateSender -->
* LcmPublisherSystem
*
*/
#include <memory>
#include <thread>
#include <gflags/gflags.h>
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_lcm.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/lcmt_acrobot_u.hpp"
#include "drake/lcmt_acrobot_x.hpp"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/lcm/lcm_interface_system.h"
#include "drake/systems/lcm/lcm_publisher_system.h"
#include "drake/systems/lcm/lcm_subscriber_system.h"
DEFINE_double(simulation_sec, std::numeric_limits<double>::infinity(),
"Number of seconds to simulate.");
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
using std::chrono::milliseconds;
using std::this_thread::sleep_for;
namespace drake {
namespace examples {
namespace acrobot {
namespace {
int DoMain() {
drake::systems::DiagramBuilder<double> builder;
const std::string channel_x = "acrobot_xhat";
const std::string channel_u = "acrobot_u";
auto lcm = builder.AddSystem<systems::lcm::LcmInterfaceSystem>();
auto acrobot = builder.AddSystem<AcrobotPlant>();
acrobot->set_name("acrobot");
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(
&builder, acrobot->get_output_port(0), scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph, lcm);
// Creates command receiver and subscriber.
auto command_sub = builder.AddSystem(
systems::lcm::LcmSubscriberSystem::Make<lcmt_acrobot_u>(channel_u, lcm));
auto command_receiver = builder.AddSystem<AcrobotCommandReceiver>();
builder.Connect(command_sub->get_output_port(),
command_receiver->get_input_port(0));
// Creates state sender and publisher.
auto state_pub = builder.AddSystem(
systems::lcm::LcmPublisherSystem::Make<lcmt_acrobot_x>(channel_x, lcm));
auto state_sender = builder.AddSystem<AcrobotStateSender>();
builder.Connect(state_sender->get_output_port(0),
state_pub->get_input_port());
// Connects plant to command receiver and state sender.
builder.Connect(command_receiver->get_output_port(0),
acrobot->get_input_port(0));
builder.Connect(acrobot->get_output_port(0), state_sender->get_input_port(0));
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
// Sets an initial condition near the stable fixed point.
systems::Context<double>& acrobot_context =
diagram->GetMutableSubsystemContext(*acrobot,
&simulator.get_mutable_context());
AcrobotState<double>* x0 = dynamic_cast<AcrobotState<double>*>(
&acrobot_context.get_mutable_continuous_state_vector());
DRAKE_DEMAND(x0 != nullptr);
x0->set_theta1(0.1);
x0->set_theta2(0.1);
x0->set_theta1dot(0.0);
x0->set_theta2dot(0.0);
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.Initialize();
simulator.AdvanceTo(FLAGS_simulation_sec);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::DoMain();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/BUILD.bazel | load("//tools/install:install_data.bzl", "install_data")
load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_binary",
"drake_cc_googletest",
"drake_cc_library",
)
load("//tools/skylark:drake_data.bzl", "models_filegroup")
load(
"//tools/skylark:drake_py.bzl",
"drake_py_binary",
"drake_py_library",
"drake_py_test",
"drake_py_unittest",
)
package(default_visibility = ["//visibility:private"])
models_filegroup(
name = "models",
visibility = ["//visibility:public"],
)
install_data(
name = "install_data",
data = [":models"],
visibility = ["//visibility:public"],
)
drake_cc_library(
name = "acrobot_input",
srcs = ["acrobot_input.cc"],
hdrs = [
"acrobot_input.h",
"gen/acrobot_input.h",
],
visibility = ["//visibility:public"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_py_library(
name = "acrobot_io",
srcs = ["acrobot_io.py"],
deps = [
"//bindings/pydrake",
],
)
drake_cc_library(
name = "acrobot_params",
srcs = ["acrobot_params.cc"],
hdrs = [
"acrobot_params.h",
"gen/acrobot_params.h",
],
visibility = ["//visibility:public"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_cc_library(
name = "acrobot_state",
srcs = ["acrobot_state.cc"],
hdrs = [
"acrobot_state.h",
"gen/acrobot_state.h",
],
visibility = ["//visibility:public"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_cc_library(
name = "spong_controller_params",
srcs = ["spong_controller_params.cc"],
hdrs = [
"gen/spong_controller_params.h",
"spong_controller_params.h",
],
visibility = ["//visibility:public"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_cc_library(
name = "acrobot_lcm",
srcs = ["acrobot_lcm.cc"],
hdrs = ["acrobot_lcm.h"],
deps = [
":acrobot_state",
"//lcmtypes:acrobot",
"//systems/framework:leaf_system",
"//systems/framework:vector",
],
)
drake_cc_library(
name = "acrobot_plant",
srcs = ["acrobot_plant.cc"],
hdrs = ["acrobot_plant.h"],
visibility = ["//visibility:public"],
deps = [
":acrobot_input",
":acrobot_params",
":acrobot_state",
"//common:default_scalars",
"//systems/controllers:linear_quadratic_regulator",
"//systems/framework",
"//systems/sensors:rotary_encoders",
],
)
drake_cc_library(
name = "acrobot_geometry",
srcs = ["acrobot_geometry.cc"],
hdrs = ["acrobot_geometry.h"],
visibility = ["//visibility:public"],
deps = [
":acrobot_params",
":acrobot_plant",
"//geometry:geometry_roles",
"//geometry:scene_graph",
"//math:geometric_transform",
"//systems/framework:diagram_builder",
"//systems/framework:leaf_system",
],
)
drake_py_library(
name = "metrics",
srcs = ["metrics.py"],
)
drake_cc_library(
name = "spong_controller",
srcs = ["spong_controller.cc"],
hdrs = ["spong_controller.h"],
visibility = ["//visibility:public"],
deps = [
":acrobot_plant",
":spong_controller_params",
"//common:default_scalars",
"//math:wrap_to",
"//systems/controllers:linear_quadratic_regulator",
"//systems/framework:leaf_system",
"//systems/framework:vector",
"//systems/primitives:linear_system",
],
)
drake_py_library(
# This is the library form of spong_sim.py.
name = "spong_sim_lib_py",
srcs = ["spong_sim.py"],
deps = [
":acrobot_io",
"//bindings/pydrake",
],
)
drake_py_binary(
# This is the directly-runnable form of spong_sim.py.
name = "spong_sim_main_py",
srcs = ["spong_sim.py"],
main = "spong_sim.py",
deps = [
":acrobot_io",
"//bindings/pydrake",
],
)
drake_cc_binary(
name = "spong_sim_main_cc",
srcs = ["spong_sim.cc"],
deps = [
"//common:add_text_logging_gflags",
"//common:name_value",
"//common/schema:stochastic",
"//common/yaml",
"//examples/acrobot:acrobot_plant",
"//examples/acrobot:spong_controller",
"//systems/analysis:simulator",
"//systems/framework:diagram_builder",
"//systems/primitives:vector_log_sink",
],
)
# Note: This is a development tool for testing LCM communication
# but does not actually provide test coverage as a stand-alone
# executable.
drake_cc_binary(
name = "acrobot_lcm_msg_generator",
testonly = 1,
srcs = ["test/acrobot_lcm_msg_generator.cc"],
deps = [
"//lcm",
"//lcmtypes:acrobot",
"//systems/analysis:simulator",
],
)
drake_py_binary(
name = "optimizer_demo",
srcs = ["optimizer_demo.py"],
add_test_rule = True,
data = [
":spong_sim_main_cc",
":test/example_stochastic_scenario.yaml",
],
test_rule_args = [
"--ensemble_size=3",
"--num_evaluations=3",
],
deps = [
":acrobot_io",
":metrics",
],
)
drake_cc_binary(
name = "run_lqr",
srcs = ["run_lqr.cc"],
add_test_rule = 1,
test_rule_args = [
"-simulation_sec=1.0",
"-realtime_factor=0.0",
],
deps = [
":acrobot_geometry",
":acrobot_plant",
"//geometry:drake_visualizer",
"//systems/analysis:simulator",
"@gflags",
],
)
drake_cc_binary(
name = "run_lqr_w_estimator",
testonly = 1,
srcs = ["run_lqr_w_estimator.cc"],
add_test_rule = 1,
test_rule_args = [
"-simulation_sec=1.0",
"-realtime_factor=0.0",
],
deps = [
":acrobot_geometry",
":acrobot_plant",
"//common/proto:call_python",
"//geometry:drake_visualizer",
"//systems/analysis:simulator",
"//systems/estimators:kalman_filter",
"//systems/framework:diagram",
"//systems/primitives:linear_system",
"//systems/primitives:vector_log_sink",
"//systems/sensors:rotary_encoders",
"@gflags",
],
)
drake_cc_binary(
name = "run_passive",
srcs = ["run_passive.cc"],
add_test_rule = 1,
test_rule_args = [
"-simulation_sec=1.0",
"-realtime_factor=0.0",
],
deps = [
":acrobot_geometry",
":acrobot_plant",
"//geometry:drake_visualizer",
"//systems/analysis:simulator",
"//systems/framework:diagram",
"@gflags",
],
)
drake_cc_binary(
name = "run_swing_up",
srcs = [
"run_swing_up.cc",
],
add_test_rule = 1,
test_rule_args = [
# N.B. We can't set -simulation_sec here, because the demo program has
# success criteria that it asserts after the simulation expires.
"-realtime_factor=0.0",
],
deps = [
":acrobot_geometry",
":acrobot_plant",
":spong_controller",
":spong_controller_params",
"//geometry:drake_visualizer",
"//solvers:snopt_solver",
"//systems/analysis:simulator",
"@gflags",
],
)
drake_cc_binary(
name = "run_swing_up_traj_optimization",
srcs = ["test/run_swing_up_traj_optimization.cc"],
# TODO(ggould) Temporarily turned off due to mac test failures, see #10276.
add_test_rule = 0,
tags = ["snopt"],
test_rule_args = ["-realtime_factor=0.0"],
deps = [
":acrobot_geometry",
":acrobot_plant",
"//common:is_approx_equal_abstol",
"//geometry:drake_visualizer",
"//planning/trajectory_optimization:direct_collocation",
"//solvers:snopt_solver",
"//solvers:solve",
"//systems/analysis:simulator",
"//systems/controllers:finite_horizon_linear_quadratic_regulator",
"//systems/primitives:trajectory_source",
"@gflags",
],
)
drake_cc_binary(
name = "spong_controller_w_lcm",
srcs = [
"spong_controller_w_lcm.cc",
],
add_test_rule = 1,
test_rule_args = [
"-time_limit_sec=1.0",
],
deps = [
":acrobot_lcm",
":acrobot_plant",
":spong_controller",
":spong_controller_params",
"//systems/analysis:simulator",
"//systems/lcm",
"@gflags",
],
)
drake_cc_binary(
name = "run_plant_w_lcm",
srcs = ["run_plant_w_lcm.cc"],
add_test_rule = 1,
test_rule_args = [
"-simulation_sec=1.0",
"-realtime_factor=0.0",
],
test_rule_size = "medium",
deps = [
":acrobot_geometry",
":acrobot_lcm",
":acrobot_plant",
"//geometry:drake_visualizer",
"//systems/analysis:simulator",
"//systems/controllers:linear_quadratic_regulator",
"//systems/lcm",
"@gflags",
],
)
drake_py_unittest(
name = "acrobot_io_test",
data = [
":test/example_scenario.yaml",
],
deps = [
":acrobot_io",
"//bindings/pydrake",
],
)
drake_cc_googletest(
name = "acrobot_plant_test",
deps = [
":acrobot_plant",
"//common/test_utilities:eigen_matrix_compare",
"//common/test_utilities:expect_no_throw",
],
)
drake_cc_googletest(
name = "acrobot_geometry_test",
deps = [
":acrobot_geometry",
":acrobot_plant",
],
)
drake_py_unittest(
name = "metrics_test",
deps = [
":metrics",
],
)
drake_cc_googletest(
name = "multibody_dynamics_test",
data = [":models"],
deps = [
":acrobot_plant",
"//common/test_utilities:eigen_matrix_compare",
"//multibody/parsing",
],
)
drake_py_unittest(
name = "spong_sim_lib_py_test",
deps = [
":spong_sim_lib_py",
],
)
drake_py_test(
name = "spong_sim_main_py_test",
srcs = ["test/spong_sim_main_test.py"],
allow_import_unittest = True,
data = [
":spong_sim_main_py",
":test/example_scenario.yaml",
],
main = "test/spong_sim_main_test.py",
deps = [
":acrobot_io",
"//bindings/pydrake",
],
)
drake_py_test(
name = "spong_sim_main_cc_test",
srcs = ["test/spong_sim_main_test.py"],
allow_import_unittest = True,
args = ["--cc"],
data = [
":spong_sim_main_cc",
":test/example_scenario.yaml",
":test/example_stochastic_scenario.yaml",
],
main = "test/spong_sim_main_test.py",
deps = [
":acrobot_io",
"//bindings/pydrake",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_lcm.cc | #include "drake/examples/acrobot/acrobot_lcm.h"
#include "drake/lcmt_acrobot_u.hpp"
#include "drake/lcmt_acrobot_x.hpp"
namespace drake {
namespace examples {
namespace acrobot {
using systems::Context;
using systems::SystemOutput;
static const int kNumJoints = 2;
/*--------------------------------------------------------------------------*/
// methods implementation for AcrobotStateReceiver.
AcrobotStateReceiver::AcrobotStateReceiver() {
this->DeclareAbstractInputPort("lcmt_acrobot_x",
Value<lcmt_acrobot_x>{});
this->DeclareVectorOutputPort("acrobot_state",
&AcrobotStateReceiver::CopyStateOut);
}
void AcrobotStateReceiver::CopyStateOut(
const Context<double>& context, AcrobotState<double>* output) const {
const AbstractValue* input = this->EvalAbstractInput(context, 0);
DRAKE_ASSERT(input != nullptr);
const auto& state = input->get_value<lcmt_acrobot_x>();
auto output_vec = output->get_mutable_value();
output_vec(0) = state.theta1;
output_vec(1) = state.theta2;
output_vec(2) = state.theta1Dot;
output_vec(3) = state.theta2Dot;
}
/*--------------------------------------------------------------------------*/
// methods implementation for AcrobotCommandSender.
AcrobotCommandSender::AcrobotCommandSender() {
this->DeclareInputPort("elbow_torque", systems::kVectorValued, 1);
this->DeclareAbstractOutputPort("lcmt_acrobot_u",
&AcrobotCommandSender::OutputCommand);
}
void AcrobotCommandSender::OutputCommand(const Context<double>& context,
lcmt_acrobot_u* status) const {
const systems::BasicVector<double>* command =
this->EvalVectorInput(context, 0);
status->tau = command->GetAtIndex(0);
}
/*--------------------------------------------------------------------------*/
// methods implementation for AcrobotCommandReceiver
AcrobotCommandReceiver::AcrobotCommandReceiver() {
this->DeclareAbstractInputPort("lcmt_acrobot_u",
Value<lcmt_acrobot_u>());
this->DeclareVectorOutputPort("elbow_torque", 1,
&AcrobotCommandReceiver::OutputCommandAsVector);
}
void AcrobotCommandReceiver::OutputCommandAsVector(
const Context<double>& context,
systems::BasicVector<double>* output) const {
const AbstractValue* input = this->EvalAbstractInput(context, 0);
DRAKE_ASSERT(input != nullptr);
const auto& command = input->get_value<lcmt_acrobot_u>();
output->SetAtIndex(0, command.tau);
}
/*--------------------------------------------------------------------------*/
// methods implementation for AcrobotStateSender
AcrobotStateSender::AcrobotStateSender() {
this->DeclareInputPort("acrobot_state", systems::kVectorValued,
kNumJoints * 2);
this->DeclareAbstractOutputPort("lcmt_acrobot_x",
&AcrobotStateSender::OutputState);
}
void AcrobotStateSender::OutputState(const Context<double>& context,
lcmt_acrobot_x* status) const {
const systems::BasicVector<double>* state = this->EvalVectorInput(context, 0);
status->theta1 = state->GetAtIndex(0);
status->theta2 = state->GetAtIndex(1);
status->theta1Dot = state->GetAtIndex(2);
status->theta2Dot = state->GetAtIndex(3);
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/Acrobot_no_collision.urdf | <?xml version="1.0"?>
<robot name="Acrobot">
<link name="base_link">
<visual>
<geometry>
<box size="0.2 0.2 0.2"/>
</geometry>
<material name="green">
<color rgba="0 1 0 1"/>
</material>
</visual>
</link>
<link name="upper_link">
<inertial>
<origin xyz="0 0 -.5" rpy="0 0 0"/>
<mass value="1"/>
<inertia ixx="1" ixy="0" ixz="0" iyy="0.083" iyz="0" izz="1"/>
</inertial>
<visual>
<origin xyz="0 0 -0.5" rpy="0 0 0"/>
<geometry>
<cylinder length="1.1" radius="0.05"/>
</geometry>
<material name="red">
<color rgba="1 0 0 1"/>
</material>
</visual>
</link>
<link name="lower_link">
<inertial>
<origin xyz="0 0 -1" rpy="0 0 0"/>
<mass value="1"/>
<inertia ixx="1" ixy="0" ixz="0" iyy="0.33" iyz="0" izz="1"/>
</inertial>
<visual>
<origin xyz="0 0 -1" rpy="0 0 0"/>
<geometry>
<cylinder length="2.1" radius=".05"/>
</geometry>
<material name="blue">
<color rgba="0 0 1 1"/>
</material>
</visual>
</link>
<joint name="shoulder" type="continuous">
<parent link="base_link"/>
<child link="upper_link"/>
<origin xyz="0 0.15 0"/>
<axis xyz="0 1 0"/>
<dynamics damping="0.1"/>
</joint>
<joint name="elbow" type="continuous">
<parent link="upper_link"/>
<child link="lower_link"/>
<origin xyz="0 0.1 -1"/>
<axis xyz="0 1 0"/>
<dynamics damping="0.1"/>
</joint>
<transmission type="SimpleTransmission" name="elbow_trans">
<actuator name="elbow"/>
<joint name="elbow"/>
<mechanicalReduction>1</mechanicalReduction>
</transmission>
<frame name="hand" link="lower_link" xyz="0 0 -2.1" rpy="0 0 0"/>
</robot>
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/run_lqr.cc | #include <memory>
#include <gflags/gflags.h>
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
// Simple example which simulates the Acrobot, started near the upright, with an
// LQR controller designed to stabilize the unstable fixed point. Run meldis to
// see the animated result.
//
// Note: See also examples/multibody/acrobot for an almost identical test
// using the MultibodyPlant version of the Acrobot dynamics.
DEFINE_double(simulation_sec, 10.0,
"Number of seconds to simulate.");
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
int do_main() {
systems::DiagramBuilder<double> builder;
auto acrobot = builder.AddSystem<AcrobotPlant>();
acrobot->set_name("acrobot");
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(
&builder, acrobot->get_output_port(0), scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
auto controller = builder.AddSystem(BalancingLQRController(*acrobot));
controller->set_name("controller");
builder.Connect(acrobot->get_output_port(0), controller->get_input_port());
builder.Connect(controller->get_output_port(), acrobot->get_input_port(0));
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
systems::Context<double>& acrobot_context =
diagram->GetMutableSubsystemContext(*acrobot,
&simulator.get_mutable_context());
// Set an initial condition near the upright fixed point.
AcrobotState<double>* x0 = dynamic_cast<AcrobotState<double>*>(
&acrobot_context.get_mutable_continuous_state_vector());
DRAKE_DEMAND(x0 != nullptr);
x0->set_theta1(M_PI + 0.1);
x0->set_theta2(-.1);
x0->set_theta1dot(0.0);
x0->set_theta2dot(0.0);
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.Initialize();
simulator.AdvanceTo(FLAGS_simulation_sec);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::do_main();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_state.cc | #include "drake/examples/acrobot/acrobot_state.h"
namespace drake {
namespace examples {
namespace acrobot {
const int AcrobotStateIndices::kNumCoordinates;
const int AcrobotStateIndices::kTheta1;
const int AcrobotStateIndices::kTheta2;
const int AcrobotStateIndices::kTheta1dot;
const int AcrobotStateIndices::kTheta2dot;
const std::vector<std::string>& AcrobotStateIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"theta1",
"theta2",
"theta1dot",
"theta2dot",
});
return coordinates.access();
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_controller_params.cc | #include "drake/examples/acrobot/spong_controller_params.h"
namespace drake {
namespace examples {
namespace acrobot {
const int SpongControllerParamsIndices::kNumCoordinates;
const int SpongControllerParamsIndices::kKE;
const int SpongControllerParamsIndices::kKP;
const int SpongControllerParamsIndices::kKD;
const int SpongControllerParamsIndices::kBalancingThreshold;
const std::vector<std::string>&
SpongControllerParamsIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"k_e",
"k_p",
"k_d",
"balancing_threshold",
});
return coordinates.access();
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_input.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Describes the row indices of a AcrobotInput.
struct AcrobotInputIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 1;
// The index of each individual coordinate.
static const int kTau = 0;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `AcrobotInputIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class AcrobotInput final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef AcrobotInputIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c tau defaults to 0.0 Nm.
AcrobotInput() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_tau(0.0);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
AcrobotInput(const AcrobotInput& other)
: drake::systems::BasicVector<T>(other.values()) {}
AcrobotInput(AcrobotInput&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
AcrobotInput& operator=(const AcrobotInput& other) {
this->values() = other.values();
return *this;
}
AcrobotInput& operator=(AcrobotInput&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_tau(symbolic::Variable("tau"));
}
[[nodiscard]] AcrobotInput<T>* DoClone() const final {
return new AcrobotInput;
}
/// @name Getters and Setters
//@{
/// Torque at the elbow
/// @note @c tau is expressed in units of Nm.
const T& tau() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTau);
}
/// Setter that matches tau().
void set_tau(const T& tau) {
ThrowIfEmpty();
this->SetAtIndex(K::kTau, tau);
}
/// Fluent setter that matches tau().
/// Returns a copy of `this` with tau set to a new value.
[[nodiscard]] AcrobotInput<T> with_tau(const T& tau) const {
AcrobotInput<T> result(*this);
result.set_tau(tau);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& tau_ref = this->GetAtIndex(K::kTau);
a->Visit(drake::MakeNameValue("tau", &tau_ref));
}
/// See AcrobotInputIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return AcrobotInputIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(tau());
return result;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The AcrobotInput vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/README.md | Drake Acrobot Examples
======================
The files in this directory implement a basic Drake acrobot (a double pendulum
actuated at its "waist") and use the acrobot to demonstrate various drake
control features.
The most common task for the acrobot is to achieve and hold an upright (both
links pointing in the positive-Z direction) position.
For more information on the acrobot, see
[Chapter 3 of Underactuated Robotics](http://underactuated.mit.edu/underactuated.html?chapter=3)
Basic Acrobot Simulation and Control
------------------------------------
The basic acrobot and its simple controllers are implemented in the files:
* `acrobot_geometry.{cc,h}`
* `acrobot_plant.{cc,h}`
* `acrobot_{params,input,state}.{cc,h}`
* `spong_controller.{cc,h}`
The acrobot plant is implemented analytically rather than from a URDF or
SDFormat file.
### `//examples/acrobot:`*run_passive*
This program runs a passive acrobot with no applied torque. It publishes
visualization data over LCM, so if you run `meldis` it will appear there.
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_passive
```
You can leave the same meldis display open for all of the demos below;
you don't need to close and re-open it each time.
### `//examples/acrobot:`*run_lqr*
Like *run_passive*, this runs an acrobot and publishes its visualization
information to LCM. Unlike *run_passive*
* It starts very near the upright position.
* It attaches an LQR controller that can maintain the upright position.
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_lqr
```
### `//examples/acrobot:`*run_swing_up*
Like the above, but this attaches a Spong controller to the acrobot. The
Spong controller is a hybrid controller that performs energy shaping to swing
the acrobot up to near its vertical posture, then switches to the LQR
controller to hold it there.
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_swing_up
```
Benchmarks and Demonstrations
-----------------------------
### `//examples/acrobot:`*run_lqr_w_estimator*
This is a demonstration of how to use a Kalman filter observer so that the
controller does not need direct access to the robot's state. The acrobot's
output is limited to joint positions, and an observer system with a model of
the acrobot estimates the model state from the observed positions. The
controller then relies only on the estimated positions.
The observer trajectory does not show up in the visualizer, but can be
visualized via the `call_python_client_cli` remote python interpreter.
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_lqr_w_estimator
bazel run //common/proto:call_python_client_cli
```
### `//examples/acrobot:`*run_swing_up_traj_optimization*
This demonstrates the use of trajectory optimization with acrobot: An
optimized trajectory is computed using direct collocation to limit the
required power on the joint; the acrobot is then controlled with LQR to the
trajectory.
This demonstration requires SNOPT and will not run without it.
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_swing_up_traj_optimization
```
LCM-based Control Stack
-----------------------
Real-world robotic systems in Drake are often implemented with the controller
in one process and the model in a separate process, so that the same
controller process can be run against a real robot. An example of this
pattern is provided here.
### `//examples/acrobot:`*spong_controller_w_lcm*
This runs the same control as `run_swing_up` above, but instead of connecting
to a plant it is attached to an LCM publisher
### `//examples/acrobot:`*run_plant_w_lcm*
This implements the same plant as the acrobots above, but its control is
attached to an LCM receiver.
### Putting them together
```
bazel run //tools:meldis -- --open-window &
bazel run //examples/acrobot:run_plant_w_lcm &
bazel run //examples/acrobot:spong_controller_w_lcm
```
Monte Carlo Parameter Search
----------------------------
A demonstration of how to use Drake stochastic schemas to do deterministic
Monte Carlo testing.
### The scenario and output schemas
A schema is a structure that uses the mechanisms in `drake::schema` to define
a yaml language.
In C++, the scenario and output schemas are defined in the `Scenario` and
`Output` structs of [spong_sim.cc](spong_sim.cc).
A simulation runner is a binary that takes a scenario and a random seed in and
outputs the scenario that was actually run (i.e. with no stochastic elements)
and the simulation output.
`//examples/acrobot:`*spong_sim_main_cc* works this way:
```
bazel build //examples/acrobot:spong_sim_main_cc
./bazel-bin/examples/acrobot/spong_sim_main_cc --scenario examples/acrobot/test/example_stochastic_scenario.yaml --output out.yaml --random_seed 12
cat out.yaml
```
### Python
`//examples/acrobot:`*spong_sim_main_py* demonstrates a similar system in
python. However stochastic schemas are not yet supported in python so this is
only useful as a demonstration of the python schema mechanism.
### `//examples/acrobot:`*optimizer_demo*
A demonstration of running parameter search optimization. This loads a
scenario file and optimizes scenario parameters. For instance, given the
scenario from `test/example_stochastic_scenario.yaml`:
```
example:
controller_params: !UniformVector
min: [4, 40, 4, 0.9e3]
max: [6, 60, 6, 1.1e3]
initial_state: !UniformVector
min: [1.1, -0.1, -0.1, -0.1]
max: [1.3, 0.1, 0.1, 0.1]
t_final: 30.0
tape_period: 0.05
```
running the command
```
bazel run //examples/acrobot:optimizer_demo -- --ensemble_size=30 --num_evaluations=300
```
will produce a (much!) better set of `controller_params` in a few minutes.
### Practical considerations
This demo is just a proof of concept, not a sensible way to solve serious
parameter optimization problems. Optimizing a more computationally expensive
problem would want a more sophisticated optimizer (the Nevergrad collection
works well), parallel or cloud-based evaluation of the metric, and more
careful curation of the ensemble. In addition you would want separate
training and test sets.
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/run_swing_up.cc | #include <memory>
#include <gflags/gflags.h>
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/examples/acrobot/spong_controller.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/math/wrap_to.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
// Simple example which simulates the Acrobot, started near its stable fixed
// point, with a Spong swing-up controller designed to reach the unstable
// fixed point. Run meldis to see the animated result.
DEFINE_double(simulation_sec, 10.0,
"Number of seconds to simulate.");
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
int do_main() {
systems::DiagramBuilder<double> builder;
auto acrobot = builder.AddSystem<AcrobotPlant>();
acrobot->set_name("acrobot");
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(
&builder, acrobot->get_output_port(0), scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
auto controller = builder.AddSystem<AcrobotSpongController>();
builder.Connect(acrobot->get_output_port(0), controller->get_input_port(0));
builder.Connect(controller->get_output_port(0), acrobot->get_input_port(0));
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
systems::Context<double>& acrobot_context =
diagram->GetMutableSubsystemContext(*acrobot,
&simulator.get_mutable_context());
// Sets an initial condition near the upright fixed point.
AcrobotState<double>* state = dynamic_cast<AcrobotState<double>*>(
&acrobot_context.get_mutable_continuous_state_vector());
DRAKE_DEMAND(state != nullptr);
state->set_theta1(0.1);
state->set_theta2(-0.1);
state->set_theta1dot(0.0);
state->set_theta2dot(0.02);
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.AdvanceTo(FLAGS_simulation_sec);
DRAKE_DEMAND(std::abs(math::wrap_to(state->theta1(), 0., 2. * M_PI) - M_PI) <
1e-2);
DRAKE_DEMAND(std::abs(math::wrap_to(state->theta2(), -M_PI, M_PI)) < 1e-2);
DRAKE_DEMAND(std::abs(state->theta1dot()) < 0.1);
DRAKE_DEMAND(std::abs(state->theta2dot()) < 0.1);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::do_main();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_controller_w_lcm.cc | /*
* An acrobot Spong controller that communicates to run_plant_w_lcm
* through LCM, implemented by the following diagram system:
*
* LcmSubscriberSystem—>
* AcrobotStateReceiver—>
* AcrobotSpongController—>
* AcrobotCommandSender—>
* LcmPublisherSystem
*
*/
#include <chrono>
#include <memory>
#include <gflags/gflags.h>
#include "drake/examples/acrobot/acrobot_lcm.h"
#include "drake/examples/acrobot/spong_controller.h"
#include "drake/lcmt_acrobot_u.hpp"
#include "drake/lcmt_acrobot_x.hpp"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/lcm/lcm_interface_system.h"
#include "drake/systems/lcm/lcm_publisher_system.h"
#include "drake/systems/lcm/lcm_subscriber_system.h"
DEFINE_double(time_limit_sec, std::numeric_limits<double>::infinity(),
"Number of seconds to run (default: infinity).");
namespace drake {
namespace examples {
namespace acrobot {
namespace {
int DoMain() {
drake::systems::DiagramBuilder<double> builder;
const std::string channel_x = "acrobot_xhat";
const std::string channel_u = "acrobot_u";
// -----------------controller--------------------------------------
// Create state receiver.
auto lcm = builder.AddSystem<systems::lcm::LcmInterfaceSystem>();
auto state_sub = builder.AddSystem(
systems::lcm::LcmSubscriberSystem::Make<lcmt_acrobot_x>(channel_x, lcm));
auto state_receiver = builder.AddSystem<AcrobotStateReceiver>();
builder.Connect(state_sub->get_output_port(),
state_receiver->get_input_port(0));
// Create command sender.
auto command_pub = builder.AddSystem(
systems::lcm::LcmPublisherSystem::Make<lcmt_acrobot_u>(
channel_u, lcm, 0.001));
auto command_sender = builder.AddSystem<AcrobotCommandSender>();
builder.Connect(command_sender->get_output_port(0),
command_pub->get_input_port());
auto controller = builder.AddSystem<AcrobotSpongController>();
builder.Connect(controller->get_output_port(0),
command_sender->get_input_port(0));
builder.Connect(state_receiver->get_output_port(0),
controller->get_input_port(0));
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
simulator.set_target_realtime_rate(1.0);
simulator.Initialize();
simulator.AdvanceTo(FLAGS_time_limit_sec);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::DoMain();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_io.py | import numpy as np
from pydrake.common.yaml import yaml_load, yaml_dump
def load_scenario(*, filename=None, data=None):
"""Given a scenario `filename` xor `data` loads and
returns the acrobot scenario from the file.
"""
return yaml_load(filename=filename, data=data)
def save_scenario(*, scenario):
"""Given a scenario, returns a yaml-formatted str for it.
"""
# For a known list of scenario-specific items, convert numpy arrays into
# lists for serialization purposes.
scrubbed = dict(scenario)
for key in ["controller_params", "initial_state"]:
if isinstance(scenario[key], dict):
for subkey in ["min", "max"]:
scrubbed[key][subkey] = [
float(x) for x in scenario[key][subkey]
]
else:
scrubbed[key] = [float(x) for x in scenario[key]]
return yaml_dump(scrubbed)
def load_output(*, filename=None, data=None):
"""Given an acrobot output `filename` xor `data`, loads and returns the
np.ndarray.
"""
x_tape_data = yaml_load(filename=filename, data=data)
if not x_tape_data:
raise RuntimeError("Could not load acrobot output")
if "x_tape" not in x_tape_data:
raise RuntimeError(f"Did not find 'x_tape' in {x_tape_data}")
return np.array(x_tape_data["x_tape"])
def save_output(*, x_tape):
"""Given an acrobot output `x_tape`, returns a yaml-formatter str for it.
"""
return yaml_dump({"x_tape": x_tape.tolist()})
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_controller.h | #pragma once
#include <memory>
#include "drake/examples/acrobot/acrobot_input.h"
#include "drake/examples/acrobot/acrobot_params.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/examples/acrobot/spong_controller_params.h"
#include "drake/math/wrap_to.h"
#include "drake/systems/controllers/linear_quadratic_regulator.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/primitives/linear_system.h"
namespace drake {
namespace examples {
namespace acrobot {
/// The Spong acrobot swing-up controller as described in:
/// Spong, Mark W. "Swing up control of the acrobot." Robotics and Automation,
/// 1994. Proceedings., 1994 IEEE International Conference on. IEEE, 1994.
///
/// Note that the Spong controller works well on the default set of parameters,
/// which Spong used in his paper. In contrast, it is difficult to find a
/// functional set of gains to stabilize the robot about its upright fixed
/// point using the parameters of the physical robot we have in lab.
///
/// @system
/// name: AcrobotSpongController
/// input_ports:
/// - acrobot_state
/// output_ports:
/// - elbow_torque
/// @endsystem
///
/// @ingroup acrobot_systems
template <typename T>
class AcrobotSpongController : public systems::LeafSystem<T> {
public:
AcrobotSpongController()
: acrobot_{}, acrobot_context_(acrobot_.CreateDefaultContext()) {
this->DeclareVectorInputPort("acrobot_state", AcrobotState<T>());
this->DeclareVectorOutputPort("elbow_torque", AcrobotInput<T>(),
&AcrobotSpongController::CalcControlTorque);
this->DeclareNumericParameter(SpongControllerParams<T>());
// Set nominal state to the upright fixed point.
AcrobotState<T>& state =
AcrobotPlant<T>::get_mutable_state(acrobot_context_.get());
state.set_theta1(M_PI);
state.set_theta2(0.0);
state.set_theta1dot(0.0);
state.set_theta2dot(0.0);
const AcrobotPlant<double> acrobot_double{};
// Setup context for linearization.
std::unique_ptr<drake::systems::Context<double>> acrobot_context_double =
acrobot_double.CreateDefaultContext();
acrobot_context_double->SetTimeStateAndParametersFrom(*acrobot_context_);
acrobot_double.GetInputPort("elbow_torque")
.FixValue(acrobot_context_double.get(), 0.0);
auto linear_system = Linearize(acrobot_double, *acrobot_context_double);
Eigen::Matrix4d Q = Eigen::Matrix4d::Identity();
Q(0, 0) = 10;
Q(1, 1) = 10;
Vector1d R(1);
systems::controllers::LinearQuadraticRegulatorResult lqr_result =
systems::controllers::LinearQuadraticRegulator(
linear_system->A(), linear_system->B(), Q, R);
S_ = lqr_result.S;
K_ = lqr_result.K;
}
const SpongControllerParams<T>& get_parameters(
const systems::Context<T>& context) const {
return this->template GetNumericParameter<SpongControllerParams>(context,
0);
}
SpongControllerParams<T>& get_mutable_parameters(
systems::Context<T>* context) {
return this->template GetMutableNumericParameter<SpongControllerParams>(
context, 0);
}
void CalcControlTorque(const systems::Context<T>& context,
AcrobotInput<T>* output) const {
acrobot_context_->get_mutable_continuous_state_vector().SetFromVector(
this->EvalVectorInput(context, 0)->CopyToVector());
const AcrobotState<T>& state =
AcrobotPlant<T>::get_state(*acrobot_context_);
const AcrobotParams<T>& p = acrobot_.get_parameters(*acrobot_context_);
const Vector4<T> x0(M_PI, 0, 0, 0);
Vector4<T> x = state.CopyToVector();
// Wrap theta1 and theta2.
x(0) = math::wrap_to(x(0), 0., 2. * M_PI);
x(1) = math::wrap_to(x(1), -M_PI, M_PI);
const T cost = (x - x0).dot(S_ * (x - x0));
T u;
if (cost < get_parameters(context).balancing_threshold()) {
/*
* Balancing control law
* When the robot is close enough to the upright fixed point, i.e.
* (x-x0)'*S*(x-x0) < some_threshold, an LQR controller linearized about
* the upright fixed point takes over and balances the robot about its
* upright position.
*/
const Vector1<T> u_v = K_ * (x0 - x);
u = u_v(0);
} else {
/*
* Swing-up control law: u = u_p + u_e;
* u_e is the energy shaping controller.
* u_e = k_e * (E_desired - E) * q̇₂, so that
* Edot = k_e * (E_desired - E) * q̇₂^2.
*
* u_p is the partial feedback linearization controller which stabilizes
* q₂.
* We want
* q̈ = y = - k_p * q₂ - k_d * q̇₂
* Given Acrobot's manipulator equation:
* q̈ =M⁻¹ * (Bu - bias),
* where
* M⁻¹ = [a1,a2; a2,a3],
* B = [0;1],
* bias=[C0;C1]
* we have:
* q̈₂ = -C0*a2 + a3*(u-C1)
* Equating the above equation to y gives
* u_p = (a2 * C0 + y) / a3 + C1.
*
* See http://underactuated.mit.edu/underactuated.html?chapter=acrobot
*/
const Matrix2<T> M = acrobot_.MassMatrix(*acrobot_context_);
const Vector2<T> bias = acrobot_.DynamicsBiasTerm(*acrobot_context_);
const Matrix2<T> M_inverse = M.inverse();
// controller gains
const T& k_e = get_parameters(context).k_e();
const T& k_p = get_parameters(context).k_p();
const T& k_d = get_parameters(context).k_d();
const T PE = acrobot_.EvalPotentialEnergy(*acrobot_context_);
const T KE = acrobot_.EvalKineticEnergy(*acrobot_context_);
const T E = PE + KE;
const T E_desired =
(p.m1() * p.lc1() + p.m2() * (p.l1() + p.lc2())) * p.gravity();
const T E_tilde = E - E_desired;
const T u_e = -k_e * E_tilde * state.theta2dot();
const T y = -k_p * state.theta2() - k_d * state.theta2dot();
T a3 = M_inverse(1, 1), a2 = M_inverse(0, 1);
T u_p = (a2 * bias(0) + y) / a3 + bias(1);
u = u_e + u_p;
}
// Saturation.
const T ku_upper_bound = 20;
const T ku_lower_bound = -20;
if (u >= ku_upper_bound) u = ku_upper_bound;
if (u <= ku_lower_bound) u = ku_lower_bound;
output->set_tau(u);
}
private:
AcrobotPlant<T> acrobot_;
// The implementation above is (and must remain) careful to not store hidden
// state in here. This is only used to avoid runtime allocations.
const std::unique_ptr<systems::Context<T>> acrobot_context_;
Eigen::Matrix4d S_;
Eigen::RowVector4d K_;
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/Acrobot.sdf | <?xml version="1.0"?>
<sdf version="1.7">
<model name="Acrobot">
<link name="base_link">
<collision name="base_link_collision">
<geometry>
<box>
<size>0.2 0.2 0.2</size>
</box>
</geometry>
</collision>
<visual name="base_link_visual">
<geometry>
<box>
<size>0.2 0.2 0.2</size>
</box>
</geometry>
</visual>
</link>
<joint name="base_weld" type="fixed">
<child>base_link</child>
<parent>world</parent>
</joint>
<link name="upper_link">
<pose>0 0.15 0 0 0 0</pose>
<inertial>
<pose>0 0 -0.5 0 0 0</pose>
<mass>1</mass>
<inertia>
<ixx>1</ixx>
<ixy>0</ixy>
<ixz>0</ixz>
<iyy>0.083</iyy>
<iyz>0</iyz>
<izz>1</izz>
</inertia>
</inertial>
<collision name="upper_link_collision">
<pose>0 0 -0.5 0 0 0</pose>
<geometry>
<cylinder>
<length>1.1</length>
<radius>0.05</radius>
</cylinder>
</geometry>
</collision>
<visual name="upper_link_visual">
<pose>0 0 -0.5 0 0 0</pose>
<geometry>
<cylinder>
<length>1.1</length>
<radius>0.05</radius>
</cylinder>
</geometry>
</visual>
</link>
<joint name="shoulder" type="revolute">
<child>upper_link</child>
<parent>base_link</parent>
<axis>
<xyz expressed_in="__model__">0 1 0</xyz>
<limit>
<effort>0</effort>
</limit>
<dynamics>
<damping>0.1</damping>
<friction>0</friction>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
</dynamics>
</axis>
</joint>
<link name="lower_link">
<pose>0 0.25 -1 0 0 0</pose>
<inertial>
<pose>0 0 -1 0 0 0</pose>
<mass>1</mass>
<inertia>
<ixx>1</ixx>
<ixy>0</ixy>
<ixz>0</ixz>
<iyy>0.33</iyy>
<iyz>0</iyz>
<izz>1</izz>
</inertia>
</inertial>
<collision name="lower_link_collision">
<pose>0 0 -1 0 0 0</pose>
<geometry>
<cylinder>
<length>2.1</length>
<radius>0.05</radius>
</cylinder>
</geometry>
</collision>
<visual name="lower_link_visual">
<pose>0 0 -1 0 0 0</pose>
<geometry>
<cylinder>
<length>2.1</length>
<radius>0.05</radius>
</cylinder>
</geometry>
</visual>
</link>
<joint name="elbow" type="revolute">
<child>lower_link</child>
<parent>upper_link</parent>
<axis>
<xyz expressed_in="__model__">0 1 0</xyz>
<limit>
</limit>
<dynamics>
<damping>0.1</damping>
<friction>0</friction>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
</dynamics>
</axis>
</joint>
<frame name="hand">
<pose relative_to="lower_link">0 0 -2.1 0 0 0</pose>
</frame>
</model>
</sdf>
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_controller_params.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Describes the row indices of a SpongControllerParams.
struct SpongControllerParamsIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 4;
// The index of each individual coordinate.
static const int kKE = 0;
static const int kKP = 1;
static const int kKD = 2;
static const int kBalancingThreshold = 3;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `SpongControllerParamsIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class SpongControllerParams final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef SpongControllerParamsIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c k_e defaults to 5.0 s.
/// @arg @c k_p defaults to 50.0 s^-2.
/// @arg @c k_d defaults to 5.0 s^-1.
/// @arg @c balancing_threshold defaults to 1e3 None.
SpongControllerParams() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_k_e(5.0);
this->set_k_p(50.0);
this->set_k_d(5.0);
this->set_balancing_threshold(1e3);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
SpongControllerParams(const SpongControllerParams& other)
: drake::systems::BasicVector<T>(other.values()) {}
SpongControllerParams(SpongControllerParams&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
SpongControllerParams& operator=(const SpongControllerParams& other) {
this->values() = other.values();
return *this;
}
SpongControllerParams& operator=(SpongControllerParams&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_k_e(symbolic::Variable("k_e"));
this->set_k_p(symbolic::Variable("k_p"));
this->set_k_d(symbolic::Variable("k_d"));
this->set_balancing_threshold(symbolic::Variable("balancing_threshold"));
}
[[nodiscard]] SpongControllerParams<T>* DoClone() const final {
return new SpongControllerParams;
}
/// @name Getters and Setters
//@{
/// Energy shaping gain.
/// @note @c k_e is expressed in units of s.
/// @note @c k_e has a limited domain of [0.0, +Inf].
const T& k_e() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kKE);
}
/// Setter that matches k_e().
void set_k_e(const T& k_e) {
ThrowIfEmpty();
this->SetAtIndex(K::kKE, k_e);
}
/// Fluent setter that matches k_e().
/// Returns a copy of `this` with k_e set to a new value.
[[nodiscard]] SpongControllerParams<T> with_k_e(const T& k_e) const {
SpongControllerParams<T> result(*this);
result.set_k_e(k_e);
return result;
}
/// Partial feedback linearization proportional gain.
/// @note @c k_p is expressed in units of s^-2.
/// @note @c k_p has a limited domain of [0.0, +Inf].
const T& k_p() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kKP);
}
/// Setter that matches k_p().
void set_k_p(const T& k_p) {
ThrowIfEmpty();
this->SetAtIndex(K::kKP, k_p);
}
/// Fluent setter that matches k_p().
/// Returns a copy of `this` with k_p set to a new value.
[[nodiscard]] SpongControllerParams<T> with_k_p(const T& k_p) const {
SpongControllerParams<T> result(*this);
result.set_k_p(k_p);
return result;
}
/// Partial feedback linearization derivative gain.
/// @note @c k_d is expressed in units of s^-1.
/// @note @c k_d has a limited domain of [0.0, +Inf].
const T& k_d() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kKD);
}
/// Setter that matches k_d().
void set_k_d(const T& k_d) {
ThrowIfEmpty();
this->SetAtIndex(K::kKD, k_d);
}
/// Fluent setter that matches k_d().
/// Returns a copy of `this` with k_d set to a new value.
[[nodiscard]] SpongControllerParams<T> with_k_d(const T& k_d) const {
SpongControllerParams<T> result(*this);
result.set_k_d(k_d);
return result;
}
/// Cost value at which to switch from swing up to balancing.
/// @note @c balancing_threshold is expressed in units of None.
/// @note @c balancing_threshold has a limited domain of [0.0, +Inf].
const T& balancing_threshold() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kBalancingThreshold);
}
/// Setter that matches balancing_threshold().
void set_balancing_threshold(const T& balancing_threshold) {
ThrowIfEmpty();
this->SetAtIndex(K::kBalancingThreshold, balancing_threshold);
}
/// Fluent setter that matches balancing_threshold().
/// Returns a copy of `this` with balancing_threshold set to a new value.
[[nodiscard]] SpongControllerParams<T> with_balancing_threshold(
const T& balancing_threshold) const {
SpongControllerParams<T> result(*this);
result.set_balancing_threshold(balancing_threshold);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& k_e_ref = this->GetAtIndex(K::kKE);
a->Visit(drake::MakeNameValue("k_e", &k_e_ref));
T& k_p_ref = this->GetAtIndex(K::kKP);
a->Visit(drake::MakeNameValue("k_p", &k_p_ref));
T& k_d_ref = this->GetAtIndex(K::kKD);
a->Visit(drake::MakeNameValue("k_d", &k_d_ref));
T& balancing_threshold_ref = this->GetAtIndex(K::kBalancingThreshold);
a->Visit(
drake::MakeNameValue("balancing_threshold", &balancing_threshold_ref));
}
/// See SpongControllerParamsIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return SpongControllerParamsIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(k_e());
result = result && (k_e() >= T(0.0));
result = result && !isnan(k_p());
result = result && (k_p() >= T(0.0));
result = result && !isnan(k_d());
result = result && (k_d() >= T(0.0));
result = result && !isnan(balancing_threshold());
result = result && (balancing_threshold() >= T(0.0));
return result;
}
void GetElementBounds(Eigen::VectorXd* lower,
Eigen::VectorXd* upper) const final {
const double kInf = std::numeric_limits<double>::infinity();
*lower = Eigen::Matrix<double, 4, 1>::Constant(-kInf);
*upper = Eigen::Matrix<double, 4, 1>::Constant(kInf);
(*lower)(K::kKE) = 0.0;
(*lower)(K::kKP) = 0.0;
(*lower)(K::kKD) = 0.0;
(*lower)(K::kBalancingThreshold) = 0.0;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The SpongControllerParams vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_lcm.h | #pragma once
/// @file
/// This file contains classes dealing with sending/receiving LCM messages
/// related to acrobot. The classes in this file are based on iiwa_lcm.h
#include <memory>
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/lcmt_acrobot_u.hpp"
#include "drake/lcmt_acrobot_x.hpp"
#include "drake/systems/framework/basic_vector.h"
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Receives the output of an LcmSubsriberSystem that subsribes to the
/// acrobot state channel with LCM type lcmt_acrobot_x, and outputs the
/// acrobot states as an AcrobotState.
///
/// @system
/// name: AcrobotStateReceiver
/// input_ports:
/// - lcmt_acrobot_x
/// output_ports:
/// - acrobot_state
/// @endsystem
///
/// @ingroup acrobot_systems
class AcrobotStateReceiver : public systems::LeafSystem<double> {
public:
AcrobotStateReceiver();
private:
void CopyStateOut(const systems::Context<double>& context,
AcrobotState<double>* output) const;
};
/// Receives the output of an acrobot controller, and outputs it as an LCM
/// message with type lcm_acrobot_u. Its output port is usually connected to
/// an LcmPublisherSystem to publish the messages it generates.
///
/// @system
/// name : AcrobotCommandSender
/// input_ports:
/// - elbow_torque
/// output_ports:
/// - lcm_acrobot_u
/// @endsystem
///
/// @ingroup acrobot_systems
class AcrobotCommandSender : public systems::LeafSystem<double> {
public:
AcrobotCommandSender();
private:
void OutputCommand(const systems::Context<double>& context,
lcmt_acrobot_u* output) const;
};
/// Receives the output of an LcmSubscriberSystem that subscribes to the
/// acrobot input channel with LCM type lcmt_acrobot_u, and outputs the
/// acrobot input as a BasicVector.
///
/// @system
/// name: AcrobotCommandReceiver
/// input_ports:
/// - lcmt_acrobot_u
/// output_ports:
/// - elbow_torque
/// @endsystem
///
/// @ingroup acrobot_systems
class AcrobotCommandReceiver : public systems::LeafSystem<double> {
public:
AcrobotCommandReceiver();
private:
void OutputCommandAsVector(const systems::Context<double>& context,
systems::BasicVector<double>* output) const;
};
/// Receives the output of an acrobot_plant, and outputs it as an LCM
/// message with type lcm_acrobot_x. Its output port is usually connected to
/// an LcmPublisherSystem to publish the messages it generates.
///
/// @system
/// name: AcrobotStateSender
/// input_ports:
/// - acrobot_state
/// output_ports:
/// - lcmt_acrobot_x
/// @endsystem
///
/// @ingroup acrobot_systems
class AcrobotStateSender : public systems::LeafSystem<double> {
public:
AcrobotStateSender();
private:
void OutputState(const systems::Context<double>& context,
lcmt_acrobot_x* output) const;
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_sim.cc | #include <fstream>
#include <iostream>
#include <gflags/gflags.h>
#include "drake/common/name_value.h"
#include "drake/common/schema/stochastic.h"
#include "drake/common/yaml/yaml_io.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/spong_controller.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/primitives/vector_log_sink.h"
using drake::examples::acrobot::AcrobotParams;
using drake::examples::acrobot::AcrobotPlant;
using drake::examples::acrobot::AcrobotSpongController;
using drake::systems::DiagramBuilder;
using drake::systems::Simulator;
using drake::yaml::SaveYamlFile;
using drake::yaml::LoadYamlFile;
namespace drake {
namespace examples {
namespace acrobot {
namespace {
DEFINE_string(scenario, "", "Scenario file to load (required).");
DEFINE_string(dump_scenario, "", "Scenario file to save.");
DEFINE_string(output, "", "Output file to save (required).");
DEFINE_int32(random_seed, drake::RandomGenerator::default_seed,
"Random seed");
// The YAML format for --scenario.
struct Scenario {
// TODO(jeremy.nimmer) Maybe use AcrobotParams class for this one?
drake::schema::DistributionVectorVariant<4> controller_params =
Eigen::Vector4d::Constant(NAN);
drake::schema::DistributionVectorVariant<4> initial_state =
Eigen::Vector4d::Constant(NAN);
double t_final = NAN;
double tape_period = NAN;
template <typename Archive>
void Serialize(Archive* a) {
a->Visit(DRAKE_NVP(controller_params));
a->Visit(DRAKE_NVP(initial_state));
a->Visit(DRAKE_NVP(t_final));
a->Visit(DRAKE_NVP(tape_period));
}
};
// The YAML format for --output.
struct Output {
Eigen::MatrixXd x_tape;
template <typename Archive>
void Serialize(Archive* a) {
a->Visit(DRAKE_NVP(x_tape));
}
};
// If the Scenario `input` has any randomness, sample it; return a Scenario
// with no randomness (ie, IsDeterministic() is guaranteed true on all
// elements).
Scenario SampleScenario(const Scenario& input) {
drake::RandomGenerator random(FLAGS_random_seed);
Scenario result = input;
result.controller_params =
drake::schema::ToDistributionVector(input.controller_params)->
Sample(&random);
result.initial_state =
drake::schema::ToDistributionVector(input.initial_state)->
Sample(&random);
return result;
}
// Simulates an Acrobot + Spong controller from the given initial state and
// parameters until the given final time. Returns the state as output.
Output Simulate(const Scenario& stochastic_scenario) {
// Resolve scenario randomness and write out the resolved scenario.
const Scenario scenario = SampleScenario(stochastic_scenario);
if (!FLAGS_dump_scenario.empty()) {
SaveYamlFile(FLAGS_dump_scenario, scenario);
}
DiagramBuilder<double> builder;
auto plant = builder.AddSystem<AcrobotPlant>();
auto controller = builder.AddSystem<AcrobotSpongController>();
builder.Connect(plant->get_output_port(0), controller->get_input_port(0));
builder.Connect(controller->get_output_port(0), plant->get_input_port(0));
auto state_logger = LogVectorOutput(plant->get_output_port(0), &builder,
scenario.tape_period);
auto diagram = builder.Build();
Simulator<double> simulator(*diagram);
auto& context = simulator.get_mutable_context();
auto& plant_context = diagram->GetMutableSubsystemContext(
*plant, &context);
DRAKE_DEMAND(drake::schema::IsDeterministic(scenario.initial_state));
plant_context.SetContinuousState(
drake::schema::GetDeterministicValue(scenario.initial_state));
auto& controller_context = diagram->GetMutableSubsystemContext(
*controller, &context);
DRAKE_DEMAND(drake::schema::IsDeterministic(
scenario.controller_params));
controller_context.get_mutable_numeric_parameter(0).SetFromVector(
drake::schema::GetDeterministicValue(scenario.controller_params));
simulator.AdvanceTo(scenario.t_final);
// Create an output string that looks like this:
// x_tape: [[0,1,2,3,4,5],[0,1,2,3,4,5],[0,1,2,3,4,5],[0,1,2,3,4,5]]
Output output;
output.x_tape = state_logger->FindLog(context).data();
return output;
}
int Main() {
if (FLAGS_scenario.empty()) {
std::cerr << "A --scenario file is required.\n";
return 1;
}
if (FLAGS_output.empty()) {
std::cerr << "An --output file is required.\n";
return 1;
}
const Scenario stochastic_scenario = LoadYamlFile<Scenario>(FLAGS_scenario);
const Output output = Simulate(stochastic_scenario);
SaveYamlFile(FLAGS_output, output);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::SetUsageMessage(
"A main() program simulates a spong-controlled acrobot.");
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::Main();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_sim.py | """A main() program (plus an reusable standalone function) that simulates a
spong-controlled acrobot.
"""
import argparse
import sys
from pydrake.examples import (
AcrobotPlant,
AcrobotSpongController,
AcrobotState,
)
from pydrake.systems.analysis import Simulator
from pydrake.systems.framework import DiagramBuilder
from pydrake.systems.primitives import LogVectorOutput
from examples.acrobot.acrobot_io import load_scenario, save_output
def simulate(*, initial_state, controller_params, t_final, tape_period):
"""Simulates an Acrobot + Spong controller from the given initial state and
parameters until the given final time. Returns the state sampled at the
given tape_period.
"""
builder = DiagramBuilder()
plant = builder.AddSystem(AcrobotPlant())
controller = builder.AddSystem(AcrobotSpongController())
builder.Connect(plant.get_output_port(0), controller.get_input_port(0))
builder.Connect(controller.get_output_port(0), plant.get_input_port(0))
state_logger = LogVectorOutput(plant.get_output_port(0), builder,
tape_period)
diagram = builder.Build()
simulator = Simulator(diagram)
context = simulator.get_mutable_context()
plant_context = diagram.GetMutableSubsystemContext(plant, context)
controller_context = diagram.GetMutableSubsystemContext(
controller, context)
plant_context.SetContinuousState(initial_state)
controller_context.get_mutable_numeric_parameter(0).SetFromVector(
controller_params)
simulator.AdvanceTo(t_final)
x_tape = state_logger.FindLog(context).data()
return x_tape
def main():
parser = argparse.ArgumentParser(description=__doc__)
parser.add_argument(
"--scenario", metavar="*.yaml", required=True,
help="Scenario file to load (required).")
parser.add_argument(
"--output", metavar="*.yaml", required=True,
help="Output file to save (required).")
args = parser.parse_args()
scenario = load_scenario(filename=args.scenario)
x_tape = simulate(**scenario)
text = save_output(x_tape=x_tape)
with open(args.output, 'w') as handle:
handle.write(text)
return 0
if __name__ == "__main__":
sys.exit(main())
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/run_passive.cc | #include <memory>
#include <gflags/gflags.h>
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
// Simple example which simulates the (passive) Acrobot. Run meldis to see
// the animated result.
DEFINE_double(simulation_sec, 10.0,
"Number of seconds to simulate.");
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
int do_main() {
systems::DiagramBuilder<double> builder;
auto acrobot = builder.AddSystem<AcrobotPlant>();
acrobot->set_name("acrobot");
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(
&builder, acrobot->get_output_port(0), scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
systems::Context<double>& acrobot_context =
diagram->GetMutableSubsystemContext(*acrobot,
&simulator.get_mutable_context());
const double tau = 0;
acrobot->GetInputPort("elbow_torque").FixValue(&acrobot_context, tau);
// Set an initial condition that is sufficiently far from the downright fixed
// point.
AcrobotState<double>* x0 = dynamic_cast<AcrobotState<double>*>(
&acrobot_context.get_mutable_continuous_state_vector());
DRAKE_DEMAND(x0 != nullptr);
x0->set_theta1(1.0);
x0->set_theta2(1.0);
x0->set_theta1dot(0.0);
x0->set_theta2dot(0.0);
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.Initialize();
simulator.AdvanceTo(FLAGS_simulation_sec);
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::do_main();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_geometry.cc | #include "drake/examples/acrobot/acrobot_geometry.h"
#include <memory>
#include "drake/examples/acrobot/acrobot_params.h"
#include "drake/geometry/geometry_frame.h"
#include "drake/geometry/geometry_ids.h"
#include "drake/geometry/geometry_instance.h"
#include "drake/geometry/geometry_roles.h"
#include "drake/math/rigid_transform.h"
#include "drake/math/rotation_matrix.h"
namespace drake {
namespace examples {
namespace acrobot {
using Eigen::Vector3d;
using Eigen::Vector4d;
using geometry::Box;
using geometry::Cylinder;
using geometry::GeometryFrame;
using geometry::GeometryId;
using geometry::GeometryInstance;
using geometry::MakePhongIllustrationProperties;
using geometry::Sphere;
using std::make_unique;
const AcrobotGeometry* AcrobotGeometry::AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& acrobot_state_port,
const AcrobotParams<double>& acrobot_params,
geometry::SceneGraph<double>* scene_graph) {
DRAKE_THROW_UNLESS(builder != nullptr);
DRAKE_THROW_UNLESS(scene_graph != nullptr);
auto acrobot_geometry = builder->AddSystem(std::unique_ptr<AcrobotGeometry>(
new AcrobotGeometry(acrobot_params, scene_graph)));
builder->Connect(acrobot_state_port, acrobot_geometry->get_input_port(0));
builder->Connect(
acrobot_geometry->get_output_port(0),
scene_graph->get_source_pose_port(acrobot_geometry->source_id_));
return acrobot_geometry;
}
AcrobotGeometry::AcrobotGeometry(const AcrobotParams<double>& params,
geometry::SceneGraph<double>* scene_graph)
: l1_(params.l1()) {
DRAKE_THROW_UNLESS(scene_graph != nullptr);
source_id_ = scene_graph->RegisterSource();
// Note: using AcrobotState as the port type would have complicated the
// trajectory playback workflow used in run_swing_up_traj_optimization.cc.
this->DeclareVectorInputPort("state", 4);
this->DeclareAbstractOutputPort("geometry_pose",
&AcrobotGeometry::OutputGeometryPose);
// The base.
GeometryId id = scene_graph->RegisterAnchoredGeometry(
source_id_,
make_unique<GeometryInstance>(math::RigidTransformd::Identity(),
make_unique<Box>(.2, 0.2, 0.2), "base"));
scene_graph->AssignRole(
source_id_, id, MakePhongIllustrationProperties(Vector4d(0, 1, 0, 1)));
// The upper link.
upper_link_frame_id_ =
scene_graph->RegisterFrame(source_id_, GeometryFrame("upper_link"));
id = scene_graph->RegisterGeometry(
source_id_, upper_link_frame_id_,
make_unique<GeometryInstance>(
math::RigidTransformd(Vector3d(0., 0.15, -params.l1() / 2.)),
make_unique<Cylinder>(0.05, params.l1()), "upper_link"));
scene_graph->AssignRole(
source_id_, id, MakePhongIllustrationProperties(Vector4d(1, 0, 0, 1)));
// The lower link.
lower_link_frame_id_ =
scene_graph->RegisterFrame(source_id_, GeometryFrame("lower_link"));
id = scene_graph->RegisterGeometry(
source_id_, lower_link_frame_id_,
make_unique<GeometryInstance>(
math::RigidTransformd(Vector3d(0., 0.25, -params.l2() / 2.)),
make_unique<Cylinder>(0.05, params.l2()), "lower_link"));
scene_graph->AssignRole(
source_id_, id, MakePhongIllustrationProperties(Vector4d(0, 0, 1, 1)));
}
AcrobotGeometry::~AcrobotGeometry() = default;
void AcrobotGeometry::OutputGeometryPose(
const systems::Context<double>& context,
geometry::FramePoseVector<double>* poses) const {
DRAKE_DEMAND(upper_link_frame_id_.is_valid());
DRAKE_DEMAND(lower_link_frame_id_.is_valid());
// TODO(russt): Use AcrobotState here upon resolution of #12566.
const auto& input =
get_input_port(0).Eval<systems::BasicVector<double>>(context);
const double theta1 = input[0], theta2 = input[1];
const math::RigidTransformd upper_link_pose(
math::RotationMatrixd::MakeYRotation(theta1));
const math::RigidTransformd lower_link_pose(
math::RotationMatrixd::MakeYRotation(theta1 + theta2),
Vector3d(-l1_ * std::sin(theta1), 0, -l1_ * std::cos(theta1)));
*poses = {{upper_link_frame_id_, upper_link_pose},
{lower_link_frame_id_, lower_link_pose}};
}
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_plant.h | #pragma once
#include <memory>
#include "drake/examples/acrobot/acrobot_input.h"
#include "drake/examples/acrobot/acrobot_params.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/systems/framework/basic_vector.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/primitives/affine_system.h"
namespace drake {
namespace examples {
namespace acrobot {
/// @defgroup acrobot_systems Acrobot
/// @{
/// @brief Systems related to the Acrobot example.
/// @ingroup example_systems
/// @}
/// The Acrobot - a canonical underactuated system as described in <a
/// href="http://underactuated.mit.edu/underactuated.html?chapter=3">Chapter 3
/// of Underactuated Robotics</a>.
///
/// @system
/// name: AcrobotPlant
/// input_ports:
/// - elbow_torque (optional)
/// output_ports:
/// - acrobot_state
/// @endsystem
///
/// Note: If the elbow_torque input port is not connected, then the torque is
/// taken to be zero.
///
/// @tparam_default_scalar
/// @ingroup acrobot_systems
template <typename T>
class AcrobotPlant : public systems::LeafSystem<T> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(AcrobotPlant)
/// Constructs the plant. The parameters of the system are stored as
/// Parameters in the Context (see acrobot_params.h).
AcrobotPlant();
/// Scalar-converting copy constructor. See @ref system_scalar_conversion.
template <typename U>
explicit AcrobotPlant(const AcrobotPlant<U>&);
/// Sets the parameters to describe MIT Robot Locomotion Group's hardware
/// acrobot.
void SetMitAcrobotParameters(AcrobotParams<T>* parameters) const;
///@{
/// Manipulator equation of Acrobot: M(q)q̈ + bias(q,q̇) = B*u.
///
/// - M[2x2] is the mass matrix.
/// - bias[2x1] includes the Coriolis term, gravity term and the damping term,
/// i.e. bias[2x1] = C(q,v)*v - τ_g(q) + [b1*q̇₁;b2*q̇₂].
// TODO(russt): Update this to the newest conventions.
Vector2<T> DynamicsBiasTerm(const systems::Context<T> &context) const;
Matrix2<T> MassMatrix(const systems::Context<T> &context) const;
///@}
/// Evaluates the input port and returns the scalar value of the commanded
/// torque. If the input port is not connected, then the torque is taken to
/// be zero.
const T get_tau(const systems::Context<T>& context) const {
const systems::BasicVector<T>* u_vec = this->EvalVectorInput(context, 0);
return u_vec ? u_vec->GetAtIndex(0) : 0.0;
}
static const AcrobotState<T>& get_state(
const systems::ContinuousState<T>& cstate) {
return dynamic_cast<const AcrobotState<T>&>(cstate.get_vector());
}
static const AcrobotState<T>& get_state(const systems::Context<T>& context) {
return get_state(context.get_continuous_state());
}
static AcrobotState<T>& get_mutable_state(
systems::ContinuousState<T>* cstate) {
return dynamic_cast<AcrobotState<T>&>(cstate->get_mutable_vector());
}
static AcrobotState<T>& get_mutable_state(systems::Context<T>* context) {
return get_mutable_state(&context->get_mutable_continuous_state());
}
const AcrobotParams<T>& get_parameters(
const systems::Context<T>& context) const {
return this->template GetNumericParameter<AcrobotParams>(context, 0);
}
AcrobotParams<T>& get_mutable_parameters(systems::Context<T>* context) const {
return this->template GetMutableNumericParameter<AcrobotParams>(context, 0);
}
private:
T DoCalcKineticEnergy(const systems::Context<T>& context) const override;
T DoCalcPotentialEnergy(const systems::Context<T>& context) const override;
void DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const override;
void DoCalcImplicitTimeDerivativesResidual(
const systems::Context<T>& context,
const systems::ContinuousState<T>& proposed_derivatives,
EigenPtr<VectorX<T>> residual) const override;
};
/// Constructs the Acrobot with (only) encoder outputs.
///
/// @system
/// name: AcrobotWEncoder
/// input_ports:
/// - elbow_torque
/// output_ports:
/// - measured_joint_positions
/// - <span style="color:gray">acrobot_state</span>
/// @endsystem
///
/// The `acrobot_state` output port is present only if the construction
/// parameter `acrobot_state_as_second_output` is true.
///
/// @ingroup acrobot_systems
template <typename T>
class AcrobotWEncoder : public systems::Diagram<T> {
public:
explicit AcrobotWEncoder(bool acrobot_state_as_second_output = false);
const AcrobotPlant<T>* acrobot_plant() const { return acrobot_plant_; }
AcrobotState<T>& get_mutable_acrobot_state(
systems::Context<T>* context) const;
private:
AcrobotPlant<T>* acrobot_plant_{nullptr};
};
/// Constructs the LQR controller for stabilizing the upright fixed point using
/// default LQR cost matrices which have been tested for this system.
/// @ingroup acrobot_systems
std::unique_ptr<systems::AffineSystem<double>> BalancingLQRController(
const AcrobotPlant<double>& acrobot);
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/optimizer_demo.py | """Demonstration of optimizing Spong controller parameters for an acrobot
using a Monte Carlo scenario.
"""
import argparse
from contextlib import closing
import os
import subprocess
import sys
import tempfile
import numpy as np
from pydrake.common import FindResourceOrThrow
from examples.acrobot.acrobot_io import (
load_output, load_scenario, save_scenario)
from examples.acrobot.metrics import (
ensemble_cost, success_rate)
try:
from scipy.optimize import fmin
except ImportError:
print("WARNING: scipy not installed, using stubbed non-minimizing "
"version of fmin.", file=sys.stderr)
def fmin(func, x0, func_args=(), full_output=False, *args, **kwargs):
"""Dummy version of scipy.optimize.fmin.
It allows scipy to be an optional dependency."""
if full_output:
fopt = func(x0, *func_args)
return x0, fopt, 1, 1, 0
else:
return x0
METRICS = {"ensemble_cost": ensemble_cost,
"success_rate": success_rate}
def evaluate_metric_once(scenario, metric, seeds):
"""Runs one evaluation of metric by running a roll out of scenario
with each random seed in seeds.
"""
runner = FindResourceOrThrow(
"drake/examples/acrobot/spong_sim_main_cc")
env_tmpdir = os.getenv("TEST_TEMPDIR") or os.getenv("TMPDIR") or "/tmp"
with tempfile.TemporaryDirectory(prefix="optimizer_demo",
dir=env_tmpdir) as temp_dir:
scenario_filename = os.path.join(temp_dir, "scenario.yaml")
with open(scenario_filename, "w") as scenario_file:
scenario_file.write(save_scenario(scenario=scenario))
tapes = []
for seed in seeds:
output_filename = os.path.join(temp_dir, f"output_{seed}.yaml")
subprocess.check_call(
[runner,
"--scenario", scenario_filename,
"--output", output_filename,
"--random_seed", str(seed)])
tapes += [load_output(filename=output_filename)]
metric_value = metric(tapes)
return metric_value
def optimize_controller_params(
scenario, metric, ensemble_size, num_evaluations):
"""Runs `scipy.optimize.fmin` over the `controller_params` of scenario
(treating the existing parameter values there as the starting point). The
metric is run over ensemble_size fixed random seeds. The optimizer
is given a budget of num_evaluations metric evaluations.
Because each metric evaluation runs a full ensemble, the total number of
simulations is ensemble_size * num_evaluations.
"""
seeds = list(range(1, 1 + ensemble_size))
metric_evaluation_count = 0
def function_to_optimize(params):
nonlocal metric_evaluation_count
metric_evaluation_count += 1
print(f"Iteration {metric_evaluation_count}: {len(seeds)} rollouts...")
new_scenario = scenario
new_scenario["controller_params"] = list(params)
metric_value = evaluate_metric_once(new_scenario, metric, seeds)
print(f" ...{metric_evaluation_count}: metric is {metric_value}")
return metric_value
try:
x0 = np.asfarray(scenario["controller_params"])
except TypeError:
x0 = (np.asfarray(scenario["controller_params"]["min"])
+ np.asfarray(scenario["controller_params"]["max"])) / 2
result = fmin(func=function_to_optimize,
x0=x0,
maxfun=num_evaluations)
return result
def main():
parser = argparse.ArgumentParser(__doc__)
# There is a subtlety to the default `scenario_file`: any default must be
# a bazel `data=` dependency, but any user-specified file cannot be relied
# on to be a bazel dependency, so we do `FindResourceOrThrow` resolution
# only on the default and not on any user-provided argument.
parser.add_argument(
"--scenario_file", "-f", type=str, default=None,
help="Scenario to run (default: example_stochastic_scenario.yaml)")
parser.add_argument(
"--metric", "-m", type=str, choices=METRICS.keys(),
help="Choice of metric to optimize (default: %(default)s)",
default="ensemble_cost")
parser.add_argument(
"--ensemble_size", "-e", type=int, default=10,
help=("Size of ensemble for each cost function evaluation "
"(default: %(default)s)"))
parser.add_argument(
"--num_evaluations", "-n", type=int, default=250,
help=("Cost function call budget of the optimizer "
"(default: %(default)s)"))
parser.add_argument(
"--output", "-o", type=argparse.FileType("w"), default=sys.stdout,
help="File to write the optimized output (default: stdout)")
args = parser.parse_args()
with closing(args.output) as output:
scenario_file = args.scenario_file or FindResourceOrThrow(
"drake/examples/acrobot/test/example_stochastic_scenario.yaml")
input_scenario = load_scenario(filename=scenario_file)
result = optimize_controller_params(
scenario=input_scenario,
metric=METRICS[args.metric],
ensemble_size=args.ensemble_size,
num_evaluations=args.num_evaluations)
output_scenario = input_scenario
output_scenario["controller_params"] = result
output.write(save_scenario(scenario=output_scenario))
if __name__ == "__main__":
main()
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_params.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Describes the row indices of a AcrobotParams.
struct AcrobotParamsIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 11;
// The index of each individual coordinate.
static const int kM1 = 0;
static const int kM2 = 1;
static const int kL1 = 2;
static const int kL2 = 3;
static const int kLc1 = 4;
static const int kLc2 = 5;
static const int kIc1 = 6;
static const int kIc2 = 7;
static const int kB1 = 8;
static const int kB2 = 9;
static const int kGravity = 10;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `AcrobotParamsIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class AcrobotParams final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef AcrobotParamsIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c m1 defaults to 1.0 kg.
/// @arg @c m2 defaults to 1.0 kg.
/// @arg @c l1 defaults to 1.0 m.
/// @arg @c l2 defaults to 2.0 m.
/// @arg @c lc1 defaults to 0.5 m.
/// @arg @c lc2 defaults to 1.0 m.
/// @arg @c Ic1 defaults to 0.083 kg*m^2.
/// @arg @c Ic2 defaults to 0.33 kg*m^2.
/// @arg @c b1 defaults to 0.1 kg*m^2/s.
/// @arg @c b2 defaults to 0.1 kg*m^2/s.
/// @arg @c gravity defaults to 9.81 m/s^2.
AcrobotParams() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_m1(1.0);
this->set_m2(1.0);
this->set_l1(1.0);
this->set_l2(2.0);
this->set_lc1(0.5);
this->set_lc2(1.0);
this->set_Ic1(0.083);
this->set_Ic2(0.33);
this->set_b1(0.1);
this->set_b2(0.1);
this->set_gravity(9.81);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
AcrobotParams(const AcrobotParams& other)
: drake::systems::BasicVector<T>(other.values()) {}
AcrobotParams(AcrobotParams&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
AcrobotParams& operator=(const AcrobotParams& other) {
this->values() = other.values();
return *this;
}
AcrobotParams& operator=(AcrobotParams&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_m1(symbolic::Variable("m1"));
this->set_m2(symbolic::Variable("m2"));
this->set_l1(symbolic::Variable("l1"));
this->set_l2(symbolic::Variable("l2"));
this->set_lc1(symbolic::Variable("lc1"));
this->set_lc2(symbolic::Variable("lc2"));
this->set_Ic1(symbolic::Variable("Ic1"));
this->set_Ic2(symbolic::Variable("Ic2"));
this->set_b1(symbolic::Variable("b1"));
this->set_b2(symbolic::Variable("b2"));
this->set_gravity(symbolic::Variable("gravity"));
}
[[nodiscard]] AcrobotParams<T>* DoClone() const final {
return new AcrobotParams;
}
/// @name Getters and Setters
//@{
/// Mass of link 1.
/// @note @c m1 is expressed in units of kg.
/// @note @c m1 has a limited domain of [0.0, +Inf].
const T& m1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kM1);
}
/// Setter that matches m1().
void set_m1(const T& m1) {
ThrowIfEmpty();
this->SetAtIndex(K::kM1, m1);
}
/// Fluent setter that matches m1().
/// Returns a copy of `this` with m1 set to a new value.
[[nodiscard]] AcrobotParams<T> with_m1(const T& m1) const {
AcrobotParams<T> result(*this);
result.set_m1(m1);
return result;
}
/// Mass of link 2.
/// @note @c m2 is expressed in units of kg.
/// @note @c m2 has a limited domain of [0.0, +Inf].
const T& m2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kM2);
}
/// Setter that matches m2().
void set_m2(const T& m2) {
ThrowIfEmpty();
this->SetAtIndex(K::kM2, m2);
}
/// Fluent setter that matches m2().
/// Returns a copy of `this` with m2 set to a new value.
[[nodiscard]] AcrobotParams<T> with_m2(const T& m2) const {
AcrobotParams<T> result(*this);
result.set_m2(m2);
return result;
}
/// Length of link 1.
/// @note @c l1 is expressed in units of m.
/// @note @c l1 has a limited domain of [0.0, +Inf].
const T& l1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kL1);
}
/// Setter that matches l1().
void set_l1(const T& l1) {
ThrowIfEmpty();
this->SetAtIndex(K::kL1, l1);
}
/// Fluent setter that matches l1().
/// Returns a copy of `this` with l1 set to a new value.
[[nodiscard]] AcrobotParams<T> with_l1(const T& l1) const {
AcrobotParams<T> result(*this);
result.set_l1(l1);
return result;
}
/// Length of link 2.
/// @note @c l2 is expressed in units of m.
/// @note @c l2 has a limited domain of [0.0, +Inf].
const T& l2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kL2);
}
/// Setter that matches l2().
void set_l2(const T& l2) {
ThrowIfEmpty();
this->SetAtIndex(K::kL2, l2);
}
/// Fluent setter that matches l2().
/// Returns a copy of `this` with l2 set to a new value.
[[nodiscard]] AcrobotParams<T> with_l2(const T& l2) const {
AcrobotParams<T> result(*this);
result.set_l2(l2);
return result;
}
/// Vertical distance from shoulder joint to center of mass of link 1.
/// @note @c lc1 is expressed in units of m.
/// @note @c lc1 has a limited domain of [0.0, +Inf].
const T& lc1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kLc1);
}
/// Setter that matches lc1().
void set_lc1(const T& lc1) {
ThrowIfEmpty();
this->SetAtIndex(K::kLc1, lc1);
}
/// Fluent setter that matches lc1().
/// Returns a copy of `this` with lc1 set to a new value.
[[nodiscard]] AcrobotParams<T> with_lc1(const T& lc1) const {
AcrobotParams<T> result(*this);
result.set_lc1(lc1);
return result;
}
/// Vertical distance from elbow joint to center of mass of link 1.
/// @note @c lc2 is expressed in units of m.
/// @note @c lc2 has a limited domain of [0.0, +Inf].
const T& lc2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kLc2);
}
/// Setter that matches lc2().
void set_lc2(const T& lc2) {
ThrowIfEmpty();
this->SetAtIndex(K::kLc2, lc2);
}
/// Fluent setter that matches lc2().
/// Returns a copy of `this` with lc2 set to a new value.
[[nodiscard]] AcrobotParams<T> with_lc2(const T& lc2) const {
AcrobotParams<T> result(*this);
result.set_lc2(lc2);
return result;
}
/// Inertia of link 1 about the center of mass of link 1.
/// @note @c Ic1 is expressed in units of kg*m^2.
/// @note @c Ic1 has a limited domain of [0.0, +Inf].
const T& Ic1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kIc1);
}
/// Setter that matches Ic1().
void set_Ic1(const T& Ic1) {
ThrowIfEmpty();
this->SetAtIndex(K::kIc1, Ic1);
}
/// Fluent setter that matches Ic1().
/// Returns a copy of `this` with Ic1 set to a new value.
[[nodiscard]] AcrobotParams<T> with_Ic1(const T& Ic1) const {
AcrobotParams<T> result(*this);
result.set_Ic1(Ic1);
return result;
}
/// Inertia of link 2 about the center of mass of link 2.
/// @note @c Ic2 is expressed in units of kg*m^2.
/// @note @c Ic2 has a limited domain of [0.0, +Inf].
const T& Ic2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kIc2);
}
/// Setter that matches Ic2().
void set_Ic2(const T& Ic2) {
ThrowIfEmpty();
this->SetAtIndex(K::kIc2, Ic2);
}
/// Fluent setter that matches Ic2().
/// Returns a copy of `this` with Ic2 set to a new value.
[[nodiscard]] AcrobotParams<T> with_Ic2(const T& Ic2) const {
AcrobotParams<T> result(*this);
result.set_Ic2(Ic2);
return result;
}
/// Damping coefficient of the shoulder joint.
/// @note @c b1 is expressed in units of kg*m^2/s.
/// @note @c b1 has a limited domain of [0.0, +Inf].
const T& b1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kB1);
}
/// Setter that matches b1().
void set_b1(const T& b1) {
ThrowIfEmpty();
this->SetAtIndex(K::kB1, b1);
}
/// Fluent setter that matches b1().
/// Returns a copy of `this` with b1 set to a new value.
[[nodiscard]] AcrobotParams<T> with_b1(const T& b1) const {
AcrobotParams<T> result(*this);
result.set_b1(b1);
return result;
}
/// Damping coefficient of the elbow joint.
/// @note @c b2 is expressed in units of kg*m^2/s.
/// @note @c b2 has a limited domain of [0.0, +Inf].
const T& b2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kB2);
}
/// Setter that matches b2().
void set_b2(const T& b2) {
ThrowIfEmpty();
this->SetAtIndex(K::kB2, b2);
}
/// Fluent setter that matches b2().
/// Returns a copy of `this` with b2 set to a new value.
[[nodiscard]] AcrobotParams<T> with_b2(const T& b2) const {
AcrobotParams<T> result(*this);
result.set_b2(b2);
return result;
}
/// Gravitational constant.
/// @note @c gravity is expressed in units of m/s^2.
/// @note @c gravity has a limited domain of [0.0, +Inf].
const T& gravity() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kGravity);
}
/// Setter that matches gravity().
void set_gravity(const T& gravity) {
ThrowIfEmpty();
this->SetAtIndex(K::kGravity, gravity);
}
/// Fluent setter that matches gravity().
/// Returns a copy of `this` with gravity set to a new value.
[[nodiscard]] AcrobotParams<T> with_gravity(const T& gravity) const {
AcrobotParams<T> result(*this);
result.set_gravity(gravity);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& m1_ref = this->GetAtIndex(K::kM1);
a->Visit(drake::MakeNameValue("m1", &m1_ref));
T& m2_ref = this->GetAtIndex(K::kM2);
a->Visit(drake::MakeNameValue("m2", &m2_ref));
T& l1_ref = this->GetAtIndex(K::kL1);
a->Visit(drake::MakeNameValue("l1", &l1_ref));
T& l2_ref = this->GetAtIndex(K::kL2);
a->Visit(drake::MakeNameValue("l2", &l2_ref));
T& lc1_ref = this->GetAtIndex(K::kLc1);
a->Visit(drake::MakeNameValue("lc1", &lc1_ref));
T& lc2_ref = this->GetAtIndex(K::kLc2);
a->Visit(drake::MakeNameValue("lc2", &lc2_ref));
T& Ic1_ref = this->GetAtIndex(K::kIc1);
a->Visit(drake::MakeNameValue("Ic1", &Ic1_ref));
T& Ic2_ref = this->GetAtIndex(K::kIc2);
a->Visit(drake::MakeNameValue("Ic2", &Ic2_ref));
T& b1_ref = this->GetAtIndex(K::kB1);
a->Visit(drake::MakeNameValue("b1", &b1_ref));
T& b2_ref = this->GetAtIndex(K::kB2);
a->Visit(drake::MakeNameValue("b2", &b2_ref));
T& gravity_ref = this->GetAtIndex(K::kGravity);
a->Visit(drake::MakeNameValue("gravity", &gravity_ref));
}
/// See AcrobotParamsIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return AcrobotParamsIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(m1());
result = result && (m1() >= T(0.0));
result = result && !isnan(m2());
result = result && (m2() >= T(0.0));
result = result && !isnan(l1());
result = result && (l1() >= T(0.0));
result = result && !isnan(l2());
result = result && (l2() >= T(0.0));
result = result && !isnan(lc1());
result = result && (lc1() >= T(0.0));
result = result && !isnan(lc2());
result = result && (lc2() >= T(0.0));
result = result && !isnan(Ic1());
result = result && (Ic1() >= T(0.0));
result = result && !isnan(Ic2());
result = result && (Ic2() >= T(0.0));
result = result && !isnan(b1());
result = result && (b1() >= T(0.0));
result = result && !isnan(b2());
result = result && (b2() >= T(0.0));
result = result && !isnan(gravity());
result = result && (gravity() >= T(0.0));
return result;
}
void GetElementBounds(Eigen::VectorXd* lower,
Eigen::VectorXd* upper) const final {
const double kInf = std::numeric_limits<double>::infinity();
*lower = Eigen::Matrix<double, 11, 1>::Constant(-kInf);
*upper = Eigen::Matrix<double, 11, 1>::Constant(kInf);
(*lower)(K::kM1) = 0.0;
(*lower)(K::kM2) = 0.0;
(*lower)(K::kL1) = 0.0;
(*lower)(K::kL2) = 0.0;
(*lower)(K::kLc1) = 0.0;
(*lower)(K::kLc2) = 0.0;
(*lower)(K::kIc1) = 0.0;
(*lower)(K::kIc2) = 0.0;
(*lower)(K::kB1) = 0.0;
(*lower)(K::kB2) = 0.0;
(*lower)(K::kGravity) = 0.0;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The AcrobotParams vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_state.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Describes the row indices of a AcrobotState.
struct AcrobotStateIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 4;
// The index of each individual coordinate.
static const int kTheta1 = 0;
static const int kTheta2 = 1;
static const int kTheta1dot = 2;
static const int kTheta2dot = 3;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `AcrobotStateIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class AcrobotState final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef AcrobotStateIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c theta1 defaults to 0.0 rad.
/// @arg @c theta2 defaults to 0.0 rad.
/// @arg @c theta1dot defaults to 0.0 rad/s.
/// @arg @c theta2dot defaults to 0.0 rad/s.
AcrobotState() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_theta1(0.0);
this->set_theta2(0.0);
this->set_theta1dot(0.0);
this->set_theta2dot(0.0);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
AcrobotState(const AcrobotState& other)
: drake::systems::BasicVector<T>(other.values()) {}
AcrobotState(AcrobotState&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
AcrobotState& operator=(const AcrobotState& other) {
this->values() = other.values();
return *this;
}
AcrobotState& operator=(AcrobotState&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_theta1(symbolic::Variable("theta1"));
this->set_theta2(symbolic::Variable("theta2"));
this->set_theta1dot(symbolic::Variable("theta1dot"));
this->set_theta2dot(symbolic::Variable("theta2dot"));
}
[[nodiscard]] AcrobotState<T>* DoClone() const final {
return new AcrobotState;
}
/// @name Getters and Setters
//@{
/// The shoulder joint angle
/// @note @c theta1 is expressed in units of rad.
const T& theta1() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta1);
}
/// Setter that matches theta1().
void set_theta1(const T& theta1) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta1, theta1);
}
/// Fluent setter that matches theta1().
/// Returns a copy of `this` with theta1 set to a new value.
[[nodiscard]] AcrobotState<T> with_theta1(const T& theta1) const {
AcrobotState<T> result(*this);
result.set_theta1(theta1);
return result;
}
/// The elbow joint angle
/// @note @c theta2 is expressed in units of rad.
const T& theta2() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta2);
}
/// Setter that matches theta2().
void set_theta2(const T& theta2) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta2, theta2);
}
/// Fluent setter that matches theta2().
/// Returns a copy of `this` with theta2 set to a new value.
[[nodiscard]] AcrobotState<T> with_theta2(const T& theta2) const {
AcrobotState<T> result(*this);
result.set_theta2(theta2);
return result;
}
/// The shoulder joint velocity
/// @note @c theta1dot is expressed in units of rad/s.
const T& theta1dot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta1dot);
}
/// Setter that matches theta1dot().
void set_theta1dot(const T& theta1dot) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta1dot, theta1dot);
}
/// Fluent setter that matches theta1dot().
/// Returns a copy of `this` with theta1dot set to a new value.
[[nodiscard]] AcrobotState<T> with_theta1dot(const T& theta1dot) const {
AcrobotState<T> result(*this);
result.set_theta1dot(theta1dot);
return result;
}
/// The elbow joint velocity
/// @note @c theta2dot is expressed in units of rad/s.
const T& theta2dot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta2dot);
}
/// Setter that matches theta2dot().
void set_theta2dot(const T& theta2dot) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta2dot, theta2dot);
}
/// Fluent setter that matches theta2dot().
/// Returns a copy of `this` with theta2dot set to a new value.
[[nodiscard]] AcrobotState<T> with_theta2dot(const T& theta2dot) const {
AcrobotState<T> result(*this);
result.set_theta2dot(theta2dot);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& theta1_ref = this->GetAtIndex(K::kTheta1);
a->Visit(drake::MakeNameValue("theta1", &theta1_ref));
T& theta2_ref = this->GetAtIndex(K::kTheta2);
a->Visit(drake::MakeNameValue("theta2", &theta2_ref));
T& theta1dot_ref = this->GetAtIndex(K::kTheta1dot);
a->Visit(drake::MakeNameValue("theta1dot", &theta1dot_ref));
T& theta2dot_ref = this->GetAtIndex(K::kTheta2dot);
a->Visit(drake::MakeNameValue("theta2dot", &theta2dot_ref));
}
/// See AcrobotStateIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return AcrobotStateIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(theta1());
result = result && !isnan(theta2());
result = result && !isnan(theta1dot());
result = result && !isnan(theta2dot());
return result;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The AcrobotState vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_geometry.h | #pragma once
#include "drake/examples/acrobot/acrobot_params.h"
#include "drake/geometry/scene_graph.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace acrobot {
/// Expresses an AcrobotPlant's geometry to a SceneGraph.
///
/// @system
/// name: AcrobotGeometry
/// input_ports:
/// - state
/// output_ports:
/// - geometry_pose
/// @endsystem
///
/// This class has no public constructor; instead use the AddToBuilder() static
/// method to create and add it to a DiagramBuilder directly.
///
/// @ingroup acrobot_systems
class AcrobotGeometry final : public systems::LeafSystem<double> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(AcrobotGeometry);
~AcrobotGeometry() final;
/// Creates, adds, and connects an AcrobotGeometry system into the given
/// `builder`. Both the `acrobot_state.get_system()` and `scene_graph`
/// systems must have been added to the given `builder` already.
///
/// @param acrobot_params sets the parameters of the geometry registered
/// with `scene_graph`.
///
/// The `scene_graph` pointer is not retained by the %AcrobotGeometry
/// system. The return value pointer is an alias of the new
/// %AcrobotGeometry system that is owned by the `builder`.
static const AcrobotGeometry* AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& acrobot_state_port,
const AcrobotParams<double>& acrobot_params,
geometry::SceneGraph<double>* scene_graph);
/// Creates, adds, and connects an AcrobotGeometry system into the given
/// `builder`. Both the `acrobot_state.get_system()` and `scene_graph`
/// systems must have been added to the given `builder` already.
///
/// Acrobot parameters are set to their default values.
///
/// The `scene_graph` pointer is not retained by the %AcrobotGeometry
/// system. The return value pointer is an alias of the new
/// %AcrobotGeometry system that is owned by the `builder`.
static const AcrobotGeometry* AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& acrobot_state_port,
geometry::SceneGraph<double>* scene_graph) {
return AddToBuilder(builder, acrobot_state_port, AcrobotParams<double>(),
scene_graph);
}
private:
AcrobotGeometry(const AcrobotParams<double>& acrobot_params,
geometry::SceneGraph<double>*);
void OutputGeometryPose(const systems::Context<double>&,
geometry::FramePoseVector<double>*) const;
// Geometry source identifier for this system to interact with SceneGraph.
geometry::SourceId source_id_{};
// The frames for the two links.
geometry::FrameId upper_link_frame_id_{};
geometry::FrameId lower_link_frame_id_{};
// Local copy of the parameter required for the forward kinematics (the
// length of the upper link).
const double l1_;
};
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/spong_controller.cc | #include "drake/examples/acrobot/spong_controller.h"
#include "drake/common/default_scalars.h"
DRAKE_DEFINE_CLASS_TEMPLATE_INSTANTIATIONS_ON_DEFAULT_SCALARS(
class ::drake::examples::acrobot::AcrobotSpongController)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/Acrobot.urdf | <?xml version="1.0"?>
<robot name="Acrobot">
<link name="base_link">
<visual>
<geometry>
<box size="0.2 0.2 0.2"/>
</geometry>
<material name="green">
<color rgba="0 1 0 1"/>
</material>
</visual>
<collision name="base_link_collision">
<geometry>
<box size="0.2 0.2 0.2"/>
</geometry>
</collision>
</link>
<link name="upper_link">
<inertial>
<origin xyz="0 0 -.5" rpy="0 0 0"/>
<mass value="1"/>
<inertia ixx="1" ixy="0" ixz="0" iyy="0.083" iyz="0" izz="1"/>
</inertial>
<visual>
<origin xyz="0 0 -0.5" rpy="0 0 0"/>
<geometry>
<cylinder length="1.1" radius="0.05"/>
</geometry>
<material name="red">
<color rgba="1 0 0 1"/>
</material>
</visual>
<collision name="upper_link_collision">
<origin xyz="0 0 -0.5" rpy="0 0 0"/>
<geometry>
<cylinder length="1.1" radius="0.05"/>
</geometry>
</collision>
</link>
<link name="lower_link">
<inertial>
<origin xyz="0 0 -1" rpy="0 0 0"/>
<mass value="1"/>
<inertia ixx="1" ixy="0" ixz="0" iyy="0.33" iyz="0" izz="1"/>
</inertial>
<visual>
<origin xyz="0 0 -1" rpy="0 0 0"/>
<geometry>
<cylinder length="2.1" radius=".05"/>
</geometry>
<material name="blue">
<color rgba="0 0 1 1"/>
</material>
</visual>
<collision name="lower_link_collision">
<origin xyz="0 0 -1" rpy="0 0 0"/>
<geometry>
<cylinder length="2.1" radius=".05"/>
</geometry>
</collision>
</link>
<joint name="base_weld" type="fixed">
<parent link="world"/>
<child link="base_link"/>
</joint>
<joint name="shoulder" type="continuous">
<parent link="base_link"/>
<child link="upper_link"/>
<origin xyz="0 0.15 0"/>
<axis xyz="0 1 0"/>
<dynamics damping="0.1"/>
</joint>
<joint name="elbow" type="continuous">
<parent link="upper_link"/>
<child link="lower_link"/>
<origin xyz="0 0.1 -1"/>
<axis xyz="0 1 0"/>
<dynamics damping="0.1"/>
</joint>
<transmission type="SimpleTransmission" name="elbow_trans">
<actuator name="elbow"/>
<joint name="elbow"/>
<mechanicalReduction>1</mechanicalReduction>
</transmission>
<frame name="hand" link="lower_link" xyz="0 0 -2.1" rpy="0 0 0"/>
</robot>
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/acrobot_plant.cc | #include "drake/examples/acrobot/acrobot_plant.h"
#include <cmath>
#include <vector>
#include "drake/common/default_scalars.h"
#include "drake/common/drake_throw.h"
#include "drake/systems/controllers/linear_quadratic_regulator.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/sensors/rotary_encoders.h"
using std::sin;
using std::cos;
namespace drake {
namespace examples {
namespace acrobot {
template <typename T>
AcrobotPlant<T>::AcrobotPlant()
: systems::LeafSystem<T>(systems::SystemTypeTag<AcrobotPlant>{}) {
this->DeclareNumericParameter(AcrobotParams<T>());
this->DeclareVectorInputPort("elbow_torque", AcrobotInput<T>());
auto state_index = this->DeclareContinuousState(
AcrobotState<T>(), 2 /* num_q */, 2 /* num_v */, 0 /* num_z */);
this->DeclareStateOutputPort("acrobot_state", state_index);
}
template <typename T>
template <typename U>
AcrobotPlant<T>::AcrobotPlant(const AcrobotPlant<U>&) : AcrobotPlant<T>() {}
template <typename T>
void AcrobotPlant<T>::SetMitAcrobotParameters(
AcrobotParams<T>* parameters) const {
DRAKE_DEMAND(parameters != nullptr);
parameters->set_m1(2.4367);
parameters->set_m2(0.6178);
parameters->set_l1(0.2563);
parameters->set_lc1(1.6738);
parameters->set_lc2(1.5651);
parameters->set_Ic1(
-4.7443); // Notes: Yikes! Negative inertias (from sysid).
parameters->set_Ic2(-1.0068);
parameters->set_b1(0.0320);
parameters->set_b2(0.0413);
// Note: parameters are identified in a way that torque has the unit of
// current (Amps), in order to simplify the implementation of torque
// constraint on motors. Therefore, some of the numbers here have incorrect
// units.
}
template <typename T>
Matrix2<T> AcrobotPlant<T>::MassMatrix(
const systems::Context<T> &context) const {
const AcrobotState<T>& state = get_state(context);
const AcrobotParams<T>& p = get_parameters(context);
const T c2 = cos(state.theta2());
const T I1 = p.Ic1() + p.m1() * p.lc1() * p.lc1();
const T I2 = p.Ic2() + p.m2() * p.lc2() * p.lc2();
const T m2l1lc2 = p.m2() * p.l1() * p.lc2();
const T m12 = I2 + m2l1lc2 * c2;
Matrix2<T> M;
M << I1 + I2 + p.m2() * p.l1() * p.l1() + 2 * m2l1lc2 * c2, m12, m12,
I2;
return M;
}
template <typename T>
Vector2<T> AcrobotPlant<T>::DynamicsBiasTerm(const systems::Context<T> &
context)
const {
const AcrobotState<T>& state = get_state(context);
const AcrobotParams<T>& p = get_parameters(context);
const T s1 = sin(state.theta1()), s2 = sin(state.theta2());
const T s12 = sin(state.theta1() + state.theta2());
const T m2l1lc2 = p.m2() * p.l1() * p.lc2();
Vector2<T> bias;
// C(q,v)*v terms.
bias << -2 * m2l1lc2 * s2 * state.theta2dot() * state.theta1dot() +
-m2l1lc2 * s2 * state.theta2dot() * state.theta2dot(),
m2l1lc2 * s2 * state.theta1dot() * state.theta1dot();
// -τ_g(q) terms.
bias(0) += p.gravity() * p.m1() * p.lc1() * s1 +
p.gravity() * p.m2() * (p.l1() * s1 + p.lc2() * s12);
bias(1) += p.gravity() * p.m2() * p.lc2() * s12;
// Damping terms.
bias(0) += p.b1() * state.theta1dot();
bias(1) += p.b2() * state.theta2dot();
return bias;
}
// Compute the actual physics.
template <typename T>
void AcrobotPlant<T>::DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const {
const AcrobotState<T>& state = get_state(context);
const T& tau = get_tau(context);
const Matrix2<T> M = MassMatrix(context);
const Vector2<T> bias = DynamicsBiasTerm(context);
const Vector2<T> B(0, 1); // input matrix
Vector4<T> xdot;
xdot << state.theta1dot(), state.theta2dot(),
M.inverse() * (B * tau - bias);
derivatives->SetFromVector(xdot);
}
template <typename T>
void AcrobotPlant<T>::DoCalcImplicitTimeDerivativesResidual(
const systems::Context<T>& context,
const systems::ContinuousState<T>& proposed_derivatives,
EigenPtr<VectorX<T>> residual) const {
DRAKE_DEMAND(residual != nullptr);
const AcrobotState<T>& state = get_state(context);
const T& tau = get_tau(context);
const Matrix2<T> M = MassMatrix(context);
const Vector2<T> bias = DynamicsBiasTerm(context);
const Vector2<T> B(0, 1); // input matrix
const auto& proposed_qdot = proposed_derivatives.get_generalized_position();
const auto proposed_vdot =
proposed_derivatives.get_generalized_velocity().CopyToVector();
*residual << proposed_qdot[0] - state.theta1dot(),
proposed_qdot[1] - state.theta2dot(),
M * proposed_vdot - (B * tau - bias);
}
template <typename T>
T AcrobotPlant<T>::DoCalcKineticEnergy(
const systems::Context<T>& context) const {
const AcrobotState<T>& state = dynamic_cast<const AcrobotState<T>&>(
context.get_continuous_state_vector());
Matrix2<T> M = MassMatrix(context);
Vector2<T> qdot(state.theta1dot(), state.theta2dot());
return 0.5 * qdot.transpose() * M * qdot;
}
template <typename T>
T AcrobotPlant<T>::DoCalcPotentialEnergy(
const systems::Context<T>& context) const {
const AcrobotState<T>& state = get_state(context);
const AcrobotParams<T>& p = get_parameters(context);
using std::cos;
const T c1 = cos(state.theta1());
const T c12 = cos(state.theta1() + state.theta2());
return -p.m1() * p.gravity() * p.lc1() * c1 -
p.m2() * p.gravity() * (p.l1() * c1 + p.lc2() * c12);
}
template <typename T>
AcrobotWEncoder<T>::AcrobotWEncoder(bool acrobot_state_as_second_output) {
systems::DiagramBuilder<T> builder;
acrobot_plant_ = builder.template AddSystem<AcrobotPlant<T>>();
acrobot_plant_->set_name("acrobot_plant");
auto encoder =
builder.template AddSystem<systems::sensors::RotaryEncoders<T>>(
4, std::vector<int>{0, 1});
encoder->set_name("encoder");
builder.Cascade(*acrobot_plant_, *encoder);
builder.ExportInput(acrobot_plant_->get_input_port(0), "elbow_torque");
builder.ExportOutput(encoder->get_output_port(), "measured_joint_positions");
if (acrobot_state_as_second_output)
builder.ExportOutput(acrobot_plant_->get_output_port(0), "acrobot_state");
builder.BuildInto(this);
}
template <typename T>
AcrobotState<T>& AcrobotWEncoder<T>::get_mutable_acrobot_state(
systems::Context<T>* context) const {
AcrobotState<T>* x = dynamic_cast<AcrobotState<T>*>(
&this->GetMutableSubsystemContext(*acrobot_plant_, context)
.get_mutable_continuous_state_vector());
DRAKE_DEMAND(x != nullptr);
return *x;
}
std::unique_ptr<systems::AffineSystem<double>> BalancingLQRController(
const AcrobotPlant<double>& acrobot) {
auto context = acrobot.CreateDefaultContext();
// Set nominal torque to zero.
acrobot.GetInputPort("elbow_torque").FixValue(context.get(), 0.0);
// Set nominal state to the upright fixed point.
AcrobotState<double>* x = dynamic_cast<AcrobotState<double>*>(
&context->get_mutable_continuous_state_vector());
DRAKE_ASSERT(x != nullptr);
x->set_theta1(M_PI);
x->set_theta2(0.0);
x->set_theta1dot(0.0);
x->set_theta2dot(0.0);
// Setup LQR Cost matrices (penalize position error 10x more than velocity
// to roughly address difference in units, using sqrt(g/l) as the time
// constant.
Eigen::Matrix4d Q = Eigen::Matrix4d::Identity();
Q(0, 0) = 10;
Q(1, 1) = 10;
Vector1d R = Vector1d::Constant(1);
return systems::controllers::LinearQuadraticRegulator(acrobot, *context, Q,
R);
}
} // namespace acrobot
} // namespace examples
} // namespace drake
DRAKE_DEFINE_CLASS_TEMPLATE_INSTANTIATIONS_ON_DEFAULT_SCALARS(
class ::drake::examples::acrobot::AcrobotPlant)
DRAKE_DEFINE_CLASS_TEMPLATE_INSTANTIATIONS_ON_DEFAULT_NONSYMBOLIC_SCALARS(
class ::drake::examples::acrobot::AcrobotWEncoder)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/acrobot/run_lqr_w_estimator.cc | #include <cmath>
#include <iostream>
#include <memory>
#include <gflags/gflags.h>
#include "drake/common/fmt_eigen.h"
#include "drake/common/proto/call_python.h"
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/examples/acrobot/acrobot_state.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/estimators/kalman_filter.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/primitives/linear_system.h"
#include "drake/systems/primitives/vector_log_sink.h"
#include "drake/systems/sensors/rotary_encoders.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
// Simple example which simulates the Acrobot, started near the upright
// configuration, with an LQR controller designed to stabilize the unstable
// fixed point and a state estimator in the loop. Run meldis to see the
// animated result.
DEFINE_double(simulation_sec, 5.0,
"Number of seconds to simulate.");
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
int do_main() {
// Make the robot.
systems::DiagramBuilder<double> builder;
auto acrobot_w_encoder = builder.AddSystem<AcrobotWEncoder<double>>(true);
acrobot_w_encoder->set_name("acrobot_w_encoder");
// Get a pointer to the actual plant subsystem (will be used below).
auto acrobot = acrobot_w_encoder->acrobot_plant();
// Attach a DrakeVisualizer so we can animate the robot.
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(
&builder, acrobot_w_encoder->get_output_port(1), scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
// Make a Kalman filter observer.
auto observer_acrobot = std::make_unique<AcrobotWEncoder<double>>();
auto observer_context = observer_acrobot->CreateDefaultContext();
{ // Set context to upright fixed point.
AcrobotState<double>& x0 =
observer_acrobot->get_mutable_acrobot_state(observer_context.get());
x0.set_theta1(M_PI);
x0.set_theta2(0.0);
x0.set_theta1dot(0.0);
x0.set_theta2dot(0.0);
observer_acrobot->GetInputPort("elbow_torque")
.FixValue(observer_context.get(), 0.0);
}
// Make a linearization here for the exercise below. Need to do it before I
// std::move the pointers.
auto linearized_acrobot =
systems::Linearize(*observer_acrobot, *observer_context);
Eigen::Matrix4d W = Eigen::Matrix4d::Identity();
Eigen::Matrix2d V =
0.1 * Eigen::Matrix2d::Identity(); // Position measurements are clean.
auto observer =
builder.AddSystem(systems::estimators::SteadyStateKalmanFilter(
std::move(observer_acrobot), std::move(observer_context), W, V));
observer->set_name("observer");
builder.Connect(acrobot_w_encoder->get_output_port(0),
observer->get_input_port(0));
{ // As a simple exercise, analyze the closed-loop estimation error dynamics.
std::cout << "Is this system observable? "
<< systems::IsObservable(*linearized_acrobot) << std::endl;
Eigen::Matrix4d error_sys =
linearized_acrobot->A() - observer->L() * linearized_acrobot->C();
fmt::print("L = {}\n", fmt_eigen(observer->L()));
fmt::print("A - LC =\n{}\n", fmt_eigen(error_sys));
fmt::print("eig(A-LC) = {}\n", fmt_eigen(error_sys.eigenvalues().real()));
}
// Make the LQR Controller.
auto controller = builder.AddSystem(BalancingLQRController(*acrobot));
controller->set_name("controller");
builder.Connect(observer->get_output_port(0), controller->get_input_port());
builder.Connect(controller->get_output_port(),
acrobot_w_encoder->get_input_port(0));
builder.Connect(controller->get_output_port(), observer->get_input_port(1));
// Log the true state and the estimated state.
auto x_logger = LogVectorOutput(acrobot_w_encoder->get_output_port(1),
&builder);
x_logger->set_name("x_logger");
auto xhat_logger = LogVectorOutput(observer->get_output_port(0), &builder);
xhat_logger->set_name("xhat_logger");
// Build the system/simulator.
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
// Set an initial condition near the upright fixed point.
AcrobotState<double>& x0 = acrobot_w_encoder->get_mutable_acrobot_state(
&(diagram->GetMutableSubsystemContext(*acrobot_w_encoder,
&simulator.get_mutable_context())));
x0.set_theta1(M_PI + 0.1);
x0.set_theta2(-.1);
x0.set_theta1dot(0.0);
x0.set_theta2dot(0.0);
// Set the initial conditions of the observer.
auto& xhat0 = diagram
->GetMutableSubsystemContext(
*observer, &simulator.get_mutable_context())
.get_mutable_continuous_state_vector();
xhat0.SetAtIndex(0, M_PI);
xhat0.SetAtIndex(1, 0.0);
xhat0.SetAtIndex(2, 0.0);
xhat0.SetAtIndex(3, 0.0);
// Simulate.
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.get_mutable_integrator().set_maximum_step_size(0.01);
simulator.get_mutable_integrator().set_fixed_step_mode(true);
simulator.Initialize();
simulator.AdvanceTo(FLAGS_simulation_sec);
// Plot the results (launch call_python_client to see the plots).
const auto& x_log = x_logger->FindLog(simulator.get_context());
const auto& xhat_log = xhat_logger->FindLog(simulator.get_context());
using common::CallPython;
using common::ToPythonTuple;
CallPython("figure", 1);
CallPython("clf");
CallPython("plot", x_log.sample_times(),
(x_log.data().row(0).array() - M_PI)
.matrix().transpose());
CallPython("plot", x_log.sample_times(), x_log.data().row(1).transpose());
CallPython("legend", ToPythonTuple("theta1 - PI", "theta2"));
CallPython("axis", "tight");
CallPython("figure", 2);
CallPython("clf");
CallPython("plot", x_log.sample_times(),
(x_log.data().array() - xhat_log.data().array())
.matrix().transpose());
CallPython("ylabel", "error");
CallPython("legend", ToPythonTuple("theta1", "theta2", "theta1dot",
"theta2dot"));
CallPython("axis", "tight");
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::do_main();
}
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/gen/acrobot_input.h | #pragma once
#include "drake/examples/acrobot/acrobot_input.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/gen/spong_controller_params.h | #pragma once
#include "drake/examples/acrobot/spong_controller_params.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/gen/acrobot_params.h | #pragma once
#include "drake/examples/acrobot/acrobot_params.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/gen/acrobot_state.h | #pragma once
#include "drake/examples/acrobot/acrobot_state.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/metrics_test.py | import unittest
import numpy as np
from examples.acrobot.metrics import (
deviation_from_upright_equilibrium,
ensemble_cost,
final_state_cost,
is_success,
success_rate,
)
class TestMetrics(unittest.TestCase):
def setUp(self):
self.x_equilibrium = [np.pi, 0, 0, 0]
self.x_wrapped_equilibrium = [-np.pi, 2 * np.pi, 0, 0]
self.equilibrium_tapes = 2 * [np.zeros([4, 10])]
self.equilibrium_tapes[0][:, -1] = self.x_equilibrium
self.equilibrium_tapes[1][:, -1] = self.x_wrapped_equilibrium
self.nonequilibrium_tapes = 6 * [np.zeros([4, 10])]
self.nonequilibrium_tapes[0][:, -1] = [0, 0, 0, 0]
self.nonequilibrium_tapes[1][:, -1] = [np.pi, np.pi / 2, 0, 0]
self.nonequilibrium_tapes[2][:, -1] = [np.pi, 0, 1, 0]
self.nonequilibrium_tapes[3][:, -1] = [np.pi, 0, 0, 1]
self.nonequilibrium_tapes[4][:, -1] = [-np.pi, -np.pi / 2, -1, -1]
self.nonequilibrium_tapes[5][:, -1] = [-0.1, 0.2, -0.3, 0.4]
def test_deviation_from_upright_equilibrium(self):
# Verify that the deviation is 0 at the upright equilibrium.
self.assertTrue(
np.linalg.norm(
deviation_from_upright_equilibrium(self.x_equilibrium)) == 0)
# Verify that the deviation is 0 if we add or subtract 2 * pi
self.assertTrue(
np.linalg.norm(
deviation_from_upright_equilibrium(self.x_wrapped_equilibrium))
== 0)
# Verify a few other values.
self.assertTrue((deviation_from_upright_equilibrium(
[np.pi, np.pi / 2, 0, 0]) == np.array([0, np.pi / 2, 0, 0])).all())
self.assertTrue((deviation_from_upright_equilibrium(
[np.pi, 0, 1, 0]) == np.array([0, 0, 1, 0])).all())
self.assertTrue((deviation_from_upright_equilibrium(
[np.pi, 0, 0, 1]) == np.array([0, 0, 0, 1])).all())
self.assertTrue(np.allclose(deviation_from_upright_equilibrium(
[3 * np.pi + 0.01, -2 * np.pi - 0.02, 0.03, -0.04]),
np.array([0.01, -0.02, 0.03, -0.04])))
def test_final_state_cost(self):
# Verify that tapes ending at equilibrium have zero cost.
for x_tape in self.equilibrium_tapes:
self.assertTrue(final_state_cost(x_tape) == 0)
self.assertEqual(ensemble_cost(self.equilibrium_tapes), 0)
# Verify that tapes ending at non-equilibrium points have positive
# cost.
for x_tape in self.nonequilibrium_tapes:
self.assertGreater(final_state_cost(x_tape), 0)
self.assertGreater(ensemble_cost(self.nonequilibrium_tapes), 0)
def test_is_success(self):
# Verify that ending at the upright equilibrium yields true.
for x_tape in self.equilibrium_tapes:
self.assertTrue(is_success(x_tape))
# Verify that ending at non-equilibrium points yields false.
for x_tape in self.nonequilibrium_tapes:
self.assertFalse(is_success(x_tape))
def test_success_rate(self):
self.assertEqual(success_rate(self.nonequilibrium_tapes), 0.)
self.assertEqual(
success_rate(self.nonequilibrium_tapes
+ (self.equilibrium_tapes * 3)),
0.5)
self.assertEqual(success_rate(self.equilibrium_tapes), 1.)
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/spong_sim_lib_py_test.py | import unittest
from examples.acrobot.spong_sim import simulate
class TestSpongControlledAcrobot(unittest.TestCase):
def test_simulate(self):
x_tape = simulate(
initial_state=[0.01, 0.02, 0.03, 0.04],
controller_params=[0.001, 0.002, 0.003, 0.004],
t_final=30.0, tape_period=0.05)
# 4 states x 30 seconds of samples at 20 Hz per sample_scenario.
self.assertEqual(x_tape.shape, (4, 601))
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/example_stochastic_scenario.yaml | # An example scenario for acrobot, with randomness.
controller_params: !UniformVector
min: [4, 40, 4, 0.9e3]
max: [6, 60, 6, 1.1e3]
initial_state: !UniformVector
min: [1.1, -0.1, -0.1, -0.1]
max: [1.3, 0.1, 0.1, 0.1]
t_final: 30.0
tape_period: 0.05
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/run_swing_up_traj_optimization.cc | // Generates a swing-up trajectory for acrobot and displays the trajectory
// in DrakeVisualizer. Trajectory generation code is based on
// pendulum_swing_up.cc.
#include <iostream>
#include <limits>
#include <memory>
#include <gflags/gflags.h>
#include "drake/common/is_approx_equal_abstol.h"
#include "drake/examples/acrobot/acrobot_geometry.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/planning/trajectory_optimization/direct_collocation.h"
#include "drake/solvers/snopt_solver.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/controllers/finite_horizon_linear_quadratic_regulator.h"
#include "drake/systems/framework/diagram.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/primitives/trajectory_source.h"
using drake::solvers::SolutionResult;
namespace drake {
namespace examples {
namespace acrobot {
typedef trajectories::PiecewisePolynomial<double> PiecewisePolynomialType;
using planning::trajectory_optimization::DirectCollocation;
namespace {
DEFINE_double(realtime_factor, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
int do_main() {
if (!(solvers::SnoptSolver::is_available() &&
solvers::SnoptSolver::is_enabled())) {
std::cout << "This test was flaky with IPOPT, so currently requires SNOPT."
<< std::endl;
return 0;
}
AcrobotPlant<double> acrobot;
auto context = acrobot.CreateDefaultContext();
const int kNumTimeSamples = 21;
const double kMinimumTimeStep = 0.05;
const double kMaximumTimeStep = 0.2;
DirectCollocation dircol(&acrobot, *context, kNumTimeSamples,
kMinimumTimeStep, kMaximumTimeStep);
auto& prog = dircol.prog();
dircol.AddEqualTimeIntervalsConstraints();
// Current limit for MIT's acrobot is 7-9 Amps, according to Michael Posa.
const double kTorqueLimit = 8;
auto u = dircol.input();
dircol.AddConstraintToAllKnotPoints(-kTorqueLimit <= u(0));
dircol.AddConstraintToAllKnotPoints(u(0) <= kTorqueLimit);
const Eigen::Vector4d x0(0, 0, 0, 0);
const Eigen::Vector4d xG(M_PI, 0, 0, 0);
prog.AddLinearConstraint(dircol.initial_state() == x0);
prog.AddLinearConstraint(dircol.final_state() == xG);
const double R = 10; // Cost on input "effort".
dircol.AddRunningCost((R * u) * u);
const double timespan_init = 4;
auto traj_init_x = PiecewisePolynomialType::FirstOrderHold(
{0, timespan_init}, {x0, xG});
dircol.SetInitialTrajectory(PiecewisePolynomialType(), traj_init_x);
solvers::SnoptSolver solver;
const auto result = solver.Solve(dircol.prog());
if (!result.is_success()) {
std::cerr << "No solution found.\n";
return 1;
}
// Stabilize the trajectory with LQR and simulate.
systems::DiagramBuilder<double> builder;
auto plant = builder.AddSystem<AcrobotPlant>();
auto plant_context = plant->CreateDefaultContext();
systems::controllers::FiniteHorizonLinearQuadraticRegulatorOptions options;
const trajectories::PiecewisePolynomial<double> xtraj =
dircol.ReconstructStateTrajectory(result);
const trajectories::PiecewisePolynomial<double> utraj =
dircol.ReconstructInputTrajectory(result);
options.x0 = &xtraj;
options.u0 = &utraj;
const Eigen::Matrix4d Q = Eigen::Vector4d(10.0, 10.0, 1.0, 1.0).asDiagonal();
options.Qf = Q;
auto regulator = builder.AddSystem(
systems::controllers::MakeFiniteHorizonLinearQuadraticRegulator(
*plant, *plant_context, options.u0->start_time(),
options.u0->end_time(), Q, Vector1d::Constant(1), options));
builder.Connect(regulator->get_output_port(0), plant->get_input_port(0));
builder.Connect(plant->get_output_port(0), regulator->get_input_port(0));
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
AcrobotGeometry::AddToBuilder(&builder, plant->get_output_port(0),
scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
simulator.set_target_realtime_rate(FLAGS_realtime_factor);
simulator.Initialize();
simulator.get_mutable_context().SetTime(options.u0->start_time());
simulator.AdvanceTo(options.u0->end_time());
// Confirm that the stabilized system is in the vicinity of the upright.
DRAKE_DEMAND(is_approx_equal_abstol(
simulator.get_context().get_continuous_state_vector().CopyToVector(), xG,
0.1));
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::acrobot::do_main();
}
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/acrobot_plant_test.cc | #include "drake/examples/acrobot/acrobot_plant.h"
#include <gtest/gtest.h>
#include "drake/common/test_utilities/eigen_matrix_compare.h"
#include "drake/common/test_utilities/expect_no_throw.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
GTEST_TEST(AcrobotPlantTest, ImplicitTimeDerivatives) {
const double kEpsilon = std::numeric_limits<double>::epsilon();
AcrobotPlant<double> plant;
auto context = plant.CreateDefaultContext();
plant.get_input_port(0).FixValue(context.get(), -.32);
context->SetContinuousState(Eigen::Vector4d(.1, .2, .3, .4));
Eigen::VectorXd residual = plant.AllocateImplicitTimeDerivativesResidual();
// Try perfect proposed derivatives -- should get near-zero residual.
const systems::ContinuousState<double>& proposed_derivatives =
plant.EvalTimeDerivatives(*context);
plant.CalcImplicitTimeDerivativesResidual(*context, proposed_derivatives,
&residual);
// Expected accuracy depends on the mass matrix condition number (about 41
// here) and the solve accuracy. We're limited to using Eigen's M.inverse()
// here to permit this to be done symbolically, which is not the most
// accurate method. 100ε (~2e-14) should be achievable.
EXPECT_LT(residual.lpNorm<Eigen::Infinity>(), 100*kEpsilon);
}
// Ensure that DoCalcTimeDerivatives succeeds even if the input port is
// disconnected.
GTEST_TEST(AcrobotPlantTest, NoInput) {
const AcrobotPlant<double> plant;
auto context = plant.CreateDefaultContext();
DRAKE_EXPECT_NO_THROW(plant.EvalTimeDerivatives(*context));
}
GTEST_TEST(AcrobotPlantTest, SetMitAcrobotParameters) {
const AcrobotPlant<double> plant;
auto context = plant.CreateDefaultContext();
auto& parameters = plant.get_mutable_parameters(context.get());
const double m1_old = parameters.m1();
plant.SetMitAcrobotParameters(¶meters);
const double m1_mit = parameters.m1();
EXPECT_NE(m1_old, m1_mit);
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/example_scenario.yaml | # An example scenario for acrobot.
controller_params: [5, 50, 5, 1e3]
initial_state: [1.2, 0, 0, 0]
t_final: 30.0
tape_period: 0.05
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/multibody_dynamics_test.cc | #include <memory>
#include <gtest/gtest.h>
#include "drake/common/test_utilities/eigen_matrix_compare.h"
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/multibody/parsing/parser.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
// Tests that the hand-derived dynamics (from the textbook) match the dynamics
// generated from the urdf and sdf files via the MultibodyPlant class.
GTEST_TEST(MultibodyDynamicsTest, AllTests) {
for (const char* const ext : {"urdf", "sdf"}) {
const double kTimeStep = 0.0;
multibody::MultibodyPlant<double> mbp(kTimeStep);
multibody::Parser(&mbp).AddModelsFromUrl(
fmt::format("package://drake/examples/acrobot/Acrobot.{}", ext));
mbp.Finalize();
AcrobotPlant<double> p;
auto context_mbp = mbp.CreateDefaultContext();
auto context_p = p.CreateDefaultContext();
auto& u_mbp = mbp.get_actuation_input_port().FixValue(context_mbp.get(),
0.0);
auto& u_p = p.get_input_port(0).FixValue(context_p.get(), 0.0);
Eigen::Vector4d x;
Vector1d u;
auto xdot_mbp = mbp.AllocateTimeDerivatives();
auto xdot_p = p.AllocateTimeDerivatives();
auto y_mbp = mbp.AllocateOutput();
auto y_p = p.AllocateOutput();
srand(42);
for (int i = 0; i < 100; ++i) {
x = Eigen::Vector4d::Random();
u = Vector1d::Random();
context_mbp->get_mutable_continuous_state_vector().SetFromVector(x);
context_p->get_mutable_continuous_state_vector().SetFromVector(x);
u_mbp.GetMutableVectorData<double>()->SetFromVector(u);
u_p.GetMutableVectorData<double>()->SetFromVector(u);
mbp.CalcTimeDerivatives(*context_mbp, xdot_mbp.get());
p.CalcTimeDerivatives(*context_p, xdot_p.get());
EXPECT_TRUE(CompareMatrices(xdot_mbp->CopyToVector(),
xdot_p->CopyToVector(), 1e-8,
MatrixCompareType::absolute));
mbp.CalcOutput(*context_mbp, y_mbp.get());
p.CalcOutput(*context_p, y_p.get());
EXPECT_TRUE(CompareMatrices(
y_mbp->get_vector_data(mbp.get_state_output_port().get_index())
->CopyToVector(),
y_p->get_vector_data(0)->CopyToVector(), 1e-8,
MatrixCompareType::absolute));
}
}
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/acrobot_lcm_msg_generator.cc | // Generates simple LCM messages to test acrobot_plant and controllers.
#include <memory>
#include <string>
#include <thread>
#include <vector>
#include "drake/lcm/drake_lcm.h"
#include "drake/lcmt_acrobot_u.hpp"
#include "drake/lcmt_acrobot_x.hpp"
#include "drake/systems/analysis/simulator.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
using std::chrono::milliseconds;
using std::this_thread::sleep_for;
int DoMain() {
drake::lcm::DrakeLcm lcm;
const std::string channel_x = "acrobot_xhat";
const std::string channel_u = "acrobot_u";
// Decode channel_x into msg_x.
drake::lcm::Subscriber<lcmt_acrobot_x> subscription(&lcm, channel_x);
const lcmt_acrobot_x& msg_x = subscription.message();
for (int i = 0; i < 1e5; ++i) {
// Publishes a dummy msg_x.
{
lcmt_acrobot_x dummy{};
dummy.theta1 = i * M_PI / 6;
dummy.theta2 = i * M_PI / 6;
dummy.theta1Dot = std::sin(dummy.theta1);
dummy.theta2Dot = std::cos(dummy.theta2);
Publish(&lcm, channel_x, dummy);
}
// Publishes msg_u using received msg_x.
lcm.HandleSubscriptions(0 /* timeout */);
if (msg_x.timestamp > 0) {
// Calculates some output from received state.
lcmt_acrobot_u msg_u{};
msg_u.tau = msg_x.theta1 + msg_x.theta2;
Publish(&lcm, channel_u, msg_u);
}
sleep_for(milliseconds(500));
}
return 0;
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
int main() { return drake::examples::acrobot::DoMain(); }
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/acrobot_io_test.py | import numpy as np
import unittest
from pydrake.common import FindResourceOrThrow
from examples.acrobot.acrobot_io import (
load_scenario, save_scenario,
load_output, save_output)
class TestIo(unittest.TestCase):
def setUp(self):
self.maxDiff = None
self.example = FindResourceOrThrow(
"drake/examples/acrobot/test/example_scenario.yaml")
# When saving, everything comes out as floats (not `int`, etc.).
self.expected_save = """\
controller_params: [5.0, 50.0, 5.0, 1000.0]
initial_state: [1.2, 0.0, 0.0, 0.0]
t_final: 30.0
tape_period: 0.05
"""
def test_load_scenario(self):
scenario = load_scenario(filename=self.example)
expected = ", ".join([
"{'controller_params': [5, 50, 5, '1e3']",
"'initial_state': [1.2, 0, 0, 0]",
"'t_final': 30.0",
"'tape_period': 0.05}"])
self.assertEqual(str(scenario), expected)
def test_save_scenario(self):
scenario = load_scenario(filename=self.example)
actual = save_scenario(scenario=scenario)
self.assertEqual(actual, self.expected_save)
def test_save_scenario_numpy(self):
scenario = {
"controller_params": np.array([5, 50, 5, 1e3]),
"initial_state": np.array([1.2, 0, 0, 0]),
"t_final": 30.0,
"tape_period": 0.05,
}
actual = save_scenario(scenario=scenario)
self.assertEqual(actual, self.expected_save)
def test_save_scenario_stochastic(self):
scenario = {
"controller_params": {
"max": np.array([5, 50, 5, 1e3]),
"min": np.array([1, 10, 1, 1e2]),
},
"initial_state": np.array([1.2, 0, 0, 0]),
"t_final": 30.0,
"tape_period": 0.05,
}
actual = save_scenario(scenario=scenario)
self.assertEqual(actual, """\
controller_params:
max: [5.0, 50.0, 5.0, 1000.0]
min: [1.0, 10.0, 1.0, 100.0]
initial_state: [1.2, 0.0, 0.0, 0.0]
t_final: 30.0
tape_period: 0.05
""")
def test_save_output_and_load_output(self):
values = [
[0, 1, 2, 3, 4, 5, 6, 7],
[10, 11, 12, 13, 14, 15, 16, 17],
[20, 21, 22, 23, 24, 25, 26, 27],
[30, 31, 32, 33, 34, 35, 36, 37],
]
x_tape = np.array(values)
actual = save_output(x_tape=x_tape)
expected = """x_tape:
- [0, 1, 2, 3, 4, 5, 6, 7]
- [10, 11, 12, 13, 14, 15, 16, 17]
- [20, 21, 22, 23, 24, 25, 26, 27]
- [30, 31, 32, 33, 34, 35, 36, 37]
"""
self.assertEqual(actual, expected)
readback = load_output(data=expected)
self.assertEqual(x_tape.tolist(), values)
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/spong_sim_main_test.py | import os
import subprocess
import sys
import unittest
from pydrake.common import FindResourceOrThrow
from examples.acrobot.acrobot_io import load_output, load_scenario
_backend = "py"
class TestRunSpongControlledAcrobot(unittest.TestCase):
def setUp(self):
self.dut = FindResourceOrThrow(
f"drake/examples/acrobot/spong_sim_main_{_backend}")
# 4 states x 30 seconds of samples at 20 Hz per sample_scenario.
self.nominal_x_tape_shape = (4, 601)
def test_help(self):
result = subprocess.run(
[self.dut, "--help"],
stdout=subprocess.PIPE,
stderr=subprocess.PIPE,
encoding="utf-8")
self.assertEqual(result.stderr, "")
self.assertIn("spong-controlled acrobot", result.stdout)
self.assertEqual(result.returncode, 0 if _backend == "py" else 1)
def test_example_scenario(self):
scenario = FindResourceOrThrow(
"drake/examples/acrobot/test/example_scenario.yaml")
output = os.path.join(os.environ["TEST_TMPDIR"], "output.yaml")
subprocess.check_call([
self.dut, "--scenario", scenario, "--output", output])
x_tape = load_output(filename=output)
self.assertEqual(x_tape.shape, self.nominal_x_tape_shape)
def test_stochastic_scenario(self):
if _backend == "py":
return # Python backend does not support stochastic scenarios.
scenario = FindResourceOrThrow(
"drake/examples/acrobot/test/example_stochastic_scenario.yaml")
output = os.path.join(os.environ["TEST_TMPDIR"], "output.yaml")
dump_file = os.path.join(os.environ["TEST_TMPDIR"],
"scenario_out.yaml")
subprocess.check_call([
self.dut, "--scenario", scenario, "--output", output,
"--dump_scenario", dump_file])
x_tape = load_output(filename=output)
# 4 states x 30 seconds of samples at 20 Hz per sample_scenario.
self.assertEqual(x_tape.shape, (4, 601))
# Load the scenario dump; don't bother checking exact distribution
# semantics; just check that we have the right data shape and type.
dump = load_scenario(filename=dump_file)
self.assertEqual(len(dump["controller_params"]), 4)
self.assertTrue(all(type(x) is float
for x in dump["controller_params"]))
self.assertEqual(len(dump["initial_state"]), 4)
self.assertTrue(all(type(x) is float
for x in dump["initial_state"]))
if __name__ == "__main__":
if "--cc" in sys.argv:
sys.argv.remove("--cc")
_backend = "cc"
unittest.main()
| 0 |
/home/johnshepherd/drake/examples/acrobot | /home/johnshepherd/drake/examples/acrobot/test/acrobot_geometry_test.cc | #include "drake/examples/acrobot/acrobot_geometry.h"
#include <gtest/gtest.h>
#include "drake/examples/acrobot/acrobot_plant.h"
#include "drake/geometry/scene_graph.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace acrobot {
namespace {
GTEST_TEST(AcrobotGeometryTest, AcceptanceTest) {
// Just make sure nothing faults out.
systems::DiagramBuilder<double> builder;
auto plant = builder.AddSystem<AcrobotPlant>();
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
auto geom = AcrobotGeometry::AddToBuilder(
&builder, plant->get_output_port(0), scene_graph);
auto diagram = builder.Build();
ASSERT_NE(geom, nullptr);
}
} // namespace
} // namespace acrobot
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/bead_on_a_wire/bead_on_a_wire.h | #pragma once
#include <Eigen/Core>
#include <unsupported/Eigen/AutoDiff>
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace bead_on_a_wire {
/// Dynamical system of a point (unit) mass constrained to lie on a wire. The
/// system is currently frictionless. The equation for the wire can be provided
/// parametrically by the user. Dynamics equations can be computed in both
/// minimal coordinates or absolute coordinates (with Lagrange Multipliers).
///
/// The presence of readily available solutions coupled with the potential
/// for highly irregular geometric constraints (which can be viewed
/// as complex contact constraints), can make this a challenging problem
/// to simulate.
///
/// The dynamic equations for the bead in minimal coordinates comes from
/// Lagrangian Dynamics of the "second kind": forming the Lagrangian and
/// using the Euler-Lagrange equation. The potential energy (V) of the bead with
/// respect to the parametric function f(s) : ℝ → ℝ³ is:
/// <pre>
/// -f₃(s)⋅ag
/// </pre>
/// where `ag` is the magnitude (i.e., non-negative value) of the acceleration
/// due to gravity. The velocity of the bead is df/ds⋅ṡ, and the kinetic
/// energy of the bead (T) is therefore 1/2⋅(df/ds⋅ṡ)².
///
/// The Lagrangian is then defined as:
/// <pre>
/// ℒ = T - V = 1/2⋅(df/ds)²⋅ṡ² + f₃(s)⋅ag
/// </pre>
/// And Lagrangian Dynamics specifies that the dynamics for the system are
/// defined by:
/// <pre>
/// ∂ℒ/∂s - d/dt ∂ℒ/∂ṡ = τ̇⋅
/// </pre>
/// where τ is the generalized force on the system. Thus:
/// <pre>
/// df(s)/ds⋅ṡ²⋅d²f/ds² + (df(s)/ds)₃⋅ag - (df/ds)²⋅dṡ/dt = τ
/// </pre>
/// The dynamic equations for the bead in absolute coordinates comes from the
/// Lagrangian Dynamics of the "first kind" (i.e., by formulating the problem
/// as an Index-3 DAE):
/// <pre>
/// d²x/dt² = fg + fext + Jᵀλ
/// g(x) = 0
/// </pre>
/// where `x` is the three dimensional position of the bead, `fg` is a three
/// dimensional gravitational acceleration vector, `fext` is a three dimensional
/// vector of "external" (user applied) forces acting on the bead, and J ≡ ∂g/∂x
/// is the Jacobian matrix of the positional constraints (computed with respect
/// to the bead location). We define `g(x) : ℝ³ → ℝ³` as the following function:
/// <pre>
/// g(x) = f(f⁻¹(x)) - x
/// </pre>
/// where f⁻¹ : ℝ³ → ℝ maps points in Cartesian space to wire parameters (this
/// class expects that this inverse function may be ill-defined).
/// g(x) = 0 will only be satisfied if x corresponds to a point on the wire.
///
/// Inputs: One input (generalized external force) for the bead on a wire
/// simulated in minimal coordinates, three inputs (three dimensional
/// external force applied to the center of mass) for the bead on a
/// wire simulated in absolute coordinates.
///
/// States: Two state variables (arc length of the bead along the wire and the
/// velocity of the bead along the wire) for the bead simulated in
/// minimal coordinates; 3D position (state indices 0,1, and 2), and
/// 3D linear velocity (state indices 3, 4, and 5) in units of m and
/// m/s, respectively, for the bead simulated in absolute coordinates.
///
/// Outputs: same as state.
///
/// @system
/// name: BeadOnAWire
/// input_ports:
/// - u0
/// output_ports:
/// - y0
/// @endsystem
///
/// @tparam_double_only
template <typename T>
class BeadOnAWire : public systems::LeafSystem<T> {
public:
/// AutoDiff scalar used for constraint function automatic differentiation.
/// The constraint function maps from an arc length (s) to a point in
/// three-dimensional Cartesian space. This type represents the input
/// variable s and- on return from that constraint function- would hold the
/// first derivative of the constraint function computed with respect to s.
typedef Eigen::AutoDiffScalar<drake::Vector1d> AScalar;
/// AutoDiff scalar used for computing the second derivative of the constraint
/// function using automatic differentiation. The constraint function maps
/// from an arc length (s) to a point in three-dimensional Cartesian space.
/// This type represents the input variable s and- on return from that
/// constraint function- would hold the second derivative of the constraint
/// function computed with respect to s.
typedef Eigen::AutoDiffScalar<Eigen::Matrix<AScalar, 1, 1>> ArcLength;
/// The type of coordinate representation to use for kinematics and dynamics.
enum CoordinateType {
/// Coordinate representation will be wire parameter.
kMinimalCoordinates,
/// Coordinate representation will be the bead location (in 3D).
kAbsoluteCoordinates
};
/// Constructs the object using either minimal or absolute coordinates (the
/// latter uses the method of Lagrange Multipliers to compute the time
/// derivatives).
explicit BeadOnAWire(CoordinateType type);
/// Sets the acceleration (with respect to the positive y-axis) due to
/// gravity (i.e., this number should generally be negative).
void set_gravitational_acceleration(double g) { g_ = g; }
/// Gets the acceleration (with respect to the positive y-axis) due to
/// gravity (i.e., this number should generally be negative).
double get_gravitational_acceleration() const { return g_; }
/// Allows the user to reset the wire parameter function and its inverse
/// (which points to helix_function and inverse_helix_function by
/// default.
/// @param f The pointer to a function that takes a wire parameter
/// (scalar `s`) as input and outputs a point in 3D as output.
/// @param inv_f The pointer to a function that takes a point in 3D as input
/// and outputs a floating point scalar as output.
/// @throws std::exception if f or inv_f is a nullptr (the functions must
/// always be set).
void reset_wire_parameter_functions(
std::function<Eigen::Matrix<ArcLength, 3, 1>(const ArcLength&)> f,
std::function<ArcLength(const Eigen::Matrix<ArcLength, 3, 1>&)> inv_f) {
if (!f || !inv_f) throw std::logic_error("Function must be non-null.");
f_ = f;
inv_f_ = inv_f;
}
/// Example wire parametric function for the bead on a wire example. The
/// exact function definition is:
/// <pre>
/// | cos(s) |
/// f(s) = | sin(s) |
/// | s |
/// </pre>
static Eigen::Matrix<ArcLength, 3, 1> helix_function(const ArcLength &s);
/// Inverse parametric function for the bead on a wire system that uses the
/// helix parametric example function.
static ArcLength inverse_helix_function(const Vector3<ArcLength> &v);
/// Gets the output from the parametric function in Vector3d form.
/// @param m the output from the parametric wire function.
static Eigen::Vector3d get_pfunction_output(
const Eigen::Matrix<ArcLength, 3, 1>& m);
/// Gets the first derivative from the parametric function, in Vector3d form,
/// using the output from that parametric function.
/// @param m the output from the parametric wire function.
static Eigen::Vector3d get_pfunction_first_derivative(
const Eigen::Matrix<ArcLength, 3, 1>& m);
/// Gets the second derivative from the parametric function, in Vector3d form,
/// using the output from that parametric function.
/// @param m the output from the parametric wire function.
static Eigen::Vector3d get_pfunction_second_derivative(
const Eigen::Matrix<ArcLength, 3, 1>& m);
/// Gets the output from the inverse parametric function as a double, using
/// the output from that inverse parametric function.
/// @param m the output from the inverse parametric wire function.
static double get_inv_pfunction_output(const ArcLength& m);
/// Gets the first derivative from the inverse parametric function as a
/// double, using the output from that inverse parametric function.
/// @param m the output from the inverse parametric wire function.
static double get_inv_pfunction_first_derivative(const ArcLength& m);
/// Gets the second derivative from the inverse parametric function as a
/// double, using the output from that inverse parametric function.
/// @param m the output from the inverse parametric wire function.
static double get_inv_pfunction_second_derivative(const ArcLength& m);
/// Gets the number of constraint equations used for dynamics.
int get_num_constraint_equations(const systems::Context<T>& context) const;
/// Evaluates the constraint equations for a bead represented in absolute
/// coordinates (no constraint equations are used for a bead represented in
/// minimal coordinates).
Eigen::VectorXd EvalConstraintEquations(
const systems::Context<T>& context) const;
/// Computes the time derivative of the constraint equations, evaluated at
/// the current generalized coordinates and generalized velocity.
Eigen::VectorXd EvalConstraintEquationsDot(
const systems::Context<T>& context) const;
/// Computes the change in generalized velocity from applying constraint
/// impulses @p lambda.
/// @param context The current state of the system.
/// @param lambda The vector of constraint forces.
/// @returns a `n` dimensional vector, where `n` is the dimension of the
/// quasi-coordinates.
Eigen::VectorXd CalcVelocityChangeFromConstraintImpulses(
const systems::Context<T>& context, const Eigen::MatrixXd& J,
const Eigen::VectorXd& lambda) const;
protected:
void DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const override;
void SetDefaultState(const systems::Context<T>& context,
systems::State<T>* state) const override;
private:
// The coordinate representation used for kinematics and dynamics.
CoordinateType coordinate_type_;
// The wire parameter function. See set_wire_parameter_function() for more
// information. This pointer is expected to never be null.
std::function<Eigen::Matrix<ArcLength, 3, 1>(const ArcLength&)> f_{
&helix_function};
// The inverse of the wire parameter function.
// This function takes a point in 3D as input and outputs a scalar
// in ℝ as output. Constraint stabilization methods for DAEs can use this
// function (via EvaluateConstraintEquations()) to efficiently project the
// bead represented in absolute coordinates back onto the wire. If this
// function is null, EvaluateConstraintEquations() will use generic,
// presumably less efficient methods instead. This pointer is expected to
// never be null.
std::function<ArcLength(const Eigen::Matrix<ArcLength, 3, 1>&)> inv_f_{
&inverse_helix_function};
// Signed acceleration due to gravity.
double g_{-9.81};
};
} // namespace bead_on_a_wire
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/bead_on_a_wire/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_googletest",
"drake_cc_library",
)
package(default_visibility = ["//visibility:private"])
drake_cc_library(
name = "bead_on_a_wire",
srcs = ["bead_on_a_wire.cc"],
hdrs = [
"bead_on_a_wire.h",
],
deps = [
"//common:essential",
"//systems/framework:leaf_system",
],
)
# === test/ ===
drake_cc_googletest(
name = "bead_on_a_wire_test",
deps = [
":bead_on_a_wire",
"//systems/analysis:simulator",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/bead_on_a_wire/bead_on_a_wire.cc | #include "drake/examples/bead_on_a_wire/bead_on_a_wire.h"
#include <limits>
#include "drake/common/drake_assert.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace bead_on_a_wire {
template <typename T>
BeadOnAWire<T>::BeadOnAWire(BeadOnAWire<T>::CoordinateType type)
: coordinate_type_(type) {
const int n = (type == BeadOnAWire<T>::kMinimalCoordinates) ? 1 : 3;
auto state_index = this->DeclareContinuousState(n, n, 0);
this->DeclareInputPort(systems::kUseDefaultName, systems::kVectorValued, n);
this->DeclareStateOutputPort(systems::kUseDefaultName, state_index);
}
template <class T>
Eigen::Matrix<typename BeadOnAWire<T>::ArcLength, 3, 1>
BeadOnAWire<T>::helix_function(
const typename BeadOnAWire<T>::ArcLength& s) {
using std::cos;
using std::sin;
return Vector3<BeadOnAWire<T>::ArcLength>(cos(s),
sin(s),
s);
}
template <class T>
typename BeadOnAWire<T>::ArcLength
BeadOnAWire<T>::inverse_helix_function(
const Vector3<typename BeadOnAWire<T>::ArcLength>& v) {
using std::atan2;
return atan2(v(1), v(0));
}
template <class T>
Eigen::VectorXd BeadOnAWire<T>::EvalConstraintEquations(
const systems::Context<T>& context) const {
// Return a zero vector if this system is in minimal coordinates.
if (coordinate_type_ == BeadOnAWire<T>::kMinimalCoordinates)
return Eigen::VectorXd(0);
// The constraint function is defined as:
// g(x) = f(f⁻¹(x)) - x
// where x is the position of the bead and f() is the parametric wire
// function.
// Get the position of the bead.
constexpr int three_d = 3;
Eigen::Matrix<ArcLength, three_d, 1> x;
const auto position = context.get_continuous_state().
get_generalized_position().CopyToVector();
for (int i = 0; i < three_d; ++i)
x(i).value() = position[i];
// Call the inverse function.
ArcLength s = inv_f_(x);
// Get the output position.
Eigen::Matrix<ArcLength, three_d, 1> fs = f_(s);
Eigen::VectorXd xprime(three_d);
for (int i = 0; i < three_d; ++i)
xprime[i] = fs(i).value().value() - x(i).value().value();
return xprime;
}
template <class T>
Eigen::VectorXd BeadOnAWire<T>::EvalConstraintEquationsDot(
const systems::Context<T>& context) const {
constexpr int three_d = 3;
// Return a zero vector if this system is in minimal coordinates.
if (coordinate_type_ == BeadOnAWire<T>::kMinimalCoordinates)
return Eigen::VectorXd(0);
// The constraint function is defined as:
// g(x) = f(f⁻¹(x)) - x
// where x is the position of the bead and f() is the parametric wire
// function. Therefore:
// dg/dt(x) = df/dt (f⁻¹(x)) ⋅ df⁻¹/dt (x) - v
// For example, assume that f(s) = | cos(s) sin(s) s | and that
// f⁻¹(x) = atan2(x(2),x(1)).
//
// Then dg/dt = | -sin(atan2(x(2),x(1))) cos(atan2(x(2),x(1))) 1 | ⋅
// d/dt atan2(x(2),x(1)) - | v(1) v(2) v(3) |
// Compute df/dt (f⁻¹(x)). The result will be a vector.
const auto& xc = context.get_continuous_state().get_vector();
Eigen::Matrix<BeadOnAWire<T>::ArcLength, three_d, 1> x;
x(0).value() = xc.GetAtIndex(0);
x(1).value() = xc.GetAtIndex(1);
x(2).value() = xc.GetAtIndex(2);
BeadOnAWire<T>::ArcLength sprime = inv_f_(x);
sprime.derivatives()(0) = 1;
const Eigen::Matrix<BeadOnAWire<T>::ArcLength, three_d, 1> fprime =
f_(sprime);
// Compute df⁻¹/dt (x). This result will be a scalar.
Eigen::Matrix<BeadOnAWire<T>::ArcLength, three_d, 1> xprime;
xprime(0).value() = xc.GetAtIndex(0);
xprime(1).value() = xc.GetAtIndex(1);
xprime(2).value() = xc.GetAtIndex(2);
xprime(0).value().derivatives()(0) = xc.GetAtIndex(3);
xprime(1).value().derivatives()(0) = xc.GetAtIndex(4);
xprime(2).value().derivatives()(0) = xc.GetAtIndex(5);
const double dinvf_dt = inv_f_(xprime).value().derivatives()(0);
// Set the velocity vector.
const Eigen::Vector3d v(xc.GetAtIndex(3), xc.GetAtIndex(4),
xc.GetAtIndex(5));
// Compute the result.
Eigen::VectorXd result = get_pfunction_first_derivative(fprime)*dinvf_dt - v;
return result;
}
template <class T>
int BeadOnAWire<T>::get_num_constraint_equations(
const systems::Context<T>&) const {
return (coordinate_type_ == kAbsoluteCoordinates) ? 3 : 0;
}
template <class T>
Eigen::VectorXd BeadOnAWire<T>::CalcVelocityChangeFromConstraintImpulses(
const systems::Context<T>&, const Eigen::MatrixXd& J,
const Eigen::VectorXd& lambda) const {
// TODO(edrumwri): Test this method as soon as DAE solver is available,
// (necessarily removing abort() first).
if (true) {
throw std::logic_error(
"CalcVelocityChangeFromConstraintImpulses requires testing.");
}
// The bead on the wire is unit mass, so the velocity change is equal to
// simply Jᵀλ
if (coordinate_type_ == kAbsoluteCoordinates)
return J.transpose() * lambda;
else
return Eigen::Matrix<T, 1, 1>(0);
}
template <class T>
double BeadOnAWire<T>::get_inv_pfunction_output(const ArcLength& m) {
return m.value().value();
}
template <class T>
double BeadOnAWire<T>::get_inv_pfunction_first_derivative(const ArcLength& m) {
return m.value().derivatives()(0);
}
template <class T>
double BeadOnAWire<T>::get_inv_pfunction_second_derivative(const ArcLength& m) {
return m.derivatives()(0).derivatives()(0);
}
template <class T>
Eigen::Vector3d BeadOnAWire<T>::get_pfunction_output(
const Eigen::Matrix<ArcLength, 3, 1>& m) {
const double x = m(0).value().value();
const double y = m(1).value().value();
const double z = m(2).value().value();
return Eigen::Vector3d(x, y, z);
}
template <class T>
Eigen::Vector3d BeadOnAWire<T>::get_pfunction_first_derivative(
const Eigen::Matrix<ArcLength, 3, 1>& m) {
const double x = m(0).derivatives()(0).value();
const double y = m(1).derivatives()(0).value();
const double z = m(2).derivatives()(0).value();
return Eigen::Vector3d(x, y, z);
}
template <class T>
Eigen::Vector3d BeadOnAWire<T>::get_pfunction_second_derivative(
const Eigen::Matrix<ArcLength, 3, 1>& m) {
const double x = m(0).derivatives()(0).derivatives()(0);
const double y = m(1).derivatives()(0).derivatives()(0);
const double z = m(2).derivatives()(0).derivatives()(0);
return Eigen::Vector3d(x, y, z);
}
template <typename T>
void BeadOnAWire<T>::DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const {
using std::sin;
using std::cos;
using std::abs;
const systems::VectorBase<T>& state = context.get_continuous_state_vector();
// Obtain the structure we need to write into.
DRAKE_ASSERT(derivatives != nullptr);
systems::VectorBase<T>& f = derivatives->get_mutable_vector();
// Get the inputs.
const VectorX<T>& input = this->get_input_port(0).Eval(context);
// Compute the derivatives using the desired coordinate representation.
if (coordinate_type_ == kMinimalCoordinates) {
// Get the necessary parts of the state.
const T s = state.GetAtIndex(0);
const T s_dot = state.GetAtIndex(1);
// Get the external force.
const double tau = input(0);
// Compute derivatives.
ArcLength s_in;
s_in.value().value() = s;
s_in.derivatives()(0) = 1;
s_in.value().derivatives()(0) = 1;
Eigen::Matrix<ArcLength, 3, 1> foutput = f_(s_in);
// From the description in the header file, the dynamics of the bead in
// minimal coordinates is:
// df/ds⋅ṡ²⋅d²f/ds² + (df/ds)₃⋅ag - (df/ds)²⋅dṡ/dt = τ
// Implying that:
// dṡ/dt = (-τ + (df(s)/ds)₃⋅ag - df(s)/ds⋅ṡ²⋅d²f/ds²) / (df/ds)²
// This derivation was double-checked with the following Mathematica code:
// L:= 1/2*(f'[s[t]] * s'[t])^2 + Dot[z, f[s[t]]]*ag
// Solve[D[L, s[t]] - D[D[L, s'[t]], t] == tau, s''[t]]
// where L is the definition of the Lagrangian and Dot[z, f[s[t]]] is
// equivalent to f₃ (i.e., assuming that z is the unit vector [0 0 1]ᵀ).
const double ag = get_gravitational_acceleration();
const Eigen::Vector3d dfds = get_pfunction_first_derivative(foutput);
const Eigen::Vector3d d2fds2 = get_pfunction_second_derivative(foutput);
const double s_ddot = (tau + dfds(2)*ag - dfds.dot(d2fds2)*s_dot*s_dot) /
dfds.dot(dfds);
// Set derivative.
f.SetAtIndex(0, s_dot);
f.SetAtIndex(1, s_ddot);
} else {
// Compute acceleration from unconstrained Newtonian dynamics.
const T xdot = state.GetAtIndex(3);
const T ydot = state.GetAtIndex(4);
const T zdot = state.GetAtIndex(5);
// Get the external force.
const Vector3<T> fext = input.segment(0, 3);
// Set velocity components of derivative.
f.SetAtIndex(0, xdot);
f.SetAtIndex(1, ydot);
f.SetAtIndex(2, zdot);
f.SetAtIndex(3, fext(0));
f.SetAtIndex(4, fext(1));
f.SetAtIndex(5, fext(2) + get_gravitational_acceleration());
}
}
/// Sets the default state for the bead-on-a-wire system to `s = 0, ds/dt = 0`
/// for the bead represented in minimal coordinates; for the bead represented
/// in absolute coordinates, the default state is set to `f(0), ds/dt(0)⋅0 = 0`.
template <typename T>
void BeadOnAWire<T>::SetDefaultState(const systems::Context<T>&,
systems::State<T>* state) const {
// Use a consistent default state for the helix bead-on-the-wire
// example.
const double s = 1.0, s_dot = 1.0;
VectorX<T> x0;
if (coordinate_type_ == BeadOnAWire<T>::kAbsoluteCoordinates) {
const int state_size = 6;
// Evaluate the wire parameter function at s.
ArcLength sprime;
sprime.value() = s;
sprime.derivatives()(0).value() = 1;
const Eigen::Matrix<ArcLength, 3, 1> q = f_(sprime);
// Set x0 appropriately.
x0.resize(state_size);
x0 << q(0).value().value(),
q(1).value().value(),
q(2).value().value(),
q(0).derivatives()(0).value() * s_dot,
q(1).derivatives()(0).value() * s_dot,
q(2).derivatives()(0).value() * s_dot;
} else {
const int state_size = 2;
x0.resize(state_size);
x0 << s, s_dot;
}
state->get_mutable_continuous_state().SetFromVector(x0);
}
template class BeadOnAWire<double>;
} // namespace bead_on_a_wire
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/bead_on_a_wire | /home/johnshepherd/drake/examples/bead_on_a_wire/test/bead_on_a_wire_test.cc | #include "drake/examples/bead_on_a_wire/bead_on_a_wire.h"
#include <memory>
#include <gtest/gtest.h>
namespace drake {
namespace examples {
namespace bead_on_a_wire {
namespace {
class BeadOnAWireTest : public ::testing::Test {
protected:
void SetUp() override {
// Construct beads on the wire in absolute and minimal coordinates.
dut_abs_ = std::make_unique<BeadOnAWire<double>>(
BeadOnAWire<double>::kAbsoluteCoordinates);
dut_min_ = std::make_unique<BeadOnAWire<double>>(
BeadOnAWire<double>::kMinimalCoordinates);
// Construct contexts.
context_abs_ = dut_abs_->CreateDefaultContext();
context_min_ = dut_min_->CreateDefaultContext();
// Construct outputs.
output_abs_ = dut_abs_->AllocateOutput();
output_min_ = dut_min_->AllocateOutput();
// Allocate storage for derivatives.
derivatives_abs_ = dut_abs_->AllocateTimeDerivatives();
derivatives_min_ = dut_min_->AllocateTimeDerivatives();
// Fix unused input ports.
const Vector3<double> input_abs(0.0, 0.0, 0.0);
const double input_min = 0.0;
dut_abs_->get_input_port(0).FixValue(context_abs_.get(), input_abs);
dut_min_->get_input_port(0).FixValue(context_min_.get(), input_min);
}
std::unique_ptr<BeadOnAWire<double>> dut_abs_; //< A device under test.
std::unique_ptr<BeadOnAWire<double>> dut_min_; //< A device under test.
std::unique_ptr<systems::Context<double>> context_abs_, context_min_;
std::unique_ptr<systems::SystemOutput<double>> output_abs_, output_min_;
std::unique_ptr<systems::ContinuousState<double>> derivatives_abs_,
derivatives_min_;
};
// Checks output is as expected.
TEST_F(BeadOnAWireTest, Output) {
const systems::ContinuousState<double>& v = context_abs_->
get_continuous_state();
dut_abs_->CalcOutput(*context_abs_, output_abs_.get());
for (int i = 0; i < v.size(); ++i)
EXPECT_EQ(v[i], output_abs_->get_vector_data(0)->value()(i));
}
// Tests parameter getting and setting.
TEST_F(BeadOnAWireTest, Parameters) {
// Set parameters to non-default values.
const double g = -1.0;
dut_abs_->set_gravitational_acceleration(g);
EXPECT_EQ(dut_abs_->get_gravitational_acceleration(), g);
}
// Tests that helix parameter function produces the values and
// derivatives we expect.
TEST_F(BeadOnAWireTest, Helix) {
// Set small tolerance value.
const double tol = std::numeric_limits<double>::epsilon() * 10.0;
// Compute the value at pi/3.
BeadOnAWire<double>::ArcLength s;
s = M_PI / 3.0;
auto v = dut_abs_->get_pfunction_output(dut_abs_->helix_function(s));
const double svalue = s.value().value();
EXPECT_NEAR(v(0), std::cos(svalue), tol);
EXPECT_NEAR(v(1), std::sin(svalue), tol);
EXPECT_NEAR(v(2), svalue, tol);
// Compute the derivative at pi/3.
s.derivatives()(0) = 1.0;
s.value().derivatives()(0) = 1.0;
const auto w = dut_abs_->get_pfunction_first_derivative(
dut_abs_->helix_function(s));
EXPECT_NEAR(w(0), -std::sin(svalue), tol);
EXPECT_NEAR(w(1), std::cos(svalue), tol);
EXPECT_NEAR(w(2), 1.0, tol);
// Compute the second derivative at pi/3.
const auto w2 = dut_abs_->get_pfunction_second_derivative(
dut_abs_->helix_function(s));
EXPECT_NEAR(w2(0), -std::cos(svalue), tol);
EXPECT_NEAR(w2(1), -std::sin(svalue), tol);
EXPECT_NEAR(w2(2), 0.0, tol);
}
// Tests that the inverse of the helix parameter function produces the
// values and derivatives we expect.
TEST_F(BeadOnAWireTest, InverseHelix) {
// Set small tolerance value.
const double tol = std::numeric_limits<double>::epsilon() * 10.0;
// Compute the value at pi/3.
const double test_value = M_PI / 3.0;
BeadOnAWire<double>::ArcLength s;
s = test_value;
auto v = dut_abs_->helix_function(s);
auto sprime = dut_abs_->inverse_helix_function(v);
EXPECT_NEAR(dut_abs_->get_inv_pfunction_output(s),
dut_abs_->get_inv_pfunction_output(sprime), tol);
// Compute the derivative of the inverse helix function.
s.derivatives()(0) = 1.0;
s.value().derivatives()(0) = 1.0;
auto wprime = dut_abs_->inverse_helix_function(dut_abs_->
helix_function(s));
const double candidate_value = dut_abs_->get_inv_pfunction_first_derivative(
wprime);
// Apply numerical differentiation to the inverse helix function. We
// do this by computing the output:
// f⁻¹(f(s+ds)) - f⁻¹(f(s))
// ------------------------
// ds
const double ds = std::numeric_limits<double>::epsilon();
double fprime = dut_abs_->get_inv_pfunction_output(
dut_abs_->inverse_helix_function(dut_abs_->helix_function(s + ds)));
double f = dut_abs_->get_inv_pfunction_output(
dut_abs_->inverse_helix_function(dut_abs_->helix_function(s)));
const double num_value = (fprime - f) / ds;
EXPECT_NEAR(candidate_value, num_value, tol);
// Compute the derivative of the inverse helix function using the
// velocity.
const double x = std::cos(test_value);
const double y = std::sin(test_value);
const double z = test_value;
const double xdot = 1.0;
const double ydot = 2.0;
const double zdot = 3.0;
const double inv_helix_dot = -y / (x * x + y * y) * xdot +
x / (x * x + y * y) * ydot;
Eigen::Matrix<BeadOnAWire<double>::ArcLength, 3, 1> xx;
xx(0).value() = x;
xx(1).value() = y;
xx(2).value() = z;
xx(0).value().derivatives()(0) = xdot;
xx(1).value().derivatives()(0) = ydot;
xx(2).value().derivatives()(0) = zdot;
double tprime = dut_abs_->get_inv_pfunction_first_derivative(
dut_abs_->inverse_helix_function(xx));
EXPECT_NEAR(inv_helix_dot, tprime, tol);
}
// A parametric wire function that allows the bead to move along x² - y² = 0
// (which is orthogonal to gravity).
static Eigen::Matrix<BeadOnAWire<double>::ArcLength, 3, 1>
horz_line_function(const BeadOnAWire<double>::ArcLength& s) {
return Vector3<BeadOnAWire<double>::ArcLength>(s*s, s*s, s*0);
}
// The inverse of the parametric wire function that allows the bead to move
// along x² - y² = 0 (which is orthogonal to gravity).
static BeadOnAWire<double>::ArcLength inverse_horz_line_function(
const Vector3<BeadOnAWire<double>::ArcLength>& v) {
using std::sqrt;
return (sqrt(v(1))+sqrt(v(0)))/2;
}
// A parametric wire function that allows the bead to along the z-axis (i.e.,
// parallel to gravity).
static Eigen::Matrix<BeadOnAWire<double>::ArcLength, 3, 1>
vert_line_function(const BeadOnAWire<double>::ArcLength& s) {
return Vector3<BeadOnAWire<double>::ArcLength>(s*0, s*0, s);
}
// The inverse of the parametric wire function that allows the bead to along
// the z-axis.
static BeadOnAWire<double>::ArcLength inverse_vert_line_function(
const Vector3<BeadOnAWire<double>::ArcLength>& v) {
return v(2);
}
// Tests the constraint function evaluation using the helix function.
TEST_F(BeadOnAWireTest, ConstraintFunctionEval) {
// Use the vertical wire function, which allows us to determine error
// precisely.
dut_abs_->reset_wire_parameter_functions(&vert_line_function,
&inverse_vert_line_function);
// Put the bead directly onto the wire.
systems::ContinuousState<double>& xc =
context_abs_->get_mutable_continuous_state();
const double s = 1.0;
xc[0] = 0.0;
xc[1] = 0.0;
xc[2] = s;
// Verify that the constraint error is effectively zero.
const double tol = std::numeric_limits<double>::epsilon() * 10;
EXPECT_NEAR(dut_abs_->EvalConstraintEquations(*context_abs_).norm(),
0.0, tol);
// Move the bead off of the wire and verify the expected error.
const double err = 1.0;
xc[0] += err;
EXPECT_LE(dut_abs_->EvalConstraintEquations(*context_abs_).norm() - err, tol);
}
// Tests the evaluation of the time derivative of the constraint functions
// using the helix function.
TEST_F(BeadOnAWireTest, ConstraintDotFunctionEval) {
// Put the bead directly onto the wire and make its velocity such that
// it is not instantaneously leaving the wire.
systems::ContinuousState<double>& xc =
context_abs_->get_mutable_continuous_state();
const double s = 1.0;
xc[0] = std::cos(s);
xc[1] = std::sin(s);
xc[2] = s;
xc[3] = -std::sin(s);
xc[4] = std::cos(s);
xc[5] = 1.0;
// Verify that the constraint error is effectively zero.
const double tol = std::numeric_limits<double>::epsilon() * 10;
EXPECT_NEAR(dut_abs_->EvalConstraintEquationsDot(*context_abs_).norm(),
0.0, tol);
}
// Verify that the minimal coordinate dynamics computations are reasonable.
TEST_F(BeadOnAWireTest, MinimalCoordinateDeriv) {
const int n_vars = derivatives_min_->get_vector().size();
const double tol = std::numeric_limits<double>::epsilon();
// For horizontal wire function, velocity derivatives should be due to
// centripetal acceleration only. Centripetal acceleration is v^2/R, where
// v is the velocity and R is the radius. In this test case, the
// instantaneous radius (i.e., that for initial s = 1.0) is 1.0 and the
// initial velocity is 1.0.
const double ca = 1.0; // centripetal acceleration
dut_min_->reset_wire_parameter_functions(&horz_line_function,
&inverse_horz_line_function);
dut_min_->CalcTimeDerivatives(*context_min_, derivatives_min_.get());
Eigen::VectorXd deriv = derivatives_min_->get_vector().CopyToVector();
EXPECT_LT(std::abs(deriv.segment(n_vars/2, n_vars/2).norm() - ca), tol);
// For vertical wire function, velocity derivatives should be gravitational
// acceleration.
dut_min_->reset_wire_parameter_functions(&vert_line_function,
&inverse_vert_line_function);
dut_min_->CalcTimeDerivatives(*context_min_, derivatives_min_.get());
deriv = derivatives_min_->get_vector().CopyToVector();
EXPECT_NEAR(deriv.segment(n_vars/2, n_vars/2).norm(),
std::abs(dut_min_->get_gravitational_acceleration()), tol);
}
} // namespace
} // namespace bead_on_a_wire
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/cubic_polynomial/region_of_attraction.cc | // A simple example of computing a region of attraction for a (polynomial)
// dynamical system. Note that this is a C++ version of
// CubicPolynomialExample.m which is available at the following link:
//
// https://github.com/RobotLocomotion/drake/blob/00ec5a9836871d5a22579963d45376b5979e41d5/drake/examples/CubicPolynomialExample.m.
//
// TODO(russt): Provide an additional python-only implementation of this
// example.
#include <cmath>
#include <iostream>
#include <gflags/gflags.h>
#include "drake/common/symbolic/polynomial.h"
#include "drake/common/unused.h"
#include "drake/solvers/mathematical_program.h"
#include "drake/solvers/solve.h"
#include "drake/systems/framework/vector_system.h"
namespace drake {
using std::cout;
using std::endl;
using symbolic::Expression;
using symbolic::Polynomial;
using symbolic::Variable;
using symbolic::Variables;
/// Cubic Polynomial System:
/// ẋ = -x + x³
/// y = x
template <typename T>
class CubicPolynomialSystem : public systems::VectorSystem<T> {
public:
CubicPolynomialSystem()
: systems::VectorSystem<T>(0, 0) { // Zero inputs, zero outputs.
this->DeclareContinuousState(1); // One state variable.
}
private:
// ẋ = -x + x³
virtual void DoCalcVectorTimeDerivatives(
const systems::Context<T>& context,
const Eigen::VectorBlock<const VectorX<T>>& input,
const Eigen::VectorBlock<const VectorX<T>>& state,
Eigen::VectorBlock<VectorX<T>>* derivatives) const {
unused(context, input);
using std::pow;
(*derivatives)(0) = -state(0) + pow(state(0), 3.0);
}
};
void ComputeRegionOfAttraction() {
// Create the simple system.
CubicPolynomialSystem<Expression> system;
auto context = system.CreateDefaultContext();
auto derivatives = system.AllocateTimeDerivatives();
// Setup the optimization problem.
solvers::MathematicalProgram prog;
const VectorX<Variable> xvar{prog.NewIndeterminates<1>("x")};
const VectorX<Expression> x = xvar.cast<Expression>();
// Extract the polynomial dynamics.
context->get_mutable_continuous_state_vector().SetFromVector(x);
system.CalcTimeDerivatives(*context, derivatives.get());
// Define the Lyapunov function.
const Expression V = x.dot(x);
const Expression Vdot = (Jacobian(Vector1<Expression>(V), xvar) *
derivatives->CopyToVector())[0];
// Maximize ρ s.t. V̇ ≥ 0 ∧ x ≠ 0 ⇒ V ≥ ρ,
// implemented as (V(x) - ρ)x² - λ(x)V̇(x) is SOS;
// λ(x) is SOS.
const Variable rho{prog.NewContinuousVariables<1>("rho").coeff(0)};
const Expression lambda{
prog.NewSosPolynomial(Variables(xvar), 4).first.ToExpression()};
prog.AddSosConstraint((V - rho) * x.dot(x) - lambda * Vdot);
prog.AddLinearCost(-rho);
const solvers::MathematicalProgramResult result = Solve(prog);
DRAKE_DEMAND(result.is_success());
cout << "Verified that " << V << " < " << result.GetSolution(rho)
<< " is in the region of attraction." << endl;
// Check that ρ ≃ 1.0.
DRAKE_DEMAND(std::abs(result.GetSolution(rho) - 1.0) < 1e-6);
}
} // namespace drake
int main(int argc, char* argv[]) {
// Process the add_text_logging_gflags from our BUILD file.
gflags::ParseCommandLineFlags(&argc, &argv, true);
drake::ComputeRegionOfAttraction();
return 0;
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/cubic_polynomial/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load("//tools/skylark:drake_cc.bzl", "drake_cc_binary")
load("//tools/skylark:test_tags.bzl", "mosek_test_tags")
package(default_visibility = ["//visibility:private"])
drake_cc_binary(
name = "region_of_attraction",
srcs = ["region_of_attraction.cc"],
add_test_rule = 1,
deps = [
"//common:add_text_logging_gflags",
"//solvers:mathematical_program",
"//solvers:solve",
"//systems/framework:vector_system",
],
)
drake_cc_binary(
name = "backward_reachability",
srcs = ["backward_reachability.cc"],
add_test_rule = 1,
test_rule_tags = mosek_test_tags(),
deps = [
"//common/proto:call_python",
"//solvers:mathematical_program",
"//solvers:mosek_solver",
"//solvers:solve",
"//systems/framework:vector_system",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/cubic_polynomial/backward_reachability.cc | // A simple "hello world" example of computing a finite-time backward reachable
// set for a polynomial system using convex optimization.
//
// This implements the occupation measure-based SDP relaxation described in
// "Convex computation of the region of attraction of polynomial control
// systems" by Didier Henrion and Milan Korda, IEEE Transactions on Automatic
// Control, vol. 59, no. 2, pp. 297-312, Feb. 2014.
//
// TODO(jadecastro) Transcribe this example into Python.
#include <cmath>
#include <iostream>
#include "drake/common/proto/call_python.h"
#include "drake/common/symbolic/polynomial.h"
#include "drake/solvers/mathematical_program.h"
#include "drake/solvers/mosek_solver.h"
#include "drake/solvers/solve.h"
#include "drake/systems/framework/vector_system.h"
namespace drake {
namespace {
using std::cout;
using std::endl;
using std::pow;
using common::CallPython;
using symbolic::Environment;
using symbolic::Expression;
using symbolic::Polynomial;
using symbolic::Variable;
using symbolic::Variables;
using MapType = Polynomial::MapType;
using Bound = std::map<Variable, double>;
using BoundingBox = std::pair<Bound, Bound>;
/// Defines the autonomous cubic polynomial system:
/// ẋ = 100 x(x - 0.5)(x + 0.5) = 100 x³ - 25 x
template <typename T>
class CubicSystem : public systems::VectorSystem<T> {
public:
CubicSystem() : systems::VectorSystem<T>(0, 0) {
// 0 inputs, 0 outputs, 1 continuous state.
this->DeclareContinuousState(1);
}
private:
virtual void DoCalcVectorTimeDerivatives(
const systems::Context<T>&, const Eigen::VectorBlock<const VectorX<T>>&,
const Eigen::VectorBlock<const VectorX<T>>& state,
Eigen::VectorBlock<VectorX<T>>* derivatives) const {
(*derivatives)(0) = 100. * pow(state(0), 3.0) - 25. * state(0);
}
};
/// Makes a cost equal to the volume of polynomial @p p over with respect to the
/// indeterminates over the domain defined by @p b. For polynomials, this is
/// equivalent to the product of `coeff(p) * l`, where `coeff(p)` is the vector
/// of coefficients of `p` and `l` is the corresponding vector of moments of the
/// Lebesgue measure over the domain.
Expression MakeVolumetricCost(const Polynomial& p, const BoundingBox& b) {
DRAKE_DEMAND(b.first.size() == b.second.size());
DRAKE_DEMAND(p.indeterminates().size() == b.first.size());
// Build a moment vector.
const MapType& map = p.monomial_to_coefficient_map();
Expression result;
const Variables& indeterminates = p.indeterminates();
for (const auto& monomial : map) {
const std::map<Variable, int>& powers = monomial.first.get_powers();
Expression moment{1.};
for (const auto& var : indeterminates) {
if (powers.find(var) == powers.end()) {
moment *= b.second.at(var) - b.first.at(var); // zero exponent case.
continue;
}
moment *= (pow(b.second.at(var), powers.at(var) + 1) -
pow(b.first.at(var), powers.at(var) + 1)) /
(powers.at(var) + 1);
}
result += moment * monomial.second;
}
return result;
}
/// Solves the backward reachable set over T seconds on the above univariate
/// example, given a terminal set. Specifically, we solve the dual of the
/// polynomial relaxation to an infinite-dimensional LP over the space of
/// occupation measures capturing the reachable set. By restricting the space
/// of functions to polynomials and representing measures by their moments, we
/// solve the dual relaxation over the space of nonnegative functions in order
/// to find a tight over-approximation to the reachable set.
///
/// Given the target set {x | ‖x(1)‖ ≤ 0.1}, the cubic polynomial system
/// ẋ = 100x(x - 0.5)(x + 0.5) is known to have a backward reachable set
/// B = {x | ‖x(0)‖ < 0.5}.
void ComputeBackwardReachableSet() {
// Construct the system.
CubicSystem<Expression> system;
auto context = system.CreateDefaultContext();
auto derivatives = system.AllocateTimeDerivatives();
// Set up the optimization problem and declare the indeterminates.
solvers::MathematicalProgram prog;
const VectorX<Variable> tvec{prog.NewIndeterminates<1>("t")};
const VectorX<Variable> xvec{prog.NewIndeterminates<1>("x")};
const Variable& t = tvec(0);
const Variable& x = xvec(0);
// The desired order of the polynomial function approximations (noting that
// the SOS multipliers, constructed below, have order d-2).
const int d = 8;
// Extract the polynomial dynamics.
context->get_mutable_continuous_state_vector().SetAtIndex(0, x);
system.CalcTimeDerivatives(*context, derivatives.get());
// Define the domain bounds on t: 0 ≤ t ≤ T.
const double T = 1.;
const Polynomial gt = Polynomial{t * (T - t)};
// Define the (symmetric) domain bounds on x: ‖x‖ ≤ x_bound
// (X = {x | gx ≥ 0}).
const double x_bound = 1.;
const Polynomial gx = Polynomial{-(x - x_bound) * (x + x_bound)};
// Define the terminal set: ‖x(T)‖ ≤ 0.1 (X_T = {x | gxT ≥ 0}).
const Polynomial gxT{-(x - 0.1) * (x + 0.1)};
// Define the ground-truth backward reachable set (for plotting only):
// ‖x(0)‖ < 0.5 (a.k.a. X₀).
const Polynomial gx0{-(x - 0.5) * (x + 0.5)};
// Set up and solve the following optimization problem:
// Inf w'l over v, w, qₓ, qt, qT, q₀, sₓ
// s.t. ℒv ≤ 0 on [0, T] × X (see below and Henrion and Korda 2014)
// v ≥ 0 on {T} × X_T
// w ≥ v + 1 on {0} × X
// w ≥ 0 on X
//
// The result is the T-horizon outer-approximated backward reachable set,
// B = X₀ = {x ∈ X | w ≥ 1}.
const Polynomial v{prog.NewFreePolynomial({t, x}, d)};
const Polynomial w{prog.NewFreePolynomial({x}, d)};
// ℒ operator, defined as v ↦ ℒv := ∂v/∂t + Σ_{i=1,…,n} ∂v/∂x fᵢ(t,x).
const Polynomial Lv{v.Jacobian(tvec).coeff(0) +
v.Jacobian(xvec).coeff(0) *
Polynomial((*derivatives)[0])};
// v decreases along trajectories over t ∈ [0, T], x ∈ X.
const Polynomial qx{prog.NewSosPolynomial({t, x}, d - 2).first};
const Polynomial qt{prog.NewSosPolynomial({t, x}, d - 2).first};
prog.AddSosConstraint(-Lv - qx * gx - qt * gt);
// v(t=T, x) is SOS for all x ∈ X_T. Note that this constraint, coupled with
// the one above, implies that v(t=0, x) ≥ 0 for all x ∈ B.
// Notice that, by replacing v_at_T with v, we may solve the "free final-time"
// problem.
const Polynomial v_at_T = v.EvaluatePartial({{t, T}});
const Polynomial qT{prog.NewSosPolynomial({x}, d - 2).first};
prog.AddSosConstraint(v_at_T - qT * gxT);
// w is an outer-approximation to B over x ∈ X.
const Polynomial v_at_0 = v.EvaluatePartial({{t, 0.}});
const Polynomial q0{prog.NewSosPolynomial({x}, d - 2).first};
prog.AddSosConstraint(w - v_at_0 - 1. - q0 * gx);
// w is SOS for all x ∈ X.
const Polynomial sx{prog.NewSosPolynomial({x}, d - 2).first};
prog.AddSosConstraint(w - sx * gx);
// w represents a *tight* outer-approximation.
prog.AddCost(MakeVolumetricCost(
w, std::make_pair(Bound{{x, -x_bound}}, Bound{{x, x_bound}})));
cout << " Program attributes: " << endl;
cout << " number of decision variables: " << prog.num_vars() << endl;
cout << " number of PSD constraints: "
<< prog.positive_semidefinite_constraints().size() << endl;
const solvers::MathematicalProgramResult result = Solve(prog);
DRAKE_DEMAND(result.get_solver_id() == solvers::MosekSolver::id());
DRAKE_DEMAND(result.is_success());
// Print the solution (if one is found).
cout << " Solution found with optimal cost: " << result.get_optimal_cost()
<< endl;
Environment w_env;
for (const auto& var : w.decision_variables()) {
w_env.insert(var, result.GetSolution(var));
}
const Polynomial w_sol{w.EvaluatePartial(w_env)};
// Serialize the result and generate Python plots. In particular, plot the
// true indicator function of B and w (its polynomial outer-approximation).
// Execute:
// > bazel run //examples/cubic_polynomial:backward_reachability -c dbg
// --config mosek
// > bazel run //common/proto:call_python_client_cli
const int N{1000};
Eigen::VectorXd x_val(N), w_val(N), v_val(N), ground_val(N);
for (int i = 0; i < N; i++) {
x_val[i] = x_bound * (2. * i / N - 1.);
w_val[i] = w_sol.Evaluate({{x, x_val[i]}});
ground_val[i] = (gx0.Evaluate({{x, x_val[i]}}) >= 0.) ? 1. : 0.;
}
CallPython("figure", 1);
CallPython("clf");
CallPython("plot", x_val, w_val);
CallPython("setvars", "x_val", x_val, "w_val", w_val);
CallPython("plot", x_val, ground_val);
CallPython("setvars", "x_val", x_val, "ground_val", ground_val);
CallPython("plt.xlabel", "x");
CallPython("plt.ylabel", "w, I_B");
}
} // namespace
} // namespace drake
int main() {
drake::ComputeBackwardReachableSet();
return 0;
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/atlas/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_binary",
)
package(default_visibility = ["//visibility:private"])
drake_cc_binary(
name = "atlas_run_dynamics",
srcs = [
"atlas_run_dynamics.cc",
],
add_test_rule = 1,
data = ["@drake_models//:atlas"],
# Smoke test.
test_rule_args = [
"--simulation_time=0.01",
"--simulator_target_realtime_rate=0.0",
],
deps = [
"//common:add_text_logging_gflags",
"//multibody/parsing",
"//systems/analysis:simulator",
"//systems/analysis:simulator_gflags",
"//systems/framework:diagram",
"//visualization:visualization_config_functions",
"@gflags",
],
)
add_lint_tests()
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/atlas/README.md |
This directory contains a small demo of Atlas dynamics.
Historical note
---------------
Prior to 2016, Drake was built around a substantial base of MATLAB software.
Most of that was removed from the head of git master during 2017.
To view or use the original MATLAB implementation of Atlas you may use this tag:
https://github.com/RobotLocomotion/drake/tree/last_sha_with_original_matlab/drake/examples/Atlas
For progress updates on the re-implementation, see
https://github.com/RobotLocomotion/drake/issues/6381.
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/atlas/atlas_run_dynamics.cc | #include <memory>
#include <gflags/gflags.h>
#include "drake/geometry/scene_graph.h"
#include "drake/multibody/parsing/parser.h"
#include "drake/multibody/plant/multibody_plant_config_functions.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/analysis/simulator_gflags.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/visualization/visualization_config_functions.h"
DEFINE_double(simulation_time, 2.0, "Simulation duration in seconds");
DEFINE_double(penetration_allowance, 1.0E-3, "Allowable penetration (meters).");
DEFINE_double(stiction_tolerance, 1.0E-3,
"Allowable drift speed during stiction (m/s).");
DEFINE_double(
mbp_discrete_update_period, 0.01,
"The fixed-time step period (in seconds) of discrete updates for the "
"multibody plant modeled as a discrete system. Strictly positive. "
"Set to zero for a continuous plant model. When using TAMSI, a smaller "
"time step of 1.0e-3 is recommended.");
DEFINE_string(contact_approximation, "sap",
"Discrete contact approximation. Options are: 'tamsi', 'sap', "
"'similar', 'lagged'");
namespace drake {
namespace examples {
namespace atlas {
namespace {
using drake::math::RigidTransformd;
using drake::multibody::MultibodyPlant;
using drake::multibody::MultibodyPlantConfig;
using Eigen::Translation3d;
using Eigen::VectorXd;
int do_main() {
if (FLAGS_mbp_discrete_update_period < 0) {
throw std::runtime_error(
"mbp_discrete_update_period must be a non-negative number.");
}
// Build a generic multibody plant.
systems::DiagramBuilder<double> builder;
MultibodyPlantConfig plant_config;
plant_config.time_step = FLAGS_mbp_discrete_update_period;
plant_config.stiction_tolerance = FLAGS_stiction_tolerance;
plant_config.discrete_contact_approximation = FLAGS_contact_approximation;
auto [plant, scene_graph] =
multibody::AddMultibodyPlant(plant_config, &builder);
multibody::Parser(&plant).AddModelsFromUrl(
"package://drake_models/atlas/atlas_convex_hull.urdf");
// Add model of the ground.
const double static_friction = 1.0;
const Vector4<double> green(0.5, 1.0, 0.5, 1.0);
plant.RegisterVisualGeometry(plant.world_body(), RigidTransformd(),
geometry::HalfSpace(), "GroundVisualGeometry",
green);
// For a time-stepping model only static friction is used.
const multibody::CoulombFriction<double> ground_friction(static_friction,
static_friction);
plant.RegisterCollisionGeometry(plant.world_body(), RigidTransformd(),
geometry::HalfSpace(),
"GroundCollisionGeometry", ground_friction);
plant.Finalize();
plant.set_penetration_allowance(FLAGS_penetration_allowance);
// Set the speed tolerance (m/s) for the underlying Stribeck friction model
// For two points in contact, this is the maximum allowable drift speed at the
// edge of the friction cone, an approximation to true stiction.
plant.set_stiction_tolerance(FLAGS_stiction_tolerance);
// Sanity check model size.
DRAKE_DEMAND(plant.num_velocities() == 36);
DRAKE_DEMAND(plant.num_positions() == 37);
// Verify the "pelvis" body is free and modeled with quaternions dofs before
// moving on with that assumption.
const drake::multibody::RigidBody<double>& pelvis =
plant.GetBodyByName("pelvis");
DRAKE_DEMAND(pelvis.is_floating());
DRAKE_DEMAND(pelvis.has_quaternion_dofs());
// Since there is a single floating body, we know that the positions for it
// lie first in the state vector.
DRAKE_DEMAND(pelvis.floating_positions_start() == 0);
// Similarly for velocities. The velocities for this floating pelvis are the
// first set of velocities and should start at the beginning of v.
DRAKE_DEMAND(pelvis.floating_velocities_start_in_v() == 0);
visualization::AddDefaultVisualization(&builder);
auto diagram = builder.Build();
// Create a context for this system:
std::unique_ptr<systems::Context<double>> diagram_context =
diagram->CreateDefaultContext();
systems::Context<double>& plant_context =
diagram->GetMutableSubsystemContext(plant, diagram_context.get());
const VectorXd tau = VectorXd::Zero(plant.num_actuated_dofs());
plant.get_actuation_input_port().FixValue(&plant_context, tau);
// Set the pelvis frame P initial pose.
const Translation3d X_WP(0.0, 0.0, 0.95);
plant.SetFreeBodyPoseInWorldFrame(&plant_context, pelvis, X_WP);
auto simulator =
MakeSimulatorFromGflags(*diagram, std::move(diagram_context));
simulator->AdvanceTo(FLAGS_simulation_time);
return 0;
}
} // namespace
} // namespace atlas
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::SetUsageMessage(
"\nPassive simulation of the Atlas robot. With the default time step of "
"\n1 ms this simulation typically runs slightly faster than real-time. "
"\nThe time step has an effect on the joint limits stiffnesses, which "
"\nconverge quadratically to the rigid limit as the time step is "
"\ndecreased. Thus, decrease the time step for more accurately resolved "
"\njoint limits. "
"\nLaunch meldis before running this example.");
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::atlas::do_main();
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/zmp/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_binary",
)
package(default_visibility = ["//visibility:private"])
drake_cc_binary(
name = "zmp_example",
testonly = 1,
srcs = ["zmp_example.cc"],
deps = [
"//common/proto:call_python",
"//planning/locomotion/test_utilities",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/zmp/zmp_example.cc | #include <chrono>
#include <thread>
#include "drake/common/proto/call_python.h"
#include "drake/planning/locomotion/test_utilities/zmp_test_util.h"
namespace drake {
namespace examples {
namespace zmp {
namespace {
void PlotResults(const planning::ZmpTestTraj& traj) {
using common::CallPython;
using common::ToPythonTuple;
CallPython("figure", 1);
CallPython("clf");
CallPython("subplot", 2, 1, 1);
CallPython("plot", traj.time.transpose(),
traj.desired_zmp.row(0).transpose(), "r");
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(0).transpose(), "b");
CallPython("plot", traj.time.transpose(), traj.cop.row(0).transpose(), "g");
CallPython("plot", traj.time.transpose(), traj.x.row(0).transpose(), "c");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "x [m]");
CallPython("legend", ToPythonTuple("desired zmp", "planned com",
"planned cop", "actual com"));
CallPython("subplot", 2, 1, 2);
CallPython("plot", traj.time.transpose(),
traj.desired_zmp.row(1).transpose(), "r");
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(1).transpose(), "b");
CallPython("plot", traj.time.transpose(), traj.cop.row(1).transpose(), "g");
CallPython("plot", traj.time.transpose(), traj.x.row(1).transpose(), "c");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "y [m]");
CallPython("legend", ToPythonTuple("desired zmp", "planned com",
"planned cop", "actual com"));
// Give time for Python to plot.
std::this_thread::sleep_for(std::chrono::seconds(1));
CallPython("figure", 2);
CallPython("clf");
CallPython("subplot", 2, 1, 1);
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(2).transpose(), "b");
CallPython("plot", traj.time.transpose(), traj.x.row(2).transpose(), "c");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "xd [m/s]");
CallPython("legend", ToPythonTuple("planned comd", "actual comd"));
CallPython("subplot", 2, 1, 2);
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(3).transpose(), "b");
CallPython("plot", traj.time.transpose(), traj.x.row(3).transpose(), "c");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "yd [m/s]");
CallPython("legend", ToPythonTuple("planned comd", "actual comd"));
// Give time for Python to plot.
std::this_thread::sleep_for(std::chrono::seconds(1));
CallPython("figure", 3);
CallPython("clf");
CallPython("subplot", 2, 1, 1);
CallPython("plot", traj.time.transpose(), traj.u.row(0).transpose(), "r");
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(4).transpose(), "b.");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "xdd [m/s2]");
CallPython("legend", ToPythonTuple("comdd from policy", "nominal comdd"));
CallPython("subplot", 2, 1, 2);
CallPython("plot", traj.time.transpose(), traj.u.row(1).transpose(), "r");
CallPython("plot", traj.time.transpose(),
traj.nominal_com.row(5).transpose(), "b.");
CallPython("xlabel", "time [s]");
CallPython("ylabel", "ydd [m/s2]");
CallPython("legend", ToPythonTuple("comdd from policy", "nominal comdd"));
// Give time for Python to plot.
std::this_thread::sleep_for(std::chrono::seconds(1));
}
void do_main() {
std::vector<Eigen::Vector2d> footsteps = {
Eigen::Vector2d(0, 0), Eigen::Vector2d(0.5, 0.1),
Eigen::Vector2d(1, -0.1), Eigen::Vector2d(1.5, 0.1),
Eigen::Vector2d(2, -0.1), Eigen::Vector2d(2.5, 0)};
std::vector<trajectories::PiecewisePolynomial<double>> zmp_trajs =
planning::GenerateDesiredZmpTrajs(footsteps, 0.5, 1);
Eigen::Vector4d x0(0, 0, 0, 0);
double z = 1;
planning::ZmpPlanner zmp_planner;
zmp_planner.Plan(zmp_trajs[0], x0, z);
double sample_dt = 0.01;
// Perturb the initial state a bit.
x0 << 0, 0, 0.2, -0.1;
planning::ZmpTestTraj result =
planning::SimulateZmpPolicy(zmp_planner, x0, sample_dt, 2);
PlotResults(result);
}
} // namespace
} // namespace zmp
} // namespace examples
} // namespace drake
int main() {
drake::examples::zmp::do_main();
return 0;
}
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_continuous_state.cc | #include "drake/examples/rimless_wheel/rimless_wheel_continuous_state.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
const int RimlessWheelContinuousStateIndices::kNumCoordinates;
const int RimlessWheelContinuousStateIndices::kTheta;
const int RimlessWheelContinuousStateIndices::kThetadot;
const std::vector<std::string>&
RimlessWheelContinuousStateIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"theta",
"thetadot",
});
return coordinates.access();
}
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_params.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
/// Describes the row indices of a RimlessWheelParams.
struct RimlessWheelParamsIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 5;
// The index of each individual coordinate.
static const int kMass = 0;
static const int kLength = 1;
static const int kGravity = 2;
static const int kNumberOfSpokes = 3;
static const int kSlope = 4;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words, `RimlessWheelParamsIndices::GetCoordinateNames()[i]`
/// is the name for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class RimlessWheelParams final : public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef RimlessWheelParamsIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c mass defaults to 1.0 kg.
/// @arg @c length defaults to 1.0 m.
/// @arg @c gravity defaults to 9.81 m/s^2.
/// @arg @c number_of_spokes defaults to 8 integer.
/// @arg @c slope defaults to 0.08 radians.
RimlessWheelParams() : drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_mass(1.0);
this->set_length(1.0);
this->set_gravity(9.81);
this->set_number_of_spokes(8);
this->set_slope(0.08);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
RimlessWheelParams(const RimlessWheelParams& other)
: drake::systems::BasicVector<T>(other.values()) {}
RimlessWheelParams(RimlessWheelParams&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
RimlessWheelParams& operator=(const RimlessWheelParams& other) {
this->values() = other.values();
return *this;
}
RimlessWheelParams& operator=(RimlessWheelParams&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_mass(symbolic::Variable("mass"));
this->set_length(symbolic::Variable("length"));
this->set_gravity(symbolic::Variable("gravity"));
this->set_number_of_spokes(symbolic::Variable("number_of_spokes"));
this->set_slope(symbolic::Variable("slope"));
}
[[nodiscard]] RimlessWheelParams<T>* DoClone() const final {
return new RimlessWheelParams;
}
/// @name Getters and Setters
//@{
/// The rimless wheel has a single point mass at the hub.
/// @note @c mass is expressed in units of kg.
/// @note @c mass has a limited domain of [0.0, +Inf].
const T& mass() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kMass);
}
/// Setter that matches mass().
void set_mass(const T& mass) {
ThrowIfEmpty();
this->SetAtIndex(K::kMass, mass);
}
/// Fluent setter that matches mass().
/// Returns a copy of `this` with mass set to a new value.
[[nodiscard]] RimlessWheelParams<T> with_mass(const T& mass) const {
RimlessWheelParams<T> result(*this);
result.set_mass(mass);
return result;
}
/// The length of each spoke.
/// @note @c length is expressed in units of m.
/// @note @c length has a limited domain of [0.0, +Inf].
const T& length() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kLength);
}
/// Setter that matches length().
void set_length(const T& length) {
ThrowIfEmpty();
this->SetAtIndex(K::kLength, length);
}
/// Fluent setter that matches length().
/// Returns a copy of `this` with length set to a new value.
[[nodiscard]] RimlessWheelParams<T> with_length(const T& length) const {
RimlessWheelParams<T> result(*this);
result.set_length(length);
return result;
}
/// An approximate value for gravitational acceleration.
/// @note @c gravity is expressed in units of m/s^2.
/// @note @c gravity has a limited domain of [0.0, +Inf].
const T& gravity() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kGravity);
}
/// Setter that matches gravity().
void set_gravity(const T& gravity) {
ThrowIfEmpty();
this->SetAtIndex(K::kGravity, gravity);
}
/// Fluent setter that matches gravity().
/// Returns a copy of `this` with gravity set to a new value.
[[nodiscard]] RimlessWheelParams<T> with_gravity(const T& gravity) const {
RimlessWheelParams<T> result(*this);
result.set_gravity(gravity);
return result;
}
/// Total number of spokes on the wheel
/// @note @c number_of_spokes is expressed in units of integer.
/// @note @c number_of_spokes has a limited domain of [4, +Inf].
const T& number_of_spokes() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kNumberOfSpokes);
}
/// Setter that matches number_of_spokes().
void set_number_of_spokes(const T& number_of_spokes) {
ThrowIfEmpty();
this->SetAtIndex(K::kNumberOfSpokes, number_of_spokes);
}
/// Fluent setter that matches number_of_spokes().
/// Returns a copy of `this` with number_of_spokes set to a new value.
[[nodiscard]] RimlessWheelParams<T> with_number_of_spokes(
const T& number_of_spokes) const {
RimlessWheelParams<T> result(*this);
result.set_number_of_spokes(number_of_spokes);
return result;
}
/// The angle of the ramp on which the rimless wheel is walking.
/// @note @c slope is expressed in units of radians.
const T& slope() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kSlope);
}
/// Setter that matches slope().
void set_slope(const T& slope) {
ThrowIfEmpty();
this->SetAtIndex(K::kSlope, slope);
}
/// Fluent setter that matches slope().
/// Returns a copy of `this` with slope set to a new value.
[[nodiscard]] RimlessWheelParams<T> with_slope(const T& slope) const {
RimlessWheelParams<T> result(*this);
result.set_slope(slope);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& mass_ref = this->GetAtIndex(K::kMass);
a->Visit(drake::MakeNameValue("mass", &mass_ref));
T& length_ref = this->GetAtIndex(K::kLength);
a->Visit(drake::MakeNameValue("length", &length_ref));
T& gravity_ref = this->GetAtIndex(K::kGravity);
a->Visit(drake::MakeNameValue("gravity", &gravity_ref));
T& number_of_spokes_ref = this->GetAtIndex(K::kNumberOfSpokes);
a->Visit(drake::MakeNameValue("number_of_spokes", &number_of_spokes_ref));
T& slope_ref = this->GetAtIndex(K::kSlope);
a->Visit(drake::MakeNameValue("slope", &slope_ref));
}
/// See RimlessWheelParamsIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return RimlessWheelParamsIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(mass());
result = result && (mass() >= T(0.0));
result = result && !isnan(length());
result = result && (length() >= T(0.0));
result = result && !isnan(gravity());
result = result && (gravity() >= T(0.0));
result = result && !isnan(number_of_spokes());
result = result && (number_of_spokes() >= T(4));
result = result && !isnan(slope());
return result;
}
void GetElementBounds(Eigen::VectorXd* lower,
Eigen::VectorXd* upper) const final {
const double kInf = std::numeric_limits<double>::infinity();
*lower = Eigen::Matrix<double, 5, 1>::Constant(-kInf);
*upper = Eigen::Matrix<double, 5, 1>::Constant(kInf);
(*lower)(K::kMass) = 0.0;
(*lower)(K::kLength) = 0.0;
(*lower)(K::kGravity) = 0.0;
(*lower)(K::kNumberOfSpokes) = 4;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The RimlessWheelParams vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_geometry.cc | #include "drake/examples/rimless_wheel/rimless_wheel_geometry.h"
#include <memory>
#include <fmt/format.h>
#include "drake/examples/rimless_wheel/rimless_wheel_params.h"
#include "drake/geometry/geometry_frame.h"
#include "drake/geometry/geometry_ids.h"
#include "drake/geometry/geometry_instance.h"
#include "drake/geometry/geometry_roles.h"
#include "drake/math/rigid_transform.h"
#include "drake/math/roll_pitch_yaw.h"
#include "drake/math/rotation_matrix.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
using Eigen::Vector3d;
using Eigen::Vector4d;
using Eigen::VectorXd;
using geometry::Box;
using geometry::Cylinder;
using geometry::GeometryFrame;
using geometry::GeometryId;
using geometry::GeometryInstance;
using geometry::MakePhongIllustrationProperties;
using geometry::Sphere;
using std::make_unique;
const RimlessWheelGeometry* RimlessWheelGeometry::AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& floating_base_state_port,
const RimlessWheelParams<double>& rimless_wheel_params,
geometry::SceneGraph<double>* scene_graph) {
DRAKE_THROW_UNLESS(builder != nullptr);
DRAKE_THROW_UNLESS(scene_graph != nullptr);
auto rimless_wheel_geometry =
builder->AddSystem(std::unique_ptr<RimlessWheelGeometry>(
new RimlessWheelGeometry(rimless_wheel_params, scene_graph)));
builder->Connect(floating_base_state_port,
rimless_wheel_geometry->get_input_port(0));
builder->Connect(
rimless_wheel_geometry->get_output_port(0),
scene_graph->get_source_pose_port(rimless_wheel_geometry->source_id_));
return rimless_wheel_geometry;
}
RimlessWheelGeometry::RimlessWheelGeometry(
const RimlessWheelParams<double>& params,
geometry::SceneGraph<double>* scene_graph) {
DRAKE_THROW_UNLESS(scene_graph != nullptr);
source_id_ = scene_graph->RegisterSource();
// Note: the floating-base state output from RimlessWheel uses RPY.
this->DeclareVectorInputPort("floating_base_state", 12);
this->DeclareAbstractOutputPort("geometry_pose",
&RimlessWheelGeometry::OutputGeometryPose);
// Add the ramp.
// Positioned so that the top center of the box is at x=y=z=0.
GeometryId id = scene_graph->RegisterAnchoredGeometry(
source_id_,
make_unique<GeometryInstance>(
math::RigidTransformd(
math::RotationMatrixd::MakeYRotation(params.slope())) *
math::RigidTransformd(Vector3d(0, 0, -10. / 2.)),
make_unique<Box>(100, 1, 10), "ramp"));
// Color is "desert sand" according to htmlcsscolor.com.
scene_graph->AssignRole(
source_id_, id,
MakePhongIllustrationProperties(Vector4d(0.9297, 0.7930, 0.6758, 1)));
// All of the remaining geometry is on one link.
frame_id_ = scene_graph->RegisterFrame(source_id_, GeometryFrame("center"));
// Add the hub.
id = scene_graph->RegisterGeometry(
source_id_, frame_id_,
make_unique<GeometryInstance>(
math::RigidTransformd(math::RotationMatrixd::MakeXRotation(M_PI_2)),
make_unique<Cylinder>(.2, .2), "hub"));
scene_graph->AssignRole(
source_id_, id, MakePhongIllustrationProperties(Vector4d(.6, .2, .2, 1)));
// Add the spokes.
for (int i = 0; i < params.number_of_spokes(); i++) {
id = scene_graph->RegisterGeometry(
source_id_, frame_id_,
make_unique<GeometryInstance>(
math::RigidTransformd(math::RotationMatrixd::MakeYRotation(
(2. * M_PI * i) / params.number_of_spokes())) *
math::RigidTransformd(Vector3d(0, 0, -params.length() / 2.)),
make_unique<Cylinder>(0.0075, params.length()),
fmt::format("spoke{}", i)));
scene_graph->AssignRole(
source_id_, id, MakePhongIllustrationProperties(Vector4d(0, 0, 0, 1)));
}
}
RimlessWheelGeometry::~RimlessWheelGeometry() = default;
void RimlessWheelGeometry::OutputGeometryPose(
const systems::Context<double>& context,
geometry::FramePoseVector<double>* poses) const {
DRAKE_DEMAND(frame_id_.is_valid());
const VectorXd& input = get_input_port(0).Eval(context);
const math::RigidTransformd pose(math::RollPitchYawd(input.segment<3>(3)),
input.head<3>());
*poses = {{frame_id_, pose}};
}
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel.cc | #include "drake/examples/rimless_wheel/rimless_wheel.h"
#include <limits>
#include "drake/common/default_scalars.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
template <typename T>
RimlessWheel<T>::RimlessWheel()
: systems::LeafSystem<T>(systems::SystemTypeTag<RimlessWheel>{}) {
this->DeclareContinuousState(RimlessWheelContinuousState<T>(), 1, 1, 0);
// Discrete state for stance toe distance along the ramp.
this->DeclareDiscreteState(1);
// Abstract state for indicating that we are in "double support" mode (e.g.
// two feet/spokes are touching the ground, and angular velocity is
// approximately zero).
const bool double_support = false;
this->DeclareAbstractState(Value<bool>(double_support));
// The minimal state of the system.
this->DeclareVectorOutputPort(systems::kUseDefaultName,
RimlessWheelContinuousState<T>(),
&RimlessWheel::MinimalStateOut);
// The floating-base (RPY) state of the system (useful for visualization).
this->DeclareVectorOutputPort(systems::kUseDefaultName, 12,
&RimlessWheel::FloatingBaseStateOut);
this->DeclareNumericParameter(RimlessWheelParams<T>());
// Create the witness functions.
step_backward_ = this->MakeWitnessFunction(
"step backward",
systems::WitnessFunctionDirection::kPositiveThenNonPositive,
&RimlessWheel::StepBackwardGuard, &RimlessWheel::StepBackwardReset);
step_forward_ = this->MakeWitnessFunction(
"step forward",
systems::WitnessFunctionDirection::kPositiveThenNonPositive,
&RimlessWheel::StepForwardGuard, &RimlessWheel::StepForwardReset);
}
template <typename T>
T RimlessWheel<T>::CalcTotalEnergy(const systems::Context<T>& context) const {
using std::pow;
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
const RimlessWheelParams<T>& params = get_parameters(context);
// Kinetic energy = 1/2 m l² θ̇ ².
const T kinetic_energy =
0.5 * params.mass() * pow(params.length() * rw_state.thetadot(), 2);
// Potential energy = mgl cos θ.
const T potential_energy = params.mass() * params.gravity() *
params.length() * cos(rw_state.theta());
return kinetic_energy + potential_energy;
}
template <typename T>
T RimlessWheel<T>::StepForwardGuard(const systems::Context<T>& context) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
const RimlessWheelParams<T>& params = get_parameters(context);
// Triggers when θ = slope + α.
return params.slope() + calc_alpha(params) - rw_state.theta();
}
template <typename T>
void RimlessWheel<T>::StepForwardReset(
const systems::Context<T>& context,
const systems::UnrestrictedUpdateEvent<T>&,
systems::State<T>* state) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
RimlessWheelContinuousState<T>& next_state =
get_mutable_continuous_state(&(state->get_mutable_continuous_state()));
const RimlessWheelParams<T>& params = get_parameters(context);
T& toe = get_mutable_toe_position(state);
const T alpha = calc_alpha(params);
// Stance foot changes to the new support leg.
next_state.set_theta(rw_state.theta() - 2. * alpha +
std::numeric_limits<T>::epsilon());
// Event isolation guarantees that the penetration was non-negative. We add
// epsilon because the post-impact state needs to be above the ground, so
// that the witness function evaluation that occurs immediately after the
// impact has a positive value (we have declared the WitnessFunction with
// kPositiveThenNonPositive). The failure mode before this improved
// implementation was the robot falling through the ground after an impact.
DRAKE_DEMAND(next_state.theta() > params.slope() - alpha);
// θ̇ ⇐ θ̇ cos(2α)
next_state.set_thetadot(rw_state.thetadot() * cos(2. * alpha));
// toe += stance length.
toe += 2. * params.length() * sin(alpha);
// If thetadot is very small, then transition to double support (this is
// only for efficiency, to avoid simulation at the Zeno).
// Note: I already know that thetadot > 0 since the guard triggered.
DRAKE_ASSERT(next_state.thetadot() >= 0.);
// The threshold value below impacts early termination. Setting it closer to
// zero will not cause the wheel to miss an event, but will cause the
// simulator to perform arbitrarily more event detection calculations. If the
// threshold is smaller than Simulator::GetCurrentWitnessTimeIsolation(), it
// can result in an infinite loop (a numerical Zeno's paradox). The
// threshold is multiplied by sqrt(g/l) to make it dimensionless.
const T kThreshold = 0.01 * sqrt(params.gravity() / params.length());
if (next_state.thetadot() < kThreshold) {
bool& double_support = get_mutable_double_support(state);
double_support = true;
next_state.set_thetadot(0.0);
}
}
template <typename T>
T RimlessWheel<T>::StepBackwardGuard(const systems::Context<T>& context) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
const RimlessWheelParams<T>& params = get_parameters(context);
// Triggers when θ = slope - α.
return rw_state.theta() - params.slope() + calc_alpha(params);
}
template <typename T>
void RimlessWheel<T>::StepBackwardReset(
const systems::Context<T>& context,
const systems::UnrestrictedUpdateEvent<T>&,
systems::State<T>* state) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
RimlessWheelContinuousState<T>& next_state =
get_mutable_continuous_state(&(state->get_mutable_continuous_state()));
const RimlessWheelParams<T>& params = get_parameters(context);
T& toe = get_mutable_toe_position(state);
const T alpha = calc_alpha(params);
// Stance foot changes to the new support leg.
next_state.set_theta(rw_state.theta() + 2. * alpha -
std::numeric_limits<T>::epsilon());
// Event isolation guarantees that the penetration was non-negative. We add
// epsilon because the post-impact state needs to be above the ground, so
// that the witness function evaluation that occurs immediately after the
// impact has a positive value (we have declared the WitnessFunction with
// kPositiveThenNonPositive). The failure mode before this improved
// implementation was the robot falling through the ground after an impact.
DRAKE_DEMAND(next_state.theta() < params.slope() + alpha);
// θ̇ ⇐ θ̇ cos(2α)
next_state.set_thetadot(rw_state.thetadot() * cos(2. * alpha));
// toe -= stance length.
toe -= 2. * params.length() * sin(alpha);
// If thetadot is very small, then transition to double support.
// Note: I already know that thetadot < 0 since the guard triggered.
DRAKE_ASSERT(next_state.thetadot() <= 0.);
// The threshold value below impacts early termination. Setting it closer to
// zero will not cause the wheel to miss an event, but will cause the
// simulator to perform arbitrarily more event detection calculations. If the
// threshold is smaller than Simulator::GetCurrentWitnessTimeIsolation(), it
// can result in an infinite loop (a numerical Zeno's paradox). The
// threshold is multiplied by sqrt(g/l) to make it dimensionless.
const T kThreshold = 0.01 * sqrt(params.gravity() / params.length());
if (next_state.thetadot() > -kThreshold) {
bool& double_support = get_mutable_double_support(state);
double_support = true;
next_state.set_thetadot(0.0);
}
}
template <typename T>
void RimlessWheel<T>::MinimalStateOut(
const systems::Context<T>& context,
RimlessWheelContinuousState<T>* output) const {
output->SetFromVector(get_continuous_state(context).CopyToVector());
}
template <typename T>
void RimlessWheel<T>::FloatingBaseStateOut(
const systems::Context<T>& context, systems::BasicVector<T>* output) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
const RimlessWheelParams<T>& params = get_parameters(context);
const T toe = get_toe_position(context);
const T alpha = calc_alpha(params);
// x, y, z.
output->SetAtIndex(
0, toe * cos(params.slope()) + params.length() * sin(rw_state.theta()));
output->SetAtIndex(1, 0.);
output->SetAtIndex(
2, -toe * sin(params.slope()) + params.length() * cos(rw_state.theta()));
// roll, pitch, yaw.
output->SetAtIndex(3, 0.);
// Unroll theta (add 2 alpha * number of steps, where number of steps = toe /
// stance length)
const T theta =
rw_state.theta() + alpha * toe / (params.length() * sin(alpha));
output->SetAtIndex(4, theta);
output->SetAtIndex(5, 0.);
// x, y, z derivatives.
output->SetAtIndex(
6, -rw_state.thetadot() * params.length() * cos(rw_state.theta()));
output->SetAtIndex(7, 0.);
output->SetAtIndex(
8, rw_state.thetadot() * params.length() * sin(rw_state.theta()));
// roll, pitch, yaw derivatives.
output->SetAtIndex(9, 0.);
output->SetAtIndex(10, rw_state.thetadot());
output->SetAtIndex(11, 0.);
}
template <typename T>
void RimlessWheel<T>::DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const {
const RimlessWheelContinuousState<T>& rw_state =
get_continuous_state(context);
const RimlessWheelParams<T>& params = get_parameters(context);
RimlessWheelContinuousState<T>& rw_derivatives =
get_mutable_continuous_state(derivatives);
const bool double_support = get_double_support(context);
// Handle double-support (to avoid simulating at the Zeno fixed point).
if (double_support) {
rw_derivatives.set_theta(0.0);
rw_derivatives.set_thetadot(0.0);
} else {
rw_derivatives.set_theta(rw_state.thetadot());
rw_derivatives.set_thetadot(sin(rw_state.theta()) * params.gravity() /
params.length());
}
}
template <typename T>
void RimlessWheel<T>::DoGetWitnessFunctions(
const systems::Context<T>& context,
std::vector<const systems::WitnessFunction<T>*>* witnesses) const {
const bool double_support = get_double_support(context);
if (!double_support) {
witnesses->push_back(step_backward_.get());
witnesses->push_back(step_forward_.get());
}
}
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
DRAKE_DEFINE_CLASS_TEMPLATE_INSTANTIATIONS_ON_DEFAULT_NONSYMBOLIC_SCALARS(
class ::drake::examples::rimless_wheel::RimlessWheel)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load(
"//tools/skylark:drake_cc.bzl",
"drake_cc_binary",
"drake_cc_googletest",
"drake_cc_library",
)
package(default_visibility = ["//visibility:private"])
drake_cc_library(
name = "rimless_wheel_vector_types",
srcs = [
"rimless_wheel_continuous_state.cc",
"rimless_wheel_params.cc",
],
hdrs = [
"gen/rimless_wheel_continuous_state.h",
"gen/rimless_wheel_params.h",
"rimless_wheel_continuous_state.h",
"rimless_wheel_params.h",
],
visibility = ["//visibility:public"],
deps = [
"//common:dummy_value",
"//common:essential",
"//common:name_value",
"//common/symbolic:expression",
"//systems/framework:vector",
],
)
drake_cc_library(
name = "rimless_wheel",
srcs = ["rimless_wheel.cc"],
hdrs = [
"rimless_wheel.h",
],
visibility = ["//visibility:public"],
deps = [
":rimless_wheel_vector_types",
"//common:default_scalars",
"//common:essential",
"//systems/framework:leaf_system",
],
)
drake_cc_library(
name = "rimless_wheel_geometry",
srcs = ["rimless_wheel_geometry.cc"],
hdrs = ["rimless_wheel_geometry.h"],
visibility = ["//visibility:public"],
deps = [
":rimless_wheel",
"//geometry:geometry_roles",
"//geometry:scene_graph",
"//math:geometric_transform",
"//systems/framework:diagram_builder",
"//systems/framework:leaf_system",
],
)
drake_cc_binary(
name = "simulate",
srcs = ["simulate.cc"],
add_test_rule = 1,
test_rule_args = ["--target_realtime_rate=0.0"],
deps = [
":rimless_wheel",
":rimless_wheel_geometry",
"//geometry:drake_visualizer",
"//systems/analysis:simulator",
"//systems/framework:diagram_builder",
"@gflags",
],
)
drake_cc_googletest(
name = "rimless_wheel_test",
deps = [
":rimless_wheel",
"//systems/analysis:simulator",
"//systems/framework/test_utilities:scalar_conversion",
],
)
drake_cc_googletest(
name = "rimless_wheel_geometry_test",
deps = [
":rimless_wheel",
":rimless_wheel_geometry",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_geometry.h | #pragma once
#include "drake/examples/rimless_wheel/rimless_wheel_params.h"
#include "drake/geometry/scene_graph.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
/// Expresses a RimlessWheel's geometry to a SceneGraph.
///
/// @system
/// name: RimlessWheelGeometry
/// input_ports:
/// - floating_base_state
/// output_ports:
/// - geometry_pose
/// @endsystem
///
/// This class has no public constructor; instead use the AddToBuilder() static
/// method to create and add it to a DiagramBuilder directly.
class RimlessWheelGeometry final : public systems::LeafSystem<double> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(RimlessWheelGeometry);
~RimlessWheelGeometry() final;
/// Creates, adds, and connects a RimlessWheelGeometry system into the given
/// `builder`. Both the `floating_base_state_port.get_system()`
/// and `scene_graph` systems must have been added to the given `builder`
/// already. The `rimless_wheel_params` sets the parameters of the
/// geometry registered with `scene_graph`.
///
/// The `scene_graph` pointer is not retained by the %RimlessWheelGeometry
/// system. The return value pointer is an alias of the new
/// %RimlessWheelGeometry system that is owned by the `builder`.
static const RimlessWheelGeometry* AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& floating_base_state_port,
const RimlessWheelParams<double>& rimless_wheel_params,
geometry::SceneGraph<double>* scene_graph);
/// Creates, adds, and connects a RimlessWheelGeometry system into the given
/// `builder`. Both the `floating_base_state_port.get_system()` and
/// `scene_graph` systems must have been added to the given `builder` already.
/// RimlessWheelParams are set to their default values.
///
/// The `scene_graph` pointer is not retained by the %RimlessWheelGeometry
/// system. The return value pointer is an alias of the new
/// %RimlessWheelGeometry system that is owned by the `builder`.
static const RimlessWheelGeometry* AddToBuilder(
systems::DiagramBuilder<double>* builder,
const systems::OutputPort<double>& floating_base_state_port,
geometry::SceneGraph<double>* scene_graph) {
return AddToBuilder(builder, floating_base_state_port,
RimlessWheelParams<double>(), scene_graph);
}
private:
RimlessWheelGeometry(const RimlessWheelParams<double>& rimless_wheel_params,
geometry::SceneGraph<double>*);
void OutputGeometryPose(const systems::Context<double>&,
geometry::FramePoseVector<double>*) const;
// Geometry source identifier for this system to interact with SceneGraph.
geometry::SourceId source_id_{};
geometry::FrameId frame_id_{};
};
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_continuous_state.h | #pragma once
// This file was previously auto-generated, but now is just a normal source
// file in git. However, it still retains some historical oddities from its
// heritage. In general, we do recommend against subclassing BasicVector in
// new code.
#include <cmath>
#include <limits>
#include <stdexcept>
#include <string>
#include <utility>
#include <vector>
#include <Eigen/Core>
#include "drake/common/drake_bool.h"
#include "drake/common/dummy_value.h"
#include "drake/common/name_value.h"
#include "drake/common/never_destroyed.h"
#include "drake/common/symbolic/expression.h"
#include "drake/systems/framework/basic_vector.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
/// Describes the row indices of a RimlessWheelContinuousState.
struct RimlessWheelContinuousStateIndices {
/// The total number of rows (coordinates).
static const int kNumCoordinates = 2;
// The index of each individual coordinate.
static const int kTheta = 0;
static const int kThetadot = 1;
/// Returns a vector containing the names of each coordinate within this
/// class. The indices within the returned vector matches that of this class.
/// In other words,
/// `RimlessWheelContinuousStateIndices::GetCoordinateNames()[i]` is the name
/// for `BasicVector::GetAtIndex(i)`.
static const std::vector<std::string>& GetCoordinateNames();
};
/// Specializes BasicVector with specific getters and setters.
template <typename T>
class RimlessWheelContinuousState final
: public drake::systems::BasicVector<T> {
public:
/// An abbreviation for our row index constants.
typedef RimlessWheelContinuousStateIndices K;
/// Default constructor. Sets all rows to their default value:
/// @arg @c theta defaults to 0.0 radians.
/// @arg @c thetadot defaults to 0.0 rad/sec.
RimlessWheelContinuousState()
: drake::systems::BasicVector<T>(K::kNumCoordinates) {
this->set_theta(0.0);
this->set_thetadot(0.0);
}
// Note: It's safe to implement copy and move because this class is final.
/// @name Implements CopyConstructible, CopyAssignable, MoveConstructible,
/// MoveAssignable
//@{
RimlessWheelContinuousState(const RimlessWheelContinuousState& other)
: drake::systems::BasicVector<T>(other.values()) {}
RimlessWheelContinuousState(RimlessWheelContinuousState&& other) noexcept
: drake::systems::BasicVector<T>(std::move(other.values())) {}
RimlessWheelContinuousState& operator=(
const RimlessWheelContinuousState& other) {
this->values() = other.values();
return *this;
}
RimlessWheelContinuousState& operator=(
RimlessWheelContinuousState&& other) noexcept {
this->values() = std::move(other.values());
other.values().resize(0);
return *this;
}
//@}
/// Create a symbolic::Variable for each element with the known variable
/// name. This is only available for T == symbolic::Expression.
template <typename U = T>
typename std::enable_if_t<std::is_same_v<U, symbolic::Expression>>
SetToNamedVariables() {
this->set_theta(symbolic::Variable("theta"));
this->set_thetadot(symbolic::Variable("thetadot"));
}
[[nodiscard]] RimlessWheelContinuousState<T>* DoClone() const final {
return new RimlessWheelContinuousState;
}
/// @name Getters and Setters
//@{
/// The orientation of the stance leg, measured clockwise from the vertical
/// axis.
/// @note @c theta is expressed in units of radians.
const T& theta() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kTheta);
}
/// Setter that matches theta().
void set_theta(const T& theta) {
ThrowIfEmpty();
this->SetAtIndex(K::kTheta, theta);
}
/// Fluent setter that matches theta().
/// Returns a copy of `this` with theta set to a new value.
[[nodiscard]] RimlessWheelContinuousState<T> with_theta(
const T& theta) const {
RimlessWheelContinuousState<T> result(*this);
result.set_theta(theta);
return result;
}
/// The angular velocity of the stance leg.
/// @note @c thetadot is expressed in units of rad/sec.
const T& thetadot() const {
ThrowIfEmpty();
return this->GetAtIndex(K::kThetadot);
}
/// Setter that matches thetadot().
void set_thetadot(const T& thetadot) {
ThrowIfEmpty();
this->SetAtIndex(K::kThetadot, thetadot);
}
/// Fluent setter that matches thetadot().
/// Returns a copy of `this` with thetadot set to a new value.
[[nodiscard]] RimlessWheelContinuousState<T> with_thetadot(
const T& thetadot) const {
RimlessWheelContinuousState<T> result(*this);
result.set_thetadot(thetadot);
return result;
}
//@}
/// Visit each field of this named vector, passing them (in order) to the
/// given Archive. The archive can read and/or write to the vector values.
/// One common use of Serialize is the //common/yaml tools.
template <typename Archive>
void Serialize(Archive* a) {
T& theta_ref = this->GetAtIndex(K::kTheta);
a->Visit(drake::MakeNameValue("theta", &theta_ref));
T& thetadot_ref = this->GetAtIndex(K::kThetadot);
a->Visit(drake::MakeNameValue("thetadot", &thetadot_ref));
}
/// See RimlessWheelContinuousStateIndices::GetCoordinateNames().
static const std::vector<std::string>& GetCoordinateNames() {
return RimlessWheelContinuousStateIndices::GetCoordinateNames();
}
/// Returns whether the current values of this vector are well-formed.
drake::boolean<T> IsValid() const {
using std::isnan;
drake::boolean<T> result{true};
result = result && !isnan(theta());
result = result && !isnan(thetadot());
return result;
}
private:
void ThrowIfEmpty() const {
if (this->values().size() == 0) {
throw std::out_of_range(
"The RimlessWheelContinuousState vector has been moved-from; "
"accessor methods may no longer be used");
}
}
};
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel.h | #pragma once
#include <memory>
#include <vector>
#include "drake/examples/rimless_wheel/rimless_wheel_continuous_state.h"
#include "drake/examples/rimless_wheel/rimless_wheel_params.h"
#include "drake/systems/framework/event.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/framework/scalar_conversion_traits.h"
#include "drake/systems/framework/witness_function.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
/// Dynamical representation of the idealized hybrid dynamics of a "rimless
/// wheel", as described in
/// http://underactuated.mit.edu/underactuated.html?chapter=simple_legs
/// In addition, this model has two additional (discrete) state variables that
/// are not required in the mathematical model:
///
/// - the position of the stance toe along the ramp (helpful for outputting
/// a floating-base model coordinate, e.g. for visualization),
/// - a boolean indicator for "double support" (to avoid the numerical
/// challenges of simulation around the Zeno phenomenon at the standing
/// fixed point).
///
/// @system
/// name: RimlessWheel
/// output_ports:
/// - minimal_state
/// - floating_base_state
/// @endsystem
///
/// Continuous States: theta, thetadot (emitted at `minimal_state` port).
/// Discrete States: stance toe position (emitted at `floating_base_state`
/// port), double support indicator.
/// Parameters: mass, length, number of spokes, etc, are all set as Context
/// parameters using RimlessWheelParams.
///
/// @tparam_nonsymbolic_scalar
template <typename T>
class RimlessWheel final : public systems::LeafSystem<T> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(RimlessWheel);
/// Constructs the plant.
RimlessWheel();
/// Scalar-converting copy constructor. See @ref system_scalar_conversion.
template <typename U>
explicit RimlessWheel(const RimlessWheel<U>&) : RimlessWheel<T>() {}
/// Return reference to the output port that publishes only [theta,
/// thetatdot].
const systems::OutputPort<T>& get_minimal_state_output_port() const {
return this->get_output_port(0);
}
/// Returns reference to the output port that provides a 12 dimensional state
/// (FloatingBaseType::kRollPitchYaw positions then velocities). This is
/// useful, e.g., for visualization. θ of the rimless wheel is the pitch
/// of the floating base (rotation around global y), and downhill moves toward
/// positive x. As always, we use vehicle coordinates (x-y on the ground, z
/// is up).
const systems::OutputPort<T>& get_floating_base_state_output_port() const {
return this->get_output_port(1);
}
/// Access the RimlessWheelContinuousState.
static const RimlessWheelContinuousState<T>& get_continuous_state(
const systems::ContinuousState<T>& cstate) {
return dynamic_cast<const RimlessWheelContinuousState<T>&>(
cstate.get_vector());
}
/// Access the RimlessWheelContinuousState.
static const RimlessWheelContinuousState<T>& get_continuous_state(
const systems::Context<T>& context) {
return get_continuous_state(context.get_continuous_state());
}
/// Access the mutable RimlessWheelContinuousState.
static RimlessWheelContinuousState<T>& get_mutable_continuous_state(
systems::ContinuousState<T>* cstate) {
return dynamic_cast<RimlessWheelContinuousState<T>&>(
cstate->get_mutable_vector());
}
/// Access the mutable RimlessWheelContinuousState.
static RimlessWheelContinuousState<T>& get_mutable_continuous_state(
systems::Context<T>* context) {
return get_mutable_continuous_state(
&context->get_mutable_continuous_state());
}
static const T& get_toe_position(const systems::Context<T>& context) {
return context.get_discrete_state(0).GetAtIndex(0);
}
static T& get_mutable_toe_position(systems::State<T>* state) {
return state->get_mutable_discrete_state().get_mutable_vector(0).GetAtIndex(
0);
}
static bool get_double_support(const systems::Context<T>& context) {
return context.template get_abstract_state<bool>(0);
}
static bool& get_mutable_double_support(systems::State<T>* state) {
return state->template get_mutable_abstract_state<bool>(0);
}
/// Access the RimlessWheelParams.
const RimlessWheelParams<T>& get_parameters(
const systems::Context<T>& context) const {
return this->template GetNumericParameter<RimlessWheelParams>(context, 0);
}
/// Alpha is half the interleg angle, and is used frequently.
static T calc_alpha(const RimlessWheelParams<T>& params) {
return M_PI / params.number_of_spokes();
}
/// Calculates the kinetic + potential energy.
T CalcTotalEnergy(const systems::Context<T>& context) const;
private:
// Check when the foot hits the ramp (rolling downhill). This occurs when
// θ = slope + the interleg angle (α).
T StepForwardGuard(const systems::Context<T>& context) const;
// Handles the impact dynamics, including resetting theta to the angle of the
// new stance leg, and updating the toe position by the step length.
void StepForwardReset(const systems::Context<T>& context,
const systems::UnrestrictedUpdateEvent<T>&,
systems::State<T>* state) const;
// Check when the foot hits the ramp (rolling uphill). This occurs when
// θ = slope - the interleg angle (α).
T StepBackwardGuard(const systems::Context<T>& context) const;
// Handles the impact dynamics, including resetting theta to the angle of the
// new stance leg, and updating the toe position by the step length.
void StepBackwardReset(const systems::Context<T>& context,
const systems::UnrestrictedUpdateEvent<T>&,
systems::State<T>* state) const;
void MinimalStateOut(const systems::Context<T>& context,
RimlessWheelContinuousState<T>* output) const;
void FloatingBaseStateOut(const systems::Context<T>& context,
systems::BasicVector<T>* output) const;
// Implements the simple pendulum dynamics during stance.
void DoCalcTimeDerivatives(
const systems::Context<T>& context,
systems::ContinuousState<T>* derivatives) const override;
void DoGetWitnessFunctions(const systems::Context<T>&,
std::vector<const systems::WitnessFunction<T>*>*
witnesses) const override;
// The system stores its witness functions internally.
std::unique_ptr<systems::WitnessFunction<T>> step_backward_;
std::unique_ptr<systems::WitnessFunction<T>> step_forward_;
};
} // namespace rimless_wheel
} // namespace examples
// Explicitly disable symbolic::Expression (for now).
namespace systems {
namespace scalar_conversion {
template <>
struct Traits<examples::rimless_wheel::RimlessWheel>
: public NonSymbolicTraits {};
} // namespace scalar_conversion
} // namespace systems
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/rimless_wheel_params.cc | #include "drake/examples/rimless_wheel/rimless_wheel_params.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
const int RimlessWheelParamsIndices::kNumCoordinates;
const int RimlessWheelParamsIndices::kMass;
const int RimlessWheelParamsIndices::kLength;
const int RimlessWheelParamsIndices::kGravity;
const int RimlessWheelParamsIndices::kNumberOfSpokes;
const int RimlessWheelParamsIndices::kSlope;
const std::vector<std::string>&
RimlessWheelParamsIndices::GetCoordinateNames() {
static const drake::never_destroyed<std::vector<std::string>> coordinates(
std::vector<std::string>{
"mass",
"length",
"gravity",
"number_of_spokes",
"slope",
});
return coordinates.access();
}
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples | /home/johnshepherd/drake/examples/rimless_wheel/simulate.cc | #include <memory>
#include <gflags/gflags.h>
#include "drake/examples/rimless_wheel/rimless_wheel.h"
#include "drake/examples/rimless_wheel/rimless_wheel_geometry.h"
#include "drake/geometry/drake_visualizer.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
namespace {
DEFINE_double(accuracy, 1e-4, "Accuracy of the rimless wheel system (unitless);"
" must be positive.");
DEFINE_double(initial_angle, 0.0, "Initial angle of the wheel (rad). Must be"
" in the interval (slope - alpha, slope + alpha), as described in "
"http://underactuated.mit.edu/underactuated.html?chapter=simple_legs .");
DEFINE_double(initial_angular_velocity, 5.0,
"Initial angular velocity of the wheel (rad/sec).");
DEFINE_double(target_realtime_rate, 1.0,
"Playback speed. See documentation for "
"Simulator::set_target_realtime_rate() for details.");
/// Simulates the rimless wheel from various initial velocities (accepted as
/// command-line arguments. Run meldis to watch the results.
int DoMain() {
systems::DiagramBuilder<double> builder;
auto rimless_wheel = builder.AddSystem<RimlessWheel>();
rimless_wheel->set_name("rimless_wheel");
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
RimlessWheelGeometry::AddToBuilder(
&builder, rimless_wheel->get_floating_base_state_output_port(),
scene_graph);
geometry::DrakeVisualizerd::AddToBuilder(&builder, *scene_graph);
auto diagram = builder.Build();
systems::Simulator<double> simulator(*diagram);
systems::Context<double>& rw_context = diagram->GetMutableSubsystemContext(
*rimless_wheel, &simulator.get_mutable_context());
RimlessWheelContinuousState<double>& state =
rimless_wheel->get_mutable_continuous_state(&rw_context);
// Check that command line argument puts the wheel above the ground.
const RimlessWheelParams<double>& params =
rimless_wheel->get_parameters(rw_context);
const double alpha = rimless_wheel->calc_alpha(params);
DRAKE_DEMAND(FLAGS_initial_angle > params.slope() - alpha);
DRAKE_DEMAND(FLAGS_initial_angle < params.slope() + alpha);
state.set_theta(FLAGS_initial_angle);
state.set_thetadot(FLAGS_initial_angular_velocity);
simulator.set_target_realtime_rate(FLAGS_target_realtime_rate);
simulator.get_mutable_context().SetAccuracy(FLAGS_accuracy);
simulator.AdvanceTo(10);
// Check that the state is still inside the expected region (I did not miss
// any collisions).
DRAKE_DEMAND(state.theta() >= params.slope() - alpha);
DRAKE_DEMAND(state.theta() <= params.slope() + alpha);
return 0;
}
} // namespace
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::rimless_wheel::DoMain();
}
| 0 |
/home/johnshepherd/drake/examples/rimless_wheel | /home/johnshepherd/drake/examples/rimless_wheel/gen/rimless_wheel_params.h | #pragma once
#include "drake/examples/rimless_wheel/rimless_wheel_params.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/rimless_wheel | /home/johnshepherd/drake/examples/rimless_wheel/gen/rimless_wheel_continuous_state.h | #pragma once
#include "drake/examples/rimless_wheel/rimless_wheel_continuous_state.h"
// clang-format off
// NOLINTNEXTLINE
#warning "DRAKE_DEPRECATED: This include path is deprecated and will be removed on or after 2024-09-01. Remove the /gen/ part of your code's include statement."
// clang-format on
| 0 |
/home/johnshepherd/drake/examples/rimless_wheel | /home/johnshepherd/drake/examples/rimless_wheel/test/rimless_wheel_test.cc | #include "drake/examples/rimless_wheel/rimless_wheel.h"
#include <gtest/gtest.h>
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/framework/test_utilities/scalar_conversion.h"
using std::cos;
using std::sin;
using std::sqrt;
namespace drake {
namespace examples {
namespace rimless_wheel {
namespace {
GTEST_TEST(RimlessWheelTest, ToAutoDiff) {
RimlessWheel<double> rw;
EXPECT_TRUE(is_autodiffxd_convertible(rw));
}
// Given the desired energy and theta, compute thetadot.
void set_thetadot_to_achieve_energy(const RimlessWheel<double>& rw,
double desired_energy,
systems::Context<double>* context) {
RimlessWheelContinuousState<double>& state =
rw.get_mutable_continuous_state(context);
const RimlessWheelParams<double>& params = rw.get_parameters(*context);
state.set_thetadot(0.0);
const double energy = rw.CalcTotalEnergy(*context);
// Note: returns the positive solution. (-thetadot will have the same
// energy).
state.set_thetadot(sqrt(2. * (desired_energy - energy) /
(params.mass() * params.length() * params.length())));
}
// Simulate across one (foot)step and check against analytical solutions.
GTEST_TEST(RimlessWheelTest, StepTest) {
const RimlessWheel<double> rw;
systems::Simulator<double> simulator(rw);
auto& context = simulator.get_mutable_context();
context.SetAccuracy(1e-8);
RimlessWheelContinuousState<double>& state =
rw.get_mutable_continuous_state(&context);
double& toe = rw.get_mutable_toe_position(&context.get_mutable_state());
bool& double_support =
rw.get_mutable_double_support(&context.get_mutable_state());
const RimlessWheelParams<double>& params = rw.get_parameters(context);
const double alpha = rw.calc_alpha(params);
// From the course notes, the rolling fixed point is at:
state.set_theta(params.slope() - alpha);
state.set_thetadot(cos(2 * alpha) / sin(2 * alpha) *
sqrt(4 * params.gravity() / params.length() * sin(alpha) *
sin(params.slope())));
const double steady_state_energy = rw.CalcTotalEnergy(context);
const double step_length = 2 * params.length() * sin(alpha);
// A point on that limit cycle just before a forward step:
context.SetTime(0.0);
state.set_theta(params.slope() + alpha / 2.0);
toe = 0.0;
double_support = false;
set_thetadot_to_achieve_energy(rw, steady_state_energy, &context);
simulator.AdvanceTo(.2);
// Theta should now be on the other side of zero.
EXPECT_LT(state.theta(), 0.0);
// Should have taken one step forward.
EXPECT_NEAR(toe, step_length, 1e-8);
// Should still have the same energy (dissipation energy lost = potential
// energy gained). The error tolerance below seems to work well.
EXPECT_NEAR(rw.CalcTotalEnergy(context), steady_state_energy, 1e-5);
// Floating-base state should unroll theta (pitch).
Eigen::VectorXd floating_base_state =
rw.get_floating_base_state_output_port().Eval(context);
EXPECT_GT(floating_base_state[4], params.slope() + alpha);
// Lose at least this much energy (chosen as an arbitrary small positive
// number).
const double threshold = 0.01;
// Walking too fast should lose energy through impact.
context.SetTime(0.0);
state.set_theta(params.slope() + alpha / 2.0);
toe = 0.0;
double_support = false;
set_thetadot_to_achieve_energy(rw, steady_state_energy, &context);
state.set_thetadot(state.thetadot() + 0.2);
double initial_energy = rw.CalcTotalEnergy(context);
simulator.Initialize();
simulator.AdvanceTo(.2);
// Theta should now be on the other side of zero.
EXPECT_LT(state.theta(), 0.0);
// Should have taken one step forward.
EXPECT_NEAR(toe, step_length, 1e-8);
// Should have lost total energy on collision.
EXPECT_LT(rw.CalcTotalEnergy(context), initial_energy - threshold);
// Walking too slow should gain energy through impact.
context.SetTime(0.0);
state.set_theta(params.slope() + alpha / 2.0);
toe = 0.0;
double_support = false;
set_thetadot_to_achieve_energy(rw, steady_state_energy, &context);
state.set_thetadot(state.thetadot() - 0.2);
initial_energy = rw.CalcTotalEnergy(context);
simulator.Initialize();
simulator.AdvanceTo(.2);
// Theta should now be on the other side of zero.
EXPECT_LT(state.theta(), 0.);
// Should have taken one step forward.
EXPECT_NEAR(toe, step_length, 1e-8);
// Should have gained total energy on collision.
EXPECT_GT(rw.CalcTotalEnergy(context), initial_energy + threshold);
// Rolling uphill should always lose energy.
context.SetTime(0.0);
// Leaning uphill.
state.set_theta(params.slope() - alpha / 2.0);
state.set_thetadot(-4.);
toe = 0.0;
double_support = false;
simulator.Initialize();
initial_energy = rw.CalcTotalEnergy(context);
simulator.AdvanceTo(.2);
// Theta should now be on the other side of zero.
EXPECT_GT(state.theta(), 0.);
// Should have taken one step backward.
EXPECT_NEAR(toe, -step_length, 1e-8);
// Should have gained total energy on collision.
EXPECT_LT(rw.CalcTotalEnergy(context), initial_energy + 0.1);
}
// Check that the double-support logic makes the standing fixed point a fixed
// point.
GTEST_TEST(RimlessWheelTest, FixedPointTest) {
const RimlessWheel<double> rw;
systems::Simulator<double> simulator(rw);
auto& context = simulator.get_mutable_context();
context.SetAccuracy(1e-8);
RimlessWheelContinuousState<double>& state =
rw.get_mutable_continuous_state(&context);
double& toe = rw.get_mutable_toe_position(&context.get_mutable_state());
bool& double_support =
rw.get_mutable_double_support(&context.get_mutable_state());
const RimlessWheelParams<double>& params = rw.get_parameters(context);
const double alpha = rw.calc_alpha(params);
// Start the foot just above the ground (in each direction). Chosen as
// positive number that is sufficiently small to trigger double support on
// first collision.
const double angle_above_touchdown = 1e-5;
// Front foot down.
context.SetTime(0.0);
state.set_theta(params.slope() + alpha - angle_above_touchdown);
state.set_thetadot(0.0);
toe = 0.0;
double_support = false;
simulator.AdvanceTo(0.2);
EXPECT_TRUE(double_support);
EXPECT_NEAR(std::abs(state.theta() - params.slope()), alpha, 1e-8);
EXPECT_EQ(state.thetadot(), 0.0);
// Back foot down.
context.SetTime(0.0);
state.set_theta(params.slope() - alpha + angle_above_touchdown);
state.set_thetadot(0.0);
toe = 0.0;
double_support = false;
simulator.Initialize();
simulator.AdvanceTo(0.2);
EXPECT_TRUE(double_support);
EXPECT_NEAR(std::abs(state.theta() - params.slope()), alpha, 1e-8);
EXPECT_EQ(state.thetadot(), 0.0);
}
// Use the integrator's dense output to check the details of integration.
GTEST_TEST(RimlessWheelTest, DenseOutput) {
const RimlessWheel<double> rw;
systems::Simulator<double> simulator(rw);
auto& context = simulator.get_mutable_context();
context.SetAccuracy(1e-8);
auto& integrator = simulator.get_mutable_integrator();
RimlessWheelContinuousState<double>& state =
rw.get_mutable_continuous_state(&context);
double& toe = rw.get_mutable_toe_position(&context.get_mutable_state());
const RimlessWheelParams<double>& params = rw.get_parameters(context);
const double alpha = rw.calc_alpha(params);
// A point that is just before a forward step:
state.set_theta(params.slope() + alpha / 2.0);
state.set_thetadot(1.);
toe = 0.0;
integrator.StartDenseIntegration();
simulator.AdvanceTo(.2);
const std::unique_ptr<trajectories::PiecewisePolynomial<double>>
dense_output = integrator.StopDenseIntegration();
// Check that I indeed took a step.
EXPECT_GE(toe, 0.0);
// Ensure that I can find the discontinuity in the dense output.
int num_forward_steps = 0;
const double kEpsilon = std::numeric_limits<double>::epsilon();
for (const double time : dense_output->get_segment_times()) {
// We have theta > 0 before impact, and theta < 0 after the impact.
if (dense_output->value(time - kEpsilon)(0) > 0 &&
dense_output->value(time + kEpsilon)(0) < 0) {
num_forward_steps++;
}
}
EXPECT_EQ(num_forward_steps, 1);
}
} // namespace
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/rimless_wheel | /home/johnshepherd/drake/examples/rimless_wheel/test/rimless_wheel_geometry_test.cc | #include "drake/examples/rimless_wheel/rimless_wheel_geometry.h"
#include <gtest/gtest.h>
#include "drake/examples/rimless_wheel/rimless_wheel.h"
#include "drake/geometry/scene_graph.h"
#include "drake/systems/framework/diagram_builder.h"
namespace drake {
namespace examples {
namespace rimless_wheel {
namespace {
GTEST_TEST(RimlessWheelGeometryTest, AcceptanceTest) {
// Just make sure nothing faults out.
systems::DiagramBuilder<double> builder;
auto plant = builder.AddSystem<RimlessWheel>();
auto scene_graph = builder.AddSystem<geometry::SceneGraph>();
auto geom = RimlessWheelGeometry::AddToBuilder(
&builder, plant->get_floating_base_state_output_port(), scene_graph);
auto diagram = builder.Build();
ASSERT_NE(geom, nullptr);
}
} // namespace
} // namespace rimless_wheel
} // namespace examples
} // namespace drake
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/spatula_slip_control/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load("//tools/skylark:drake_cc.bzl", "drake_cc_binary")
load("//tools/skylark:drake_data.bzl", "models_filegroup")
package(default_visibility = ["//visibility:private"])
models_filegroup(
name = "glob_models",
glob_exclude = ["images/**/*"],
)
filegroup(
name = "models",
srcs = [
":glob_models",
],
visibility = [
"//:__pkg__",
"//bindings/pydrake/visualization:__pkg__",
],
)
drake_cc_binary(
name = "spatula_slip_control",
srcs = ["spatula_slip_control.cc"],
add_test_rule = True,
data = [
":models",
"@drake_models//:wsg_50_description",
],
test_rule_args = [
"--simulation_sec=0.1",
"--realtime_rate=0.0",
],
deps = [
"//multibody/parsing",
"//multibody/plant:multibody_plant_config_functions",
"//systems/analysis:simulator",
"//systems/analysis:simulator_config_functions",
"//systems/analysis:simulator_gflags",
"//systems/analysis:simulator_print_stats",
"//systems/framework:diagram",
"//systems/primitives",
"//visualization",
"@gflags",
],
)
add_lint_tests(enable_clang_format_lint = False)
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/spatula_slip_control/README.md | # Spatula Slip Control
This is an example of using the hydroelastic contact model with a
robot gripper with compliant bubble fingers and a compliant spatula handle.
This example is described in the paper
[Discrete Approximation of Pressure Field Contact Patches](https://arxiv.org/abs/2110.04157)
and a discussion of the example can be found in
[the accompanying video](https://youtu.be/TOsd5LAEPmU?t=85) at 1:25.
The example poses the spatula in the closed grip of the gripper and
uses an open loop square wave controller to perform a controlled
rotational slip of the spatula while maintaining the spatula in
the gripper's grasp. This demonstrates that the hydroelastic contact patch
captures important behavior missed in point contact -- in this case,
pressure-dependent torsional friction emerges naturally.
In the source code, this example shows how to set up bodies by loading SDFormat
files and also calling C++ APIs.


## Run the example
```
bazel run //examples/hydroelastic/spatula_slip_control:spatula_slip_control
```
Use the MeshCat URL from the console log messages for visualization. In
the example console output below, the last line contains the log message
with the MeshCat URL `http://localhost:7002`.
```
drake (master)$ bazel run //examples/hydroelastic/spatula_slip_control:spatula_slip_control
INFO: Analyzed target //examples/hydroelastic/spatula_slip_control:spatula_slip_control (0 packages loaded, 0 targets configured).
INFO: Found 1 target...
Target //examples/hydroelastic/spatula_slip_control:spatula_slip_control up-to-date:
bazel-bin/examples/hydroelastic/spatula_slip_control/spatula_slip_control
INFO: Elapsed time: 0.428s, Critical Path: 0.00s
INFO: 1 process: 1 internal.
INFO: Build completed successfully, 1 total action
INFO: Running command line: bazel-bin/examples/hydroelastic/spatula_slip_control/spatula_slip_control
[2023-11-27 15:10:48.768] [console] [info] Meshcat listening for connections at http://localhost:7002
```
Please note that other examples may instruct users to run `meldis` for
visualization. However, this example recommends using the in-process MeshCat
URL from the log messages because it supports Animations playback (see the
section *Playback simulation* below). You can use `meldis` with this example,
but it will not show Animations playback when the simulation finishes.
Animations playback comes from code like this in
[spatula_slip_control.cc](https://github.com/RobotLocomotion/drake/blob/master/examples/hydroelastic/spatula_slip_control/spatula_slip_control.cc):
```C++
meshcat->StartRecording();
simulator.AdvanceTo(FLAGS_simulation_sec);
meshcat->StopRecording();
meshcat->PublishRecording();
```
## Illustration and Collision geometries
In Meshcat, you can view illustration geometries or
collision (aka, proximity) geometries or both as shown
in the following picture.
Click on `illustration` or `proximity` as you wish.

## Playback simulation
After the simulation ends, it will publish the result for playback to Meshcat.
In Meshcat, you can use `Animations/play` or `pause` or `reset` to control
the playback.
## Use polygon or triangle contact surfaces
This example provides the option for either triangulated or polygonal
contact surface representation. The polygonal representation of a contact
surface contains significantly fewer elements which in practice corresponds
to a more efficient simulation, though may produce unwanted artifacts when
working with very coarse meshes. By default, this example uses polygonal
contact surfaces. Refer to the
[Hydroelastic User Guide](https://drake.mit.edu/doxygen_cxx/group__hydroelastic__user__guide.html)
for more details about hydroelastic representations. You can also try a more
conventional point-contact model by specifying `--contact_model=point` on the
command line.
The option `--contact_surface_representation=triangle` specifies triangle
contact surfaces:
```
bazel run //examples/hydroelastic/spatula_slip_control:spatula_slip_control \
-- --contact_surface_representation=triangle
```
**WARNING**: Using a more dense contact surface representation may cause simulation
convergence failures. Using a smaller step size can help in convergence:
```
bazel run //examples/hydroelastic/spatula_slip_control:spatula_slip_control \
-- --contact_surface_representation=triangle --mbp_discrete_update_period=5e-3
```
**WARNING**: According to issue
[17681](https://github.com/RobotLocomotion/drake/issues/17681),
neither MeshCat nor Meldis can draw wireframe of the contact surfaces.
As a result, you cannot currently see the difference between the `triangle`
option and the default `polygon` option.
## Other Options
There are other command-line options that you can use. Use `--help` to see
the list. `gripper_force, amplitude, frequency, pulse_width, period` are all
parameters that affect the control signal of the gripper. It is tuned to show
a controlled slip where when the gripper is fully engaged the spatula is in
stiction. Turning down the `amplitude` parameter will affect when and if the
spatula is in stiction. Other options affect the parameters of the
MultibodyPlant running the dynamics as well as the Simulator running the
simulation.
```
bazel run //examples/hydroelastic/spatula_slip_control:spatula_slip_control \
-- --help
```
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/spatula_slip_control/spatula_slip_control.cc | #include <gflags/gflags.h>
#include "drake/common/drake_copyable.h"
#include "drake/common/eigen_types.h"
#include "drake/multibody/parsing/parser.h"
#include "drake/multibody/plant/multibody_plant_config_functions.h"
#include "drake/multibody/tree/prismatic_joint.h"
#include "drake/systems/analysis/simulator.h"
#include "drake/systems/analysis/simulator_config_functions.h"
#include "drake/systems/analysis/simulator_print_stats.h"
#include "drake/systems/framework/diagram_builder.h"
#include "drake/systems/framework/leaf_system.h"
#include "drake/systems/primitives/adder.h"
#include "drake/systems/primitives/constant_vector_source.h"
#include "drake/visualization/visualization_config.h"
#include "drake/visualization/visualization_config_functions.h"
// Parameters for squeezing the spatula.
DEFINE_double(gripper_force, 1,
"The baseline force to be applied by the gripper. [N].");
DEFINE_double(amplitude, 5,
"The amplitude of the oscillations "
"carried out by the gripper. [N].");
DEFINE_double(duty_cycle, 0.5, "Duty cycle of the control signal.");
DEFINE_double(period, 3, "Period of the control signal. [s].");
// MultibodyPlant settings.
DEFINE_double(stiction_tolerance, 1e-4, "Default stiction tolerance. [m/s].");
DEFINE_double(mbp_discrete_update_period, 4.0e-2,
"If zero, the plant is modeled as a continuous system. "
"If positive, the period (in seconds) of the discrete updates "
"for the plant modeled as a discrete system."
"This parameter must be non-negative.");
DEFINE_string(contact_model, "hydroelastic",
"Contact model. Options are: 'point', 'hydroelastic', "
"'hydroelastic_with_fallback'.");
DEFINE_string(contact_surface_representation, "polygon",
"Contact-surface representation for hydroelastics. "
"Options are: 'triangle' or 'polygon'.");
DEFINE_string(contact_approximation, "tamsi",
"Discrete contact approximation. Options are: 'tamsi', "
"'sap', 'similar', 'lagged'");
// Simulator settings.
DEFINE_double(realtime_rate, 1,
"Desired rate of the simulation compared to realtime."
"A value of 1 indicates real time.");
DEFINE_double(simulation_sec, 30, "Number of seconds to simulate. [s].");
// The following flags are only in effect in continuous mode.
DEFINE_double(accuracy, 1.0e-3, "The integration accuracy.");
DEFINE_double(max_time_step, 1.0e-2,
"The maximum time step the integrator is allowed to take, [s].");
DEFINE_string(integration_scheme, "implicit_euler",
"Integration scheme to be used. Available options are: "
"'semi_explicit_euler','runge_kutta2','runge_kutta3',"
"'implicit_euler'");
namespace drake {
using geometry::SceneGraph;
using math::RigidTransform;
using math::RollPitchYaw;
using multibody::MultibodyPlant;
using multibody::MultibodyPlantConfig;
using multibody::PrismaticJoint;
using systems::ApplySimulatorConfig;
using systems::BasicVector;
using systems::Context;
using systems::SimulatorConfig;
namespace examples {
namespace spatula_slip_control {
namespace {
// We create a simple leaf system that outputs a square wave signal for our
// open loop controller. The Square system here supports an arbitrarily
// dimensional signal, but we will use a 2-dimensional signal for our gripper.
class Square final : public systems::LeafSystem<double> {
public:
DRAKE_NO_COPY_NO_MOVE_NO_ASSIGN(Square)
// Constructs a %Square system where different amplitudes, duty cycles,
// periods, and phases can be applied to each square wave.
//
// @param[in] amplitudes the square wave amplitudes. (unitless)
// @param[in] duty_cycles the square wave duty cycles.
// (ratio of pulse duration to period of the waveform)
// @param[in] periods the square wave periods. (seconds)
// @param[in] phases the square wave phases. (radians)
Square(const Eigen::VectorXd& amplitudes, const Eigen::VectorXd& duty_cycles,
const Eigen::VectorXd& periods, const Eigen::VectorXd& phases)
: amplitude_(amplitudes),
duty_cycle_(duty_cycles),
period_(periods),
phase_(phases) {
// Ensure the incoming vectors are all the same size.
DRAKE_THROW_UNLESS(duty_cycles.size() == amplitudes.size());
DRAKE_THROW_UNLESS(duty_cycles.size() == periods.size());
DRAKE_THROW_UNLESS(duty_cycles.size() == phases.size());
this->DeclareVectorOutputPort("Square Wave Output", duty_cycles.size(),
&Square::CalcValueOutput);
}
private:
void CalcValueOutput(const Context<double>& context,
BasicVector<double>* output) const {
Eigen::VectorBlock<VectorX<double>> output_block =
output->get_mutable_value();
const double time = context.get_time();
for (int i = 0; i < duty_cycle_.size(); ++i) {
// Add phase offset.
double t = time + (period_[i] * phase_[i] / (2 * M_PI));
output_block[i] =
amplitude_[i] *
(t - floor(t / period_[i]) * period_[i] < duty_cycle_[i] * period_[i]
? 1
: 0);
}
}
const Eigen::VectorXd amplitude_;
const Eigen::VectorXd duty_cycle_;
const Eigen::VectorXd period_;
const Eigen::VectorXd phase_;
};
int DoMain() {
// Construct a MultibodyPlant and a SceneGraph.
systems::DiagramBuilder<double> builder;
MultibodyPlantConfig plant_config;
plant_config.time_step = FLAGS_mbp_discrete_update_period;
plant_config.stiction_tolerance = FLAGS_stiction_tolerance;
plant_config.contact_model = FLAGS_contact_model;
plant_config.discrete_contact_approximation = FLAGS_contact_approximation;
plant_config.contact_surface_representation =
FLAGS_contact_surface_representation;
DRAKE_DEMAND(FLAGS_mbp_discrete_update_period >= 0);
auto [plant, scene_graph] =
multibody::AddMultibodyPlant(plant_config, &builder);
// Parse the gripper and spatula models.
multibody::Parser parser(&plant, &scene_graph);
parser.AddModelsFromUrl(
"package://drake_models/wsg_50_description/sdf/"
"schunk_wsg_50_hydro_bubble.sdf");
parser.AddModelsFromUrl(
"package://drake/examples/hydroelastic/spatula_slip_control/"
"spatula.sdf");
// Pose the gripper and weld it to the world.
const math::RigidTransform<double> X_WF0 = math::RigidTransform<double>(
math::RollPitchYaw(0.0, -1.57, 0.0), Eigen::Vector3d(0, 0, 0.25));
plant.WeldFrames(plant.world_frame(), plant.GetFrameByName("gripper"), X_WF0);
plant.Finalize();
// Construct the open loop square wave controller. To oscillate around a
// constant force, we construct a ConstantVectorSource and combine it with
// the square wave output using an Adder.
const double f0 = FLAGS_gripper_force;
const Eigen::Vector2d amplitudes(FLAGS_amplitude, -FLAGS_amplitude);
const Eigen::Vector2d duty_cycles(FLAGS_duty_cycle, FLAGS_duty_cycle);
const Eigen::Vector2d periods(FLAGS_period, FLAGS_period);
const Eigen::Vector2d phases(0, 0);
const auto& square_force =
*builder.AddSystem<Square>(amplitudes, duty_cycles, periods, phases);
const auto& constant_force =
*builder.AddSystem<systems::ConstantVectorSource<double>>(
Eigen::Vector2d(f0, -f0));
const auto& adder = *builder.AddSystem<systems::Adder<double>>(2, 2);
builder.Connect(square_force.get_output_port(), adder.get_input_port(0));
builder.Connect(constant_force.get_output_port(), adder.get_input_port(1));
// Connect the output of the adder to the plant's actuation input.
builder.Connect(adder.get_output_port(0), plant.get_actuation_input_port());
auto meshcat = std::make_shared<geometry::Meshcat>();
visualization::ApplyVisualizationConfig(
visualization::VisualizationConfig{
.default_proximity_color = geometry::Rgba{1, 0, 0, 0.25},
.enable_alpha_sliders = true,
},
&builder, nullptr, nullptr, nullptr, meshcat);
// Construct a simulator.
std::unique_ptr<systems::Diagram<double>> diagram = builder.Build();
SimulatorConfig sim_config;
sim_config.integration_scheme = FLAGS_integration_scheme;
sim_config.max_step_size = FLAGS_max_time_step;
sim_config.accuracy = FLAGS_accuracy;
sim_config.target_realtime_rate = FLAGS_realtime_rate;
sim_config.publish_every_time_step = false;
systems::Simulator<double> simulator(*diagram);
ApplySimulatorConfig(sim_config, &simulator);
// Set the initial conditions for the spatula pose and the gripper finger
// positions.
Context<double>& mutable_root_context = simulator.get_mutable_context();
Context<double>& plant_context =
diagram->GetMutableSubsystemContext(plant, &mutable_root_context);
// Set spatula's free body pose.
const math::RigidTransform<double> X_WF1 = math::RigidTransform<double>(
math::RollPitchYaw(-0.4, 0.0, 1.57), Eigen::Vector3d(0.35, 0, 0.25));
const auto& base_link = plant.GetBodyByName("spatula");
plant.SetFreeBodyPose(&plant_context, base_link, X_WF1);
// Set finger joint positions.
const PrismaticJoint<double>& left_joint =
plant.GetJointByName<PrismaticJoint>("left_finger_sliding_joint");
left_joint.set_translation(&plant_context, -0.01);
const PrismaticJoint<double>& right_joint =
plant.GetJointByName<PrismaticJoint>("right_finger_sliding_joint");
right_joint.set_translation(&plant_context, 0.01);
// Simulate.
simulator.Initialize();
meshcat->StartRecording();
simulator.AdvanceTo(FLAGS_simulation_sec);
meshcat->StopRecording();
// TODO(#19142) According to issue 19142, we can playback contact forces and
// torques; however, contact surfaces are not recorded properly.
// For now, we delete contact surfaces to prevent confusion in the playback.
// Remove deletion when 19142 is resovled.
meshcat->Delete("/drake/contact_forces/hydroelastic/"
"left_finger_bubble+spatula/"
"contact_surface");
meshcat->Delete("/drake/contact_forces/hydroelastic/"
"right_finger_bubble+spatula/"
"contact_surface");
meshcat->PublishRecording();
systems::PrintSimulatorStatistics(simulator);
return 0;
}
} // namespace
} // namespace spatula_slip_control
} // namespace examples
} // namespace drake
int main(int argc, char* argv[]) {
gflags::SetUsageMessage(
"This is an example of using the hydroelastic contact model with a\n"
"robot gripper with compliant bubble fingers and a compliant spatula.\n"
"The example poses the spatula in the closed grip of the gripper and\n"
"uses an open loop square wave controller to perform a controlled\n"
"rotational slip of the spatula while maintaining the spatula in\n"
"the gripper's grasp. Use the MeshCat URL from the console log\n"
"messages for visualization. See the README.md file for more\n"
"information.\n");
gflags::ParseCommandLineFlags(&argc, &argv, true);
return drake::examples::spatula_slip_control::DoMain();
}
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/spatula_slip_control/spatula.sdf | <?xml version="1.0"?>
<sdf version="1.7"
xmlns:drake='drake.mit.edu'>
<model name="spatula">
<pose>0 0 0 0 0 0</pose>
<link name="spatula">
<inertial>
<mass>0.068</mass>
<inertia>
<ixx>0.21095403274864072</ixx>
<ixy>-4.264472553729218e-08</ixy>
<ixz>0.0</ixz>
<iyy>0.03710803288660893</iyy>
<iyz>-0.06982169738550205</iyz>
<izz>0.18858265237650276</izz>
</inertia>
<pose>0.0 0.115649 0.044471 0 0 0</pose>
</inertial>
<visual name="spatula_connector_visual">
<pose>0 0.0675 0.015 -0.94 0 0</pose>
<geometry>
<cylinder>
<radius>0.002</radius>
<length>0.048</length>
</cylinder>
</geometry>
</visual>
<visual name="spatula_bottom_visual">
<geometry>
<box>
<size>0.08 0.1 0.001</size>
</box>
</geometry>
</visual>
<visual name="spatula_handle_visual">
<pose>0 0.135 0.052 2 0 0</pose>
<material>
<diffuse>0 0 0 0.5</diffuse>
</material>
<geometry>
<cylinder>
<radius>0.015</radius>
<length>0.11</length>
</cylinder>
</geometry>
</visual>
<collision name="spatula_handle_collision">
<pose>0 0.135 0.052 2 0 0</pose>
<geometry>
<cylinder>
<radius>0.015</radius>
<length>0.11</length>
</cylinder>
</geometry>
<drake:proximity_properties>
<drake:mesh_resolution_hint>0.005</drake:mesh_resolution_hint>
<drake:compliant_hydroelastic/>
<drake:hydroelastic_modulus>1e8</drake:hydroelastic_modulus>
<drake:hunt_crossley_dissipation>5</drake:hunt_crossley_dissipation>
<drake:mu_dynamic>1.0</drake:mu_dynamic>
</drake:proximity_properties>
</collision>
</link>
</model>
</sdf>
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_nonconvex_mesh/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load("//tools/skylark:drake_py.bzl", "drake_py_binary")
package(default_visibility = ["//visibility:private"])
filegroup(
name = "models",
srcs = [
"table.sdf",
],
visibility = ["//:__pkg__"],
)
drake_py_binary(
name = "drop_pepper_py",
srcs = ["drop_pepper.py"],
add_test_rule = 1,
data = [
":models",
"@drake_models//:dishes",
"@drake_models//:veggies",
],
test_rule_args = [
"--simulation_time=0.01",
"--target_realtime_rate=0",
],
# The debug test can be 100x slower than the release.
# The debug test takes about 90 seconds.
# The release test takes about 3 seconds.
test_rule_timeout = "moderate",
deps = [
"//bindings/pydrake",
],
)
add_lint_tests()
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_nonconvex_mesh/README.md | # Drop a bell pepper
This is a simple example of using non-convex meshes with the hydroelastic
contact model in Python.
The example drops a compliant-hydroelastic yellow bell pepper onto a
rigid-hydroelastic bowl.
The bell pepper is a non-convex compliant mesh.
The bowl is a non-convex rigid mesh.
The table is a compliant box primitive.
## Run the visualizer
```
bazel run //tools:meldis -- --open-window &
```
## Run the example
```
bazel run //examples/hydroelastic/python_nonconvex_mesh:drop_pepper_py
```

## Inspect the initial position
```
bazel run //examples/hydroelastic/python_nonconvex_mesh:drop_pepper_py \
-- --simulation_time=0
```
With `--simulation_time=0`, the simulation stops at the beginning, so we can
see the initial position of the bell pepper.

## Slow down to observe dynamics
```
bazel run //examples/hydroelastic/python_nonconvex_mesh:drop_pepper_py \
-- --target_realtime_rate=0.1
```
By default, the simulation runs at 1X realtime rate.
With `--target_realtime_rate=0.1`, the simulation slows down ten times, so
we can observe how the bowl rocks back and forth and how the bell pepper
spins and moves around.
## Inspect the contact surface
After the simulation finishes, in the visualizer adjust the alpha blending to
add transparency.

We can see multiple pieces of the contact patch between the bell pepper and
the bowl.
Rotate around and zoom-in to inspect the contact patches.
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_nonconvex_mesh/table.sdf | <?xml version="1.0"?>
<sdf version="1.7">
<model name="table">
<link name="table">
<pose>0 0 0 0 0 0</pose>
<inertial>
<pose>0 0 0 0 0 0</pose>
<mass>5.0e3</mass>
<inertia>
<!-- The code will likely fix it to World frame, so the calculation
here is just for completeness. Solid cuboid of width w, height h,
depth d, and mass m has:
Inertia tensor = (m/12)Diag(h^2+d^2, w^2+d^2, w^2+h^2)
= Diag(16.8333, 16.8333, 33.3333) -->
<ixx>16.8333</ixx>
<ixy>0</ixy>
<ixz>0</ixz>
<iyy>16.8333</iyy>
<iyz>0</iyz>
<izz>33.3333</izz>
</inertia>
</inertial>
<visual name="visual">
<pose>0 0 0 0 0 0</pose>
<geometry>
<box>
<size>0.2 0.2 0.02</size>
</box>
</geometry>
<material>
<diffuse>0.8 0.5 0.5 0.5</diffuse>
</material>
</visual>
<collision name="collision">
<pose>0 0 0 0 0 0</pose>
<geometry>
<box>
<size>0.2 0.2 0.02</size>
</box>
</geometry>
<drake:proximity_properties>
<drake:compliant_hydroelastic/>
<drake:hydroelastic_modulus>5.0e6</drake:hydroelastic_modulus>
<!-- geometry/box does not take mesh_resolution_hint into account
because it always uses the coarsest mesh. We place it here in
case you want to change the box to something else like capsules,
cylinders, ellipsoids, or spheres.
-->
<drake:mesh_resolution_hint>0.01</drake:mesh_resolution_hint>
<!-- Both mu_dynamic and mu_static are used in Continuous system.
Only mu_dynamic is used in Discrete system.
-->
<drake:mu_dynamic>1.0</drake:mu_dynamic>
<drake:mu_static>1.0</drake:mu_static>
</drake:proximity_properties>
</collision>
</link>
</model>
</sdf>
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_nonconvex_mesh/drop_pepper.py | """
This is an example of using hydroelastic contact model through pydrake with
non-convex meshes. It reads SDFormat files of:
- a non-convex mesh of a yellow bell pepper with compliant-hydroelastic
properties,
- a non-convex mesh of a bowl with rigid-hydroelastic properties,
- and a table top (anchored to the World) represented as a box primitive with
compliant-hydroelastic properties.
"""
import argparse
import numpy as np
from pydrake.math import RigidTransform
from pydrake.multibody.parsing import Parser
from pydrake.multibody.plant import AddMultibodyPlant
from pydrake.multibody.plant import MultibodyPlantConfig
from pydrake.systems.analysis import ApplySimulatorConfig
from pydrake.systems.analysis import Simulator
from pydrake.systems.analysis import SimulatorConfig
from pydrake.systems.analysis import PrintSimulatorStatistics
from pydrake.systems.framework import DiagramBuilder
from pydrake.visualization import AddDefaultVisualization
def make_pepper_bowl_table(contact_model, time_step):
builder = DiagramBuilder()
plant, scene_graph = AddMultibodyPlant(
MultibodyPlantConfig(
time_step=time_step,
contact_model=contact_model,
contact_surface_representation="polygon",
discrete_contact_solver="sap"),
builder)
parser = Parser(plant)
parser.AddModels(
url="package://drake_models/veggies/"
"yellow_bell_pepper_no_stem_low.sdf")
parser.AddModels(
url="package://drake_models/dishes/evo_bowl.sdf")
parser.AddModels(
url="package://drake/examples/hydroelastic/python_nonconvex_mesh/"
"table.sdf")
# We pose the table with its top surface on World's X-Y plane.
# Intuitively we push it down 1 cm because the box is 2 cm thick.
p_WTable_fixed = RigidTransform(np.array([0, 0, -0.01]))
plant.WeldFrames(
frame_on_parent_F=plant.world_frame(),
frame_on_child_M=plant.GetFrameByName("table"),
X_FM=p_WTable_fixed)
plant.Finalize()
AddDefaultVisualization(builder=builder)
diagram = builder.Build()
return diagram, plant
def simulate_diagram(diagram, plant,
pepper_position, pepper_wz,
bowl_position,
simulation_time, target_realtime_rate):
simulator = Simulator(diagram)
ApplySimulatorConfig(
SimulatorConfig(target_realtime_rate=target_realtime_rate,
publish_every_time_step=True),
simulator)
q_init_val = np.array([
1, 0, 0, 0, pepper_position[0], pepper_position[1], pepper_position[2],
1, 0, 0, 0, bowl_position[0], bowl_position[1], bowl_position[2]
])
v_init_val = np.hstack((np.array([0, 0, pepper_wz]), np.zeros(3),
np.zeros(3), np.zeros(3)))
plant.SetPositionsAndVelocities(
diagram.GetSubsystemContext(plant, simulator.get_context()),
np.concatenate((q_init_val, v_init_val)))
simulator.get_mutable_context().SetTime(0)
simulator.Initialize()
simulator.AdvanceTo(boundary_time=simulation_time)
PrintSimulatorStatistics(simulator)
if __name__ == "__main__":
parser = argparse.ArgumentParser(description=__doc__)
parser.add_argument(
"--simulation_time", type=float, default=2,
help="Desired duration of the simulation in seconds. "
"Default %(default)s.")
parser.add_argument(
"--contact_model", type=str, default="hydroelastic_with_fallback",
help="Contact model. Options are: 'point', 'hydroelastic', "
"'hydroelastic_with_fallback'. Default %(default)s.")
parser.add_argument(
"--time_step", type=float, default=0.01,
help="The fixed time step period (in seconds) of discrete updates "
"for the multibody plant modeled as a discrete system. "
"Strictly positive. Default %(default)s.")
parser.add_argument(
"--pepper_position", nargs=3, metavar=('x', 'y', 'z'),
default=[0, -0.15, 0.10],
help="Pepper's initial position of the bottom of the pepper: "
"x, y, z (in meters) in World frame. Default %(default)s.")
parser.add_argument(
"--pepper_wz", type=float, default=150,
help="Pepper's initial angular velocity in the z-axis in rad/s. "
"Default %(default)s.")
parser.add_argument(
"--bowl_position", nargs=3, metavar=('x', 'y', 'z'),
default=[0, -0.07, 0.061],
help="Bowl's initial position of its center: "
"x, y, z (in meters) in World frame. Default %(default)s.")
parser.add_argument(
"--target_realtime_rate", type=float, default=1.0,
help="Target realtime rate. Set to 0 to run as fast as it can. "
"Default %(default)s.")
args = parser.parse_args()
diagram, plant = make_pepper_bowl_table(args.contact_model, args.time_step)
simulate_diagram(diagram, plant,
np.array(args.pepper_position),
args.pepper_wz,
np.array(args.bowl_position),
args.simulation_time,
args.target_realtime_rate)
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_ball_paddle/BUILD.bazel | load("//tools/lint:lint.bzl", "add_lint_tests")
load("//tools/skylark:drake_py.bzl", "drake_py_binary")
package(default_visibility = ["//visibility:private"])
filegroup(
name = "models",
srcs = [
"ball.sdf",
"paddle.sdf",
],
visibility = ["//:__pkg__"],
)
drake_py_binary(
name = "contact_sim_demo_py",
srcs = ["contact_sim_demo.py"],
add_test_rule = 1,
data = [":models"],
test_rule_args = [
"--target_realtime_rate=0",
"--simulation_time=0.1",
],
deps = [
"//bindings/pydrake",
],
)
add_lint_tests()
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_ball_paddle/README.md | # Ball and Paddle in Python
This is a simple example of using the hydroelastic contact model in Python.
The example drops a compliant-hydroelastic ball on a compliant-hydroelastic
paddle (represented as a box). The two bodies are defined in SDFormat files.
The compliant paddle is a box of size 20x20x2 cm. It is stationary and
posed in World frame such that its top surface is on and aligned with the
World's X-Y plane. The center of its top surface is at World origin.
The compliant ball has radius of 2 cm. By default, it is dropped 4 cm above
the paddle.
## Run the visualizer
```
bazel run //tools:meldis -- --open-window &
```
## Run the example
```
bazel run //examples/hydroelastic/python_ball_paddle:contact_sim_demo_py
```

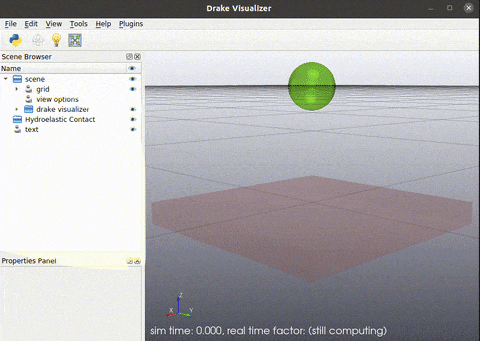
## Drop the ball near the paddle's edge
We specify very low `target_realtime_rate` so that we can see it easier.
```
bazel run //examples/hydroelastic/python_ball_paddle:contact_sim_demo_py -- \
--ball_initial_position 0.101 0 0.05 \
--target_realtime_rate=0.04 \
--simulation_time=0.09
```

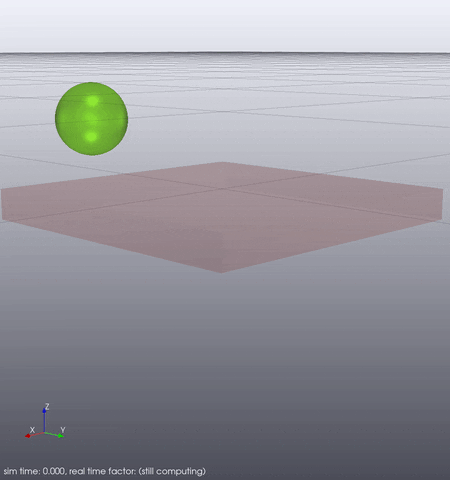
## Drop the ball near the paddle's corner
We specify even lower `target_realtime_rate` so that we can see it easier.
```
bazel run //examples/hydroelastic/python_ball_paddle:contact_sim_demo_py -- \
--ball_initial_position 0.102 0.102 0.05 \
--target_realtime_rate=0.02 \
--simulation_time=0.09
```

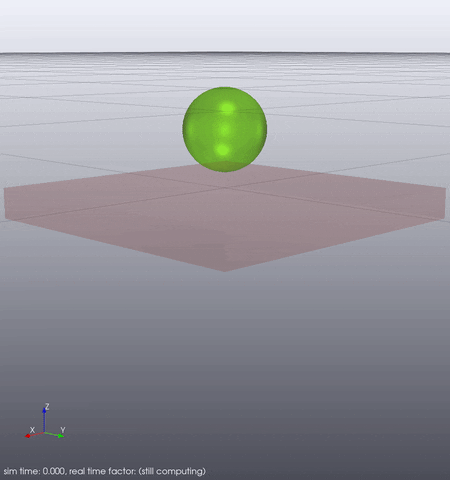
## Other Options
There are other command-line options that you can use.
Use `--help` to see the list.
For example, you can use `--contact_model=point` to test point contact.
```
bazel run //examples/hydroelastic/python_ball_paddle:contact_sim_demo_py -- \
--help
```
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_ball_paddle/ball.sdf | <?xml version="1.0"?>
<sdf version="1.7">
<model name="ball">
<link name="ball">
<pose>0 0 0 0 0 0</pose>
<inertial>
<pose>0 0 0 0 0 0</pose>
<mass>0.1</mass>
<inertia>
<!-- Solid sphere of radius r and mass m has:
Inertia tensor = (2/5)mr^2 I_3x3 = 1.6e-5 I_3x3 -->
<ixx>1.6e-5</ixx>
<ixy>0</ixy>
<ixz>0</ixz>
<iyy>1.6e-5</iyy>
<iyz>0</iyz>
<izz>1.6e-5</izz>
</inertia>
</inertial>
<visual name="visual">
<pose>0 0 0 0 0 0</pose>
<geometry>
<sphere>
<radius>0.02</radius>
</sphere>
</geometry>
<material>
<diffuse>0.5 1.0 0.0 0.5</diffuse>
</material>
</visual>
<collision name="collision">
<pose>0 0 0 0 0 0</pose>
<geometry>
<sphere>
<radius>0.02</radius>
</sphere>
</geometry>
<drake:proximity_properties>
<drake:point_contact_stiffness>980</drake:point_contact_stiffness>
<drake:compliant_hydroelastic/>
<drake:hydroelastic_modulus>5.0e6</drake:hydroelastic_modulus>
<drake:mesh_resolution_hint>0.005</drake:mesh_resolution_hint>
<!-- Little dissipation means the ball can bounce off. -->
<drake:hunt_crossley_dissipation>
0.1
</drake:hunt_crossley_dissipation>
<!-- Both mu_dynamic and mu_static are used in Continuous system.
Only mu_dynamic is used in Discrete system.
-->
<drake:mu_dynamic>1.0</drake:mu_dynamic>
<drake:mu_static>1.0</drake:mu_static>
</drake:proximity_properties>
</collision>
</link>
</model>
</sdf>
| 0 |
/home/johnshepherd/drake/examples/hydroelastic | /home/johnshepherd/drake/examples/hydroelastic/python_ball_paddle/paddle.sdf | <?xml version="1.0"?>
<sdf version="1.7">
<model name="paddle">
<link name="paddle">
<pose>0 0 0 0 0 0</pose>
<inertial>
<pose>0 0 0 0 0 0</pose>
<mass>5.0e3</mass>
<inertia>
<!-- The code will likely fix it to World frame, so the calculation
here is just for completeness. Solid cuboid of width w, height h,
depth d, and mass m has:
Inertia tensor = (m/12)Diag(h^2+d^2, w^2+d^2, w^2+h^2)
= Diag(16.8333, 16.8333, 33.3333) -->
<ixx>16.8333</ixx>
<ixy>0</ixy>
<ixz>0</ixz>
<iyy>16.8333</iyy>
<iyz>0</iyz>
<izz>33.3333</izz>
</inertia>
</inertial>
<visual name="visual">
<pose>0 0 0 0 0 0</pose>
<geometry>
<box>
<size>0.2 0.2 0.02</size>
</box>
</geometry>
<material>
<diffuse>0.8 0.5 0.5 0.2</diffuse>
</material>
</visual>
<collision name="collision">
<pose>0 0 0 0 0 0</pose>
<geometry>
<box>
<size>0.2 0.2 0.02</size>
</box>
</geometry>
<drake:proximity_properties>
<drake:compliant_hydroelastic/>
<drake:hydroelastic_modulus>2.5e6</drake:hydroelastic_modulus>
<!-- geometry/box does not take mesh_resolution_hint into account
because it always uses the coarsest mesh. We place it here in
case you want to change the box to something else like capsules,
cylinders, ellipsoids, or spheres.
-->
<drake:mesh_resolution_hint>100</drake:mesh_resolution_hint>
<!-- Both mu_dynamic and mu_static are used in Continuous system.
Only mu_dynamic is used in Discrete system.
-->
<drake:mu_dynamic>1.0</drake:mu_dynamic>
<drake:mu_static>1.0</drake:mu_static>
</drake:proximity_properties>
</collision>
</link>
</model>
</sdf>
| 0 |